custom pandas.DataFrame class
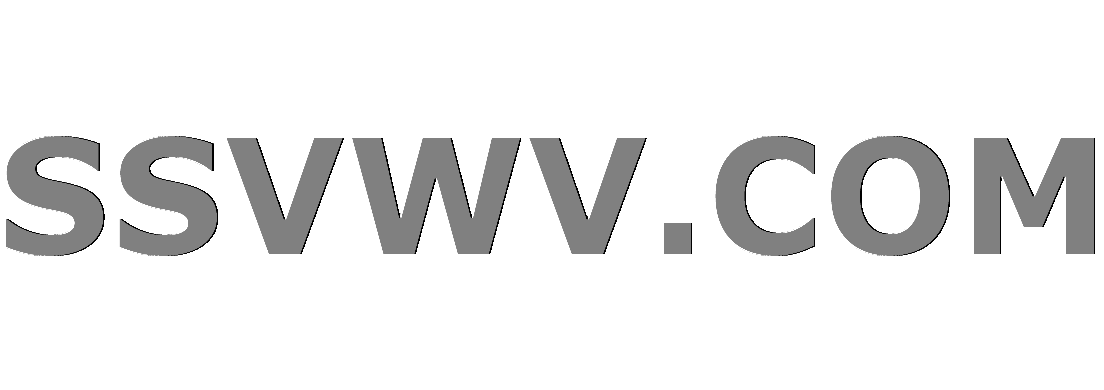
Multi tool use
I have created a class based on a pandas.DataFrame
object which initializes with a csv
file as shown here:
import pandas as pd
class CustomDataFrame(pd.DataFrame):
def __init__(self, input_file):
df = pd.read_csv(input_file)
super().__init__(df)
#...
This way, I have a CustomDataFrame
type that has additional specific methods to operate on itself. The problem I have with this setup is that when I take a slice of the object, it returns a pandas.DataFrame
object instead of keeping the same type. In other words:
> blip = mypackage.CustomDataFrame('test.csv')
> type(blip)
mypackage.CustomDataFrame
> type(blip[1:3])
pandas.core.frame.DataFrame
Is there a simple way to correct my custom class such that it can operate on itself in all the ways that a pandas.DataFrame
can, while returning this custom class each time rather than just the built-in version of the DataFrame
?
python pandas oop dataframe inheritance
add a comment |
I have created a class based on a pandas.DataFrame
object which initializes with a csv
file as shown here:
import pandas as pd
class CustomDataFrame(pd.DataFrame):
def __init__(self, input_file):
df = pd.read_csv(input_file)
super().__init__(df)
#...
This way, I have a CustomDataFrame
type that has additional specific methods to operate on itself. The problem I have with this setup is that when I take a slice of the object, it returns a pandas.DataFrame
object instead of keeping the same type. In other words:
> blip = mypackage.CustomDataFrame('test.csv')
> type(blip)
mypackage.CustomDataFrame
> type(blip[1:3])
pandas.core.frame.DataFrame
Is there a simple way to correct my custom class such that it can operate on itself in all the ways that a pandas.DataFrame
can, while returning this custom class each time rather than just the built-in version of the DataFrame
?
python pandas oop dataframe inheritance
1
I have seen it said by one of the top 3 Pandas answerers that subclassing a DataFrame is a bad idea.
– roganjosh
Dec 31 '18 at 19:05
Oh really? That throws a wrench in my plans. Could you please link me to some of those answers? And do you know what a better alternative would be? Thanks.
– teepee
Dec 31 '18 at 19:30
1
I don't recall exactly what the other issue was, but I spend plenty of time looking at SO questions and this is only the second that I recall that was using subclassing tbh. It might be better to flesh out your plan with one of the custom methods that makes you think you need to subclass in the first place.Why can't you just have an object that applies methods to its own DF attribute?
– roganjosh
Dec 31 '18 at 19:55
1
stackoverflow.com/q/22155951/6361531
– Scott Boston
Jan 1 at 6:04
add a comment |
I have created a class based on a pandas.DataFrame
object which initializes with a csv
file as shown here:
import pandas as pd
class CustomDataFrame(pd.DataFrame):
def __init__(self, input_file):
df = pd.read_csv(input_file)
super().__init__(df)
#...
This way, I have a CustomDataFrame
type that has additional specific methods to operate on itself. The problem I have with this setup is that when I take a slice of the object, it returns a pandas.DataFrame
object instead of keeping the same type. In other words:
> blip = mypackage.CustomDataFrame('test.csv')
> type(blip)
mypackage.CustomDataFrame
> type(blip[1:3])
pandas.core.frame.DataFrame
Is there a simple way to correct my custom class such that it can operate on itself in all the ways that a pandas.DataFrame
can, while returning this custom class each time rather than just the built-in version of the DataFrame
?
python pandas oop dataframe inheritance
I have created a class based on a pandas.DataFrame
object which initializes with a csv
file as shown here:
import pandas as pd
class CustomDataFrame(pd.DataFrame):
def __init__(self, input_file):
df = pd.read_csv(input_file)
super().__init__(df)
#...
This way, I have a CustomDataFrame
type that has additional specific methods to operate on itself. The problem I have with this setup is that when I take a slice of the object, it returns a pandas.DataFrame
object instead of keeping the same type. In other words:
> blip = mypackage.CustomDataFrame('test.csv')
> type(blip)
mypackage.CustomDataFrame
> type(blip[1:3])
pandas.core.frame.DataFrame
Is there a simple way to correct my custom class such that it can operate on itself in all the ways that a pandas.DataFrame
can, while returning this custom class each time rather than just the built-in version of the DataFrame
?
python pandas oop dataframe inheritance
python pandas oop dataframe inheritance
asked Dec 31 '18 at 19:02
teepeeteepee
7122819
7122819
1
I have seen it said by one of the top 3 Pandas answerers that subclassing a DataFrame is a bad idea.
– roganjosh
Dec 31 '18 at 19:05
Oh really? That throws a wrench in my plans. Could you please link me to some of those answers? And do you know what a better alternative would be? Thanks.
– teepee
Dec 31 '18 at 19:30
1
I don't recall exactly what the other issue was, but I spend plenty of time looking at SO questions and this is only the second that I recall that was using subclassing tbh. It might be better to flesh out your plan with one of the custom methods that makes you think you need to subclass in the first place.Why can't you just have an object that applies methods to its own DF attribute?
– roganjosh
Dec 31 '18 at 19:55
1
stackoverflow.com/q/22155951/6361531
– Scott Boston
Jan 1 at 6:04
add a comment |
1
I have seen it said by one of the top 3 Pandas answerers that subclassing a DataFrame is a bad idea.
– roganjosh
Dec 31 '18 at 19:05
Oh really? That throws a wrench in my plans. Could you please link me to some of those answers? And do you know what a better alternative would be? Thanks.
– teepee
Dec 31 '18 at 19:30
1
I don't recall exactly what the other issue was, but I spend plenty of time looking at SO questions and this is only the second that I recall that was using subclassing tbh. It might be better to flesh out your plan with one of the custom methods that makes you think you need to subclass in the first place.Why can't you just have an object that applies methods to its own DF attribute?
– roganjosh
Dec 31 '18 at 19:55
1
stackoverflow.com/q/22155951/6361531
– Scott Boston
Jan 1 at 6:04
1
1
I have seen it said by one of the top 3 Pandas answerers that subclassing a DataFrame is a bad idea.
– roganjosh
Dec 31 '18 at 19:05
I have seen it said by one of the top 3 Pandas answerers that subclassing a DataFrame is a bad idea.
– roganjosh
Dec 31 '18 at 19:05
Oh really? That throws a wrench in my plans. Could you please link me to some of those answers? And do you know what a better alternative would be? Thanks.
– teepee
Dec 31 '18 at 19:30
Oh really? That throws a wrench in my plans. Could you please link me to some of those answers? And do you know what a better alternative would be? Thanks.
– teepee
Dec 31 '18 at 19:30
1
1
I don't recall exactly what the other issue was, but I spend plenty of time looking at SO questions and this is only the second that I recall that was using subclassing tbh. It might be better to flesh out your plan with one of the custom methods that makes you think you need to subclass in the first place.Why can't you just have an object that applies methods to its own DF attribute?
– roganjosh
Dec 31 '18 at 19:55
I don't recall exactly what the other issue was, but I spend plenty of time looking at SO questions and this is only the second that I recall that was using subclassing tbh. It might be better to flesh out your plan with one of the custom methods that makes you think you need to subclass in the first place.Why can't you just have an object that applies methods to its own DF attribute?
– roganjosh
Dec 31 '18 at 19:55
1
1
stackoverflow.com/q/22155951/6361531
– Scott Boston
Jan 1 at 6:04
stackoverflow.com/q/22155951/6361531
– Scott Boston
Jan 1 at 6:04
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990630%2fcustom-pandas-dataframe-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990630%2fcustom-pandas-dataframe-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WS jNbWh1xOv mNPcHtIbCNKm644U9
1
I have seen it said by one of the top 3 Pandas answerers that subclassing a DataFrame is a bad idea.
– roganjosh
Dec 31 '18 at 19:05
Oh really? That throws a wrench in my plans. Could you please link me to some of those answers? And do you know what a better alternative would be? Thanks.
– teepee
Dec 31 '18 at 19:30
1
I don't recall exactly what the other issue was, but I spend plenty of time looking at SO questions and this is only the second that I recall that was using subclassing tbh. It might be better to flesh out your plan with one of the custom methods that makes you think you need to subclass in the first place.Why can't you just have an object that applies methods to its own DF attribute?
– roganjosh
Dec 31 '18 at 19:55
1
stackoverflow.com/q/22155951/6361531
– Scott Boston
Jan 1 at 6:04