How to see why a keras / tensorflow model is getting stuck?
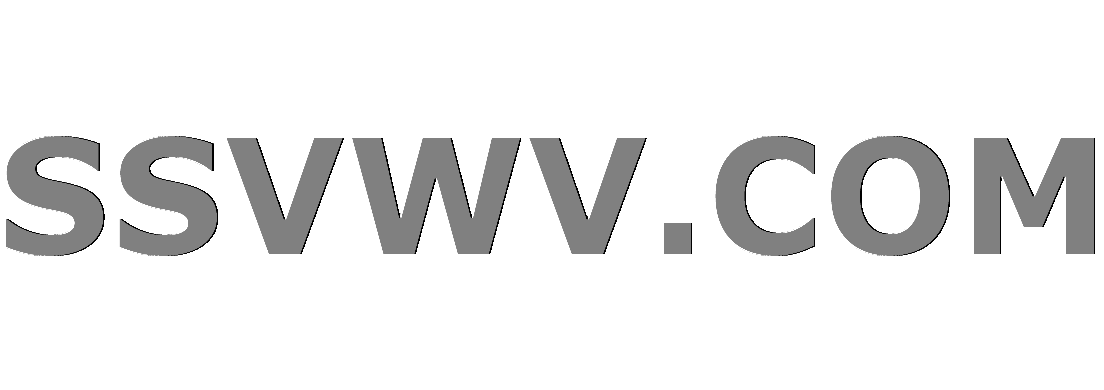
Multi tool use
My code is:
from keras.models import Sequential
from keras.layers import Dense
import numpy
import pandas as pd
X = pd.read_csv(
"data/train.csv", usecols=['Type', 'Age', 'Breed1', 'Breed2', 'Gender', 'Color1', 'Color2', 'Color3', 'MaturitySize', 'FurLength', 'Vaccinated', 'Dewormed', 'Sterilized', 'Health', 'Quantity', 'Fee', 'VideoAmt', 'PhotoAmt'])
Y = pd.read_csv(
"data/train.csv", usecols=['AdoptionSpeed'])
model = Sequential()
model.add(Dense(18, input_dim=18, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
model.compile(loss='binary_crossentropy',
optimizer='adam', metrics=['accuracy'])
model.fit(X, Y, epochs=150, batch_size=100)
scores = model.evaluate(X, Y)
print("n%s: %.2f%%" % (model.metrics_names[1], scores[1]*100))
I am trying to train to see how the various factors (type, age, etc) affect the AdoptionSpeed
. However, the accuracy gets stuck at 20.6% and doesn't really move from there.
Epoch 2/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1539 - acc: 0.2061
Epoch 3/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1591 - acc: 0.2061
Epoch 4/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1626 - acc: 0.2061
...
Epoch 150/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1757 - acc: 0.2061
14993/14993 [==============================] - 0s 11us/step
acc: 20.61%
Is there anything I can do to nudge to get unstuck?
python tensorflow keras neural-network
|
show 4 more comments
My code is:
from keras.models import Sequential
from keras.layers import Dense
import numpy
import pandas as pd
X = pd.read_csv(
"data/train.csv", usecols=['Type', 'Age', 'Breed1', 'Breed2', 'Gender', 'Color1', 'Color2', 'Color3', 'MaturitySize', 'FurLength', 'Vaccinated', 'Dewormed', 'Sterilized', 'Health', 'Quantity', 'Fee', 'VideoAmt', 'PhotoAmt'])
Y = pd.read_csv(
"data/train.csv", usecols=['AdoptionSpeed'])
model = Sequential()
model.add(Dense(18, input_dim=18, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
model.compile(loss='binary_crossentropy',
optimizer='adam', metrics=['accuracy'])
model.fit(X, Y, epochs=150, batch_size=100)
scores = model.evaluate(X, Y)
print("n%s: %.2f%%" % (model.metrics_names[1], scores[1]*100))
I am trying to train to see how the various factors (type, age, etc) affect the AdoptionSpeed
. However, the accuracy gets stuck at 20.6% and doesn't really move from there.
Epoch 2/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1539 - acc: 0.2061
Epoch 3/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1591 - acc: 0.2061
Epoch 4/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1626 - acc: 0.2061
...
Epoch 150/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1757 - acc: 0.2061
14993/14993 [==============================] - 0s 11us/step
acc: 20.61%
Is there anything I can do to nudge to get unstuck?
python tensorflow keras neural-network
Did you normalize your data before feeding to the network? see here for an example.
– Amir
Dec 28 '18 at 19:21
What are the values in your Y? AdoptionSpeed sounds like a continuous value.
– Matias Valdenegro
Dec 28 '18 at 19:39
And the values of the loss suggest that the speed is not between 0 and 1 (as your output is a sigmoid activation)
– Daniel Möller
Dec 28 '18 at 20:54
I did not normalize. I guess I was somewhat hoping thatkeras
would be able to do that?Y
is days until adoption.
– Shamoon
Dec 29 '18 at 3:51
@DanielMöller good point. Wouldrelu
be a better output?
– Shamoon
Dec 29 '18 at 3:53
|
show 4 more comments
My code is:
from keras.models import Sequential
from keras.layers import Dense
import numpy
import pandas as pd
X = pd.read_csv(
"data/train.csv", usecols=['Type', 'Age', 'Breed1', 'Breed2', 'Gender', 'Color1', 'Color2', 'Color3', 'MaturitySize', 'FurLength', 'Vaccinated', 'Dewormed', 'Sterilized', 'Health', 'Quantity', 'Fee', 'VideoAmt', 'PhotoAmt'])
Y = pd.read_csv(
"data/train.csv", usecols=['AdoptionSpeed'])
model = Sequential()
model.add(Dense(18, input_dim=18, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
model.compile(loss='binary_crossentropy',
optimizer='adam', metrics=['accuracy'])
model.fit(X, Y, epochs=150, batch_size=100)
scores = model.evaluate(X, Y)
print("n%s: %.2f%%" % (model.metrics_names[1], scores[1]*100))
I am trying to train to see how the various factors (type, age, etc) affect the AdoptionSpeed
. However, the accuracy gets stuck at 20.6% and doesn't really move from there.
Epoch 2/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1539 - acc: 0.2061
Epoch 3/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1591 - acc: 0.2061
Epoch 4/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1626 - acc: 0.2061
...
Epoch 150/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1757 - acc: 0.2061
14993/14993 [==============================] - 0s 11us/step
acc: 20.61%
Is there anything I can do to nudge to get unstuck?
python tensorflow keras neural-network
My code is:
from keras.models import Sequential
from keras.layers import Dense
import numpy
import pandas as pd
X = pd.read_csv(
"data/train.csv", usecols=['Type', 'Age', 'Breed1', 'Breed2', 'Gender', 'Color1', 'Color2', 'Color3', 'MaturitySize', 'FurLength', 'Vaccinated', 'Dewormed', 'Sterilized', 'Health', 'Quantity', 'Fee', 'VideoAmt', 'PhotoAmt'])
Y = pd.read_csv(
"data/train.csv", usecols=['AdoptionSpeed'])
model = Sequential()
model.add(Dense(18, input_dim=18, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
model.compile(loss='binary_crossentropy',
optimizer='adam', metrics=['accuracy'])
model.fit(X, Y, epochs=150, batch_size=100)
scores = model.evaluate(X, Y)
print("n%s: %.2f%%" % (model.metrics_names[1], scores[1]*100))
I am trying to train to see how the various factors (type, age, etc) affect the AdoptionSpeed
. However, the accuracy gets stuck at 20.6% and doesn't really move from there.
Epoch 2/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1539 - acc: 0.2061
Epoch 3/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1591 - acc: 0.2061
Epoch 4/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1626 - acc: 0.2061
...
Epoch 150/150
14993/14993 [==============================] - 0s 9us/step - loss: -24.1757 - acc: 0.2061
14993/14993 [==============================] - 0s 11us/step
acc: 20.61%
Is there anything I can do to nudge to get unstuck?
python tensorflow keras neural-network
python tensorflow keras neural-network
asked Dec 28 '18 at 18:05
ShamoonShamoon
10.7k52163339
10.7k52163339
Did you normalize your data before feeding to the network? see here for an example.
– Amir
Dec 28 '18 at 19:21
What are the values in your Y? AdoptionSpeed sounds like a continuous value.
– Matias Valdenegro
Dec 28 '18 at 19:39
And the values of the loss suggest that the speed is not between 0 and 1 (as your output is a sigmoid activation)
– Daniel Möller
Dec 28 '18 at 20:54
I did not normalize. I guess I was somewhat hoping thatkeras
would be able to do that?Y
is days until adoption.
– Shamoon
Dec 29 '18 at 3:51
@DanielMöller good point. Wouldrelu
be a better output?
– Shamoon
Dec 29 '18 at 3:53
|
show 4 more comments
Did you normalize your data before feeding to the network? see here for an example.
– Amir
Dec 28 '18 at 19:21
What are the values in your Y? AdoptionSpeed sounds like a continuous value.
– Matias Valdenegro
Dec 28 '18 at 19:39
And the values of the loss suggest that the speed is not between 0 and 1 (as your output is a sigmoid activation)
– Daniel Möller
Dec 28 '18 at 20:54
I did not normalize. I guess I was somewhat hoping thatkeras
would be able to do that?Y
is days until adoption.
– Shamoon
Dec 29 '18 at 3:51
@DanielMöller good point. Wouldrelu
be a better output?
– Shamoon
Dec 29 '18 at 3:53
Did you normalize your data before feeding to the network? see here for an example.
– Amir
Dec 28 '18 at 19:21
Did you normalize your data before feeding to the network? see here for an example.
– Amir
Dec 28 '18 at 19:21
What are the values in your Y? AdoptionSpeed sounds like a continuous value.
– Matias Valdenegro
Dec 28 '18 at 19:39
What are the values in your Y? AdoptionSpeed sounds like a continuous value.
– Matias Valdenegro
Dec 28 '18 at 19:39
And the values of the loss suggest that the speed is not between 0 and 1 (as your output is a sigmoid activation)
– Daniel Möller
Dec 28 '18 at 20:54
And the values of the loss suggest that the speed is not between 0 and 1 (as your output is a sigmoid activation)
– Daniel Möller
Dec 28 '18 at 20:54
I did not normalize. I guess I was somewhat hoping that
keras
would be able to do that? Y
is days until adoption.– Shamoon
Dec 29 '18 at 3:51
I did not normalize. I guess I was somewhat hoping that
keras
would be able to do that? Y
is days until adoption.– Shamoon
Dec 29 '18 at 3:51
@DanielMöller good point. Would
relu
be a better output?– Shamoon
Dec 29 '18 at 3:53
@DanielMöller good point. Would
relu
be a better output?– Shamoon
Dec 29 '18 at 3:53
|
show 4 more comments
1 Answer
1
active
oldest
votes
By the values of the loss, it seems your true data is not in the same range as the the model's output (sigmoid).
Sigmoid outputs between 0 and 1 only. So you should normalize your data in order to have it between 0 and 1. One possibility is simply divide y
by y.max()
.
Or you can try other possibilities, considering:
- sigmoid: between 0 and 1
- tanh: between -1 and 1
- relu: 0 to infinity
- linear: -inf to +inf
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53962586%2fhow-to-see-why-a-keras-tensorflow-model-is-getting-stuck%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
By the values of the loss, it seems your true data is not in the same range as the the model's output (sigmoid).
Sigmoid outputs between 0 and 1 only. So you should normalize your data in order to have it between 0 and 1. One possibility is simply divide y
by y.max()
.
Or you can try other possibilities, considering:
- sigmoid: between 0 and 1
- tanh: between -1 and 1
- relu: 0 to infinity
- linear: -inf to +inf
add a comment |
By the values of the loss, it seems your true data is not in the same range as the the model's output (sigmoid).
Sigmoid outputs between 0 and 1 only. So you should normalize your data in order to have it between 0 and 1. One possibility is simply divide y
by y.max()
.
Or you can try other possibilities, considering:
- sigmoid: between 0 and 1
- tanh: between -1 and 1
- relu: 0 to infinity
- linear: -inf to +inf
add a comment |
By the values of the loss, it seems your true data is not in the same range as the the model's output (sigmoid).
Sigmoid outputs between 0 and 1 only. So you should normalize your data in order to have it between 0 and 1. One possibility is simply divide y
by y.max()
.
Or you can try other possibilities, considering:
- sigmoid: between 0 and 1
- tanh: between -1 and 1
- relu: 0 to infinity
- linear: -inf to +inf
By the values of the loss, it seems your true data is not in the same range as the the model's output (sigmoid).
Sigmoid outputs between 0 and 1 only. So you should normalize your data in order to have it between 0 and 1. One possibility is simply divide y
by y.max()
.
Or you can try other possibilities, considering:
- sigmoid: between 0 and 1
- tanh: between -1 and 1
- relu: 0 to infinity
- linear: -inf to +inf
answered Dec 29 '18 at 5:39


Daniel MöllerDaniel Möller
33.6k663103
33.6k663103
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53962586%2fhow-to-see-why-a-keras-tensorflow-model-is-getting-stuck%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6aQ3Bw242Sg,Gw8mMOUzUNIsow4R,T7Bmp4J9uL yfslDoOnMb40Fwb,bnH2g3,JGfPki 6KAnqPY7E8igTxs71L4EDOtDeaK
Did you normalize your data before feeding to the network? see here for an example.
– Amir
Dec 28 '18 at 19:21
What are the values in your Y? AdoptionSpeed sounds like a continuous value.
– Matias Valdenegro
Dec 28 '18 at 19:39
And the values of the loss suggest that the speed is not between 0 and 1 (as your output is a sigmoid activation)
– Daniel Möller
Dec 28 '18 at 20:54
I did not normalize. I guess I was somewhat hoping that
keras
would be able to do that?Y
is days until adoption.– Shamoon
Dec 29 '18 at 3:51
@DanielMöller good point. Would
relu
be a better output?– Shamoon
Dec 29 '18 at 3:53