Accessing superclass method of subclass through another class in java
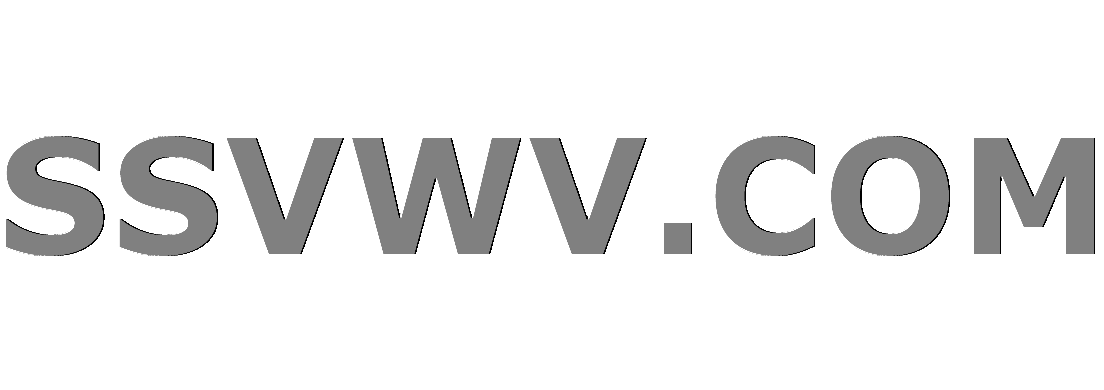
Multi tool use
What is the best solution for this problem?
This is the superClass
public class Views<T> {
private T controller;
public Views() {
}
public Stage getStage(Node object){
return (Stage) object.getScene().getWindow();
}
public T getController(){
return controller;
}
public void setController(T controller){
this.controller = controller;
}
}
I have this interface:
public interface UserView extends Observer{
public void setLoginObjects(Parent root);
public void loginStartUI() throws Exception;
public void setCriarUserObjects(Parent root, User user);
public void criarUserStartUI(User user) throws Exception;
}
I have this subclass of Views:
public class UserViewImpl extends Views<UserController> implements UserView{
...
And i have this class:
public class UserController {
private UserView view;
private UserDAOImpl model;
public UserController(UserView view, UserDAOImpl model) {
this.model = model;
this.view = view;
view.setController(this);
this.model.addObserver(view);
}
...
What is the best solution for this problem?
Should i put together Views
and UserView
? (The problem for this solution is i will have more views, for example i have ParqueView
and ParqueViewImpl
, that basically will have the same methods as Views
, so there would be repeated code and i want to avoid that)
The main problem is that inside my UserController
i pass as parameter UserView
, which does not have access to super class methods, and i need to access setController
which is located in Views
class, it doesnt make sense to change the type of UserView
to Views in userController
because of the MVC
java interface subclass superclass
|
show 4 more comments
What is the best solution for this problem?
This is the superClass
public class Views<T> {
private T controller;
public Views() {
}
public Stage getStage(Node object){
return (Stage) object.getScene().getWindow();
}
public T getController(){
return controller;
}
public void setController(T controller){
this.controller = controller;
}
}
I have this interface:
public interface UserView extends Observer{
public void setLoginObjects(Parent root);
public void loginStartUI() throws Exception;
public void setCriarUserObjects(Parent root, User user);
public void criarUserStartUI(User user) throws Exception;
}
I have this subclass of Views:
public class UserViewImpl extends Views<UserController> implements UserView{
...
And i have this class:
public class UserController {
private UserView view;
private UserDAOImpl model;
public UserController(UserView view, UserDAOImpl model) {
this.model = model;
this.view = view;
view.setController(this);
this.model.addObserver(view);
}
...
What is the best solution for this problem?
Should i put together Views
and UserView
? (The problem for this solution is i will have more views, for example i have ParqueView
and ParqueViewImpl
, that basically will have the same methods as Views
, so there would be repeated code and i want to avoid that)
The main problem is that inside my UserController
i pass as parameter UserView
, which does not have access to super class methods, and i need to access setController
which is located in Views
class, it doesnt make sense to change the type of UserView
to Views in userController
because of the MVC
java interface subclass superclass
2
"What is the best solution for this problem?" - You never explained what the problem is (the title does not suffice).
– Jacob G.
Dec 31 '18 at 18:53
WouldParqueView
also have/need the methods fromUserView
?
– user7
Dec 31 '18 at 18:55
@user7 no, ParqueView needs the methods from Views
– Hugo Modesto
Dec 31 '18 at 18:56
@JacobG. sorry i though the problem was pretty obvious, ill edit the post!
– Hugo Modesto
Dec 31 '18 at 18:56
You could make View an interface and makeUserView
implement it?
– user7
Dec 31 '18 at 19:01
|
show 4 more comments
What is the best solution for this problem?
This is the superClass
public class Views<T> {
private T controller;
public Views() {
}
public Stage getStage(Node object){
return (Stage) object.getScene().getWindow();
}
public T getController(){
return controller;
}
public void setController(T controller){
this.controller = controller;
}
}
I have this interface:
public interface UserView extends Observer{
public void setLoginObjects(Parent root);
public void loginStartUI() throws Exception;
public void setCriarUserObjects(Parent root, User user);
public void criarUserStartUI(User user) throws Exception;
}
I have this subclass of Views:
public class UserViewImpl extends Views<UserController> implements UserView{
...
And i have this class:
public class UserController {
private UserView view;
private UserDAOImpl model;
public UserController(UserView view, UserDAOImpl model) {
this.model = model;
this.view = view;
view.setController(this);
this.model.addObserver(view);
}
...
What is the best solution for this problem?
Should i put together Views
and UserView
? (The problem for this solution is i will have more views, for example i have ParqueView
and ParqueViewImpl
, that basically will have the same methods as Views
, so there would be repeated code and i want to avoid that)
The main problem is that inside my UserController
i pass as parameter UserView
, which does not have access to super class methods, and i need to access setController
which is located in Views
class, it doesnt make sense to change the type of UserView
to Views in userController
because of the MVC
java interface subclass superclass
What is the best solution for this problem?
This is the superClass
public class Views<T> {
private T controller;
public Views() {
}
public Stage getStage(Node object){
return (Stage) object.getScene().getWindow();
}
public T getController(){
return controller;
}
public void setController(T controller){
this.controller = controller;
}
}
I have this interface:
public interface UserView extends Observer{
public void setLoginObjects(Parent root);
public void loginStartUI() throws Exception;
public void setCriarUserObjects(Parent root, User user);
public void criarUserStartUI(User user) throws Exception;
}
I have this subclass of Views:
public class UserViewImpl extends Views<UserController> implements UserView{
...
And i have this class:
public class UserController {
private UserView view;
private UserDAOImpl model;
public UserController(UserView view, UserDAOImpl model) {
this.model = model;
this.view = view;
view.setController(this);
this.model.addObserver(view);
}
...
What is the best solution for this problem?
Should i put together Views
and UserView
? (The problem for this solution is i will have more views, for example i have ParqueView
and ParqueViewImpl
, that basically will have the same methods as Views
, so there would be repeated code and i want to avoid that)
The main problem is that inside my UserController
i pass as parameter UserView
, which does not have access to super class methods, and i need to access setController
which is located in Views
class, it doesnt make sense to change the type of UserView
to Views in userController
because of the MVC
java interface subclass superclass
java interface subclass superclass
edited Dec 31 '18 at 19:02
Hugo Modesto
asked Dec 31 '18 at 18:50
Hugo ModestoHugo Modesto
188
188
2
"What is the best solution for this problem?" - You never explained what the problem is (the title does not suffice).
– Jacob G.
Dec 31 '18 at 18:53
WouldParqueView
also have/need the methods fromUserView
?
– user7
Dec 31 '18 at 18:55
@user7 no, ParqueView needs the methods from Views
– Hugo Modesto
Dec 31 '18 at 18:56
@JacobG. sorry i though the problem was pretty obvious, ill edit the post!
– Hugo Modesto
Dec 31 '18 at 18:56
You could make View an interface and makeUserView
implement it?
– user7
Dec 31 '18 at 19:01
|
show 4 more comments
2
"What is the best solution for this problem?" - You never explained what the problem is (the title does not suffice).
– Jacob G.
Dec 31 '18 at 18:53
WouldParqueView
also have/need the methods fromUserView
?
– user7
Dec 31 '18 at 18:55
@user7 no, ParqueView needs the methods from Views
– Hugo Modesto
Dec 31 '18 at 18:56
@JacobG. sorry i though the problem was pretty obvious, ill edit the post!
– Hugo Modesto
Dec 31 '18 at 18:56
You could make View an interface and makeUserView
implement it?
– user7
Dec 31 '18 at 19:01
2
2
"What is the best solution for this problem?" - You never explained what the problem is (the title does not suffice).
– Jacob G.
Dec 31 '18 at 18:53
"What is the best solution for this problem?" - You never explained what the problem is (the title does not suffice).
– Jacob G.
Dec 31 '18 at 18:53
Would
ParqueView
also have/need the methods from UserView
?– user7
Dec 31 '18 at 18:55
Would
ParqueView
also have/need the methods from UserView
?– user7
Dec 31 '18 at 18:55
@user7 no, ParqueView needs the methods from Views
– Hugo Modesto
Dec 31 '18 at 18:56
@user7 no, ParqueView needs the methods from Views
– Hugo Modesto
Dec 31 '18 at 18:56
@JacobG. sorry i though the problem was pretty obvious, ill edit the post!
– Hugo Modesto
Dec 31 '18 at 18:56
@JacobG. sorry i though the problem was pretty obvious, ill edit the post!
– Hugo Modesto
Dec 31 '18 at 18:56
You could make View an interface and make
UserView
implement it?– user7
Dec 31 '18 at 19:01
You could make View an interface and make
UserView
implement it?– user7
Dec 31 '18 at 19:01
|
show 4 more comments
1 Answer
1
active
oldest
votes
It looks like you do not need generics here. Simply using an interface should suffice.
Create a Controller
interface, which your two controllers will implement. Then you can get rid of the <T>
entirely and use this interface instead.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990548%2faccessing-superclass-method-of-subclass-through-another-class-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It looks like you do not need generics here. Simply using an interface should suffice.
Create a Controller
interface, which your two controllers will implement. Then you can get rid of the <T>
entirely and use this interface instead.
add a comment |
It looks like you do not need generics here. Simply using an interface should suffice.
Create a Controller
interface, which your two controllers will implement. Then you can get rid of the <T>
entirely and use this interface instead.
add a comment |
It looks like you do not need generics here. Simply using an interface should suffice.
Create a Controller
interface, which your two controllers will implement. Then you can get rid of the <T>
entirely and use this interface instead.
It looks like you do not need generics here. Simply using an interface should suffice.
Create a Controller
interface, which your two controllers will implement. Then you can get rid of the <T>
entirely and use this interface instead.
answered Dec 31 '18 at 19:08
Joe CJoe C
11.5k62543
11.5k62543
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990548%2faccessing-superclass-method-of-subclass-through-another-class-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w0,R9TtHJgc2igH,cI5pdgU3TwO 02jX 0vl9nMq90Vvpn3VoyL7Lfo4LPLLbO2J,Cn0Oy1Aq3 09QWqp JVzOI9uWKu7Wv
2
"What is the best solution for this problem?" - You never explained what the problem is (the title does not suffice).
– Jacob G.
Dec 31 '18 at 18:53
Would
ParqueView
also have/need the methods fromUserView
?– user7
Dec 31 '18 at 18:55
@user7 no, ParqueView needs the methods from Views
– Hugo Modesto
Dec 31 '18 at 18:56
@JacobG. sorry i though the problem was pretty obvious, ill edit the post!
– Hugo Modesto
Dec 31 '18 at 18:56
You could make View an interface and make
UserView
implement it?– user7
Dec 31 '18 at 19:01