Display URL image on fragment image view using Picasso and Volley
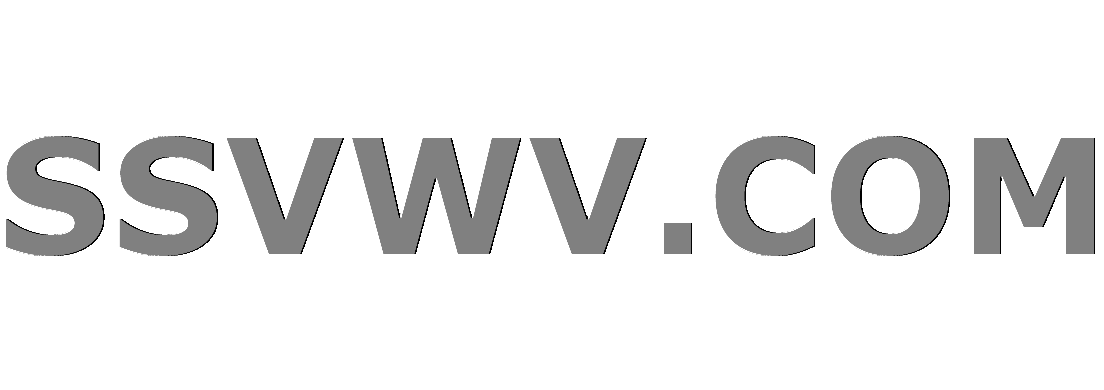
Multi tool use
I was able to load JSON data on textview using Volley but image was handled by Picasso to load it into an ImageView
. Here's my code:
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View v = inflater.inflate(R.layout.fragment_home, container, false);
Context c = getActivity().getApplicationContext();
tvQuote = (TextView) v.findViewById(R.id.quote);
tvAuthor = (TextView) v.findViewById(R.id.author);
Typeface MP = Typeface.createFromAsset(getActivity().getAssets(), "font/MP.ttf");
tvQuote.setTypeface(MP);
tvAuthor.setTypeface(MP);
imageFromURL=(ImageView)v. findViewById(R.id.imgUrl);
getQuote();
loadImageFromURL();
//Picasso.with(getActivity().getApplicationContext()).load(jsonImage).into(imageFromURL);
Log.d("Picasso","Error");
return v;
//return inflater.inflate(R.layout.fragment_home, container, false);
}
Here's my method for loading image from a URL using Volley
private void loadImageFromURL() {
List<Integer> imageList = new ArrayList<Integer>();
imageList.add(R.drawable.ic_action_loader);
//swipeRefreshLayout.setRefreshing(true);
JsonObjectRequest jsonObjReq = new JsonObjectRequest(Request.Method.GET,
imgURL, (String) null, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
try {
JSONObject urls = response.getJSONObject("urls");
String regular = urls.getString("regular");
JSONObject user = response.getJSONObject("user");
String name = user.getString("name");
String username = user.getString("username");
jsonImage = regular;
jsonName = name;
jsonUserName = username;
Picasso.with(getActivity().getApplicationContext())
.load(jsonImage)
//.placeholder(R.drawable.desert)
.into(imageFromURL);
Log.d(TAG, jsonImage);
tvPhotographer.setClickable(true);
tvPhotographer.setMovementMethod(LinkMovementMethod.getInstance());
String authorLink = "Photo By - <a href='https://unsplash.com/@" + jsonUserName + "'>" + jsonName + "</a> / <a href='https://unsplash.com/'>Unsplash</a>";
Spanned result;
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.N) {
result = Html.fromHtml(authorLink, Html.FROM_HTML_MODE_LEGACY);
} else {
result = Html.fromHtml(authorLink);
}
tvPhotographer.setText(result);
} catch (JSONException e) {
e.printStackTrace();
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
VolleyLog.d(TAG, "Error: " + error.getMessage());
}
});
// Adding request to request queue
jsonObjReq.setTag(TAG);
if (mInstance != null) {
// Add a request to your RequestQueue.
mInstance.addToRequestQueue(jsonObjReq);
// Start the queue
mInstance.getRequestQueue().start();
}
}
Texts which are loaded from stringrequest
are loaded perfectly on the textviews with Volley, but only the image is not loaded.
I have tried this method on the main activity and it works. But why isn't it loaded on the fragment part?
java


add a comment |
I was able to load JSON data on textview using Volley but image was handled by Picasso to load it into an ImageView
. Here's my code:
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View v = inflater.inflate(R.layout.fragment_home, container, false);
Context c = getActivity().getApplicationContext();
tvQuote = (TextView) v.findViewById(R.id.quote);
tvAuthor = (TextView) v.findViewById(R.id.author);
Typeface MP = Typeface.createFromAsset(getActivity().getAssets(), "font/MP.ttf");
tvQuote.setTypeface(MP);
tvAuthor.setTypeface(MP);
imageFromURL=(ImageView)v. findViewById(R.id.imgUrl);
getQuote();
loadImageFromURL();
//Picasso.with(getActivity().getApplicationContext()).load(jsonImage).into(imageFromURL);
Log.d("Picasso","Error");
return v;
//return inflater.inflate(R.layout.fragment_home, container, false);
}
Here's my method for loading image from a URL using Volley
private void loadImageFromURL() {
List<Integer> imageList = new ArrayList<Integer>();
imageList.add(R.drawable.ic_action_loader);
//swipeRefreshLayout.setRefreshing(true);
JsonObjectRequest jsonObjReq = new JsonObjectRequest(Request.Method.GET,
imgURL, (String) null, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
try {
JSONObject urls = response.getJSONObject("urls");
String regular = urls.getString("regular");
JSONObject user = response.getJSONObject("user");
String name = user.getString("name");
String username = user.getString("username");
jsonImage = regular;
jsonName = name;
jsonUserName = username;
Picasso.with(getActivity().getApplicationContext())
.load(jsonImage)
//.placeholder(R.drawable.desert)
.into(imageFromURL);
Log.d(TAG, jsonImage);
tvPhotographer.setClickable(true);
tvPhotographer.setMovementMethod(LinkMovementMethod.getInstance());
String authorLink = "Photo By - <a href='https://unsplash.com/@" + jsonUserName + "'>" + jsonName + "</a> / <a href='https://unsplash.com/'>Unsplash</a>";
Spanned result;
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.N) {
result = Html.fromHtml(authorLink, Html.FROM_HTML_MODE_LEGACY);
} else {
result = Html.fromHtml(authorLink);
}
tvPhotographer.setText(result);
} catch (JSONException e) {
e.printStackTrace();
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
VolleyLog.d(TAG, "Error: " + error.getMessage());
}
});
// Adding request to request queue
jsonObjReq.setTag(TAG);
if (mInstance != null) {
// Add a request to your RequestQueue.
mInstance.addToRequestQueue(jsonObjReq);
// Start the queue
mInstance.getRequestQueue().start();
}
}
Texts which are loaded from stringrequest
are loaded perfectly on the textviews with Volley, but only the image is not loaded.
I have tried this method on the main activity and it works. But why isn't it loaded on the fragment part?
java


are you able to get image loading url inregular
? What you are getting injsonImage
?
– pRaNaY
Mar 12 '17 at 12:49
i get the image url in jsonImage...when the control reaches picasso the app crashes
– bluetoro
Mar 12 '17 at 13:13
add a comment |
I was able to load JSON data on textview using Volley but image was handled by Picasso to load it into an ImageView
. Here's my code:
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View v = inflater.inflate(R.layout.fragment_home, container, false);
Context c = getActivity().getApplicationContext();
tvQuote = (TextView) v.findViewById(R.id.quote);
tvAuthor = (TextView) v.findViewById(R.id.author);
Typeface MP = Typeface.createFromAsset(getActivity().getAssets(), "font/MP.ttf");
tvQuote.setTypeface(MP);
tvAuthor.setTypeface(MP);
imageFromURL=(ImageView)v. findViewById(R.id.imgUrl);
getQuote();
loadImageFromURL();
//Picasso.with(getActivity().getApplicationContext()).load(jsonImage).into(imageFromURL);
Log.d("Picasso","Error");
return v;
//return inflater.inflate(R.layout.fragment_home, container, false);
}
Here's my method for loading image from a URL using Volley
private void loadImageFromURL() {
List<Integer> imageList = new ArrayList<Integer>();
imageList.add(R.drawable.ic_action_loader);
//swipeRefreshLayout.setRefreshing(true);
JsonObjectRequest jsonObjReq = new JsonObjectRequest(Request.Method.GET,
imgURL, (String) null, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
try {
JSONObject urls = response.getJSONObject("urls");
String regular = urls.getString("regular");
JSONObject user = response.getJSONObject("user");
String name = user.getString("name");
String username = user.getString("username");
jsonImage = regular;
jsonName = name;
jsonUserName = username;
Picasso.with(getActivity().getApplicationContext())
.load(jsonImage)
//.placeholder(R.drawable.desert)
.into(imageFromURL);
Log.d(TAG, jsonImage);
tvPhotographer.setClickable(true);
tvPhotographer.setMovementMethod(LinkMovementMethod.getInstance());
String authorLink = "Photo By - <a href='https://unsplash.com/@" + jsonUserName + "'>" + jsonName + "</a> / <a href='https://unsplash.com/'>Unsplash</a>";
Spanned result;
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.N) {
result = Html.fromHtml(authorLink, Html.FROM_HTML_MODE_LEGACY);
} else {
result = Html.fromHtml(authorLink);
}
tvPhotographer.setText(result);
} catch (JSONException e) {
e.printStackTrace();
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
VolleyLog.d(TAG, "Error: " + error.getMessage());
}
});
// Adding request to request queue
jsonObjReq.setTag(TAG);
if (mInstance != null) {
// Add a request to your RequestQueue.
mInstance.addToRequestQueue(jsonObjReq);
// Start the queue
mInstance.getRequestQueue().start();
}
}
Texts which are loaded from stringrequest
are loaded perfectly on the textviews with Volley, but only the image is not loaded.
I have tried this method on the main activity and it works. But why isn't it loaded on the fragment part?
java


I was able to load JSON data on textview using Volley but image was handled by Picasso to load it into an ImageView
. Here's my code:
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View v = inflater.inflate(R.layout.fragment_home, container, false);
Context c = getActivity().getApplicationContext();
tvQuote = (TextView) v.findViewById(R.id.quote);
tvAuthor = (TextView) v.findViewById(R.id.author);
Typeface MP = Typeface.createFromAsset(getActivity().getAssets(), "font/MP.ttf");
tvQuote.setTypeface(MP);
tvAuthor.setTypeface(MP);
imageFromURL=(ImageView)v. findViewById(R.id.imgUrl);
getQuote();
loadImageFromURL();
//Picasso.with(getActivity().getApplicationContext()).load(jsonImage).into(imageFromURL);
Log.d("Picasso","Error");
return v;
//return inflater.inflate(R.layout.fragment_home, container, false);
}
Here's my method for loading image from a URL using Volley
private void loadImageFromURL() {
List<Integer> imageList = new ArrayList<Integer>();
imageList.add(R.drawable.ic_action_loader);
//swipeRefreshLayout.setRefreshing(true);
JsonObjectRequest jsonObjReq = new JsonObjectRequest(Request.Method.GET,
imgURL, (String) null, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
try {
JSONObject urls = response.getJSONObject("urls");
String regular = urls.getString("regular");
JSONObject user = response.getJSONObject("user");
String name = user.getString("name");
String username = user.getString("username");
jsonImage = regular;
jsonName = name;
jsonUserName = username;
Picasso.with(getActivity().getApplicationContext())
.load(jsonImage)
//.placeholder(R.drawable.desert)
.into(imageFromURL);
Log.d(TAG, jsonImage);
tvPhotographer.setClickable(true);
tvPhotographer.setMovementMethod(LinkMovementMethod.getInstance());
String authorLink = "Photo By - <a href='https://unsplash.com/@" + jsonUserName + "'>" + jsonName + "</a> / <a href='https://unsplash.com/'>Unsplash</a>";
Spanned result;
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.N) {
result = Html.fromHtml(authorLink, Html.FROM_HTML_MODE_LEGACY);
} else {
result = Html.fromHtml(authorLink);
}
tvPhotographer.setText(result);
} catch (JSONException e) {
e.printStackTrace();
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
VolleyLog.d(TAG, "Error: " + error.getMessage());
}
});
// Adding request to request queue
jsonObjReq.setTag(TAG);
if (mInstance != null) {
// Add a request to your RequestQueue.
mInstance.addToRequestQueue(jsonObjReq);
// Start the queue
mInstance.getRequestQueue().start();
}
}
Texts which are loaded from stringrequest
are loaded perfectly on the textviews with Volley, but only the image is not loaded.
I have tried this method on the main activity and it works. But why isn't it loaded on the fragment part?
java


java


edited Mar 12 '17 at 15:26


mustaccio
14.3k83738
14.3k83738
asked Mar 12 '17 at 12:43
bluetorobluetoro
82
82
are you able to get image loading url inregular
? What you are getting injsonImage
?
– pRaNaY
Mar 12 '17 at 12:49
i get the image url in jsonImage...when the control reaches picasso the app crashes
– bluetoro
Mar 12 '17 at 13:13
add a comment |
are you able to get image loading url inregular
? What you are getting injsonImage
?
– pRaNaY
Mar 12 '17 at 12:49
i get the image url in jsonImage...when the control reaches picasso the app crashes
– bluetoro
Mar 12 '17 at 13:13
are you able to get image loading url in
regular
? What you are getting in jsonImage
?– pRaNaY
Mar 12 '17 at 12:49
are you able to get image loading url in
regular
? What you are getting in jsonImage
?– pRaNaY
Mar 12 '17 at 12:49
i get the image url in jsonImage...when the control reaches picasso the app crashes
– bluetoro
Mar 12 '17 at 13:13
i get the image url in jsonImage...when the control reaches picasso the app crashes
– bluetoro
Mar 12 '17 at 13:13
add a comment |
1 Answer
1
active
oldest
votes
do it like this
Picasso.with(getContext())
.load(jsonImage)
.into(imageFromURL);
i have tried your code and its not working neither does with getActivity() nor getApplicationContext() nor getActivity().getApplicationContext()
– bluetoro
Mar 12 '17 at 13:14
working great now. Thanks.
– bluetoro
Mar 14 '17 at 15:43
@bluetoro You're welcome man
– Sepehr
Mar 14 '17 at 20:14
I'm using the same code on a fragment and it is not working for me. Please help.
– Maryoomi1
Dec 6 '18 at 7:41
@bluetoro how did you solve it?
– Maryoomi1
Dec 6 '18 at 7:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f42747531%2fdisplay-url-image-on-fragment-image-view-using-picasso-and-volley%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
do it like this
Picasso.with(getContext())
.load(jsonImage)
.into(imageFromURL);
i have tried your code and its not working neither does with getActivity() nor getApplicationContext() nor getActivity().getApplicationContext()
– bluetoro
Mar 12 '17 at 13:14
working great now. Thanks.
– bluetoro
Mar 14 '17 at 15:43
@bluetoro You're welcome man
– Sepehr
Mar 14 '17 at 20:14
I'm using the same code on a fragment and it is not working for me. Please help.
– Maryoomi1
Dec 6 '18 at 7:41
@bluetoro how did you solve it?
– Maryoomi1
Dec 6 '18 at 7:42
add a comment |
do it like this
Picasso.with(getContext())
.load(jsonImage)
.into(imageFromURL);
i have tried your code and its not working neither does with getActivity() nor getApplicationContext() nor getActivity().getApplicationContext()
– bluetoro
Mar 12 '17 at 13:14
working great now. Thanks.
– bluetoro
Mar 14 '17 at 15:43
@bluetoro You're welcome man
– Sepehr
Mar 14 '17 at 20:14
I'm using the same code on a fragment and it is not working for me. Please help.
– Maryoomi1
Dec 6 '18 at 7:41
@bluetoro how did you solve it?
– Maryoomi1
Dec 6 '18 at 7:42
add a comment |
do it like this
Picasso.with(getContext())
.load(jsonImage)
.into(imageFromURL);
do it like this
Picasso.with(getContext())
.load(jsonImage)
.into(imageFromURL);
answered Mar 12 '17 at 13:04
SepehrSepehr
218211
218211
i have tried your code and its not working neither does with getActivity() nor getApplicationContext() nor getActivity().getApplicationContext()
– bluetoro
Mar 12 '17 at 13:14
working great now. Thanks.
– bluetoro
Mar 14 '17 at 15:43
@bluetoro You're welcome man
– Sepehr
Mar 14 '17 at 20:14
I'm using the same code on a fragment and it is not working for me. Please help.
– Maryoomi1
Dec 6 '18 at 7:41
@bluetoro how did you solve it?
– Maryoomi1
Dec 6 '18 at 7:42
add a comment |
i have tried your code and its not working neither does with getActivity() nor getApplicationContext() nor getActivity().getApplicationContext()
– bluetoro
Mar 12 '17 at 13:14
working great now. Thanks.
– bluetoro
Mar 14 '17 at 15:43
@bluetoro You're welcome man
– Sepehr
Mar 14 '17 at 20:14
I'm using the same code on a fragment and it is not working for me. Please help.
– Maryoomi1
Dec 6 '18 at 7:41
@bluetoro how did you solve it?
– Maryoomi1
Dec 6 '18 at 7:42
i have tried your code and its not working neither does with getActivity() nor getApplicationContext() nor getActivity().getApplicationContext()
– bluetoro
Mar 12 '17 at 13:14
i have tried your code and its not working neither does with getActivity() nor getApplicationContext() nor getActivity().getApplicationContext()
– bluetoro
Mar 12 '17 at 13:14
working great now. Thanks.
– bluetoro
Mar 14 '17 at 15:43
working great now. Thanks.
– bluetoro
Mar 14 '17 at 15:43
@bluetoro You're welcome man
– Sepehr
Mar 14 '17 at 20:14
@bluetoro You're welcome man
– Sepehr
Mar 14 '17 at 20:14
I'm using the same code on a fragment and it is not working for me. Please help.
– Maryoomi1
Dec 6 '18 at 7:41
I'm using the same code on a fragment and it is not working for me. Please help.
– Maryoomi1
Dec 6 '18 at 7:41
@bluetoro how did you solve it?
– Maryoomi1
Dec 6 '18 at 7:42
@bluetoro how did you solve it?
– Maryoomi1
Dec 6 '18 at 7:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f42747531%2fdisplay-url-image-on-fragment-image-view-using-picasso-and-volley%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pBSJnRehLl,LMfta ZXAy8rK7JLSeTG cY8D v fo,1vhrF
are you able to get image loading url in
regular
? What you are getting injsonImage
?– pRaNaY
Mar 12 '17 at 12:49
i get the image url in jsonImage...when the control reaches picasso the app crashes
– bluetoro
Mar 12 '17 at 13:13