Unit Test Spring-boot Rest Controller
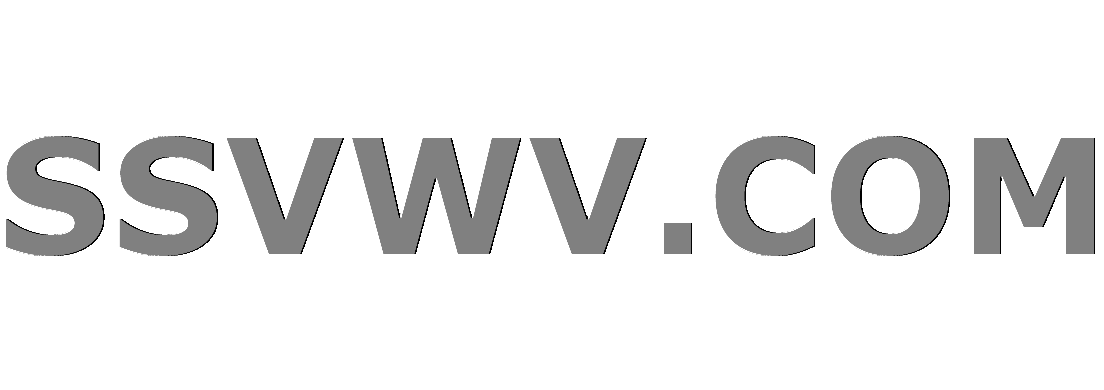
Multi tool use
Is there anyway to write unit test for my RestController without mocking the service using @MockBean?
@Autowired
private MockMvc mockMvc;
@MockBean
private CarService carService;
@Test
public void shouldReturnCarDetails() {
//when(carService.getCarDetails(1)).thenReturn(new Car(1, 300));
try {
mockMvc.perform(MockMvcRequestBuilders.get("/api/cars/1")).andExpect(MockMvcResultMatchers.status().isOk())
.andExpect(MockMvcResultMatchers.jsonPath("speed").value(300));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}`
java spring-boot junit mockito
add a comment |
Is there anyway to write unit test for my RestController without mocking the service using @MockBean?
@Autowired
private MockMvc mockMvc;
@MockBean
private CarService carService;
@Test
public void shouldReturnCarDetails() {
//when(carService.getCarDetails(1)).thenReturn(new Car(1, 300));
try {
mockMvc.perform(MockMvcRequestBuilders.get("/api/cars/1")).andExpect(MockMvcResultMatchers.status().isOk())
.andExpect(MockMvcResultMatchers.jsonPath("speed").value(300));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}`
java spring-boot junit mockito
1
Can you specify what issue you have with your current implementation?
– Assafs
Dec 31 '18 at 17:02
I heard about classist approch that they don't use mocks and i wonder how i can implement it in this case
– Kira Layto
Dec 31 '18 at 17:12
are you looking for integration tests?
– stacker
Dec 31 '18 at 17:18
So you don't want to write a unit test, but instead you want an integration test, testing your complete application? docs.spring.io/spring-boot/docs/current/reference/htmlsingle/…
– JB Nizet
Dec 31 '18 at 17:19
stackoverflow.com/questions/50907132/… -: This will help you to write integration test.
– Shaunak Patel
Dec 31 '18 at 18:04
add a comment |
Is there anyway to write unit test for my RestController without mocking the service using @MockBean?
@Autowired
private MockMvc mockMvc;
@MockBean
private CarService carService;
@Test
public void shouldReturnCarDetails() {
//when(carService.getCarDetails(1)).thenReturn(new Car(1, 300));
try {
mockMvc.perform(MockMvcRequestBuilders.get("/api/cars/1")).andExpect(MockMvcResultMatchers.status().isOk())
.andExpect(MockMvcResultMatchers.jsonPath("speed").value(300));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}`
java spring-boot junit mockito
Is there anyway to write unit test for my RestController without mocking the service using @MockBean?
@Autowired
private MockMvc mockMvc;
@MockBean
private CarService carService;
@Test
public void shouldReturnCarDetails() {
//when(carService.getCarDetails(1)).thenReturn(new Car(1, 300));
try {
mockMvc.perform(MockMvcRequestBuilders.get("/api/cars/1")).andExpect(MockMvcResultMatchers.status().isOk())
.andExpect(MockMvcResultMatchers.jsonPath("speed").value(300));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}`
java spring-boot junit mockito
java spring-boot junit mockito
edited Dec 31 '18 at 17:00


Assafs
2,82631828
2,82631828
asked Dec 31 '18 at 16:59
Kira LaytoKira Layto
4315
4315
1
Can you specify what issue you have with your current implementation?
– Assafs
Dec 31 '18 at 17:02
I heard about classist approch that they don't use mocks and i wonder how i can implement it in this case
– Kira Layto
Dec 31 '18 at 17:12
are you looking for integration tests?
– stacker
Dec 31 '18 at 17:18
So you don't want to write a unit test, but instead you want an integration test, testing your complete application? docs.spring.io/spring-boot/docs/current/reference/htmlsingle/…
– JB Nizet
Dec 31 '18 at 17:19
stackoverflow.com/questions/50907132/… -: This will help you to write integration test.
– Shaunak Patel
Dec 31 '18 at 18:04
add a comment |
1
Can you specify what issue you have with your current implementation?
– Assafs
Dec 31 '18 at 17:02
I heard about classist approch that they don't use mocks and i wonder how i can implement it in this case
– Kira Layto
Dec 31 '18 at 17:12
are you looking for integration tests?
– stacker
Dec 31 '18 at 17:18
So you don't want to write a unit test, but instead you want an integration test, testing your complete application? docs.spring.io/spring-boot/docs/current/reference/htmlsingle/…
– JB Nizet
Dec 31 '18 at 17:19
stackoverflow.com/questions/50907132/… -: This will help you to write integration test.
– Shaunak Patel
Dec 31 '18 at 18:04
1
1
Can you specify what issue you have with your current implementation?
– Assafs
Dec 31 '18 at 17:02
Can you specify what issue you have with your current implementation?
– Assafs
Dec 31 '18 at 17:02
I heard about classist approch that they don't use mocks and i wonder how i can implement it in this case
– Kira Layto
Dec 31 '18 at 17:12
I heard about classist approch that they don't use mocks and i wonder how i can implement it in this case
– Kira Layto
Dec 31 '18 at 17:12
are you looking for integration tests?
– stacker
Dec 31 '18 at 17:18
are you looking for integration tests?
– stacker
Dec 31 '18 at 17:18
So you don't want to write a unit test, but instead you want an integration test, testing your complete application? docs.spring.io/spring-boot/docs/current/reference/htmlsingle/…
– JB Nizet
Dec 31 '18 at 17:19
So you don't want to write a unit test, but instead you want an integration test, testing your complete application? docs.spring.io/spring-boot/docs/current/reference/htmlsingle/…
– JB Nizet
Dec 31 '18 at 17:19
stackoverflow.com/questions/50907132/… -: This will help you to write integration test.
– Shaunak Patel
Dec 31 '18 at 18:04
stackoverflow.com/questions/50907132/… -: This will help you to write integration test.
– Shaunak Patel
Dec 31 '18 at 18:04
add a comment |
1 Answer
1
active
oldest
votes
There is another option called, @EnableWebMvc / mockMvc through which you can test your controller layer.
Code snippet given below,
*
* The TransactionsControllerTest class implements Junit functionality that
* simply connect to the controller layer (TransactionsController) and test the
* controller layer with some pre-defined/test value. This class also provides
* the details result for each test cases.
*
* @author Sibsankar Bera
* @version 1.0
* @since 2018-08-31
*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = { TransactionsController.class, AppConfig.class, AppInitializer.class })
@EnableWebMvc
public class TransactionsControllerTest {
private static final Logger logger = Logger.getLogger(TransactionsControllerTest.class.getName());
private MockMvc mockMvc = null;
String values = null;
@InjectMocks
private WebApplicationContext wac;
/**
* This Junit test method assigns required resources value before use.
*/
@Before
public void setup() throws Exception {
values = TransactionConstant.AUTHORIZATION_CODE;
mockMvc = MockMvcBuilders.webAppContextSetup(this.wac).build();
}
/**
* This Junit test method is used to test controller layer api,
* "/transactions/getAllTransactionList"
*/
@Test
public void getAllTransactionList_Test() {
try {
logger.info("Logger Name: getAllTransactionList_Test() :: " + logger.getName());
ObjectMapper mapper = new ObjectMapper();
MvcResult result = mockMvc.perform(get("/getAllTransactionList").header("authorization_code", values))
.andReturn();
JsonNode root = mapper.readTree(result.getResponse().getContentAsString());
JsonNode resultNodes = root.path("result");
logger.debug("Junit Response :: resultNodes :: getAllTransactionList_Test() :: " + resultNodes.asText());
assertEquals(resultNodes.asText(), "success");
} catch (Exception e) {
logger.error("Junit :: Exception :: getAllTransactionList_Test() :: ", e);
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53989725%2funit-test-spring-boot-rest-controller%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is another option called, @EnableWebMvc / mockMvc through which you can test your controller layer.
Code snippet given below,
*
* The TransactionsControllerTest class implements Junit functionality that
* simply connect to the controller layer (TransactionsController) and test the
* controller layer with some pre-defined/test value. This class also provides
* the details result for each test cases.
*
* @author Sibsankar Bera
* @version 1.0
* @since 2018-08-31
*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = { TransactionsController.class, AppConfig.class, AppInitializer.class })
@EnableWebMvc
public class TransactionsControllerTest {
private static final Logger logger = Logger.getLogger(TransactionsControllerTest.class.getName());
private MockMvc mockMvc = null;
String values = null;
@InjectMocks
private WebApplicationContext wac;
/**
* This Junit test method assigns required resources value before use.
*/
@Before
public void setup() throws Exception {
values = TransactionConstant.AUTHORIZATION_CODE;
mockMvc = MockMvcBuilders.webAppContextSetup(this.wac).build();
}
/**
* This Junit test method is used to test controller layer api,
* "/transactions/getAllTransactionList"
*/
@Test
public void getAllTransactionList_Test() {
try {
logger.info("Logger Name: getAllTransactionList_Test() :: " + logger.getName());
ObjectMapper mapper = new ObjectMapper();
MvcResult result = mockMvc.perform(get("/getAllTransactionList").header("authorization_code", values))
.andReturn();
JsonNode root = mapper.readTree(result.getResponse().getContentAsString());
JsonNode resultNodes = root.path("result");
logger.debug("Junit Response :: resultNodes :: getAllTransactionList_Test() :: " + resultNodes.asText());
assertEquals(resultNodes.asText(), "success");
} catch (Exception e) {
logger.error("Junit :: Exception :: getAllTransactionList_Test() :: ", e);
}
}
add a comment |
There is another option called, @EnableWebMvc / mockMvc through which you can test your controller layer.
Code snippet given below,
*
* The TransactionsControllerTest class implements Junit functionality that
* simply connect to the controller layer (TransactionsController) and test the
* controller layer with some pre-defined/test value. This class also provides
* the details result for each test cases.
*
* @author Sibsankar Bera
* @version 1.0
* @since 2018-08-31
*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = { TransactionsController.class, AppConfig.class, AppInitializer.class })
@EnableWebMvc
public class TransactionsControllerTest {
private static final Logger logger = Logger.getLogger(TransactionsControllerTest.class.getName());
private MockMvc mockMvc = null;
String values = null;
@InjectMocks
private WebApplicationContext wac;
/**
* This Junit test method assigns required resources value before use.
*/
@Before
public void setup() throws Exception {
values = TransactionConstant.AUTHORIZATION_CODE;
mockMvc = MockMvcBuilders.webAppContextSetup(this.wac).build();
}
/**
* This Junit test method is used to test controller layer api,
* "/transactions/getAllTransactionList"
*/
@Test
public void getAllTransactionList_Test() {
try {
logger.info("Logger Name: getAllTransactionList_Test() :: " + logger.getName());
ObjectMapper mapper = new ObjectMapper();
MvcResult result = mockMvc.perform(get("/getAllTransactionList").header("authorization_code", values))
.andReturn();
JsonNode root = mapper.readTree(result.getResponse().getContentAsString());
JsonNode resultNodes = root.path("result");
logger.debug("Junit Response :: resultNodes :: getAllTransactionList_Test() :: " + resultNodes.asText());
assertEquals(resultNodes.asText(), "success");
} catch (Exception e) {
logger.error("Junit :: Exception :: getAllTransactionList_Test() :: ", e);
}
}
add a comment |
There is another option called, @EnableWebMvc / mockMvc through which you can test your controller layer.
Code snippet given below,
*
* The TransactionsControllerTest class implements Junit functionality that
* simply connect to the controller layer (TransactionsController) and test the
* controller layer with some pre-defined/test value. This class also provides
* the details result for each test cases.
*
* @author Sibsankar Bera
* @version 1.0
* @since 2018-08-31
*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = { TransactionsController.class, AppConfig.class, AppInitializer.class })
@EnableWebMvc
public class TransactionsControllerTest {
private static final Logger logger = Logger.getLogger(TransactionsControllerTest.class.getName());
private MockMvc mockMvc = null;
String values = null;
@InjectMocks
private WebApplicationContext wac;
/**
* This Junit test method assigns required resources value before use.
*/
@Before
public void setup() throws Exception {
values = TransactionConstant.AUTHORIZATION_CODE;
mockMvc = MockMvcBuilders.webAppContextSetup(this.wac).build();
}
/**
* This Junit test method is used to test controller layer api,
* "/transactions/getAllTransactionList"
*/
@Test
public void getAllTransactionList_Test() {
try {
logger.info("Logger Name: getAllTransactionList_Test() :: " + logger.getName());
ObjectMapper mapper = new ObjectMapper();
MvcResult result = mockMvc.perform(get("/getAllTransactionList").header("authorization_code", values))
.andReturn();
JsonNode root = mapper.readTree(result.getResponse().getContentAsString());
JsonNode resultNodes = root.path("result");
logger.debug("Junit Response :: resultNodes :: getAllTransactionList_Test() :: " + resultNodes.asText());
assertEquals(resultNodes.asText(), "success");
} catch (Exception e) {
logger.error("Junit :: Exception :: getAllTransactionList_Test() :: ", e);
}
}
There is another option called, @EnableWebMvc / mockMvc through which you can test your controller layer.
Code snippet given below,
*
* The TransactionsControllerTest class implements Junit functionality that
* simply connect to the controller layer (TransactionsController) and test the
* controller layer with some pre-defined/test value. This class also provides
* the details result for each test cases.
*
* @author Sibsankar Bera
* @version 1.0
* @since 2018-08-31
*/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = { TransactionsController.class, AppConfig.class, AppInitializer.class })
@EnableWebMvc
public class TransactionsControllerTest {
private static final Logger logger = Logger.getLogger(TransactionsControllerTest.class.getName());
private MockMvc mockMvc = null;
String values = null;
@InjectMocks
private WebApplicationContext wac;
/**
* This Junit test method assigns required resources value before use.
*/
@Before
public void setup() throws Exception {
values = TransactionConstant.AUTHORIZATION_CODE;
mockMvc = MockMvcBuilders.webAppContextSetup(this.wac).build();
}
/**
* This Junit test method is used to test controller layer api,
* "/transactions/getAllTransactionList"
*/
@Test
public void getAllTransactionList_Test() {
try {
logger.info("Logger Name: getAllTransactionList_Test() :: " + logger.getName());
ObjectMapper mapper = new ObjectMapper();
MvcResult result = mockMvc.perform(get("/getAllTransactionList").header("authorization_code", values))
.andReturn();
JsonNode root = mapper.readTree(result.getResponse().getContentAsString());
JsonNode resultNodes = root.path("result");
logger.debug("Junit Response :: resultNodes :: getAllTransactionList_Test() :: " + resultNodes.asText());
assertEquals(resultNodes.asText(), "success");
} catch (Exception e) {
logger.error("Junit :: Exception :: getAllTransactionList_Test() :: ", e);
}
}
answered Jan 1 at 20:02
Sibsankar BeraSibsankar Bera
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53989725%2funit-test-spring-boot-rest-controller%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1VY qPSq pWVY,kvkMqyxejH2kpTDJBn9Alr4pAZ 1YTCToARd4djsQGlb,zO0BboJzk1nsDqo7pYF77zmq,mGLbPmG
1
Can you specify what issue you have with your current implementation?
– Assafs
Dec 31 '18 at 17:02
I heard about classist approch that they don't use mocks and i wonder how i can implement it in this case
– Kira Layto
Dec 31 '18 at 17:12
are you looking for integration tests?
– stacker
Dec 31 '18 at 17:18
So you don't want to write a unit test, but instead you want an integration test, testing your complete application? docs.spring.io/spring-boot/docs/current/reference/htmlsingle/…
– JB Nizet
Dec 31 '18 at 17:19
stackoverflow.com/questions/50907132/… -: This will help you to write integration test.
– Shaunak Patel
Dec 31 '18 at 18:04