How to get the msbuild path returned from “nuget.exe restore”
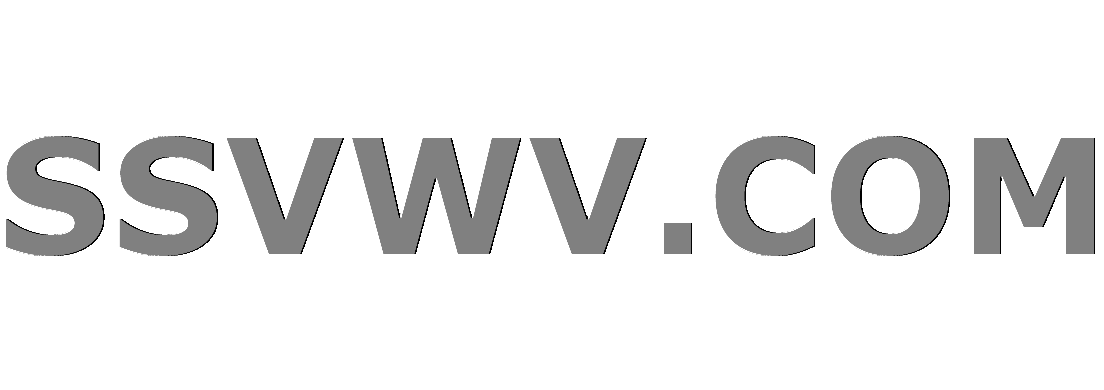
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm new to using git hooks and now I'm trying to restore nuget packages for the projects in my solution and after that build the solution.
What I did is create a git hook 'post-merge' and added the following code:
#!/bin/sh
RED='33[0;31m'
GREEN='33[0;32m'
NC='33[0m'
projectRoot="$(git rev-parse --show-toplevel)"
solutionFile="solution.sln"
msBuild="C:Program Files (x86)Microsoft Visual Studio2017CommunityMSBuild15.0BinMSBuild.exe"
echo -e "n${RED}Restoring nuget packages for ${solutionFile}...${NC}"
./nuget.exe restore $projectRoot/development/$solutionFile
echo -e "${GREEN}Done restoring nuget packages for ${solutionFile}.${NC}n"
echo -e "n${RED}Building ${solutionFile}...${NC}"
"$msBuild" $projectRoot/development/$solutionFile
echo -e "${GREEN}Done building ${solutionFile}.${NC}n"
exit 1
I added exit 1 so that I can test my commands without actually pulling the project.
Now after I run nuget.exe on the solution path, it returns a msbuild auto-detection path, I want to use this path to locate msbuild.exe so I can run msbuild on the solution based on the version it's using.
How do I do this?
And if there's any way to improve or make this code better please let me know!
Thank you!
git msbuild nuget githooks
add a comment |
I'm new to using git hooks and now I'm trying to restore nuget packages for the projects in my solution and after that build the solution.
What I did is create a git hook 'post-merge' and added the following code:
#!/bin/sh
RED='33[0;31m'
GREEN='33[0;32m'
NC='33[0m'
projectRoot="$(git rev-parse --show-toplevel)"
solutionFile="solution.sln"
msBuild="C:Program Files (x86)Microsoft Visual Studio2017CommunityMSBuild15.0BinMSBuild.exe"
echo -e "n${RED}Restoring nuget packages for ${solutionFile}...${NC}"
./nuget.exe restore $projectRoot/development/$solutionFile
echo -e "${GREEN}Done restoring nuget packages for ${solutionFile}.${NC}n"
echo -e "n${RED}Building ${solutionFile}...${NC}"
"$msBuild" $projectRoot/development/$solutionFile
echo -e "${GREEN}Done building ${solutionFile}.${NC}n"
exit 1
I added exit 1 so that I can test my commands without actually pulling the project.
Now after I run nuget.exe on the solution path, it returns a msbuild auto-detection path, I want to use this path to locate msbuild.exe so I can run msbuild on the solution based on the version it's using.
How do I do this?
And if there's any way to improve or make this code better please let me know!
Thank you!
git msbuild nuget githooks
add a comment |
I'm new to using git hooks and now I'm trying to restore nuget packages for the projects in my solution and after that build the solution.
What I did is create a git hook 'post-merge' and added the following code:
#!/bin/sh
RED='33[0;31m'
GREEN='33[0;32m'
NC='33[0m'
projectRoot="$(git rev-parse --show-toplevel)"
solutionFile="solution.sln"
msBuild="C:Program Files (x86)Microsoft Visual Studio2017CommunityMSBuild15.0BinMSBuild.exe"
echo -e "n${RED}Restoring nuget packages for ${solutionFile}...${NC}"
./nuget.exe restore $projectRoot/development/$solutionFile
echo -e "${GREEN}Done restoring nuget packages for ${solutionFile}.${NC}n"
echo -e "n${RED}Building ${solutionFile}...${NC}"
"$msBuild" $projectRoot/development/$solutionFile
echo -e "${GREEN}Done building ${solutionFile}.${NC}n"
exit 1
I added exit 1 so that I can test my commands without actually pulling the project.
Now after I run nuget.exe on the solution path, it returns a msbuild auto-detection path, I want to use this path to locate msbuild.exe so I can run msbuild on the solution based on the version it's using.
How do I do this?
And if there's any way to improve or make this code better please let me know!
Thank you!
git msbuild nuget githooks
I'm new to using git hooks and now I'm trying to restore nuget packages for the projects in my solution and after that build the solution.
What I did is create a git hook 'post-merge' and added the following code:
#!/bin/sh
RED='33[0;31m'
GREEN='33[0;32m'
NC='33[0m'
projectRoot="$(git rev-parse --show-toplevel)"
solutionFile="solution.sln"
msBuild="C:Program Files (x86)Microsoft Visual Studio2017CommunityMSBuild15.0BinMSBuild.exe"
echo -e "n${RED}Restoring nuget packages for ${solutionFile}...${NC}"
./nuget.exe restore $projectRoot/development/$solutionFile
echo -e "${GREEN}Done restoring nuget packages for ${solutionFile}.${NC}n"
echo -e "n${RED}Building ${solutionFile}...${NC}"
"$msBuild" $projectRoot/development/$solutionFile
echo -e "${GREEN}Done building ${solutionFile}.${NC}n"
exit 1
I added exit 1 so that I can test my commands without actually pulling the project.
Now after I run nuget.exe on the solution path, it returns a msbuild auto-detection path, I want to use this path to locate msbuild.exe so I can run msbuild on the solution based on the version it's using.
How do I do this?
And if there's any way to improve or make this code better please let me know!
Thank you!
git msbuild nuget githooks
git msbuild nuget githooks
asked Jan 4 at 10:35


Koen van RasKoen van Ras
159
159
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Rather than capturing and parsing NuGet's output, you can use VSWhere to find MSBuild.
From the wiki page:
With Visual Studio 2017 Update 2 or newer installed, you can find vswhere at %ProgramFiles(x86)%Microsoft Visual StudioInstallervswhere.exe, or to make sure it's always available in your repo see Installing for an option using NuGet.
powershell example that you'll need to adapt to Bash if you want to keep using bash
$path = vswhere -latest -products * -requires Microsoft.Component.MSBuild -property installationPath
if ($path) {
$path = join-path $path 'MSBuild15.0BinMSBuild.exe'
if (test-path $path) {
& $path $args
}
}
EDIT: If you have the .NET Core SDK installed, you can just use dotnet msbuild
to run msbuild, since installing the .NET Core SDK puts dotnet.exe
on the path. The only real difference between using a Visual Studio MSBuild and the .NET Core MSBuild is that the extensions path will be different. But if your build doesn't depend on an MSBuild extension, then using dotnet msbuild
works without having to find the path to an executable first.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54037254%2fhow-to-get-the-msbuild-path-returned-from-nuget-exe-restore%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Rather than capturing and parsing NuGet's output, you can use VSWhere to find MSBuild.
From the wiki page:
With Visual Studio 2017 Update 2 or newer installed, you can find vswhere at %ProgramFiles(x86)%Microsoft Visual StudioInstallervswhere.exe, or to make sure it's always available in your repo see Installing for an option using NuGet.
powershell example that you'll need to adapt to Bash if you want to keep using bash
$path = vswhere -latest -products * -requires Microsoft.Component.MSBuild -property installationPath
if ($path) {
$path = join-path $path 'MSBuild15.0BinMSBuild.exe'
if (test-path $path) {
& $path $args
}
}
EDIT: If you have the .NET Core SDK installed, you can just use dotnet msbuild
to run msbuild, since installing the .NET Core SDK puts dotnet.exe
on the path. The only real difference between using a Visual Studio MSBuild and the .NET Core MSBuild is that the extensions path will be different. But if your build doesn't depend on an MSBuild extension, then using dotnet msbuild
works without having to find the path to an executable first.
add a comment |
Rather than capturing and parsing NuGet's output, you can use VSWhere to find MSBuild.
From the wiki page:
With Visual Studio 2017 Update 2 or newer installed, you can find vswhere at %ProgramFiles(x86)%Microsoft Visual StudioInstallervswhere.exe, or to make sure it's always available in your repo see Installing for an option using NuGet.
powershell example that you'll need to adapt to Bash if you want to keep using bash
$path = vswhere -latest -products * -requires Microsoft.Component.MSBuild -property installationPath
if ($path) {
$path = join-path $path 'MSBuild15.0BinMSBuild.exe'
if (test-path $path) {
& $path $args
}
}
EDIT: If you have the .NET Core SDK installed, you can just use dotnet msbuild
to run msbuild, since installing the .NET Core SDK puts dotnet.exe
on the path. The only real difference between using a Visual Studio MSBuild and the .NET Core MSBuild is that the extensions path will be different. But if your build doesn't depend on an MSBuild extension, then using dotnet msbuild
works without having to find the path to an executable first.
add a comment |
Rather than capturing and parsing NuGet's output, you can use VSWhere to find MSBuild.
From the wiki page:
With Visual Studio 2017 Update 2 or newer installed, you can find vswhere at %ProgramFiles(x86)%Microsoft Visual StudioInstallervswhere.exe, or to make sure it's always available in your repo see Installing for an option using NuGet.
powershell example that you'll need to adapt to Bash if you want to keep using bash
$path = vswhere -latest -products * -requires Microsoft.Component.MSBuild -property installationPath
if ($path) {
$path = join-path $path 'MSBuild15.0BinMSBuild.exe'
if (test-path $path) {
& $path $args
}
}
EDIT: If you have the .NET Core SDK installed, you can just use dotnet msbuild
to run msbuild, since installing the .NET Core SDK puts dotnet.exe
on the path. The only real difference between using a Visual Studio MSBuild and the .NET Core MSBuild is that the extensions path will be different. But if your build doesn't depend on an MSBuild extension, then using dotnet msbuild
works without having to find the path to an executable first.
Rather than capturing and parsing NuGet's output, you can use VSWhere to find MSBuild.
From the wiki page:
With Visual Studio 2017 Update 2 or newer installed, you can find vswhere at %ProgramFiles(x86)%Microsoft Visual StudioInstallervswhere.exe, or to make sure it's always available in your repo see Installing for an option using NuGet.
powershell example that you'll need to adapt to Bash if you want to keep using bash
$path = vswhere -latest -products * -requires Microsoft.Component.MSBuild -property installationPath
if ($path) {
$path = join-path $path 'MSBuild15.0BinMSBuild.exe'
if (test-path $path) {
& $path $args
}
}
EDIT: If you have the .NET Core SDK installed, you can just use dotnet msbuild
to run msbuild, since installing the .NET Core SDK puts dotnet.exe
on the path. The only real difference between using a Visual Studio MSBuild and the .NET Core MSBuild is that the extensions path will be different. But if your build doesn't depend on an MSBuild extension, then using dotnet msbuild
works without having to find the path to an executable first.
edited Jan 6 at 19:10
answered Jan 4 at 21:40
zivkanzivkan
1,8541918
1,8541918
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54037254%2fhow-to-get-the-msbuild-path-returned-from-nuget-exe-restore%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5 l DC6pCNk9ehFG joblDysM3zs,D1 fr,FQj6ggAeB8j,G48N 3GnXRG6j8 xzj1XBNofZkUnGcSQFb1ZH NOyv,nM,jusM4HMfBu n