How to modify class variable in a separated running process?
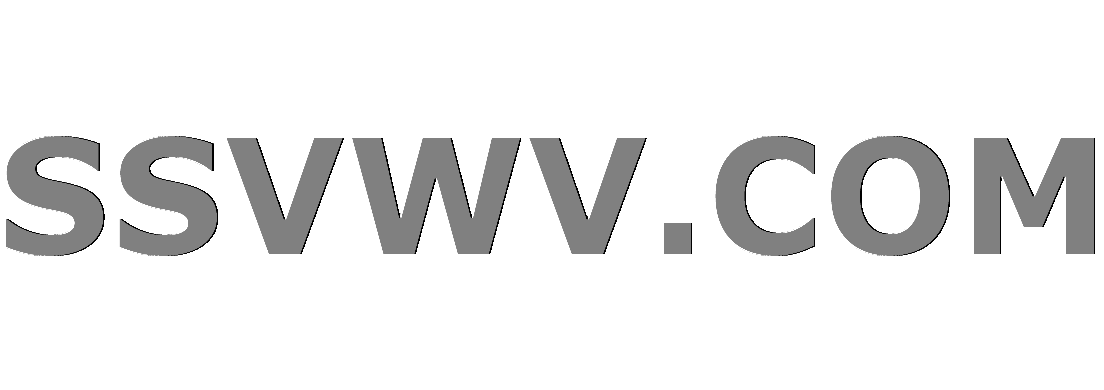
Multi tool use
I am trying to write a Python class method that execute for a fixed amount of time and modify a class instance variable during execution if needed.
For my project, I need read_key
method in the following code to run exactly 5 seconds (no more no less).
During this 5 seconds, I use getch.getch()
to try reading
one character from the keyboard and assign it to instance variable self.key
. Because getch.getch()
will block the execution of its process until a keystroke is detected. If the user doesn't press any key in this 5 seconds window, let self.key
be None
.
So far, I can only think of using multiprocessing
and its join()
to realize this 5 seconds "timer" effect.
import getch
import multiprocessing
class A:
def __init__(self):
self.key = None
self.read_key()
# to check whether instance variable is successfully modified
print(self.key)
def read_key(self):
def scan():
self.key = None
while True:
key = getch.getch()
if key == 'a':
self.key = key
print('updated self key to ' + str(self.key))
else:
print('invalid entry ' + str(key))
p = multiprocessing.Process(target=scan)
p.start()
p.join(5)
p.terminate()
if __name__ == '__main__':
A()
Running the above code, it does take 5 seconds to finish. If user presses a key after program is launched, I expect the print out to be:
'updated self key to a'
'a'
What I actually see:
'updated self key to a'
None
Apparently, even though self.key = key
is executed, the actual instance variable self.key
. Any idea why and how to modify self.key
if the user presses the a key?
python timer multiprocessing instance instance-variables
add a comment |
I am trying to write a Python class method that execute for a fixed amount of time and modify a class instance variable during execution if needed.
For my project, I need read_key
method in the following code to run exactly 5 seconds (no more no less).
During this 5 seconds, I use getch.getch()
to try reading
one character from the keyboard and assign it to instance variable self.key
. Because getch.getch()
will block the execution of its process until a keystroke is detected. If the user doesn't press any key in this 5 seconds window, let self.key
be None
.
So far, I can only think of using multiprocessing
and its join()
to realize this 5 seconds "timer" effect.
import getch
import multiprocessing
class A:
def __init__(self):
self.key = None
self.read_key()
# to check whether instance variable is successfully modified
print(self.key)
def read_key(self):
def scan():
self.key = None
while True:
key = getch.getch()
if key == 'a':
self.key = key
print('updated self key to ' + str(self.key))
else:
print('invalid entry ' + str(key))
p = multiprocessing.Process(target=scan)
p.start()
p.join(5)
p.terminate()
if __name__ == '__main__':
A()
Running the above code, it does take 5 seconds to finish. If user presses a key after program is launched, I expect the print out to be:
'updated self key to a'
'a'
What I actually see:
'updated self key to a'
None
Apparently, even though self.key = key
is executed, the actual instance variable self.key
. Any idea why and how to modify self.key
if the user presses the a key?
python timer multiprocessing instance instance-variables
You cannot share information like that between processes. See the Python docs about this. For your specific problem see Keyboard input with timeout in Python.
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Python multiprocessing global variable updates not returned to parent
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Cannot change class variables with multiprocessing.Process object in Python3
– finefoot
Dec 31 '18 at 12:43
thanks Jayjayyy, I managed to solve the issue using a workaround. Solution posted below.
– Rookie
Jan 1 at 5:39
add a comment |
I am trying to write a Python class method that execute for a fixed amount of time and modify a class instance variable during execution if needed.
For my project, I need read_key
method in the following code to run exactly 5 seconds (no more no less).
During this 5 seconds, I use getch.getch()
to try reading
one character from the keyboard and assign it to instance variable self.key
. Because getch.getch()
will block the execution of its process until a keystroke is detected. If the user doesn't press any key in this 5 seconds window, let self.key
be None
.
So far, I can only think of using multiprocessing
and its join()
to realize this 5 seconds "timer" effect.
import getch
import multiprocessing
class A:
def __init__(self):
self.key = None
self.read_key()
# to check whether instance variable is successfully modified
print(self.key)
def read_key(self):
def scan():
self.key = None
while True:
key = getch.getch()
if key == 'a':
self.key = key
print('updated self key to ' + str(self.key))
else:
print('invalid entry ' + str(key))
p = multiprocessing.Process(target=scan)
p.start()
p.join(5)
p.terminate()
if __name__ == '__main__':
A()
Running the above code, it does take 5 seconds to finish. If user presses a key after program is launched, I expect the print out to be:
'updated self key to a'
'a'
What I actually see:
'updated self key to a'
None
Apparently, even though self.key = key
is executed, the actual instance variable self.key
. Any idea why and how to modify self.key
if the user presses the a key?
python timer multiprocessing instance instance-variables
I am trying to write a Python class method that execute for a fixed amount of time and modify a class instance variable during execution if needed.
For my project, I need read_key
method in the following code to run exactly 5 seconds (no more no less).
During this 5 seconds, I use getch.getch()
to try reading
one character from the keyboard and assign it to instance variable self.key
. Because getch.getch()
will block the execution of its process until a keystroke is detected. If the user doesn't press any key in this 5 seconds window, let self.key
be None
.
So far, I can only think of using multiprocessing
and its join()
to realize this 5 seconds "timer" effect.
import getch
import multiprocessing
class A:
def __init__(self):
self.key = None
self.read_key()
# to check whether instance variable is successfully modified
print(self.key)
def read_key(self):
def scan():
self.key = None
while True:
key = getch.getch()
if key == 'a':
self.key = key
print('updated self key to ' + str(self.key))
else:
print('invalid entry ' + str(key))
p = multiprocessing.Process(target=scan)
p.start()
p.join(5)
p.terminate()
if __name__ == '__main__':
A()
Running the above code, it does take 5 seconds to finish. If user presses a key after program is launched, I expect the print out to be:
'updated self key to a'
'a'
What I actually see:
'updated self key to a'
None
Apparently, even though self.key = key
is executed, the actual instance variable self.key
. Any idea why and how to modify self.key
if the user presses the a key?
python timer multiprocessing instance instance-variables
python timer multiprocessing instance instance-variables
edited Dec 31 '18 at 12:22


finefoot
2,71531834
2,71531834
asked Dec 31 '18 at 11:47
RookieRookie
741210
741210
You cannot share information like that between processes. See the Python docs about this. For your specific problem see Keyboard input with timeout in Python.
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Python multiprocessing global variable updates not returned to parent
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Cannot change class variables with multiprocessing.Process object in Python3
– finefoot
Dec 31 '18 at 12:43
thanks Jayjayyy, I managed to solve the issue using a workaround. Solution posted below.
– Rookie
Jan 1 at 5:39
add a comment |
You cannot share information like that between processes. See the Python docs about this. For your specific problem see Keyboard input with timeout in Python.
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Python multiprocessing global variable updates not returned to parent
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Cannot change class variables with multiprocessing.Process object in Python3
– finefoot
Dec 31 '18 at 12:43
thanks Jayjayyy, I managed to solve the issue using a workaround. Solution posted below.
– Rookie
Jan 1 at 5:39
You cannot share information like that between processes. See the Python docs about this. For your specific problem see Keyboard input with timeout in Python.
– finefoot
Dec 31 '18 at 12:40
You cannot share information like that between processes. See the Python docs about this. For your specific problem see Keyboard input with timeout in Python.
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Python multiprocessing global variable updates not returned to parent
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Python multiprocessing global variable updates not returned to parent
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Cannot change class variables with multiprocessing.Process object in Python3
– finefoot
Dec 31 '18 at 12:43
Possible duplicate of Cannot change class variables with multiprocessing.Process object in Python3
– finefoot
Dec 31 '18 at 12:43
thanks Jayjayyy, I managed to solve the issue using a workaround. Solution posted below.
– Rookie
Jan 1 at 5:39
thanks Jayjayyy, I managed to solve the issue using a workaround. Solution posted below.
– Rookie
Jan 1 at 5:39
add a comment |
1 Answer
1
active
oldest
votes
I managed to come up with the following solution using multiprocessing
's shared variable. Using a shared variable detected
, I am able to detect whether user has pressed any of the special keys I would like to detect. Then I manually set the class instance variable at the end of the method to achieve the effect of having self.key
attribute set in exactly 5 seconds.
https://docs.python.org/2/library/multiprocessing.html#multiprocessing.Value
def read_key(self):
def scan(detected):
while True:
key = getch.getch()
if key == 'a':
detected.value = b'a'
elif key == 'd':
detected.value = b'd'
elif key == 'w':
detected.value = b'w'
elif key == 's':
detected.value = b's'
detected = Value('c', b'z')
p = Process(target=scan, args=(detected,))
p.start()
p.join(self.time_interval)
p.terminate()
byte_str_map = {b'a': 'a',
b's': 's',
b'w': 'w',
b'd': 'd'}
self.key = byte_str_map.get(detected.value)
I'm glad to hear that you figured it out :-) Out of curiosity: why are you using Python 2? Or did you just link the wrong docs?
– finefoot
Jan 1 at 13:02
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987117%2fhow-to-modify-class-variable-in-a-separated-running-process%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I managed to come up with the following solution using multiprocessing
's shared variable. Using a shared variable detected
, I am able to detect whether user has pressed any of the special keys I would like to detect. Then I manually set the class instance variable at the end of the method to achieve the effect of having self.key
attribute set in exactly 5 seconds.
https://docs.python.org/2/library/multiprocessing.html#multiprocessing.Value
def read_key(self):
def scan(detected):
while True:
key = getch.getch()
if key == 'a':
detected.value = b'a'
elif key == 'd':
detected.value = b'd'
elif key == 'w':
detected.value = b'w'
elif key == 's':
detected.value = b's'
detected = Value('c', b'z')
p = Process(target=scan, args=(detected,))
p.start()
p.join(self.time_interval)
p.terminate()
byte_str_map = {b'a': 'a',
b's': 's',
b'w': 'w',
b'd': 'd'}
self.key = byte_str_map.get(detected.value)
I'm glad to hear that you figured it out :-) Out of curiosity: why are you using Python 2? Or did you just link the wrong docs?
– finefoot
Jan 1 at 13:02
add a comment |
I managed to come up with the following solution using multiprocessing
's shared variable. Using a shared variable detected
, I am able to detect whether user has pressed any of the special keys I would like to detect. Then I manually set the class instance variable at the end of the method to achieve the effect of having self.key
attribute set in exactly 5 seconds.
https://docs.python.org/2/library/multiprocessing.html#multiprocessing.Value
def read_key(self):
def scan(detected):
while True:
key = getch.getch()
if key == 'a':
detected.value = b'a'
elif key == 'd':
detected.value = b'd'
elif key == 'w':
detected.value = b'w'
elif key == 's':
detected.value = b's'
detected = Value('c', b'z')
p = Process(target=scan, args=(detected,))
p.start()
p.join(self.time_interval)
p.terminate()
byte_str_map = {b'a': 'a',
b's': 's',
b'w': 'w',
b'd': 'd'}
self.key = byte_str_map.get(detected.value)
I'm glad to hear that you figured it out :-) Out of curiosity: why are you using Python 2? Or did you just link the wrong docs?
– finefoot
Jan 1 at 13:02
add a comment |
I managed to come up with the following solution using multiprocessing
's shared variable. Using a shared variable detected
, I am able to detect whether user has pressed any of the special keys I would like to detect. Then I manually set the class instance variable at the end of the method to achieve the effect of having self.key
attribute set in exactly 5 seconds.
https://docs.python.org/2/library/multiprocessing.html#multiprocessing.Value
def read_key(self):
def scan(detected):
while True:
key = getch.getch()
if key == 'a':
detected.value = b'a'
elif key == 'd':
detected.value = b'd'
elif key == 'w':
detected.value = b'w'
elif key == 's':
detected.value = b's'
detected = Value('c', b'z')
p = Process(target=scan, args=(detected,))
p.start()
p.join(self.time_interval)
p.terminate()
byte_str_map = {b'a': 'a',
b's': 's',
b'w': 'w',
b'd': 'd'}
self.key = byte_str_map.get(detected.value)
I managed to come up with the following solution using multiprocessing
's shared variable. Using a shared variable detected
, I am able to detect whether user has pressed any of the special keys I would like to detect. Then I manually set the class instance variable at the end of the method to achieve the effect of having self.key
attribute set in exactly 5 seconds.
https://docs.python.org/2/library/multiprocessing.html#multiprocessing.Value
def read_key(self):
def scan(detected):
while True:
key = getch.getch()
if key == 'a':
detected.value = b'a'
elif key == 'd':
detected.value = b'd'
elif key == 'w':
detected.value = b'w'
elif key == 's':
detected.value = b's'
detected = Value('c', b'z')
p = Process(target=scan, args=(detected,))
p.start()
p.join(self.time_interval)
p.terminate()
byte_str_map = {b'a': 'a',
b's': 's',
b'w': 'w',
b'd': 'd'}
self.key = byte_str_map.get(detected.value)
edited Jan 1 at 13:00


finefoot
2,71531834
2,71531834
answered Jan 1 at 5:45
RookieRookie
741210
741210
I'm glad to hear that you figured it out :-) Out of curiosity: why are you using Python 2? Or did you just link the wrong docs?
– finefoot
Jan 1 at 13:02
add a comment |
I'm glad to hear that you figured it out :-) Out of curiosity: why are you using Python 2? Or did you just link the wrong docs?
– finefoot
Jan 1 at 13:02
I'm glad to hear that you figured it out :-) Out of curiosity: why are you using Python 2? Or did you just link the wrong docs?
– finefoot
Jan 1 at 13:02
I'm glad to hear that you figured it out :-) Out of curiosity: why are you using Python 2? Or did you just link the wrong docs?
– finefoot
Jan 1 at 13:02
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987117%2fhow-to-modify-class-variable-in-a-separated-running-process%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eTk3WEP J,IkR CkHtOe 8hrgeMZM,1N2,n8 2P2WLi0Jtaauqh5jblY viZUen8cqPCAyv,T,QOQBQH1Cr3
You cannot share information like that between processes. See the Python docs about this. For your specific problem see Keyboard input with timeout in Python.
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Python multiprocessing global variable updates not returned to parent
– finefoot
Dec 31 '18 at 12:40
Possible duplicate of Cannot change class variables with multiprocessing.Process object in Python3
– finefoot
Dec 31 '18 at 12:43
thanks Jayjayyy, I managed to solve the issue using a workaround. Solution posted below.
– Rookie
Jan 1 at 5:39