How to loop through a dictionary to remove values that match a string?
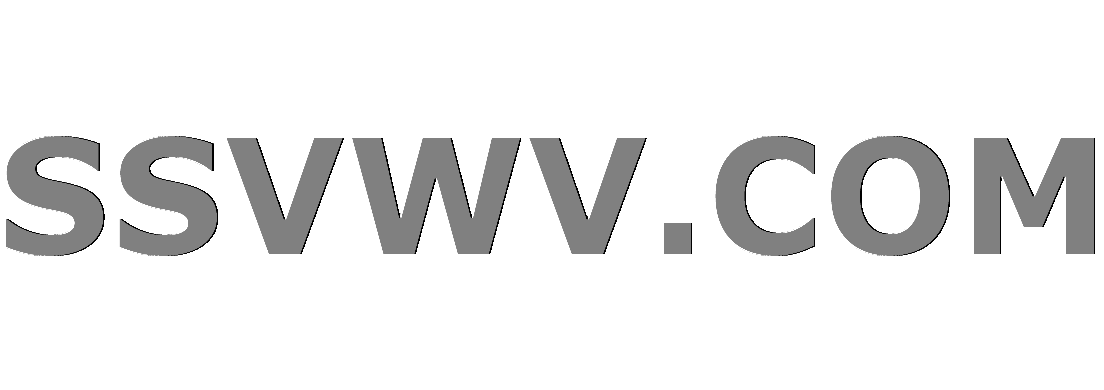
Multi tool use
I want to remove values from a dictionary if they contain a particular string and consequentially remove any keys that have an empty list as value.
mydict = {
'Getting links from: https://www.foo.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
],
'Getting links from: https://www.bar.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.boo.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'
]
}
val = "is"
for k, v in mydict.iteritems():
if v.contains(val):
del mydict[v]
The result I want is:
{
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
],
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
]
}
How can I remove all dictionary values that contain a string, and then any keys that have no values as a result?
python string python-2.7 list dictionary
add a comment |
I want to remove values from a dictionary if they contain a particular string and consequentially remove any keys that have an empty list as value.
mydict = {
'Getting links from: https://www.foo.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
],
'Getting links from: https://www.bar.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.boo.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'
]
}
val = "is"
for k, v in mydict.iteritems():
if v.contains(val):
del mydict[v]
The result I want is:
{
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
],
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
]
}
How can I remove all dictionary values that contain a string, and then any keys that have no values as a result?
python string python-2.7 list dictionary
2
you should notdel
an item of a dictionary within a loop that runs through this dictionary. You could use dict-comprehension to solve your issue.
– jojo
Dec 31 '18 at 12:33
add a comment |
I want to remove values from a dictionary if they contain a particular string and consequentially remove any keys that have an empty list as value.
mydict = {
'Getting links from: https://www.foo.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
],
'Getting links from: https://www.bar.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.boo.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'
]
}
val = "is"
for k, v in mydict.iteritems():
if v.contains(val):
del mydict[v]
The result I want is:
{
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
],
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
]
}
How can I remove all dictionary values that contain a string, and then any keys that have no values as a result?
python string python-2.7 list dictionary
I want to remove values from a dictionary if they contain a particular string and consequentially remove any keys that have an empty list as value.
mydict = {
'Getting links from: https://www.foo.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
],
'Getting links from: https://www.bar.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.boo.com/':
[
'+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'
]
}
val = "is"
for k, v in mydict.iteritems():
if v.contains(val):
del mydict[v]
The result I want is:
{
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
],
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
]
}
How can I remove all dictionary values that contain a string, and then any keys that have no values as a result?
python string python-2.7 list dictionary
python string python-2.7 list dictionary
edited Dec 31 '18 at 14:26
jojo
3,66122244
3,66122244
asked Dec 31 '18 at 12:12
lanelane
13512
13512
2
you should notdel
an item of a dictionary within a loop that runs through this dictionary. You could use dict-comprehension to solve your issue.
– jojo
Dec 31 '18 at 12:33
add a comment |
2
you should notdel
an item of a dictionary within a loop that runs through this dictionary. You could use dict-comprehension to solve your issue.
– jojo
Dec 31 '18 at 12:33
2
2
you should not
del
an item of a dictionary within a loop that runs through this dictionary. You could use dict-comprehension to solve your issue.– jojo
Dec 31 '18 at 12:33
you should not
del
an item of a dictionary within a loop that runs through this dictionary. You could use dict-comprehension to solve your issue.– jojo
Dec 31 '18 at 12:33
add a comment |
5 Answers
5
active
oldest
votes
You can use a list comprehension within a dictionary comprehension. You shouldn't change the number of items in a dictionary while you iterate that dictionary.
res = {k: [x for x in v if 'is' not in x] for k, v in mydict.items()}
# {'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/',
# '+---OK--- http://www.set.com/',
# '+---OK--- http://www.one.com/'],
# 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/'],
# 'Getting links from: https://www.boo.com/': }
If you wish to remove items with empty list values, you can in a subsequent step:
res = {k: v for k, v in res.items() if v}
Awesome. Thanks! One last question: if I wanted to remove multiple string values at once, could I add some sort of an 'or' argument? Such that, 'if 'is' or 'foo' or 'bar' not in x'
– lane
Dec 31 '18 at 13:02
1
[x for x in v if not any(item in x for item in ['foo', 'bar'])]
would work
– jpp
Dec 31 '18 at 13:16
1
Thank you again!
– lane
Dec 31 '18 at 13:23
add a comment |
With simple loop:
val = "is"
new_dict = dict()
for k, v in mydict.items():
values = [i for i in v if val not in i]
if values: new_dict[k] = values
print(new_dict)
The output:
{'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/', '+---OK--- http://www.set.com/', '+---OK--- http://www.one.com/'], 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/']}
add a comment |
This is a one-liner:
{k: [e for e in v if val not in e] for k, v in mydict.items() if any([val not in e for e in v])}
The expected output:
Out[1]: {
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
]
}
1
The one-liner works, of course, but hides the fact you are explicitly checking for'is'
in your values twice. That double string checking isn't required, better to use 2 lines.
– jpp
Dec 31 '18 at 12:35
1
@jpp in a 2 liner, on the other hand, you create an additional intermediary dict object, which is also not strictly required.
– jojo
Dec 31 '18 at 12:38
Except the expensive part isn't checking if a list is non-empty, it's checking if any strings within a list contain a substring. I suggest timing this for a larger input to satisfy yourself this is the case.
– jpp
Dec 31 '18 at 12:40
add a comment |
Using dict comprehension
, you can try the following:
import re
val = 'is'
# step 1 - remove line having is
mydict = {k:[re.sub(r'.*is*.', '', x) for x in v] for k,v in mydict.items()}
# filtering out keys if there is no value - if needed
mydict = {k:v for k,v in mydict.items() if len(v) > 0}
print(mydict)
{'Getting links from: https://www.foo.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'],
'Getting links from: https://www.bar.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['com/', 'com/']}
add a comment |
There are a couple of ways you could do it. One using regex and one without.
if you're not familiar with regex you could try this:
for key, value in mydict.items():
if val in value:
mydict.pop(key)
output would be:
mydict = {'Getting links from: https://www.bar.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'],
'Getting links from: https://www.foo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/']}
This do not match the expected output
– Daniel Mesejo
Dec 31 '18 at 12:19
The pop() method removes the specified item from the dictionary. The value of the removed item is the return value of the pop() method
– Brandon Bailey
Dec 31 '18 at 12:20
I'm referring to the fact that the OP include an expected output, what you wrote as the output in your answer do not match the OP expected output
– Daniel Mesejo
Dec 31 '18 at 12:21
its a starting point, you could refine it to strip away what you want
– Brandon Bailey
Dec 31 '18 at 12:23
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987387%2fhow-to-loop-through-a-dictionary-to-remove-values-that-match-a-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use a list comprehension within a dictionary comprehension. You shouldn't change the number of items in a dictionary while you iterate that dictionary.
res = {k: [x for x in v if 'is' not in x] for k, v in mydict.items()}
# {'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/',
# '+---OK--- http://www.set.com/',
# '+---OK--- http://www.one.com/'],
# 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/'],
# 'Getting links from: https://www.boo.com/': }
If you wish to remove items with empty list values, you can in a subsequent step:
res = {k: v for k, v in res.items() if v}
Awesome. Thanks! One last question: if I wanted to remove multiple string values at once, could I add some sort of an 'or' argument? Such that, 'if 'is' or 'foo' or 'bar' not in x'
– lane
Dec 31 '18 at 13:02
1
[x for x in v if not any(item in x for item in ['foo', 'bar'])]
would work
– jpp
Dec 31 '18 at 13:16
1
Thank you again!
– lane
Dec 31 '18 at 13:23
add a comment |
You can use a list comprehension within a dictionary comprehension. You shouldn't change the number of items in a dictionary while you iterate that dictionary.
res = {k: [x for x in v if 'is' not in x] for k, v in mydict.items()}
# {'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/',
# '+---OK--- http://www.set.com/',
# '+---OK--- http://www.one.com/'],
# 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/'],
# 'Getting links from: https://www.boo.com/': }
If you wish to remove items with empty list values, you can in a subsequent step:
res = {k: v for k, v in res.items() if v}
Awesome. Thanks! One last question: if I wanted to remove multiple string values at once, could I add some sort of an 'or' argument? Such that, 'if 'is' or 'foo' or 'bar' not in x'
– lane
Dec 31 '18 at 13:02
1
[x for x in v if not any(item in x for item in ['foo', 'bar'])]
would work
– jpp
Dec 31 '18 at 13:16
1
Thank you again!
– lane
Dec 31 '18 at 13:23
add a comment |
You can use a list comprehension within a dictionary comprehension. You shouldn't change the number of items in a dictionary while you iterate that dictionary.
res = {k: [x for x in v if 'is' not in x] for k, v in mydict.items()}
# {'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/',
# '+---OK--- http://www.set.com/',
# '+---OK--- http://www.one.com/'],
# 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/'],
# 'Getting links from: https://www.boo.com/': }
If you wish to remove items with empty list values, you can in a subsequent step:
res = {k: v for k, v in res.items() if v}
You can use a list comprehension within a dictionary comprehension. You shouldn't change the number of items in a dictionary while you iterate that dictionary.
res = {k: [x for x in v if 'is' not in x] for k, v in mydict.items()}
# {'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/',
# '+---OK--- http://www.set.com/',
# '+---OK--- http://www.one.com/'],
# 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/'],
# 'Getting links from: https://www.boo.com/': }
If you wish to remove items with empty list values, you can in a subsequent step:
res = {k: v for k, v in res.items() if v}
edited Dec 31 '18 at 12:34
answered Dec 31 '18 at 12:28


jppjpp
100k2161111
100k2161111
Awesome. Thanks! One last question: if I wanted to remove multiple string values at once, could I add some sort of an 'or' argument? Such that, 'if 'is' or 'foo' or 'bar' not in x'
– lane
Dec 31 '18 at 13:02
1
[x for x in v if not any(item in x for item in ['foo', 'bar'])]
would work
– jpp
Dec 31 '18 at 13:16
1
Thank you again!
– lane
Dec 31 '18 at 13:23
add a comment |
Awesome. Thanks! One last question: if I wanted to remove multiple string values at once, could I add some sort of an 'or' argument? Such that, 'if 'is' or 'foo' or 'bar' not in x'
– lane
Dec 31 '18 at 13:02
1
[x for x in v if not any(item in x for item in ['foo', 'bar'])]
would work
– jpp
Dec 31 '18 at 13:16
1
Thank you again!
– lane
Dec 31 '18 at 13:23
Awesome. Thanks! One last question: if I wanted to remove multiple string values at once, could I add some sort of an 'or' argument? Such that, 'if 'is' or 'foo' or 'bar' not in x'
– lane
Dec 31 '18 at 13:02
Awesome. Thanks! One last question: if I wanted to remove multiple string values at once, could I add some sort of an 'or' argument? Such that, 'if 'is' or 'foo' or 'bar' not in x'
– lane
Dec 31 '18 at 13:02
1
1
[x for x in v if not any(item in x for item in ['foo', 'bar'])]
would work– jpp
Dec 31 '18 at 13:16
[x for x in v if not any(item in x for item in ['foo', 'bar'])]
would work– jpp
Dec 31 '18 at 13:16
1
1
Thank you again!
– lane
Dec 31 '18 at 13:23
Thank you again!
– lane
Dec 31 '18 at 13:23
add a comment |
With simple loop:
val = "is"
new_dict = dict()
for k, v in mydict.items():
values = [i for i in v if val not in i]
if values: new_dict[k] = values
print(new_dict)
The output:
{'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/', '+---OK--- http://www.set.com/', '+---OK--- http://www.one.com/'], 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/']}
add a comment |
With simple loop:
val = "is"
new_dict = dict()
for k, v in mydict.items():
values = [i for i in v if val not in i]
if values: new_dict[k] = values
print(new_dict)
The output:
{'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/', '+---OK--- http://www.set.com/', '+---OK--- http://www.one.com/'], 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/']}
add a comment |
With simple loop:
val = "is"
new_dict = dict()
for k, v in mydict.items():
values = [i for i in v if val not in i]
if values: new_dict[k] = values
print(new_dict)
The output:
{'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/', '+---OK--- http://www.set.com/', '+---OK--- http://www.one.com/'], 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/']}
With simple loop:
val = "is"
new_dict = dict()
for k, v in mydict.items():
values = [i for i in v if val not in i]
if values: new_dict[k] = values
print(new_dict)
The output:
{'Getting links from: https://www.foo.com/': ['+-BROKEN- http://www.broken.com/', '+---OK--- http://www.set.com/', '+---OK--- http://www.one.com/'], 'Getting links from: https://www.bar.com/': ['+-BROKEN- http://www.broken.com/']}
answered Dec 31 '18 at 12:36


RomanPerekhrestRomanPerekhrest
55.7k32253
55.7k32253
add a comment |
add a comment |
This is a one-liner:
{k: [e for e in v if val not in e] for k, v in mydict.items() if any([val not in e for e in v])}
The expected output:
Out[1]: {
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
]
}
1
The one-liner works, of course, but hides the fact you are explicitly checking for'is'
in your values twice. That double string checking isn't required, better to use 2 lines.
– jpp
Dec 31 '18 at 12:35
1
@jpp in a 2 liner, on the other hand, you create an additional intermediary dict object, which is also not strictly required.
– jojo
Dec 31 '18 at 12:38
Except the expensive part isn't checking if a list is non-empty, it's checking if any strings within a list contain a substring. I suggest timing this for a larger input to satisfy yourself this is the case.
– jpp
Dec 31 '18 at 12:40
add a comment |
This is a one-liner:
{k: [e for e in v if val not in e] for k, v in mydict.items() if any([val not in e for e in v])}
The expected output:
Out[1]: {
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
]
}
1
The one-liner works, of course, but hides the fact you are explicitly checking for'is'
in your values twice. That double string checking isn't required, better to use 2 lines.
– jpp
Dec 31 '18 at 12:35
1
@jpp in a 2 liner, on the other hand, you create an additional intermediary dict object, which is also not strictly required.
– jojo
Dec 31 '18 at 12:38
Except the expensive part isn't checking if a list is non-empty, it's checking if any strings within a list contain a substring. I suggest timing this for a larger input to satisfy yourself this is the case.
– jpp
Dec 31 '18 at 12:40
add a comment |
This is a one-liner:
{k: [e for e in v if val not in e] for k, v in mydict.items() if any([val not in e for e in v])}
The expected output:
Out[1]: {
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
]
}
This is a one-liner:
{k: [e for e in v if val not in e] for k, v in mydict.items() if any([val not in e for e in v])}
The expected output:
Out[1]: {
'Getting links from: https://www.bar.com/':
[
'+-BROKEN- http://www.broken.com/'
],
'Getting links from: https://www.foo.com/':
[
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'
]
}
edited Dec 31 '18 at 14:27
answered Dec 31 '18 at 12:26
jojojojo
3,66122244
3,66122244
1
The one-liner works, of course, but hides the fact you are explicitly checking for'is'
in your values twice. That double string checking isn't required, better to use 2 lines.
– jpp
Dec 31 '18 at 12:35
1
@jpp in a 2 liner, on the other hand, you create an additional intermediary dict object, which is also not strictly required.
– jojo
Dec 31 '18 at 12:38
Except the expensive part isn't checking if a list is non-empty, it's checking if any strings within a list contain a substring. I suggest timing this for a larger input to satisfy yourself this is the case.
– jpp
Dec 31 '18 at 12:40
add a comment |
1
The one-liner works, of course, but hides the fact you are explicitly checking for'is'
in your values twice. That double string checking isn't required, better to use 2 lines.
– jpp
Dec 31 '18 at 12:35
1
@jpp in a 2 liner, on the other hand, you create an additional intermediary dict object, which is also not strictly required.
– jojo
Dec 31 '18 at 12:38
Except the expensive part isn't checking if a list is non-empty, it's checking if any strings within a list contain a substring. I suggest timing this for a larger input to satisfy yourself this is the case.
– jpp
Dec 31 '18 at 12:40
1
1
The one-liner works, of course, but hides the fact you are explicitly checking for
'is'
in your values twice. That double string checking isn't required, better to use 2 lines.– jpp
Dec 31 '18 at 12:35
The one-liner works, of course, but hides the fact you are explicitly checking for
'is'
in your values twice. That double string checking isn't required, better to use 2 lines.– jpp
Dec 31 '18 at 12:35
1
1
@jpp in a 2 liner, on the other hand, you create an additional intermediary dict object, which is also not strictly required.
– jojo
Dec 31 '18 at 12:38
@jpp in a 2 liner, on the other hand, you create an additional intermediary dict object, which is also not strictly required.
– jojo
Dec 31 '18 at 12:38
Except the expensive part isn't checking if a list is non-empty, it's checking if any strings within a list contain a substring. I suggest timing this for a larger input to satisfy yourself this is the case.
– jpp
Dec 31 '18 at 12:40
Except the expensive part isn't checking if a list is non-empty, it's checking if any strings within a list contain a substring. I suggest timing this for a larger input to satisfy yourself this is the case.
– jpp
Dec 31 '18 at 12:40
add a comment |
Using dict comprehension
, you can try the following:
import re
val = 'is'
# step 1 - remove line having is
mydict = {k:[re.sub(r'.*is*.', '', x) for x in v] for k,v in mydict.items()}
# filtering out keys if there is no value - if needed
mydict = {k:v for k,v in mydict.items() if len(v) > 0}
print(mydict)
{'Getting links from: https://www.foo.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'],
'Getting links from: https://www.bar.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['com/', 'com/']}
add a comment |
Using dict comprehension
, you can try the following:
import re
val = 'is'
# step 1 - remove line having is
mydict = {k:[re.sub(r'.*is*.', '', x) for x in v] for k,v in mydict.items()}
# filtering out keys if there is no value - if needed
mydict = {k:v for k,v in mydict.items() if len(v) > 0}
print(mydict)
{'Getting links from: https://www.foo.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'],
'Getting links from: https://www.bar.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['com/', 'com/']}
add a comment |
Using dict comprehension
, you can try the following:
import re
val = 'is'
# step 1 - remove line having is
mydict = {k:[re.sub(r'.*is*.', '', x) for x in v] for k,v in mydict.items()}
# filtering out keys if there is no value - if needed
mydict = {k:v for k,v in mydict.items() if len(v) > 0}
print(mydict)
{'Getting links from: https://www.foo.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'],
'Getting links from: https://www.bar.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['com/', 'com/']}
Using dict comprehension
, you can try the following:
import re
val = 'is'
# step 1 - remove line having is
mydict = {k:[re.sub(r'.*is*.', '', x) for x in v] for k,v in mydict.items()}
# filtering out keys if there is no value - if needed
mydict = {k:v for k,v in mydict.items() if len(v) > 0}
print(mydict)
{'Getting links from: https://www.foo.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/'],
'Getting links from: https://www.bar.com/': ['com/',
'com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['com/', 'com/']}
answered Dec 31 '18 at 12:25


YOLOYOLO
5,4721424
5,4721424
add a comment |
add a comment |
There are a couple of ways you could do it. One using regex and one without.
if you're not familiar with regex you could try this:
for key, value in mydict.items():
if val in value:
mydict.pop(key)
output would be:
mydict = {'Getting links from: https://www.bar.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'],
'Getting links from: https://www.foo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/']}
This do not match the expected output
– Daniel Mesejo
Dec 31 '18 at 12:19
The pop() method removes the specified item from the dictionary. The value of the removed item is the return value of the pop() method
– Brandon Bailey
Dec 31 '18 at 12:20
I'm referring to the fact that the OP include an expected output, what you wrote as the output in your answer do not match the OP expected output
– Daniel Mesejo
Dec 31 '18 at 12:21
its a starting point, you could refine it to strip away what you want
– Brandon Bailey
Dec 31 '18 at 12:23
add a comment |
There are a couple of ways you could do it. One using regex and one without.
if you're not familiar with regex you could try this:
for key, value in mydict.items():
if val in value:
mydict.pop(key)
output would be:
mydict = {'Getting links from: https://www.bar.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'],
'Getting links from: https://www.foo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/']}
This do not match the expected output
– Daniel Mesejo
Dec 31 '18 at 12:19
The pop() method removes the specified item from the dictionary. The value of the removed item is the return value of the pop() method
– Brandon Bailey
Dec 31 '18 at 12:20
I'm referring to the fact that the OP include an expected output, what you wrote as the output in your answer do not match the OP expected output
– Daniel Mesejo
Dec 31 '18 at 12:21
its a starting point, you could refine it to strip away what you want
– Brandon Bailey
Dec 31 '18 at 12:23
add a comment |
There are a couple of ways you could do it. One using regex and one without.
if you're not familiar with regex you could try this:
for key, value in mydict.items():
if val in value:
mydict.pop(key)
output would be:
mydict = {'Getting links from: https://www.bar.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'],
'Getting links from: https://www.foo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/']}
There are a couple of ways you could do it. One using regex and one without.
if you're not familiar with regex you could try this:
for key, value in mydict.items():
if val in value:
mydict.pop(key)
output would be:
mydict = {'Getting links from: https://www.bar.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/'],
'Getting links from: https://www.boo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/'],
'Getting links from: https://www.foo.com/': ['+---OK--- http://www.this.com/',
'+---OK--- http://www.is.com/',
'+-BROKEN- http://www.broken.com/',
'+---OK--- http://www.set.com/',
'+---OK--- http://www.one.com/']}
edited Dec 31 '18 at 14:08


Aaron_ab
1,0531823
1,0531823
answered Dec 31 '18 at 12:18


Brandon BaileyBrandon Bailey
1477
1477
This do not match the expected output
– Daniel Mesejo
Dec 31 '18 at 12:19
The pop() method removes the specified item from the dictionary. The value of the removed item is the return value of the pop() method
– Brandon Bailey
Dec 31 '18 at 12:20
I'm referring to the fact that the OP include an expected output, what you wrote as the output in your answer do not match the OP expected output
– Daniel Mesejo
Dec 31 '18 at 12:21
its a starting point, you could refine it to strip away what you want
– Brandon Bailey
Dec 31 '18 at 12:23
add a comment |
This do not match the expected output
– Daniel Mesejo
Dec 31 '18 at 12:19
The pop() method removes the specified item from the dictionary. The value of the removed item is the return value of the pop() method
– Brandon Bailey
Dec 31 '18 at 12:20
I'm referring to the fact that the OP include an expected output, what you wrote as the output in your answer do not match the OP expected output
– Daniel Mesejo
Dec 31 '18 at 12:21
its a starting point, you could refine it to strip away what you want
– Brandon Bailey
Dec 31 '18 at 12:23
This do not match the expected output
– Daniel Mesejo
Dec 31 '18 at 12:19
This do not match the expected output
– Daniel Mesejo
Dec 31 '18 at 12:19
The pop() method removes the specified item from the dictionary. The value of the removed item is the return value of the pop() method
– Brandon Bailey
Dec 31 '18 at 12:20
The pop() method removes the specified item from the dictionary. The value of the removed item is the return value of the pop() method
– Brandon Bailey
Dec 31 '18 at 12:20
I'm referring to the fact that the OP include an expected output, what you wrote as the output in your answer do not match the OP expected output
– Daniel Mesejo
Dec 31 '18 at 12:21
I'm referring to the fact that the OP include an expected output, what you wrote as the output in your answer do not match the OP expected output
– Daniel Mesejo
Dec 31 '18 at 12:21
its a starting point, you could refine it to strip away what you want
– Brandon Bailey
Dec 31 '18 at 12:23
its a starting point, you could refine it to strip away what you want
– Brandon Bailey
Dec 31 '18 at 12:23
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987387%2fhow-to-loop-through-a-dictionary-to-remove-values-that-match-a-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WBg,ceYZJ,aiPAU 7pqWzc3d5cs9oDt0S9OJG fKqkWgx DlL6VX ykPhDjyhFN,9R
2
you should not
del
an item of a dictionary within a loop that runs through this dictionary. You could use dict-comprehension to solve your issue.– jojo
Dec 31 '18 at 12:33