CORS Angular and SpringBoot - request blocked
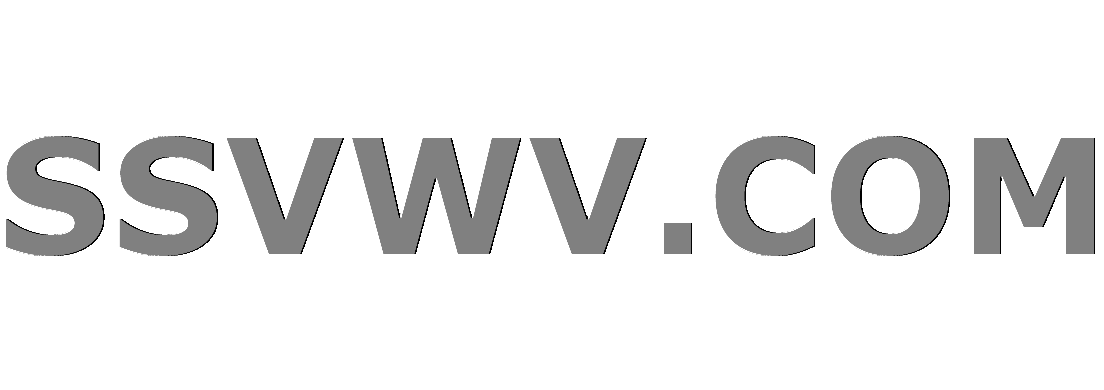
Multi tool use
I have a problem that I don't seem to figure out.
I want to send a http request from my
Angular client:
const url = 'http://localhost:8080/api';
console.log(this.http.get(url).subscribe(data => this.greeting = data));
to SpringBoot backend where I use CORS annotation:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/api/")
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
but I'm getting an error that it's blocked and redirects me to login all the time.
Failed to load http://localhost:8080/api: Redirect from 'http://localhost:8080/api' to 'http://localhost:8080/login' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Is there a way to change that?
I appreciate any hint or help regarding this. I would like to understand why this problem is happening.
spring angular spring-boot
add a comment |
I have a problem that I don't seem to figure out.
I want to send a http request from my
Angular client:
const url = 'http://localhost:8080/api';
console.log(this.http.get(url).subscribe(data => this.greeting = data));
to SpringBoot backend where I use CORS annotation:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/api/")
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
but I'm getting an error that it's blocked and redirects me to login all the time.
Failed to load http://localhost:8080/api: Redirect from 'http://localhost:8080/api' to 'http://localhost:8080/login' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Is there a way to change that?
I appreciate any hint or help regarding this. I would like to understand why this problem is happening.
spring angular spring-boot
Have you tried adding CORS to/login
?
– acdcjunior
Apr 3 '18 at 0:37
add a comment |
I have a problem that I don't seem to figure out.
I want to send a http request from my
Angular client:
const url = 'http://localhost:8080/api';
console.log(this.http.get(url).subscribe(data => this.greeting = data));
to SpringBoot backend where I use CORS annotation:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/api/")
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
but I'm getting an error that it's blocked and redirects me to login all the time.
Failed to load http://localhost:8080/api: Redirect from 'http://localhost:8080/api' to 'http://localhost:8080/login' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Is there a way to change that?
I appreciate any hint or help regarding this. I would like to understand why this problem is happening.
spring angular spring-boot
I have a problem that I don't seem to figure out.
I want to send a http request from my
Angular client:
const url = 'http://localhost:8080/api';
console.log(this.http.get(url).subscribe(data => this.greeting = data));
to SpringBoot backend where I use CORS annotation:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/api/")
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
but I'm getting an error that it's blocked and redirects me to login all the time.
Failed to load http://localhost:8080/api: Redirect from 'http://localhost:8080/api' to 'http://localhost:8080/login' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Is there a way to change that?
I appreciate any hint or help regarding this. I would like to understand why this problem is happening.
spring angular spring-boot
spring angular spring-boot
asked Apr 3 '18 at 0:33


Maciej CzarnotaMaciej Czarnota
3618
3618
Have you tried adding CORS to/login
?
– acdcjunior
Apr 3 '18 at 0:37
add a comment |
Have you tried adding CORS to/login
?
– acdcjunior
Apr 3 '18 at 0:37
Have you tried adding CORS to
/login
?– acdcjunior
Apr 3 '18 at 0:37
Have you tried adding CORS to
/login
?– acdcjunior
Apr 3 '18 at 0:37
add a comment |
4 Answers
4
active
oldest
votes
You have error in your RequestMapping
, as you have used @RequestMapping("/api/")
, this will be evaluated as http://your_url/api//
. Such mapping is not present in your controller, hence it is giving you CORS Origin error.
Just remove trailing /
from @RequestMapping("/api/")
so that it will be @RequestMapping("/api")
.
Your class should look like follows,
@RestController
@CrossOrigin(origins = "http://localhost:4200")
public class DemoController {
@RequestMapping(value = "/api", method = RequestMethod.GET)
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
}
Thank you it helped!! Thanks everyone for your help
– Maciej Czarnota
Apr 3 '18 at 19:41
add a comment |
This is the problem with your server-side. all you will have to do is do a component in your server-side and it will solve the issue. or refer here
follow this code:
@Component
@Order(Ordered.HIGHEST_PRECEDENCE)
public class RequestFilter implements Filter {
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) {
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest request = (HttpServletRequest) req;
response.setHeader("Access-Control-Allow-Origin", "http://localhost:4200");
response.setHeader("Access-Control-Allow-Methods", "POST, PUT, GET, OPTIONS, DELETE");
response.setHeader("Access-Control-Allow-Headers", "x-requested-with");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Credentials", "true");
if (!(request.getMethod().equalsIgnoreCase("OPTIONS"))) {
try {
chain.doFilter(req, res);
} catch(Exception e) {
e.printStackTrace();
}
} else {
System.out.println("Pre-flight");
response.setHeader("Access-Control-Allow-Methods", "POST,GET,DELETE");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Headers", "authorization, content-type," +
"access-control-request-headers,access-control-request-method,accept,origin,authorization,x-requested-with");
response.setStatus(HttpServletResponse.SC_OK);
}
}
}
add a comment |
As you've chosen to annotate your app, you must provide the @CrossOrigin
annotation for each method individually. That means you must also do:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/login/")
public Map<String,Object> login() {
/* ... */
}
Reference: https://spring.io/guides/gs/rest-service-cors/#_enabling_cors
add a comment |
Instead of annotating every method with @Crossorigin
you can use a filter
in web.xml
as mentioned below
Add Below filter to web.xml
file.
<filter>
<filter-name>cors</filter-name>
<filter-class>com.thetransactioncompany.cors.CORSFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>cors</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Dependency for the mentioned jar is given below.
<dependency>
<groupId>com.thetransactioncompany</groupId>
<artifactId>cors-filter</artifactId>
<version>2.5</version>
</dependency>
Thats it -Done.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49620597%2fcors-angular-and-springboot-request-blocked%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have error in your RequestMapping
, as you have used @RequestMapping("/api/")
, this will be evaluated as http://your_url/api//
. Such mapping is not present in your controller, hence it is giving you CORS Origin error.
Just remove trailing /
from @RequestMapping("/api/")
so that it will be @RequestMapping("/api")
.
Your class should look like follows,
@RestController
@CrossOrigin(origins = "http://localhost:4200")
public class DemoController {
@RequestMapping(value = "/api", method = RequestMethod.GET)
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
}
Thank you it helped!! Thanks everyone for your help
– Maciej Czarnota
Apr 3 '18 at 19:41
add a comment |
You have error in your RequestMapping
, as you have used @RequestMapping("/api/")
, this will be evaluated as http://your_url/api//
. Such mapping is not present in your controller, hence it is giving you CORS Origin error.
Just remove trailing /
from @RequestMapping("/api/")
so that it will be @RequestMapping("/api")
.
Your class should look like follows,
@RestController
@CrossOrigin(origins = "http://localhost:4200")
public class DemoController {
@RequestMapping(value = "/api", method = RequestMethod.GET)
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
}
Thank you it helped!! Thanks everyone for your help
– Maciej Czarnota
Apr 3 '18 at 19:41
add a comment |
You have error in your RequestMapping
, as you have used @RequestMapping("/api/")
, this will be evaluated as http://your_url/api//
. Such mapping is not present in your controller, hence it is giving you CORS Origin error.
Just remove trailing /
from @RequestMapping("/api/")
so that it will be @RequestMapping("/api")
.
Your class should look like follows,
@RestController
@CrossOrigin(origins = "http://localhost:4200")
public class DemoController {
@RequestMapping(value = "/api", method = RequestMethod.GET)
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
}
You have error in your RequestMapping
, as you have used @RequestMapping("/api/")
, this will be evaluated as http://your_url/api//
. Such mapping is not present in your controller, hence it is giving you CORS Origin error.
Just remove trailing /
from @RequestMapping("/api/")
so that it will be @RequestMapping("/api")
.
Your class should look like follows,
@RestController
@CrossOrigin(origins = "http://localhost:4200")
public class DemoController {
@RequestMapping(value = "/api", method = RequestMethod.GET)
public Map<String,Object> home() {
Map<String,Object> model = new HashMap<String,Object>();
model.put("id", UUID.randomUUID().toString());
model.put("content", "Hello World");
return model;
}
}
edited Apr 3 '18 at 4:22
answered Apr 3 '18 at 4:17
Paresh LomateParesh Lomate
319317
319317
Thank you it helped!! Thanks everyone for your help
– Maciej Czarnota
Apr 3 '18 at 19:41
add a comment |
Thank you it helped!! Thanks everyone for your help
– Maciej Czarnota
Apr 3 '18 at 19:41
Thank you it helped!! Thanks everyone for your help
– Maciej Czarnota
Apr 3 '18 at 19:41
Thank you it helped!! Thanks everyone for your help
– Maciej Czarnota
Apr 3 '18 at 19:41
add a comment |
This is the problem with your server-side. all you will have to do is do a component in your server-side and it will solve the issue. or refer here
follow this code:
@Component
@Order(Ordered.HIGHEST_PRECEDENCE)
public class RequestFilter implements Filter {
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) {
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest request = (HttpServletRequest) req;
response.setHeader("Access-Control-Allow-Origin", "http://localhost:4200");
response.setHeader("Access-Control-Allow-Methods", "POST, PUT, GET, OPTIONS, DELETE");
response.setHeader("Access-Control-Allow-Headers", "x-requested-with");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Credentials", "true");
if (!(request.getMethod().equalsIgnoreCase("OPTIONS"))) {
try {
chain.doFilter(req, res);
} catch(Exception e) {
e.printStackTrace();
}
} else {
System.out.println("Pre-flight");
response.setHeader("Access-Control-Allow-Methods", "POST,GET,DELETE");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Headers", "authorization, content-type," +
"access-control-request-headers,access-control-request-method,accept,origin,authorization,x-requested-with");
response.setStatus(HttpServletResponse.SC_OK);
}
}
}
add a comment |
This is the problem with your server-side. all you will have to do is do a component in your server-side and it will solve the issue. or refer here
follow this code:
@Component
@Order(Ordered.HIGHEST_PRECEDENCE)
public class RequestFilter implements Filter {
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) {
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest request = (HttpServletRequest) req;
response.setHeader("Access-Control-Allow-Origin", "http://localhost:4200");
response.setHeader("Access-Control-Allow-Methods", "POST, PUT, GET, OPTIONS, DELETE");
response.setHeader("Access-Control-Allow-Headers", "x-requested-with");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Credentials", "true");
if (!(request.getMethod().equalsIgnoreCase("OPTIONS"))) {
try {
chain.doFilter(req, res);
} catch(Exception e) {
e.printStackTrace();
}
} else {
System.out.println("Pre-flight");
response.setHeader("Access-Control-Allow-Methods", "POST,GET,DELETE");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Headers", "authorization, content-type," +
"access-control-request-headers,access-control-request-method,accept,origin,authorization,x-requested-with");
response.setStatus(HttpServletResponse.SC_OK);
}
}
}
add a comment |
This is the problem with your server-side. all you will have to do is do a component in your server-side and it will solve the issue. or refer here
follow this code:
@Component
@Order(Ordered.HIGHEST_PRECEDENCE)
public class RequestFilter implements Filter {
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) {
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest request = (HttpServletRequest) req;
response.setHeader("Access-Control-Allow-Origin", "http://localhost:4200");
response.setHeader("Access-Control-Allow-Methods", "POST, PUT, GET, OPTIONS, DELETE");
response.setHeader("Access-Control-Allow-Headers", "x-requested-with");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Credentials", "true");
if (!(request.getMethod().equalsIgnoreCase("OPTIONS"))) {
try {
chain.doFilter(req, res);
} catch(Exception e) {
e.printStackTrace();
}
} else {
System.out.println("Pre-flight");
response.setHeader("Access-Control-Allow-Methods", "POST,GET,DELETE");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Headers", "authorization, content-type," +
"access-control-request-headers,access-control-request-method,accept,origin,authorization,x-requested-with");
response.setStatus(HttpServletResponse.SC_OK);
}
}
}
This is the problem with your server-side. all you will have to do is do a component in your server-side and it will solve the issue. or refer here
follow this code:
@Component
@Order(Ordered.HIGHEST_PRECEDENCE)
public class RequestFilter implements Filter {
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) {
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest request = (HttpServletRequest) req;
response.setHeader("Access-Control-Allow-Origin", "http://localhost:4200");
response.setHeader("Access-Control-Allow-Methods", "POST, PUT, GET, OPTIONS, DELETE");
response.setHeader("Access-Control-Allow-Headers", "x-requested-with");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Credentials", "true");
if (!(request.getMethod().equalsIgnoreCase("OPTIONS"))) {
try {
chain.doFilter(req, res);
} catch(Exception e) {
e.printStackTrace();
}
} else {
System.out.println("Pre-flight");
response.setHeader("Access-Control-Allow-Methods", "POST,GET,DELETE");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Headers", "authorization, content-type," +
"access-control-request-headers,access-control-request-method,accept,origin,authorization,x-requested-with");
response.setStatus(HttpServletResponse.SC_OK);
}
}
}
answered Apr 3 '18 at 5:06
Abhishek EkaanthAbhishek Ekaanth
1,1851023
1,1851023
add a comment |
add a comment |
As you've chosen to annotate your app, you must provide the @CrossOrigin
annotation for each method individually. That means you must also do:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/login/")
public Map<String,Object> login() {
/* ... */
}
Reference: https://spring.io/guides/gs/rest-service-cors/#_enabling_cors
add a comment |
As you've chosen to annotate your app, you must provide the @CrossOrigin
annotation for each method individually. That means you must also do:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/login/")
public Map<String,Object> login() {
/* ... */
}
Reference: https://spring.io/guides/gs/rest-service-cors/#_enabling_cors
add a comment |
As you've chosen to annotate your app, you must provide the @CrossOrigin
annotation for each method individually. That means you must also do:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/login/")
public Map<String,Object> login() {
/* ... */
}
Reference: https://spring.io/guides/gs/rest-service-cors/#_enabling_cors
As you've chosen to annotate your app, you must provide the @CrossOrigin
annotation for each method individually. That means you must also do:
@CrossOrigin(origins = "http://localhost:4200/", maxAge = 3600)
@RequestMapping("/login/")
public Map<String,Object> login() {
/* ... */
}
Reference: https://spring.io/guides/gs/rest-service-cors/#_enabling_cors
answered Apr 3 '18 at 4:05
Randy CasburnRandy Casburn
4,9641318
4,9641318
add a comment |
add a comment |
Instead of annotating every method with @Crossorigin
you can use a filter
in web.xml
as mentioned below
Add Below filter to web.xml
file.
<filter>
<filter-name>cors</filter-name>
<filter-class>com.thetransactioncompany.cors.CORSFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>cors</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Dependency for the mentioned jar is given below.
<dependency>
<groupId>com.thetransactioncompany</groupId>
<artifactId>cors-filter</artifactId>
<version>2.5</version>
</dependency>
Thats it -Done.
add a comment |
Instead of annotating every method with @Crossorigin
you can use a filter
in web.xml
as mentioned below
Add Below filter to web.xml
file.
<filter>
<filter-name>cors</filter-name>
<filter-class>com.thetransactioncompany.cors.CORSFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>cors</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Dependency for the mentioned jar is given below.
<dependency>
<groupId>com.thetransactioncompany</groupId>
<artifactId>cors-filter</artifactId>
<version>2.5</version>
</dependency>
Thats it -Done.
add a comment |
Instead of annotating every method with @Crossorigin
you can use a filter
in web.xml
as mentioned below
Add Below filter to web.xml
file.
<filter>
<filter-name>cors</filter-name>
<filter-class>com.thetransactioncompany.cors.CORSFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>cors</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Dependency for the mentioned jar is given below.
<dependency>
<groupId>com.thetransactioncompany</groupId>
<artifactId>cors-filter</artifactId>
<version>2.5</version>
</dependency>
Thats it -Done.
Instead of annotating every method with @Crossorigin
you can use a filter
in web.xml
as mentioned below
Add Below filter to web.xml
file.
<filter>
<filter-name>cors</filter-name>
<filter-class>com.thetransactioncompany.cors.CORSFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>cors</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
Dependency for the mentioned jar is given below.
<dependency>
<groupId>com.thetransactioncompany</groupId>
<artifactId>cors-filter</artifactId>
<version>2.5</version>
</dependency>
Thats it -Done.
answered Apr 3 '18 at 4:57


vipin cpvipin cp
1,51711632
1,51711632
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49620597%2fcors-angular-and-springboot-request-blocked%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
TrQsbYvG4rQmrRaPQFk27cuK9,ou9ze7Ce,UxzSFScV,4xnwhaMGJsn8V,M18
Have you tried adding CORS to
/login
?– acdcjunior
Apr 3 '18 at 0:37