Python argparse skip if not int
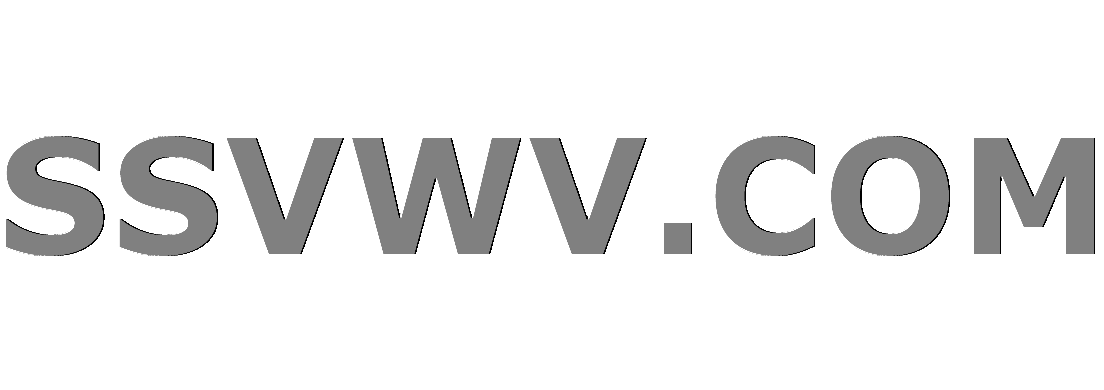
Multi tool use
I would like to have 3 optional positional arguments (int
, int
, then str
).
What I want:
$ ./args.py 0 100 vid
start=0
end=100
video='vid'
$ ./args.py 0 100
start=0
end=100
video=None
$ ./args.py 0
start=0
end=None
video=None
$ ./args.py vid
start=None
end=None
video='vid'
$ ./args.py
start=None
end=None
video=None
What I have tried:
#!/usr/bin/python3
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('start', type=int, nargs='?')
parser.add_argument('end', type=int, nargs='?')
parser.add_argument('video', type=str, nargs='?')
print(parser.parse_args())
The problem with that code is:
$ ./args.py vid
usage: args.py [-h] [start] [end] [video]
args.py: error: argument start: invalid int value: 'vid'
argparse knows that the value 'vid' is not an integer, so I would like it to "skip" the two first arguments start
and end
since they don't match.
When I make the video
argument mandatory, it works a bit better:
parser.add_argument('start', type=int, nargs='?')
parser.add_argument('end', type=int, nargs='?')
parser.add_argument('video', type=str)
Demo:
# Fine!
$ ./args.py 0 100 vid
start=0
end=100
video='vid'
# Fine!
$ ./args.py vid
start=None
end=None
video='vid'
# Not fine
./args.py
usage: args.py [-h] [start] [end] video
args.py: error: the following arguments are required: video
python argparse
add a comment |
I would like to have 3 optional positional arguments (int
, int
, then str
).
What I want:
$ ./args.py 0 100 vid
start=0
end=100
video='vid'
$ ./args.py 0 100
start=0
end=100
video=None
$ ./args.py 0
start=0
end=None
video=None
$ ./args.py vid
start=None
end=None
video='vid'
$ ./args.py
start=None
end=None
video=None
What I have tried:
#!/usr/bin/python3
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('start', type=int, nargs='?')
parser.add_argument('end', type=int, nargs='?')
parser.add_argument('video', type=str, nargs='?')
print(parser.parse_args())
The problem with that code is:
$ ./args.py vid
usage: args.py [-h] [start] [end] [video]
args.py: error: argument start: invalid int value: 'vid'
argparse knows that the value 'vid' is not an integer, so I would like it to "skip" the two first arguments start
and end
since they don't match.
When I make the video
argument mandatory, it works a bit better:
parser.add_argument('start', type=int, nargs='?')
parser.add_argument('end', type=int, nargs='?')
parser.add_argument('video', type=str)
Demo:
# Fine!
$ ./args.py 0 100 vid
start=0
end=100
video='vid'
# Fine!
$ ./args.py vid
start=None
end=None
video='vid'
# Not fine
./args.py
usage: args.py [-h] [start] [end] video
args.py: error: the following arguments are required: video
python argparse
did you try setting a default value for video?
– miraculixx
2 days ago
argparse
raises an error when thetype
doesn't match; it doesn't skip that argument or go on to try another. Positional values like this are assigned strictly on position, not on value.
– hpaulj
2 days ago
add a comment |
I would like to have 3 optional positional arguments (int
, int
, then str
).
What I want:
$ ./args.py 0 100 vid
start=0
end=100
video='vid'
$ ./args.py 0 100
start=0
end=100
video=None
$ ./args.py 0
start=0
end=None
video=None
$ ./args.py vid
start=None
end=None
video='vid'
$ ./args.py
start=None
end=None
video=None
What I have tried:
#!/usr/bin/python3
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('start', type=int, nargs='?')
parser.add_argument('end', type=int, nargs='?')
parser.add_argument('video', type=str, nargs='?')
print(parser.parse_args())
The problem with that code is:
$ ./args.py vid
usage: args.py [-h] [start] [end] [video]
args.py: error: argument start: invalid int value: 'vid'
argparse knows that the value 'vid' is not an integer, so I would like it to "skip" the two first arguments start
and end
since they don't match.
When I make the video
argument mandatory, it works a bit better:
parser.add_argument('start', type=int, nargs='?')
parser.add_argument('end', type=int, nargs='?')
parser.add_argument('video', type=str)
Demo:
# Fine!
$ ./args.py 0 100 vid
start=0
end=100
video='vid'
# Fine!
$ ./args.py vid
start=None
end=None
video='vid'
# Not fine
./args.py
usage: args.py [-h] [start] [end] video
args.py: error: the following arguments are required: video
python argparse
I would like to have 3 optional positional arguments (int
, int
, then str
).
What I want:
$ ./args.py 0 100 vid
start=0
end=100
video='vid'
$ ./args.py 0 100
start=0
end=100
video=None
$ ./args.py 0
start=0
end=None
video=None
$ ./args.py vid
start=None
end=None
video='vid'
$ ./args.py
start=None
end=None
video=None
What I have tried:
#!/usr/bin/python3
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('start', type=int, nargs='?')
parser.add_argument('end', type=int, nargs='?')
parser.add_argument('video', type=str, nargs='?')
print(parser.parse_args())
The problem with that code is:
$ ./args.py vid
usage: args.py [-h] [start] [end] [video]
args.py: error: argument start: invalid int value: 'vid'
argparse knows that the value 'vid' is not an integer, so I would like it to "skip" the two first arguments start
and end
since they don't match.
When I make the video
argument mandatory, it works a bit better:
parser.add_argument('start', type=int, nargs='?')
parser.add_argument('end', type=int, nargs='?')
parser.add_argument('video', type=str)
Demo:
# Fine!
$ ./args.py 0 100 vid
start=0
end=100
video='vid'
# Fine!
$ ./args.py vid
start=None
end=None
video='vid'
# Not fine
./args.py
usage: args.py [-h] [start] [end] video
args.py: error: the following arguments are required: video
python argparse
python argparse
asked 2 days ago
Bilow
856913
856913
did you try setting a default value for video?
– miraculixx
2 days ago
argparse
raises an error when thetype
doesn't match; it doesn't skip that argument or go on to try another. Positional values like this are assigned strictly on position, not on value.
– hpaulj
2 days ago
add a comment |
did you try setting a default value for video?
– miraculixx
2 days ago
argparse
raises an error when thetype
doesn't match; it doesn't skip that argument or go on to try another. Positional values like this are assigned strictly on position, not on value.
– hpaulj
2 days ago
did you try setting a default value for video?
– miraculixx
2 days ago
did you try setting a default value for video?
– miraculixx
2 days ago
argparse
raises an error when the type
doesn't match; it doesn't skip that argument or go on to try another. Positional values like this are assigned strictly on position, not on value.– hpaulj
2 days ago
argparse
raises an error when the type
doesn't match; it doesn't skip that argument or go on to try another. Positional values like this are assigned strictly on position, not on value.– hpaulj
2 days ago
add a comment |
2 Answers
2
active
oldest
votes
There is no built-in way to do this cleanly, I think. Thus, you'll just have to gather all of the options into a list and parse them out yourself.
e.g.
parser.add_argument('start_end_video', nargs='*')
args=parser.parse_args()
if len(args.start_end_video) == 1:
video = args.start_end_video
elif len(args.start_end_video) == 3:
start, end, video = args.start_end_video
etc.
add a comment |
I think you should add what your arguments are called:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-s', '--start', type=int)
parser.add_argument('-e', '--end', type=int)
parser.add_argument('-v', '--video', type=str)
args = parser.parse_args()
for arg in vars(args):
print(arg, '=', getattr(args, arg))
That way you can specify the arguments and no confusion can occur:
$ ./args.py -s 0 -e 100 -v vid
start = 0
end = 100
video = vid
$ ./args.py -s 0 -e 100
start = 0
end = 100
video = None
$ ./args.py -s 0
start = 0
end = None
video = None
$ ./args.py -v vid
start = None
end = None
video = vid
$ ./args.py
start = None
end = None
video = None
$ ./args.py vid
usage: args.py [-h] [-s START] [-e END] [-v VIDEO]
args.py: error: unrecognized arguments: vid
Note: I have included short aliases in the arguments above. E.g. You can call -s
instead of --start
.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53944971%2fpython-argparse-skip-if-not-int%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is no built-in way to do this cleanly, I think. Thus, you'll just have to gather all of the options into a list and parse them out yourself.
e.g.
parser.add_argument('start_end_video', nargs='*')
args=parser.parse_args()
if len(args.start_end_video) == 1:
video = args.start_end_video
elif len(args.start_end_video) == 3:
start, end, video = args.start_end_video
etc.
add a comment |
There is no built-in way to do this cleanly, I think. Thus, you'll just have to gather all of the options into a list and parse them out yourself.
e.g.
parser.add_argument('start_end_video', nargs='*')
args=parser.parse_args()
if len(args.start_end_video) == 1:
video = args.start_end_video
elif len(args.start_end_video) == 3:
start, end, video = args.start_end_video
etc.
add a comment |
There is no built-in way to do this cleanly, I think. Thus, you'll just have to gather all of the options into a list and parse them out yourself.
e.g.
parser.add_argument('start_end_video', nargs='*')
args=parser.parse_args()
if len(args.start_end_video) == 1:
video = args.start_end_video
elif len(args.start_end_video) == 3:
start, end, video = args.start_end_video
etc.
There is no built-in way to do this cleanly, I think. Thus, you'll just have to gather all of the options into a list and parse them out yourself.
e.g.
parser.add_argument('start_end_video', nargs='*')
args=parser.parse_args()
if len(args.start_end_video) == 1:
video = args.start_end_video
elif len(args.start_end_video) == 3:
start, end, video = args.start_end_video
etc.
answered 2 days ago


Scott
1,729824
1,729824
add a comment |
add a comment |
I think you should add what your arguments are called:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-s', '--start', type=int)
parser.add_argument('-e', '--end', type=int)
parser.add_argument('-v', '--video', type=str)
args = parser.parse_args()
for arg in vars(args):
print(arg, '=', getattr(args, arg))
That way you can specify the arguments and no confusion can occur:
$ ./args.py -s 0 -e 100 -v vid
start = 0
end = 100
video = vid
$ ./args.py -s 0 -e 100
start = 0
end = 100
video = None
$ ./args.py -s 0
start = 0
end = None
video = None
$ ./args.py -v vid
start = None
end = None
video = vid
$ ./args.py
start = None
end = None
video = None
$ ./args.py vid
usage: args.py [-h] [-s START] [-e END] [-v VIDEO]
args.py: error: unrecognized arguments: vid
Note: I have included short aliases in the arguments above. E.g. You can call -s
instead of --start
.
add a comment |
I think you should add what your arguments are called:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-s', '--start', type=int)
parser.add_argument('-e', '--end', type=int)
parser.add_argument('-v', '--video', type=str)
args = parser.parse_args()
for arg in vars(args):
print(arg, '=', getattr(args, arg))
That way you can specify the arguments and no confusion can occur:
$ ./args.py -s 0 -e 100 -v vid
start = 0
end = 100
video = vid
$ ./args.py -s 0 -e 100
start = 0
end = 100
video = None
$ ./args.py -s 0
start = 0
end = None
video = None
$ ./args.py -v vid
start = None
end = None
video = vid
$ ./args.py
start = None
end = None
video = None
$ ./args.py vid
usage: args.py [-h] [-s START] [-e END] [-v VIDEO]
args.py: error: unrecognized arguments: vid
Note: I have included short aliases in the arguments above. E.g. You can call -s
instead of --start
.
add a comment |
I think you should add what your arguments are called:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-s', '--start', type=int)
parser.add_argument('-e', '--end', type=int)
parser.add_argument('-v', '--video', type=str)
args = parser.parse_args()
for arg in vars(args):
print(arg, '=', getattr(args, arg))
That way you can specify the arguments and no confusion can occur:
$ ./args.py -s 0 -e 100 -v vid
start = 0
end = 100
video = vid
$ ./args.py -s 0 -e 100
start = 0
end = 100
video = None
$ ./args.py -s 0
start = 0
end = None
video = None
$ ./args.py -v vid
start = None
end = None
video = vid
$ ./args.py
start = None
end = None
video = None
$ ./args.py vid
usage: args.py [-h] [-s START] [-e END] [-v VIDEO]
args.py: error: unrecognized arguments: vid
Note: I have included short aliases in the arguments above. E.g. You can call -s
instead of --start
.
I think you should add what your arguments are called:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-s', '--start', type=int)
parser.add_argument('-e', '--end', type=int)
parser.add_argument('-v', '--video', type=str)
args = parser.parse_args()
for arg in vars(args):
print(arg, '=', getattr(args, arg))
That way you can specify the arguments and no confusion can occur:
$ ./args.py -s 0 -e 100 -v vid
start = 0
end = 100
video = vid
$ ./args.py -s 0 -e 100
start = 0
end = 100
video = None
$ ./args.py -s 0
start = 0
end = None
video = None
$ ./args.py -v vid
start = None
end = None
video = vid
$ ./args.py
start = None
end = None
video = None
$ ./args.py vid
usage: args.py [-h] [-s START] [-e END] [-v VIDEO]
args.py: error: unrecognized arguments: vid
Note: I have included short aliases in the arguments above. E.g. You can call -s
instead of --start
.
edited 2 days ago
answered 2 days ago


RoadRunner
10.3k31340
10.3k31340
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53944971%2fpython-argparse-skip-if-not-int%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YoiH8T8zkm2nhu,0oRpcAjiDY VHLqx0DU8C8
did you try setting a default value for video?
– miraculixx
2 days ago
argparse
raises an error when thetype
doesn't match; it doesn't skip that argument or go on to try another. Positional values like this are assigned strictly on position, not on value.– hpaulj
2 days ago