iOS swift delegate with more than 1 uitextfield in a uiview
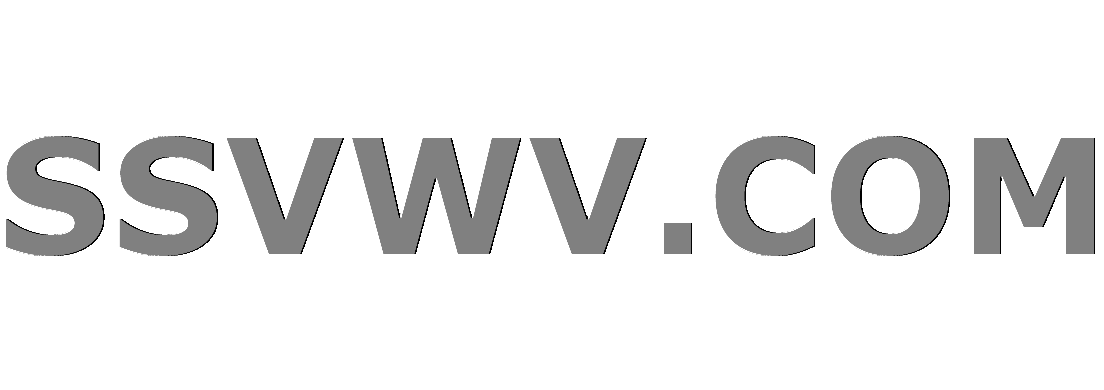
Multi tool use
I have an iOS app, with one UIView
and three UITextField
(more than 1)
I would to understand what are the best practices for my class ViewController to manage the UITextField
.
- class MainViewController: UIViewController, UITextFieldDelegate ?
I wonder that, because I have more than one UITextField
and only one func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool
ios swift uitextfielddelegate
add a comment |
I have an iOS app, with one UIView
and three UITextField
(more than 1)
I would to understand what are the best practices for my class ViewController to manage the UITextField
.
- class MainViewController: UIViewController, UITextFieldDelegate ?
I wonder that, because I have more than one UITextField
and only one func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool
ios swift uitextfielddelegate
add a comment |
I have an iOS app, with one UIView
and three UITextField
(more than 1)
I would to understand what are the best practices for my class ViewController to manage the UITextField
.
- class MainViewController: UIViewController, UITextFieldDelegate ?
I wonder that, because I have more than one UITextField
and only one func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool
ios swift uitextfielddelegate
I have an iOS app, with one UIView
and three UITextField
(more than 1)
I would to understand what are the best practices for my class ViewController to manage the UITextField
.
- class MainViewController: UIViewController, UITextFieldDelegate ?
I wonder that, because I have more than one UITextField
and only one func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool
ios swift uitextfielddelegate
ios swift uitextfielddelegate
edited Jun 3 '15 at 22:31


Victor Sigler
18.2k86887
18.2k86887
asked Jun 3 '15 at 20:58
FREDERIC1405FREDERIC1405
3014
3014
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Easiest way is to know what text field to use in delegate methods. I.e. you have 3 text fields: field1, field2, field3 and when delegate called you can detect what to do:
func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool {
if textField == field1 {
// do something
} else if textField == field2 {
// do something
} else if textField == field3 {
// do something
}
return true
}
Do not forget to make all field's delegate as self: field1.delegate = self
etc.
In your case it will work fine.
If you want to know a better solution if you have much more fields (10, 20?) let me know and I'll update my answer.
1
What to do with 10 or 20 fields? Could you please update your answer.
– Vitaliy Litvinov
Mar 23 '17 at 11:07
Is there any better solution(s)? Would be best if someone could write down all possible alternatives.
– In-Vogue
Nov 24 '17 at 5:44
@anatoliy_v Please write the alternatives for multiple fields, It would help so much.
– Aashish
Apr 3 '18 at 9:40
@VitaliyLitvinov answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:12
@Aashish I've answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:13
add a comment |
Best way to do this is using the tag
attribute.
As seen on the Apple Docs:
- (void)textFieldDidEndEditing:(UITextField *)textField {
switch (textField.tag) {
case NameFieldTag:
// do something with this text field
break;
case EmailFieldTag:
// do something with this text field
break;
// remainder of switch statement....
}
}
enum {
NameFieldTag = 0,
EmailFieldTag,
DOBFieldTag,
SSNFieldTag
};
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f30630582%2fios-swift-delegate-with-more-than-1-uitextfield-in-a-uiview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Easiest way is to know what text field to use in delegate methods. I.e. you have 3 text fields: field1, field2, field3 and when delegate called you can detect what to do:
func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool {
if textField == field1 {
// do something
} else if textField == field2 {
// do something
} else if textField == field3 {
// do something
}
return true
}
Do not forget to make all field's delegate as self: field1.delegate = self
etc.
In your case it will work fine.
If you want to know a better solution if you have much more fields (10, 20?) let me know and I'll update my answer.
1
What to do with 10 or 20 fields? Could you please update your answer.
– Vitaliy Litvinov
Mar 23 '17 at 11:07
Is there any better solution(s)? Would be best if someone could write down all possible alternatives.
– In-Vogue
Nov 24 '17 at 5:44
@anatoliy_v Please write the alternatives for multiple fields, It would help so much.
– Aashish
Apr 3 '18 at 9:40
@VitaliyLitvinov answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:12
@Aashish I've answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:13
add a comment |
Easiest way is to know what text field to use in delegate methods. I.e. you have 3 text fields: field1, field2, field3 and when delegate called you can detect what to do:
func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool {
if textField == field1 {
// do something
} else if textField == field2 {
// do something
} else if textField == field3 {
// do something
}
return true
}
Do not forget to make all field's delegate as self: field1.delegate = self
etc.
In your case it will work fine.
If you want to know a better solution if you have much more fields (10, 20?) let me know and I'll update my answer.
1
What to do with 10 or 20 fields? Could you please update your answer.
– Vitaliy Litvinov
Mar 23 '17 at 11:07
Is there any better solution(s)? Would be best if someone could write down all possible alternatives.
– In-Vogue
Nov 24 '17 at 5:44
@anatoliy_v Please write the alternatives for multiple fields, It would help so much.
– Aashish
Apr 3 '18 at 9:40
@VitaliyLitvinov answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:12
@Aashish I've answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:13
add a comment |
Easiest way is to know what text field to use in delegate methods. I.e. you have 3 text fields: field1, field2, field3 and when delegate called you can detect what to do:
func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool {
if textField == field1 {
// do something
} else if textField == field2 {
// do something
} else if textField == field3 {
// do something
}
return true
}
Do not forget to make all field's delegate as self: field1.delegate = self
etc.
In your case it will work fine.
If you want to know a better solution if you have much more fields (10, 20?) let me know and I'll update my answer.
Easiest way is to know what text field to use in delegate methods. I.e. you have 3 text fields: field1, field2, field3 and when delegate called you can detect what to do:
func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool {
if textField == field1 {
// do something
} else if textField == field2 {
// do something
} else if textField == field3 {
// do something
}
return true
}
Do not forget to make all field's delegate as self: field1.delegate = self
etc.
In your case it will work fine.
If you want to know a better solution if you have much more fields (10, 20?) let me know and I'll update my answer.
edited Jan 4 '16 at 21:31


Roman
6791829
6791829
answered Jun 3 '15 at 21:21
anatoliy_vanatoliy_v
1,2471322
1,2471322
1
What to do with 10 or 20 fields? Could you please update your answer.
– Vitaliy Litvinov
Mar 23 '17 at 11:07
Is there any better solution(s)? Would be best if someone could write down all possible alternatives.
– In-Vogue
Nov 24 '17 at 5:44
@anatoliy_v Please write the alternatives for multiple fields, It would help so much.
– Aashish
Apr 3 '18 at 9:40
@VitaliyLitvinov answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:12
@Aashish I've answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:13
add a comment |
1
What to do with 10 or 20 fields? Could you please update your answer.
– Vitaliy Litvinov
Mar 23 '17 at 11:07
Is there any better solution(s)? Would be best if someone could write down all possible alternatives.
– In-Vogue
Nov 24 '17 at 5:44
@anatoliy_v Please write the alternatives for multiple fields, It would help so much.
– Aashish
Apr 3 '18 at 9:40
@VitaliyLitvinov answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:12
@Aashish I've answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:13
1
1
What to do with 10 or 20 fields? Could you please update your answer.
– Vitaliy Litvinov
Mar 23 '17 at 11:07
What to do with 10 or 20 fields? Could you please update your answer.
– Vitaliy Litvinov
Mar 23 '17 at 11:07
Is there any better solution(s)? Would be best if someone could write down all possible alternatives.
– In-Vogue
Nov 24 '17 at 5:44
Is there any better solution(s)? Would be best if someone could write down all possible alternatives.
– In-Vogue
Nov 24 '17 at 5:44
@anatoliy_v Please write the alternatives for multiple fields, It would help so much.
– Aashish
Apr 3 '18 at 9:40
@anatoliy_v Please write the alternatives for multiple fields, It would help so much.
– Aashish
Apr 3 '18 at 9:40
@VitaliyLitvinov answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:12
@VitaliyLitvinov answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:12
@Aashish I've answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:13
@Aashish I've answered below a way to do this for any number of fields.
– dordio
Dec 28 '18 at 18:13
add a comment |
Best way to do this is using the tag
attribute.
As seen on the Apple Docs:
- (void)textFieldDidEndEditing:(UITextField *)textField {
switch (textField.tag) {
case NameFieldTag:
// do something with this text field
break;
case EmailFieldTag:
// do something with this text field
break;
// remainder of switch statement....
}
}
enum {
NameFieldTag = 0,
EmailFieldTag,
DOBFieldTag,
SSNFieldTag
};
add a comment |
Best way to do this is using the tag
attribute.
As seen on the Apple Docs:
- (void)textFieldDidEndEditing:(UITextField *)textField {
switch (textField.tag) {
case NameFieldTag:
// do something with this text field
break;
case EmailFieldTag:
// do something with this text field
break;
// remainder of switch statement....
}
}
enum {
NameFieldTag = 0,
EmailFieldTag,
DOBFieldTag,
SSNFieldTag
};
add a comment |
Best way to do this is using the tag
attribute.
As seen on the Apple Docs:
- (void)textFieldDidEndEditing:(UITextField *)textField {
switch (textField.tag) {
case NameFieldTag:
// do something with this text field
break;
case EmailFieldTag:
// do something with this text field
break;
// remainder of switch statement....
}
}
enum {
NameFieldTag = 0,
EmailFieldTag,
DOBFieldTag,
SSNFieldTag
};
Best way to do this is using the tag
attribute.
As seen on the Apple Docs:
- (void)textFieldDidEndEditing:(UITextField *)textField {
switch (textField.tag) {
case NameFieldTag:
// do something with this text field
break;
case EmailFieldTag:
// do something with this text field
break;
// remainder of switch statement....
}
}
enum {
NameFieldTag = 0,
EmailFieldTag,
DOBFieldTag,
SSNFieldTag
};
answered Dec 28 '18 at 18:08


dordiodordio
1187
1187
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f30630582%2fios-swift-delegate-with-more-than-1-uitextfield-in-a-uiview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KZWXG1k0 Vn0eEa tJYtWuuHT,45CO8R 4E2t4,l0dHm7f2 MKjBPIqk3Q2tl FYfNtgR,DN C up9a,VivOSkijb