web api returning no response but data is formed at return
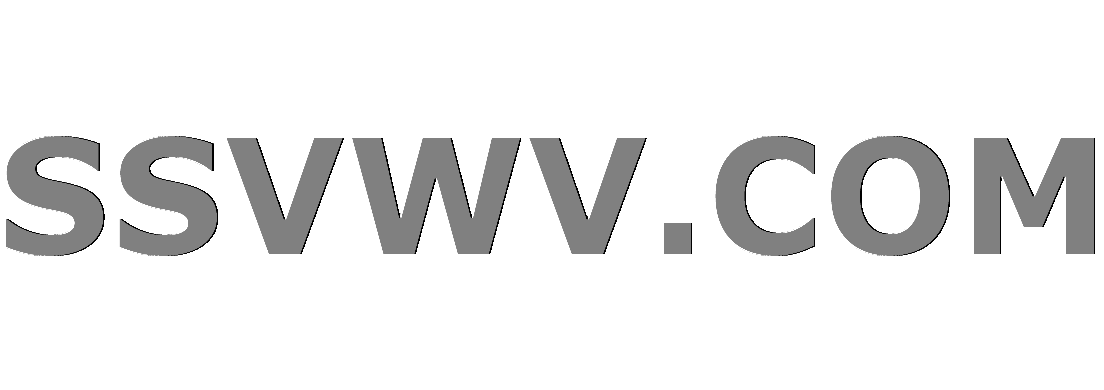
Multi tool use
The GetPlaces
method should return all users with their shares(homes), but I keep getting no response when I try to include the Shares.
The API
gets called and it gets all data but when I try to return the data back,then I get no response.
It's not only that API
call but every time I want to include another table from database, I get the same response. I am testing it with Postman
public class PlaceController : ControllerBase
{
PlaceService _PlaceService;
UserService _UserService;
ApplicationDbContext _db;
public PlaceController(PlaceService PlaceServicea, UserService _UserServicea, ApplicationDbContext db)
{
_db = db;
_PlaceService = PlaceServicea;
_UserService = _UserServicea;
}
//get all places
[HttpGet]
[Route("all")]
public async Task<ActionResult> GetPlaces()
{
var usersWithShares = await _db.Set<User>().Include(x => x.Shares).ToListAsync();
return Ok(usersWithShares);
// return Ok(await _PlaceService.GetAll());
}
}
This is my entity User
public class User
{
public int Id { get; set; }
public string Email { get; set; }
public string Password { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public long PhoneNumber { get; set; }
public DateTime BirthDate { get; set; }
public string Token { get; internal set; }
public ICollection<Place> Shares { get; set; }
}
and this is my Entity place
public class Place
{
public int Id { get; set; }
public int Rooms { get; set; }
public int Garage { get; set; }
public bool Garden { get; set; }
public int Bathrooms { get; set; }
public int Toilet { get; set; }
public int Kitchen { get; set; }
public int LivingRoom { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
public User User { get; set; }
public Adres Adres { get; set; }
public ICollection<PlacePicture> PlacePictures { get; set; }
}
and this is my ConfigureServices
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(
options => options.UseSqlServer(Configuration.GetConnectionString("Homesharing"))
);
// configure DI for application services
services.AddScoped<UserService>();
services.AddScoped<PlaceService>();
}
See image for more
c# entity-framework asp.net-web-api postman
|
show 2 more comments
The GetPlaces
method should return all users with their shares(homes), but I keep getting no response when I try to include the Shares.
The API
gets called and it gets all data but when I try to return the data back,then I get no response.
It's not only that API
call but every time I want to include another table from database, I get the same response. I am testing it with Postman
public class PlaceController : ControllerBase
{
PlaceService _PlaceService;
UserService _UserService;
ApplicationDbContext _db;
public PlaceController(PlaceService PlaceServicea, UserService _UserServicea, ApplicationDbContext db)
{
_db = db;
_PlaceService = PlaceServicea;
_UserService = _UserServicea;
}
//get all places
[HttpGet]
[Route("all")]
public async Task<ActionResult> GetPlaces()
{
var usersWithShares = await _db.Set<User>().Include(x => x.Shares).ToListAsync();
return Ok(usersWithShares);
// return Ok(await _PlaceService.GetAll());
}
}
This is my entity User
public class User
{
public int Id { get; set; }
public string Email { get; set; }
public string Password { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public long PhoneNumber { get; set; }
public DateTime BirthDate { get; set; }
public string Token { get; internal set; }
public ICollection<Place> Shares { get; set; }
}
and this is my Entity place
public class Place
{
public int Id { get; set; }
public int Rooms { get; set; }
public int Garage { get; set; }
public bool Garden { get; set; }
public int Bathrooms { get; set; }
public int Toilet { get; set; }
public int Kitchen { get; set; }
public int LivingRoom { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
public User User { get; set; }
public Adres Adres { get; set; }
public ICollection<PlacePicture> PlacePictures { get; set; }
}
and this is my ConfigureServices
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(
options => options.UseSqlServer(Configuration.GetConnectionString("Homesharing"))
);
// configure DI for application services
services.AddScoped<UserService>();
services.AddScoped<PlaceService>();
}
See image for more
c# entity-framework asp.net-web-api postman
Please sharePOSTMAN
result/error.
– Umair Anwaar
Jan 1 at 8:48
You probably get a circular reference error from the Json serializer.
– Gert Arnold
Jan 1 at 10:26
Yes it was the circular reference error, I placed Jsonignore above ICollection and now it works great, is there alternative fix for that? btw how can i mark you're commend as answere
– DES PRO
Jan 1 at 14:10
A (better) alternative is this. If you agree with that you may want to close your question as a duplicate of that one.
– Gert Arnold
Jan 1 at 20:46
@DES PRO you can answer your own question after (I think) 48hrs. What you should probably think about doing is mapping your entities to a DTO class before returning from your API, exposing everything from your DB is not always a good idea and returning a DTO will mean your data structure can change without effecting the API contract.
– matt_lethargic
Jan 2 at 10:45
|
show 2 more comments
The GetPlaces
method should return all users with their shares(homes), but I keep getting no response when I try to include the Shares.
The API
gets called and it gets all data but when I try to return the data back,then I get no response.
It's not only that API
call but every time I want to include another table from database, I get the same response. I am testing it with Postman
public class PlaceController : ControllerBase
{
PlaceService _PlaceService;
UserService _UserService;
ApplicationDbContext _db;
public PlaceController(PlaceService PlaceServicea, UserService _UserServicea, ApplicationDbContext db)
{
_db = db;
_PlaceService = PlaceServicea;
_UserService = _UserServicea;
}
//get all places
[HttpGet]
[Route("all")]
public async Task<ActionResult> GetPlaces()
{
var usersWithShares = await _db.Set<User>().Include(x => x.Shares).ToListAsync();
return Ok(usersWithShares);
// return Ok(await _PlaceService.GetAll());
}
}
This is my entity User
public class User
{
public int Id { get; set; }
public string Email { get; set; }
public string Password { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public long PhoneNumber { get; set; }
public DateTime BirthDate { get; set; }
public string Token { get; internal set; }
public ICollection<Place> Shares { get; set; }
}
and this is my Entity place
public class Place
{
public int Id { get; set; }
public int Rooms { get; set; }
public int Garage { get; set; }
public bool Garden { get; set; }
public int Bathrooms { get; set; }
public int Toilet { get; set; }
public int Kitchen { get; set; }
public int LivingRoom { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
public User User { get; set; }
public Adres Adres { get; set; }
public ICollection<PlacePicture> PlacePictures { get; set; }
}
and this is my ConfigureServices
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(
options => options.UseSqlServer(Configuration.GetConnectionString("Homesharing"))
);
// configure DI for application services
services.AddScoped<UserService>();
services.AddScoped<PlaceService>();
}
See image for more
c# entity-framework asp.net-web-api postman
The GetPlaces
method should return all users with their shares(homes), but I keep getting no response when I try to include the Shares.
The API
gets called and it gets all data but when I try to return the data back,then I get no response.
It's not only that API
call but every time I want to include another table from database, I get the same response. I am testing it with Postman
public class PlaceController : ControllerBase
{
PlaceService _PlaceService;
UserService _UserService;
ApplicationDbContext _db;
public PlaceController(PlaceService PlaceServicea, UserService _UserServicea, ApplicationDbContext db)
{
_db = db;
_PlaceService = PlaceServicea;
_UserService = _UserServicea;
}
//get all places
[HttpGet]
[Route("all")]
public async Task<ActionResult> GetPlaces()
{
var usersWithShares = await _db.Set<User>().Include(x => x.Shares).ToListAsync();
return Ok(usersWithShares);
// return Ok(await _PlaceService.GetAll());
}
}
This is my entity User
public class User
{
public int Id { get; set; }
public string Email { get; set; }
public string Password { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public long PhoneNumber { get; set; }
public DateTime BirthDate { get; set; }
public string Token { get; internal set; }
public ICollection<Place> Shares { get; set; }
}
and this is my Entity place
public class Place
{
public int Id { get; set; }
public int Rooms { get; set; }
public int Garage { get; set; }
public bool Garden { get; set; }
public int Bathrooms { get; set; }
public int Toilet { get; set; }
public int Kitchen { get; set; }
public int LivingRoom { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
public User User { get; set; }
public Adres Adres { get; set; }
public ICollection<PlacePicture> PlacePictures { get; set; }
}
and this is my ConfigureServices
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(
options => options.UseSqlServer(Configuration.GetConnectionString("Homesharing"))
);
// configure DI for application services
services.AddScoped<UserService>();
services.AddScoped<PlaceService>();
}
See image for more
c# entity-framework asp.net-web-api postman
c# entity-framework asp.net-web-api postman
edited Jan 1 at 6:40


Aria
2,7031832
2,7031832
asked Dec 31 '18 at 19:06


DES PRODES PRO
15
15
Please sharePOSTMAN
result/error.
– Umair Anwaar
Jan 1 at 8:48
You probably get a circular reference error from the Json serializer.
– Gert Arnold
Jan 1 at 10:26
Yes it was the circular reference error, I placed Jsonignore above ICollection and now it works great, is there alternative fix for that? btw how can i mark you're commend as answere
– DES PRO
Jan 1 at 14:10
A (better) alternative is this. If you agree with that you may want to close your question as a duplicate of that one.
– Gert Arnold
Jan 1 at 20:46
@DES PRO you can answer your own question after (I think) 48hrs. What you should probably think about doing is mapping your entities to a DTO class before returning from your API, exposing everything from your DB is not always a good idea and returning a DTO will mean your data structure can change without effecting the API contract.
– matt_lethargic
Jan 2 at 10:45
|
show 2 more comments
Please sharePOSTMAN
result/error.
– Umair Anwaar
Jan 1 at 8:48
You probably get a circular reference error from the Json serializer.
– Gert Arnold
Jan 1 at 10:26
Yes it was the circular reference error, I placed Jsonignore above ICollection and now it works great, is there alternative fix for that? btw how can i mark you're commend as answere
– DES PRO
Jan 1 at 14:10
A (better) alternative is this. If you agree with that you may want to close your question as a duplicate of that one.
– Gert Arnold
Jan 1 at 20:46
@DES PRO you can answer your own question after (I think) 48hrs. What you should probably think about doing is mapping your entities to a DTO class before returning from your API, exposing everything from your DB is not always a good idea and returning a DTO will mean your data structure can change without effecting the API contract.
– matt_lethargic
Jan 2 at 10:45
Please share
POSTMAN
result/error.– Umair Anwaar
Jan 1 at 8:48
Please share
POSTMAN
result/error.– Umair Anwaar
Jan 1 at 8:48
You probably get a circular reference error from the Json serializer.
– Gert Arnold
Jan 1 at 10:26
You probably get a circular reference error from the Json serializer.
– Gert Arnold
Jan 1 at 10:26
Yes it was the circular reference error, I placed Jsonignore above ICollection and now it works great, is there alternative fix for that? btw how can i mark you're commend as answere
– DES PRO
Jan 1 at 14:10
Yes it was the circular reference error, I placed Jsonignore above ICollection and now it works great, is there alternative fix for that? btw how can i mark you're commend as answere
– DES PRO
Jan 1 at 14:10
A (better) alternative is this. If you agree with that you may want to close your question as a duplicate of that one.
– Gert Arnold
Jan 1 at 20:46
A (better) alternative is this. If you agree with that you may want to close your question as a duplicate of that one.
– Gert Arnold
Jan 1 at 20:46
@DES PRO you can answer your own question after (I think) 48hrs. What you should probably think about doing is mapping your entities to a DTO class before returning from your API, exposing everything from your DB is not always a good idea and returning a DTO will mean your data structure can change without effecting the API contract.
– matt_lethargic
Jan 2 at 10:45
@DES PRO you can answer your own question after (I think) 48hrs. What you should probably think about doing is mapping your entities to a DTO class before returning from your API, exposing everything from your DB is not always a good idea and returning a DTO will mean your data structure can change without effecting the API contract.
– matt_lethargic
Jan 2 at 10:45
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990662%2fweb-api-returning-no-response-but-data-is-formed-at-return%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990662%2fweb-api-returning-no-response-but-data-is-formed-at-return%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6ptK6sE,JLBB1WhvIR3Cud,3LXPSOKwzf9E,kAp5TskpConLJ3rF1zVp6,ReUAXAzRd2xGnPGofAl7Tgkzj42
Please share
POSTMAN
result/error.– Umair Anwaar
Jan 1 at 8:48
You probably get a circular reference error from the Json serializer.
– Gert Arnold
Jan 1 at 10:26
Yes it was the circular reference error, I placed Jsonignore above ICollection and now it works great, is there alternative fix for that? btw how can i mark you're commend as answere
– DES PRO
Jan 1 at 14:10
A (better) alternative is this. If you agree with that you may want to close your question as a duplicate of that one.
– Gert Arnold
Jan 1 at 20:46
@DES PRO you can answer your own question after (I think) 48hrs. What you should probably think about doing is mapping your entities to a DTO class before returning from your API, exposing everything from your DB is not always a good idea and returning a DTO will mean your data structure can change without effecting the API contract.
– matt_lethargic
Jan 2 at 10:45