How to fix “cannot find function name” error in Angular
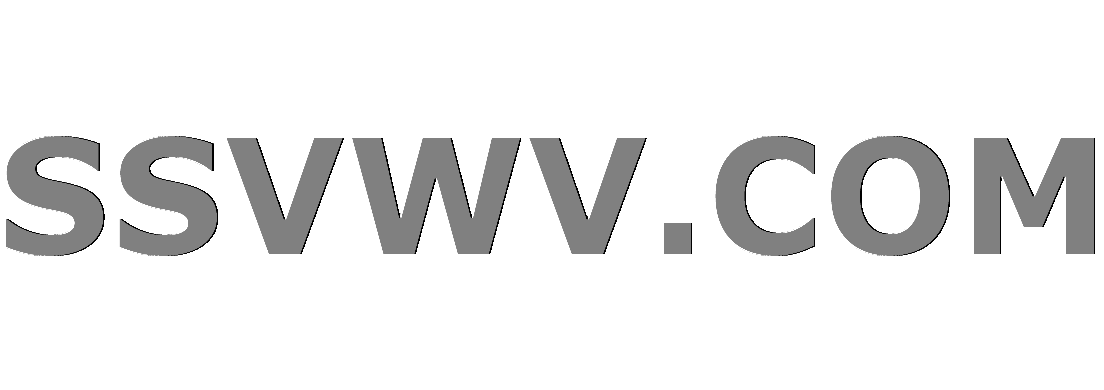
Multi tool use
I'm using Angular 6 to implement a google maps based app with google maps direction API. I used following initial code in my project. But when ng serve, it says Cannot find name 'calculateAndDisplayRoute'
.
I already tried with declare the function name 'calculateAndDisplayRoute' as commented above the class but seems not working either.
What is the problem here?
import { Component, OnInit } from '@angular/core';
declare var google: any;
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['../../resources/css/style.min.css', '../../resources/css/bt.min.css']
})
// declare var calculateAndDisplayRoute(directionsService, directionsDisplay) : any;
export class HomeComponent implements OnInit {
initMap() {
var directionsService = new google.maps.DirectionsService;
var directionsDisplay = new google.maps.DirectionsRenderer;
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 7,
center: {lat: 41.85, lng: -87.65}
});
directionsDisplay.setMap(map);
var onChangeHandler = function() {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
document.getElementById('start').addEventListener('change', onChangeHandler);
document.getElementById('end').addEventListener('change', onChangeHandler);
}
calculateAndDisplayRoute(directionsService, directionsDisplay) {
directionsService.route({
origin: (<HTMLInputElement>document.getElementById('start')).value,
destination: (<HTMLInputElement>document.getElementById('end')).value,
travelMode: 'DRIVING'
}, function(response, status) {
if (status === 'OK') {
directionsDisplay.setDirections(response);
}
else {
window.alert('Directions request failed due to ' + status);
}
});
}
constructor() {
}
ngOnInit() {
this.initMap();
}
}
angular google-maps
add a comment |
I'm using Angular 6 to implement a google maps based app with google maps direction API. I used following initial code in my project. But when ng serve, it says Cannot find name 'calculateAndDisplayRoute'
.
I already tried with declare the function name 'calculateAndDisplayRoute' as commented above the class but seems not working either.
What is the problem here?
import { Component, OnInit } from '@angular/core';
declare var google: any;
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['../../resources/css/style.min.css', '../../resources/css/bt.min.css']
})
// declare var calculateAndDisplayRoute(directionsService, directionsDisplay) : any;
export class HomeComponent implements OnInit {
initMap() {
var directionsService = new google.maps.DirectionsService;
var directionsDisplay = new google.maps.DirectionsRenderer;
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 7,
center: {lat: 41.85, lng: -87.65}
});
directionsDisplay.setMap(map);
var onChangeHandler = function() {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
document.getElementById('start').addEventListener('change', onChangeHandler);
document.getElementById('end').addEventListener('change', onChangeHandler);
}
calculateAndDisplayRoute(directionsService, directionsDisplay) {
directionsService.route({
origin: (<HTMLInputElement>document.getElementById('start')).value,
destination: (<HTMLInputElement>document.getElementById('end')).value,
travelMode: 'DRIVING'
}, function(response, status) {
if (status === 'OK') {
directionsDisplay.setDirections(response);
}
else {
window.alert('Directions request failed due to ' + status);
}
});
}
constructor() {
}
ngOnInit() {
this.initMap();
}
}
angular google-maps
1
You have to call the function asthis.calculateAndDisplayRoute(directionsService, directionsDisplay);
– Saddam Pojee
Dec 31 '18 at 12:23
add a comment |
I'm using Angular 6 to implement a google maps based app with google maps direction API. I used following initial code in my project. But when ng serve, it says Cannot find name 'calculateAndDisplayRoute'
.
I already tried with declare the function name 'calculateAndDisplayRoute' as commented above the class but seems not working either.
What is the problem here?
import { Component, OnInit } from '@angular/core';
declare var google: any;
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['../../resources/css/style.min.css', '../../resources/css/bt.min.css']
})
// declare var calculateAndDisplayRoute(directionsService, directionsDisplay) : any;
export class HomeComponent implements OnInit {
initMap() {
var directionsService = new google.maps.DirectionsService;
var directionsDisplay = new google.maps.DirectionsRenderer;
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 7,
center: {lat: 41.85, lng: -87.65}
});
directionsDisplay.setMap(map);
var onChangeHandler = function() {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
document.getElementById('start').addEventListener('change', onChangeHandler);
document.getElementById('end').addEventListener('change', onChangeHandler);
}
calculateAndDisplayRoute(directionsService, directionsDisplay) {
directionsService.route({
origin: (<HTMLInputElement>document.getElementById('start')).value,
destination: (<HTMLInputElement>document.getElementById('end')).value,
travelMode: 'DRIVING'
}, function(response, status) {
if (status === 'OK') {
directionsDisplay.setDirections(response);
}
else {
window.alert('Directions request failed due to ' + status);
}
});
}
constructor() {
}
ngOnInit() {
this.initMap();
}
}
angular google-maps
I'm using Angular 6 to implement a google maps based app with google maps direction API. I used following initial code in my project. But when ng serve, it says Cannot find name 'calculateAndDisplayRoute'
.
I already tried with declare the function name 'calculateAndDisplayRoute' as commented above the class but seems not working either.
What is the problem here?
import { Component, OnInit } from '@angular/core';
declare var google: any;
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['../../resources/css/style.min.css', '../../resources/css/bt.min.css']
})
// declare var calculateAndDisplayRoute(directionsService, directionsDisplay) : any;
export class HomeComponent implements OnInit {
initMap() {
var directionsService = new google.maps.DirectionsService;
var directionsDisplay = new google.maps.DirectionsRenderer;
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 7,
center: {lat: 41.85, lng: -87.65}
});
directionsDisplay.setMap(map);
var onChangeHandler = function() {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
document.getElementById('start').addEventListener('change', onChangeHandler);
document.getElementById('end').addEventListener('change', onChangeHandler);
}
calculateAndDisplayRoute(directionsService, directionsDisplay) {
directionsService.route({
origin: (<HTMLInputElement>document.getElementById('start')).value,
destination: (<HTMLInputElement>document.getElementById('end')).value,
travelMode: 'DRIVING'
}, function(response, status) {
if (status === 'OK') {
directionsDisplay.setDirections(response);
}
else {
window.alert('Directions request failed due to ' + status);
}
});
}
constructor() {
}
ngOnInit() {
this.initMap();
}
}
angular google-maps
angular google-maps
asked Dec 31 '18 at 11:48


David JohnsDavid Johns
10111
10111
1
You have to call the function asthis.calculateAndDisplayRoute(directionsService, directionsDisplay);
– Saddam Pojee
Dec 31 '18 at 12:23
add a comment |
1
You have to call the function asthis.calculateAndDisplayRoute(directionsService, directionsDisplay);
– Saddam Pojee
Dec 31 '18 at 12:23
1
1
You have to call the function as
this.calculateAndDisplayRoute(directionsService, directionsDisplay);
– Saddam Pojee
Dec 31 '18 at 12:23
You have to call the function as
this.calculateAndDisplayRoute(directionsService, directionsDisplay);
– Saddam Pojee
Dec 31 '18 at 12:23
add a comment |
1 Answer
1
active
oldest
votes
You should be using arrow function in typescript
var onChangeHandler = () => {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987129%2fhow-to-fix-cannot-find-function-name-error-in-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You should be using arrow function in typescript
var onChangeHandler = () => {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
add a comment |
You should be using arrow function in typescript
var onChangeHandler = () => {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
add a comment |
You should be using arrow function in typescript
var onChangeHandler = () => {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
You should be using arrow function in typescript
var onChangeHandler = () => {
calculateAndDisplayRoute(directionsService, directionsDisplay);
};
answered Dec 31 '18 at 12:11
Abhishek EkaanthAbhishek Ekaanth
1,1851023
1,1851023
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987129%2fhow-to-fix-cannot-find-function-name-error-in-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NDoESRFiiaBgcu90 7,FZ12Et9
1
You have to call the function as
this.calculateAndDisplayRoute(directionsService, directionsDisplay);
– Saddam Pojee
Dec 31 '18 at 12:23