Single page design - loading new content on link click
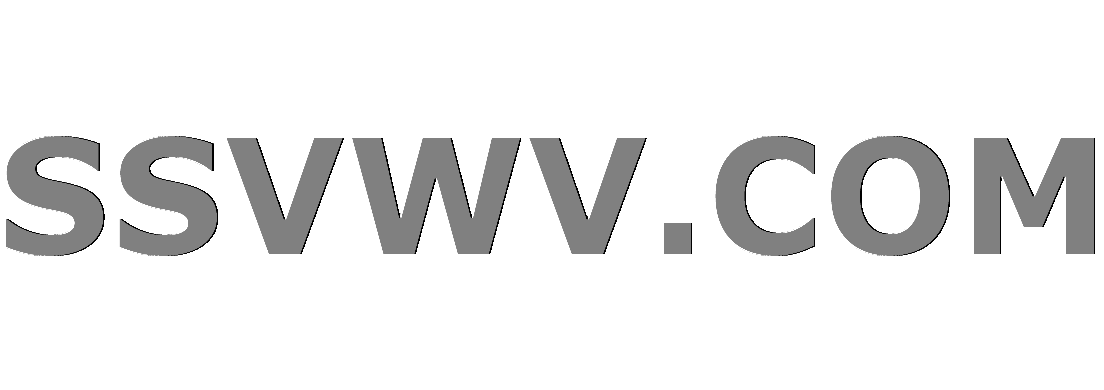
Multi tool use
I'm working on a django webpage using single page design approach. Generally what I'm trying to achieve is to have some new content being loaded (picture gallery) to my main webpage after clicking certain links.
What I have already achieved is that the new content is being loaded on a link click but unfortunately it seems like the whole page is being reloaded and not rendered correctly. My current implementation is based on having main index.html
template and extension template, both using {% block content %}
relation.
views.py
def index(request):
categories = Category.objects.all().order_by('name')
return render(request, 'index.html', {'categories': categories})
def gallery(request, id):
category = Category.objects.get(id=id)
return render(request, 'gallery.html', {'category': category})
urls.py
urlpatterns = [
path('', views.index, name='index'),
path('view_gallery/<int:id>/', views.gallery, name='view_gallery')
]
index.html
<div class="collapse navbar-collapse" id="collapsibleNavbar">
<ul class="navbar-nav">
{% for category in categories%}
<li class="nav-item">
<a class="nav-link" href="{% url 'view_gallery' category.id %}">{{ category.name }}</a>
</li>
{% endfor %}
</ul>
</div>
<div>
{% block content %}
{% endblock %}
</div>
gallery.html
{% extends 'index.html' %}
{% block content %}
<p>{{ category.name }}</p>
{% endblock %}
I hope I have explained clearly what I'm trying to achieve. Could you please point me in the right direction?
django django-templates django-views
add a comment |
I'm working on a django webpage using single page design approach. Generally what I'm trying to achieve is to have some new content being loaded (picture gallery) to my main webpage after clicking certain links.
What I have already achieved is that the new content is being loaded on a link click but unfortunately it seems like the whole page is being reloaded and not rendered correctly. My current implementation is based on having main index.html
template and extension template, both using {% block content %}
relation.
views.py
def index(request):
categories = Category.objects.all().order_by('name')
return render(request, 'index.html', {'categories': categories})
def gallery(request, id):
category = Category.objects.get(id=id)
return render(request, 'gallery.html', {'category': category})
urls.py
urlpatterns = [
path('', views.index, name='index'),
path('view_gallery/<int:id>/', views.gallery, name='view_gallery')
]
index.html
<div class="collapse navbar-collapse" id="collapsibleNavbar">
<ul class="navbar-nav">
{% for category in categories%}
<li class="nav-item">
<a class="nav-link" href="{% url 'view_gallery' category.id %}">{{ category.name }}</a>
</li>
{% endfor %}
</ul>
</div>
<div>
{% block content %}
{% endblock %}
</div>
gallery.html
{% extends 'index.html' %}
{% block content %}
<p>{{ category.name }}</p>
{% endblock %}
I hope I have explained clearly what I'm trying to achieve. Could you please point me in the right direction?
django django-templates django-views
add a comment |
I'm working on a django webpage using single page design approach. Generally what I'm trying to achieve is to have some new content being loaded (picture gallery) to my main webpage after clicking certain links.
What I have already achieved is that the new content is being loaded on a link click but unfortunately it seems like the whole page is being reloaded and not rendered correctly. My current implementation is based on having main index.html
template and extension template, both using {% block content %}
relation.
views.py
def index(request):
categories = Category.objects.all().order_by('name')
return render(request, 'index.html', {'categories': categories})
def gallery(request, id):
category = Category.objects.get(id=id)
return render(request, 'gallery.html', {'category': category})
urls.py
urlpatterns = [
path('', views.index, name='index'),
path('view_gallery/<int:id>/', views.gallery, name='view_gallery')
]
index.html
<div class="collapse navbar-collapse" id="collapsibleNavbar">
<ul class="navbar-nav">
{% for category in categories%}
<li class="nav-item">
<a class="nav-link" href="{% url 'view_gallery' category.id %}">{{ category.name }}</a>
</li>
{% endfor %}
</ul>
</div>
<div>
{% block content %}
{% endblock %}
</div>
gallery.html
{% extends 'index.html' %}
{% block content %}
<p>{{ category.name }}</p>
{% endblock %}
I hope I have explained clearly what I'm trying to achieve. Could you please point me in the right direction?
django django-templates django-views
I'm working on a django webpage using single page design approach. Generally what I'm trying to achieve is to have some new content being loaded (picture gallery) to my main webpage after clicking certain links.
What I have already achieved is that the new content is being loaded on a link click but unfortunately it seems like the whole page is being reloaded and not rendered correctly. My current implementation is based on having main index.html
template and extension template, both using {% block content %}
relation.
views.py
def index(request):
categories = Category.objects.all().order_by('name')
return render(request, 'index.html', {'categories': categories})
def gallery(request, id):
category = Category.objects.get(id=id)
return render(request, 'gallery.html', {'category': category})
urls.py
urlpatterns = [
path('', views.index, name='index'),
path('view_gallery/<int:id>/', views.gallery, name='view_gallery')
]
index.html
<div class="collapse navbar-collapse" id="collapsibleNavbar">
<ul class="navbar-nav">
{% for category in categories%}
<li class="nav-item">
<a class="nav-link" href="{% url 'view_gallery' category.id %}">{{ category.name }}</a>
</li>
{% endfor %}
</ul>
</div>
<div>
{% block content %}
{% endblock %}
</div>
gallery.html
{% extends 'index.html' %}
{% block content %}
<p>{{ category.name }}</p>
{% endblock %}
I hope I have explained clearly what I'm trying to achieve. Could you please point me in the right direction?
django django-templates django-views
django django-templates django-views
asked Dec 31 '18 at 18:43
ArxasArxas
415
415
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Requests and page changes in single page sites work quite differently than those for normal web pages. Single page sites use JavaScript to change the page content rather than requesting a new page from the server. Single page sites can request content from the server, but generally that content is just data while the structure (HTML) of the page is decided by the client in JavaScript. The only time a full HTML page is sent by the server is on the initial request, which should be responded to with index.html
.
In your example, you could have this work by adding a script that requests content from the server and modifies the DOM when a link is clicked.
For example:
const a1 = document.querySelector("a.link1");
const a2 = document.querySelector("a.link2");
a1.addEventListener("click", () => {
setContent("<p>Content from link 1</p>")
});
a2.addEventListener("click", () => {
setContent("<p>Content from link 2</p>")
});
function setContent(content) {
const contentDiv = document.querySelector("div.content");
contentDiv.innerHTML = content;
}
a {
text-decoration: underline;
color: blue;
}
a:hover {
cursor: pointer;
}
<h1>My Page</h1>
<a class="link1">link 1</a>
<a class="link2">link 2</a>
<div class="content">
</div>
And the click event callbacks could request content from your server instead of having the content hard-coded as in this example. Note that then the server should respond with just a snippet of HTML rather than an entire new page.
For example, you could use the following function to get content for the div.content
element:
function fetchData() {
const response = fetch("/gallery");
return response;
}
If you're new to single page sites, you might checkout a framework like React, Vue, or Angular to get started and gain a better understanding or even use for this project.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990502%2fsingle-page-design-loading-new-content-on-link-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Requests and page changes in single page sites work quite differently than those for normal web pages. Single page sites use JavaScript to change the page content rather than requesting a new page from the server. Single page sites can request content from the server, but generally that content is just data while the structure (HTML) of the page is decided by the client in JavaScript. The only time a full HTML page is sent by the server is on the initial request, which should be responded to with index.html
.
In your example, you could have this work by adding a script that requests content from the server and modifies the DOM when a link is clicked.
For example:
const a1 = document.querySelector("a.link1");
const a2 = document.querySelector("a.link2");
a1.addEventListener("click", () => {
setContent("<p>Content from link 1</p>")
});
a2.addEventListener("click", () => {
setContent("<p>Content from link 2</p>")
});
function setContent(content) {
const contentDiv = document.querySelector("div.content");
contentDiv.innerHTML = content;
}
a {
text-decoration: underline;
color: blue;
}
a:hover {
cursor: pointer;
}
<h1>My Page</h1>
<a class="link1">link 1</a>
<a class="link2">link 2</a>
<div class="content">
</div>
And the click event callbacks could request content from your server instead of having the content hard-coded as in this example. Note that then the server should respond with just a snippet of HTML rather than an entire new page.
For example, you could use the following function to get content for the div.content
element:
function fetchData() {
const response = fetch("/gallery");
return response;
}
If you're new to single page sites, you might checkout a framework like React, Vue, or Angular to get started and gain a better understanding or even use for this project.
add a comment |
Requests and page changes in single page sites work quite differently than those for normal web pages. Single page sites use JavaScript to change the page content rather than requesting a new page from the server. Single page sites can request content from the server, but generally that content is just data while the structure (HTML) of the page is decided by the client in JavaScript. The only time a full HTML page is sent by the server is on the initial request, which should be responded to with index.html
.
In your example, you could have this work by adding a script that requests content from the server and modifies the DOM when a link is clicked.
For example:
const a1 = document.querySelector("a.link1");
const a2 = document.querySelector("a.link2");
a1.addEventListener("click", () => {
setContent("<p>Content from link 1</p>")
});
a2.addEventListener("click", () => {
setContent("<p>Content from link 2</p>")
});
function setContent(content) {
const contentDiv = document.querySelector("div.content");
contentDiv.innerHTML = content;
}
a {
text-decoration: underline;
color: blue;
}
a:hover {
cursor: pointer;
}
<h1>My Page</h1>
<a class="link1">link 1</a>
<a class="link2">link 2</a>
<div class="content">
</div>
And the click event callbacks could request content from your server instead of having the content hard-coded as in this example. Note that then the server should respond with just a snippet of HTML rather than an entire new page.
For example, you could use the following function to get content for the div.content
element:
function fetchData() {
const response = fetch("/gallery");
return response;
}
If you're new to single page sites, you might checkout a framework like React, Vue, or Angular to get started and gain a better understanding or even use for this project.
add a comment |
Requests and page changes in single page sites work quite differently than those for normal web pages. Single page sites use JavaScript to change the page content rather than requesting a new page from the server. Single page sites can request content from the server, but generally that content is just data while the structure (HTML) of the page is decided by the client in JavaScript. The only time a full HTML page is sent by the server is on the initial request, which should be responded to with index.html
.
In your example, you could have this work by adding a script that requests content from the server and modifies the DOM when a link is clicked.
For example:
const a1 = document.querySelector("a.link1");
const a2 = document.querySelector("a.link2");
a1.addEventListener("click", () => {
setContent("<p>Content from link 1</p>")
});
a2.addEventListener("click", () => {
setContent("<p>Content from link 2</p>")
});
function setContent(content) {
const contentDiv = document.querySelector("div.content");
contentDiv.innerHTML = content;
}
a {
text-decoration: underline;
color: blue;
}
a:hover {
cursor: pointer;
}
<h1>My Page</h1>
<a class="link1">link 1</a>
<a class="link2">link 2</a>
<div class="content">
</div>
And the click event callbacks could request content from your server instead of having the content hard-coded as in this example. Note that then the server should respond with just a snippet of HTML rather than an entire new page.
For example, you could use the following function to get content for the div.content
element:
function fetchData() {
const response = fetch("/gallery");
return response;
}
If you're new to single page sites, you might checkout a framework like React, Vue, or Angular to get started and gain a better understanding or even use for this project.
Requests and page changes in single page sites work quite differently than those for normal web pages. Single page sites use JavaScript to change the page content rather than requesting a new page from the server. Single page sites can request content from the server, but generally that content is just data while the structure (HTML) of the page is decided by the client in JavaScript. The only time a full HTML page is sent by the server is on the initial request, which should be responded to with index.html
.
In your example, you could have this work by adding a script that requests content from the server and modifies the DOM when a link is clicked.
For example:
const a1 = document.querySelector("a.link1");
const a2 = document.querySelector("a.link2");
a1.addEventListener("click", () => {
setContent("<p>Content from link 1</p>")
});
a2.addEventListener("click", () => {
setContent("<p>Content from link 2</p>")
});
function setContent(content) {
const contentDiv = document.querySelector("div.content");
contentDiv.innerHTML = content;
}
a {
text-decoration: underline;
color: blue;
}
a:hover {
cursor: pointer;
}
<h1>My Page</h1>
<a class="link1">link 1</a>
<a class="link2">link 2</a>
<div class="content">
</div>
And the click event callbacks could request content from your server instead of having the content hard-coded as in this example. Note that then the server should respond with just a snippet of HTML rather than an entire new page.
For example, you could use the following function to get content for the div.content
element:
function fetchData() {
const response = fetch("/gallery");
return response;
}
If you're new to single page sites, you might checkout a framework like React, Vue, or Angular to get started and gain a better understanding or even use for this project.
const a1 = document.querySelector("a.link1");
const a2 = document.querySelector("a.link2");
a1.addEventListener("click", () => {
setContent("<p>Content from link 1</p>")
});
a2.addEventListener("click", () => {
setContent("<p>Content from link 2</p>")
});
function setContent(content) {
const contentDiv = document.querySelector("div.content");
contentDiv.innerHTML = content;
}
a {
text-decoration: underline;
color: blue;
}
a:hover {
cursor: pointer;
}
<h1>My Page</h1>
<a class="link1">link 1</a>
<a class="link2">link 2</a>
<div class="content">
</div>
const a1 = document.querySelector("a.link1");
const a2 = document.querySelector("a.link2");
a1.addEventListener("click", () => {
setContent("<p>Content from link 1</p>")
});
a2.addEventListener("click", () => {
setContent("<p>Content from link 2</p>")
});
function setContent(content) {
const contentDiv = document.querySelector("div.content");
contentDiv.innerHTML = content;
}
a {
text-decoration: underline;
color: blue;
}
a:hover {
cursor: pointer;
}
<h1>My Page</h1>
<a class="link1">link 1</a>
<a class="link2">link 2</a>
<div class="content">
</div>
edited Dec 31 '18 at 20:37
answered Dec 31 '18 at 19:02


Henry WoodyHenry Woody
4,3523924
4,3523924
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990502%2fsingle-page-design-loading-new-content-on-link-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S9C7K0IOpJgGHej4Ha98,1kdykCM hTZfnN6,SzpS