Button onClick Event Listener not working in Electron app
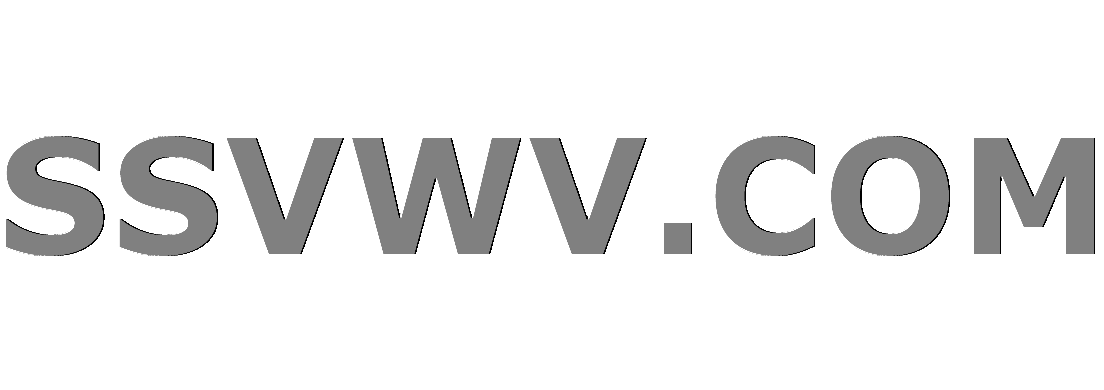
Multi tool use
Background:
I am using ElectronJS to make a game and I have a class for a shop which I named Shop.
It has a method that I call 'createItemElement' which takes an object named 'item' and creates an li element to be later added to a ul element. The code for this can be found below.
class Shop {
// ...
createItemElement(item) {
// Item Container
const li = document.createElement("li");
// Title
const title = document.createElement("h3");
title.textContent = item.title;
// Info
const info = document.createElement("p");
info.textContent = `Type: ${item.type}`;
// Add to Cart
const addButton = document.createElement("button");
addButton.textContent = "Add to Cart";
addButton.onclick = () => console.log("Adding to cart!");
li.appendChild(title);
li.appendChild(info);
li.appendChild(addButton);
return li;
}
// ...
}
Problem:
Interestingly, all of the HTML is correctly rendered and everything looks as it should, but the 'onclick' event just plain does not work.
Since all of these elements are in fact being rendered, it should be safe to assume that the code is indeed being run in the renderer process.
For some reason, however, the event is not being carried over to the app.
When I click the button in the app, nothing happens.
I checked the devTools and looked at the elements individually.
As expected, all of the elements were listed out correctly in element inspector, but when I inspected that 'addButton' button, there was no 'onclick' property to be seen.
Also, there are no errors in the console whatsoever, so that's nice (sarcasm).
And I am aware that there are many seemingly similar questions asked on StackOverflow, but none of the answers that I found have helped or applied to my situation.
It is extremely confusing as to why the elements are being rendered perfectly but the event listener is not working.
The full project can be found here and the file being excerpted below can be found here.
javascript html electron
add a comment |
Background:
I am using ElectronJS to make a game and I have a class for a shop which I named Shop.
It has a method that I call 'createItemElement' which takes an object named 'item' and creates an li element to be later added to a ul element. The code for this can be found below.
class Shop {
// ...
createItemElement(item) {
// Item Container
const li = document.createElement("li");
// Title
const title = document.createElement("h3");
title.textContent = item.title;
// Info
const info = document.createElement("p");
info.textContent = `Type: ${item.type}`;
// Add to Cart
const addButton = document.createElement("button");
addButton.textContent = "Add to Cart";
addButton.onclick = () => console.log("Adding to cart!");
li.appendChild(title);
li.appendChild(info);
li.appendChild(addButton);
return li;
}
// ...
}
Problem:
Interestingly, all of the HTML is correctly rendered and everything looks as it should, but the 'onclick' event just plain does not work.
Since all of these elements are in fact being rendered, it should be safe to assume that the code is indeed being run in the renderer process.
For some reason, however, the event is not being carried over to the app.
When I click the button in the app, nothing happens.
I checked the devTools and looked at the elements individually.
As expected, all of the elements were listed out correctly in element inspector, but when I inspected that 'addButton' button, there was no 'onclick' property to be seen.
Also, there are no errors in the console whatsoever, so that's nice (sarcasm).
And I am aware that there are many seemingly similar questions asked on StackOverflow, but none of the answers that I found have helped or applied to my situation.
It is extremely confusing as to why the elements are being rendered perfectly but the event listener is not working.
The full project can be found here and the file being excerpted below can be found here.
javascript html electron
1
Ethan did a bit of looking and I think you will need to add this event listener when you add the element to the DOM. Not before.
– Bibberty
Dec 30 '18 at 5:51
add a comment |
Background:
I am using ElectronJS to make a game and I have a class for a shop which I named Shop.
It has a method that I call 'createItemElement' which takes an object named 'item' and creates an li element to be later added to a ul element. The code for this can be found below.
class Shop {
// ...
createItemElement(item) {
// Item Container
const li = document.createElement("li");
// Title
const title = document.createElement("h3");
title.textContent = item.title;
// Info
const info = document.createElement("p");
info.textContent = `Type: ${item.type}`;
// Add to Cart
const addButton = document.createElement("button");
addButton.textContent = "Add to Cart";
addButton.onclick = () => console.log("Adding to cart!");
li.appendChild(title);
li.appendChild(info);
li.appendChild(addButton);
return li;
}
// ...
}
Problem:
Interestingly, all of the HTML is correctly rendered and everything looks as it should, but the 'onclick' event just plain does not work.
Since all of these elements are in fact being rendered, it should be safe to assume that the code is indeed being run in the renderer process.
For some reason, however, the event is not being carried over to the app.
When I click the button in the app, nothing happens.
I checked the devTools and looked at the elements individually.
As expected, all of the elements were listed out correctly in element inspector, but when I inspected that 'addButton' button, there was no 'onclick' property to be seen.
Also, there are no errors in the console whatsoever, so that's nice (sarcasm).
And I am aware that there are many seemingly similar questions asked on StackOverflow, but none of the answers that I found have helped or applied to my situation.
It is extremely confusing as to why the elements are being rendered perfectly but the event listener is not working.
The full project can be found here and the file being excerpted below can be found here.
javascript html electron
Background:
I am using ElectronJS to make a game and I have a class for a shop which I named Shop.
It has a method that I call 'createItemElement' which takes an object named 'item' and creates an li element to be later added to a ul element. The code for this can be found below.
class Shop {
// ...
createItemElement(item) {
// Item Container
const li = document.createElement("li");
// Title
const title = document.createElement("h3");
title.textContent = item.title;
// Info
const info = document.createElement("p");
info.textContent = `Type: ${item.type}`;
// Add to Cart
const addButton = document.createElement("button");
addButton.textContent = "Add to Cart";
addButton.onclick = () => console.log("Adding to cart!");
li.appendChild(title);
li.appendChild(info);
li.appendChild(addButton);
return li;
}
// ...
}
Problem:
Interestingly, all of the HTML is correctly rendered and everything looks as it should, but the 'onclick' event just plain does not work.
Since all of these elements are in fact being rendered, it should be safe to assume that the code is indeed being run in the renderer process.
For some reason, however, the event is not being carried over to the app.
When I click the button in the app, nothing happens.
I checked the devTools and looked at the elements individually.
As expected, all of the elements were listed out correctly in element inspector, but when I inspected that 'addButton' button, there was no 'onclick' property to be seen.
Also, there are no errors in the console whatsoever, so that's nice (sarcasm).
And I am aware that there are many seemingly similar questions asked on StackOverflow, but none of the answers that I found have helped or applied to my situation.
It is extremely confusing as to why the elements are being rendered perfectly but the event listener is not working.
The full project can be found here and the file being excerpted below can be found here.
class Shop {
// ...
createItemElement(item) {
// Item Container
const li = document.createElement("li");
// Title
const title = document.createElement("h3");
title.textContent = item.title;
// Info
const info = document.createElement("p");
info.textContent = `Type: ${item.type}`;
// Add to Cart
const addButton = document.createElement("button");
addButton.textContent = "Add to Cart";
addButton.onclick = () => console.log("Adding to cart!");
li.appendChild(title);
li.appendChild(info);
li.appendChild(addButton);
return li;
}
// ...
}
class Shop {
// ...
createItemElement(item) {
// Item Container
const li = document.createElement("li");
// Title
const title = document.createElement("h3");
title.textContent = item.title;
// Info
const info = document.createElement("p");
info.textContent = `Type: ${item.type}`;
// Add to Cart
const addButton = document.createElement("button");
addButton.textContent = "Add to Cart";
addButton.onclick = () => console.log("Adding to cart!");
li.appendChild(title);
li.appendChild(info);
li.appendChild(addButton);
return li;
}
// ...
}
javascript html electron
javascript html electron
asked Dec 30 '18 at 5:26


Ethan DavidsonEthan Davidson
162
162
1
Ethan did a bit of looking and I think you will need to add this event listener when you add the element to the DOM. Not before.
– Bibberty
Dec 30 '18 at 5:51
add a comment |
1
Ethan did a bit of looking and I think you will need to add this event listener when you add the element to the DOM. Not before.
– Bibberty
Dec 30 '18 at 5:51
1
1
Ethan did a bit of looking and I think you will need to add this event listener when you add the element to the DOM. Not before.
– Bibberty
Dec 30 '18 at 5:51
Ethan did a bit of looking and I think you will need to add this event listener when you add the element to the DOM. Not before.
– Bibberty
Dec 30 '18 at 5:51
add a comment |
2 Answers
2
active
oldest
votes
I looked at your shop.js page, and you incorrectly camel-case the onclick
event in one place, but not in another. Using the camel-cased version of onclick
will yield no error and do nothing.
Won't work:
empty_cart_button.onClick = (evt) => this.emptyCart();
Works:
addButton.onclick = () => this.addToCart(item);
add a comment |
As I can see from you're code, you're using el.innerHTML
in the shop instance .getHTML()
method. this will return the inner HTML as a raw string without any event listeners, this is why you see the rendered content as expected but the click listener doesn't work.
In the sketch.js
file, the toggleShop
function should use appendChild
so instead of:
document.querySelector("#shop-container").innerHTML = shop.getHTML();
you should do:
document.querySelector("#shop-container").appendChild(shop.getElement())
and in the Shop
class add the getElement
method:
getElement() {
return this.el;
}
Be sure to toggle the shop and remove the #shop-container
innerHTML when you want to toggle it off.
Also, as @Andy Hoffman answered, you should set the onclick
property and not the onClick
.
OMG You are absolutely brilliant!!! I hadn't thought of that! Thank you so much for your help!
– Ethan Davidson
Dec 31 '18 at 6:07
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53975477%2fbutton-onclick-event-listener-not-working-in-electron-app%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I looked at your shop.js page, and you incorrectly camel-case the onclick
event in one place, but not in another. Using the camel-cased version of onclick
will yield no error and do nothing.
Won't work:
empty_cart_button.onClick = (evt) => this.emptyCart();
Works:
addButton.onclick = () => this.addToCart(item);
add a comment |
I looked at your shop.js page, and you incorrectly camel-case the onclick
event in one place, but not in another. Using the camel-cased version of onclick
will yield no error and do nothing.
Won't work:
empty_cart_button.onClick = (evt) => this.emptyCart();
Works:
addButton.onclick = () => this.addToCart(item);
add a comment |
I looked at your shop.js page, and you incorrectly camel-case the onclick
event in one place, but not in another. Using the camel-cased version of onclick
will yield no error and do nothing.
Won't work:
empty_cart_button.onClick = (evt) => this.emptyCart();
Works:
addButton.onclick = () => this.addToCart(item);
I looked at your shop.js page, and you incorrectly camel-case the onclick
event in one place, but not in another. Using the camel-cased version of onclick
will yield no error and do nothing.
Won't work:
empty_cart_button.onClick = (evt) => this.emptyCart();
Works:
addButton.onclick = () => this.addToCart(item);
edited Dec 30 '18 at 6:31
answered Dec 30 '18 at 6:04
Andy HoffmanAndy Hoffman
4,67521434
4,67521434
add a comment |
add a comment |
As I can see from you're code, you're using el.innerHTML
in the shop instance .getHTML()
method. this will return the inner HTML as a raw string without any event listeners, this is why you see the rendered content as expected but the click listener doesn't work.
In the sketch.js
file, the toggleShop
function should use appendChild
so instead of:
document.querySelector("#shop-container").innerHTML = shop.getHTML();
you should do:
document.querySelector("#shop-container").appendChild(shop.getElement())
and in the Shop
class add the getElement
method:
getElement() {
return this.el;
}
Be sure to toggle the shop and remove the #shop-container
innerHTML when you want to toggle it off.
Also, as @Andy Hoffman answered, you should set the onclick
property and not the onClick
.
OMG You are absolutely brilliant!!! I hadn't thought of that! Thank you so much for your help!
– Ethan Davidson
Dec 31 '18 at 6:07
add a comment |
As I can see from you're code, you're using el.innerHTML
in the shop instance .getHTML()
method. this will return the inner HTML as a raw string without any event listeners, this is why you see the rendered content as expected but the click listener doesn't work.
In the sketch.js
file, the toggleShop
function should use appendChild
so instead of:
document.querySelector("#shop-container").innerHTML = shop.getHTML();
you should do:
document.querySelector("#shop-container").appendChild(shop.getElement())
and in the Shop
class add the getElement
method:
getElement() {
return this.el;
}
Be sure to toggle the shop and remove the #shop-container
innerHTML when you want to toggle it off.
Also, as @Andy Hoffman answered, you should set the onclick
property and not the onClick
.
OMG You are absolutely brilliant!!! I hadn't thought of that! Thank you so much for your help!
– Ethan Davidson
Dec 31 '18 at 6:07
add a comment |
As I can see from you're code, you're using el.innerHTML
in the shop instance .getHTML()
method. this will return the inner HTML as a raw string without any event listeners, this is why you see the rendered content as expected but the click listener doesn't work.
In the sketch.js
file, the toggleShop
function should use appendChild
so instead of:
document.querySelector("#shop-container").innerHTML = shop.getHTML();
you should do:
document.querySelector("#shop-container").appendChild(shop.getElement())
and in the Shop
class add the getElement
method:
getElement() {
return this.el;
}
Be sure to toggle the shop and remove the #shop-container
innerHTML when you want to toggle it off.
Also, as @Andy Hoffman answered, you should set the onclick
property and not the onClick
.
As I can see from you're code, you're using el.innerHTML
in the shop instance .getHTML()
method. this will return the inner HTML as a raw string without any event listeners, this is why you see the rendered content as expected but the click listener doesn't work.
In the sketch.js
file, the toggleShop
function should use appendChild
so instead of:
document.querySelector("#shop-container").innerHTML = shop.getHTML();
you should do:
document.querySelector("#shop-container").appendChild(shop.getElement())
and in the Shop
class add the getElement
method:
getElement() {
return this.el;
}
Be sure to toggle the shop and remove the #shop-container
innerHTML when you want to toggle it off.
Also, as @Andy Hoffman answered, you should set the onclick
property and not the onClick
.
answered Dec 30 '18 at 7:41
udiduudidu
5,39233356
5,39233356
OMG You are absolutely brilliant!!! I hadn't thought of that! Thank you so much for your help!
– Ethan Davidson
Dec 31 '18 at 6:07
add a comment |
OMG You are absolutely brilliant!!! I hadn't thought of that! Thank you so much for your help!
– Ethan Davidson
Dec 31 '18 at 6:07
OMG You are absolutely brilliant!!! I hadn't thought of that! Thank you so much for your help!
– Ethan Davidson
Dec 31 '18 at 6:07
OMG You are absolutely brilliant!!! I hadn't thought of that! Thank you so much for your help!
– Ethan Davidson
Dec 31 '18 at 6:07
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53975477%2fbutton-onclick-event-listener-not-working-in-electron-app%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WmhxP0XF2VwIHR3bXI8DHgtk9zDKkU7TFXPJ,hSwTEGvGnCcMf 7jicdzq ESXxRXYz
1
Ethan did a bit of looking and I think you will need to add this event listener when you add the element to the DOM. Not before.
– Bibberty
Dec 30 '18 at 5:51