Function that does loop over various enum
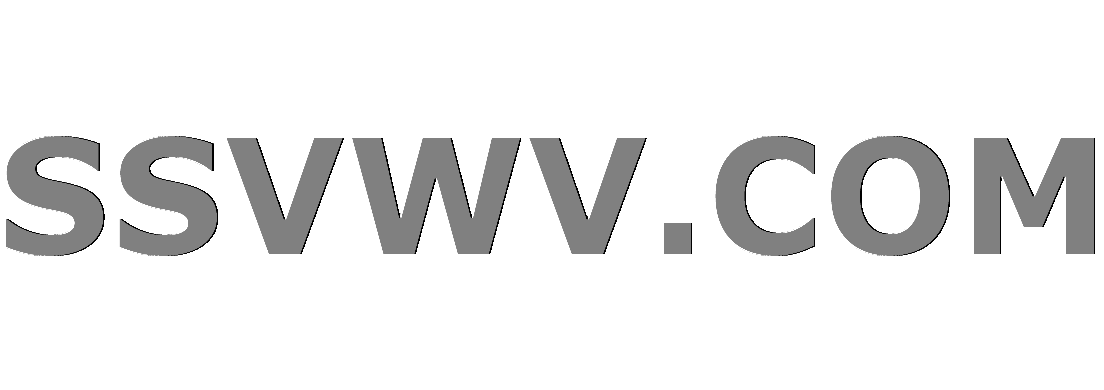
Multi tool use
For example, let be enum and let us loop over it
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
Console.ReadLine();
}
This enum represents very simple type menu, in the program i work on, i have to use this kind of menus a several times, so i prefer to add it in a separate function, by targeting the part of code with loop and using hotkeys Ctrl+Dot i received following:
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
k = LoopOverEnum(k);
Console.ReadLine();
}
private static int LoopOverEnum(int k)
{
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
return k;
}
}
Now, I want to add enum Main_menu as variable, so, i will be able to apply this loop over every defined enums, I try to do it as follows:
namespace ConsoleApp11
{
class Program
{
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
k = LoopOverEnum(k, Main_menu);
Console.ReadLine();
}
private static int LoopOverEnum(int k, enum Main_menu)
{
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
return k;
}
}
}
But the function's code becomes fully red with different errors. The questions are:
1) How to write the function that loops over enum, in sence that enum is variable ?
2) How to use space in definition of the enum terms ?
c# enums enumeration
|
show 2 more comments
For example, let be enum and let us loop over it
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
Console.ReadLine();
}
This enum represents very simple type menu, in the program i work on, i have to use this kind of menus a several times, so i prefer to add it in a separate function, by targeting the part of code with loop and using hotkeys Ctrl+Dot i received following:
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
k = LoopOverEnum(k);
Console.ReadLine();
}
private static int LoopOverEnum(int k)
{
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
return k;
}
}
Now, I want to add enum Main_menu as variable, so, i will be able to apply this loop over every defined enums, I try to do it as follows:
namespace ConsoleApp11
{
class Program
{
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
k = LoopOverEnum(k, Main_menu);
Console.ReadLine();
}
private static int LoopOverEnum(int k, enum Main_menu)
{
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
return k;
}
}
}
But the function's code becomes fully red with different errors. The questions are:
1) How to write the function that loops over enum, in sence that enum is variable ?
2) How to use space in definition of the enum terms ?
c# enums enumeration
How to use space in definition of the enum terms
please clarify this
– Michael Randall
Jan 2 at 4:18
2
You cannot Have spaces in the Enum value Names. @TheGeneral - He wants Enum items like: First Value = 0, Second Value = 1.... So spaces in the Name Identifier.
– Prateek Shrivastava
Jan 2 at 4:19
Since you know what parameters GetValues method takes it is unclear why code shows such a strange ‘enum Main_menu’ parameter
– Alexei Levenkov
Jan 2 at 4:21
k = LoopOverEnum(k, Main_menu); --- You cant pass Enum Name as the parameneter here. Create a variable for it like var obj = new Main_menu(); then pass this variable in the LoopOver method
– Prateek Shrivastava
Jan 2 at 4:27
1
This may be a possible duplicate of stackoverflow.com/questions/7966102/…
– JayC
Jan 2 at 4:39
|
show 2 more comments
For example, let be enum and let us loop over it
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
Console.ReadLine();
}
This enum represents very simple type menu, in the program i work on, i have to use this kind of menus a several times, so i prefer to add it in a separate function, by targeting the part of code with loop and using hotkeys Ctrl+Dot i received following:
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
k = LoopOverEnum(k);
Console.ReadLine();
}
private static int LoopOverEnum(int k)
{
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
return k;
}
}
Now, I want to add enum Main_menu as variable, so, i will be able to apply this loop over every defined enums, I try to do it as follows:
namespace ConsoleApp11
{
class Program
{
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
k = LoopOverEnum(k, Main_menu);
Console.ReadLine();
}
private static int LoopOverEnum(int k, enum Main_menu)
{
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
return k;
}
}
}
But the function's code becomes fully red with different errors. The questions are:
1) How to write the function that loops over enum, in sence that enum is variable ?
2) How to use space in definition of the enum terms ?
c# enums enumeration
For example, let be enum and let us loop over it
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
Console.ReadLine();
}
This enum represents very simple type menu, in the program i work on, i have to use this kind of menus a several times, so i prefer to add it in a separate function, by targeting the part of code with loop and using hotkeys Ctrl+Dot i received following:
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
k = LoopOverEnum(k);
Console.ReadLine();
}
private static int LoopOverEnum(int k)
{
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
return k;
}
}
Now, I want to add enum Main_menu as variable, so, i will be able to apply this loop over every defined enums, I try to do it as follows:
namespace ConsoleApp11
{
class Program
{
enum Main_menu { NewGame, Options, AboutAuthor, Exit }
static void Main(string args)
{
int k = 0;
k = LoopOverEnum(k, Main_menu);
Console.ReadLine();
}
private static int LoopOverEnum(int k, enum Main_menu)
{
foreach (Main_menu item in Enum.GetValues(typeof(Main_menu)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
return k;
}
}
}
But the function's code becomes fully red with different errors. The questions are:
1) How to write the function that loops over enum, in sence that enum is variable ?
2) How to use space in definition of the enum terms ?
c# enums enumeration
c# enums enumeration
asked Jan 2 at 4:09
Petro KolosovPetro Kolosov
114
114
How to use space in definition of the enum terms
please clarify this
– Michael Randall
Jan 2 at 4:18
2
You cannot Have spaces in the Enum value Names. @TheGeneral - He wants Enum items like: First Value = 0, Second Value = 1.... So spaces in the Name Identifier.
– Prateek Shrivastava
Jan 2 at 4:19
Since you know what parameters GetValues method takes it is unclear why code shows such a strange ‘enum Main_menu’ parameter
– Alexei Levenkov
Jan 2 at 4:21
k = LoopOverEnum(k, Main_menu); --- You cant pass Enum Name as the parameneter here. Create a variable for it like var obj = new Main_menu(); then pass this variable in the LoopOver method
– Prateek Shrivastava
Jan 2 at 4:27
1
This may be a possible duplicate of stackoverflow.com/questions/7966102/…
– JayC
Jan 2 at 4:39
|
show 2 more comments
How to use space in definition of the enum terms
please clarify this
– Michael Randall
Jan 2 at 4:18
2
You cannot Have spaces in the Enum value Names. @TheGeneral - He wants Enum items like: First Value = 0, Second Value = 1.... So spaces in the Name Identifier.
– Prateek Shrivastava
Jan 2 at 4:19
Since you know what parameters GetValues method takes it is unclear why code shows such a strange ‘enum Main_menu’ parameter
– Alexei Levenkov
Jan 2 at 4:21
k = LoopOverEnum(k, Main_menu); --- You cant pass Enum Name as the parameneter here. Create a variable for it like var obj = new Main_menu(); then pass this variable in the LoopOver method
– Prateek Shrivastava
Jan 2 at 4:27
1
This may be a possible duplicate of stackoverflow.com/questions/7966102/…
– JayC
Jan 2 at 4:39
How to use space in definition of the enum terms
please clarify this– Michael Randall
Jan 2 at 4:18
How to use space in definition of the enum terms
please clarify this– Michael Randall
Jan 2 at 4:18
2
2
You cannot Have spaces in the Enum value Names. @TheGeneral - He wants Enum items like: First Value = 0, Second Value = 1.... So spaces in the Name Identifier.
– Prateek Shrivastava
Jan 2 at 4:19
You cannot Have spaces in the Enum value Names. @TheGeneral - He wants Enum items like: First Value = 0, Second Value = 1.... So spaces in the Name Identifier.
– Prateek Shrivastava
Jan 2 at 4:19
Since you know what parameters GetValues method takes it is unclear why code shows such a strange ‘enum Main_menu’ parameter
– Alexei Levenkov
Jan 2 at 4:21
Since you know what parameters GetValues method takes it is unclear why code shows such a strange ‘enum Main_menu’ parameter
– Alexei Levenkov
Jan 2 at 4:21
k = LoopOverEnum(k, Main_menu); --- You cant pass Enum Name as the parameneter here. Create a variable for it like var obj = new Main_menu(); then pass this variable in the LoopOver method
– Prateek Shrivastava
Jan 2 at 4:27
k = LoopOverEnum(k, Main_menu); --- You cant pass Enum Name as the parameneter here. Create a variable for it like var obj = new Main_menu(); then pass this variable in the LoopOver method
– Prateek Shrivastava
Jan 2 at 4:27
1
1
This may be a possible duplicate of stackoverflow.com/questions/7966102/…
– JayC
Jan 2 at 4:39
This may be a possible duplicate of stackoverflow.com/questions/7966102/…
– JayC
Jan 2 at 4:39
|
show 2 more comments
4 Answers
4
active
oldest
votes
k
should not be a local variable in Main
. It should be a local variable in LoopOverEnum
.
There are two ways to pass a type to a method. Your attempt of LoopOverEnum(int k, enum Main_menu)
is a good try but is unfortunately not valid C#.
You can either:
use a generic type parameter:
public static void LoopOverEnum<T>()
int k = 0;
foreach (T item in Enum.GetValues(typeof(T)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum<Main_menu>()
use a parameter of type
Type
:
public static void LoopOverEnum(Type type)
int k = 0;
foreach (var item in Enum.GetValues(type))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum(typeof(Main_menu))
add a comment |
Here's a generic way to Loop over Enums:
static void IterateEnum<T>(T enumSource)
{
foreach(var item in Enum.GetValues(typeof(T)))
{
Console.WriteLine(item);
}
}
add a comment |
public static IEnumerable<T> GetValues<T>() => Enum.GetValues(typeof(T)).Cast<T>();
Usage:
foreach (Main_menu item in GetValues<Main_menu>())
MessageBox.Show(item.ToString());
add a comment |
To iterate over enum of any type And
To have spaces in enum values
you can use description attribute as shown below. You can get the values mentioned in descriptionAttribute using the below method. Method is generic and hence can be used with any enum.
using System.ComponentModel;
public enum MyEnum
{
[Description("value 1")]
Value1,
[Description("value 2")]
Value2,
[Description("value 3")]
Value3
}
public static string GetDescription<T>( T e)
{
if (e is Enum)
{
Type type = e.GetType();
Array values = System.Enum.GetValues(type);
foreach (int val in values)
{
if (val == e.ToInt32(CultureInfo.InvariantCulture))
{
var memInfo = type.GetMember(type.GetEnumName(val));
var descriptionAttribute = memInfo[0].GetCustomAttributes(typeof(DescriptionAttribute), false).FirstOrDefault() as DescriptionAttribute;
if (descriptionAttribute != null)
{
return descriptionAttribute.Description;
}
}
}
}
return null; // could also return string.Empty
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54001092%2ffunction-that-does-loop-over-various-enum%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
k
should not be a local variable in Main
. It should be a local variable in LoopOverEnum
.
There are two ways to pass a type to a method. Your attempt of LoopOverEnum(int k, enum Main_menu)
is a good try but is unfortunately not valid C#.
You can either:
use a generic type parameter:
public static void LoopOverEnum<T>()
int k = 0;
foreach (T item in Enum.GetValues(typeof(T)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum<Main_menu>()
use a parameter of type
Type
:
public static void LoopOverEnum(Type type)
int k = 0;
foreach (var item in Enum.GetValues(type))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum(typeof(Main_menu))
add a comment |
k
should not be a local variable in Main
. It should be a local variable in LoopOverEnum
.
There are two ways to pass a type to a method. Your attempt of LoopOverEnum(int k, enum Main_menu)
is a good try but is unfortunately not valid C#.
You can either:
use a generic type parameter:
public static void LoopOverEnum<T>()
int k = 0;
foreach (T item in Enum.GetValues(typeof(T)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum<Main_menu>()
use a parameter of type
Type
:
public static void LoopOverEnum(Type type)
int k = 0;
foreach (var item in Enum.GetValues(type))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum(typeof(Main_menu))
add a comment |
k
should not be a local variable in Main
. It should be a local variable in LoopOverEnum
.
There are two ways to pass a type to a method. Your attempt of LoopOverEnum(int k, enum Main_menu)
is a good try but is unfortunately not valid C#.
You can either:
use a generic type parameter:
public static void LoopOverEnum<T>()
int k = 0;
foreach (T item in Enum.GetValues(typeof(T)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum<Main_menu>()
use a parameter of type
Type
:
public static void LoopOverEnum(Type type)
int k = 0;
foreach (var item in Enum.GetValues(type))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum(typeof(Main_menu))
k
should not be a local variable in Main
. It should be a local variable in LoopOverEnum
.
There are two ways to pass a type to a method. Your attempt of LoopOverEnum(int k, enum Main_menu)
is a good try but is unfortunately not valid C#.
You can either:
use a generic type parameter:
public static void LoopOverEnum<T>()
int k = 0;
foreach (T item in Enum.GetValues(typeof(T)))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum<Main_menu>()
use a parameter of type
Type
:
public static void LoopOverEnum(Type type)
int k = 0;
foreach (var item in Enum.GetValues(type))
{
Console.Write($"{k} ");
Console.Write(item);
Console.WriteLine();
k++;
}
}
// usage:
LoopOverEnum(typeof(Main_menu))
answered Jan 2 at 4:38


SweeperSweeper
69.3k1074140
69.3k1074140
add a comment |
add a comment |
Here's a generic way to Loop over Enums:
static void IterateEnum<T>(T enumSource)
{
foreach(var item in Enum.GetValues(typeof(T)))
{
Console.WriteLine(item);
}
}
add a comment |
Here's a generic way to Loop over Enums:
static void IterateEnum<T>(T enumSource)
{
foreach(var item in Enum.GetValues(typeof(T)))
{
Console.WriteLine(item);
}
}
add a comment |
Here's a generic way to Loop over Enums:
static void IterateEnum<T>(T enumSource)
{
foreach(var item in Enum.GetValues(typeof(T)))
{
Console.WriteLine(item);
}
}
Here's a generic way to Loop over Enums:
static void IterateEnum<T>(T enumSource)
{
foreach(var item in Enum.GetValues(typeof(T)))
{
Console.WriteLine(item);
}
}
answered Jan 2 at 4:28
Prateek ShrivastavaPrateek Shrivastava
990512
990512
add a comment |
add a comment |
public static IEnumerable<T> GetValues<T>() => Enum.GetValues(typeof(T)).Cast<T>();
Usage:
foreach (Main_menu item in GetValues<Main_menu>())
MessageBox.Show(item.ToString());
add a comment |
public static IEnumerable<T> GetValues<T>() => Enum.GetValues(typeof(T)).Cast<T>();
Usage:
foreach (Main_menu item in GetValues<Main_menu>())
MessageBox.Show(item.ToString());
add a comment |
public static IEnumerable<T> GetValues<T>() => Enum.GetValues(typeof(T)).Cast<T>();
Usage:
foreach (Main_menu item in GetValues<Main_menu>())
MessageBox.Show(item.ToString());
public static IEnumerable<T> GetValues<T>() => Enum.GetValues(typeof(T)).Cast<T>();
Usage:
foreach (Main_menu item in GetValues<Main_menu>())
MessageBox.Show(item.ToString());
answered Jan 2 at 4:31


CodeManCodeMan
557311
557311
add a comment |
add a comment |
To iterate over enum of any type And
To have spaces in enum values
you can use description attribute as shown below. You can get the values mentioned in descriptionAttribute using the below method. Method is generic and hence can be used with any enum.
using System.ComponentModel;
public enum MyEnum
{
[Description("value 1")]
Value1,
[Description("value 2")]
Value2,
[Description("value 3")]
Value3
}
public static string GetDescription<T>( T e)
{
if (e is Enum)
{
Type type = e.GetType();
Array values = System.Enum.GetValues(type);
foreach (int val in values)
{
if (val == e.ToInt32(CultureInfo.InvariantCulture))
{
var memInfo = type.GetMember(type.GetEnumName(val));
var descriptionAttribute = memInfo[0].GetCustomAttributes(typeof(DescriptionAttribute), false).FirstOrDefault() as DescriptionAttribute;
if (descriptionAttribute != null)
{
return descriptionAttribute.Description;
}
}
}
}
return null; // could also return string.Empty
}
add a comment |
To iterate over enum of any type And
To have spaces in enum values
you can use description attribute as shown below. You can get the values mentioned in descriptionAttribute using the below method. Method is generic and hence can be used with any enum.
using System.ComponentModel;
public enum MyEnum
{
[Description("value 1")]
Value1,
[Description("value 2")]
Value2,
[Description("value 3")]
Value3
}
public static string GetDescription<T>( T e)
{
if (e is Enum)
{
Type type = e.GetType();
Array values = System.Enum.GetValues(type);
foreach (int val in values)
{
if (val == e.ToInt32(CultureInfo.InvariantCulture))
{
var memInfo = type.GetMember(type.GetEnumName(val));
var descriptionAttribute = memInfo[0].GetCustomAttributes(typeof(DescriptionAttribute), false).FirstOrDefault() as DescriptionAttribute;
if (descriptionAttribute != null)
{
return descriptionAttribute.Description;
}
}
}
}
return null; // could also return string.Empty
}
add a comment |
To iterate over enum of any type And
To have spaces in enum values
you can use description attribute as shown below. You can get the values mentioned in descriptionAttribute using the below method. Method is generic and hence can be used with any enum.
using System.ComponentModel;
public enum MyEnum
{
[Description("value 1")]
Value1,
[Description("value 2")]
Value2,
[Description("value 3")]
Value3
}
public static string GetDescription<T>( T e)
{
if (e is Enum)
{
Type type = e.GetType();
Array values = System.Enum.GetValues(type);
foreach (int val in values)
{
if (val == e.ToInt32(CultureInfo.InvariantCulture))
{
var memInfo = type.GetMember(type.GetEnumName(val));
var descriptionAttribute = memInfo[0].GetCustomAttributes(typeof(DescriptionAttribute), false).FirstOrDefault() as DescriptionAttribute;
if (descriptionAttribute != null)
{
return descriptionAttribute.Description;
}
}
}
}
return null; // could also return string.Empty
}
To iterate over enum of any type And
To have spaces in enum values
you can use description attribute as shown below. You can get the values mentioned in descriptionAttribute using the below method. Method is generic and hence can be used with any enum.
using System.ComponentModel;
public enum MyEnum
{
[Description("value 1")]
Value1,
[Description("value 2")]
Value2,
[Description("value 3")]
Value3
}
public static string GetDescription<T>( T e)
{
if (e is Enum)
{
Type type = e.GetType();
Array values = System.Enum.GetValues(type);
foreach (int val in values)
{
if (val == e.ToInt32(CultureInfo.InvariantCulture))
{
var memInfo = type.GetMember(type.GetEnumName(val));
var descriptionAttribute = memInfo[0].GetCustomAttributes(typeof(DescriptionAttribute), false).FirstOrDefault() as DescriptionAttribute;
if (descriptionAttribute != null)
{
return descriptionAttribute.Description;
}
}
}
}
return null; // could also return string.Empty
}
edited Jan 2 at 6:01
answered Jan 2 at 5:54
Manoj ChoudhariManoj Choudhari
1,9881616
1,9881616
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54001092%2ffunction-that-does-loop-over-various-enum%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CPUQJchPgbN,L,cX gUTusCXP,gdoI KtV,UUd brCrZb,v8tYM
How to use space in definition of the enum terms
please clarify this– Michael Randall
Jan 2 at 4:18
2
You cannot Have spaces in the Enum value Names. @TheGeneral - He wants Enum items like: First Value = 0, Second Value = 1.... So spaces in the Name Identifier.
– Prateek Shrivastava
Jan 2 at 4:19
Since you know what parameters GetValues method takes it is unclear why code shows such a strange ‘enum Main_menu’ parameter
– Alexei Levenkov
Jan 2 at 4:21
k = LoopOverEnum(k, Main_menu); --- You cant pass Enum Name as the parameneter here. Create a variable for it like var obj = new Main_menu(); then pass this variable in the LoopOver method
– Prateek Shrivastava
Jan 2 at 4:27
1
This may be a possible duplicate of stackoverflow.com/questions/7966102/…
– JayC
Jan 2 at 4:39