R Yahoo Fantasy API - Permission
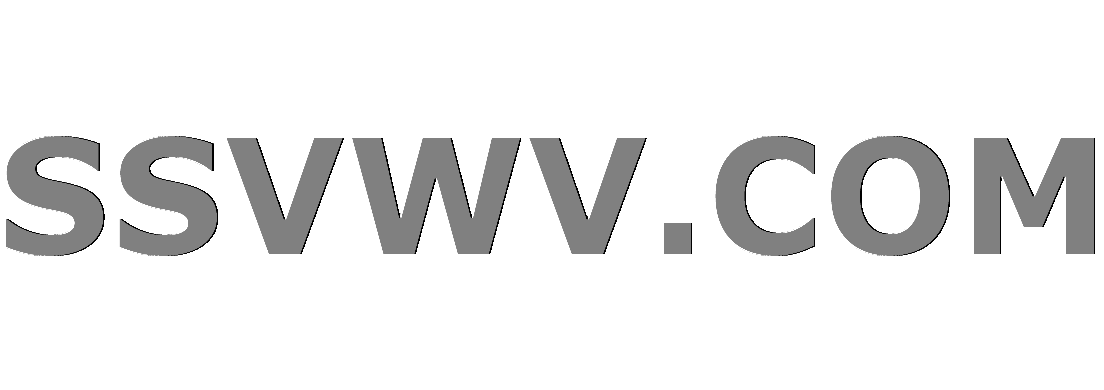
Multi tool use
I am having issues using the Yahoo Fantasy API. Using examples here and here, I have developed a pretty hacky solution to get my connection set.
First, I create an oauth1 token with the following code:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
oauth_endpoints("yahoo")
myapp <- oauth_app("yahoo", key = cKey, secret = cSecret)
token <- oauth1.0_token(oauth_endpoints("yahoo"), myapp)
Then I have another piece of code that gets me a signature:
yahoo <-oauth_endpoints("yahoo")
myapp <- oauth_app("yahoo", key=cKey, secret=cSecret)
yahoo_token<- oauth2.0_token(yahoo, myapp, cache=T, use_oob = T)
sig <- sign_oauth1.0(myapp, yahoo_token$oauth_token, yahoo_token$oauth_token_secret)
It seems to me like I should only need one of these to access the API, but I can't make it work with just one.
Anyways, this does allow me to get my connection set up. In order to access the API, I need the game ID. Per instructions in one of the above-linked walkthroughs, I use this code to do that:
page_mlb <-GET("http://fantasysports.yahooapis.com/fantasy/v2/game/mlb?format=json", sig)
page_mlb_parse <- content(page_mlb, as="parsed", encoding="utf-8")
game_key <- page_mlb_parse[["fantasy_content"]][["game"]][[1]][["game_key"]]
That game_key
ends up being 378
. Thus, I should be able to access something like the league standings for my league using the game key I just found along with my league ID of 94107
which is unique to my league.
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "http://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL<-paste(baseURL, leagueKey, "/standings", sep="")
standings_page <- GET(standingsURL,sig)
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
But when I print that to the screen, I get:
> standings_parse
{xml_document}
<error lang="en-us" uri="http://fantasysports.yahooapis.com/fantasy/v2/league/378.l.94107/standings" xmlns:yahoo="http://www.yahooapis.com/v1/base.rng" xmlns="http://www.yahooapis.com/v1/base.rng">
[1] <description>You are not allowed to view this page because you are not in this league.</description>
[2] <detail/>
The response: You are not allowed to view this page because you are not in this league
is what has me hung up here. I am using the same Yahoo login to create the API that I used to set up my fantasy team.
Any suggestions?
r oauth yahoo-api
add a comment |
I am having issues using the Yahoo Fantasy API. Using examples here and here, I have developed a pretty hacky solution to get my connection set.
First, I create an oauth1 token with the following code:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
oauth_endpoints("yahoo")
myapp <- oauth_app("yahoo", key = cKey, secret = cSecret)
token <- oauth1.0_token(oauth_endpoints("yahoo"), myapp)
Then I have another piece of code that gets me a signature:
yahoo <-oauth_endpoints("yahoo")
myapp <- oauth_app("yahoo", key=cKey, secret=cSecret)
yahoo_token<- oauth2.0_token(yahoo, myapp, cache=T, use_oob = T)
sig <- sign_oauth1.0(myapp, yahoo_token$oauth_token, yahoo_token$oauth_token_secret)
It seems to me like I should only need one of these to access the API, but I can't make it work with just one.
Anyways, this does allow me to get my connection set up. In order to access the API, I need the game ID. Per instructions in one of the above-linked walkthroughs, I use this code to do that:
page_mlb <-GET("http://fantasysports.yahooapis.com/fantasy/v2/game/mlb?format=json", sig)
page_mlb_parse <- content(page_mlb, as="parsed", encoding="utf-8")
game_key <- page_mlb_parse[["fantasy_content"]][["game"]][[1]][["game_key"]]
That game_key
ends up being 378
. Thus, I should be able to access something like the league standings for my league using the game key I just found along with my league ID of 94107
which is unique to my league.
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "http://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL<-paste(baseURL, leagueKey, "/standings", sep="")
standings_page <- GET(standingsURL,sig)
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
But when I print that to the screen, I get:
> standings_parse
{xml_document}
<error lang="en-us" uri="http://fantasysports.yahooapis.com/fantasy/v2/league/378.l.94107/standings" xmlns:yahoo="http://www.yahooapis.com/v1/base.rng" xmlns="http://www.yahooapis.com/v1/base.rng">
[1] <description>You are not allowed to view this page because you are not in this league.</description>
[2] <detail/>
The response: You are not allowed to view this page because you are not in this league
is what has me hung up here. I am using the same Yahoo login to create the API that I used to set up my fantasy team.
Any suggestions?
r oauth yahoo-api
I have the same problem. Have you made any progress on this issue?
– Phil
Aug 26 '18 at 23:51
@Phil, nope, no progress to report.
– Nick Criswell
Aug 27 '18 at 12:43
add a comment |
I am having issues using the Yahoo Fantasy API. Using examples here and here, I have developed a pretty hacky solution to get my connection set.
First, I create an oauth1 token with the following code:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
oauth_endpoints("yahoo")
myapp <- oauth_app("yahoo", key = cKey, secret = cSecret)
token <- oauth1.0_token(oauth_endpoints("yahoo"), myapp)
Then I have another piece of code that gets me a signature:
yahoo <-oauth_endpoints("yahoo")
myapp <- oauth_app("yahoo", key=cKey, secret=cSecret)
yahoo_token<- oauth2.0_token(yahoo, myapp, cache=T, use_oob = T)
sig <- sign_oauth1.0(myapp, yahoo_token$oauth_token, yahoo_token$oauth_token_secret)
It seems to me like I should only need one of these to access the API, but I can't make it work with just one.
Anyways, this does allow me to get my connection set up. In order to access the API, I need the game ID. Per instructions in one of the above-linked walkthroughs, I use this code to do that:
page_mlb <-GET("http://fantasysports.yahooapis.com/fantasy/v2/game/mlb?format=json", sig)
page_mlb_parse <- content(page_mlb, as="parsed", encoding="utf-8")
game_key <- page_mlb_parse[["fantasy_content"]][["game"]][[1]][["game_key"]]
That game_key
ends up being 378
. Thus, I should be able to access something like the league standings for my league using the game key I just found along with my league ID of 94107
which is unique to my league.
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "http://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL<-paste(baseURL, leagueKey, "/standings", sep="")
standings_page <- GET(standingsURL,sig)
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
But when I print that to the screen, I get:
> standings_parse
{xml_document}
<error lang="en-us" uri="http://fantasysports.yahooapis.com/fantasy/v2/league/378.l.94107/standings" xmlns:yahoo="http://www.yahooapis.com/v1/base.rng" xmlns="http://www.yahooapis.com/v1/base.rng">
[1] <description>You are not allowed to view this page because you are not in this league.</description>
[2] <detail/>
The response: You are not allowed to view this page because you are not in this league
is what has me hung up here. I am using the same Yahoo login to create the API that I used to set up my fantasy team.
Any suggestions?
r oauth yahoo-api
I am having issues using the Yahoo Fantasy API. Using examples here and here, I have developed a pretty hacky solution to get my connection set.
First, I create an oauth1 token with the following code:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
oauth_endpoints("yahoo")
myapp <- oauth_app("yahoo", key = cKey, secret = cSecret)
token <- oauth1.0_token(oauth_endpoints("yahoo"), myapp)
Then I have another piece of code that gets me a signature:
yahoo <-oauth_endpoints("yahoo")
myapp <- oauth_app("yahoo", key=cKey, secret=cSecret)
yahoo_token<- oauth2.0_token(yahoo, myapp, cache=T, use_oob = T)
sig <- sign_oauth1.0(myapp, yahoo_token$oauth_token, yahoo_token$oauth_token_secret)
It seems to me like I should only need one of these to access the API, but I can't make it work with just one.
Anyways, this does allow me to get my connection set up. In order to access the API, I need the game ID. Per instructions in one of the above-linked walkthroughs, I use this code to do that:
page_mlb <-GET("http://fantasysports.yahooapis.com/fantasy/v2/game/mlb?format=json", sig)
page_mlb_parse <- content(page_mlb, as="parsed", encoding="utf-8")
game_key <- page_mlb_parse[["fantasy_content"]][["game"]][[1]][["game_key"]]
That game_key
ends up being 378
. Thus, I should be able to access something like the league standings for my league using the game key I just found along with my league ID of 94107
which is unique to my league.
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "http://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL<-paste(baseURL, leagueKey, "/standings", sep="")
standings_page <- GET(standingsURL,sig)
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
But when I print that to the screen, I get:
> standings_parse
{xml_document}
<error lang="en-us" uri="http://fantasysports.yahooapis.com/fantasy/v2/league/378.l.94107/standings" xmlns:yahoo="http://www.yahooapis.com/v1/base.rng" xmlns="http://www.yahooapis.com/v1/base.rng">
[1] <description>You are not allowed to view this page because you are not in this league.</description>
[2] <detail/>
The response: You are not allowed to view this page because you are not in this league
is what has me hung up here. I am using the same Yahoo login to create the API that I used to set up my fantasy team.
Any suggestions?
r oauth yahoo-api
r oauth yahoo-api
asked Apr 7 '18 at 17:23
Nick CriswellNick Criswell
1,2201821
1,2201821
I have the same problem. Have you made any progress on this issue?
– Phil
Aug 26 '18 at 23:51
@Phil, nope, no progress to report.
– Nick Criswell
Aug 27 '18 at 12:43
add a comment |
I have the same problem. Have you made any progress on this issue?
– Phil
Aug 26 '18 at 23:51
@Phil, nope, no progress to report.
– Nick Criswell
Aug 27 '18 at 12:43
I have the same problem. Have you made any progress on this issue?
– Phil
Aug 26 '18 at 23:51
I have the same problem. Have you made any progress on this issue?
– Phil
Aug 26 '18 at 23:51
@Phil, nope, no progress to report.
– Nick Criswell
Aug 27 '18 at 12:43
@Phil, nope, no progress to report.
– Nick Criswell
Aug 27 '18 at 12:43
add a comment |
2 Answers
2
active
oldest
votes
Not sure if you're still having issues, but I struggled with this for a while until I found the solution.
Issue: Yahoo has switched from OAuth1.0 to OAuth2.0. That means many of the sample scripts you find online -- nearly all of which were created before this change -- are no longer functional.
In the sample code you've provided, it looks like both 1.0 and 2.0 are being used (one interesting note here: 1.0 functionality is used to create the "sig" variable -- a signed token -- which is no longer necessary in 2.0).
Here's a rewrite that should accomplish what you're trying to do without the pesky authorization issues:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
b_url <- "https://fantasysports.yahooapis.com" #base url
#Create Endpoint
yahoo <- httr::oauth_endpoint(authorize = "https://api.login.yahoo.com/oauth2/request_auth"
, access = "https://api.login.yahoo.com/oauth2/get_token"
, base_url = b_url)
#Create App
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
#Open Browser to Authorization Code
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r"
, redirect_uri = yahoo_app$redirect_uri))
#Create Token
yahoo_token <- httr::oauth2.0_access_token(yahoo,yahoo_app,code="[ENTER CODE FROM BROWSER HERE]")
save(yahoo_token,file="yahoo_token.Rdata")
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL <- paste(baseURL, leagueKey, "/standings")
standings_page <- GET(standingsURL,
add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
Thank you, this is getting me much further. I think there might be a change needed on theyahoo_app
. Should that just bemyapp
? When I make that change, things seem to go a little more smoothly. Thestandings_parse
is returning a non-nonsensical result, but it is better than the access denied I was getting before. I probably just need to read more through the API docs.
– Nick Criswell
Oct 3 '18 at 21:59
add a comment |
I was having similar struggles and I used Birchman's answer and a lot of trial and error.
Here's how I solved it.
Once you key and secret code from yahoo, you can do the following. Of course, I've not shown mine.
options("httr_oob_default" = T)
cKey <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
cSecret <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
yahoo <- httr::oauth_endpoint(authorize ="https://api.login.yahoo.com/oauth2/request_auth", access = "https://api.login.yahoo.com/oauth2/get_token", base_url = "https://fantasysports.yahooapis.com")
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
Now, when you do the next section, you'll have a browser pop up. You'll have to copy and paste the code provided.
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r", redirect_uri = myapp$redirect_uri))
passcode = "xxxxxxx"
yahoo_token <- httr::oauth2.0_access_token(yahoo,myapp,code=passcode)
The next section helps you build the url to get the data you need.
standings_page <- GET("https://fantasysports.yahooapis.com/fantasy/v2/game/nfl", add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
XMLstandings<- content(standings_page, as="parsed", encoding="utf-8")
doc<-xmlTreeParse(XMLstandings, useInternal=TRUE)
myList<- xmlToList(xmlRoot(doc))
game_key = myList$game$game_key
game_key
Now, the next chunk of code will extract the data you are looking for.
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
leagueID <- "1244633"
tag <- "/scoreboard;week=1"
standingsURL <-paste0(baseURL,game_key,".l.",leagueID,tag)
standings_page <- GET(standingsURL, add_headers(Authorization =
paste0("Bearer ", yahoo_token$access_token)))
XMLstandings <- content(standings_page, as = "parsed", encoding = "utf-8")
doc <- xmlTreeParse(XMLstandings, useInternal = TRUE)
myList <- xmlToList(xmlRoot(doc))
For more details, here is a Fantasy Football Blog Post I wrote.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49709988%2fr-yahoo-fantasy-api-permission%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Not sure if you're still having issues, but I struggled with this for a while until I found the solution.
Issue: Yahoo has switched from OAuth1.0 to OAuth2.0. That means many of the sample scripts you find online -- nearly all of which were created before this change -- are no longer functional.
In the sample code you've provided, it looks like both 1.0 and 2.0 are being used (one interesting note here: 1.0 functionality is used to create the "sig" variable -- a signed token -- which is no longer necessary in 2.0).
Here's a rewrite that should accomplish what you're trying to do without the pesky authorization issues:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
b_url <- "https://fantasysports.yahooapis.com" #base url
#Create Endpoint
yahoo <- httr::oauth_endpoint(authorize = "https://api.login.yahoo.com/oauth2/request_auth"
, access = "https://api.login.yahoo.com/oauth2/get_token"
, base_url = b_url)
#Create App
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
#Open Browser to Authorization Code
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r"
, redirect_uri = yahoo_app$redirect_uri))
#Create Token
yahoo_token <- httr::oauth2.0_access_token(yahoo,yahoo_app,code="[ENTER CODE FROM BROWSER HERE]")
save(yahoo_token,file="yahoo_token.Rdata")
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL <- paste(baseURL, leagueKey, "/standings")
standings_page <- GET(standingsURL,
add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
Thank you, this is getting me much further. I think there might be a change needed on theyahoo_app
. Should that just bemyapp
? When I make that change, things seem to go a little more smoothly. Thestandings_parse
is returning a non-nonsensical result, but it is better than the access denied I was getting before. I probably just need to read more through the API docs.
– Nick Criswell
Oct 3 '18 at 21:59
add a comment |
Not sure if you're still having issues, but I struggled with this for a while until I found the solution.
Issue: Yahoo has switched from OAuth1.0 to OAuth2.0. That means many of the sample scripts you find online -- nearly all of which were created before this change -- are no longer functional.
In the sample code you've provided, it looks like both 1.0 and 2.0 are being used (one interesting note here: 1.0 functionality is used to create the "sig" variable -- a signed token -- which is no longer necessary in 2.0).
Here's a rewrite that should accomplish what you're trying to do without the pesky authorization issues:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
b_url <- "https://fantasysports.yahooapis.com" #base url
#Create Endpoint
yahoo <- httr::oauth_endpoint(authorize = "https://api.login.yahoo.com/oauth2/request_auth"
, access = "https://api.login.yahoo.com/oauth2/get_token"
, base_url = b_url)
#Create App
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
#Open Browser to Authorization Code
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r"
, redirect_uri = yahoo_app$redirect_uri))
#Create Token
yahoo_token <- httr::oauth2.0_access_token(yahoo,yahoo_app,code="[ENTER CODE FROM BROWSER HERE]")
save(yahoo_token,file="yahoo_token.Rdata")
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL <- paste(baseURL, leagueKey, "/standings")
standings_page <- GET(standingsURL,
add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
Thank you, this is getting me much further. I think there might be a change needed on theyahoo_app
. Should that just bemyapp
? When I make that change, things seem to go a little more smoothly. Thestandings_parse
is returning a non-nonsensical result, but it is better than the access denied I was getting before. I probably just need to read more through the API docs.
– Nick Criswell
Oct 3 '18 at 21:59
add a comment |
Not sure if you're still having issues, but I struggled with this for a while until I found the solution.
Issue: Yahoo has switched from OAuth1.0 to OAuth2.0. That means many of the sample scripts you find online -- nearly all of which were created before this change -- are no longer functional.
In the sample code you've provided, it looks like both 1.0 and 2.0 are being used (one interesting note here: 1.0 functionality is used to create the "sig" variable -- a signed token -- which is no longer necessary in 2.0).
Here's a rewrite that should accomplish what you're trying to do without the pesky authorization issues:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
b_url <- "https://fantasysports.yahooapis.com" #base url
#Create Endpoint
yahoo <- httr::oauth_endpoint(authorize = "https://api.login.yahoo.com/oauth2/request_auth"
, access = "https://api.login.yahoo.com/oauth2/get_token"
, base_url = b_url)
#Create App
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
#Open Browser to Authorization Code
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r"
, redirect_uri = yahoo_app$redirect_uri))
#Create Token
yahoo_token <- httr::oauth2.0_access_token(yahoo,yahoo_app,code="[ENTER CODE FROM BROWSER HERE]")
save(yahoo_token,file="yahoo_token.Rdata")
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL <- paste(baseURL, leagueKey, "/standings")
standings_page <- GET(standingsURL,
add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
Not sure if you're still having issues, but I struggled with this for a while until I found the solution.
Issue: Yahoo has switched from OAuth1.0 to OAuth2.0. That means many of the sample scripts you find online -- nearly all of which were created before this change -- are no longer functional.
In the sample code you've provided, it looks like both 1.0 and 2.0 are being used (one interesting note here: 1.0 functionality is used to create the "sig" variable -- a signed token -- which is no longer necessary in 2.0).
Here's a rewrite that should accomplish what you're trying to do without the pesky authorization issues:
library(httr)
cKey <- "mykey"
cSecret <- "mysecret"
b_url <- "https://fantasysports.yahooapis.com" #base url
#Create Endpoint
yahoo <- httr::oauth_endpoint(authorize = "https://api.login.yahoo.com/oauth2/request_auth"
, access = "https://api.login.yahoo.com/oauth2/get_token"
, base_url = b_url)
#Create App
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
#Open Browser to Authorization Code
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r"
, redirect_uri = yahoo_app$redirect_uri))
#Create Token
yahoo_token <- httr::oauth2.0_access_token(yahoo,yahoo_app,code="[ENTER CODE FROM BROWSER HERE]")
save(yahoo_token,file="yahoo_token.Rdata")
leagueKey <- paste0(game_key,'.l.',lg_id)
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
standingsURL <- paste(baseURL, leagueKey, "/standings")
standings_page <- GET(standingsURL,
add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
standings_parse <- content(standings_page, as = "parsed", encoding = "utf-8")
answered Oct 1 '18 at 20:48
BirchmanBirchman
111
111
Thank you, this is getting me much further. I think there might be a change needed on theyahoo_app
. Should that just bemyapp
? When I make that change, things seem to go a little more smoothly. Thestandings_parse
is returning a non-nonsensical result, but it is better than the access denied I was getting before. I probably just need to read more through the API docs.
– Nick Criswell
Oct 3 '18 at 21:59
add a comment |
Thank you, this is getting me much further. I think there might be a change needed on theyahoo_app
. Should that just bemyapp
? When I make that change, things seem to go a little more smoothly. Thestandings_parse
is returning a non-nonsensical result, but it is better than the access denied I was getting before. I probably just need to read more through the API docs.
– Nick Criswell
Oct 3 '18 at 21:59
Thank you, this is getting me much further. I think there might be a change needed on the
yahoo_app
. Should that just be myapp
? When I make that change, things seem to go a little more smoothly. The standings_parse
is returning a non-nonsensical result, but it is better than the access denied I was getting before. I probably just need to read more through the API docs.– Nick Criswell
Oct 3 '18 at 21:59
Thank you, this is getting me much further. I think there might be a change needed on the
yahoo_app
. Should that just be myapp
? When I make that change, things seem to go a little more smoothly. The standings_parse
is returning a non-nonsensical result, but it is better than the access denied I was getting before. I probably just need to read more through the API docs.– Nick Criswell
Oct 3 '18 at 21:59
add a comment |
I was having similar struggles and I used Birchman's answer and a lot of trial and error.
Here's how I solved it.
Once you key and secret code from yahoo, you can do the following. Of course, I've not shown mine.
options("httr_oob_default" = T)
cKey <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
cSecret <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
yahoo <- httr::oauth_endpoint(authorize ="https://api.login.yahoo.com/oauth2/request_auth", access = "https://api.login.yahoo.com/oauth2/get_token", base_url = "https://fantasysports.yahooapis.com")
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
Now, when you do the next section, you'll have a browser pop up. You'll have to copy and paste the code provided.
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r", redirect_uri = myapp$redirect_uri))
passcode = "xxxxxxx"
yahoo_token <- httr::oauth2.0_access_token(yahoo,myapp,code=passcode)
The next section helps you build the url to get the data you need.
standings_page <- GET("https://fantasysports.yahooapis.com/fantasy/v2/game/nfl", add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
XMLstandings<- content(standings_page, as="parsed", encoding="utf-8")
doc<-xmlTreeParse(XMLstandings, useInternal=TRUE)
myList<- xmlToList(xmlRoot(doc))
game_key = myList$game$game_key
game_key
Now, the next chunk of code will extract the data you are looking for.
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
leagueID <- "1244633"
tag <- "/scoreboard;week=1"
standingsURL <-paste0(baseURL,game_key,".l.",leagueID,tag)
standings_page <- GET(standingsURL, add_headers(Authorization =
paste0("Bearer ", yahoo_token$access_token)))
XMLstandings <- content(standings_page, as = "parsed", encoding = "utf-8")
doc <- xmlTreeParse(XMLstandings, useInternal = TRUE)
myList <- xmlToList(xmlRoot(doc))
For more details, here is a Fantasy Football Blog Post I wrote.
add a comment |
I was having similar struggles and I used Birchman's answer and a lot of trial and error.
Here's how I solved it.
Once you key and secret code from yahoo, you can do the following. Of course, I've not shown mine.
options("httr_oob_default" = T)
cKey <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
cSecret <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
yahoo <- httr::oauth_endpoint(authorize ="https://api.login.yahoo.com/oauth2/request_auth", access = "https://api.login.yahoo.com/oauth2/get_token", base_url = "https://fantasysports.yahooapis.com")
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
Now, when you do the next section, you'll have a browser pop up. You'll have to copy and paste the code provided.
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r", redirect_uri = myapp$redirect_uri))
passcode = "xxxxxxx"
yahoo_token <- httr::oauth2.0_access_token(yahoo,myapp,code=passcode)
The next section helps you build the url to get the data you need.
standings_page <- GET("https://fantasysports.yahooapis.com/fantasy/v2/game/nfl", add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
XMLstandings<- content(standings_page, as="parsed", encoding="utf-8")
doc<-xmlTreeParse(XMLstandings, useInternal=TRUE)
myList<- xmlToList(xmlRoot(doc))
game_key = myList$game$game_key
game_key
Now, the next chunk of code will extract the data you are looking for.
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
leagueID <- "1244633"
tag <- "/scoreboard;week=1"
standingsURL <-paste0(baseURL,game_key,".l.",leagueID,tag)
standings_page <- GET(standingsURL, add_headers(Authorization =
paste0("Bearer ", yahoo_token$access_token)))
XMLstandings <- content(standings_page, as = "parsed", encoding = "utf-8")
doc <- xmlTreeParse(XMLstandings, useInternal = TRUE)
myList <- xmlToList(xmlRoot(doc))
For more details, here is a Fantasy Football Blog Post I wrote.
add a comment |
I was having similar struggles and I used Birchman's answer and a lot of trial and error.
Here's how I solved it.
Once you key and secret code from yahoo, you can do the following. Of course, I've not shown mine.
options("httr_oob_default" = T)
cKey <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
cSecret <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
yahoo <- httr::oauth_endpoint(authorize ="https://api.login.yahoo.com/oauth2/request_auth", access = "https://api.login.yahoo.com/oauth2/get_token", base_url = "https://fantasysports.yahooapis.com")
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
Now, when you do the next section, you'll have a browser pop up. You'll have to copy and paste the code provided.
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r", redirect_uri = myapp$redirect_uri))
passcode = "xxxxxxx"
yahoo_token <- httr::oauth2.0_access_token(yahoo,myapp,code=passcode)
The next section helps you build the url to get the data you need.
standings_page <- GET("https://fantasysports.yahooapis.com/fantasy/v2/game/nfl", add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
XMLstandings<- content(standings_page, as="parsed", encoding="utf-8")
doc<-xmlTreeParse(XMLstandings, useInternal=TRUE)
myList<- xmlToList(xmlRoot(doc))
game_key = myList$game$game_key
game_key
Now, the next chunk of code will extract the data you are looking for.
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
leagueID <- "1244633"
tag <- "/scoreboard;week=1"
standingsURL <-paste0(baseURL,game_key,".l.",leagueID,tag)
standings_page <- GET(standingsURL, add_headers(Authorization =
paste0("Bearer ", yahoo_token$access_token)))
XMLstandings <- content(standings_page, as = "parsed", encoding = "utf-8")
doc <- xmlTreeParse(XMLstandings, useInternal = TRUE)
myList <- xmlToList(xmlRoot(doc))
For more details, here is a Fantasy Football Blog Post I wrote.
I was having similar struggles and I used Birchman's answer and a lot of trial and error.
Here's how I solved it.
Once you key and secret code from yahoo, you can do the following. Of course, I've not shown mine.
options("httr_oob_default" = T)
cKey <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
cSecret <- "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
yahoo <- httr::oauth_endpoint(authorize ="https://api.login.yahoo.com/oauth2/request_auth", access = "https://api.login.yahoo.com/oauth2/get_token", base_url = "https://fantasysports.yahooapis.com")
myapp <- httr::oauth_app("yahoo", key=cKey, secret = cSecret,redirect_uri = "oob")
Now, when you do the next section, you'll have a browser pop up. You'll have to copy and paste the code provided.
httr::BROWSE(httr::oauth2.0_authorize_url(yahoo, myapp, scope="fspt-r", redirect_uri = myapp$redirect_uri))
passcode = "xxxxxxx"
yahoo_token <- httr::oauth2.0_access_token(yahoo,myapp,code=passcode)
The next section helps you build the url to get the data you need.
standings_page <- GET("https://fantasysports.yahooapis.com/fantasy/v2/game/nfl", add_headers(Authorization=paste0("Bearer ", yahoo_token$access_token)))
XMLstandings<- content(standings_page, as="parsed", encoding="utf-8")
doc<-xmlTreeParse(XMLstandings, useInternal=TRUE)
myList<- xmlToList(xmlRoot(doc))
game_key = myList$game$game_key
game_key
Now, the next chunk of code will extract the data you are looking for.
baseURL <- "https://fantasysports.yahooapis.com/fantasy/v2/league/"
leagueID <- "1244633"
tag <- "/scoreboard;week=1"
standingsURL <-paste0(baseURL,game_key,".l.",leagueID,tag)
standings_page <- GET(standingsURL, add_headers(Authorization =
paste0("Bearer ", yahoo_token$access_token)))
XMLstandings <- content(standings_page, as = "parsed", encoding = "utf-8")
doc <- xmlTreeParse(XMLstandings, useInternal = TRUE)
myList <- xmlToList(xmlRoot(doc))
For more details, here is a Fantasy Football Blog Post I wrote.
edited Jan 3 at 2:39
answered Jan 2 at 1:15
DustyDusty
365
365
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49709988%2fr-yahoo-fantasy-api-permission%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Mnoy2VBkOAQ0Qbfgb,P9L mVX9QSVq40QLjpO7ho2Te3YHQ
I have the same problem. Have you made any progress on this issue?
– Phil
Aug 26 '18 at 23:51
@Phil, nope, no progress to report.
– Nick Criswell
Aug 27 '18 at 12:43