How to render list of hundreds of Images using FlatList and JSON data
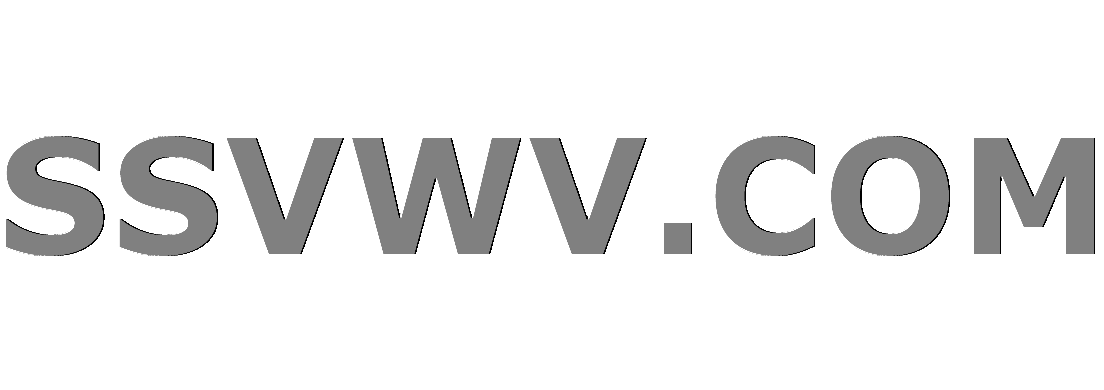
Multi tool use
Hi I would like to learn how I can map() through this JSON and grab the href of each image and place into a FlatList?
Currently the information I am trying to grab is collection.items.links[0].href and would like to place this href into the Image uri
The problem is I can grab one image easy and place it into a FlatList, but cannot work out how I can grab all of them and render a list of hundreds of images using the FlatList. This is because somehow I need to use map() at this point it seems:
collection.items map() .links[0].href
Thank you in advance!
Example of Json
{
"collection": {
"links": [
{
"prompt": "Next",
"rel": "next",
"href": "https://images-api.nasa.gov/search?media_type=image&q=moon&page=2"
}
],
"version": "1.0",
"metadata": {
"total_hits": 6726
},
"items": [
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12235/PIA12235~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12235",
"secondary_creator": "ISRO/NASA/JPL-Caltech/Brown Univ.",
"keywords": [
"Moon",
"Chandrayaan-1"
],
"date_created": "2009-09-24T18:00:22Z",
"media_type": "image",
"title": "Nearside of the Moon",
"description_508": "Nearside of the Moon",
"center": "JPL",
"description": "Nearside of the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12235/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA13517/PIA13517~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA13517",
"secondary_creator": "NASA/GSFC/Arizona State University",
"keywords": [
"Moon",
"Lunar Reconnaissance Orbiter LRO"
],
"date_created": "2010-09-10T22:24:40Z",
"media_type": "image",
"title": "Color of the Moon",
"description_508": "Color of the Moon",
"center": "JPL",
"description": "Color of the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA13517/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12233/PIA12233~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12233",
"secondary_creator": "NASA/JPL-Caltech",
"keywords": [
"Moon",
"Chandrayaan-1"
],
"date_created": "2009-09-24T18:00:20Z",
"media_type": "image",
"title": "Mapping the Moon, Point by Point",
"description_508": "Mapping the Moon, Point by Point",
"center": "JPL",
"description": "Mapping the Moon, Point by Point"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12233/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12228/PIA12228~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12228",
"secondary_creator": "NASA/JPL-Caltech/USGS",
"keywords": [
"Moon",
"Cassini-Huygens"
],
"date_created": "2009-09-24T18:00:15Z",
"media_type": "image",
"title": "Cassini Look at Water on the Moon",
"description_508": "Cassini Look at Water on the Moon",
"center": "JPL",
"description": "Cassini Look at Water on the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12228/collection.json"
},
Also here is my code:
export default class ThirdScreen extends React.Component {
state = {
search: "",
data: "",
}
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items[0].links[0].href
}))
}
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: this.state.data}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
render() {
const {data} = this.state
return (
<View style={{ flex: 1, alignItems: "center", justifyContent: "center" }}>
<FlatList
data={data}
renderItem={this.renderItem}
keyExtractor={(item, index) => index.toString()}
/>
</View>
);
}
}
json reactjs api react-native
add a comment |
Hi I would like to learn how I can map() through this JSON and grab the href of each image and place into a FlatList?
Currently the information I am trying to grab is collection.items.links[0].href and would like to place this href into the Image uri
The problem is I can grab one image easy and place it into a FlatList, but cannot work out how I can grab all of them and render a list of hundreds of images using the FlatList. This is because somehow I need to use map() at this point it seems:
collection.items map() .links[0].href
Thank you in advance!
Example of Json
{
"collection": {
"links": [
{
"prompt": "Next",
"rel": "next",
"href": "https://images-api.nasa.gov/search?media_type=image&q=moon&page=2"
}
],
"version": "1.0",
"metadata": {
"total_hits": 6726
},
"items": [
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12235/PIA12235~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12235",
"secondary_creator": "ISRO/NASA/JPL-Caltech/Brown Univ.",
"keywords": [
"Moon",
"Chandrayaan-1"
],
"date_created": "2009-09-24T18:00:22Z",
"media_type": "image",
"title": "Nearside of the Moon",
"description_508": "Nearside of the Moon",
"center": "JPL",
"description": "Nearside of the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12235/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA13517/PIA13517~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA13517",
"secondary_creator": "NASA/GSFC/Arizona State University",
"keywords": [
"Moon",
"Lunar Reconnaissance Orbiter LRO"
],
"date_created": "2010-09-10T22:24:40Z",
"media_type": "image",
"title": "Color of the Moon",
"description_508": "Color of the Moon",
"center": "JPL",
"description": "Color of the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA13517/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12233/PIA12233~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12233",
"secondary_creator": "NASA/JPL-Caltech",
"keywords": [
"Moon",
"Chandrayaan-1"
],
"date_created": "2009-09-24T18:00:20Z",
"media_type": "image",
"title": "Mapping the Moon, Point by Point",
"description_508": "Mapping the Moon, Point by Point",
"center": "JPL",
"description": "Mapping the Moon, Point by Point"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12233/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12228/PIA12228~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12228",
"secondary_creator": "NASA/JPL-Caltech/USGS",
"keywords": [
"Moon",
"Cassini-Huygens"
],
"date_created": "2009-09-24T18:00:15Z",
"media_type": "image",
"title": "Cassini Look at Water on the Moon",
"description_508": "Cassini Look at Water on the Moon",
"center": "JPL",
"description": "Cassini Look at Water on the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12228/collection.json"
},
Also here is my code:
export default class ThirdScreen extends React.Component {
state = {
search: "",
data: "",
}
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items[0].links[0].href
}))
}
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: this.state.data}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
render() {
const {data} = this.state
return (
<View style={{ flex: 1, alignItems: "center", justifyContent: "center" }}>
<FlatList
data={data}
renderItem={this.renderItem}
keyExtractor={(item, index) => index.toString()}
/>
</View>
);
}
}
json reactjs api react-native
due to memory and other resource constraints, not to mention time constraints, it's impossible to render an infinite list. Maybe you mean a list of unknown size?
– Dan Farrell
Dec 31 '18 at 19:11
Correct a list of unknown size :)
– SwiftyCON
Dec 31 '18 at 19:12
add a comment |
Hi I would like to learn how I can map() through this JSON and grab the href of each image and place into a FlatList?
Currently the information I am trying to grab is collection.items.links[0].href and would like to place this href into the Image uri
The problem is I can grab one image easy and place it into a FlatList, but cannot work out how I can grab all of them and render a list of hundreds of images using the FlatList. This is because somehow I need to use map() at this point it seems:
collection.items map() .links[0].href
Thank you in advance!
Example of Json
{
"collection": {
"links": [
{
"prompt": "Next",
"rel": "next",
"href": "https://images-api.nasa.gov/search?media_type=image&q=moon&page=2"
}
],
"version": "1.0",
"metadata": {
"total_hits": 6726
},
"items": [
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12235/PIA12235~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12235",
"secondary_creator": "ISRO/NASA/JPL-Caltech/Brown Univ.",
"keywords": [
"Moon",
"Chandrayaan-1"
],
"date_created": "2009-09-24T18:00:22Z",
"media_type": "image",
"title": "Nearside of the Moon",
"description_508": "Nearside of the Moon",
"center": "JPL",
"description": "Nearside of the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12235/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA13517/PIA13517~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA13517",
"secondary_creator": "NASA/GSFC/Arizona State University",
"keywords": [
"Moon",
"Lunar Reconnaissance Orbiter LRO"
],
"date_created": "2010-09-10T22:24:40Z",
"media_type": "image",
"title": "Color of the Moon",
"description_508": "Color of the Moon",
"center": "JPL",
"description": "Color of the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA13517/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12233/PIA12233~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12233",
"secondary_creator": "NASA/JPL-Caltech",
"keywords": [
"Moon",
"Chandrayaan-1"
],
"date_created": "2009-09-24T18:00:20Z",
"media_type": "image",
"title": "Mapping the Moon, Point by Point",
"description_508": "Mapping the Moon, Point by Point",
"center": "JPL",
"description": "Mapping the Moon, Point by Point"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12233/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12228/PIA12228~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12228",
"secondary_creator": "NASA/JPL-Caltech/USGS",
"keywords": [
"Moon",
"Cassini-Huygens"
],
"date_created": "2009-09-24T18:00:15Z",
"media_type": "image",
"title": "Cassini Look at Water on the Moon",
"description_508": "Cassini Look at Water on the Moon",
"center": "JPL",
"description": "Cassini Look at Water on the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12228/collection.json"
},
Also here is my code:
export default class ThirdScreen extends React.Component {
state = {
search: "",
data: "",
}
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items[0].links[0].href
}))
}
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: this.state.data}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
render() {
const {data} = this.state
return (
<View style={{ flex: 1, alignItems: "center", justifyContent: "center" }}>
<FlatList
data={data}
renderItem={this.renderItem}
keyExtractor={(item, index) => index.toString()}
/>
</View>
);
}
}
json reactjs api react-native
Hi I would like to learn how I can map() through this JSON and grab the href of each image and place into a FlatList?
Currently the information I am trying to grab is collection.items.links[0].href and would like to place this href into the Image uri
The problem is I can grab one image easy and place it into a FlatList, but cannot work out how I can grab all of them and render a list of hundreds of images using the FlatList. This is because somehow I need to use map() at this point it seems:
collection.items map() .links[0].href
Thank you in advance!
Example of Json
{
"collection": {
"links": [
{
"prompt": "Next",
"rel": "next",
"href": "https://images-api.nasa.gov/search?media_type=image&q=moon&page=2"
}
],
"version": "1.0",
"metadata": {
"total_hits": 6726
},
"items": [
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12235/PIA12235~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12235",
"secondary_creator": "ISRO/NASA/JPL-Caltech/Brown Univ.",
"keywords": [
"Moon",
"Chandrayaan-1"
],
"date_created": "2009-09-24T18:00:22Z",
"media_type": "image",
"title": "Nearside of the Moon",
"description_508": "Nearside of the Moon",
"center": "JPL",
"description": "Nearside of the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12235/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA13517/PIA13517~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA13517",
"secondary_creator": "NASA/GSFC/Arizona State University",
"keywords": [
"Moon",
"Lunar Reconnaissance Orbiter LRO"
],
"date_created": "2010-09-10T22:24:40Z",
"media_type": "image",
"title": "Color of the Moon",
"description_508": "Color of the Moon",
"center": "JPL",
"description": "Color of the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA13517/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12233/PIA12233~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12233",
"secondary_creator": "NASA/JPL-Caltech",
"keywords": [
"Moon",
"Chandrayaan-1"
],
"date_created": "2009-09-24T18:00:20Z",
"media_type": "image",
"title": "Mapping the Moon, Point by Point",
"description_508": "Mapping the Moon, Point by Point",
"center": "JPL",
"description": "Mapping the Moon, Point by Point"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12233/collection.json"
},
{
"links": [
{
"render": "image",
"rel": "preview",
"href": "https://images-assets.nasa.gov/image/PIA12228/PIA12228~thumb.jpg"
}
],
"data": [
{
"nasa_id": "PIA12228",
"secondary_creator": "NASA/JPL-Caltech/USGS",
"keywords": [
"Moon",
"Cassini-Huygens"
],
"date_created": "2009-09-24T18:00:15Z",
"media_type": "image",
"title": "Cassini Look at Water on the Moon",
"description_508": "Cassini Look at Water on the Moon",
"center": "JPL",
"description": "Cassini Look at Water on the Moon"
}
],
"href": "https://images-assets.nasa.gov/image/PIA12228/collection.json"
},
Also here is my code:
export default class ThirdScreen extends React.Component {
state = {
search: "",
data: "",
}
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items[0].links[0].href
}))
}
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: this.state.data}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
render() {
const {data} = this.state
return (
<View style={{ flex: 1, alignItems: "center", justifyContent: "center" }}>
<FlatList
data={data}
renderItem={this.renderItem}
keyExtractor={(item, index) => index.toString()}
/>
</View>
);
}
}
json reactjs api react-native
json reactjs api react-native
edited Dec 31 '18 at 19:13
SwiftyCON
asked Dec 31 '18 at 19:01
SwiftyCONSwiftyCON
327
327
due to memory and other resource constraints, not to mention time constraints, it's impossible to render an infinite list. Maybe you mean a list of unknown size?
– Dan Farrell
Dec 31 '18 at 19:11
Correct a list of unknown size :)
– SwiftyCON
Dec 31 '18 at 19:12
add a comment |
due to memory and other resource constraints, not to mention time constraints, it's impossible to render an infinite list. Maybe you mean a list of unknown size?
– Dan Farrell
Dec 31 '18 at 19:11
Correct a list of unknown size :)
– SwiftyCON
Dec 31 '18 at 19:12
due to memory and other resource constraints, not to mention time constraints, it's impossible to render an infinite list. Maybe you mean a list of unknown size?
– Dan Farrell
Dec 31 '18 at 19:11
due to memory and other resource constraints, not to mention time constraints, it's impossible to render an infinite list. Maybe you mean a list of unknown size?
– Dan Farrell
Dec 31 '18 at 19:11
Correct a list of unknown size :)
– SwiftyCON
Dec 31 '18 at 19:12
Correct a list of unknown size :)
– SwiftyCON
Dec 31 '18 at 19:12
add a comment |
1 Answer
1
active
oldest
votes
In JavaScript, Array.map() takes a function that takes each item of the original array and returns the new item that replaces it. You can use it to transform arrays from one structure to another. For example, you can transform the JSON data in your post from its original, complicated form to simply an array of strings of the HREFs:
const data = result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
});
Now plug something like that into your fetchNasa
function so that your data
variable in state is an array of strings representing HREFs:
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
})
}));
}
Finally, renderItem
should use the item passed to it as an argument in order to return what to render. In fact, renderItem
is essentially a version of an Array.map
function: it takes each item of the original data structure and returns some kind of React elements to render:
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: item}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
Because each item in your this.state.data
array is a string representing an HREF, you can pass that directly into your <Image>
source prop as the URI.
Really appreciate the long explanation and learnt a lot from it! Only one problem, I am now getting this error?
– SwiftyCON
Dec 31 '18 at 19:28
@SwiftyCON what error...?
– jered
Dec 31 '18 at 19:29
JSON Value '0' of type NSNumber cannot be converted to NSString
– SwiftyCON
Dec 31 '18 at 19:29
@SwiftyCON Does it indicate a line number? It probably has to do withreturn Array.isArray(eachItem) && eachItem[0] && eachItem[0].href
– jered
Dec 31 '18 at 20:58
@SwiftyCON Ah it's probably supposed to beArray.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
. I've edited my answer.
– jered
Dec 31 '18 at 21:01
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990628%2fhow-to-render-list-of-hundreds-of-images-using-flatlist-and-json-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In JavaScript, Array.map() takes a function that takes each item of the original array and returns the new item that replaces it. You can use it to transform arrays from one structure to another. For example, you can transform the JSON data in your post from its original, complicated form to simply an array of strings of the HREFs:
const data = result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
});
Now plug something like that into your fetchNasa
function so that your data
variable in state is an array of strings representing HREFs:
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
})
}));
}
Finally, renderItem
should use the item passed to it as an argument in order to return what to render. In fact, renderItem
is essentially a version of an Array.map
function: it takes each item of the original data structure and returns some kind of React elements to render:
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: item}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
Because each item in your this.state.data
array is a string representing an HREF, you can pass that directly into your <Image>
source prop as the URI.
Really appreciate the long explanation and learnt a lot from it! Only one problem, I am now getting this error?
– SwiftyCON
Dec 31 '18 at 19:28
@SwiftyCON what error...?
– jered
Dec 31 '18 at 19:29
JSON Value '0' of type NSNumber cannot be converted to NSString
– SwiftyCON
Dec 31 '18 at 19:29
@SwiftyCON Does it indicate a line number? It probably has to do withreturn Array.isArray(eachItem) && eachItem[0] && eachItem[0].href
– jered
Dec 31 '18 at 20:58
@SwiftyCON Ah it's probably supposed to beArray.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
. I've edited my answer.
– jered
Dec 31 '18 at 21:01
|
show 1 more comment
In JavaScript, Array.map() takes a function that takes each item of the original array and returns the new item that replaces it. You can use it to transform arrays from one structure to another. For example, you can transform the JSON data in your post from its original, complicated form to simply an array of strings of the HREFs:
const data = result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
});
Now plug something like that into your fetchNasa
function so that your data
variable in state is an array of strings representing HREFs:
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
})
}));
}
Finally, renderItem
should use the item passed to it as an argument in order to return what to render. In fact, renderItem
is essentially a version of an Array.map
function: it takes each item of the original data structure and returns some kind of React elements to render:
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: item}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
Because each item in your this.state.data
array is a string representing an HREF, you can pass that directly into your <Image>
source prop as the URI.
Really appreciate the long explanation and learnt a lot from it! Only one problem, I am now getting this error?
– SwiftyCON
Dec 31 '18 at 19:28
@SwiftyCON what error...?
– jered
Dec 31 '18 at 19:29
JSON Value '0' of type NSNumber cannot be converted to NSString
– SwiftyCON
Dec 31 '18 at 19:29
@SwiftyCON Does it indicate a line number? It probably has to do withreturn Array.isArray(eachItem) && eachItem[0] && eachItem[0].href
– jered
Dec 31 '18 at 20:58
@SwiftyCON Ah it's probably supposed to beArray.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
. I've edited my answer.
– jered
Dec 31 '18 at 21:01
|
show 1 more comment
In JavaScript, Array.map() takes a function that takes each item of the original array and returns the new item that replaces it. You can use it to transform arrays from one structure to another. For example, you can transform the JSON data in your post from its original, complicated form to simply an array of strings of the HREFs:
const data = result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
});
Now plug something like that into your fetchNasa
function so that your data
variable in state is an array of strings representing HREFs:
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
})
}));
}
Finally, renderItem
should use the item passed to it as an argument in order to return what to render. In fact, renderItem
is essentially a version of an Array.map
function: it takes each item of the original data structure and returns some kind of React elements to render:
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: item}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
Because each item in your this.state.data
array is a string representing an HREF, you can pass that directly into your <Image>
source prop as the URI.
In JavaScript, Array.map() takes a function that takes each item of the original array and returns the new item that replaces it. You can use it to transform arrays from one structure to another. For example, you can transform the JSON data in your post from its original, complicated form to simply an array of strings of the HREFs:
const data = result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
});
Now plug something like that into your fetchNasa
function so that your data
variable in state is an array of strings representing HREFs:
fetchNasa = () => {
const {search} = this.state;
fetch(`https://images-api.nasa.gov/search?q=${search}&media_type=image`)
.then((response) => response.json())
.then((result) => this.setState({
data: result.collection.items.map(eachItem => {
return Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
})
}));
}
Finally, renderItem
should use the item passed to it as an argument in order to return what to render. In fact, renderItem
is essentially a version of an Array.map
function: it takes each item of the original data structure and returns some kind of React elements to render:
renderItem = ({item}) => {
return (
<View>
<TouchableOpacity
style={{margin: 5, backgroundColor: 'black', padding: 15}}
>
<Image
source={{uri: item}}
style={{width: 60, height: 60}}
/>
</TouchableOpacity>
</View>
)
}
Because each item in your this.state.data
array is a string representing an HREF, you can pass that directly into your <Image>
source prop as the URI.
edited Dec 31 '18 at 21:50
answered Dec 31 '18 at 19:23


jeredjered
5,2642827
5,2642827
Really appreciate the long explanation and learnt a lot from it! Only one problem, I am now getting this error?
– SwiftyCON
Dec 31 '18 at 19:28
@SwiftyCON what error...?
– jered
Dec 31 '18 at 19:29
JSON Value '0' of type NSNumber cannot be converted to NSString
– SwiftyCON
Dec 31 '18 at 19:29
@SwiftyCON Does it indicate a line number? It probably has to do withreturn Array.isArray(eachItem) && eachItem[0] && eachItem[0].href
– jered
Dec 31 '18 at 20:58
@SwiftyCON Ah it's probably supposed to beArray.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
. I've edited my answer.
– jered
Dec 31 '18 at 21:01
|
show 1 more comment
Really appreciate the long explanation and learnt a lot from it! Only one problem, I am now getting this error?
– SwiftyCON
Dec 31 '18 at 19:28
@SwiftyCON what error...?
– jered
Dec 31 '18 at 19:29
JSON Value '0' of type NSNumber cannot be converted to NSString
– SwiftyCON
Dec 31 '18 at 19:29
@SwiftyCON Does it indicate a line number? It probably has to do withreturn Array.isArray(eachItem) && eachItem[0] && eachItem[0].href
– jered
Dec 31 '18 at 20:58
@SwiftyCON Ah it's probably supposed to beArray.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
. I've edited my answer.
– jered
Dec 31 '18 at 21:01
Really appreciate the long explanation and learnt a lot from it! Only one problem, I am now getting this error?
– SwiftyCON
Dec 31 '18 at 19:28
Really appreciate the long explanation and learnt a lot from it! Only one problem, I am now getting this error?
– SwiftyCON
Dec 31 '18 at 19:28
@SwiftyCON what error...?
– jered
Dec 31 '18 at 19:29
@SwiftyCON what error...?
– jered
Dec 31 '18 at 19:29
JSON Value '0' of type NSNumber cannot be converted to NSString
– SwiftyCON
Dec 31 '18 at 19:29
JSON Value '0' of type NSNumber cannot be converted to NSString
– SwiftyCON
Dec 31 '18 at 19:29
@SwiftyCON Does it indicate a line number? It probably has to do with
return Array.isArray(eachItem) && eachItem[0] && eachItem[0].href
– jered
Dec 31 '18 at 20:58
@SwiftyCON Does it indicate a line number? It probably has to do with
return Array.isArray(eachItem) && eachItem[0] && eachItem[0].href
– jered
Dec 31 '18 at 20:58
@SwiftyCON Ah it's probably supposed to be
Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
. I've edited my answer.– jered
Dec 31 '18 at 21:01
@SwiftyCON Ah it's probably supposed to be
Array.isArray(eachItem.links) && eachItem.links[0] && eachItem.links[0].href
. I've edited my answer.– jered
Dec 31 '18 at 21:01
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53990628%2fhow-to-render-list-of-hundreds-of-images-using-flatlist-and-json-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
evlOLWIvJc9E5,54qG6nsRGlYc 6zPXA2vhqT,qTyfT3O,JO0iOFLixXW609Ww xI,VUF45xpPujWsjbq
due to memory and other resource constraints, not to mention time constraints, it's impossible to render an infinite list. Maybe you mean a list of unknown size?
– Dan Farrell
Dec 31 '18 at 19:11
Correct a list of unknown size :)
– SwiftyCON
Dec 31 '18 at 19:12