C++ : good practice of declare map of custom type
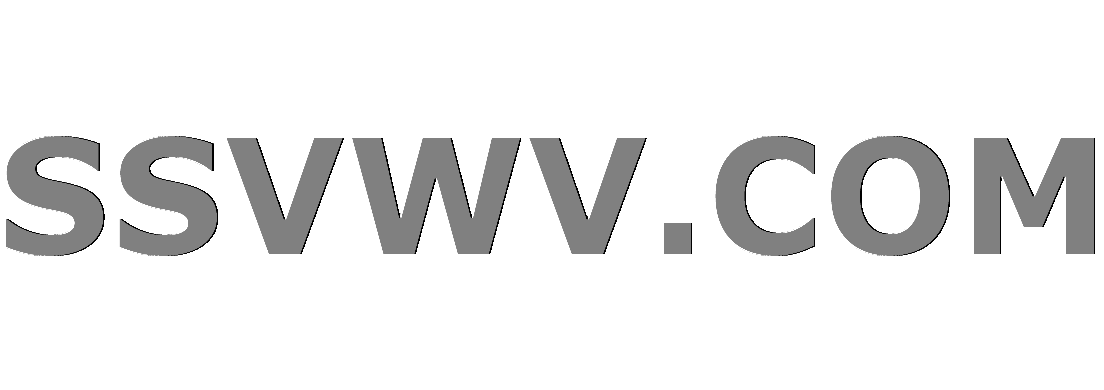
Multi tool use
I am trying to define a map like below within my MyTreeNode
class:
std::unordered_map<int, MyTreeNode> children_map;
This seems to be fine. However, I am wondering would it be better to define the map using the pointer like?
std::unordered_map<int, MyTreeNode*> children_map;
For my specific case, I have
class MyTreeNode {
// some code
std::unordered_map<int, MyTreeNode> children_map; // line_1
// std::unordered_map<int, MyTreeNode*> children_map; // line_2
}
Is there a good practice for such scenario? Should I use line_1 or line_2? Thanks!
c++ pointers treenode
|
show 5 more comments
I am trying to define a map like below within my MyTreeNode
class:
std::unordered_map<int, MyTreeNode> children_map;
This seems to be fine. However, I am wondering would it be better to define the map using the pointer like?
std::unordered_map<int, MyTreeNode*> children_map;
For my specific case, I have
class MyTreeNode {
// some code
std::unordered_map<int, MyTreeNode> children_map; // line_1
// std::unordered_map<int, MyTreeNode*> children_map; // line_2
}
Is there a good practice for such scenario? Should I use line_1 or line_2? Thanks!
c++ pointers treenode
7
That depends entirely on the context. If you already have the instances ofMyTreeNode
stored e.g. in a vector and only want to use thestd::unordered_map
as a kind of index into the vector then use the second version. If the map owns theMyTreeNode
instances then use the first version.
– Corristo
Dec 31 '18 at 23:19
1
@edamame - Um..... Is this supposed to be a map that holds custom types, or is it some device that holds entities in special clothing?
– enhzflep
Dec 31 '18 at 23:26
@enhzflep it is a map that holds custom types. Thanks!
– Edamame
Dec 31 '18 at 23:31
2
If you are able to do either then use the first one (don't use pointers if you don't have to). Otherwise they have different uses.
– Galik
Dec 31 '18 at 23:45
1
If you are relying onunordered_map
for your search tree, why not just use a singleunordered_map
for all of your nodes? What advantage does a tree structure give you for children that a singleunordered_map
would not be able to provide?
– jxh
Dec 31 '18 at 23:57
|
show 5 more comments
I am trying to define a map like below within my MyTreeNode
class:
std::unordered_map<int, MyTreeNode> children_map;
This seems to be fine. However, I am wondering would it be better to define the map using the pointer like?
std::unordered_map<int, MyTreeNode*> children_map;
For my specific case, I have
class MyTreeNode {
// some code
std::unordered_map<int, MyTreeNode> children_map; // line_1
// std::unordered_map<int, MyTreeNode*> children_map; // line_2
}
Is there a good practice for such scenario? Should I use line_1 or line_2? Thanks!
c++ pointers treenode
I am trying to define a map like below within my MyTreeNode
class:
std::unordered_map<int, MyTreeNode> children_map;
This seems to be fine. However, I am wondering would it be better to define the map using the pointer like?
std::unordered_map<int, MyTreeNode*> children_map;
For my specific case, I have
class MyTreeNode {
// some code
std::unordered_map<int, MyTreeNode> children_map; // line_1
// std::unordered_map<int, MyTreeNode*> children_map; // line_2
}
Is there a good practice for such scenario? Should I use line_1 or line_2? Thanks!
c++ pointers treenode
c++ pointers treenode
edited Dec 31 '18 at 23:40
Edamame
asked Dec 31 '18 at 23:17
EdamameEdamame
4,7252379157
4,7252379157
7
That depends entirely on the context. If you already have the instances ofMyTreeNode
stored e.g. in a vector and only want to use thestd::unordered_map
as a kind of index into the vector then use the second version. If the map owns theMyTreeNode
instances then use the first version.
– Corristo
Dec 31 '18 at 23:19
1
@edamame - Um..... Is this supposed to be a map that holds custom types, or is it some device that holds entities in special clothing?
– enhzflep
Dec 31 '18 at 23:26
@enhzflep it is a map that holds custom types. Thanks!
– Edamame
Dec 31 '18 at 23:31
2
If you are able to do either then use the first one (don't use pointers if you don't have to). Otherwise they have different uses.
– Galik
Dec 31 '18 at 23:45
1
If you are relying onunordered_map
for your search tree, why not just use a singleunordered_map
for all of your nodes? What advantage does a tree structure give you for children that a singleunordered_map
would not be able to provide?
– jxh
Dec 31 '18 at 23:57
|
show 5 more comments
7
That depends entirely on the context. If you already have the instances ofMyTreeNode
stored e.g. in a vector and only want to use thestd::unordered_map
as a kind of index into the vector then use the second version. If the map owns theMyTreeNode
instances then use the first version.
– Corristo
Dec 31 '18 at 23:19
1
@edamame - Um..... Is this supposed to be a map that holds custom types, or is it some device that holds entities in special clothing?
– enhzflep
Dec 31 '18 at 23:26
@enhzflep it is a map that holds custom types. Thanks!
– Edamame
Dec 31 '18 at 23:31
2
If you are able to do either then use the first one (don't use pointers if you don't have to). Otherwise they have different uses.
– Galik
Dec 31 '18 at 23:45
1
If you are relying onunordered_map
for your search tree, why not just use a singleunordered_map
for all of your nodes? What advantage does a tree structure give you for children that a singleunordered_map
would not be able to provide?
– jxh
Dec 31 '18 at 23:57
7
7
That depends entirely on the context. If you already have the instances of
MyTreeNode
stored e.g. in a vector and only want to use the std::unordered_map
as a kind of index into the vector then use the second version. If the map owns the MyTreeNode
instances then use the first version.– Corristo
Dec 31 '18 at 23:19
That depends entirely on the context. If you already have the instances of
MyTreeNode
stored e.g. in a vector and only want to use the std::unordered_map
as a kind of index into the vector then use the second version. If the map owns the MyTreeNode
instances then use the first version.– Corristo
Dec 31 '18 at 23:19
1
1
@edamame - Um..... Is this supposed to be a map that holds custom types, or is it some device that holds entities in special clothing?
– enhzflep
Dec 31 '18 at 23:26
@edamame - Um..... Is this supposed to be a map that holds custom types, or is it some device that holds entities in special clothing?
– enhzflep
Dec 31 '18 at 23:26
@enhzflep it is a map that holds custom types. Thanks!
– Edamame
Dec 31 '18 at 23:31
@enhzflep it is a map that holds custom types. Thanks!
– Edamame
Dec 31 '18 at 23:31
2
2
If you are able to do either then use the first one (don't use pointers if you don't have to). Otherwise they have different uses.
– Galik
Dec 31 '18 at 23:45
If you are able to do either then use the first one (don't use pointers if you don't have to). Otherwise they have different uses.
– Galik
Dec 31 '18 at 23:45
1
1
If you are relying on
unordered_map
for your search tree, why not just use a single unordered_map
for all of your nodes? What advantage does a tree structure give you for children that a single unordered_map
would not be able to provide?– jxh
Dec 31 '18 at 23:57
If you are relying on
unordered_map
for your search tree, why not just use a single unordered_map
for all of your nodes? What advantage does a tree structure give you for children that a single unordered_map
would not be able to provide?– jxh
Dec 31 '18 at 23:57
|
show 5 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53992078%2fc-good-practice-of-declare-map-of-custom-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53992078%2fc-good-practice-of-declare-map-of-custom-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DBEZ57JLA4SfFpBeQ5mDA6jJiKtincsVPxOm,4fIBirNrJBN,REMZ,fX,Xe7foc8iVG S,RW,YFS UT8gfBor
7
That depends entirely on the context. If you already have the instances of
MyTreeNode
stored e.g. in a vector and only want to use thestd::unordered_map
as a kind of index into the vector then use the second version. If the map owns theMyTreeNode
instances then use the first version.– Corristo
Dec 31 '18 at 23:19
1
@edamame - Um..... Is this supposed to be a map that holds custom types, or is it some device that holds entities in special clothing?
– enhzflep
Dec 31 '18 at 23:26
@enhzflep it is a map that holds custom types. Thanks!
– Edamame
Dec 31 '18 at 23:31
2
If you are able to do either then use the first one (don't use pointers if you don't have to). Otherwise they have different uses.
– Galik
Dec 31 '18 at 23:45
1
If you are relying on
unordered_map
for your search tree, why not just use a singleunordered_map
for all of your nodes? What advantage does a tree structure give you for children that a singleunordered_map
would not be able to provide?– jxh
Dec 31 '18 at 23:57