Prevent null check in Mapstruct on update
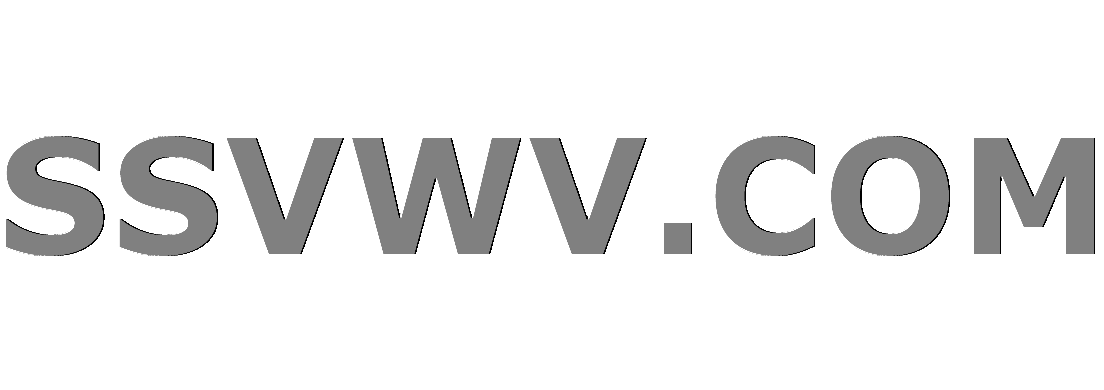
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm struggling with form update. Please consider this example:
// Entity with which I need to perform CRUD operations
public class User {
private String name;
private String email;
private String phone;
private String address;
}
I send to UI is UserDTO:
public class UserDTO {
private String name;
private ContactDataDTO contactDataDTO;
}
public class ContactDataDTO {
private String email;
private String phone;
private String address;
}
My mapper:
@Mapper
public interface UserMapper {
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
@InheritInverseConfiguration
User updateUserFromUserDTO(UserDTO userDTO, @MappingTarget User user);
}
userToUserDTO() works as expected, but generated userDTOToUser() for me seems wierd:
@Override
public User updateUserFromUserDTO(UserDTO userDTO, User user) {
if ( userDTO == null ) {
return null;
}
String address = userDTOContactDataDTOAddress( userDTO );
if ( address != null ) {
user.setAddress( address );
}
String phone = userDTOContactDataDTOPhone( userDTO );
if ( phone != null ) {
user.setPhone( phone );
}
String email = userDTOContactDataDTOEmail( userDTO );
if ( email != null ) {
user.setEmail( email );
}
user.setName( userDTO.getName() );
return user;
}
Problematic use case:
- Fill in all fields for User.
- Open form again and clear phone field.
- That means to backend I will send smth like this:
userDTO: {
name: 'John Doe';
contactDataDTO: {
email: 'johndoe@gmail.com',
phone: null,
address: 'Home'
}
}
So, user.phone won't be updated, as far as I have null check for it in generated code.
I thought NullValueCheckStrategy is what I need, but there no option which fits me.
For now the only option I see - write my own implementation of userDTOToUser() without null checks.
Maybe you can advise better solution, cause for me it looks like a problem that can happen in any mapper for a target update from DTO with non-primitive source.
Runnable demo: https://repl.it/@aksankin/SlateblueUnimportantStack
Thanks a lot.
java mapstruct
add a comment |
I'm struggling with form update. Please consider this example:
// Entity with which I need to perform CRUD operations
public class User {
private String name;
private String email;
private String phone;
private String address;
}
I send to UI is UserDTO:
public class UserDTO {
private String name;
private ContactDataDTO contactDataDTO;
}
public class ContactDataDTO {
private String email;
private String phone;
private String address;
}
My mapper:
@Mapper
public interface UserMapper {
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
@InheritInverseConfiguration
User updateUserFromUserDTO(UserDTO userDTO, @MappingTarget User user);
}
userToUserDTO() works as expected, but generated userDTOToUser() for me seems wierd:
@Override
public User updateUserFromUserDTO(UserDTO userDTO, User user) {
if ( userDTO == null ) {
return null;
}
String address = userDTOContactDataDTOAddress( userDTO );
if ( address != null ) {
user.setAddress( address );
}
String phone = userDTOContactDataDTOPhone( userDTO );
if ( phone != null ) {
user.setPhone( phone );
}
String email = userDTOContactDataDTOEmail( userDTO );
if ( email != null ) {
user.setEmail( email );
}
user.setName( userDTO.getName() );
return user;
}
Problematic use case:
- Fill in all fields for User.
- Open form again and clear phone field.
- That means to backend I will send smth like this:
userDTO: {
name: 'John Doe';
contactDataDTO: {
email: 'johndoe@gmail.com',
phone: null,
address: 'Home'
}
}
So, user.phone won't be updated, as far as I have null check for it in generated code.
I thought NullValueCheckStrategy is what I need, but there no option which fits me.
For now the only option I see - write my own implementation of userDTOToUser() without null checks.
Maybe you can advise better solution, cause for me it looks like a problem that can happen in any mapper for a target update from DTO with non-primitive source.
Runnable demo: https://repl.it/@aksankin/SlateblueUnimportantStack
Thanks a lot.
java mapstruct
one of the prettiest code styles i've seen.
– aran
Jan 4 at 13:49
In MapStruct 1.3Beta2 we introduced theNullValuePropertyMappingStrategy
specifically to control update methods. It seems to me this is not working for nested properties. I need to investigate a bit further.
– Sjaak
Jan 7 at 10:24
@sjaak tried it, didn't help. Tried basically all annotation and all options anyhow connected to "null") The cleanest from all dirty workaround I can think about now is to create new User object from UserDTO and then just update User.id with UserDTO.id. But I would be happy to know the recommended way to do it if it's possible.
– Oksana Mykhalets'
Jan 7 at 10:45
I tried it as wel (and you are right). Please write an issue on MapStruct (github.com/mapstruct/mapstruct/issues).. I already started to take a look :). In the mean time, don't use target nesting in this scenario (this is what happens when you revert source nesting). I'll provide a work-around as answer.
– Sjaak
Jan 7 at 14:12
@OksanaMykhalets': As an afterburner: it will work out of the box with your current example (I used your example to implement this, thanks). 1.3 should be released soon.
– Sjaak
Jan 29 at 20:18
add a comment |
I'm struggling with form update. Please consider this example:
// Entity with which I need to perform CRUD operations
public class User {
private String name;
private String email;
private String phone;
private String address;
}
I send to UI is UserDTO:
public class UserDTO {
private String name;
private ContactDataDTO contactDataDTO;
}
public class ContactDataDTO {
private String email;
private String phone;
private String address;
}
My mapper:
@Mapper
public interface UserMapper {
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
@InheritInverseConfiguration
User updateUserFromUserDTO(UserDTO userDTO, @MappingTarget User user);
}
userToUserDTO() works as expected, but generated userDTOToUser() for me seems wierd:
@Override
public User updateUserFromUserDTO(UserDTO userDTO, User user) {
if ( userDTO == null ) {
return null;
}
String address = userDTOContactDataDTOAddress( userDTO );
if ( address != null ) {
user.setAddress( address );
}
String phone = userDTOContactDataDTOPhone( userDTO );
if ( phone != null ) {
user.setPhone( phone );
}
String email = userDTOContactDataDTOEmail( userDTO );
if ( email != null ) {
user.setEmail( email );
}
user.setName( userDTO.getName() );
return user;
}
Problematic use case:
- Fill in all fields for User.
- Open form again and clear phone field.
- That means to backend I will send smth like this:
userDTO: {
name: 'John Doe';
contactDataDTO: {
email: 'johndoe@gmail.com',
phone: null,
address: 'Home'
}
}
So, user.phone won't be updated, as far as I have null check for it in generated code.
I thought NullValueCheckStrategy is what I need, but there no option which fits me.
For now the only option I see - write my own implementation of userDTOToUser() without null checks.
Maybe you can advise better solution, cause for me it looks like a problem that can happen in any mapper for a target update from DTO with non-primitive source.
Runnable demo: https://repl.it/@aksankin/SlateblueUnimportantStack
Thanks a lot.
java mapstruct
I'm struggling with form update. Please consider this example:
// Entity with which I need to perform CRUD operations
public class User {
private String name;
private String email;
private String phone;
private String address;
}
I send to UI is UserDTO:
public class UserDTO {
private String name;
private ContactDataDTO contactDataDTO;
}
public class ContactDataDTO {
private String email;
private String phone;
private String address;
}
My mapper:
@Mapper
public interface UserMapper {
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
@InheritInverseConfiguration
User updateUserFromUserDTO(UserDTO userDTO, @MappingTarget User user);
}
userToUserDTO() works as expected, but generated userDTOToUser() for me seems wierd:
@Override
public User updateUserFromUserDTO(UserDTO userDTO, User user) {
if ( userDTO == null ) {
return null;
}
String address = userDTOContactDataDTOAddress( userDTO );
if ( address != null ) {
user.setAddress( address );
}
String phone = userDTOContactDataDTOPhone( userDTO );
if ( phone != null ) {
user.setPhone( phone );
}
String email = userDTOContactDataDTOEmail( userDTO );
if ( email != null ) {
user.setEmail( email );
}
user.setName( userDTO.getName() );
return user;
}
Problematic use case:
- Fill in all fields for User.
- Open form again and clear phone field.
- That means to backend I will send smth like this:
userDTO: {
name: 'John Doe';
contactDataDTO: {
email: 'johndoe@gmail.com',
phone: null,
address: 'Home'
}
}
So, user.phone won't be updated, as far as I have null check for it in generated code.
I thought NullValueCheckStrategy is what I need, but there no option which fits me.
For now the only option I see - write my own implementation of userDTOToUser() without null checks.
Maybe you can advise better solution, cause for me it looks like a problem that can happen in any mapper for a target update from DTO with non-primitive source.
Runnable demo: https://repl.it/@aksankin/SlateblueUnimportantStack
Thanks a lot.
java mapstruct
java mapstruct
edited Jan 7 at 10:46
Oksana Mykhalets'
asked Jan 4 at 10:42
Oksana Mykhalets'Oksana Mykhalets'
134
134
one of the prettiest code styles i've seen.
– aran
Jan 4 at 13:49
In MapStruct 1.3Beta2 we introduced theNullValuePropertyMappingStrategy
specifically to control update methods. It seems to me this is not working for nested properties. I need to investigate a bit further.
– Sjaak
Jan 7 at 10:24
@sjaak tried it, didn't help. Tried basically all annotation and all options anyhow connected to "null") The cleanest from all dirty workaround I can think about now is to create new User object from UserDTO and then just update User.id with UserDTO.id. But I would be happy to know the recommended way to do it if it's possible.
– Oksana Mykhalets'
Jan 7 at 10:45
I tried it as wel (and you are right). Please write an issue on MapStruct (github.com/mapstruct/mapstruct/issues).. I already started to take a look :). In the mean time, don't use target nesting in this scenario (this is what happens when you revert source nesting). I'll provide a work-around as answer.
– Sjaak
Jan 7 at 14:12
@OksanaMykhalets': As an afterburner: it will work out of the box with your current example (I used your example to implement this, thanks). 1.3 should be released soon.
– Sjaak
Jan 29 at 20:18
add a comment |
one of the prettiest code styles i've seen.
– aran
Jan 4 at 13:49
In MapStruct 1.3Beta2 we introduced theNullValuePropertyMappingStrategy
specifically to control update methods. It seems to me this is not working for nested properties. I need to investigate a bit further.
– Sjaak
Jan 7 at 10:24
@sjaak tried it, didn't help. Tried basically all annotation and all options anyhow connected to "null") The cleanest from all dirty workaround I can think about now is to create new User object from UserDTO and then just update User.id with UserDTO.id. But I would be happy to know the recommended way to do it if it's possible.
– Oksana Mykhalets'
Jan 7 at 10:45
I tried it as wel (and you are right). Please write an issue on MapStruct (github.com/mapstruct/mapstruct/issues).. I already started to take a look :). In the mean time, don't use target nesting in this scenario (this is what happens when you revert source nesting). I'll provide a work-around as answer.
– Sjaak
Jan 7 at 14:12
@OksanaMykhalets': As an afterburner: it will work out of the box with your current example (I used your example to implement this, thanks). 1.3 should be released soon.
– Sjaak
Jan 29 at 20:18
one of the prettiest code styles i've seen.
– aran
Jan 4 at 13:49
one of the prettiest code styles i've seen.
– aran
Jan 4 at 13:49
In MapStruct 1.3Beta2 we introduced the
NullValuePropertyMappingStrategy
specifically to control update methods. It seems to me this is not working for nested properties. I need to investigate a bit further.– Sjaak
Jan 7 at 10:24
In MapStruct 1.3Beta2 we introduced the
NullValuePropertyMappingStrategy
specifically to control update methods. It seems to me this is not working for nested properties. I need to investigate a bit further.– Sjaak
Jan 7 at 10:24
@sjaak tried it, didn't help. Tried basically all annotation and all options anyhow connected to "null") The cleanest from all dirty workaround I can think about now is to create new User object from UserDTO and then just update User.id with UserDTO.id. But I would be happy to know the recommended way to do it if it's possible.
– Oksana Mykhalets'
Jan 7 at 10:45
@sjaak tried it, didn't help. Tried basically all annotation and all options anyhow connected to "null") The cleanest from all dirty workaround I can think about now is to create new User object from UserDTO and then just update User.id with UserDTO.id. But I would be happy to know the recommended way to do it if it's possible.
– Oksana Mykhalets'
Jan 7 at 10:45
I tried it as wel (and you are right). Please write an issue on MapStruct (github.com/mapstruct/mapstruct/issues).. I already started to take a look :). In the mean time, don't use target nesting in this scenario (this is what happens when you revert source nesting). I'll provide a work-around as answer.
– Sjaak
Jan 7 at 14:12
I tried it as wel (and you are right). Please write an issue on MapStruct (github.com/mapstruct/mapstruct/issues).. I already started to take a look :). In the mean time, don't use target nesting in this scenario (this is what happens when you revert source nesting). I'll provide a work-around as answer.
– Sjaak
Jan 7 at 14:12
@OksanaMykhalets': As an afterburner: it will work out of the box with your current example (I used your example to implement this, thanks). 1.3 should be released soon.
– Sjaak
Jan 29 at 20:18
@OksanaMykhalets': As an afterburner: it will work out of the box with your current example (I used your example to implement this, thanks). 1.3 should be released soon.
– Sjaak
Jan 29 at 20:18
add a comment |
3 Answers
3
active
oldest
votes
If you want null
to have a certain value for you then you are looking for source presence checking. You can then control in the setPhone
of the DTO whether it was set or not and add hasPhone
that would use the flag. Then MapStruct will use the presence check method when setting the value.
thank you for your response. I tried to add hasPhone() method, but it still don't work for me. Could I ask you to check this runnable demo, maybe you can see a mistake? repl.it/@aksankin/SlateblueUnimportantStack
– Oksana Mykhalets'
Jan 7 at 9:32
OK this is something that we were adding in the latest versions. Can you try to addhasContactDataDTO
to theUserDTO
? That should generate something that you are looking for. I can update the answer accordingly later. Another option would be to update the user from the contact as well. You won't need to use nested source
– Filip
Jan 7 at 13:09
thank you, hasContactDataDTO() worked perfectly!
– Oksana Mykhalets'
Jan 7 at 13:37
add a comment |
Probably Optional<> is what you're searching for. In this case you would have null for empty field, Optional if null was send from UI and Optional for actual value. But probably you need to create different DTO for request and response.
add a comment |
try:
@Mapper( )
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper( UserMapper.class );
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
default void updateUserFromUserDTO(UserDTO userDTO, User user) {
intUpdateUserFromUserDTO( userDTO, userDTO.getContactDataDTO(), user );
}
void intUpdateUserFromUserDTO(UserDTO userDTO, ContactDataDTO contactDataDTO, @MappingTarget User user);
}
(note: I returned void iso a type, which is strictly not needed).
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54037352%2fprevent-null-check-in-mapstruct-on-update%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want null
to have a certain value for you then you are looking for source presence checking. You can then control in the setPhone
of the DTO whether it was set or not and add hasPhone
that would use the flag. Then MapStruct will use the presence check method when setting the value.
thank you for your response. I tried to add hasPhone() method, but it still don't work for me. Could I ask you to check this runnable demo, maybe you can see a mistake? repl.it/@aksankin/SlateblueUnimportantStack
– Oksana Mykhalets'
Jan 7 at 9:32
OK this is something that we were adding in the latest versions. Can you try to addhasContactDataDTO
to theUserDTO
? That should generate something that you are looking for. I can update the answer accordingly later. Another option would be to update the user from the contact as well. You won't need to use nested source
– Filip
Jan 7 at 13:09
thank you, hasContactDataDTO() worked perfectly!
– Oksana Mykhalets'
Jan 7 at 13:37
add a comment |
If you want null
to have a certain value for you then you are looking for source presence checking. You can then control in the setPhone
of the DTO whether it was set or not and add hasPhone
that would use the flag. Then MapStruct will use the presence check method when setting the value.
thank you for your response. I tried to add hasPhone() method, but it still don't work for me. Could I ask you to check this runnable demo, maybe you can see a mistake? repl.it/@aksankin/SlateblueUnimportantStack
– Oksana Mykhalets'
Jan 7 at 9:32
OK this is something that we were adding in the latest versions. Can you try to addhasContactDataDTO
to theUserDTO
? That should generate something that you are looking for. I can update the answer accordingly later. Another option would be to update the user from the contact as well. You won't need to use nested source
– Filip
Jan 7 at 13:09
thank you, hasContactDataDTO() worked perfectly!
– Oksana Mykhalets'
Jan 7 at 13:37
add a comment |
If you want null
to have a certain value for you then you are looking for source presence checking. You can then control in the setPhone
of the DTO whether it was set or not and add hasPhone
that would use the flag. Then MapStruct will use the presence check method when setting the value.
If you want null
to have a certain value for you then you are looking for source presence checking. You can then control in the setPhone
of the DTO whether it was set or not and add hasPhone
that would use the flag. Then MapStruct will use the presence check method when setting the value.
answered Jan 4 at 17:20
FilipFilip
3,77731531
3,77731531
thank you for your response. I tried to add hasPhone() method, but it still don't work for me. Could I ask you to check this runnable demo, maybe you can see a mistake? repl.it/@aksankin/SlateblueUnimportantStack
– Oksana Mykhalets'
Jan 7 at 9:32
OK this is something that we were adding in the latest versions. Can you try to addhasContactDataDTO
to theUserDTO
? That should generate something that you are looking for. I can update the answer accordingly later. Another option would be to update the user from the contact as well. You won't need to use nested source
– Filip
Jan 7 at 13:09
thank you, hasContactDataDTO() worked perfectly!
– Oksana Mykhalets'
Jan 7 at 13:37
add a comment |
thank you for your response. I tried to add hasPhone() method, but it still don't work for me. Could I ask you to check this runnable demo, maybe you can see a mistake? repl.it/@aksankin/SlateblueUnimportantStack
– Oksana Mykhalets'
Jan 7 at 9:32
OK this is something that we were adding in the latest versions. Can you try to addhasContactDataDTO
to theUserDTO
? That should generate something that you are looking for. I can update the answer accordingly later. Another option would be to update the user from the contact as well. You won't need to use nested source
– Filip
Jan 7 at 13:09
thank you, hasContactDataDTO() worked perfectly!
– Oksana Mykhalets'
Jan 7 at 13:37
thank you for your response. I tried to add hasPhone() method, but it still don't work for me. Could I ask you to check this runnable demo, maybe you can see a mistake? repl.it/@aksankin/SlateblueUnimportantStack
– Oksana Mykhalets'
Jan 7 at 9:32
thank you for your response. I tried to add hasPhone() method, but it still don't work for me. Could I ask you to check this runnable demo, maybe you can see a mistake? repl.it/@aksankin/SlateblueUnimportantStack
– Oksana Mykhalets'
Jan 7 at 9:32
OK this is something that we were adding in the latest versions. Can you try to add
hasContactDataDTO
to the UserDTO
? That should generate something that you are looking for. I can update the answer accordingly later. Another option would be to update the user from the contact as well. You won't need to use nested source– Filip
Jan 7 at 13:09
OK this is something that we were adding in the latest versions. Can you try to add
hasContactDataDTO
to the UserDTO
? That should generate something that you are looking for. I can update the answer accordingly later. Another option would be to update the user from the contact as well. You won't need to use nested source– Filip
Jan 7 at 13:09
thank you, hasContactDataDTO() worked perfectly!
– Oksana Mykhalets'
Jan 7 at 13:37
thank you, hasContactDataDTO() worked perfectly!
– Oksana Mykhalets'
Jan 7 at 13:37
add a comment |
Probably Optional<> is what you're searching for. In this case you would have null for empty field, Optional if null was send from UI and Optional for actual value. But probably you need to create different DTO for request and response.
add a comment |
Probably Optional<> is what you're searching for. In this case you would have null for empty field, Optional if null was send from UI and Optional for actual value. But probably you need to create different DTO for request and response.
add a comment |
Probably Optional<> is what you're searching for. In this case you would have null for empty field, Optional if null was send from UI and Optional for actual value. But probably you need to create different DTO for request and response.
Probably Optional<> is what you're searching for. In this case you would have null for empty field, Optional if null was send from UI and Optional for actual value. But probably you need to create different DTO for request and response.
answered Jan 4 at 16:11
vansanvansan
112
112
add a comment |
add a comment |
try:
@Mapper( )
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper( UserMapper.class );
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
default void updateUserFromUserDTO(UserDTO userDTO, User user) {
intUpdateUserFromUserDTO( userDTO, userDTO.getContactDataDTO(), user );
}
void intUpdateUserFromUserDTO(UserDTO userDTO, ContactDataDTO contactDataDTO, @MappingTarget User user);
}
(note: I returned void iso a type, which is strictly not needed).
add a comment |
try:
@Mapper( )
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper( UserMapper.class );
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
default void updateUserFromUserDTO(UserDTO userDTO, User user) {
intUpdateUserFromUserDTO( userDTO, userDTO.getContactDataDTO(), user );
}
void intUpdateUserFromUserDTO(UserDTO userDTO, ContactDataDTO contactDataDTO, @MappingTarget User user);
}
(note: I returned void iso a type, which is strictly not needed).
add a comment |
try:
@Mapper( )
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper( UserMapper.class );
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
default void updateUserFromUserDTO(UserDTO userDTO, User user) {
intUpdateUserFromUserDTO( userDTO, userDTO.getContactDataDTO(), user );
}
void intUpdateUserFromUserDTO(UserDTO userDTO, ContactDataDTO contactDataDTO, @MappingTarget User user);
}
(note: I returned void iso a type, which is strictly not needed).
try:
@Mapper( )
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper( UserMapper.class );
@Mappings({
@Mapping(source="email", target="contactDataDTO.email"),
@Mapping(source="phone", target="contactDataDTO.phone"),
@Mapping(source="address", target="contactDataDTO.address")
})
UserDTO userToUserDTO(User user);
default void updateUserFromUserDTO(UserDTO userDTO, User user) {
intUpdateUserFromUserDTO( userDTO, userDTO.getContactDataDTO(), user );
}
void intUpdateUserFromUserDTO(UserDTO userDTO, ContactDataDTO contactDataDTO, @MappingTarget User user);
}
(note: I returned void iso a type, which is strictly not needed).
answered Jan 7 at 14:13
SjaakSjaak
602515
602515
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54037352%2fprevent-null-check-in-mapstruct-on-update%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XOMdo3,xU R q9nrYHzfho NFqcqq4Xkjy,UEiIe FQ7A1NeZLSQNd5,JK2lFHI
one of the prettiest code styles i've seen.
– aran
Jan 4 at 13:49
In MapStruct 1.3Beta2 we introduced the
NullValuePropertyMappingStrategy
specifically to control update methods. It seems to me this is not working for nested properties. I need to investigate a bit further.– Sjaak
Jan 7 at 10:24
@sjaak tried it, didn't help. Tried basically all annotation and all options anyhow connected to "null") The cleanest from all dirty workaround I can think about now is to create new User object from UserDTO and then just update User.id with UserDTO.id. But I would be happy to know the recommended way to do it if it's possible.
– Oksana Mykhalets'
Jan 7 at 10:45
I tried it as wel (and you are right). Please write an issue on MapStruct (github.com/mapstruct/mapstruct/issues).. I already started to take a look :). In the mean time, don't use target nesting in this scenario (this is what happens when you revert source nesting). I'll provide a work-around as answer.
– Sjaak
Jan 7 at 14:12
@OksanaMykhalets': As an afterburner: it will work out of the box with your current example (I used your example to implement this, thanks). 1.3 should be released soon.
– Sjaak
Jan 29 at 20:18