How can I test a Keras model on a group of images?
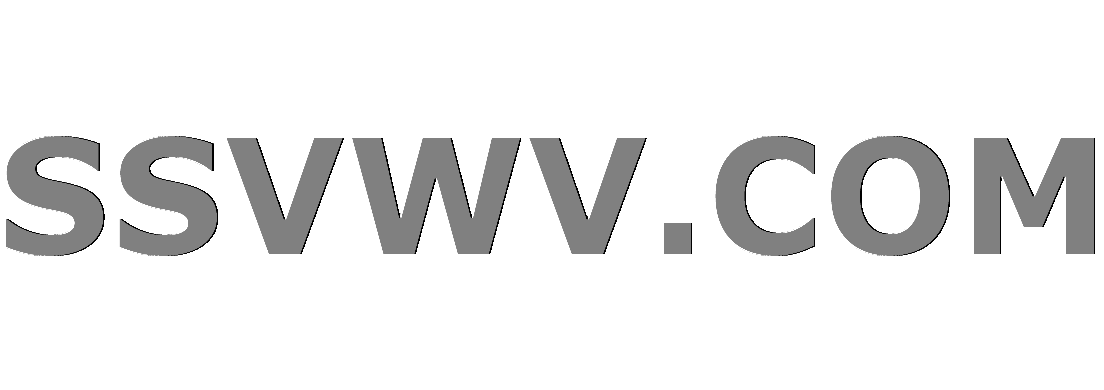
Multi tool use
I have the following in Keras:
train_data = ImageDataGenerator(rescale=1.0/255)
validation_data = ImageDataGenerator(rescale=1.0/255)
test_data = ImageDataGenerator(rescale=1.0/255)
train_features = np.zeros(shape=(number_of_training_samples,16,16,512))
train_labels = np.zeros(shape=(number_of_training_samples))
train_generator = train_data.flow_from_directory(
train_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=True)
i = 0
for inputs_batch, labels_batch in train_generator:
features_batch = conv_base.predict(inputs_batch)
train_features[i*batch_size:(i+1)*batch_size] = features_batch
train_labels[i*batch_size:(i+1)*batch_size] = labels_batch
i += 1
if i * batch_size >= number_of_training_samples:
break
train_features = np.reshape(train_features, (number_of_training_samples,16*16*512))
validation_features = np.zeros(shape=(number_of_validation_samples,16,16,512))
validation_labels = np.zeros(shape=(number_of_validation_samples))
validation_generator = validation_data.flow_from_directory(
validation_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=False)
i = 0
for inputs_batch, labels_batch in validation_generator:
features_batch = conv_base.predict(inputs_batch)
validation_features[i*batch_size:(i+1)*batch_size] = features_batch
validation_labels[i*batch_size:(i+1)*batch_size] = labels_batch
i += 1
if i * batch_size >= number_of_validation_samples:
break
validation_features = np.reshape(validation_features, (number_of_validation_samples,16*16*512))
test_generator = test_data.flow_from_directory(
test_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=False)
# define the Convolutional Neural Network (CNN) model
model = models.Sequential()
model.add(layers.Dense(1024,activation='relu',input_dim=16*16*512))
model.add(layers.Dense(1,activation='sigmoid'))
# compile the model
model.compile(loss='binary_crossentropy',
optimizer=optimizers.Adam(lr=0.01),
metrics=['acc'])
# fit the model to the data
history = model.fit(train_features,
train_labels,
epochs=1,
batch_size=batch_size,
validation_data=(validation_features,validation_labels))
# save the model
model.save('model.h5')
I tried to test the model on a group of images, as follows:
for root, dirs, files in os.walk(test_directory):
for file in files:
img = cv2.imread(root + '/' + file)
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
img = cv2.resize(img, (512,512))
img_class = model.predict_classes(img)
prediction = img_class[0]
I'm however getting:
ValueError: Error when checking : expected dense_1_input to have shape (None, 131072) but got array with shape (512, 512)
On the line:
img_class = model.predict_classes(img)
Why is that?
Thanks.
python keras
|
show 1 more comment
I have the following in Keras:
train_data = ImageDataGenerator(rescale=1.0/255)
validation_data = ImageDataGenerator(rescale=1.0/255)
test_data = ImageDataGenerator(rescale=1.0/255)
train_features = np.zeros(shape=(number_of_training_samples,16,16,512))
train_labels = np.zeros(shape=(number_of_training_samples))
train_generator = train_data.flow_from_directory(
train_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=True)
i = 0
for inputs_batch, labels_batch in train_generator:
features_batch = conv_base.predict(inputs_batch)
train_features[i*batch_size:(i+1)*batch_size] = features_batch
train_labels[i*batch_size:(i+1)*batch_size] = labels_batch
i += 1
if i * batch_size >= number_of_training_samples:
break
train_features = np.reshape(train_features, (number_of_training_samples,16*16*512))
validation_features = np.zeros(shape=(number_of_validation_samples,16,16,512))
validation_labels = np.zeros(shape=(number_of_validation_samples))
validation_generator = validation_data.flow_from_directory(
validation_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=False)
i = 0
for inputs_batch, labels_batch in validation_generator:
features_batch = conv_base.predict(inputs_batch)
validation_features[i*batch_size:(i+1)*batch_size] = features_batch
validation_labels[i*batch_size:(i+1)*batch_size] = labels_batch
i += 1
if i * batch_size >= number_of_validation_samples:
break
validation_features = np.reshape(validation_features, (number_of_validation_samples,16*16*512))
test_generator = test_data.flow_from_directory(
test_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=False)
# define the Convolutional Neural Network (CNN) model
model = models.Sequential()
model.add(layers.Dense(1024,activation='relu',input_dim=16*16*512))
model.add(layers.Dense(1,activation='sigmoid'))
# compile the model
model.compile(loss='binary_crossentropy',
optimizer=optimizers.Adam(lr=0.01),
metrics=['acc'])
# fit the model to the data
history = model.fit(train_features,
train_labels,
epochs=1,
batch_size=batch_size,
validation_data=(validation_features,validation_labels))
# save the model
model.save('model.h5')
I tried to test the model on a group of images, as follows:
for root, dirs, files in os.walk(test_directory):
for file in files:
img = cv2.imread(root + '/' + file)
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
img = cv2.resize(img, (512,512))
img_class = model.predict_classes(img)
prediction = img_class[0]
I'm however getting:
ValueError: Error when checking : expected dense_1_input to have shape (None, 131072) but got array with shape (512, 512)
On the line:
img_class = model.predict_classes(img)
Why is that?
Thanks.
python keras
1
Your first problem is that you define your model's input size as16*16*512
while you are trying to feed it in your test with image of shape(512, 512)
.
– pouyan
Jan 2 at 5:32
Thanks for your kind reply. I'm trying to do "transfer learning", and have the following: conv_base = VGG16(weights='imagenet',include_top=False,input_shape=(512,512,3)). I used "input_dim=16*16*512" because the input to the classifier would be that dimension. It seems that I might be confusing things here? What changes do you recommend to the code?
– Simplicity
Jan 2 at 5:44
So if I get your point correctly, at first you should feed yourimg
data into yourconv_base
which is yourVGG16
model and then feed it's out put in to yourmodel
( now you are feeding yourimg
into yourmodel
).
– pouyan
Jan 2 at 5:56
Yes, I think you are right. But, not sure what to enter in order to find the model's prediction accuracy on the "test" data
– Simplicity
Jan 2 at 19:33
I think my previous comment exactly address this issue. In the last part of your code, after loading your test image, instead of this line:img_class = model.predict_classes(img)
feed your image into yourconv_base
which is your VGG16 model for example likeout_1= conv_base.predict(img)
And then feedout_1
intor your model likeimg_class = model.predict_classes(out_1)
– pouyan
Jan 3 at 1:47
|
show 1 more comment
I have the following in Keras:
train_data = ImageDataGenerator(rescale=1.0/255)
validation_data = ImageDataGenerator(rescale=1.0/255)
test_data = ImageDataGenerator(rescale=1.0/255)
train_features = np.zeros(shape=(number_of_training_samples,16,16,512))
train_labels = np.zeros(shape=(number_of_training_samples))
train_generator = train_data.flow_from_directory(
train_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=True)
i = 0
for inputs_batch, labels_batch in train_generator:
features_batch = conv_base.predict(inputs_batch)
train_features[i*batch_size:(i+1)*batch_size] = features_batch
train_labels[i*batch_size:(i+1)*batch_size] = labels_batch
i += 1
if i * batch_size >= number_of_training_samples:
break
train_features = np.reshape(train_features, (number_of_training_samples,16*16*512))
validation_features = np.zeros(shape=(number_of_validation_samples,16,16,512))
validation_labels = np.zeros(shape=(number_of_validation_samples))
validation_generator = validation_data.flow_from_directory(
validation_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=False)
i = 0
for inputs_batch, labels_batch in validation_generator:
features_batch = conv_base.predict(inputs_batch)
validation_features[i*batch_size:(i+1)*batch_size] = features_batch
validation_labels[i*batch_size:(i+1)*batch_size] = labels_batch
i += 1
if i * batch_size >= number_of_validation_samples:
break
validation_features = np.reshape(validation_features, (number_of_validation_samples,16*16*512))
test_generator = test_data.flow_from_directory(
test_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=False)
# define the Convolutional Neural Network (CNN) model
model = models.Sequential()
model.add(layers.Dense(1024,activation='relu',input_dim=16*16*512))
model.add(layers.Dense(1,activation='sigmoid'))
# compile the model
model.compile(loss='binary_crossentropy',
optimizer=optimizers.Adam(lr=0.01),
metrics=['acc'])
# fit the model to the data
history = model.fit(train_features,
train_labels,
epochs=1,
batch_size=batch_size,
validation_data=(validation_features,validation_labels))
# save the model
model.save('model.h5')
I tried to test the model on a group of images, as follows:
for root, dirs, files in os.walk(test_directory):
for file in files:
img = cv2.imread(root + '/' + file)
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
img = cv2.resize(img, (512,512))
img_class = model.predict_classes(img)
prediction = img_class[0]
I'm however getting:
ValueError: Error when checking : expected dense_1_input to have shape (None, 131072) but got array with shape (512, 512)
On the line:
img_class = model.predict_classes(img)
Why is that?
Thanks.
python keras
I have the following in Keras:
train_data = ImageDataGenerator(rescale=1.0/255)
validation_data = ImageDataGenerator(rescale=1.0/255)
test_data = ImageDataGenerator(rescale=1.0/255)
train_features = np.zeros(shape=(number_of_training_samples,16,16,512))
train_labels = np.zeros(shape=(number_of_training_samples))
train_generator = train_data.flow_from_directory(
train_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=True)
i = 0
for inputs_batch, labels_batch in train_generator:
features_batch = conv_base.predict(inputs_batch)
train_features[i*batch_size:(i+1)*batch_size] = features_batch
train_labels[i*batch_size:(i+1)*batch_size] = labels_batch
i += 1
if i * batch_size >= number_of_training_samples:
break
train_features = np.reshape(train_features, (number_of_training_samples,16*16*512))
validation_features = np.zeros(shape=(number_of_validation_samples,16,16,512))
validation_labels = np.zeros(shape=(number_of_validation_samples))
validation_generator = validation_data.flow_from_directory(
validation_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=False)
i = 0
for inputs_batch, labels_batch in validation_generator:
features_batch = conv_base.predict(inputs_batch)
validation_features[i*batch_size:(i+1)*batch_size] = features_batch
validation_labels[i*batch_size:(i+1)*batch_size] = labels_batch
i += 1
if i * batch_size >= number_of_validation_samples:
break
validation_features = np.reshape(validation_features, (number_of_validation_samples,16*16*512))
test_generator = test_data.flow_from_directory(
test_directory,
target_size=(512,512),
batch_size=batch_size,
class_mode='binary',
shuffle=False)
# define the Convolutional Neural Network (CNN) model
model = models.Sequential()
model.add(layers.Dense(1024,activation='relu',input_dim=16*16*512))
model.add(layers.Dense(1,activation='sigmoid'))
# compile the model
model.compile(loss='binary_crossentropy',
optimizer=optimizers.Adam(lr=0.01),
metrics=['acc'])
# fit the model to the data
history = model.fit(train_features,
train_labels,
epochs=1,
batch_size=batch_size,
validation_data=(validation_features,validation_labels))
# save the model
model.save('model.h5')
I tried to test the model on a group of images, as follows:
for root, dirs, files in os.walk(test_directory):
for file in files:
img = cv2.imread(root + '/' + file)
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
img = cv2.resize(img, (512,512))
img_class = model.predict_classes(img)
prediction = img_class[0]
I'm however getting:
ValueError: Error when checking : expected dense_1_input to have shape (None, 131072) but got array with shape (512, 512)
On the line:
img_class = model.predict_classes(img)
Why is that?
Thanks.
python keras
python keras
asked Jan 2 at 4:06
SimplicitySimplicity
15.6k70191305
15.6k70191305
1
Your first problem is that you define your model's input size as16*16*512
while you are trying to feed it in your test with image of shape(512, 512)
.
– pouyan
Jan 2 at 5:32
Thanks for your kind reply. I'm trying to do "transfer learning", and have the following: conv_base = VGG16(weights='imagenet',include_top=False,input_shape=(512,512,3)). I used "input_dim=16*16*512" because the input to the classifier would be that dimension. It seems that I might be confusing things here? What changes do you recommend to the code?
– Simplicity
Jan 2 at 5:44
So if I get your point correctly, at first you should feed yourimg
data into yourconv_base
which is yourVGG16
model and then feed it's out put in to yourmodel
( now you are feeding yourimg
into yourmodel
).
– pouyan
Jan 2 at 5:56
Yes, I think you are right. But, not sure what to enter in order to find the model's prediction accuracy on the "test" data
– Simplicity
Jan 2 at 19:33
I think my previous comment exactly address this issue. In the last part of your code, after loading your test image, instead of this line:img_class = model.predict_classes(img)
feed your image into yourconv_base
which is your VGG16 model for example likeout_1= conv_base.predict(img)
And then feedout_1
intor your model likeimg_class = model.predict_classes(out_1)
– pouyan
Jan 3 at 1:47
|
show 1 more comment
1
Your first problem is that you define your model's input size as16*16*512
while you are trying to feed it in your test with image of shape(512, 512)
.
– pouyan
Jan 2 at 5:32
Thanks for your kind reply. I'm trying to do "transfer learning", and have the following: conv_base = VGG16(weights='imagenet',include_top=False,input_shape=(512,512,3)). I used "input_dim=16*16*512" because the input to the classifier would be that dimension. It seems that I might be confusing things here? What changes do you recommend to the code?
– Simplicity
Jan 2 at 5:44
So if I get your point correctly, at first you should feed yourimg
data into yourconv_base
which is yourVGG16
model and then feed it's out put in to yourmodel
( now you are feeding yourimg
into yourmodel
).
– pouyan
Jan 2 at 5:56
Yes, I think you are right. But, not sure what to enter in order to find the model's prediction accuracy on the "test" data
– Simplicity
Jan 2 at 19:33
I think my previous comment exactly address this issue. In the last part of your code, after loading your test image, instead of this line:img_class = model.predict_classes(img)
feed your image into yourconv_base
which is your VGG16 model for example likeout_1= conv_base.predict(img)
And then feedout_1
intor your model likeimg_class = model.predict_classes(out_1)
– pouyan
Jan 3 at 1:47
1
1
Your first problem is that you define your model's input size as
16*16*512
while you are trying to feed it in your test with image of shape (512, 512)
.– pouyan
Jan 2 at 5:32
Your first problem is that you define your model's input size as
16*16*512
while you are trying to feed it in your test with image of shape (512, 512)
.– pouyan
Jan 2 at 5:32
Thanks for your kind reply. I'm trying to do "transfer learning", and have the following: conv_base = VGG16(weights='imagenet',include_top=False,input_shape=(512,512,3)). I used "input_dim=16*16*512" because the input to the classifier would be that dimension. It seems that I might be confusing things here? What changes do you recommend to the code?
– Simplicity
Jan 2 at 5:44
Thanks for your kind reply. I'm trying to do "transfer learning", and have the following: conv_base = VGG16(weights='imagenet',include_top=False,input_shape=(512,512,3)). I used "input_dim=16*16*512" because the input to the classifier would be that dimension. It seems that I might be confusing things here? What changes do you recommend to the code?
– Simplicity
Jan 2 at 5:44
So if I get your point correctly, at first you should feed your
img
data into your conv_base
which is your VGG16
model and then feed it's out put in to your model
( now you are feeding your img
into your model
).– pouyan
Jan 2 at 5:56
So if I get your point correctly, at first you should feed your
img
data into your conv_base
which is your VGG16
model and then feed it's out put in to your model
( now you are feeding your img
into your model
).– pouyan
Jan 2 at 5:56
Yes, I think you are right. But, not sure what to enter in order to find the model's prediction accuracy on the "test" data
– Simplicity
Jan 2 at 19:33
Yes, I think you are right. But, not sure what to enter in order to find the model's prediction accuracy on the "test" data
– Simplicity
Jan 2 at 19:33
I think my previous comment exactly address this issue. In the last part of your code, after loading your test image, instead of this line:
img_class = model.predict_classes(img)
feed your image into your conv_base
which is your VGG16 model for example like out_1= conv_base.predict(img)
And then feed out_1
intor your model like img_class = model.predict_classes(out_1)
– pouyan
Jan 3 at 1:47
I think my previous comment exactly address this issue. In the last part of your code, after loading your test image, instead of this line:
img_class = model.predict_classes(img)
feed your image into your conv_base
which is your VGG16 model for example like out_1= conv_base.predict(img)
And then feed out_1
intor your model like img_class = model.predict_classes(out_1)
– pouyan
Jan 3 at 1:47
|
show 1 more comment
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54001077%2fhow-can-i-test-a-keras-model-on-a-group-of-images%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54001077%2fhow-can-i-test-a-keras-model-on-a-group-of-images%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ReldMMAMSKLjTC4SrjtG5QFwnRv0BrLpWS,sN8UFkMLbP xahEQh
1
Your first problem is that you define your model's input size as
16*16*512
while you are trying to feed it in your test with image of shape(512, 512)
.– pouyan
Jan 2 at 5:32
Thanks for your kind reply. I'm trying to do "transfer learning", and have the following: conv_base = VGG16(weights='imagenet',include_top=False,input_shape=(512,512,3)). I used "input_dim=16*16*512" because the input to the classifier would be that dimension. It seems that I might be confusing things here? What changes do you recommend to the code?
– Simplicity
Jan 2 at 5:44
So if I get your point correctly, at first you should feed your
img
data into yourconv_base
which is yourVGG16
model and then feed it's out put in to yourmodel
( now you are feeding yourimg
into yourmodel
).– pouyan
Jan 2 at 5:56
Yes, I think you are right. But, not sure what to enter in order to find the model's prediction accuracy on the "test" data
– Simplicity
Jan 2 at 19:33
I think my previous comment exactly address this issue. In the last part of your code, after loading your test image, instead of this line:
img_class = model.predict_classes(img)
feed your image into yourconv_base
which is your VGG16 model for example likeout_1= conv_base.predict(img)
And then feedout_1
intor your model likeimg_class = model.predict_classes(out_1)
– pouyan
Jan 3 at 1:47