Libcurl authentication issues
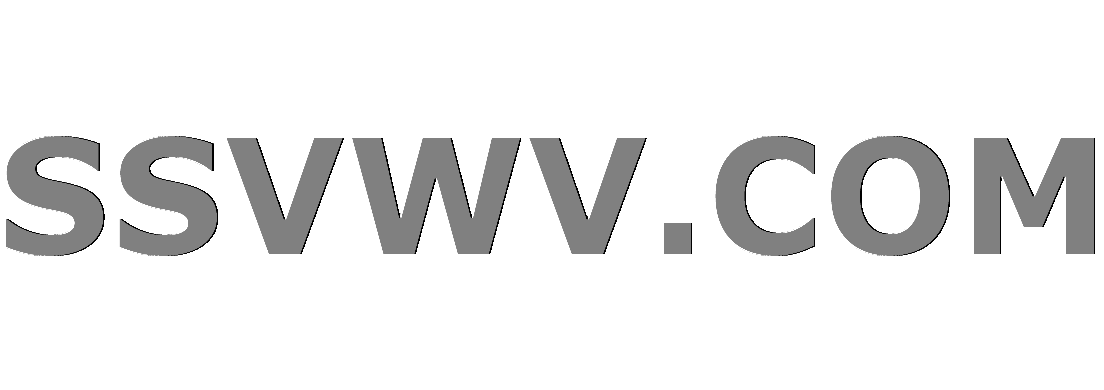
Multi tool use
I'm trying to use libcurl in C++ to send requests to a url. When I set up the request in the command line with curl, it seems to work fine:
curl -vvv -X POST -H "Authorization: <api key here>" -H "Content-Type:application/json" "<host>" --data-binary '<json data here>'
The response starts something like this:
> POST <host> HTTP/1.1
> Host: <host>
> User-Agent: curl/7.61.1
> Accept: */*
> Authorization: <api_key>
> Content-Type:application/json
> Content-Length: 80
So i can see the authorization is being sent properly.
When I try to do a similar thing in C++, using the libcurl C library, however, I don't notice the ">" in front of the request headers:
Code:
struct curl_slist *chunk = NULL;
chunk = curl_slist_append(chunk, "Authorization: <api_key>");
chunk = curl_slist_append(chunk, "Content-Type:application/json");
curl_easy_setopt(curl, CURLOPT_VERBOSE, 1);
curl_easy_setopt(curl, CURLOPT_URL, "<host>");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, chunk);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "<json>");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
Response:
> POST <host> HTTP/1.1
Host: <host>
Accept: */*
Authentication: <api_key>
Content-Type:application/json
Content-Length: 97
So I'm not even sure if the headers are even properly being processed or received by the host.
Any ideas?
I get the following response:
{
"message": "No authorization header given",
"code": 401
}
c++ authentication curl header libcurl
add a comment |
I'm trying to use libcurl in C++ to send requests to a url. When I set up the request in the command line with curl, it seems to work fine:
curl -vvv -X POST -H "Authorization: <api key here>" -H "Content-Type:application/json" "<host>" --data-binary '<json data here>'
The response starts something like this:
> POST <host> HTTP/1.1
> Host: <host>
> User-Agent: curl/7.61.1
> Accept: */*
> Authorization: <api_key>
> Content-Type:application/json
> Content-Length: 80
So i can see the authorization is being sent properly.
When I try to do a similar thing in C++, using the libcurl C library, however, I don't notice the ">" in front of the request headers:
Code:
struct curl_slist *chunk = NULL;
chunk = curl_slist_append(chunk, "Authorization: <api_key>");
chunk = curl_slist_append(chunk, "Content-Type:application/json");
curl_easy_setopt(curl, CURLOPT_VERBOSE, 1);
curl_easy_setopt(curl, CURLOPT_URL, "<host>");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, chunk);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "<json>");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
Response:
> POST <host> HTTP/1.1
Host: <host>
Accept: */*
Authentication: <api_key>
Content-Type:application/json
Content-Length: 97
So I'm not even sure if the headers are even properly being processed or received by the host.
Any ideas?
I get the following response:
{
"message": "No authorization header given",
"code": 401
}
c++ authentication curl header libcurl
1
The "> " are obviously inserted by thecurl
command itself. They are not part ofHTTP
.
– Sam Varshavchik
Dec 31 '18 at 18:55
That's true, but I assume it would be a hint given by the verbosity to what sets of parameters are sent as part of the request?
– Skorpius
Dec 31 '18 at 18:58
1
I have no idea what you meant by that. The curl command, obviously, prepends "> " as a helpful indication that this is the sent part. That's it. Nothing more can be said about it.
– Sam Varshavchik
Dec 31 '18 at 19:01
Interesting, so is there anything suspect about the code itself? the API key works with curl but not with libcurl...
– Skorpius
Dec 31 '18 at 19:48
Good catch. This fixed it. Closing question.
– Skorpius
Dec 31 '18 at 20:01
add a comment |
I'm trying to use libcurl in C++ to send requests to a url. When I set up the request in the command line with curl, it seems to work fine:
curl -vvv -X POST -H "Authorization: <api key here>" -H "Content-Type:application/json" "<host>" --data-binary '<json data here>'
The response starts something like this:
> POST <host> HTTP/1.1
> Host: <host>
> User-Agent: curl/7.61.1
> Accept: */*
> Authorization: <api_key>
> Content-Type:application/json
> Content-Length: 80
So i can see the authorization is being sent properly.
When I try to do a similar thing in C++, using the libcurl C library, however, I don't notice the ">" in front of the request headers:
Code:
struct curl_slist *chunk = NULL;
chunk = curl_slist_append(chunk, "Authorization: <api_key>");
chunk = curl_slist_append(chunk, "Content-Type:application/json");
curl_easy_setopt(curl, CURLOPT_VERBOSE, 1);
curl_easy_setopt(curl, CURLOPT_URL, "<host>");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, chunk);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "<json>");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
Response:
> POST <host> HTTP/1.1
Host: <host>
Accept: */*
Authentication: <api_key>
Content-Type:application/json
Content-Length: 97
So I'm not even sure if the headers are even properly being processed or received by the host.
Any ideas?
I get the following response:
{
"message": "No authorization header given",
"code": 401
}
c++ authentication curl header libcurl
I'm trying to use libcurl in C++ to send requests to a url. When I set up the request in the command line with curl, it seems to work fine:
curl -vvv -X POST -H "Authorization: <api key here>" -H "Content-Type:application/json" "<host>" --data-binary '<json data here>'
The response starts something like this:
> POST <host> HTTP/1.1
> Host: <host>
> User-Agent: curl/7.61.1
> Accept: */*
> Authorization: <api_key>
> Content-Type:application/json
> Content-Length: 80
So i can see the authorization is being sent properly.
When I try to do a similar thing in C++, using the libcurl C library, however, I don't notice the ">" in front of the request headers:
Code:
struct curl_slist *chunk = NULL;
chunk = curl_slist_append(chunk, "Authorization: <api_key>");
chunk = curl_slist_append(chunk, "Content-Type:application/json");
curl_easy_setopt(curl, CURLOPT_VERBOSE, 1);
curl_easy_setopt(curl, CURLOPT_URL, "<host>");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, chunk);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "<json>");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
Response:
> POST <host> HTTP/1.1
Host: <host>
Accept: */*
Authentication: <api_key>
Content-Type:application/json
Content-Length: 97
So I'm not even sure if the headers are even properly being processed or received by the host.
Any ideas?
I get the following response:
{
"message": "No authorization header given",
"code": 401
}
c++ authentication curl header libcurl
c++ authentication curl header libcurl
edited Dec 31 '18 at 19:51
Skorpius
asked Dec 31 '18 at 18:47
SkorpiusSkorpius
83921325
83921325
1
The "> " are obviously inserted by thecurl
command itself. They are not part ofHTTP
.
– Sam Varshavchik
Dec 31 '18 at 18:55
That's true, but I assume it would be a hint given by the verbosity to what sets of parameters are sent as part of the request?
– Skorpius
Dec 31 '18 at 18:58
1
I have no idea what you meant by that. The curl command, obviously, prepends "> " as a helpful indication that this is the sent part. That's it. Nothing more can be said about it.
– Sam Varshavchik
Dec 31 '18 at 19:01
Interesting, so is there anything suspect about the code itself? the API key works with curl but not with libcurl...
– Skorpius
Dec 31 '18 at 19:48
Good catch. This fixed it. Closing question.
– Skorpius
Dec 31 '18 at 20:01
add a comment |
1
The "> " are obviously inserted by thecurl
command itself. They are not part ofHTTP
.
– Sam Varshavchik
Dec 31 '18 at 18:55
That's true, but I assume it would be a hint given by the verbosity to what sets of parameters are sent as part of the request?
– Skorpius
Dec 31 '18 at 18:58
1
I have no idea what you meant by that. The curl command, obviously, prepends "> " as a helpful indication that this is the sent part. That's it. Nothing more can be said about it.
– Sam Varshavchik
Dec 31 '18 at 19:01
Interesting, so is there anything suspect about the code itself? the API key works with curl but not with libcurl...
– Skorpius
Dec 31 '18 at 19:48
Good catch. This fixed it. Closing question.
– Skorpius
Dec 31 '18 at 20:01
1
1
The "> " are obviously inserted by the
curl
command itself. They are not part of HTTP
.– Sam Varshavchik
Dec 31 '18 at 18:55
The "> " are obviously inserted by the
curl
command itself. They are not part of HTTP
.– Sam Varshavchik
Dec 31 '18 at 18:55
That's true, but I assume it would be a hint given by the verbosity to what sets of parameters are sent as part of the request?
– Skorpius
Dec 31 '18 at 18:58
That's true, but I assume it would be a hint given by the verbosity to what sets of parameters are sent as part of the request?
– Skorpius
Dec 31 '18 at 18:58
1
1
I have no idea what you meant by that. The curl command, obviously, prepends "> " as a helpful indication that this is the sent part. That's it. Nothing more can be said about it.
– Sam Varshavchik
Dec 31 '18 at 19:01
I have no idea what you meant by that. The curl command, obviously, prepends "> " as a helpful indication that this is the sent part. That's it. Nothing more can be said about it.
– Sam Varshavchik
Dec 31 '18 at 19:01
Interesting, so is there anything suspect about the code itself? the API key works with curl but not with libcurl...
– Skorpius
Dec 31 '18 at 19:48
Interesting, so is there anything suspect about the code itself? the API key works with curl but not with libcurl...
– Skorpius
Dec 31 '18 at 19:48
Good catch. This fixed it. Closing question.
– Skorpius
Dec 31 '18 at 20:01
Good catch. This fixed it. Closing question.
– Skorpius
Dec 31 '18 at 20:01
add a comment |
2 Answers
2
active
oldest
votes
Incorrect Header. Should be Authorization, not Authentication.
I have posted my comments as answer, its maybe usefull for other users.
– Steffen Mächtel
Dec 31 '18 at 20:15
add a comment |
In your command line verbose output the header is named "Authorization: ". In your libcurl vebose output its "Authentication: ". Authorization != Authentication?
Verbose output:
Its only a different output format for verbose between command line and libcurl. The headers are sent. Same output format is used for example by php curl. Only first line has ">" and then all following headers has no ">". But they are all submited.
PHP curl verbose example output:
* Trying ::1...
* TCP_NODELAY set
* Connected to localhost (::1) port 80 (#0)
> GET /XXX/api.php HTTP/1.1
Host: localhost
Accept: */*
Authorization: XXX
Content-Type: application/json
< HTTP/1.1 200 OK
< Date: Mon, 31 Dec 2018 20:12:51 GMT
< Server: Apache/2.4.34 (Win32) OpenSSL/1.1.0i PHP/7.2.10
< X-Powered-By: PHP/7.2.10
< Content-Length: 2390
< Content-Type: text/html; charset=UTF-8
<
* Connection #0 to host localhost left intact
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990526%2flibcurl-authentication-issues%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Incorrect Header. Should be Authorization, not Authentication.
I have posted my comments as answer, its maybe usefull for other users.
– Steffen Mächtel
Dec 31 '18 at 20:15
add a comment |
Incorrect Header. Should be Authorization, not Authentication.
I have posted my comments as answer, its maybe usefull for other users.
– Steffen Mächtel
Dec 31 '18 at 20:15
add a comment |
Incorrect Header. Should be Authorization, not Authentication.
Incorrect Header. Should be Authorization, not Authentication.
answered Dec 31 '18 at 20:02
SkorpiusSkorpius
83921325
83921325
I have posted my comments as answer, its maybe usefull for other users.
– Steffen Mächtel
Dec 31 '18 at 20:15
add a comment |
I have posted my comments as answer, its maybe usefull for other users.
– Steffen Mächtel
Dec 31 '18 at 20:15
I have posted my comments as answer, its maybe usefull for other users.
– Steffen Mächtel
Dec 31 '18 at 20:15
I have posted my comments as answer, its maybe usefull for other users.
– Steffen Mächtel
Dec 31 '18 at 20:15
add a comment |
In your command line verbose output the header is named "Authorization: ". In your libcurl vebose output its "Authentication: ". Authorization != Authentication?
Verbose output:
Its only a different output format for verbose between command line and libcurl. The headers are sent. Same output format is used for example by php curl. Only first line has ">" and then all following headers has no ">". But they are all submited.
PHP curl verbose example output:
* Trying ::1...
* TCP_NODELAY set
* Connected to localhost (::1) port 80 (#0)
> GET /XXX/api.php HTTP/1.1
Host: localhost
Accept: */*
Authorization: XXX
Content-Type: application/json
< HTTP/1.1 200 OK
< Date: Mon, 31 Dec 2018 20:12:51 GMT
< Server: Apache/2.4.34 (Win32) OpenSSL/1.1.0i PHP/7.2.10
< X-Powered-By: PHP/7.2.10
< Content-Length: 2390
< Content-Type: text/html; charset=UTF-8
<
* Connection #0 to host localhost left intact
add a comment |
In your command line verbose output the header is named "Authorization: ". In your libcurl vebose output its "Authentication: ". Authorization != Authentication?
Verbose output:
Its only a different output format for verbose between command line and libcurl. The headers are sent. Same output format is used for example by php curl. Only first line has ">" and then all following headers has no ">". But they are all submited.
PHP curl verbose example output:
* Trying ::1...
* TCP_NODELAY set
* Connected to localhost (::1) port 80 (#0)
> GET /XXX/api.php HTTP/1.1
Host: localhost
Accept: */*
Authorization: XXX
Content-Type: application/json
< HTTP/1.1 200 OK
< Date: Mon, 31 Dec 2018 20:12:51 GMT
< Server: Apache/2.4.34 (Win32) OpenSSL/1.1.0i PHP/7.2.10
< X-Powered-By: PHP/7.2.10
< Content-Length: 2390
< Content-Type: text/html; charset=UTF-8
<
* Connection #0 to host localhost left intact
add a comment |
In your command line verbose output the header is named "Authorization: ". In your libcurl vebose output its "Authentication: ". Authorization != Authentication?
Verbose output:
Its only a different output format for verbose between command line and libcurl. The headers are sent. Same output format is used for example by php curl. Only first line has ">" and then all following headers has no ">". But they are all submited.
PHP curl verbose example output:
* Trying ::1...
* TCP_NODELAY set
* Connected to localhost (::1) port 80 (#0)
> GET /XXX/api.php HTTP/1.1
Host: localhost
Accept: */*
Authorization: XXX
Content-Type: application/json
< HTTP/1.1 200 OK
< Date: Mon, 31 Dec 2018 20:12:51 GMT
< Server: Apache/2.4.34 (Win32) OpenSSL/1.1.0i PHP/7.2.10
< X-Powered-By: PHP/7.2.10
< Content-Length: 2390
< Content-Type: text/html; charset=UTF-8
<
* Connection #0 to host localhost left intact
In your command line verbose output the header is named "Authorization: ". In your libcurl vebose output its "Authentication: ". Authorization != Authentication?
Verbose output:
Its only a different output format for verbose between command line and libcurl. The headers are sent. Same output format is used for example by php curl. Only first line has ">" and then all following headers has no ">". But they are all submited.
PHP curl verbose example output:
* Trying ::1...
* TCP_NODELAY set
* Connected to localhost (::1) port 80 (#0)
> GET /XXX/api.php HTTP/1.1
Host: localhost
Accept: */*
Authorization: XXX
Content-Type: application/json
< HTTP/1.1 200 OK
< Date: Mon, 31 Dec 2018 20:12:51 GMT
< Server: Apache/2.4.34 (Win32) OpenSSL/1.1.0i PHP/7.2.10
< X-Powered-By: PHP/7.2.10
< Content-Length: 2390
< Content-Type: text/html; charset=UTF-8
<
* Connection #0 to host localhost left intact
answered Dec 31 '18 at 20:14


Steffen MächtelSteffen Mächtel
611511
611511
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990526%2flibcurl-authentication-issues%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tN8S3ss6 8k61 f8vbWhWF,98Clt074wDHu20ymU2hun BDHicx89znB7x2Og6
1
The "> " are obviously inserted by the
curl
command itself. They are not part ofHTTP
.– Sam Varshavchik
Dec 31 '18 at 18:55
That's true, but I assume it would be a hint given by the verbosity to what sets of parameters are sent as part of the request?
– Skorpius
Dec 31 '18 at 18:58
1
I have no idea what you meant by that. The curl command, obviously, prepends "> " as a helpful indication that this is the sent part. That's it. Nothing more can be said about it.
– Sam Varshavchik
Dec 31 '18 at 19:01
Interesting, so is there anything suspect about the code itself? the API key works with curl but not with libcurl...
– Skorpius
Dec 31 '18 at 19:48
Good catch. This fixed it. Closing question.
– Skorpius
Dec 31 '18 at 20:01