Split string into repeated characters
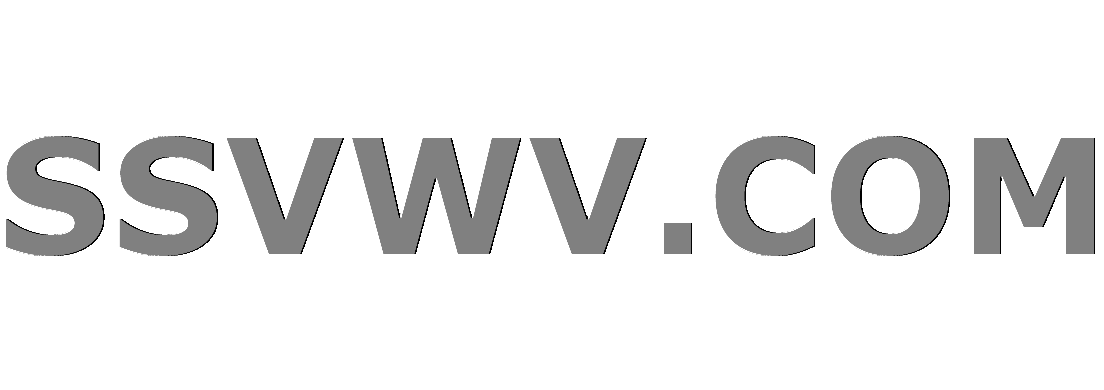
Multi tool use
I want to split the string "aaaabbbccccaaddddcfggghhhh" into "aaaa", "bbb", "cccc". "aa", "dddd", "c", "f" and so on.
I tried this:
String arr = "aaaabbbccccaaddddcfggghhhh".split("(.)(?!\1)");
But this eats away one character, so with the above regular expression I get "aaa" while I want it to be "aaaa" as the first string.
How do I achieve this?
java regex string
|
show 1 more comment
I want to split the string "aaaabbbccccaaddddcfggghhhh" into "aaaa", "bbb", "cccc". "aa", "dddd", "c", "f" and so on.
I tried this:
String arr = "aaaabbbccccaaddddcfggghhhh".split("(.)(?!\1)");
But this eats away one character, so with the above regular expression I get "aaa" while I want it to be "aaaa" as the first string.
How do I achieve this?
java regex string
1
@Adri1du40: I am open to other options but don't want to use loop.
– Lokesh
May 7 '14 at 16:52
Check this question : stackoverflow.com/questions/15101577/…
– Tofandel
May 7 '14 at 16:57
I'm not a Java guy, but wouldn'tstring.split()
be slower than a loop?
– Amal Murali
May 7 '14 at 17:49
@AmalMurali would be less readable too. I don't know about you but reading this regex(?<=(.))(?!\1)
is going to make me scratch my head.
– Cruncher
May 7 '14 at 19:04
1
Possible duplicate of Split regex to extract Strings of contiguous characters
– maxxyme
Jan 12 '17 at 8:50
|
show 1 more comment
I want to split the string "aaaabbbccccaaddddcfggghhhh" into "aaaa", "bbb", "cccc". "aa", "dddd", "c", "f" and so on.
I tried this:
String arr = "aaaabbbccccaaddddcfggghhhh".split("(.)(?!\1)");
But this eats away one character, so with the above regular expression I get "aaa" while I want it to be "aaaa" as the first string.
How do I achieve this?
java regex string
I want to split the string "aaaabbbccccaaddddcfggghhhh" into "aaaa", "bbb", "cccc". "aa", "dddd", "c", "f" and so on.
I tried this:
String arr = "aaaabbbccccaaddddcfggghhhh".split("(.)(?!\1)");
But this eats away one character, so with the above regular expression I get "aaa" while I want it to be "aaaa" as the first string.
How do I achieve this?
java regex string
java regex string
edited May 7 '14 at 22:13


Peter Mortensen
13.6k1986111
13.6k1986111
asked May 7 '14 at 16:44
LokeshLokesh
5,98562762
5,98562762
1
@Adri1du40: I am open to other options but don't want to use loop.
– Lokesh
May 7 '14 at 16:52
Check this question : stackoverflow.com/questions/15101577/…
– Tofandel
May 7 '14 at 16:57
I'm not a Java guy, but wouldn'tstring.split()
be slower than a loop?
– Amal Murali
May 7 '14 at 17:49
@AmalMurali would be less readable too. I don't know about you but reading this regex(?<=(.))(?!\1)
is going to make me scratch my head.
– Cruncher
May 7 '14 at 19:04
1
Possible duplicate of Split regex to extract Strings of contiguous characters
– maxxyme
Jan 12 '17 at 8:50
|
show 1 more comment
1
@Adri1du40: I am open to other options but don't want to use loop.
– Lokesh
May 7 '14 at 16:52
Check this question : stackoverflow.com/questions/15101577/…
– Tofandel
May 7 '14 at 16:57
I'm not a Java guy, but wouldn'tstring.split()
be slower than a loop?
– Amal Murali
May 7 '14 at 17:49
@AmalMurali would be less readable too. I don't know about you but reading this regex(?<=(.))(?!\1)
is going to make me scratch my head.
– Cruncher
May 7 '14 at 19:04
1
Possible duplicate of Split regex to extract Strings of contiguous characters
– maxxyme
Jan 12 '17 at 8:50
1
1
@Adri1du40: I am open to other options but don't want to use loop.
– Lokesh
May 7 '14 at 16:52
@Adri1du40: I am open to other options but don't want to use loop.
– Lokesh
May 7 '14 at 16:52
Check this question : stackoverflow.com/questions/15101577/…
– Tofandel
May 7 '14 at 16:57
Check this question : stackoverflow.com/questions/15101577/…
– Tofandel
May 7 '14 at 16:57
I'm not a Java guy, but wouldn't
string.split()
be slower than a loop?– Amal Murali
May 7 '14 at 17:49
I'm not a Java guy, but wouldn't
string.split()
be slower than a loop?– Amal Murali
May 7 '14 at 17:49
@AmalMurali would be less readable too. I don't know about you but reading this regex
(?<=(.))(?!\1)
is going to make me scratch my head.– Cruncher
May 7 '14 at 19:04
@AmalMurali would be less readable too. I don't know about you but reading this regex
(?<=(.))(?!\1)
is going to make me scratch my head.– Cruncher
May 7 '14 at 19:04
1
1
Possible duplicate of Split regex to extract Strings of contiguous characters
– maxxyme
Jan 12 '17 at 8:50
Possible duplicate of Split regex to extract Strings of contiguous characters
– maxxyme
Jan 12 '17 at 8:50
|
show 1 more comment
3 Answers
3
active
oldest
votes
Try this:
String str = "aaaabbbccccaaddddcfggghhhh";
String out = str.split("(?<=(.))(?!\1)");
System.out.println(Arrays.toString(out));
=> [aaaa, bbb, cccc, aa, dddd, c, f, ggg, hhhh]
Explanation: we want to split the string at groups of same chars, so we need to find out the "boundary" between each group. I'm using Java's syntax for positive look-behind to pick the previous char and then a negative look-ahead with a back reference to verify that the next char is not the same as the previous one. No characters were actually consumed, because only two look-around assertions were used (that is, the regular expresion is zero-width).
Your solution works perfectly. Can you please explain this regex. How it works.
– Lokesh
May 7 '14 at 17:00
@Lokesh you got it
– Óscar López
May 7 '14 at 17:05
add a comment |
What about capturing in a lookbehind?
(?<=(.))(?!1|$)
as a Java string:
(?<=(.))(?!\1|$)
1
@T.J.Crowder seems ok here. Why do you think its not working?
– Reimeus
May 7 '14 at 16:58
2
@Reimeus: Because I copied and pasted it without doing the escape. I really wish Java had regex literals. :-)
– T.J. Crowder
May 7 '14 at 17:03
add a comment |
here I am taking each character and Checking two conditions in the if loop i.e String can't exceed the length and if next character is not equaled to the first character continue the for loop else take new line and print it.
for (int i = 0; i < arr.length; i++) {
char chr= arr[i];
System.out.print(chr);
if (i + 1 < arr.length && arr[i + 1] != chr) {
System.out.print(" n");
}
}
For a quality answer, @Shiva can you add some explanation to your answer on how the code accomplish what the author is trying to achieve?
– pczeus
Jan 27 '17 at 5:10
I was improved the answer@pczeus
– Shivanandam
Jan 27 '17 at 14:09
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f23523597%2fsplit-string-into-repeated-characters%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try this:
String str = "aaaabbbccccaaddddcfggghhhh";
String out = str.split("(?<=(.))(?!\1)");
System.out.println(Arrays.toString(out));
=> [aaaa, bbb, cccc, aa, dddd, c, f, ggg, hhhh]
Explanation: we want to split the string at groups of same chars, so we need to find out the "boundary" between each group. I'm using Java's syntax for positive look-behind to pick the previous char and then a negative look-ahead with a back reference to verify that the next char is not the same as the previous one. No characters were actually consumed, because only two look-around assertions were used (that is, the regular expresion is zero-width).
Your solution works perfectly. Can you please explain this regex. How it works.
– Lokesh
May 7 '14 at 17:00
@Lokesh you got it
– Óscar López
May 7 '14 at 17:05
add a comment |
Try this:
String str = "aaaabbbccccaaddddcfggghhhh";
String out = str.split("(?<=(.))(?!\1)");
System.out.println(Arrays.toString(out));
=> [aaaa, bbb, cccc, aa, dddd, c, f, ggg, hhhh]
Explanation: we want to split the string at groups of same chars, so we need to find out the "boundary" between each group. I'm using Java's syntax for positive look-behind to pick the previous char and then a negative look-ahead with a back reference to verify that the next char is not the same as the previous one. No characters were actually consumed, because only two look-around assertions were used (that is, the regular expresion is zero-width).
Your solution works perfectly. Can you please explain this regex. How it works.
– Lokesh
May 7 '14 at 17:00
@Lokesh you got it
– Óscar López
May 7 '14 at 17:05
add a comment |
Try this:
String str = "aaaabbbccccaaddddcfggghhhh";
String out = str.split("(?<=(.))(?!\1)");
System.out.println(Arrays.toString(out));
=> [aaaa, bbb, cccc, aa, dddd, c, f, ggg, hhhh]
Explanation: we want to split the string at groups of same chars, so we need to find out the "boundary" between each group. I'm using Java's syntax for positive look-behind to pick the previous char and then a negative look-ahead with a back reference to verify that the next char is not the same as the previous one. No characters were actually consumed, because only two look-around assertions were used (that is, the regular expresion is zero-width).
Try this:
String str = "aaaabbbccccaaddddcfggghhhh";
String out = str.split("(?<=(.))(?!\1)");
System.out.println(Arrays.toString(out));
=> [aaaa, bbb, cccc, aa, dddd, c, f, ggg, hhhh]
Explanation: we want to split the string at groups of same chars, so we need to find out the "boundary" between each group. I'm using Java's syntax for positive look-behind to pick the previous char and then a negative look-ahead with a back reference to verify that the next char is not the same as the previous one. No characters were actually consumed, because only two look-around assertions were used (that is, the regular expresion is zero-width).
edited May 7 '14 at 17:05
answered May 7 '14 at 16:58


Óscar LópezÓscar López
178k25227322
178k25227322
Your solution works perfectly. Can you please explain this regex. How it works.
– Lokesh
May 7 '14 at 17:00
@Lokesh you got it
– Óscar López
May 7 '14 at 17:05
add a comment |
Your solution works perfectly. Can you please explain this regex. How it works.
– Lokesh
May 7 '14 at 17:00
@Lokesh you got it
– Óscar López
May 7 '14 at 17:05
Your solution works perfectly. Can you please explain this regex. How it works.
– Lokesh
May 7 '14 at 17:00
Your solution works perfectly. Can you please explain this regex. How it works.
– Lokesh
May 7 '14 at 17:00
@Lokesh you got it
– Óscar López
May 7 '14 at 17:05
@Lokesh you got it
– Óscar López
May 7 '14 at 17:05
add a comment |
What about capturing in a lookbehind?
(?<=(.))(?!1|$)
as a Java string:
(?<=(.))(?!\1|$)
1
@T.J.Crowder seems ok here. Why do you think its not working?
– Reimeus
May 7 '14 at 16:58
2
@Reimeus: Because I copied and pasted it without doing the escape. I really wish Java had regex literals. :-)
– T.J. Crowder
May 7 '14 at 17:03
add a comment |
What about capturing in a lookbehind?
(?<=(.))(?!1|$)
as a Java string:
(?<=(.))(?!\1|$)
1
@T.J.Crowder seems ok here. Why do you think its not working?
– Reimeus
May 7 '14 at 16:58
2
@Reimeus: Because I copied and pasted it without doing the escape. I really wish Java had regex literals. :-)
– T.J. Crowder
May 7 '14 at 17:03
add a comment |
What about capturing in a lookbehind?
(?<=(.))(?!1|$)
as a Java string:
(?<=(.))(?!\1|$)
What about capturing in a lookbehind?
(?<=(.))(?!1|$)
as a Java string:
(?<=(.))(?!\1|$)
edited May 7 '14 at 17:08
answered May 7 '14 at 16:51


Jonny 5Jonny 5
8,95621535
8,95621535
1
@T.J.Crowder seems ok here. Why do you think its not working?
– Reimeus
May 7 '14 at 16:58
2
@Reimeus: Because I copied and pasted it without doing the escape. I really wish Java had regex literals. :-)
– T.J. Crowder
May 7 '14 at 17:03
add a comment |
1
@T.J.Crowder seems ok here. Why do you think its not working?
– Reimeus
May 7 '14 at 16:58
2
@Reimeus: Because I copied and pasted it without doing the escape. I really wish Java had regex literals. :-)
– T.J. Crowder
May 7 '14 at 17:03
1
1
@T.J.Crowder seems ok here. Why do you think its not working?
– Reimeus
May 7 '14 at 16:58
@T.J.Crowder seems ok here. Why do you think its not working?
– Reimeus
May 7 '14 at 16:58
2
2
@Reimeus: Because I copied and pasted it without doing the escape. I really wish Java had regex literals. :-)
– T.J. Crowder
May 7 '14 at 17:03
@Reimeus: Because I copied and pasted it without doing the escape. I really wish Java had regex literals. :-)
– T.J. Crowder
May 7 '14 at 17:03
add a comment |
here I am taking each character and Checking two conditions in the if loop i.e String can't exceed the length and if next character is not equaled to the first character continue the for loop else take new line and print it.
for (int i = 0; i < arr.length; i++) {
char chr= arr[i];
System.out.print(chr);
if (i + 1 < arr.length && arr[i + 1] != chr) {
System.out.print(" n");
}
}
For a quality answer, @Shiva can you add some explanation to your answer on how the code accomplish what the author is trying to achieve?
– pczeus
Jan 27 '17 at 5:10
I was improved the answer@pczeus
– Shivanandam
Jan 27 '17 at 14:09
add a comment |
here I am taking each character and Checking two conditions in the if loop i.e String can't exceed the length and if next character is not equaled to the first character continue the for loop else take new line and print it.
for (int i = 0; i < arr.length; i++) {
char chr= arr[i];
System.out.print(chr);
if (i + 1 < arr.length && arr[i + 1] != chr) {
System.out.print(" n");
}
}
For a quality answer, @Shiva can you add some explanation to your answer on how the code accomplish what the author is trying to achieve?
– pczeus
Jan 27 '17 at 5:10
I was improved the answer@pczeus
– Shivanandam
Jan 27 '17 at 14:09
add a comment |
here I am taking each character and Checking two conditions in the if loop i.e String can't exceed the length and if next character is not equaled to the first character continue the for loop else take new line and print it.
for (int i = 0; i < arr.length; i++) {
char chr= arr[i];
System.out.print(chr);
if (i + 1 < arr.length && arr[i + 1] != chr) {
System.out.print(" n");
}
}
here I am taking each character and Checking two conditions in the if loop i.e String can't exceed the length and if next character is not equaled to the first character continue the for loop else take new line and print it.
for (int i = 0; i < arr.length; i++) {
char chr= arr[i];
System.out.print(chr);
if (i + 1 < arr.length && arr[i + 1] != chr) {
System.out.print(" n");
}
}
edited Jan 27 '17 at 14:07
answered Nov 22 '16 at 7:18


ShivanandamShivanandam
8531317
8531317
For a quality answer, @Shiva can you add some explanation to your answer on how the code accomplish what the author is trying to achieve?
– pczeus
Jan 27 '17 at 5:10
I was improved the answer@pczeus
– Shivanandam
Jan 27 '17 at 14:09
add a comment |
For a quality answer, @Shiva can you add some explanation to your answer on how the code accomplish what the author is trying to achieve?
– pczeus
Jan 27 '17 at 5:10
I was improved the answer@pczeus
– Shivanandam
Jan 27 '17 at 14:09
For a quality answer, @Shiva can you add some explanation to your answer on how the code accomplish what the author is trying to achieve?
– pczeus
Jan 27 '17 at 5:10
For a quality answer, @Shiva can you add some explanation to your answer on how the code accomplish what the author is trying to achieve?
– pczeus
Jan 27 '17 at 5:10
I was improved the answer@pczeus
– Shivanandam
Jan 27 '17 at 14:09
I was improved the answer@pczeus
– Shivanandam
Jan 27 '17 at 14:09
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f23523597%2fsplit-string-into-repeated-characters%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
H1C05vQVN7HNMyc0b3eXbO0,9jDnWdw,zgevAzaYI,akZScaB9 GFZeHbEjMxZ,wS
1
@Adri1du40: I am open to other options but don't want to use loop.
– Lokesh
May 7 '14 at 16:52
Check this question : stackoverflow.com/questions/15101577/…
– Tofandel
May 7 '14 at 16:57
I'm not a Java guy, but wouldn't
string.split()
be slower than a loop?– Amal Murali
May 7 '14 at 17:49
@AmalMurali would be less readable too. I don't know about you but reading this regex
(?<=(.))(?!\1)
is going to make me scratch my head.– Cruncher
May 7 '14 at 19:04
1
Possible duplicate of Split regex to extract Strings of contiguous characters
– maxxyme
Jan 12 '17 at 8:50