Recycler view not calling getItemCount
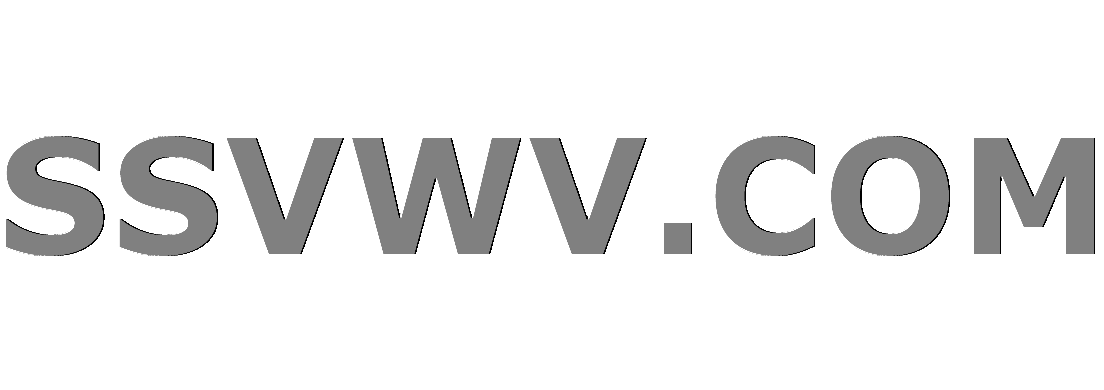
Multi tool use
I just made a recycler view and it was not working, so I put a breakpoint on getItemCount and the method is not being called. I have never seen anyone else on SO have that particular issue, I am sure it is something ridiculously obvious. Here is my code.
public class SearchAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
private ArrayList<Object> displayList;
public static class ViewHolder extends RecyclerView.ViewHolder {
public ViewHolder(View v) {
super(v);
}
}
public class ErrorSearchItem extends ViewHolder {
//this is here if there is no other viewholder
public ErrorSearchItem(View view) {
super(view);
}
}
public class HeaderViewHolder extends ViewHolder {
public HeaderViewHolder(View view) {
super(view);
}
}
@Override
public int getItemViewType(int position) {
return position;
}
public SearchAdapter(ArrayList<Object> displayList) {
this.displayList = displayList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
if (displayList.get(viewType) instanceof String){
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.row_search_title, parent, false);
return new HeaderViewHolder(itemView);
}
else{ //this is for if there is an error and no other xml files match
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.row_error_search_item, parent, false);
return new ErrorSearchItem(itemView);
}
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
}
@Override
public int getItemCount() {
return displayList.size();
}
@Override
public void onAttachedToRecyclerView(RecyclerView recyclerView) {
super.onAttachedToRecyclerView(recyclerView);
}
}
edit: fragment code:
private RecyclerView recyclerView;
private SearchAdapter searchAdapter;
recyclerView = (RecyclerView) v.findViewById(R.id.recycler_view);
searchAdapter = new SearchAdapter(categorizedArray);
recyclerView.setItemAnimator(new DefaultItemAnimator());
recyclerView.setAdapter(searchAdapter);

|
show 1 more comment
I just made a recycler view and it was not working, so I put a breakpoint on getItemCount and the method is not being called. I have never seen anyone else on SO have that particular issue, I am sure it is something ridiculously obvious. Here is my code.
public class SearchAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
private ArrayList<Object> displayList;
public static class ViewHolder extends RecyclerView.ViewHolder {
public ViewHolder(View v) {
super(v);
}
}
public class ErrorSearchItem extends ViewHolder {
//this is here if there is no other viewholder
public ErrorSearchItem(View view) {
super(view);
}
}
public class HeaderViewHolder extends ViewHolder {
public HeaderViewHolder(View view) {
super(view);
}
}
@Override
public int getItemViewType(int position) {
return position;
}
public SearchAdapter(ArrayList<Object> displayList) {
this.displayList = displayList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
if (displayList.get(viewType) instanceof String){
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.row_search_title, parent, false);
return new HeaderViewHolder(itemView);
}
else{ //this is for if there is an error and no other xml files match
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.row_error_search_item, parent, false);
return new ErrorSearchItem(itemView);
}
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
}
@Override
public int getItemCount() {
return displayList.size();
}
@Override
public void onAttachedToRecyclerView(RecyclerView recyclerView) {
super.onAttachedToRecyclerView(recyclerView);
}
}
edit: fragment code:
private RecyclerView recyclerView;
private SearchAdapter searchAdapter;
recyclerView = (RecyclerView) v.findViewById(R.id.recycler_view);
searchAdapter = new SearchAdapter(categorizedArray);
recyclerView.setItemAnimator(new DefaultItemAnimator());
recyclerView.setAdapter(searchAdapter);

Could you post your recycler view initialize code?
– Tomasz Czura
Jan 7 '17 at 14:37
sure just added it, tbh i actually never considered the issue could be there
– Adam Katz
Jan 7 '17 at 14:39
10
Did you set anyLayout
for your recyclerView? E.g.recyclerView.setLayoutManager(layout)
– Jiyeh
Jan 7 '17 at 14:46
2
Added a linear layout manager .
– khetanrajesh
Jan 7 '17 at 14:47
2
thanks that was it, works now, and i feel stupid :p
– Adam Katz
Jan 7 '17 at 15:27
|
show 1 more comment
I just made a recycler view and it was not working, so I put a breakpoint on getItemCount and the method is not being called. I have never seen anyone else on SO have that particular issue, I am sure it is something ridiculously obvious. Here is my code.
public class SearchAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
private ArrayList<Object> displayList;
public static class ViewHolder extends RecyclerView.ViewHolder {
public ViewHolder(View v) {
super(v);
}
}
public class ErrorSearchItem extends ViewHolder {
//this is here if there is no other viewholder
public ErrorSearchItem(View view) {
super(view);
}
}
public class HeaderViewHolder extends ViewHolder {
public HeaderViewHolder(View view) {
super(view);
}
}
@Override
public int getItemViewType(int position) {
return position;
}
public SearchAdapter(ArrayList<Object> displayList) {
this.displayList = displayList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
if (displayList.get(viewType) instanceof String){
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.row_search_title, parent, false);
return new HeaderViewHolder(itemView);
}
else{ //this is for if there is an error and no other xml files match
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.row_error_search_item, parent, false);
return new ErrorSearchItem(itemView);
}
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
}
@Override
public int getItemCount() {
return displayList.size();
}
@Override
public void onAttachedToRecyclerView(RecyclerView recyclerView) {
super.onAttachedToRecyclerView(recyclerView);
}
}
edit: fragment code:
private RecyclerView recyclerView;
private SearchAdapter searchAdapter;
recyclerView = (RecyclerView) v.findViewById(R.id.recycler_view);
searchAdapter = new SearchAdapter(categorizedArray);
recyclerView.setItemAnimator(new DefaultItemAnimator());
recyclerView.setAdapter(searchAdapter);

I just made a recycler view and it was not working, so I put a breakpoint on getItemCount and the method is not being called. I have never seen anyone else on SO have that particular issue, I am sure it is something ridiculously obvious. Here is my code.
public class SearchAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
private ArrayList<Object> displayList;
public static class ViewHolder extends RecyclerView.ViewHolder {
public ViewHolder(View v) {
super(v);
}
}
public class ErrorSearchItem extends ViewHolder {
//this is here if there is no other viewholder
public ErrorSearchItem(View view) {
super(view);
}
}
public class HeaderViewHolder extends ViewHolder {
public HeaderViewHolder(View view) {
super(view);
}
}
@Override
public int getItemViewType(int position) {
return position;
}
public SearchAdapter(ArrayList<Object> displayList) {
this.displayList = displayList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
if (displayList.get(viewType) instanceof String){
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.row_search_title, parent, false);
return new HeaderViewHolder(itemView);
}
else{ //this is for if there is an error and no other xml files match
View itemView = LayoutInflater.from(parent.getContext())
.inflate(R.layout.row_error_search_item, parent, false);
return new ErrorSearchItem(itemView);
}
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
}
@Override
public int getItemCount() {
return displayList.size();
}
@Override
public void onAttachedToRecyclerView(RecyclerView recyclerView) {
super.onAttachedToRecyclerView(recyclerView);
}
}
edit: fragment code:
private RecyclerView recyclerView;
private SearchAdapter searchAdapter;
recyclerView = (RecyclerView) v.findViewById(R.id.recycler_view);
searchAdapter = new SearchAdapter(categorizedArray);
recyclerView.setItemAnimator(new DefaultItemAnimator());
recyclerView.setAdapter(searchAdapter);


edited Jan 7 '17 at 14:39
Adam Katz
asked Jan 7 '17 at 14:36
Adam KatzAdam Katz
1,72832648
1,72832648
Could you post your recycler view initialize code?
– Tomasz Czura
Jan 7 '17 at 14:37
sure just added it, tbh i actually never considered the issue could be there
– Adam Katz
Jan 7 '17 at 14:39
10
Did you set anyLayout
for your recyclerView? E.g.recyclerView.setLayoutManager(layout)
– Jiyeh
Jan 7 '17 at 14:46
2
Added a linear layout manager .
– khetanrajesh
Jan 7 '17 at 14:47
2
thanks that was it, works now, and i feel stupid :p
– Adam Katz
Jan 7 '17 at 15:27
|
show 1 more comment
Could you post your recycler view initialize code?
– Tomasz Czura
Jan 7 '17 at 14:37
sure just added it, tbh i actually never considered the issue could be there
– Adam Katz
Jan 7 '17 at 14:39
10
Did you set anyLayout
for your recyclerView? E.g.recyclerView.setLayoutManager(layout)
– Jiyeh
Jan 7 '17 at 14:46
2
Added a linear layout manager .
– khetanrajesh
Jan 7 '17 at 14:47
2
thanks that was it, works now, and i feel stupid :p
– Adam Katz
Jan 7 '17 at 15:27
Could you post your recycler view initialize code?
– Tomasz Czura
Jan 7 '17 at 14:37
Could you post your recycler view initialize code?
– Tomasz Czura
Jan 7 '17 at 14:37
sure just added it, tbh i actually never considered the issue could be there
– Adam Katz
Jan 7 '17 at 14:39
sure just added it, tbh i actually never considered the issue could be there
– Adam Katz
Jan 7 '17 at 14:39
10
10
Did you set any
Layout
for your recyclerView? E.g. recyclerView.setLayoutManager(layout)
– Jiyeh
Jan 7 '17 at 14:46
Did you set any
Layout
for your recyclerView? E.g. recyclerView.setLayoutManager(layout)
– Jiyeh
Jan 7 '17 at 14:46
2
2
Added a linear layout manager .
– khetanrajesh
Jan 7 '17 at 14:47
Added a linear layout manager .
– khetanrajesh
Jan 7 '17 at 14:47
2
2
thanks that was it, works now, and i feel stupid :p
– Adam Katz
Jan 7 '17 at 15:27
thanks that was it, works now, and i feel stupid :p
– Adam Katz
Jan 7 '17 at 15:27
|
show 1 more comment
2 Answers
2
active
oldest
votes
I know It's completely nonsense but for me the problem solved when I changed the RecylerView layout_width and layout_height property from match_parent to wrap_content!!.
The trick saved my time. Cannot understand why this mandatory wrap content is not explicited
– LearningPath
Sep 17 '17 at 9:27
add a comment |
add recycler.setLayoutManager(new LinearLayoutManager(this));
before applying adapter
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f41522567%2frecycler-view-not-calling-getitemcount%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I know It's completely nonsense but for me the problem solved when I changed the RecylerView layout_width and layout_height property from match_parent to wrap_content!!.
The trick saved my time. Cannot understand why this mandatory wrap content is not explicited
– LearningPath
Sep 17 '17 at 9:27
add a comment |
I know It's completely nonsense but for me the problem solved when I changed the RecylerView layout_width and layout_height property from match_parent to wrap_content!!.
The trick saved my time. Cannot understand why this mandatory wrap content is not explicited
– LearningPath
Sep 17 '17 at 9:27
add a comment |
I know It's completely nonsense but for me the problem solved when I changed the RecylerView layout_width and layout_height property from match_parent to wrap_content!!.
I know It's completely nonsense but for me the problem solved when I changed the RecylerView layout_width and layout_height property from match_parent to wrap_content!!.
answered Sep 13 '17 at 13:58


Vahid GhadiriVahid Ghadiri
2,23142937
2,23142937
The trick saved my time. Cannot understand why this mandatory wrap content is not explicited
– LearningPath
Sep 17 '17 at 9:27
add a comment |
The trick saved my time. Cannot understand why this mandatory wrap content is not explicited
– LearningPath
Sep 17 '17 at 9:27
The trick saved my time. Cannot understand why this mandatory wrap content is not explicited
– LearningPath
Sep 17 '17 at 9:27
The trick saved my time. Cannot understand why this mandatory wrap content is not explicited
– LearningPath
Sep 17 '17 at 9:27
add a comment |
add recycler.setLayoutManager(new LinearLayoutManager(this));
before applying adapter
add a comment |
add recycler.setLayoutManager(new LinearLayoutManager(this));
before applying adapter
add a comment |
add recycler.setLayoutManager(new LinearLayoutManager(this));
before applying adapter
add recycler.setLayoutManager(new LinearLayoutManager(this));
before applying adapter
answered Dec 31 '18 at 8:25


Mohammed mansoorMohammed mansoor
661615
661615
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f41522567%2frecycler-view-not-calling-getitemcount%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jd0c,7ImGicBZ4kMRDpiS7aJNcy,7TONSX2omlD1Bn0SP7,I65ATSRT YFI6VsfthZ
Could you post your recycler view initialize code?
– Tomasz Czura
Jan 7 '17 at 14:37
sure just added it, tbh i actually never considered the issue could be there
– Adam Katz
Jan 7 '17 at 14:39
10
Did you set any
Layout
for your recyclerView? E.g.recyclerView.setLayoutManager(layout)
– Jiyeh
Jan 7 '17 at 14:46
2
Added a linear layout manager .
– khetanrajesh
Jan 7 '17 at 14:47
2
thanks that was it, works now, and i feel stupid :p
– Adam Katz
Jan 7 '17 at 15:27