What should a find function return in failure result?
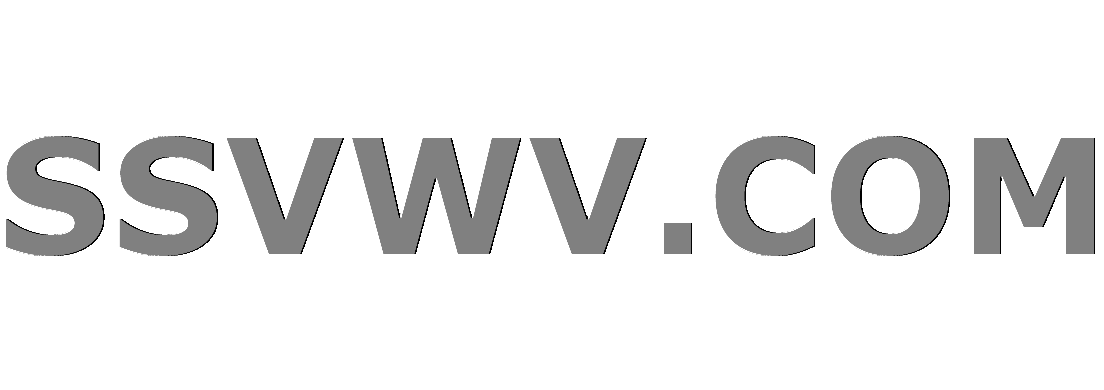
Multi tool use
The question has been bothering me since I first realized the List class, where I needed a method to find nodes which data is equal to input value. If ==, then, obviously, return immediately. But otherwise? What should it do then?
I thought about throwing an exception, but how the program will behave when from the function call it will recognizes the failure?
Node* find_node(const T &data) {
if (is_empty())
std::exit(1);
Node *temp = _head;
while (temp != nullptr) {
if (temp->_data == data)
break;
temp = temp->_next;
}
return temp;
}
Well, I kind kind of expect it to return a pointer to a node if search succeeded, so I could access its value in function's call if needed.
c++ function return
|
show 3 more comments
The question has been bothering me since I first realized the List class, where I needed a method to find nodes which data is equal to input value. If ==, then, obviously, return immediately. But otherwise? What should it do then?
I thought about throwing an exception, but how the program will behave when from the function call it will recognizes the failure?
Node* find_node(const T &data) {
if (is_empty())
std::exit(1);
Node *temp = _head;
while (temp != nullptr) {
if (temp->_data == data)
break;
temp = temp->_next;
}
return temp;
}
Well, I kind kind of expect it to return a pointer to a node if search succeeded, so I could access its value in function's call if needed.
c++ function return
4
For a function that returns a pointer, when it fails it's common to return a null pointer. Which of course needs to be checked for.
– Some programmer dude
Dec 31 '18 at 17:04
3
It is already returningnullptr
. Seems ok.
– Johnny Mopp
Dec 31 '18 at 17:05
1
It might be interesting to use this pattern: en.wikipedia.org/wiki/Option_type
– Cristiano
Dec 31 '18 at 17:06
3
Calling exit() if the list is empty is not a good idea.
– Neil Butterworth
Dec 31 '18 at 17:08
@NeilButterworth Sorry, I haven't touched this project in weeks. Will it be okay to return nullptr then?
– xt1zer
Dec 31 '18 at 17:10
|
show 3 more comments
The question has been bothering me since I first realized the List class, where I needed a method to find nodes which data is equal to input value. If ==, then, obviously, return immediately. But otherwise? What should it do then?
I thought about throwing an exception, but how the program will behave when from the function call it will recognizes the failure?
Node* find_node(const T &data) {
if (is_empty())
std::exit(1);
Node *temp = _head;
while (temp != nullptr) {
if (temp->_data == data)
break;
temp = temp->_next;
}
return temp;
}
Well, I kind kind of expect it to return a pointer to a node if search succeeded, so I could access its value in function's call if needed.
c++ function return
The question has been bothering me since I first realized the List class, where I needed a method to find nodes which data is equal to input value. If ==, then, obviously, return immediately. But otherwise? What should it do then?
I thought about throwing an exception, but how the program will behave when from the function call it will recognizes the failure?
Node* find_node(const T &data) {
if (is_empty())
std::exit(1);
Node *temp = _head;
while (temp != nullptr) {
if (temp->_data == data)
break;
temp = temp->_next;
}
return temp;
}
Well, I kind kind of expect it to return a pointer to a node if search succeeded, so I could access its value in function's call if needed.
c++ function return
c++ function return
asked Dec 31 '18 at 17:02
xt1zerxt1zer
11
11
4
For a function that returns a pointer, when it fails it's common to return a null pointer. Which of course needs to be checked for.
– Some programmer dude
Dec 31 '18 at 17:04
3
It is already returningnullptr
. Seems ok.
– Johnny Mopp
Dec 31 '18 at 17:05
1
It might be interesting to use this pattern: en.wikipedia.org/wiki/Option_type
– Cristiano
Dec 31 '18 at 17:06
3
Calling exit() if the list is empty is not a good idea.
– Neil Butterworth
Dec 31 '18 at 17:08
@NeilButterworth Sorry, I haven't touched this project in weeks. Will it be okay to return nullptr then?
– xt1zer
Dec 31 '18 at 17:10
|
show 3 more comments
4
For a function that returns a pointer, when it fails it's common to return a null pointer. Which of course needs to be checked for.
– Some programmer dude
Dec 31 '18 at 17:04
3
It is already returningnullptr
. Seems ok.
– Johnny Mopp
Dec 31 '18 at 17:05
1
It might be interesting to use this pattern: en.wikipedia.org/wiki/Option_type
– Cristiano
Dec 31 '18 at 17:06
3
Calling exit() if the list is empty is not a good idea.
– Neil Butterworth
Dec 31 '18 at 17:08
@NeilButterworth Sorry, I haven't touched this project in weeks. Will it be okay to return nullptr then?
– xt1zer
Dec 31 '18 at 17:10
4
4
For a function that returns a pointer, when it fails it's common to return a null pointer. Which of course needs to be checked for.
– Some programmer dude
Dec 31 '18 at 17:04
For a function that returns a pointer, when it fails it's common to return a null pointer. Which of course needs to be checked for.
– Some programmer dude
Dec 31 '18 at 17:04
3
3
It is already returning
nullptr
. Seems ok.– Johnny Mopp
Dec 31 '18 at 17:05
It is already returning
nullptr
. Seems ok.– Johnny Mopp
Dec 31 '18 at 17:05
1
1
It might be interesting to use this pattern: en.wikipedia.org/wiki/Option_type
– Cristiano
Dec 31 '18 at 17:06
It might be interesting to use this pattern: en.wikipedia.org/wiki/Option_type
– Cristiano
Dec 31 '18 at 17:06
3
3
Calling exit() if the list is empty is not a good idea.
– Neil Butterworth
Dec 31 '18 at 17:08
Calling exit() if the list is empty is not a good idea.
– Neil Butterworth
Dec 31 '18 at 17:08
@NeilButterworth Sorry, I haven't touched this project in weeks. Will it be okay to return nullptr then?
– xt1zer
Dec 31 '18 at 17:10
@NeilButterworth Sorry, I haven't touched this project in weeks. Will it be okay to return nullptr then?
– xt1zer
Dec 31 '18 at 17:10
|
show 3 more comments
2 Answers
2
active
oldest
votes
nullptr
is the correct return value for when the search fails. This is the advantage of using a pointer rather than a reference, as you could return a reference if you knew that you were always going to have a value.
The calling code should always check for nullptr
when receiving a pointer from the function call, and handle the fact that the search failed.
The C++ Core Guidelines state, in F.60:
Prefer T* over T& when "no argument" is a valid option
A pointer (T*) can be a nullptr and a reference (T&) cannot, there is no valid "null reference". Sometimes having nullptr as an alternative to indicated "no object" is useful, but if it is not, a reference is notationally simpler and might yield better code.
add a comment |
There are a number of common ways of handling this situation. The correct one largely depends on what type of thing you're returning.
- If you're returning an iterator, you normally want to return the "one past the end" iterator that the caller passed to define the range you were searching.
- With pointers you typically return a null pointer (which is what the code in the question actually does, though perhaps you didn't realize that, or have forgotten).
- If you're returning a reference, you have a couple of choices. One it to change the return type from
foo &
to anoptional<foo &>
. Another is to define a single "null" object, and return a reference to that upon failure.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53989750%2fwhat-should-a-find-function-return-in-failure-result%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
nullptr
is the correct return value for when the search fails. This is the advantage of using a pointer rather than a reference, as you could return a reference if you knew that you were always going to have a value.
The calling code should always check for nullptr
when receiving a pointer from the function call, and handle the fact that the search failed.
The C++ Core Guidelines state, in F.60:
Prefer T* over T& when "no argument" is a valid option
A pointer (T*) can be a nullptr and a reference (T&) cannot, there is no valid "null reference". Sometimes having nullptr as an alternative to indicated "no object" is useful, but if it is not, a reference is notationally simpler and might yield better code.
add a comment |
nullptr
is the correct return value for when the search fails. This is the advantage of using a pointer rather than a reference, as you could return a reference if you knew that you were always going to have a value.
The calling code should always check for nullptr
when receiving a pointer from the function call, and handle the fact that the search failed.
The C++ Core Guidelines state, in F.60:
Prefer T* over T& when "no argument" is a valid option
A pointer (T*) can be a nullptr and a reference (T&) cannot, there is no valid "null reference". Sometimes having nullptr as an alternative to indicated "no object" is useful, but if it is not, a reference is notationally simpler and might yield better code.
add a comment |
nullptr
is the correct return value for when the search fails. This is the advantage of using a pointer rather than a reference, as you could return a reference if you knew that you were always going to have a value.
The calling code should always check for nullptr
when receiving a pointer from the function call, and handle the fact that the search failed.
The C++ Core Guidelines state, in F.60:
Prefer T* over T& when "no argument" is a valid option
A pointer (T*) can be a nullptr and a reference (T&) cannot, there is no valid "null reference". Sometimes having nullptr as an alternative to indicated "no object" is useful, but if it is not, a reference is notationally simpler and might yield better code.
nullptr
is the correct return value for when the search fails. This is the advantage of using a pointer rather than a reference, as you could return a reference if you knew that you were always going to have a value.
The calling code should always check for nullptr
when receiving a pointer from the function call, and handle the fact that the search failed.
The C++ Core Guidelines state, in F.60:
Prefer T* over T& when "no argument" is a valid option
A pointer (T*) can be a nullptr and a reference (T&) cannot, there is no valid "null reference". Sometimes having nullptr as an alternative to indicated "no object" is useful, but if it is not, a reference is notationally simpler and might yield better code.
edited Dec 31 '18 at 17:20
answered Dec 31 '18 at 17:13
rsjaffersjaffe
3,90171631
3,90171631
add a comment |
add a comment |
There are a number of common ways of handling this situation. The correct one largely depends on what type of thing you're returning.
- If you're returning an iterator, you normally want to return the "one past the end" iterator that the caller passed to define the range you were searching.
- With pointers you typically return a null pointer (which is what the code in the question actually does, though perhaps you didn't realize that, or have forgotten).
- If you're returning a reference, you have a couple of choices. One it to change the return type from
foo &
to anoptional<foo &>
. Another is to define a single "null" object, and return a reference to that upon failure.
add a comment |
There are a number of common ways of handling this situation. The correct one largely depends on what type of thing you're returning.
- If you're returning an iterator, you normally want to return the "one past the end" iterator that the caller passed to define the range you were searching.
- With pointers you typically return a null pointer (which is what the code in the question actually does, though perhaps you didn't realize that, or have forgotten).
- If you're returning a reference, you have a couple of choices. One it to change the return type from
foo &
to anoptional<foo &>
. Another is to define a single "null" object, and return a reference to that upon failure.
add a comment |
There are a number of common ways of handling this situation. The correct one largely depends on what type of thing you're returning.
- If you're returning an iterator, you normally want to return the "one past the end" iterator that the caller passed to define the range you were searching.
- With pointers you typically return a null pointer (which is what the code in the question actually does, though perhaps you didn't realize that, or have forgotten).
- If you're returning a reference, you have a couple of choices. One it to change the return type from
foo &
to anoptional<foo &>
. Another is to define a single "null" object, and return a reference to that upon failure.
There are a number of common ways of handling this situation. The correct one largely depends on what type of thing you're returning.
- If you're returning an iterator, you normally want to return the "one past the end" iterator that the caller passed to define the range you were searching.
- With pointers you typically return a null pointer (which is what the code in the question actually does, though perhaps you didn't realize that, or have forgotten).
- If you're returning a reference, you have a couple of choices. One it to change the return type from
foo &
to anoptional<foo &>
. Another is to define a single "null" object, and return a reference to that upon failure.
edited Dec 31 '18 at 17:24
answered Dec 31 '18 at 17:14
Jerry CoffinJerry Coffin
386k50472911
386k50472911
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53989750%2fwhat-should-a-find-function-return-in-failure-result%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oU8WHyLnKT mMlJoY QB4duuFAO,Tq,LsfphQ7uUM5 tuoOgby50HgS8 xcxUPAHNCVWxaoS,ixBmlBJSx7QY oHiQp b dvY
4
For a function that returns a pointer, when it fails it's common to return a null pointer. Which of course needs to be checked for.
– Some programmer dude
Dec 31 '18 at 17:04
3
It is already returning
nullptr
. Seems ok.– Johnny Mopp
Dec 31 '18 at 17:05
1
It might be interesting to use this pattern: en.wikipedia.org/wiki/Option_type
– Cristiano
Dec 31 '18 at 17:06
3
Calling exit() if the list is empty is not a good idea.
– Neil Butterworth
Dec 31 '18 at 17:08
@NeilButterworth Sorry, I haven't touched this project in weeks. Will it be okay to return nullptr then?
– xt1zer
Dec 31 '18 at 17:10