JavaMail SMTP Transport does not throw AuthenticationFailedException when given incorrect credentials
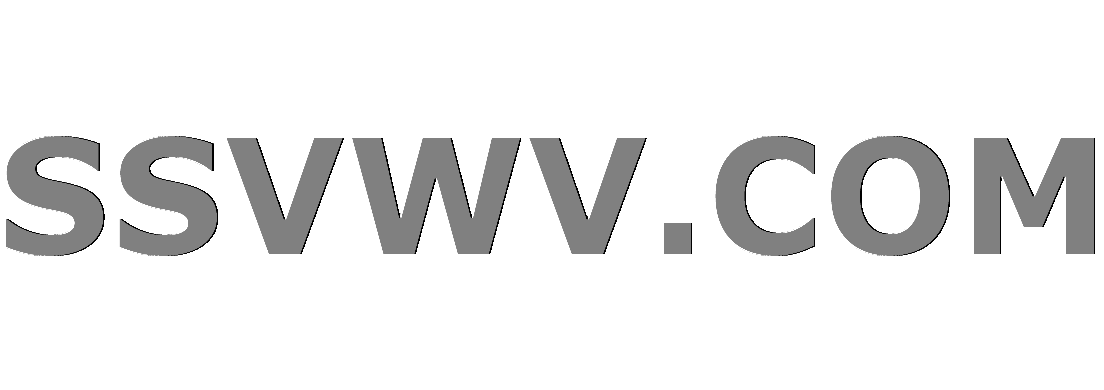
Multi tool use
I am trying to check if SMTP settings / credentials are entered correctly for an email account using the JavaMail library. The issue I am having is that the connection is successful regardless of valid or invalid credentials.
This is being tested on a GMail account that has 3rd part app access enabled, and can be connected to via IMAP and GIMAP providers included with JavaMail. If the SMTP settings are correct then it is also capable of sending mail, I am just trying to add a layer so that when you configure a new account, the SMTP credentials and settings are tested to verify a correct configuration.
The bigger picture here is that the project this code belongs to wont only be used for GMail accounts, it should support any IMAP/SMTP email service.
I have tried a number of variations with creating Session and Transports, and primarily followed the example answer in the related questions:
Javamail transport getting success to authenticate with invalid credentials
Validate smtp server credentials using java without actually sending mail
Those answers do not appear to be working for me, as the issue is that the transport is making a successful connection with the invalid credentials, trying to send a message does fail though with a MessagingException that is not an instance of AuthenticationFailedException.. the second of those 2 related questions multiple comments claim to have a similar issue with no solution provided.
// For the purposes of this code snippet getSmtpUsername() and getSmtpPassword() return a constant string value representing the username and password to be used when logging into SMTP server.
public Authenticator getSMTPAuthenticator() {
return new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication( getSmtpUsername(), getSmtpPassword() );
}
};
}
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.socketFactory.port", 465);
properties.put( "mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory" );
Transport transport = Session.getInstance( properties, getSMTPAuthenticator() ).getTransport("smtp"); //.getTransport() also has not solved this issue
transport.connect( "smtp.gmail.com", 465, getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) { //TODO: this exception just never happens even with wrong credentials...
return false;
}
}
My expected result is that if getSmtpUsername() or getSmtpPassword() return a string value that is not consistant with a valid account then the AuthenticationFailedException will be thrown, or some other method is implemented in place to determine if the credentials are incorrect.
java email authentication smtp javamail
add a comment |
I am trying to check if SMTP settings / credentials are entered correctly for an email account using the JavaMail library. The issue I am having is that the connection is successful regardless of valid or invalid credentials.
This is being tested on a GMail account that has 3rd part app access enabled, and can be connected to via IMAP and GIMAP providers included with JavaMail. If the SMTP settings are correct then it is also capable of sending mail, I am just trying to add a layer so that when you configure a new account, the SMTP credentials and settings are tested to verify a correct configuration.
The bigger picture here is that the project this code belongs to wont only be used for GMail accounts, it should support any IMAP/SMTP email service.
I have tried a number of variations with creating Session and Transports, and primarily followed the example answer in the related questions:
Javamail transport getting success to authenticate with invalid credentials
Validate smtp server credentials using java without actually sending mail
Those answers do not appear to be working for me, as the issue is that the transport is making a successful connection with the invalid credentials, trying to send a message does fail though with a MessagingException that is not an instance of AuthenticationFailedException.. the second of those 2 related questions multiple comments claim to have a similar issue with no solution provided.
// For the purposes of this code snippet getSmtpUsername() and getSmtpPassword() return a constant string value representing the username and password to be used when logging into SMTP server.
public Authenticator getSMTPAuthenticator() {
return new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication( getSmtpUsername(), getSmtpPassword() );
}
};
}
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.socketFactory.port", 465);
properties.put( "mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory" );
Transport transport = Session.getInstance( properties, getSMTPAuthenticator() ).getTransport("smtp"); //.getTransport() also has not solved this issue
transport.connect( "smtp.gmail.com", 465, getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) { //TODO: this exception just never happens even with wrong credentials...
return false;
}
}
My expected result is that if getSmtpUsername() or getSmtpPassword() return a string value that is not consistant with a valid account then the AuthenticationFailedException will be thrown, or some other method is implemented in place to determine if the credentials are incorrect.
java email authentication smtp javamail
Fix all these common JavaMail mistakes, then post the JavaMail debug output.
– Bill Shannon
Dec 29 '18 at 0:44
@BillShannon I have made the suggested changes and found out what the problem was. Thank you very much!
– Jacob Pozaic
Dec 31 '18 at 15:42
add a comment |
I am trying to check if SMTP settings / credentials are entered correctly for an email account using the JavaMail library. The issue I am having is that the connection is successful regardless of valid or invalid credentials.
This is being tested on a GMail account that has 3rd part app access enabled, and can be connected to via IMAP and GIMAP providers included with JavaMail. If the SMTP settings are correct then it is also capable of sending mail, I am just trying to add a layer so that when you configure a new account, the SMTP credentials and settings are tested to verify a correct configuration.
The bigger picture here is that the project this code belongs to wont only be used for GMail accounts, it should support any IMAP/SMTP email service.
I have tried a number of variations with creating Session and Transports, and primarily followed the example answer in the related questions:
Javamail transport getting success to authenticate with invalid credentials
Validate smtp server credentials using java without actually sending mail
Those answers do not appear to be working for me, as the issue is that the transport is making a successful connection with the invalid credentials, trying to send a message does fail though with a MessagingException that is not an instance of AuthenticationFailedException.. the second of those 2 related questions multiple comments claim to have a similar issue with no solution provided.
// For the purposes of this code snippet getSmtpUsername() and getSmtpPassword() return a constant string value representing the username and password to be used when logging into SMTP server.
public Authenticator getSMTPAuthenticator() {
return new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication( getSmtpUsername(), getSmtpPassword() );
}
};
}
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.socketFactory.port", 465);
properties.put( "mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory" );
Transport transport = Session.getInstance( properties, getSMTPAuthenticator() ).getTransport("smtp"); //.getTransport() also has not solved this issue
transport.connect( "smtp.gmail.com", 465, getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) { //TODO: this exception just never happens even with wrong credentials...
return false;
}
}
My expected result is that if getSmtpUsername() or getSmtpPassword() return a string value that is not consistant with a valid account then the AuthenticationFailedException will be thrown, or some other method is implemented in place to determine if the credentials are incorrect.
java email authentication smtp javamail
I am trying to check if SMTP settings / credentials are entered correctly for an email account using the JavaMail library. The issue I am having is that the connection is successful regardless of valid or invalid credentials.
This is being tested on a GMail account that has 3rd part app access enabled, and can be connected to via IMAP and GIMAP providers included with JavaMail. If the SMTP settings are correct then it is also capable of sending mail, I am just trying to add a layer so that when you configure a new account, the SMTP credentials and settings are tested to verify a correct configuration.
The bigger picture here is that the project this code belongs to wont only be used for GMail accounts, it should support any IMAP/SMTP email service.
I have tried a number of variations with creating Session and Transports, and primarily followed the example answer in the related questions:
Javamail transport getting success to authenticate with invalid credentials
Validate smtp server credentials using java without actually sending mail
Those answers do not appear to be working for me, as the issue is that the transport is making a successful connection with the invalid credentials, trying to send a message does fail though with a MessagingException that is not an instance of AuthenticationFailedException.. the second of those 2 related questions multiple comments claim to have a similar issue with no solution provided.
// For the purposes of this code snippet getSmtpUsername() and getSmtpPassword() return a constant string value representing the username and password to be used when logging into SMTP server.
public Authenticator getSMTPAuthenticator() {
return new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication( getSmtpUsername(), getSmtpPassword() );
}
};
}
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.socketFactory.port", 465);
properties.put( "mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory" );
Transport transport = Session.getInstance( properties, getSMTPAuthenticator() ).getTransport("smtp"); //.getTransport() also has not solved this issue
transport.connect( "smtp.gmail.com", 465, getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) { //TODO: this exception just never happens even with wrong credentials...
return false;
}
}
My expected result is that if getSmtpUsername() or getSmtpPassword() return a string value that is not consistant with a valid account then the AuthenticationFailedException will be thrown, or some other method is implemented in place to determine if the credentials are incorrect.
java email authentication smtp javamail
java email authentication smtp javamail
asked Dec 28 '18 at 17:58


Jacob PozaicJacob Pozaic
12
12
Fix all these common JavaMail mistakes, then post the JavaMail debug output.
– Bill Shannon
Dec 29 '18 at 0:44
@BillShannon I have made the suggested changes and found out what the problem was. Thank you very much!
– Jacob Pozaic
Dec 31 '18 at 15:42
add a comment |
Fix all these common JavaMail mistakes, then post the JavaMail debug output.
– Bill Shannon
Dec 29 '18 at 0:44
@BillShannon I have made the suggested changes and found out what the problem was. Thank you very much!
– Jacob Pozaic
Dec 31 '18 at 15:42
Fix all these common JavaMail mistakes, then post the JavaMail debug output.
– Bill Shannon
Dec 29 '18 at 0:44
Fix all these common JavaMail mistakes, then post the JavaMail debug output.
– Bill Shannon
Dec 29 '18 at 0:44
@BillShannon I have made the suggested changes and found out what the problem was. Thank you very much!
– Jacob Pozaic
Dec 31 '18 at 15:42
@BillShannon I have made the suggested changes and found out what the problem was. Thank you very much!
– Jacob Pozaic
Dec 31 '18 at 15:42
add a comment |
1 Answer
1
active
oldest
votes
After making the changes suggested by Bill Shannon it turned out the error was actually a logic error where getSmtpPassword() was returning the IMAP password (which led to successful login) under some circumstances, although the suggestions where not what solved the problem, the updated code is as follows:
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.ssl.enable", true);
Transport transport = Session.getInstance( properties ).getTransport();
transport.connect( getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) {
return false;
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53962499%2fjavamail-smtp-transport-does-not-throw-authenticationfailedexception-when-given%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
After making the changes suggested by Bill Shannon it turned out the error was actually a logic error where getSmtpPassword() was returning the IMAP password (which led to successful login) under some circumstances, although the suggestions where not what solved the problem, the updated code is as follows:
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.ssl.enable", true);
Transport transport = Session.getInstance( properties ).getTransport();
transport.connect( getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) {
return false;
}
}
add a comment |
After making the changes suggested by Bill Shannon it turned out the error was actually a logic error where getSmtpPassword() was returning the IMAP password (which led to successful login) under some circumstances, although the suggestions where not what solved the problem, the updated code is as follows:
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.ssl.enable", true);
Transport transport = Session.getInstance( properties ).getTransport();
transport.connect( getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) {
return false;
}
}
add a comment |
After making the changes suggested by Bill Shannon it turned out the error was actually a logic error where getSmtpPassword() was returning the IMAP password (which led to successful login) under some circumstances, although the suggestions where not what solved the problem, the updated code is as follows:
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.ssl.enable", true);
Transport transport = Session.getInstance( properties ).getTransport();
transport.connect( getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) {
return false;
}
}
After making the changes suggested by Bill Shannon it turned out the error was actually a logic error where getSmtpPassword() was returning the IMAP password (which led to successful login) under some circumstances, although the suggestions where not what solved the problem, the updated code is as follows:
public boolean authenticateSMTP( SMTPConfiguration smtpConfiguration ) throws MessagingException {
try {
Properties properties = new Properties( );
properties.put( "mail.smtp.auth", true );
properties.put( "mail.smtp.host", "smtp.gmail.com" );
properties.put( "mail.smtp.port", 465 );
properties.put( "mail.smtp.ssl.enable", true);
Transport transport = Session.getInstance( properties ).getTransport();
transport.connect( getSmtpUsername(), getSmtpPassword() );
transport.close();
return true;
} catch ( AuthenticationFailedException e ) {
return false;
}
}
answered Dec 31 '18 at 15:41


Jacob PozaicJacob Pozaic
12
12
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53962499%2fjavamail-smtp-transport-does-not-throw-authenticationfailedexception-when-given%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CI RMNkRXzICQxq8kr 5,LK64cP8qAkyr,pXFftwxCJ
Fix all these common JavaMail mistakes, then post the JavaMail debug output.
– Bill Shannon
Dec 29 '18 at 0:44
@BillShannon I have made the suggested changes and found out what the problem was. Thank you very much!
– Jacob Pozaic
Dec 31 '18 at 15:42