Callback function syntax in Swift
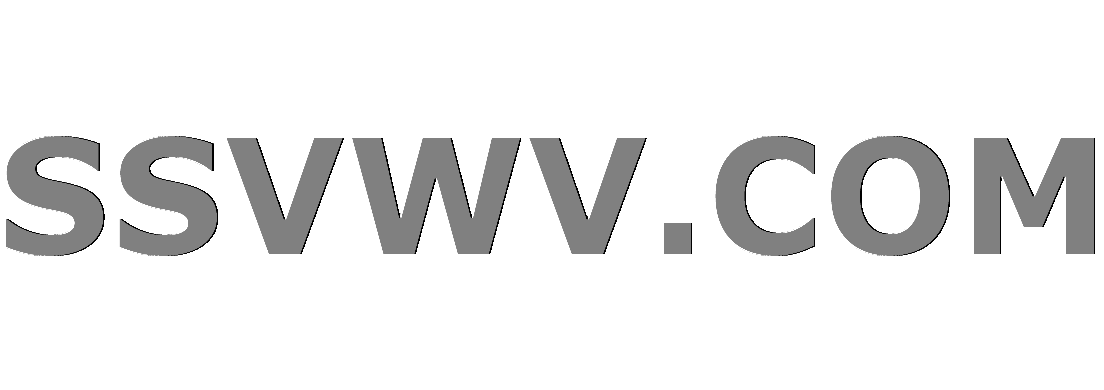
Multi tool use
I am attempting pass a function to another function and then have the passed function executed passing to it a variable.
Here is my code:
func showStandardPrompt(prompt:String,view: UIViewController,numberInput: Bool, callback: (()->(String))?) {
let alert = UIAlertController(title: "Input Data", message: prompt, preferredStyle: .Alert)
alert.addTextFieldWithConfigurationHandler { (textField) in
if numberInput {
textField.keyboardType = .NumberPad
}
}
let OKAction = UIAlertAction(title: "OK", style: .Default) { (action) in
let field = alert.textFields![0] as UITextField
callback?(field.text!)
}
alert.addAction(OKAction)
let CancelAction = UIAlertAction(title: "Cancel", style: .Default, handler: nil)
alert.addAction(CancelAction)
view.presentViewController(alert,animated: true, completion: nil)
}
The error I get is in
callback?(field.text!)
The error is "Cannot convert value type of 'String' to expected argument type '()'. I know it's a matter of syntax - just don't know what it should be.
ios swift function callback
add a comment |
I am attempting pass a function to another function and then have the passed function executed passing to it a variable.
Here is my code:
func showStandardPrompt(prompt:String,view: UIViewController,numberInput: Bool, callback: (()->(String))?) {
let alert = UIAlertController(title: "Input Data", message: prompt, preferredStyle: .Alert)
alert.addTextFieldWithConfigurationHandler { (textField) in
if numberInput {
textField.keyboardType = .NumberPad
}
}
let OKAction = UIAlertAction(title: "OK", style: .Default) { (action) in
let field = alert.textFields![0] as UITextField
callback?(field.text!)
}
alert.addAction(OKAction)
let CancelAction = UIAlertAction(title: "Cancel", style: .Default, handler: nil)
alert.addAction(CancelAction)
view.presentViewController(alert,animated: true, completion: nil)
}
The error I get is in
callback?(field.text!)
The error is "Cannot convert value type of 'String' to expected argument type '()'. I know it's a matter of syntax - just don't know what it should be.
ios swift function callback
Please try to indent your code properly, because it's difficult to understand otherwise. I fixed it for you this time.
– rob mayoff
Oct 22 '15 at 21:01
add a comment |
I am attempting pass a function to another function and then have the passed function executed passing to it a variable.
Here is my code:
func showStandardPrompt(prompt:String,view: UIViewController,numberInput: Bool, callback: (()->(String))?) {
let alert = UIAlertController(title: "Input Data", message: prompt, preferredStyle: .Alert)
alert.addTextFieldWithConfigurationHandler { (textField) in
if numberInput {
textField.keyboardType = .NumberPad
}
}
let OKAction = UIAlertAction(title: "OK", style: .Default) { (action) in
let field = alert.textFields![0] as UITextField
callback?(field.text!)
}
alert.addAction(OKAction)
let CancelAction = UIAlertAction(title: "Cancel", style: .Default, handler: nil)
alert.addAction(CancelAction)
view.presentViewController(alert,animated: true, completion: nil)
}
The error I get is in
callback?(field.text!)
The error is "Cannot convert value type of 'String' to expected argument type '()'. I know it's a matter of syntax - just don't know what it should be.
ios swift function callback
I am attempting pass a function to another function and then have the passed function executed passing to it a variable.
Here is my code:
func showStandardPrompt(prompt:String,view: UIViewController,numberInput: Bool, callback: (()->(String))?) {
let alert = UIAlertController(title: "Input Data", message: prompt, preferredStyle: .Alert)
alert.addTextFieldWithConfigurationHandler { (textField) in
if numberInput {
textField.keyboardType = .NumberPad
}
}
let OKAction = UIAlertAction(title: "OK", style: .Default) { (action) in
let field = alert.textFields![0] as UITextField
callback?(field.text!)
}
alert.addAction(OKAction)
let CancelAction = UIAlertAction(title: "Cancel", style: .Default, handler: nil)
alert.addAction(CancelAction)
view.presentViewController(alert,animated: true, completion: nil)
}
The error I get is in
callback?(field.text!)
The error is "Cannot convert value type of 'String' to expected argument type '()'. I know it's a matter of syntax - just don't know what it should be.
ios swift function callback
ios swift function callback
edited Oct 23 '15 at 0:00
rob mayoff
292k41591640
292k41591640
asked Oct 22 '15 at 20:54
Floyd ReslerFloyd Resler
63511230
63511230
Please try to indent your code properly, because it's difficult to understand otherwise. I fixed it for you this time.
– rob mayoff
Oct 22 '15 at 21:01
add a comment |
Please try to indent your code properly, because it's difficult to understand otherwise. I fixed it for you this time.
– rob mayoff
Oct 22 '15 at 21:01
Please try to indent your code properly, because it's difficult to understand otherwise. I fixed it for you this time.
– rob mayoff
Oct 22 '15 at 21:01
Please try to indent your code properly, because it's difficult to understand otherwise. I fixed it for you this time.
– rob mayoff
Oct 22 '15 at 21:01
add a comment |
5 Answers
5
active
oldest
votes
Rob's answer is correct, though I'd like to share an example of a simple working callback / completion handler, you can download an example project below and experiment with the getBoolValue
's input.
download example project
func getBoolValue(number : Int, completion: (result: Bool)->()) {
if number > 5 {
completion(result: true)
} else {
completion(result: false)
}
}
getBoolValue(8) { (result) -> () in
// do stuff with the result
print(result)
}
Important to understand:
(String)->() // takes a String returns void
()->(String) // takes void returns a String
That is not a playground.
– Price Ringo
Oct 22 '15 at 21:37
1
@PriceRingo thanks, I thought I uploaded a playground. It was a while ago
– Dan Beaulieu
Oct 22 '15 at 21:38
1
completion(result: number>5)
?
– GoZoner
Oct 23 '15 at 4:13
@GoZoner it's just a learning exercise
– Dan Beaulieu
Oct 23 '15 at 4:16
Thanks for the example. That' exactly what I needed to make my project work! And, for me, this is so much better than the old way of UIAlertView where I had to set a tag and then check the button index and tag in the delegate method.
– Floyd Resler
Oct 23 '15 at 12:52
|
show 1 more comment
You've declared callback
to take no arguments, and then you're trying to pass it an argument.
You specified type (()->(String))?
, which is an optional function that takes no arguments and returns a String
.
Perhaps you mean to specify ((String)->())?
, which is an optional function that takes a String
and returns nothing.
add a comment |
try below code updated for Swift 3
func getBoolValue(number : Int, completion: (Bool)->()) {
if number > 5 {
completion(true)
} else {
completion(false)
}
}
getBoolValue(number : 8, completion:{ result in
print(result)
})
add a comment |
In the parameters for showStandardPrompt
, you declare callback
to have the type ()->(String)
, which is a function which takes no parameters and returns a String
.
You then call it with field.text!
as a parameter, which obviously conflicts with the previously given type.
You need to correct the type given to callback
to take a String
argument and return nothing:
(String)->()
add a comment |
I use this way :
ViewController1
destination.callback = { (id) -> Void in
print("callback")
print(id)
}
ViewController2
var callback: ((_ id: Int) -> Void)?
callback?(example_id)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f33290564%2fcallback-function-syntax-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Rob's answer is correct, though I'd like to share an example of a simple working callback / completion handler, you can download an example project below and experiment with the getBoolValue
's input.
download example project
func getBoolValue(number : Int, completion: (result: Bool)->()) {
if number > 5 {
completion(result: true)
} else {
completion(result: false)
}
}
getBoolValue(8) { (result) -> () in
// do stuff with the result
print(result)
}
Important to understand:
(String)->() // takes a String returns void
()->(String) // takes void returns a String
That is not a playground.
– Price Ringo
Oct 22 '15 at 21:37
1
@PriceRingo thanks, I thought I uploaded a playground. It was a while ago
– Dan Beaulieu
Oct 22 '15 at 21:38
1
completion(result: number>5)
?
– GoZoner
Oct 23 '15 at 4:13
@GoZoner it's just a learning exercise
– Dan Beaulieu
Oct 23 '15 at 4:16
Thanks for the example. That' exactly what I needed to make my project work! And, for me, this is so much better than the old way of UIAlertView where I had to set a tag and then check the button index and tag in the delegate method.
– Floyd Resler
Oct 23 '15 at 12:52
|
show 1 more comment
Rob's answer is correct, though I'd like to share an example of a simple working callback / completion handler, you can download an example project below and experiment with the getBoolValue
's input.
download example project
func getBoolValue(number : Int, completion: (result: Bool)->()) {
if number > 5 {
completion(result: true)
} else {
completion(result: false)
}
}
getBoolValue(8) { (result) -> () in
// do stuff with the result
print(result)
}
Important to understand:
(String)->() // takes a String returns void
()->(String) // takes void returns a String
That is not a playground.
– Price Ringo
Oct 22 '15 at 21:37
1
@PriceRingo thanks, I thought I uploaded a playground. It was a while ago
– Dan Beaulieu
Oct 22 '15 at 21:38
1
completion(result: number>5)
?
– GoZoner
Oct 23 '15 at 4:13
@GoZoner it's just a learning exercise
– Dan Beaulieu
Oct 23 '15 at 4:16
Thanks for the example. That' exactly what I needed to make my project work! And, for me, this is so much better than the old way of UIAlertView where I had to set a tag and then check the button index and tag in the delegate method.
– Floyd Resler
Oct 23 '15 at 12:52
|
show 1 more comment
Rob's answer is correct, though I'd like to share an example of a simple working callback / completion handler, you can download an example project below and experiment with the getBoolValue
's input.
download example project
func getBoolValue(number : Int, completion: (result: Bool)->()) {
if number > 5 {
completion(result: true)
} else {
completion(result: false)
}
}
getBoolValue(8) { (result) -> () in
// do stuff with the result
print(result)
}
Important to understand:
(String)->() // takes a String returns void
()->(String) // takes void returns a String
Rob's answer is correct, though I'd like to share an example of a simple working callback / completion handler, you can download an example project below and experiment with the getBoolValue
's input.
download example project
func getBoolValue(number : Int, completion: (result: Bool)->()) {
if number > 5 {
completion(result: true)
} else {
completion(result: false)
}
}
getBoolValue(8) { (result) -> () in
// do stuff with the result
print(result)
}
Important to understand:
(String)->() // takes a String returns void
()->(String) // takes void returns a String
edited Oct 22 '15 at 23:56
answered Oct 22 '15 at 21:08


Dan BeaulieuDan Beaulieu
12.9k1079108
12.9k1079108
That is not a playground.
– Price Ringo
Oct 22 '15 at 21:37
1
@PriceRingo thanks, I thought I uploaded a playground. It was a while ago
– Dan Beaulieu
Oct 22 '15 at 21:38
1
completion(result: number>5)
?
– GoZoner
Oct 23 '15 at 4:13
@GoZoner it's just a learning exercise
– Dan Beaulieu
Oct 23 '15 at 4:16
Thanks for the example. That' exactly what I needed to make my project work! And, for me, this is so much better than the old way of UIAlertView where I had to set a tag and then check the button index and tag in the delegate method.
– Floyd Resler
Oct 23 '15 at 12:52
|
show 1 more comment
That is not a playground.
– Price Ringo
Oct 22 '15 at 21:37
1
@PriceRingo thanks, I thought I uploaded a playground. It was a while ago
– Dan Beaulieu
Oct 22 '15 at 21:38
1
completion(result: number>5)
?
– GoZoner
Oct 23 '15 at 4:13
@GoZoner it's just a learning exercise
– Dan Beaulieu
Oct 23 '15 at 4:16
Thanks for the example. That' exactly what I needed to make my project work! And, for me, this is so much better than the old way of UIAlertView where I had to set a tag and then check the button index and tag in the delegate method.
– Floyd Resler
Oct 23 '15 at 12:52
That is not a playground.
– Price Ringo
Oct 22 '15 at 21:37
That is not a playground.
– Price Ringo
Oct 22 '15 at 21:37
1
1
@PriceRingo thanks, I thought I uploaded a playground. It was a while ago
– Dan Beaulieu
Oct 22 '15 at 21:38
@PriceRingo thanks, I thought I uploaded a playground. It was a while ago
– Dan Beaulieu
Oct 22 '15 at 21:38
1
1
completion(result: number>5)
?– GoZoner
Oct 23 '15 at 4:13
completion(result: number>5)
?– GoZoner
Oct 23 '15 at 4:13
@GoZoner it's just a learning exercise
– Dan Beaulieu
Oct 23 '15 at 4:16
@GoZoner it's just a learning exercise
– Dan Beaulieu
Oct 23 '15 at 4:16
Thanks for the example. That' exactly what I needed to make my project work! And, for me, this is so much better than the old way of UIAlertView where I had to set a tag and then check the button index and tag in the delegate method.
– Floyd Resler
Oct 23 '15 at 12:52
Thanks for the example. That' exactly what I needed to make my project work! And, for me, this is so much better than the old way of UIAlertView where I had to set a tag and then check the button index and tag in the delegate method.
– Floyd Resler
Oct 23 '15 at 12:52
|
show 1 more comment
You've declared callback
to take no arguments, and then you're trying to pass it an argument.
You specified type (()->(String))?
, which is an optional function that takes no arguments and returns a String
.
Perhaps you mean to specify ((String)->())?
, which is an optional function that takes a String
and returns nothing.
add a comment |
You've declared callback
to take no arguments, and then you're trying to pass it an argument.
You specified type (()->(String))?
, which is an optional function that takes no arguments and returns a String
.
Perhaps you mean to specify ((String)->())?
, which is an optional function that takes a String
and returns nothing.
add a comment |
You've declared callback
to take no arguments, and then you're trying to pass it an argument.
You specified type (()->(String))?
, which is an optional function that takes no arguments and returns a String
.
Perhaps you mean to specify ((String)->())?
, which is an optional function that takes a String
and returns nothing.
You've declared callback
to take no arguments, and then you're trying to pass it an argument.
You specified type (()->(String))?
, which is an optional function that takes no arguments and returns a String
.
Perhaps you mean to specify ((String)->())?
, which is an optional function that takes a String
and returns nothing.
answered Oct 22 '15 at 21:03
rob mayoffrob mayoff
292k41591640
292k41591640
add a comment |
add a comment |
try below code updated for Swift 3
func getBoolValue(number : Int, completion: (Bool)->()) {
if number > 5 {
completion(true)
} else {
completion(false)
}
}
getBoolValue(number : 8, completion:{ result in
print(result)
})
add a comment |
try below code updated for Swift 3
func getBoolValue(number : Int, completion: (Bool)->()) {
if number > 5 {
completion(true)
} else {
completion(false)
}
}
getBoolValue(number : 8, completion:{ result in
print(result)
})
add a comment |
try below code updated for Swift 3
func getBoolValue(number : Int, completion: (Bool)->()) {
if number > 5 {
completion(true)
} else {
completion(false)
}
}
getBoolValue(number : 8, completion:{ result in
print(result)
})
try below code updated for Swift 3
func getBoolValue(number : Int, completion: (Bool)->()) {
if number > 5 {
completion(true)
} else {
completion(false)
}
}
getBoolValue(number : 8, completion:{ result in
print(result)
})
answered Nov 11 '17 at 16:53


Anand VermaAnand Verma
33938
33938
add a comment |
add a comment |
In the parameters for showStandardPrompt
, you declare callback
to have the type ()->(String)
, which is a function which takes no parameters and returns a String
.
You then call it with field.text!
as a parameter, which obviously conflicts with the previously given type.
You need to correct the type given to callback
to take a String
argument and return nothing:
(String)->()
add a comment |
In the parameters for showStandardPrompt
, you declare callback
to have the type ()->(String)
, which is a function which takes no parameters and returns a String
.
You then call it with field.text!
as a parameter, which obviously conflicts with the previously given type.
You need to correct the type given to callback
to take a String
argument and return nothing:
(String)->()
add a comment |
In the parameters for showStandardPrompt
, you declare callback
to have the type ()->(String)
, which is a function which takes no parameters and returns a String
.
You then call it with field.text!
as a parameter, which obviously conflicts with the previously given type.
You need to correct the type given to callback
to take a String
argument and return nothing:
(String)->()
In the parameters for showStandardPrompt
, you declare callback
to have the type ()->(String)
, which is a function which takes no parameters and returns a String
.
You then call it with field.text!
as a parameter, which obviously conflicts with the previously given type.
You need to correct the type given to callback
to take a String
argument and return nothing:
(String)->()
answered Oct 22 '15 at 21:06
stonesam92stonesam92
2,86711223
2,86711223
add a comment |
add a comment |
I use this way :
ViewController1
destination.callback = { (id) -> Void in
print("callback")
print(id)
}
ViewController2
var callback: ((_ id: Int) -> Void)?
callback?(example_id)
add a comment |
I use this way :
ViewController1
destination.callback = { (id) -> Void in
print("callback")
print(id)
}
ViewController2
var callback: ((_ id: Int) -> Void)?
callback?(example_id)
add a comment |
I use this way :
ViewController1
destination.callback = { (id) -> Void in
print("callback")
print(id)
}
ViewController2
var callback: ((_ id: Int) -> Void)?
callback?(example_id)
I use this way :
ViewController1
destination.callback = { (id) -> Void in
print("callback")
print(id)
}
ViewController2
var callback: ((_ id: Int) -> Void)?
callback?(example_id)
answered Dec 30 '18 at 4:40


Álvaro AgüeroÁlvaro Agüero
6115
6115
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f33290564%2fcallback-function-syntax-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sQzpytxIBp5CIkzQUw08BpeBNC,LKA1yX,xHZyoVWdH3fJPi q,vcul,Gff,T3lt4ZjIc0R cTUP1mBDth Um30vFuSOmtnPNBC,b8Q1 8O
Please try to indent your code properly, because it's difficult to understand otherwise. I fixed it for you this time.
– rob mayoff
Oct 22 '15 at 21:01