Compare two dictionaries with equal keys but different values [closed]
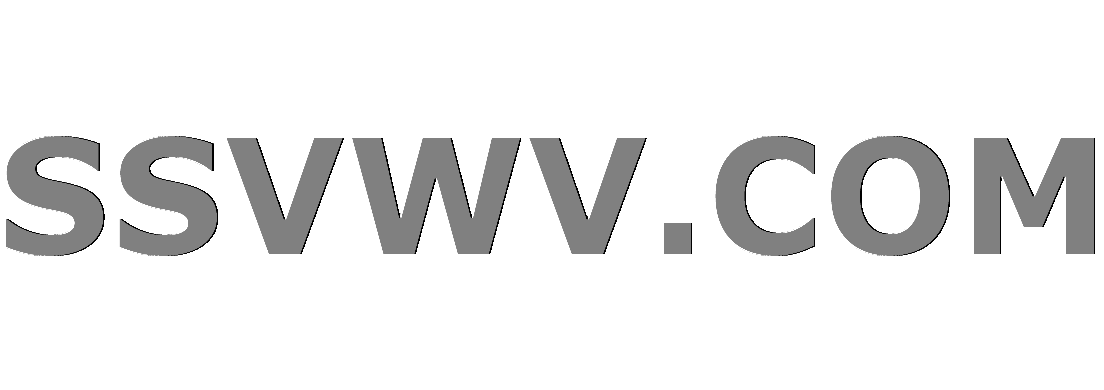
Multi tool use
I am facing a brutal mental battle with myself! I am developing a laundry system, and in this system I have 4 tables where they are: Entry, Entry Item, Output and Item output.
in the table item entry and item output I have a method that sums all the pieces made in the input and output, if the sum beat is right but if it is different I wanted to find out what the code of the clothes and the quantity that is divergent. It is in this part that I am caught.
Table ClothesOut
Table ItenEntry
Table ItemOut
This is the example I'm trying to do:
using System;
using System.Collections.Generic;
using System.Linq;
namespace Dictionary
{
class Program
{
static void Main(string args)
{
var d1 = new Dictionary<int, int>();
var d2 = new Dictionary<int, int>();
d1.Add(1, 10);
d1.Add(2, 20);
d2.Add(1, 10);
d2.Add(2, 5);
var keyAndValue = d1.Zip(d2, (first, second) => first + " " + second);
foreach (var item in keyAndValue)
{
// I need to compare the value of d1 with the value of d2. if it is different bring the difference.
}
Console.WriteLine("");
Console.ReadKey();
}
}
}
c#
closed as unclear what you're asking by Servy, NetMage, EdChum, arghtype, Rob Dec 29 '18 at 2:33
Please clarify your specific problem or add additional details to highlight exactly what you need. As it's currently written, it’s hard to tell exactly what you're asking. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
I am facing a brutal mental battle with myself! I am developing a laundry system, and in this system I have 4 tables where they are: Entry, Entry Item, Output and Item output.
in the table item entry and item output I have a method that sums all the pieces made in the input and output, if the sum beat is right but if it is different I wanted to find out what the code of the clothes and the quantity that is divergent. It is in this part that I am caught.
Table ClothesOut
Table ItenEntry
Table ItemOut
This is the example I'm trying to do:
using System;
using System.Collections.Generic;
using System.Linq;
namespace Dictionary
{
class Program
{
static void Main(string args)
{
var d1 = new Dictionary<int, int>();
var d2 = new Dictionary<int, int>();
d1.Add(1, 10);
d1.Add(2, 20);
d2.Add(1, 10);
d2.Add(2, 5);
var keyAndValue = d1.Zip(d2, (first, second) => first + " " + second);
foreach (var item in keyAndValue)
{
// I need to compare the value of d1 with the value of d2. if it is different bring the difference.
}
Console.WriteLine("");
Console.ReadKey();
}
}
}
c#
closed as unclear what you're asking by Servy, NetMage, EdChum, arghtype, Rob Dec 29 '18 at 2:33
Please clarify your specific problem or add additional details to highlight exactly what you need. As it's currently written, it’s hard to tell exactly what you're asking. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
1
Oh boy, this is gonna be SLOW as hell! Why don't you create a stored procedure in your DB and use some SQL joins to make it return the result set you need? Alternatively, you can use linq but that's gonna be way slower than the stored procedure.
– Hirasawa Yui
Dec 28 '18 at 16:25
He right, in others words, you dont have to load of your data in the application, except if youwant to work with a cache OR by working with all data each X hours for exemple.
– LeBigCat
Dec 28 '18 at 16:37
hello all, it's true had not thought about the stored procedure. I will try this solution and put the result.
– Tiago Santos Monteiro
Dec 29 '18 at 10:31
add a comment |
I am facing a brutal mental battle with myself! I am developing a laundry system, and in this system I have 4 tables where they are: Entry, Entry Item, Output and Item output.
in the table item entry and item output I have a method that sums all the pieces made in the input and output, if the sum beat is right but if it is different I wanted to find out what the code of the clothes and the quantity that is divergent. It is in this part that I am caught.
Table ClothesOut
Table ItenEntry
Table ItemOut
This is the example I'm trying to do:
using System;
using System.Collections.Generic;
using System.Linq;
namespace Dictionary
{
class Program
{
static void Main(string args)
{
var d1 = new Dictionary<int, int>();
var d2 = new Dictionary<int, int>();
d1.Add(1, 10);
d1.Add(2, 20);
d2.Add(1, 10);
d2.Add(2, 5);
var keyAndValue = d1.Zip(d2, (first, second) => first + " " + second);
foreach (var item in keyAndValue)
{
// I need to compare the value of d1 with the value of d2. if it is different bring the difference.
}
Console.WriteLine("");
Console.ReadKey();
}
}
}
c#
I am facing a brutal mental battle with myself! I am developing a laundry system, and in this system I have 4 tables where they are: Entry, Entry Item, Output and Item output.
in the table item entry and item output I have a method that sums all the pieces made in the input and output, if the sum beat is right but if it is different I wanted to find out what the code of the clothes and the quantity that is divergent. It is in this part that I am caught.
Table ClothesOut
Table ItenEntry
Table ItemOut
This is the example I'm trying to do:
using System;
using System.Collections.Generic;
using System.Linq;
namespace Dictionary
{
class Program
{
static void Main(string args)
{
var d1 = new Dictionary<int, int>();
var d2 = new Dictionary<int, int>();
d1.Add(1, 10);
d1.Add(2, 20);
d2.Add(1, 10);
d2.Add(2, 5);
var keyAndValue = d1.Zip(d2, (first, second) => first + " " + second);
foreach (var item in keyAndValue)
{
// I need to compare the value of d1 with the value of d2. if it is different bring the difference.
}
Console.WriteLine("");
Console.ReadKey();
}
}
}
c#
c#
edited Dec 28 '18 at 17:49
marc_s
572k12811071253
572k12811071253
asked Dec 28 '18 at 16:20


Tiago Santos MonteiroTiago Santos Monteiro
91
91
closed as unclear what you're asking by Servy, NetMage, EdChum, arghtype, Rob Dec 29 '18 at 2:33
Please clarify your specific problem or add additional details to highlight exactly what you need. As it's currently written, it’s hard to tell exactly what you're asking. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
closed as unclear what you're asking by Servy, NetMage, EdChum, arghtype, Rob Dec 29 '18 at 2:33
Please clarify your specific problem or add additional details to highlight exactly what you need. As it's currently written, it’s hard to tell exactly what you're asking. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
1
Oh boy, this is gonna be SLOW as hell! Why don't you create a stored procedure in your DB and use some SQL joins to make it return the result set you need? Alternatively, you can use linq but that's gonna be way slower than the stored procedure.
– Hirasawa Yui
Dec 28 '18 at 16:25
He right, in others words, you dont have to load of your data in the application, except if youwant to work with a cache OR by working with all data each X hours for exemple.
– LeBigCat
Dec 28 '18 at 16:37
hello all, it's true had not thought about the stored procedure. I will try this solution and put the result.
– Tiago Santos Monteiro
Dec 29 '18 at 10:31
add a comment |
1
Oh boy, this is gonna be SLOW as hell! Why don't you create a stored procedure in your DB and use some SQL joins to make it return the result set you need? Alternatively, you can use linq but that's gonna be way slower than the stored procedure.
– Hirasawa Yui
Dec 28 '18 at 16:25
He right, in others words, you dont have to load of your data in the application, except if youwant to work with a cache OR by working with all data each X hours for exemple.
– LeBigCat
Dec 28 '18 at 16:37
hello all, it's true had not thought about the stored procedure. I will try this solution and put the result.
– Tiago Santos Monteiro
Dec 29 '18 at 10:31
1
1
Oh boy, this is gonna be SLOW as hell! Why don't you create a stored procedure in your DB and use some SQL joins to make it return the result set you need? Alternatively, you can use linq but that's gonna be way slower than the stored procedure.
– Hirasawa Yui
Dec 28 '18 at 16:25
Oh boy, this is gonna be SLOW as hell! Why don't you create a stored procedure in your DB and use some SQL joins to make it return the result set you need? Alternatively, you can use linq but that's gonna be way slower than the stored procedure.
– Hirasawa Yui
Dec 28 '18 at 16:25
He right, in others words, you dont have to load of your data in the application, except if youwant to work with a cache OR by working with all data each X hours for exemple.
– LeBigCat
Dec 28 '18 at 16:37
He right, in others words, you dont have to load of your data in the application, except if youwant to work with a cache OR by working with all data each X hours for exemple.
– LeBigCat
Dec 28 '18 at 16:37
hello all, it's true had not thought about the stored procedure. I will try this solution and put the result.
– Tiago Santos Monteiro
Dec 29 '18 at 10:31
hello all, it's true had not thought about the stored procedure. I will try this solution and put the result.
– Tiago Santos Monteiro
Dec 29 '18 at 10:31
add a comment |
1 Answer
1
active
oldest
votes
You can use LINQ to join two dictionaries. I'm not sure what operations you want to perform on unmatched records.
var query =
from _d1 in d1
join _d2 in d2 on _d1.Key equals _d2.Key
where _d1.Value != _d2.Value
select new { _d1, _d2 };
foreach (var item in query)
{
Console.WriteLine($"Key:{item._d1.Key} Value 1: {item._d1.Value}, Value 2: {item._d2.Value}");
// do something...
}
I agree with @Hirasawa Yui. If possible try writing stored procedure to load and filter data.
– Sria Pathre
Dec 28 '18 at 16:48
pushkin, with this code I also managed to make the routine work as it has to be. thank you all!.
– Tiago Santos Monteiro
Dec 29 '18 at 10:38
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use LINQ to join two dictionaries. I'm not sure what operations you want to perform on unmatched records.
var query =
from _d1 in d1
join _d2 in d2 on _d1.Key equals _d2.Key
where _d1.Value != _d2.Value
select new { _d1, _d2 };
foreach (var item in query)
{
Console.WriteLine($"Key:{item._d1.Key} Value 1: {item._d1.Value}, Value 2: {item._d2.Value}");
// do something...
}
I agree with @Hirasawa Yui. If possible try writing stored procedure to load and filter data.
– Sria Pathre
Dec 28 '18 at 16:48
pushkin, with this code I also managed to make the routine work as it has to be. thank you all!.
– Tiago Santos Monteiro
Dec 29 '18 at 10:38
add a comment |
You can use LINQ to join two dictionaries. I'm not sure what operations you want to perform on unmatched records.
var query =
from _d1 in d1
join _d2 in d2 on _d1.Key equals _d2.Key
where _d1.Value != _d2.Value
select new { _d1, _d2 };
foreach (var item in query)
{
Console.WriteLine($"Key:{item._d1.Key} Value 1: {item._d1.Value}, Value 2: {item._d2.Value}");
// do something...
}
I agree with @Hirasawa Yui. If possible try writing stored procedure to load and filter data.
– Sria Pathre
Dec 28 '18 at 16:48
pushkin, with this code I also managed to make the routine work as it has to be. thank you all!.
– Tiago Santos Monteiro
Dec 29 '18 at 10:38
add a comment |
You can use LINQ to join two dictionaries. I'm not sure what operations you want to perform on unmatched records.
var query =
from _d1 in d1
join _d2 in d2 on _d1.Key equals _d2.Key
where _d1.Value != _d2.Value
select new { _d1, _d2 };
foreach (var item in query)
{
Console.WriteLine($"Key:{item._d1.Key} Value 1: {item._d1.Value}, Value 2: {item._d2.Value}");
// do something...
}
You can use LINQ to join two dictionaries. I'm not sure what operations you want to perform on unmatched records.
var query =
from _d1 in d1
join _d2 in d2 on _d1.Key equals _d2.Key
where _d1.Value != _d2.Value
select new { _d1, _d2 };
foreach (var item in query)
{
Console.WriteLine($"Key:{item._d1.Key} Value 1: {item._d1.Value}, Value 2: {item._d2.Value}");
// do something...
}
edited Jan 7 at 23:20
answered Dec 28 '18 at 16:44


Sria PathreSria Pathre
1046
1046
I agree with @Hirasawa Yui. If possible try writing stored procedure to load and filter data.
– Sria Pathre
Dec 28 '18 at 16:48
pushkin, with this code I also managed to make the routine work as it has to be. thank you all!.
– Tiago Santos Monteiro
Dec 29 '18 at 10:38
add a comment |
I agree with @Hirasawa Yui. If possible try writing stored procedure to load and filter data.
– Sria Pathre
Dec 28 '18 at 16:48
pushkin, with this code I also managed to make the routine work as it has to be. thank you all!.
– Tiago Santos Monteiro
Dec 29 '18 at 10:38
I agree with @Hirasawa Yui. If possible try writing stored procedure to load and filter data.
– Sria Pathre
Dec 28 '18 at 16:48
I agree with @Hirasawa Yui. If possible try writing stored procedure to load and filter data.
– Sria Pathre
Dec 28 '18 at 16:48
pushkin, with this code I also managed to make the routine work as it has to be. thank you all!.
– Tiago Santos Monteiro
Dec 29 '18 at 10:38
pushkin, with this code I also managed to make the routine work as it has to be. thank you all!.
– Tiago Santos Monteiro
Dec 29 '18 at 10:38
add a comment |
UFJUQ,Tr7TF7h8F8bozR,22sRWAabhWh Rn3GQiIxe,fv
1
Oh boy, this is gonna be SLOW as hell! Why don't you create a stored procedure in your DB and use some SQL joins to make it return the result set you need? Alternatively, you can use linq but that's gonna be way slower than the stored procedure.
– Hirasawa Yui
Dec 28 '18 at 16:25
He right, in others words, you dont have to load of your data in the application, except if youwant to work with a cache OR by working with all data each X hours for exemple.
– LeBigCat
Dec 28 '18 at 16:37
hello all, it's true had not thought about the stored procedure. I will try this solution and put the result.
– Tiago Santos Monteiro
Dec 29 '18 at 10:31