URLConnection Doesn't Follow Redirect
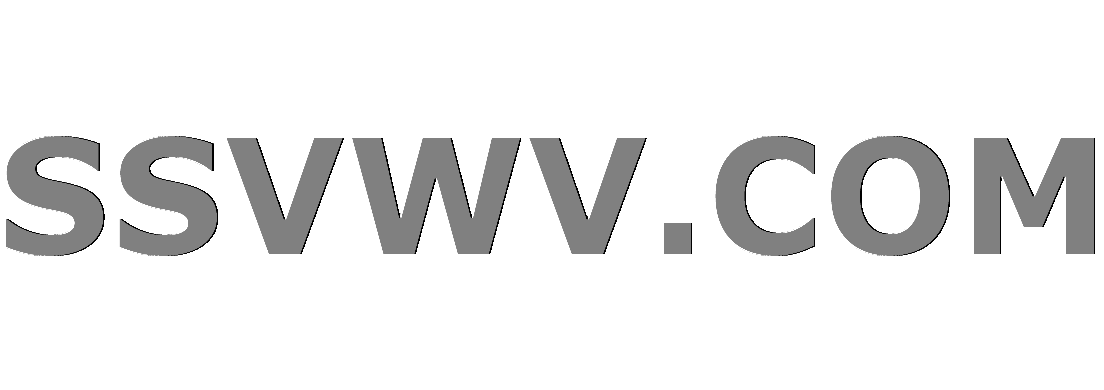
Multi tool use
I can't understand why Java's HttpURLConnection
doesn't follow redirect. I use the following code to get this page:
import java.net.URL;
import java.net.HttpURLConnection;
import java.io.InputStream;
public class Tester {
public static void main(String argv) throws Exception{
InputStream is = null;
try {
String bitlyUrl = "http://bit.ly/4hW294";
URL resourceUrl = new URL(bitlyUrl);
HttpURLConnection conn = (HttpURLConnection)resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows; U; Windows NT 6.0; ru; rv:1.9.0.11) Gecko/2009060215 Firefox/3.0.11 (.NET CLR 3.5.30729)");
conn.connect();
is = conn.getInputStream();
String res = conn.getURL().toString();
if (res.toLowerCase().contains("bit.ly"))
System.out.println("bit.ly is after resolving: "+res);
}
catch (Exception e) {
System.out.println("error happened: "+e.toString());
}
finally {
if (is != null) is.close();
}
}
}
Moreover, I get the following response (it seems absolutely right!):
GET /4hW294 HTTP/1.1
Host: bit.ly
Connection: Keep-Alive
User-Agent: Mozilla/5.0 (Windows; U; Windows NT 6.0; ru-RU; rv:1.9.1.3) Gecko/20090824 Firefox/3.5.3 (.NET CLR 3.5.30729)
HTTP/1.1 301 Moved
Server: nginx/0.7.42
Date: Thu, 10 Dec 2009 20:28:44 GMT
Content-Type: text/html; charset=utf-8
Connection: keep-alive
Location: https://www.myganocafe.com/CafeMacy
MIME-Version: 1.0
Content-Length: 297
Unfortunately, the res
variable contains the same URL and stream contains the following (obviously, Java's HttpURLConnection
doesn't follow redirect!):
<!DOCTYPE HTML PUBLIC "-//IETF//DTD HTML 2.0//EN">
<HTML>
<HEAD>
<TITLE>Moved</TITLE>
</HEAD>
<BODY>
<H2>Moved</H2>
<A HREF="https://www.myganocafe.com/CafeMacy">The requested URL has moved here.</A>
<P ALIGN=RIGHT><SMALL><I>AOLserver/4.5.1 on http://127.0.0.1:7400</I></SMALL></P>
</BODY>
</HTML>
java httpurlconnection
add a comment |
I can't understand why Java's HttpURLConnection
doesn't follow redirect. I use the following code to get this page:
import java.net.URL;
import java.net.HttpURLConnection;
import java.io.InputStream;
public class Tester {
public static void main(String argv) throws Exception{
InputStream is = null;
try {
String bitlyUrl = "http://bit.ly/4hW294";
URL resourceUrl = new URL(bitlyUrl);
HttpURLConnection conn = (HttpURLConnection)resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows; U; Windows NT 6.0; ru; rv:1.9.0.11) Gecko/2009060215 Firefox/3.0.11 (.NET CLR 3.5.30729)");
conn.connect();
is = conn.getInputStream();
String res = conn.getURL().toString();
if (res.toLowerCase().contains("bit.ly"))
System.out.println("bit.ly is after resolving: "+res);
}
catch (Exception e) {
System.out.println("error happened: "+e.toString());
}
finally {
if (is != null) is.close();
}
}
}
Moreover, I get the following response (it seems absolutely right!):
GET /4hW294 HTTP/1.1
Host: bit.ly
Connection: Keep-Alive
User-Agent: Mozilla/5.0 (Windows; U; Windows NT 6.0; ru-RU; rv:1.9.1.3) Gecko/20090824 Firefox/3.5.3 (.NET CLR 3.5.30729)
HTTP/1.1 301 Moved
Server: nginx/0.7.42
Date: Thu, 10 Dec 2009 20:28:44 GMT
Content-Type: text/html; charset=utf-8
Connection: keep-alive
Location: https://www.myganocafe.com/CafeMacy
MIME-Version: 1.0
Content-Length: 297
Unfortunately, the res
variable contains the same URL and stream contains the following (obviously, Java's HttpURLConnection
doesn't follow redirect!):
<!DOCTYPE HTML PUBLIC "-//IETF//DTD HTML 2.0//EN">
<HTML>
<HEAD>
<TITLE>Moved</TITLE>
</HEAD>
<BODY>
<H2>Moved</H2>
<A HREF="https://www.myganocafe.com/CafeMacy">The requested URL has moved here.</A>
<P ALIGN=RIGHT><SMALL><I>AOLserver/4.5.1 on http://127.0.0.1:7400</I></SMALL></P>
</BODY>
</HTML>
java httpurlconnection
add a comment |
I can't understand why Java's HttpURLConnection
doesn't follow redirect. I use the following code to get this page:
import java.net.URL;
import java.net.HttpURLConnection;
import java.io.InputStream;
public class Tester {
public static void main(String argv) throws Exception{
InputStream is = null;
try {
String bitlyUrl = "http://bit.ly/4hW294";
URL resourceUrl = new URL(bitlyUrl);
HttpURLConnection conn = (HttpURLConnection)resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows; U; Windows NT 6.0; ru; rv:1.9.0.11) Gecko/2009060215 Firefox/3.0.11 (.NET CLR 3.5.30729)");
conn.connect();
is = conn.getInputStream();
String res = conn.getURL().toString();
if (res.toLowerCase().contains("bit.ly"))
System.out.println("bit.ly is after resolving: "+res);
}
catch (Exception e) {
System.out.println("error happened: "+e.toString());
}
finally {
if (is != null) is.close();
}
}
}
Moreover, I get the following response (it seems absolutely right!):
GET /4hW294 HTTP/1.1
Host: bit.ly
Connection: Keep-Alive
User-Agent: Mozilla/5.0 (Windows; U; Windows NT 6.0; ru-RU; rv:1.9.1.3) Gecko/20090824 Firefox/3.5.3 (.NET CLR 3.5.30729)
HTTP/1.1 301 Moved
Server: nginx/0.7.42
Date: Thu, 10 Dec 2009 20:28:44 GMT
Content-Type: text/html; charset=utf-8
Connection: keep-alive
Location: https://www.myganocafe.com/CafeMacy
MIME-Version: 1.0
Content-Length: 297
Unfortunately, the res
variable contains the same URL and stream contains the following (obviously, Java's HttpURLConnection
doesn't follow redirect!):
<!DOCTYPE HTML PUBLIC "-//IETF//DTD HTML 2.0//EN">
<HTML>
<HEAD>
<TITLE>Moved</TITLE>
</HEAD>
<BODY>
<H2>Moved</H2>
<A HREF="https://www.myganocafe.com/CafeMacy">The requested URL has moved here.</A>
<P ALIGN=RIGHT><SMALL><I>AOLserver/4.5.1 on http://127.0.0.1:7400</I></SMALL></P>
</BODY>
</HTML>
java httpurlconnection
I can't understand why Java's HttpURLConnection
doesn't follow redirect. I use the following code to get this page:
import java.net.URL;
import java.net.HttpURLConnection;
import java.io.InputStream;
public class Tester {
public static void main(String argv) throws Exception{
InputStream is = null;
try {
String bitlyUrl = "http://bit.ly/4hW294";
URL resourceUrl = new URL(bitlyUrl);
HttpURLConnection conn = (HttpURLConnection)resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows; U; Windows NT 6.0; ru; rv:1.9.0.11) Gecko/2009060215 Firefox/3.0.11 (.NET CLR 3.5.30729)");
conn.connect();
is = conn.getInputStream();
String res = conn.getURL().toString();
if (res.toLowerCase().contains("bit.ly"))
System.out.println("bit.ly is after resolving: "+res);
}
catch (Exception e) {
System.out.println("error happened: "+e.toString());
}
finally {
if (is != null) is.close();
}
}
}
Moreover, I get the following response (it seems absolutely right!):
GET /4hW294 HTTP/1.1
Host: bit.ly
Connection: Keep-Alive
User-Agent: Mozilla/5.0 (Windows; U; Windows NT 6.0; ru-RU; rv:1.9.1.3) Gecko/20090824 Firefox/3.5.3 (.NET CLR 3.5.30729)
HTTP/1.1 301 Moved
Server: nginx/0.7.42
Date: Thu, 10 Dec 2009 20:28:44 GMT
Content-Type: text/html; charset=utf-8
Connection: keep-alive
Location: https://www.myganocafe.com/CafeMacy
MIME-Version: 1.0
Content-Length: 297
Unfortunately, the res
variable contains the same URL and stream contains the following (obviously, Java's HttpURLConnection
doesn't follow redirect!):
<!DOCTYPE HTML PUBLIC "-//IETF//DTD HTML 2.0//EN">
<HTML>
<HEAD>
<TITLE>Moved</TITLE>
</HEAD>
<BODY>
<H2>Moved</H2>
<A HREF="https://www.myganocafe.com/CafeMacy">The requested URL has moved here.</A>
<P ALIGN=RIGHT><SMALL><I>AOLserver/4.5.1 on http://127.0.0.1:7400</I></SMALL></P>
</BODY>
</HTML>
java httpurlconnection
java httpurlconnection
edited Jan 21 '16 at 22:39
Nathan
3,47552852
3,47552852
asked Dec 10 '09 at 21:38
ShchekleinShcheklein
2,54553243
2,54553243
add a comment |
add a comment |
6 Answers
6
active
oldest
votes
I don't think that it will automatically redirect from HTTP to HTTPS (or vice-versa).
Even though we know it mirrors HTTP, from the HTTP protocol point of view, HTTPS is just some other, completely different, unknown protocol. It would be unsafe to follow the redirect without user approval.
For example, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
58
Thanks. I've just found confiramtion: bugs.sun.com/bugdatabase/view_bug.do?bug_id=4620571 . Namely: "After discussion among Java Networking engineers, it is felt that we shouldn't automatically follow redirect from one protocol to another, for instance, from http to https and vise versa, doing so may have serious security consequences. Thus the fix is to return the server responses for redirect. Check response code and Location header field value for redirect information. It's the application's responsibility to follow the redirect."
– Shcheklein
Dec 10 '09 at 22:41
2
But does it follow redirect from http to http or https to https? Even that would be wrong. Isn't it?
– Sudarshan Bhat
Oct 31 '12 at 6:15
4
@JoshuaDavis Yes, it only applies to redirects to the same protocol. AnHttpURLConnection
won't automatically follow redirects to a different protocol, even if the redirect flag is set.
– erickson
Feb 4 '13 at 18:33
6
Java Networking engineers could offer a setFollowTransProtocol(true) option because if we need it we will program it anyway. FYI web browsers, curl and wget and may more follow redirects from HTTP to HTTPS and vice-versa.
– supercobra
Oct 22 '14 at 2:31
12
Nobody sets up auto-login on HTTPS and then expects HTTP to be "anonymous". That's nonsensical. It's perfectly safe and normal to follow redirects from HTTP to HTTPS (not the other way around). This is just a typically bad Java API.
– Glenn Maynard
Mar 27 '16 at 6:34
|
show 3 more comments
HttpURLConnection by design won't automatically redirect from HTTP to HTTPS (or vice versa). Following the redirect may have serious security consequences. SSL (hence HTTPS) creates a session that is unique to the user. This session can be reused for multiple requests. Thus, the server can track all of the requests made from a single person. This is a weak form of identity and is exploitable. Also, the SSL handshake can ask for the client's certificate. If sent to the server, then the client's identity is given to the server.
As erickson points out, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
With that understood, here's the code which will follow the redirects.
URL resourceUrl, base, next;
HttpURLConnection conn;
String location;
...
while (true)
{
resourceUrl = new URL(url);
conn = (HttpURLConnection) resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setInstanceFollowRedirects(false); // Make the logic below easier to detect redirections
conn.setRequestProperty("User-Agent", "Mozilla/5.0...");
switch (conn.getResponseCode())
{
case HttpURLConnection.HTTP_MOVED_PERM:
case HttpURLConnection.HTTP_MOVED_TEMP:
location = conn.getHeaderField("Location");
location = URLDecoder.decode(location, "UTF-8");
base = new URL(url);
next = new URL(base, location); // Deal with relative URLs
url = next.toExternalForm();
continue;
}
break;
}
is = conn.openStream();
...
This is only one solution that works for more than 1 redirects. Thank you!
– Roger Alien
Jul 31 '16 at 3:37
This works beautifully for multiple redirects (HTTPS API -> HTTP -> HTTP image)! Perfect simple solution.
– EricH206
Jan 6 '17 at 11:50
Just make you you limit the number of redirects otherwise you may get into an infinite loop
– Archimedes Trajano
Apr 15 '17 at 3:20
I have no idea how changing the URI scheme from "http" to "https" has any consequences on security. Why would that be worse to changing to another origin on "http"?
– Julian Reschke
Nov 30 '17 at 12:16
@Nathan - thanks for the details, but I still don't buy it. For instance, if's under the control of the client whether any credentials or client certs are sent. If it hurts, don't do it (in this case, do not follow the redirect).
– Julian Reschke
Dec 1 '17 at 5:30
|
show 3 more comments
Has something called HttpURLConnection.setFollowRedirects(false)
by any chance?
You could always call
conn.setInstanceFollowRedirects(true);
if you want to make sure you don't affect the rest of the behaviour of the app.
Ooo... didn't know about that... Nice find... I was about to look up the class incase there was logic like that.... It makes sense that it would be returning that header giving the single responsibility principal.... now go back to answering C# questions :P [I'm kidding]
– monksy
Dec 10 '09 at 21:47
2
Note that setFollowRedirects() should be called on the class, and not on an instance.
– karlbecker_com
Apr 4 '13 at 21:50
2
@dldnh: While karlbecker_com was absolutely right about callingsetFollowRedirects
on the type,setInstanceFollowRedirects
is an instance method and can't be called on the type.
– Jon Skeet
Apr 13 '13 at 6:43
1
uggh, how did I misread that. sorry about the incorrect edit. also tried to rollback and not sure how I bollocksed that as well.
– dldnh
Apr 13 '13 at 10:43
This does not help with https redirect I think..
– Koray Tugay
Jul 6 '18 at 16:18
add a comment |
As mentioned by some of you above, the setFollowRedirect and setInstanceFollowRedirects only work automatically when the redirected protocol is same . ie from http to http and https to https.
setFolloRedirect is at class level and sets this for all instances of the url connection, whereas setInstanceFollowRedirects is only for a given instance. This way we can have different behavior for different instances.
I found a very good example here http://www.mkyong.com/java/java-httpurlconnection-follow-redirect-example/
add a comment |
Another option can be to use Apache HttpComponents Client:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
Sample code:
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpGet httpget = new HttpGet("https://media-hearth.cursecdn.com/avatars/330/498/212.png");
CloseableHttpResponse response = httpclient.execute(httpget);
final HttpEntity entity = response.getEntity();
final InputStream is = entity.getContent();
add a comment |
HTTPUrlConnection is not responsible for handling the response of the object. It is performance as expected, it grabs the content of the URL requested. It is up to you the user of the functionality to interpret the response. It is not able to read the intentions of the developer without specification.
7
Why it has setInstanceFollowRedirects in this case? ))
– Shcheklein
Dec 10 '09 at 21:46
My guess is that it was a suggested feature to add in later, it makes sense.. my comment was more of reflected toward... the class is designed to go and grab web content and bring it back... people may want to get non HTTP 200 messages.
– monksy
Dec 10 '09 at 21:49
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f1884230%2furlconnection-doesnt-follow-redirect%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
I don't think that it will automatically redirect from HTTP to HTTPS (or vice-versa).
Even though we know it mirrors HTTP, from the HTTP protocol point of view, HTTPS is just some other, completely different, unknown protocol. It would be unsafe to follow the redirect without user approval.
For example, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
58
Thanks. I've just found confiramtion: bugs.sun.com/bugdatabase/view_bug.do?bug_id=4620571 . Namely: "After discussion among Java Networking engineers, it is felt that we shouldn't automatically follow redirect from one protocol to another, for instance, from http to https and vise versa, doing so may have serious security consequences. Thus the fix is to return the server responses for redirect. Check response code and Location header field value for redirect information. It's the application's responsibility to follow the redirect."
– Shcheklein
Dec 10 '09 at 22:41
2
But does it follow redirect from http to http or https to https? Even that would be wrong. Isn't it?
– Sudarshan Bhat
Oct 31 '12 at 6:15
4
@JoshuaDavis Yes, it only applies to redirects to the same protocol. AnHttpURLConnection
won't automatically follow redirects to a different protocol, even if the redirect flag is set.
– erickson
Feb 4 '13 at 18:33
6
Java Networking engineers could offer a setFollowTransProtocol(true) option because if we need it we will program it anyway. FYI web browsers, curl and wget and may more follow redirects from HTTP to HTTPS and vice-versa.
– supercobra
Oct 22 '14 at 2:31
12
Nobody sets up auto-login on HTTPS and then expects HTTP to be "anonymous". That's nonsensical. It's perfectly safe and normal to follow redirects from HTTP to HTTPS (not the other way around). This is just a typically bad Java API.
– Glenn Maynard
Mar 27 '16 at 6:34
|
show 3 more comments
I don't think that it will automatically redirect from HTTP to HTTPS (or vice-versa).
Even though we know it mirrors HTTP, from the HTTP protocol point of view, HTTPS is just some other, completely different, unknown protocol. It would be unsafe to follow the redirect without user approval.
For example, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
58
Thanks. I've just found confiramtion: bugs.sun.com/bugdatabase/view_bug.do?bug_id=4620571 . Namely: "After discussion among Java Networking engineers, it is felt that we shouldn't automatically follow redirect from one protocol to another, for instance, from http to https and vise versa, doing so may have serious security consequences. Thus the fix is to return the server responses for redirect. Check response code and Location header field value for redirect information. It's the application's responsibility to follow the redirect."
– Shcheklein
Dec 10 '09 at 22:41
2
But does it follow redirect from http to http or https to https? Even that would be wrong. Isn't it?
– Sudarshan Bhat
Oct 31 '12 at 6:15
4
@JoshuaDavis Yes, it only applies to redirects to the same protocol. AnHttpURLConnection
won't automatically follow redirects to a different protocol, even if the redirect flag is set.
– erickson
Feb 4 '13 at 18:33
6
Java Networking engineers could offer a setFollowTransProtocol(true) option because if we need it we will program it anyway. FYI web browsers, curl and wget and may more follow redirects from HTTP to HTTPS and vice-versa.
– supercobra
Oct 22 '14 at 2:31
12
Nobody sets up auto-login on HTTPS and then expects HTTP to be "anonymous". That's nonsensical. It's perfectly safe and normal to follow redirects from HTTP to HTTPS (not the other way around). This is just a typically bad Java API.
– Glenn Maynard
Mar 27 '16 at 6:34
|
show 3 more comments
I don't think that it will automatically redirect from HTTP to HTTPS (or vice-versa).
Even though we know it mirrors HTTP, from the HTTP protocol point of view, HTTPS is just some other, completely different, unknown protocol. It would be unsafe to follow the redirect without user approval.
For example, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
I don't think that it will automatically redirect from HTTP to HTTPS (or vice-versa).
Even though we know it mirrors HTTP, from the HTTP protocol point of view, HTTPS is just some other, completely different, unknown protocol. It would be unsafe to follow the redirect without user approval.
For example, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
answered Dec 10 '09 at 22:05
ericksonerickson
222k42331428
222k42331428
58
Thanks. I've just found confiramtion: bugs.sun.com/bugdatabase/view_bug.do?bug_id=4620571 . Namely: "After discussion among Java Networking engineers, it is felt that we shouldn't automatically follow redirect from one protocol to another, for instance, from http to https and vise versa, doing so may have serious security consequences. Thus the fix is to return the server responses for redirect. Check response code and Location header field value for redirect information. It's the application's responsibility to follow the redirect."
– Shcheklein
Dec 10 '09 at 22:41
2
But does it follow redirect from http to http or https to https? Even that would be wrong. Isn't it?
– Sudarshan Bhat
Oct 31 '12 at 6:15
4
@JoshuaDavis Yes, it only applies to redirects to the same protocol. AnHttpURLConnection
won't automatically follow redirects to a different protocol, even if the redirect flag is set.
– erickson
Feb 4 '13 at 18:33
6
Java Networking engineers could offer a setFollowTransProtocol(true) option because if we need it we will program it anyway. FYI web browsers, curl and wget and may more follow redirects from HTTP to HTTPS and vice-versa.
– supercobra
Oct 22 '14 at 2:31
12
Nobody sets up auto-login on HTTPS and then expects HTTP to be "anonymous". That's nonsensical. It's perfectly safe and normal to follow redirects from HTTP to HTTPS (not the other way around). This is just a typically bad Java API.
– Glenn Maynard
Mar 27 '16 at 6:34
|
show 3 more comments
58
Thanks. I've just found confiramtion: bugs.sun.com/bugdatabase/view_bug.do?bug_id=4620571 . Namely: "After discussion among Java Networking engineers, it is felt that we shouldn't automatically follow redirect from one protocol to another, for instance, from http to https and vise versa, doing so may have serious security consequences. Thus the fix is to return the server responses for redirect. Check response code and Location header field value for redirect information. It's the application's responsibility to follow the redirect."
– Shcheklein
Dec 10 '09 at 22:41
2
But does it follow redirect from http to http or https to https? Even that would be wrong. Isn't it?
– Sudarshan Bhat
Oct 31 '12 at 6:15
4
@JoshuaDavis Yes, it only applies to redirects to the same protocol. AnHttpURLConnection
won't automatically follow redirects to a different protocol, even if the redirect flag is set.
– erickson
Feb 4 '13 at 18:33
6
Java Networking engineers could offer a setFollowTransProtocol(true) option because if we need it we will program it anyway. FYI web browsers, curl and wget and may more follow redirects from HTTP to HTTPS and vice-versa.
– supercobra
Oct 22 '14 at 2:31
12
Nobody sets up auto-login on HTTPS and then expects HTTP to be "anonymous". That's nonsensical. It's perfectly safe and normal to follow redirects from HTTP to HTTPS (not the other way around). This is just a typically bad Java API.
– Glenn Maynard
Mar 27 '16 at 6:34
58
58
Thanks. I've just found confiramtion: bugs.sun.com/bugdatabase/view_bug.do?bug_id=4620571 . Namely: "After discussion among Java Networking engineers, it is felt that we shouldn't automatically follow redirect from one protocol to another, for instance, from http to https and vise versa, doing so may have serious security consequences. Thus the fix is to return the server responses for redirect. Check response code and Location header field value for redirect information. It's the application's responsibility to follow the redirect."
– Shcheklein
Dec 10 '09 at 22:41
Thanks. I've just found confiramtion: bugs.sun.com/bugdatabase/view_bug.do?bug_id=4620571 . Namely: "After discussion among Java Networking engineers, it is felt that we shouldn't automatically follow redirect from one protocol to another, for instance, from http to https and vise versa, doing so may have serious security consequences. Thus the fix is to return the server responses for redirect. Check response code and Location header field value for redirect information. It's the application's responsibility to follow the redirect."
– Shcheklein
Dec 10 '09 at 22:41
2
2
But does it follow redirect from http to http or https to https? Even that would be wrong. Isn't it?
– Sudarshan Bhat
Oct 31 '12 at 6:15
But does it follow redirect from http to http or https to https? Even that would be wrong. Isn't it?
– Sudarshan Bhat
Oct 31 '12 at 6:15
4
4
@JoshuaDavis Yes, it only applies to redirects to the same protocol. An
HttpURLConnection
won't automatically follow redirects to a different protocol, even if the redirect flag is set.– erickson
Feb 4 '13 at 18:33
@JoshuaDavis Yes, it only applies to redirects to the same protocol. An
HttpURLConnection
won't automatically follow redirects to a different protocol, even if the redirect flag is set.– erickson
Feb 4 '13 at 18:33
6
6
Java Networking engineers could offer a setFollowTransProtocol(true) option because if we need it we will program it anyway. FYI web browsers, curl and wget and may more follow redirects from HTTP to HTTPS and vice-versa.
– supercobra
Oct 22 '14 at 2:31
Java Networking engineers could offer a setFollowTransProtocol(true) option because if we need it we will program it anyway. FYI web browsers, curl and wget and may more follow redirects from HTTP to HTTPS and vice-versa.
– supercobra
Oct 22 '14 at 2:31
12
12
Nobody sets up auto-login on HTTPS and then expects HTTP to be "anonymous". That's nonsensical. It's perfectly safe and normal to follow redirects from HTTP to HTTPS (not the other way around). This is just a typically bad Java API.
– Glenn Maynard
Mar 27 '16 at 6:34
Nobody sets up auto-login on HTTPS and then expects HTTP to be "anonymous". That's nonsensical. It's perfectly safe and normal to follow redirects from HTTP to HTTPS (not the other way around). This is just a typically bad Java API.
– Glenn Maynard
Mar 27 '16 at 6:34
|
show 3 more comments
HttpURLConnection by design won't automatically redirect from HTTP to HTTPS (or vice versa). Following the redirect may have serious security consequences. SSL (hence HTTPS) creates a session that is unique to the user. This session can be reused for multiple requests. Thus, the server can track all of the requests made from a single person. This is a weak form of identity and is exploitable. Also, the SSL handshake can ask for the client's certificate. If sent to the server, then the client's identity is given to the server.
As erickson points out, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
With that understood, here's the code which will follow the redirects.
URL resourceUrl, base, next;
HttpURLConnection conn;
String location;
...
while (true)
{
resourceUrl = new URL(url);
conn = (HttpURLConnection) resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setInstanceFollowRedirects(false); // Make the logic below easier to detect redirections
conn.setRequestProperty("User-Agent", "Mozilla/5.0...");
switch (conn.getResponseCode())
{
case HttpURLConnection.HTTP_MOVED_PERM:
case HttpURLConnection.HTTP_MOVED_TEMP:
location = conn.getHeaderField("Location");
location = URLDecoder.decode(location, "UTF-8");
base = new URL(url);
next = new URL(base, location); // Deal with relative URLs
url = next.toExternalForm();
continue;
}
break;
}
is = conn.openStream();
...
This is only one solution that works for more than 1 redirects. Thank you!
– Roger Alien
Jul 31 '16 at 3:37
This works beautifully for multiple redirects (HTTPS API -> HTTP -> HTTP image)! Perfect simple solution.
– EricH206
Jan 6 '17 at 11:50
Just make you you limit the number of redirects otherwise you may get into an infinite loop
– Archimedes Trajano
Apr 15 '17 at 3:20
I have no idea how changing the URI scheme from "http" to "https" has any consequences on security. Why would that be worse to changing to another origin on "http"?
– Julian Reschke
Nov 30 '17 at 12:16
@Nathan - thanks for the details, but I still don't buy it. For instance, if's under the control of the client whether any credentials or client certs are sent. If it hurts, don't do it (in this case, do not follow the redirect).
– Julian Reschke
Dec 1 '17 at 5:30
|
show 3 more comments
HttpURLConnection by design won't automatically redirect from HTTP to HTTPS (or vice versa). Following the redirect may have serious security consequences. SSL (hence HTTPS) creates a session that is unique to the user. This session can be reused for multiple requests. Thus, the server can track all of the requests made from a single person. This is a weak form of identity and is exploitable. Also, the SSL handshake can ask for the client's certificate. If sent to the server, then the client's identity is given to the server.
As erickson points out, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
With that understood, here's the code which will follow the redirects.
URL resourceUrl, base, next;
HttpURLConnection conn;
String location;
...
while (true)
{
resourceUrl = new URL(url);
conn = (HttpURLConnection) resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setInstanceFollowRedirects(false); // Make the logic below easier to detect redirections
conn.setRequestProperty("User-Agent", "Mozilla/5.0...");
switch (conn.getResponseCode())
{
case HttpURLConnection.HTTP_MOVED_PERM:
case HttpURLConnection.HTTP_MOVED_TEMP:
location = conn.getHeaderField("Location");
location = URLDecoder.decode(location, "UTF-8");
base = new URL(url);
next = new URL(base, location); // Deal with relative URLs
url = next.toExternalForm();
continue;
}
break;
}
is = conn.openStream();
...
This is only one solution that works for more than 1 redirects. Thank you!
– Roger Alien
Jul 31 '16 at 3:37
This works beautifully for multiple redirects (HTTPS API -> HTTP -> HTTP image)! Perfect simple solution.
– EricH206
Jan 6 '17 at 11:50
Just make you you limit the number of redirects otherwise you may get into an infinite loop
– Archimedes Trajano
Apr 15 '17 at 3:20
I have no idea how changing the URI scheme from "http" to "https" has any consequences on security. Why would that be worse to changing to another origin on "http"?
– Julian Reschke
Nov 30 '17 at 12:16
@Nathan - thanks for the details, but I still don't buy it. For instance, if's under the control of the client whether any credentials or client certs are sent. If it hurts, don't do it (in this case, do not follow the redirect).
– Julian Reschke
Dec 1 '17 at 5:30
|
show 3 more comments
HttpURLConnection by design won't automatically redirect from HTTP to HTTPS (or vice versa). Following the redirect may have serious security consequences. SSL (hence HTTPS) creates a session that is unique to the user. This session can be reused for multiple requests. Thus, the server can track all of the requests made from a single person. This is a weak form of identity and is exploitable. Also, the SSL handshake can ask for the client's certificate. If sent to the server, then the client's identity is given to the server.
As erickson points out, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
With that understood, here's the code which will follow the redirects.
URL resourceUrl, base, next;
HttpURLConnection conn;
String location;
...
while (true)
{
resourceUrl = new URL(url);
conn = (HttpURLConnection) resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setInstanceFollowRedirects(false); // Make the logic below easier to detect redirections
conn.setRequestProperty("User-Agent", "Mozilla/5.0...");
switch (conn.getResponseCode())
{
case HttpURLConnection.HTTP_MOVED_PERM:
case HttpURLConnection.HTTP_MOVED_TEMP:
location = conn.getHeaderField("Location");
location = URLDecoder.decode(location, "UTF-8");
base = new URL(url);
next = new URL(base, location); // Deal with relative URLs
url = next.toExternalForm();
continue;
}
break;
}
is = conn.openStream();
...
HttpURLConnection by design won't automatically redirect from HTTP to HTTPS (or vice versa). Following the redirect may have serious security consequences. SSL (hence HTTPS) creates a session that is unique to the user. This session can be reused for multiple requests. Thus, the server can track all of the requests made from a single person. This is a weak form of identity and is exploitable. Also, the SSL handshake can ask for the client's certificate. If sent to the server, then the client's identity is given to the server.
As erickson points out, suppose the application is set up to perform client authentication automatically. The user expects to be surfing anonymously because he's using HTTP. But if his client follows HTTPS without asking, his identity is revealed to the server.
With that understood, here's the code which will follow the redirects.
URL resourceUrl, base, next;
HttpURLConnection conn;
String location;
...
while (true)
{
resourceUrl = new URL(url);
conn = (HttpURLConnection) resourceUrl.openConnection();
conn.setConnectTimeout(15000);
conn.setReadTimeout(15000);
conn.setInstanceFollowRedirects(false); // Make the logic below easier to detect redirections
conn.setRequestProperty("User-Agent", "Mozilla/5.0...");
switch (conn.getResponseCode())
{
case HttpURLConnection.HTTP_MOVED_PERM:
case HttpURLConnection.HTTP_MOVED_TEMP:
location = conn.getHeaderField("Location");
location = URLDecoder.decode(location, "UTF-8");
base = new URL(url);
next = new URL(base, location); // Deal with relative URLs
url = next.toExternalForm();
continue;
}
break;
}
is = conn.openStream();
...
edited Nov 30 '17 at 18:42
answered Sep 25 '14 at 18:59
NathanNathan
3,47552852
3,47552852
This is only one solution that works for more than 1 redirects. Thank you!
– Roger Alien
Jul 31 '16 at 3:37
This works beautifully for multiple redirects (HTTPS API -> HTTP -> HTTP image)! Perfect simple solution.
– EricH206
Jan 6 '17 at 11:50
Just make you you limit the number of redirects otherwise you may get into an infinite loop
– Archimedes Trajano
Apr 15 '17 at 3:20
I have no idea how changing the URI scheme from "http" to "https" has any consequences on security. Why would that be worse to changing to another origin on "http"?
– Julian Reschke
Nov 30 '17 at 12:16
@Nathan - thanks for the details, but I still don't buy it. For instance, if's under the control of the client whether any credentials or client certs are sent. If it hurts, don't do it (in this case, do not follow the redirect).
– Julian Reschke
Dec 1 '17 at 5:30
|
show 3 more comments
This is only one solution that works for more than 1 redirects. Thank you!
– Roger Alien
Jul 31 '16 at 3:37
This works beautifully for multiple redirects (HTTPS API -> HTTP -> HTTP image)! Perfect simple solution.
– EricH206
Jan 6 '17 at 11:50
Just make you you limit the number of redirects otherwise you may get into an infinite loop
– Archimedes Trajano
Apr 15 '17 at 3:20
I have no idea how changing the URI scheme from "http" to "https" has any consequences on security. Why would that be worse to changing to another origin on "http"?
– Julian Reschke
Nov 30 '17 at 12:16
@Nathan - thanks for the details, but I still don't buy it. For instance, if's under the control of the client whether any credentials or client certs are sent. If it hurts, don't do it (in this case, do not follow the redirect).
– Julian Reschke
Dec 1 '17 at 5:30
This is only one solution that works for more than 1 redirects. Thank you!
– Roger Alien
Jul 31 '16 at 3:37
This is only one solution that works for more than 1 redirects. Thank you!
– Roger Alien
Jul 31 '16 at 3:37
This works beautifully for multiple redirects (HTTPS API -> HTTP -> HTTP image)! Perfect simple solution.
– EricH206
Jan 6 '17 at 11:50
This works beautifully for multiple redirects (HTTPS API -> HTTP -> HTTP image)! Perfect simple solution.
– EricH206
Jan 6 '17 at 11:50
Just make you you limit the number of redirects otherwise you may get into an infinite loop
– Archimedes Trajano
Apr 15 '17 at 3:20
Just make you you limit the number of redirects otherwise you may get into an infinite loop
– Archimedes Trajano
Apr 15 '17 at 3:20
I have no idea how changing the URI scheme from "http" to "https" has any consequences on security. Why would that be worse to changing to another origin on "http"?
– Julian Reschke
Nov 30 '17 at 12:16
I have no idea how changing the URI scheme from "http" to "https" has any consequences on security. Why would that be worse to changing to another origin on "http"?
– Julian Reschke
Nov 30 '17 at 12:16
@Nathan - thanks for the details, but I still don't buy it. For instance, if's under the control of the client whether any credentials or client certs are sent. If it hurts, don't do it (in this case, do not follow the redirect).
– Julian Reschke
Dec 1 '17 at 5:30
@Nathan - thanks for the details, but I still don't buy it. For instance, if's under the control of the client whether any credentials or client certs are sent. If it hurts, don't do it (in this case, do not follow the redirect).
– Julian Reschke
Dec 1 '17 at 5:30
|
show 3 more comments
Has something called HttpURLConnection.setFollowRedirects(false)
by any chance?
You could always call
conn.setInstanceFollowRedirects(true);
if you want to make sure you don't affect the rest of the behaviour of the app.
Ooo... didn't know about that... Nice find... I was about to look up the class incase there was logic like that.... It makes sense that it would be returning that header giving the single responsibility principal.... now go back to answering C# questions :P [I'm kidding]
– monksy
Dec 10 '09 at 21:47
2
Note that setFollowRedirects() should be called on the class, and not on an instance.
– karlbecker_com
Apr 4 '13 at 21:50
2
@dldnh: While karlbecker_com was absolutely right about callingsetFollowRedirects
on the type,setInstanceFollowRedirects
is an instance method and can't be called on the type.
– Jon Skeet
Apr 13 '13 at 6:43
1
uggh, how did I misread that. sorry about the incorrect edit. also tried to rollback and not sure how I bollocksed that as well.
– dldnh
Apr 13 '13 at 10:43
This does not help with https redirect I think..
– Koray Tugay
Jul 6 '18 at 16:18
add a comment |
Has something called HttpURLConnection.setFollowRedirects(false)
by any chance?
You could always call
conn.setInstanceFollowRedirects(true);
if you want to make sure you don't affect the rest of the behaviour of the app.
Ooo... didn't know about that... Nice find... I was about to look up the class incase there was logic like that.... It makes sense that it would be returning that header giving the single responsibility principal.... now go back to answering C# questions :P [I'm kidding]
– monksy
Dec 10 '09 at 21:47
2
Note that setFollowRedirects() should be called on the class, and not on an instance.
– karlbecker_com
Apr 4 '13 at 21:50
2
@dldnh: While karlbecker_com was absolutely right about callingsetFollowRedirects
on the type,setInstanceFollowRedirects
is an instance method and can't be called on the type.
– Jon Skeet
Apr 13 '13 at 6:43
1
uggh, how did I misread that. sorry about the incorrect edit. also tried to rollback and not sure how I bollocksed that as well.
– dldnh
Apr 13 '13 at 10:43
This does not help with https redirect I think..
– Koray Tugay
Jul 6 '18 at 16:18
add a comment |
Has something called HttpURLConnection.setFollowRedirects(false)
by any chance?
You could always call
conn.setInstanceFollowRedirects(true);
if you want to make sure you don't affect the rest of the behaviour of the app.
Has something called HttpURLConnection.setFollowRedirects(false)
by any chance?
You could always call
conn.setInstanceFollowRedirects(true);
if you want to make sure you don't affect the rest of the behaviour of the app.
edited Apr 13 '13 at 10:41
dldnh
8,39332747
8,39332747
answered Dec 10 '09 at 21:41
Jon SkeetJon Skeet
1085k68679258428
1085k68679258428
Ooo... didn't know about that... Nice find... I was about to look up the class incase there was logic like that.... It makes sense that it would be returning that header giving the single responsibility principal.... now go back to answering C# questions :P [I'm kidding]
– monksy
Dec 10 '09 at 21:47
2
Note that setFollowRedirects() should be called on the class, and not on an instance.
– karlbecker_com
Apr 4 '13 at 21:50
2
@dldnh: While karlbecker_com was absolutely right about callingsetFollowRedirects
on the type,setInstanceFollowRedirects
is an instance method and can't be called on the type.
– Jon Skeet
Apr 13 '13 at 6:43
1
uggh, how did I misread that. sorry about the incorrect edit. also tried to rollback and not sure how I bollocksed that as well.
– dldnh
Apr 13 '13 at 10:43
This does not help with https redirect I think..
– Koray Tugay
Jul 6 '18 at 16:18
add a comment |
Ooo... didn't know about that... Nice find... I was about to look up the class incase there was logic like that.... It makes sense that it would be returning that header giving the single responsibility principal.... now go back to answering C# questions :P [I'm kidding]
– monksy
Dec 10 '09 at 21:47
2
Note that setFollowRedirects() should be called on the class, and not on an instance.
– karlbecker_com
Apr 4 '13 at 21:50
2
@dldnh: While karlbecker_com was absolutely right about callingsetFollowRedirects
on the type,setInstanceFollowRedirects
is an instance method and can't be called on the type.
– Jon Skeet
Apr 13 '13 at 6:43
1
uggh, how did I misread that. sorry about the incorrect edit. also tried to rollback and not sure how I bollocksed that as well.
– dldnh
Apr 13 '13 at 10:43
This does not help with https redirect I think..
– Koray Tugay
Jul 6 '18 at 16:18
Ooo... didn't know about that... Nice find... I was about to look up the class incase there was logic like that.... It makes sense that it would be returning that header giving the single responsibility principal.... now go back to answering C# questions :P [I'm kidding]
– monksy
Dec 10 '09 at 21:47
Ooo... didn't know about that... Nice find... I was about to look up the class incase there was logic like that.... It makes sense that it would be returning that header giving the single responsibility principal.... now go back to answering C# questions :P [I'm kidding]
– monksy
Dec 10 '09 at 21:47
2
2
Note that setFollowRedirects() should be called on the class, and not on an instance.
– karlbecker_com
Apr 4 '13 at 21:50
Note that setFollowRedirects() should be called on the class, and not on an instance.
– karlbecker_com
Apr 4 '13 at 21:50
2
2
@dldnh: While karlbecker_com was absolutely right about calling
setFollowRedirects
on the type, setInstanceFollowRedirects
is an instance method and can't be called on the type.– Jon Skeet
Apr 13 '13 at 6:43
@dldnh: While karlbecker_com was absolutely right about calling
setFollowRedirects
on the type, setInstanceFollowRedirects
is an instance method and can't be called on the type.– Jon Skeet
Apr 13 '13 at 6:43
1
1
uggh, how did I misread that. sorry about the incorrect edit. also tried to rollback and not sure how I bollocksed that as well.
– dldnh
Apr 13 '13 at 10:43
uggh, how did I misread that. sorry about the incorrect edit. also tried to rollback and not sure how I bollocksed that as well.
– dldnh
Apr 13 '13 at 10:43
This does not help with https redirect I think..
– Koray Tugay
Jul 6 '18 at 16:18
This does not help with https redirect I think..
– Koray Tugay
Jul 6 '18 at 16:18
add a comment |
As mentioned by some of you above, the setFollowRedirect and setInstanceFollowRedirects only work automatically when the redirected protocol is same . ie from http to http and https to https.
setFolloRedirect is at class level and sets this for all instances of the url connection, whereas setInstanceFollowRedirects is only for a given instance. This way we can have different behavior for different instances.
I found a very good example here http://www.mkyong.com/java/java-httpurlconnection-follow-redirect-example/
add a comment |
As mentioned by some of you above, the setFollowRedirect and setInstanceFollowRedirects only work automatically when the redirected protocol is same . ie from http to http and https to https.
setFolloRedirect is at class level and sets this for all instances of the url connection, whereas setInstanceFollowRedirects is only for a given instance. This way we can have different behavior for different instances.
I found a very good example here http://www.mkyong.com/java/java-httpurlconnection-follow-redirect-example/
add a comment |
As mentioned by some of you above, the setFollowRedirect and setInstanceFollowRedirects only work automatically when the redirected protocol is same . ie from http to http and https to https.
setFolloRedirect is at class level and sets this for all instances of the url connection, whereas setInstanceFollowRedirects is only for a given instance. This way we can have different behavior for different instances.
I found a very good example here http://www.mkyong.com/java/java-httpurlconnection-follow-redirect-example/
As mentioned by some of you above, the setFollowRedirect and setInstanceFollowRedirects only work automatically when the redirected protocol is same . ie from http to http and https to https.
setFolloRedirect is at class level and sets this for all instances of the url connection, whereas setInstanceFollowRedirects is only for a given instance. This way we can have different behavior for different instances.
I found a very good example here http://www.mkyong.com/java/java-httpurlconnection-follow-redirect-example/
answered Oct 1 '13 at 6:12
ShalvikaShalvika
32135
32135
add a comment |
add a comment |
Another option can be to use Apache HttpComponents Client:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
Sample code:
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpGet httpget = new HttpGet("https://media-hearth.cursecdn.com/avatars/330/498/212.png");
CloseableHttpResponse response = httpclient.execute(httpget);
final HttpEntity entity = response.getEntity();
final InputStream is = entity.getContent();
add a comment |
Another option can be to use Apache HttpComponents Client:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
Sample code:
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpGet httpget = new HttpGet("https://media-hearth.cursecdn.com/avatars/330/498/212.png");
CloseableHttpResponse response = httpclient.execute(httpget);
final HttpEntity entity = response.getEntity();
final InputStream is = entity.getContent();
add a comment |
Another option can be to use Apache HttpComponents Client:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
Sample code:
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpGet httpget = new HttpGet("https://media-hearth.cursecdn.com/avatars/330/498/212.png");
CloseableHttpResponse response = httpclient.execute(httpget);
final HttpEntity entity = response.getEntity();
final InputStream is = entity.getContent();
Another option can be to use Apache HttpComponents Client:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
Sample code:
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpGet httpget = new HttpGet("https://media-hearth.cursecdn.com/avatars/330/498/212.png");
CloseableHttpResponse response = httpclient.execute(httpget);
final HttpEntity entity = response.getEntity();
final InputStream is = entity.getContent();
edited Jul 9 '18 at 13:18
answered Jul 6 '18 at 16:16


Koray TugayKoray Tugay
8,82926114222
8,82926114222
add a comment |
add a comment |
HTTPUrlConnection is not responsible for handling the response of the object. It is performance as expected, it grabs the content of the URL requested. It is up to you the user of the functionality to interpret the response. It is not able to read the intentions of the developer without specification.
7
Why it has setInstanceFollowRedirects in this case? ))
– Shcheklein
Dec 10 '09 at 21:46
My guess is that it was a suggested feature to add in later, it makes sense.. my comment was more of reflected toward... the class is designed to go and grab web content and bring it back... people may want to get non HTTP 200 messages.
– monksy
Dec 10 '09 at 21:49
add a comment |
HTTPUrlConnection is not responsible for handling the response of the object. It is performance as expected, it grabs the content of the URL requested. It is up to you the user of the functionality to interpret the response. It is not able to read the intentions of the developer without specification.
7
Why it has setInstanceFollowRedirects in this case? ))
– Shcheklein
Dec 10 '09 at 21:46
My guess is that it was a suggested feature to add in later, it makes sense.. my comment was more of reflected toward... the class is designed to go and grab web content and bring it back... people may want to get non HTTP 200 messages.
– monksy
Dec 10 '09 at 21:49
add a comment |
HTTPUrlConnection is not responsible for handling the response of the object. It is performance as expected, it grabs the content of the URL requested. It is up to you the user of the functionality to interpret the response. It is not able to read the intentions of the developer without specification.
HTTPUrlConnection is not responsible for handling the response of the object. It is performance as expected, it grabs the content of the URL requested. It is up to you the user of the functionality to interpret the response. It is not able to read the intentions of the developer without specification.
answered Dec 10 '09 at 21:41
monksymonksy
10.5k1256115
10.5k1256115
7
Why it has setInstanceFollowRedirects in this case? ))
– Shcheklein
Dec 10 '09 at 21:46
My guess is that it was a suggested feature to add in later, it makes sense.. my comment was more of reflected toward... the class is designed to go and grab web content and bring it back... people may want to get non HTTP 200 messages.
– monksy
Dec 10 '09 at 21:49
add a comment |
7
Why it has setInstanceFollowRedirects in this case? ))
– Shcheklein
Dec 10 '09 at 21:46
My guess is that it was a suggested feature to add in later, it makes sense.. my comment was more of reflected toward... the class is designed to go and grab web content and bring it back... people may want to get non HTTP 200 messages.
– monksy
Dec 10 '09 at 21:49
7
7
Why it has setInstanceFollowRedirects in this case? ))
– Shcheklein
Dec 10 '09 at 21:46
Why it has setInstanceFollowRedirects in this case? ))
– Shcheklein
Dec 10 '09 at 21:46
My guess is that it was a suggested feature to add in later, it makes sense.. my comment was more of reflected toward... the class is designed to go and grab web content and bring it back... people may want to get non HTTP 200 messages.
– monksy
Dec 10 '09 at 21:49
My guess is that it was a suggested feature to add in later, it makes sense.. my comment was more of reflected toward... the class is designed to go and grab web content and bring it back... people may want to get non HTTP 200 messages.
– monksy
Dec 10 '09 at 21:49
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f1884230%2furlconnection-doesnt-follow-redirect%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
km,s,Tktx P nw ndeEYXS oQoxJm9pwmEVz n,tiXkfu7ub464i9B1Ju sxX3hm