How do I write the not/negate higher order function in swift?
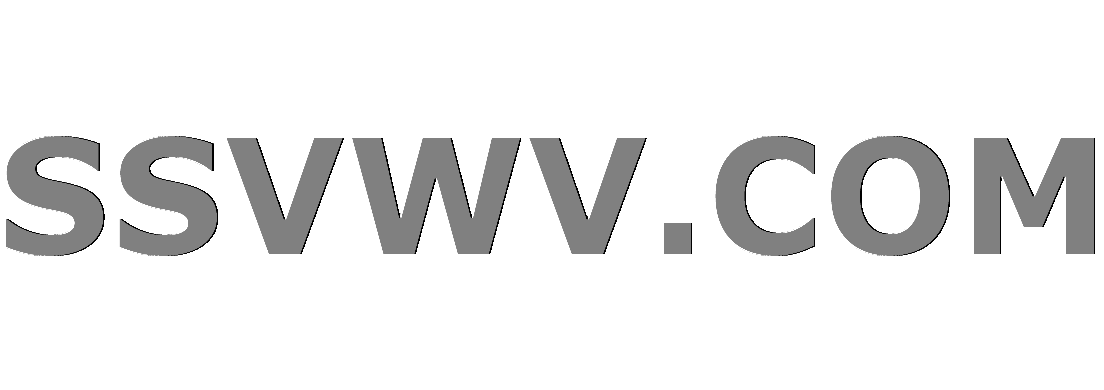
Multi tool use
I am a Javascripter and I love using the not/negate
function:
function not (predicateFunc) {
return function () {
return !predicateFunc.apply(this, arguments);
};
}
I am trying to do the same thing with swift:
func not <A> (_ f: @escaping (_ A: Any) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But I am getting errors like
generic parameter 'T' could not be inferred
and
Cannot convert value of type '(_) -> Bool' to expected argument type '(Any) -> Bool'
The outcome I am looking for is when I have a function like this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
I can just create a notEmpty
function like this:
let notEmpty = not(isEmpty)
And then use it like
notEmpty([3,4,5]) // true
What am I doing wrong?
swift functional-programming higher-order-functions
add a comment |
I am a Javascripter and I love using the not/negate
function:
function not (predicateFunc) {
return function () {
return !predicateFunc.apply(this, arguments);
};
}
I am trying to do the same thing with swift:
func not <A> (_ f: @escaping (_ A: Any) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But I am getting errors like
generic parameter 'T' could not be inferred
and
Cannot convert value of type '(_) -> Bool' to expected argument type '(Any) -> Bool'
The outcome I am looking for is when I have a function like this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
I can just create a notEmpty
function like this:
let notEmpty = not(isEmpty)
And then use it like
notEmpty([3,4,5]) // true
What am I doing wrong?
swift functional-programming higher-order-functions
add a comment |
I am a Javascripter and I love using the not/negate
function:
function not (predicateFunc) {
return function () {
return !predicateFunc.apply(this, arguments);
};
}
I am trying to do the same thing with swift:
func not <A> (_ f: @escaping (_ A: Any) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But I am getting errors like
generic parameter 'T' could not be inferred
and
Cannot convert value of type '(_) -> Bool' to expected argument type '(Any) -> Bool'
The outcome I am looking for is when I have a function like this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
I can just create a notEmpty
function like this:
let notEmpty = not(isEmpty)
And then use it like
notEmpty([3,4,5]) // true
What am I doing wrong?
swift functional-programming higher-order-functions
I am a Javascripter and I love using the not/negate
function:
function not (predicateFunc) {
return function () {
return !predicateFunc.apply(this, arguments);
};
}
I am trying to do the same thing with swift:
func not <A> (_ f: @escaping (_ A: Any) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But I am getting errors like
generic parameter 'T' could not be inferred
and
Cannot convert value of type '(_) -> Bool' to expected argument type '(Any) -> Bool'
The outcome I am looking for is when I have a function like this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
I can just create a notEmpty
function like this:
let notEmpty = not(isEmpty)
And then use it like
notEmpty([3,4,5]) // true
What am I doing wrong?
swift functional-programming higher-order-functions
swift functional-programming higher-order-functions
edited Dec 30 '18 at 4:51
Amit Erandole
asked Dec 30 '18 at 4:40
Amit ErandoleAmit Erandole
4,022164982
4,022164982
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You have two errors:
- You're using
A
as both the type parameter and as the argument name. - You're using
Any
as the argument type instead of using the type parameter (A
) as the argument type.
Try this:
func not<A>(predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
Note that in this version, I'm not using argument names for the predicate argument. You don't need an argument name in the declaration ((A) -> Bool
) and I'm using the anonymous argument name ($0
) in the body.
Okay, so you want to write this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
func not<A>(_ predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
let notEmpty = not(isEmpty)
And you get this error:
let notEmpty = not(isEmpty)
^ Generic parameter 'A' could not be inferred
The problem is that this code tries to create a generic closure, but Swift doesn't support generic closures.
That is to say, what would the type of nonEmpty
be? It would be something like:
<A: Collection>(A) -> Bool
and Swift doesn't support that.
nice! but I am getting a compiler error:generic parameter 'A' could not be inferred
– Amit Erandole
Dec 30 '18 at 6:25
1
@AmitErandole Obviously in declaration you have to tell the type to complier aslet notEmpty: ([Int])-> Bool = not(isEmpty)
– Kamran
Dec 30 '18 at 6:32
I've updated my answer.
– rob mayoff
Dec 30 '18 at 7:45
add a comment |
Using Any
is a code smell. You can just extend Collection directly:
extension Collection {
var notEmpty: Bool {
return !isEmpty
}
}
[1, 3, 5].notEmpty // true
Your functional definition of not
can work like this:
func not <A> (_ f: @escaping (_ a: A) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But to call it you would need something like this:
let arrayNotEmpty = not { (array: [Int]) in array.isEmpty }
arrayNotEmpty([1, 3, 5]) // true
Not exactly what I wanted. I would still like to work with a generic collection instead ofarray: [Int]
. Isn't there a better way?
– Amit Erandole
Dec 30 '18 at 6:22
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53975326%2fhow-do-i-write-the-not-negate-higher-order-function-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have two errors:
- You're using
A
as both the type parameter and as the argument name. - You're using
Any
as the argument type instead of using the type parameter (A
) as the argument type.
Try this:
func not<A>(predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
Note that in this version, I'm not using argument names for the predicate argument. You don't need an argument name in the declaration ((A) -> Bool
) and I'm using the anonymous argument name ($0
) in the body.
Okay, so you want to write this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
func not<A>(_ predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
let notEmpty = not(isEmpty)
And you get this error:
let notEmpty = not(isEmpty)
^ Generic parameter 'A' could not be inferred
The problem is that this code tries to create a generic closure, but Swift doesn't support generic closures.
That is to say, what would the type of nonEmpty
be? It would be something like:
<A: Collection>(A) -> Bool
and Swift doesn't support that.
nice! but I am getting a compiler error:generic parameter 'A' could not be inferred
– Amit Erandole
Dec 30 '18 at 6:25
1
@AmitErandole Obviously in declaration you have to tell the type to complier aslet notEmpty: ([Int])-> Bool = not(isEmpty)
– Kamran
Dec 30 '18 at 6:32
I've updated my answer.
– rob mayoff
Dec 30 '18 at 7:45
add a comment |
You have two errors:
- You're using
A
as both the type parameter and as the argument name. - You're using
Any
as the argument type instead of using the type parameter (A
) as the argument type.
Try this:
func not<A>(predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
Note that in this version, I'm not using argument names for the predicate argument. You don't need an argument name in the declaration ((A) -> Bool
) and I'm using the anonymous argument name ($0
) in the body.
Okay, so you want to write this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
func not<A>(_ predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
let notEmpty = not(isEmpty)
And you get this error:
let notEmpty = not(isEmpty)
^ Generic parameter 'A' could not be inferred
The problem is that this code tries to create a generic closure, but Swift doesn't support generic closures.
That is to say, what would the type of nonEmpty
be? It would be something like:
<A: Collection>(A) -> Bool
and Swift doesn't support that.
nice! but I am getting a compiler error:generic parameter 'A' could not be inferred
– Amit Erandole
Dec 30 '18 at 6:25
1
@AmitErandole Obviously in declaration you have to tell the type to complier aslet notEmpty: ([Int])-> Bool = not(isEmpty)
– Kamran
Dec 30 '18 at 6:32
I've updated my answer.
– rob mayoff
Dec 30 '18 at 7:45
add a comment |
You have two errors:
- You're using
A
as both the type parameter and as the argument name. - You're using
Any
as the argument type instead of using the type parameter (A
) as the argument type.
Try this:
func not<A>(predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
Note that in this version, I'm not using argument names for the predicate argument. You don't need an argument name in the declaration ((A) -> Bool
) and I'm using the anonymous argument name ($0
) in the body.
Okay, so you want to write this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
func not<A>(_ predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
let notEmpty = not(isEmpty)
And you get this error:
let notEmpty = not(isEmpty)
^ Generic parameter 'A' could not be inferred
The problem is that this code tries to create a generic closure, but Swift doesn't support generic closures.
That is to say, what would the type of nonEmpty
be? It would be something like:
<A: Collection>(A) -> Bool
and Swift doesn't support that.
You have two errors:
- You're using
A
as both the type parameter and as the argument name. - You're using
Any
as the argument type instead of using the type parameter (A
) as the argument type.
Try this:
func not<A>(predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
Note that in this version, I'm not using argument names for the predicate argument. You don't need an argument name in the declaration ((A) -> Bool
) and I'm using the anonymous argument name ($0
) in the body.
Okay, so you want to write this:
func isEmpty<T: Collection>(collection: T) -> Bool {
return collection.count == 0
}
func not<A>(_ predicate: @escaping (A) -> Bool) -> (A) -> Bool {
return { !predicate($0) }
}
let notEmpty = not(isEmpty)
And you get this error:
let notEmpty = not(isEmpty)
^ Generic parameter 'A' could not be inferred
The problem is that this code tries to create a generic closure, but Swift doesn't support generic closures.
That is to say, what would the type of nonEmpty
be? It would be something like:
<A: Collection>(A) -> Bool
and Swift doesn't support that.
edited Dec 30 '18 at 7:45
answered Dec 30 '18 at 6:22
rob mayoffrob mayoff
292k41591640
292k41591640
nice! but I am getting a compiler error:generic parameter 'A' could not be inferred
– Amit Erandole
Dec 30 '18 at 6:25
1
@AmitErandole Obviously in declaration you have to tell the type to complier aslet notEmpty: ([Int])-> Bool = not(isEmpty)
– Kamran
Dec 30 '18 at 6:32
I've updated my answer.
– rob mayoff
Dec 30 '18 at 7:45
add a comment |
nice! but I am getting a compiler error:generic parameter 'A' could not be inferred
– Amit Erandole
Dec 30 '18 at 6:25
1
@AmitErandole Obviously in declaration you have to tell the type to complier aslet notEmpty: ([Int])-> Bool = not(isEmpty)
– Kamran
Dec 30 '18 at 6:32
I've updated my answer.
– rob mayoff
Dec 30 '18 at 7:45
nice! but I am getting a compiler error:
generic parameter 'A' could not be inferred
– Amit Erandole
Dec 30 '18 at 6:25
nice! but I am getting a compiler error:
generic parameter 'A' could not be inferred
– Amit Erandole
Dec 30 '18 at 6:25
1
1
@AmitErandole Obviously in declaration you have to tell the type to complier as
let notEmpty: ([Int])-> Bool = not(isEmpty)
– Kamran
Dec 30 '18 at 6:32
@AmitErandole Obviously in declaration you have to tell the type to complier as
let notEmpty: ([Int])-> Bool = not(isEmpty)
– Kamran
Dec 30 '18 at 6:32
I've updated my answer.
– rob mayoff
Dec 30 '18 at 7:45
I've updated my answer.
– rob mayoff
Dec 30 '18 at 7:45
add a comment |
Using Any
is a code smell. You can just extend Collection directly:
extension Collection {
var notEmpty: Bool {
return !isEmpty
}
}
[1, 3, 5].notEmpty // true
Your functional definition of not
can work like this:
func not <A> (_ f: @escaping (_ a: A) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But to call it you would need something like this:
let arrayNotEmpty = not { (array: [Int]) in array.isEmpty }
arrayNotEmpty([1, 3, 5]) // true
Not exactly what I wanted. I would still like to work with a generic collection instead ofarray: [Int]
. Isn't there a better way?
– Amit Erandole
Dec 30 '18 at 6:22
add a comment |
Using Any
is a code smell. You can just extend Collection directly:
extension Collection {
var notEmpty: Bool {
return !isEmpty
}
}
[1, 3, 5].notEmpty // true
Your functional definition of not
can work like this:
func not <A> (_ f: @escaping (_ a: A) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But to call it you would need something like this:
let arrayNotEmpty = not { (array: [Int]) in array.isEmpty }
arrayNotEmpty([1, 3, 5]) // true
Not exactly what I wanted. I would still like to work with a generic collection instead ofarray: [Int]
. Isn't there a better way?
– Amit Erandole
Dec 30 '18 at 6:22
add a comment |
Using Any
is a code smell. You can just extend Collection directly:
extension Collection {
var notEmpty: Bool {
return !isEmpty
}
}
[1, 3, 5].notEmpty // true
Your functional definition of not
can work like this:
func not <A> (_ f: @escaping (_ a: A) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But to call it you would need something like this:
let arrayNotEmpty = not { (array: [Int]) in array.isEmpty }
arrayNotEmpty([1, 3, 5]) // true
Using Any
is a code smell. You can just extend Collection directly:
extension Collection {
var notEmpty: Bool {
return !isEmpty
}
}
[1, 3, 5].notEmpty // true
Your functional definition of not
can work like this:
func not <A> (_ f: @escaping (_ a: A) -> Bool) -> (A) -> Bool {
return { a in !f(a) }
}
But to call it you would need something like this:
let arrayNotEmpty = not { (array: [Int]) in array.isEmpty }
arrayNotEmpty([1, 3, 5]) // true
answered Dec 30 '18 at 5:58
YonatYonat
1,0141122
1,0141122
Not exactly what I wanted. I would still like to work with a generic collection instead ofarray: [Int]
. Isn't there a better way?
– Amit Erandole
Dec 30 '18 at 6:22
add a comment |
Not exactly what I wanted. I would still like to work with a generic collection instead ofarray: [Int]
. Isn't there a better way?
– Amit Erandole
Dec 30 '18 at 6:22
Not exactly what I wanted. I would still like to work with a generic collection instead of
array: [Int]
. Isn't there a better way?– Amit Erandole
Dec 30 '18 at 6:22
Not exactly what I wanted. I would still like to work with a generic collection instead of
array: [Int]
. Isn't there a better way?– Amit Erandole
Dec 30 '18 at 6:22
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53975326%2fhow-do-i-write-the-not-negate-higher-order-function-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cRQy2aG,qhn hVBskPAbfCDr,OkT0mF1Bi0VgEIuqM5sCKU3FHHe VAuri