Symfony 3.4 - Sending out an email (Call to a member function get() on null (500 Internal Server Error))
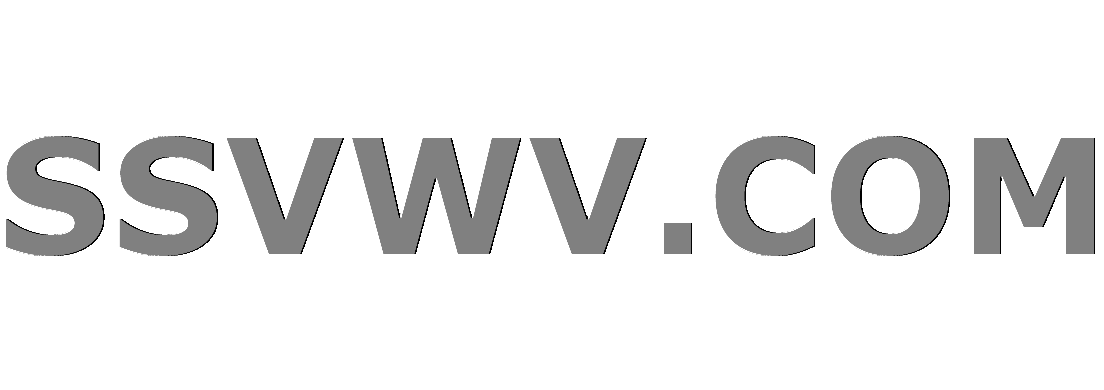
Multi tool use
I am working on the last part of my reset password system. All I need is for Symfony to send the URL that contain the token by email. When I execute the controller I get the following message:
Call to a member function get() on null (500 Internal Server Error)
If I remouve the "extends Controller" i get this error
What I don't get is that I copied the code from an other controller in the same project that sends email with out any problems.
My controller
<?php
namespace AppBundleEvent;
use CoopTilleulsForgotPasswordBundleEventForgotPasswordEvent;
use AppBundleAppBundle;
use AppBundleEntityUser;
use AppBundleFormImportForm;
use AppBundleFormUserRegistrationForm;
use AppBundleFormUserEditForm;
use SensioBundleFrameworkExtraBundleConfigurationRoute;
use SensioBundleFrameworkExtraBundleConfigurationSecurity;
use SymfonyBundleFrameworkBundleControllerController;
use SymfonyComponentHttpFoundationFileFile;
use SymfonyComponentHttpFoundationRequest;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentHttpFoundationFileUploadedFile;
use SymfonyComponentHttpFoundationStreamedResponse;
class ForgotPasswordEventListener extends Controller
{
private $templating;
private $manager;
public function __construct($templating, DoctrineORMEntityManager $manager)
{
$this->templating = $templating;
$this->manager=$manager;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$passwordToken = $event->getPasswordToken();
$user = $passwordToken->getUser();
$message = $this->templating->render(
'_Emails/change-password.html.twig',
[
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
]
);
$this->get('app.mailer')->send([
'from' => 'noreply@quebecenreseau.ca',
'to' => $user->getEmail(),
'subject' => 'Intranet Québec en réseau : Mot de passe oublier',
'body' => $message
]);
}
public function onUpdatePassword(ForgotPasswordEvent $event)
{
$passwordToken = $event->getPasswordToken();
$user = $passwordToken->getUser();
$user->setPlainPassword($event->getPassword());
$this->manager->persist($user);
}
}
My service file:
# Learn more about services, parameters and containers at
# http://symfony.com/doc/current/book/service_container.html
parameters:
# parameter_name: value
services:
app.security.login_form_authenticator:
class: AppBundleSecurityLoginFormAuthenticator
autowire: true
app.doctrine.hash_password_listener:
class: AppBundleDoctrineHashPasswordListener
autowire: true
tags:
- { name: doctrine.event_subscriber }
app.locale_listener:
class: AppBundleEventListenerLocaleListener
arguments: ['%kernel.default_locale%','@security.authorization_checker']
tags:
- { name: kernel.event_subscriber }
app.login_listener:
class: AppBundleEventListenerLoginListener
arguments: ['@session']
tags:
- { name: kernel.event_listener, event: security.interactive_login, method: onInteractiveLogin }
app.mailer:
class: AppBundleServiceMailer
arguments: ['@mailer','@templating']
app.utility:
class: AppBundleServiceUtility
arguments: ['@doctrine.orm.default_entity_manager']
app.filemanager:
class: AppBundleServiceFileManager
app.twig_extension:
class: AppBundleTwigAppExtension
arguments: ['@translator.default','@request_stack']
public: false
tags:
- { name: twig.extension }
app.sae:
class: AppBundleServiceEntitySae
arguments: ['@doctrine.orm.default_entity_manager']
app.document:
class: AppBundleServiceEntityDocument
arguments: ['@doctrine.orm.default_entity_manager']
app.professional:
class: AppBundleServiceEntityProfessional
arguments: ['@doctrine.orm.default_entity_manager']
app.article:
class: AppBundleServiceEntityArticle
arguments: ['@doctrine.orm.default_entity_manager']
app.course:
class: AppBundleServiceEntityCourse
arguments: ['@doctrine.orm.default_entity_manager']
app.useraddon:
class: AppBundleServiceEntityUserAddon
arguments: ['@doctrine.orm.default_entity_manager']
app.jwt_token_authenticator:
class: AppBundleSecurityJwtAuthenticator
arguments: ['@doctrine.orm.entity_manager', '@lexik_jwt_authentication.encoder']
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
app.ResettingController:
class: AppBundleControllerResettingController
tags:
- { name: kernel.event_listener, event: app.ResettingController }
kernel.event_listener.json_request_transformer:
class: QandidateCommonSymfonyHttpKernelEventListenerJsonRequestTransformerListener
tags:
- { name: kernel.event_listener, event: kernel.request, method: onKernelRequest, priority: 100 }
And this is the controller i based my code on
<?php
namespace AppBundleController;
use AppBundleAppBundle;
use AppBundleEntityUser;
use AppBundleFormImportForm;
use AppBundleFormUserRegistrationForm;
use AppBundleFormUserEditForm;
use SensioBundleFrameworkExtraBundleConfigurationRoute;
use SensioBundleFrameworkExtraBundleConfigurationSecurity;
use SymfonyBundleFrameworkBundleControllerController;
use SymfonyComponentHttpFoundationFileFile;
use SymfonyComponentHttpFoundationRequest;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentHttpFoundationFileUploadedFile;
use SymfonyComponentHttpFoundationStreamedResponse;
class UserController extends Controller
{
/**
* @Route("/users", name="app-users-index")
*/
public function userListAction(Request $request)
{
$em = $this->getDoctrine()->getManager();
$users = $em->getRepository('AppBundleEntityUser')
->findAll();
$title = $this->get('translator')->trans('Users');
return $this->render('Users/index.html.twig', [
'title' => $title,
'users' => $users
]);
}
/**
* @Route("/users/add", name="app-users-add")
*/
public function userAddAction(Request $request)
{
$em = $this->getDoctrine()->getManager();
$user = $this->getUser();
$usergroup = $user->getUsergroup();
$users = $this->get('app.utility')->getUsersByUsergroupId($usergroup->getId());
if ($request->get('insert')) {
$creator = $user;
$password = $this->get('app.utility')->randomPassword();
$user = new User();
$user->setPlainPassword( $password );
$user->setUsergroup( $creator->getUsergroup() );
// user
$user->setShowpro( ($request->get('showpro')) ? $request->get('showpro') : 0 );
$user->setName( $request->get('name') );
$user->setTitreid( $request->get('titreid') );
$user->setExpertise( $request->get('expertise') );
$user->setNotes( $request->get('notes') );
$user->setSaeid( $request->get('sae') );
$user->setEmail( $request->get('email') );
$user->setPhone( $request->get('phone') );
$user->setExtension( $request->get('extension') );
$user->setFax( $request->get('fax') );
$user->setAddress( $request->get('address') );
$user->setCity( $request->get('city') );
$user->setZipcode( $request->get('zipcode') );
$user->setProvince( 'QC' );
$user->setCountry( 'CA' );
$user->setLocale( 'fr' );
$user->setLanguage( 'fr' );
$em->persist($user);
$em->flush();
// user addons
// create and associate tags
$tags = $request->get('tags');
$tagsToAssociate = ;
$i = 0;
if($tags)
{
foreach($tags as $tag)
{
$checkTag = $this->get('app.professional')->getTagById($tag);
if( ! $checkTag )
{
$tag = $this->get('app.professional')->addTagByName($tag);
}
$tagsToAssociate[$i] = $tag;
$i++;
}
$this->get('app.useraddon')->associateUserTags( $user->getId(), $tagsToAssociate );
}
$secteurs = $request->get('secteurs');
if($secteurs)
{
$this->get('app.useraddon')->associateUserSecteurs( $user->getId(), $secteurs );
}
$message = $this->renderView(
'_Emails/new-account.html.twig',
[
'name' => $user->getName(),
'creator' => $creator->getName(),
'group' => $creator->getUsergroup()->getName(),
'username' => $user->getEmail(),
'password' => $password
]
);
$this->get('app.mailer')->send([
'from' => 'noreply@quebecenreseau.ca',
'to' => $user->getEmail(),
'subject' => 'Intranet Québec en réseau : Nouveau compte',
'body' => $message
]);
$this->addFlash('success', $user->getEmail() . ' ' . $this->get('translator')->trans('have been added successfully'));
return $this->redirect($this->generateUrl('app-users-index'));
}
$title = $this->get('translator')->trans('Users › Add');
$user = array(
'id' => '',
'saeid' => '',
'showpro' => '',
'name' => '',
'titreid' => '',
'expertise' => '',
'notes' => '',
'sae' => '',
'email' => '',
'phone' => '',
'extension' => '',
'fax' => '',
'address' => '',
'city' => '',
'zipcode' => ''
);
$userAddon = array(
'tags' => '',
'secteurs' => ''
);
$categories = $this->get('app.professional')->getAllCategories();
$tags = $this->get('app.professional')->getAllTags();
$saes = $this->get('app.sae')->getAllSaes();
$titres = $this->get('app.professional')->getAllTitres();
$secteurs = $this->get('app.professional')->getAllSecteurs();
return $this->render('Users/show.html.twig', [
'title' => $title,
'user' => $user,
'user_addon' => $userAddon,
'categories' => $categories,
'tags' => $tags,
'saes' => $saes,
'titres' => $titres,
'secteurs' => $secteurs,
'users' => $users
]);
}
}
It uses renderView which I never got working but render did the trick and now the get-> part that does not seem to work. I extended the controller the same way and did not find anything in the service file that would suggest I need to do something else.
php symfony
add a comment |
I am working on the last part of my reset password system. All I need is for Symfony to send the URL that contain the token by email. When I execute the controller I get the following message:
Call to a member function get() on null (500 Internal Server Error)
If I remouve the "extends Controller" i get this error
What I don't get is that I copied the code from an other controller in the same project that sends email with out any problems.
My controller
<?php
namespace AppBundleEvent;
use CoopTilleulsForgotPasswordBundleEventForgotPasswordEvent;
use AppBundleAppBundle;
use AppBundleEntityUser;
use AppBundleFormImportForm;
use AppBundleFormUserRegistrationForm;
use AppBundleFormUserEditForm;
use SensioBundleFrameworkExtraBundleConfigurationRoute;
use SensioBundleFrameworkExtraBundleConfigurationSecurity;
use SymfonyBundleFrameworkBundleControllerController;
use SymfonyComponentHttpFoundationFileFile;
use SymfonyComponentHttpFoundationRequest;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentHttpFoundationFileUploadedFile;
use SymfonyComponentHttpFoundationStreamedResponse;
class ForgotPasswordEventListener extends Controller
{
private $templating;
private $manager;
public function __construct($templating, DoctrineORMEntityManager $manager)
{
$this->templating = $templating;
$this->manager=$manager;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$passwordToken = $event->getPasswordToken();
$user = $passwordToken->getUser();
$message = $this->templating->render(
'_Emails/change-password.html.twig',
[
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
]
);
$this->get('app.mailer')->send([
'from' => 'noreply@quebecenreseau.ca',
'to' => $user->getEmail(),
'subject' => 'Intranet Québec en réseau : Mot de passe oublier',
'body' => $message
]);
}
public function onUpdatePassword(ForgotPasswordEvent $event)
{
$passwordToken = $event->getPasswordToken();
$user = $passwordToken->getUser();
$user->setPlainPassword($event->getPassword());
$this->manager->persist($user);
}
}
My service file:
# Learn more about services, parameters and containers at
# http://symfony.com/doc/current/book/service_container.html
parameters:
# parameter_name: value
services:
app.security.login_form_authenticator:
class: AppBundleSecurityLoginFormAuthenticator
autowire: true
app.doctrine.hash_password_listener:
class: AppBundleDoctrineHashPasswordListener
autowire: true
tags:
- { name: doctrine.event_subscriber }
app.locale_listener:
class: AppBundleEventListenerLocaleListener
arguments: ['%kernel.default_locale%','@security.authorization_checker']
tags:
- { name: kernel.event_subscriber }
app.login_listener:
class: AppBundleEventListenerLoginListener
arguments: ['@session']
tags:
- { name: kernel.event_listener, event: security.interactive_login, method: onInteractiveLogin }
app.mailer:
class: AppBundleServiceMailer
arguments: ['@mailer','@templating']
app.utility:
class: AppBundleServiceUtility
arguments: ['@doctrine.orm.default_entity_manager']
app.filemanager:
class: AppBundleServiceFileManager
app.twig_extension:
class: AppBundleTwigAppExtension
arguments: ['@translator.default','@request_stack']
public: false
tags:
- { name: twig.extension }
app.sae:
class: AppBundleServiceEntitySae
arguments: ['@doctrine.orm.default_entity_manager']
app.document:
class: AppBundleServiceEntityDocument
arguments: ['@doctrine.orm.default_entity_manager']
app.professional:
class: AppBundleServiceEntityProfessional
arguments: ['@doctrine.orm.default_entity_manager']
app.article:
class: AppBundleServiceEntityArticle
arguments: ['@doctrine.orm.default_entity_manager']
app.course:
class: AppBundleServiceEntityCourse
arguments: ['@doctrine.orm.default_entity_manager']
app.useraddon:
class: AppBundleServiceEntityUserAddon
arguments: ['@doctrine.orm.default_entity_manager']
app.jwt_token_authenticator:
class: AppBundleSecurityJwtAuthenticator
arguments: ['@doctrine.orm.entity_manager', '@lexik_jwt_authentication.encoder']
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
app.ResettingController:
class: AppBundleControllerResettingController
tags:
- { name: kernel.event_listener, event: app.ResettingController }
kernel.event_listener.json_request_transformer:
class: QandidateCommonSymfonyHttpKernelEventListenerJsonRequestTransformerListener
tags:
- { name: kernel.event_listener, event: kernel.request, method: onKernelRequest, priority: 100 }
And this is the controller i based my code on
<?php
namespace AppBundleController;
use AppBundleAppBundle;
use AppBundleEntityUser;
use AppBundleFormImportForm;
use AppBundleFormUserRegistrationForm;
use AppBundleFormUserEditForm;
use SensioBundleFrameworkExtraBundleConfigurationRoute;
use SensioBundleFrameworkExtraBundleConfigurationSecurity;
use SymfonyBundleFrameworkBundleControllerController;
use SymfonyComponentHttpFoundationFileFile;
use SymfonyComponentHttpFoundationRequest;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentHttpFoundationFileUploadedFile;
use SymfonyComponentHttpFoundationStreamedResponse;
class UserController extends Controller
{
/**
* @Route("/users", name="app-users-index")
*/
public function userListAction(Request $request)
{
$em = $this->getDoctrine()->getManager();
$users = $em->getRepository('AppBundleEntityUser')
->findAll();
$title = $this->get('translator')->trans('Users');
return $this->render('Users/index.html.twig', [
'title' => $title,
'users' => $users
]);
}
/**
* @Route("/users/add", name="app-users-add")
*/
public function userAddAction(Request $request)
{
$em = $this->getDoctrine()->getManager();
$user = $this->getUser();
$usergroup = $user->getUsergroup();
$users = $this->get('app.utility')->getUsersByUsergroupId($usergroup->getId());
if ($request->get('insert')) {
$creator = $user;
$password = $this->get('app.utility')->randomPassword();
$user = new User();
$user->setPlainPassword( $password );
$user->setUsergroup( $creator->getUsergroup() );
// user
$user->setShowpro( ($request->get('showpro')) ? $request->get('showpro') : 0 );
$user->setName( $request->get('name') );
$user->setTitreid( $request->get('titreid') );
$user->setExpertise( $request->get('expertise') );
$user->setNotes( $request->get('notes') );
$user->setSaeid( $request->get('sae') );
$user->setEmail( $request->get('email') );
$user->setPhone( $request->get('phone') );
$user->setExtension( $request->get('extension') );
$user->setFax( $request->get('fax') );
$user->setAddress( $request->get('address') );
$user->setCity( $request->get('city') );
$user->setZipcode( $request->get('zipcode') );
$user->setProvince( 'QC' );
$user->setCountry( 'CA' );
$user->setLocale( 'fr' );
$user->setLanguage( 'fr' );
$em->persist($user);
$em->flush();
// user addons
// create and associate tags
$tags = $request->get('tags');
$tagsToAssociate = ;
$i = 0;
if($tags)
{
foreach($tags as $tag)
{
$checkTag = $this->get('app.professional')->getTagById($tag);
if( ! $checkTag )
{
$tag = $this->get('app.professional')->addTagByName($tag);
}
$tagsToAssociate[$i] = $tag;
$i++;
}
$this->get('app.useraddon')->associateUserTags( $user->getId(), $tagsToAssociate );
}
$secteurs = $request->get('secteurs');
if($secteurs)
{
$this->get('app.useraddon')->associateUserSecteurs( $user->getId(), $secteurs );
}
$message = $this->renderView(
'_Emails/new-account.html.twig',
[
'name' => $user->getName(),
'creator' => $creator->getName(),
'group' => $creator->getUsergroup()->getName(),
'username' => $user->getEmail(),
'password' => $password
]
);
$this->get('app.mailer')->send([
'from' => 'noreply@quebecenreseau.ca',
'to' => $user->getEmail(),
'subject' => 'Intranet Québec en réseau : Nouveau compte',
'body' => $message
]);
$this->addFlash('success', $user->getEmail() . ' ' . $this->get('translator')->trans('have been added successfully'));
return $this->redirect($this->generateUrl('app-users-index'));
}
$title = $this->get('translator')->trans('Users › Add');
$user = array(
'id' => '',
'saeid' => '',
'showpro' => '',
'name' => '',
'titreid' => '',
'expertise' => '',
'notes' => '',
'sae' => '',
'email' => '',
'phone' => '',
'extension' => '',
'fax' => '',
'address' => '',
'city' => '',
'zipcode' => ''
);
$userAddon = array(
'tags' => '',
'secteurs' => ''
);
$categories = $this->get('app.professional')->getAllCategories();
$tags = $this->get('app.professional')->getAllTags();
$saes = $this->get('app.sae')->getAllSaes();
$titres = $this->get('app.professional')->getAllTitres();
$secteurs = $this->get('app.professional')->getAllSecteurs();
return $this->render('Users/show.html.twig', [
'title' => $title,
'user' => $user,
'user_addon' => $userAddon,
'categories' => $categories,
'tags' => $tags,
'saes' => $saes,
'titres' => $titres,
'secteurs' => $secteurs,
'users' => $users
]);
}
}
It uses renderView which I never got working but render did the trick and now the get-> part that does not seem to work. I extended the controller the same way and did not find anything in the service file that would suggest I need to do something else.
php symfony
1
Do you need to inject the Service Container into the EventListener (as explained in this answer stackoverflow.com/a/15472533/2915099) and then use that$container
variable to get the object? Also I don't thinkForgotPasswordEventListener
needs to extend the Controller class
– Richie Hughes
Dec 28 '18 at 23:47
Can you identify the line that is causing the error? Looking at your controller I only see aget()
call here:$this->get('app.mailer')
but the error message doesn't make sense in this context since$this
would not benull
here.
– Darragh Enright
Dec 28 '18 at 23:56
Thats exacly where the error occurs. I added a picture in the question.
– Patrick Simard
Dec 29 '18 at 0:04
there only are$this->get
and$request->get
. usexdebug
instead of guessing. see wiki.php.net/rfc/incompat_ctx
– Martin Zeitler
Dec 29 '18 at 0:05
The only thing i see diffrent is that i use namespace AppBundleEvent; could it be related? As for xdebug, i can't run in dev envirement... I have an open question here stackoverflow.com/questions/53948241/…
– Patrick Simard
Dec 29 '18 at 0:19
add a comment |
I am working on the last part of my reset password system. All I need is for Symfony to send the URL that contain the token by email. When I execute the controller I get the following message:
Call to a member function get() on null (500 Internal Server Error)
If I remouve the "extends Controller" i get this error
What I don't get is that I copied the code from an other controller in the same project that sends email with out any problems.
My controller
<?php
namespace AppBundleEvent;
use CoopTilleulsForgotPasswordBundleEventForgotPasswordEvent;
use AppBundleAppBundle;
use AppBundleEntityUser;
use AppBundleFormImportForm;
use AppBundleFormUserRegistrationForm;
use AppBundleFormUserEditForm;
use SensioBundleFrameworkExtraBundleConfigurationRoute;
use SensioBundleFrameworkExtraBundleConfigurationSecurity;
use SymfonyBundleFrameworkBundleControllerController;
use SymfonyComponentHttpFoundationFileFile;
use SymfonyComponentHttpFoundationRequest;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentHttpFoundationFileUploadedFile;
use SymfonyComponentHttpFoundationStreamedResponse;
class ForgotPasswordEventListener extends Controller
{
private $templating;
private $manager;
public function __construct($templating, DoctrineORMEntityManager $manager)
{
$this->templating = $templating;
$this->manager=$manager;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$passwordToken = $event->getPasswordToken();
$user = $passwordToken->getUser();
$message = $this->templating->render(
'_Emails/change-password.html.twig',
[
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
]
);
$this->get('app.mailer')->send([
'from' => 'noreply@quebecenreseau.ca',
'to' => $user->getEmail(),
'subject' => 'Intranet Québec en réseau : Mot de passe oublier',
'body' => $message
]);
}
public function onUpdatePassword(ForgotPasswordEvent $event)
{
$passwordToken = $event->getPasswordToken();
$user = $passwordToken->getUser();
$user->setPlainPassword($event->getPassword());
$this->manager->persist($user);
}
}
My service file:
# Learn more about services, parameters and containers at
# http://symfony.com/doc/current/book/service_container.html
parameters:
# parameter_name: value
services:
app.security.login_form_authenticator:
class: AppBundleSecurityLoginFormAuthenticator
autowire: true
app.doctrine.hash_password_listener:
class: AppBundleDoctrineHashPasswordListener
autowire: true
tags:
- { name: doctrine.event_subscriber }
app.locale_listener:
class: AppBundleEventListenerLocaleListener
arguments: ['%kernel.default_locale%','@security.authorization_checker']
tags:
- { name: kernel.event_subscriber }
app.login_listener:
class: AppBundleEventListenerLoginListener
arguments: ['@session']
tags:
- { name: kernel.event_listener, event: security.interactive_login, method: onInteractiveLogin }
app.mailer:
class: AppBundleServiceMailer
arguments: ['@mailer','@templating']
app.utility:
class: AppBundleServiceUtility
arguments: ['@doctrine.orm.default_entity_manager']
app.filemanager:
class: AppBundleServiceFileManager
app.twig_extension:
class: AppBundleTwigAppExtension
arguments: ['@translator.default','@request_stack']
public: false
tags:
- { name: twig.extension }
app.sae:
class: AppBundleServiceEntitySae
arguments: ['@doctrine.orm.default_entity_manager']
app.document:
class: AppBundleServiceEntityDocument
arguments: ['@doctrine.orm.default_entity_manager']
app.professional:
class: AppBundleServiceEntityProfessional
arguments: ['@doctrine.orm.default_entity_manager']
app.article:
class: AppBundleServiceEntityArticle
arguments: ['@doctrine.orm.default_entity_manager']
app.course:
class: AppBundleServiceEntityCourse
arguments: ['@doctrine.orm.default_entity_manager']
app.useraddon:
class: AppBundleServiceEntityUserAddon
arguments: ['@doctrine.orm.default_entity_manager']
app.jwt_token_authenticator:
class: AppBundleSecurityJwtAuthenticator
arguments: ['@doctrine.orm.entity_manager', '@lexik_jwt_authentication.encoder']
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
app.ResettingController:
class: AppBundleControllerResettingController
tags:
- { name: kernel.event_listener, event: app.ResettingController }
kernel.event_listener.json_request_transformer:
class: QandidateCommonSymfonyHttpKernelEventListenerJsonRequestTransformerListener
tags:
- { name: kernel.event_listener, event: kernel.request, method: onKernelRequest, priority: 100 }
And this is the controller i based my code on
<?php
namespace AppBundleController;
use AppBundleAppBundle;
use AppBundleEntityUser;
use AppBundleFormImportForm;
use AppBundleFormUserRegistrationForm;
use AppBundleFormUserEditForm;
use SensioBundleFrameworkExtraBundleConfigurationRoute;
use SensioBundleFrameworkExtraBundleConfigurationSecurity;
use SymfonyBundleFrameworkBundleControllerController;
use SymfonyComponentHttpFoundationFileFile;
use SymfonyComponentHttpFoundationRequest;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentHttpFoundationFileUploadedFile;
use SymfonyComponentHttpFoundationStreamedResponse;
class UserController extends Controller
{
/**
* @Route("/users", name="app-users-index")
*/
public function userListAction(Request $request)
{
$em = $this->getDoctrine()->getManager();
$users = $em->getRepository('AppBundleEntityUser')
->findAll();
$title = $this->get('translator')->trans('Users');
return $this->render('Users/index.html.twig', [
'title' => $title,
'users' => $users
]);
}
/**
* @Route("/users/add", name="app-users-add")
*/
public function userAddAction(Request $request)
{
$em = $this->getDoctrine()->getManager();
$user = $this->getUser();
$usergroup = $user->getUsergroup();
$users = $this->get('app.utility')->getUsersByUsergroupId($usergroup->getId());
if ($request->get('insert')) {
$creator = $user;
$password = $this->get('app.utility')->randomPassword();
$user = new User();
$user->setPlainPassword( $password );
$user->setUsergroup( $creator->getUsergroup() );
// user
$user->setShowpro( ($request->get('showpro')) ? $request->get('showpro') : 0 );
$user->setName( $request->get('name') );
$user->setTitreid( $request->get('titreid') );
$user->setExpertise( $request->get('expertise') );
$user->setNotes( $request->get('notes') );
$user->setSaeid( $request->get('sae') );
$user->setEmail( $request->get('email') );
$user->setPhone( $request->get('phone') );
$user->setExtension( $request->get('extension') );
$user->setFax( $request->get('fax') );
$user->setAddress( $request->get('address') );
$user->setCity( $request->get('city') );
$user->setZipcode( $request->get('zipcode') );
$user->setProvince( 'QC' );
$user->setCountry( 'CA' );
$user->setLocale( 'fr' );
$user->setLanguage( 'fr' );
$em->persist($user);
$em->flush();
// user addons
// create and associate tags
$tags = $request->get('tags');
$tagsToAssociate = ;
$i = 0;
if($tags)
{
foreach($tags as $tag)
{
$checkTag = $this->get('app.professional')->getTagById($tag);
if( ! $checkTag )
{
$tag = $this->get('app.professional')->addTagByName($tag);
}
$tagsToAssociate[$i] = $tag;
$i++;
}
$this->get('app.useraddon')->associateUserTags( $user->getId(), $tagsToAssociate );
}
$secteurs = $request->get('secteurs');
if($secteurs)
{
$this->get('app.useraddon')->associateUserSecteurs( $user->getId(), $secteurs );
}
$message = $this->renderView(
'_Emails/new-account.html.twig',
[
'name' => $user->getName(),
'creator' => $creator->getName(),
'group' => $creator->getUsergroup()->getName(),
'username' => $user->getEmail(),
'password' => $password
]
);
$this->get('app.mailer')->send([
'from' => 'noreply@quebecenreseau.ca',
'to' => $user->getEmail(),
'subject' => 'Intranet Québec en réseau : Nouveau compte',
'body' => $message
]);
$this->addFlash('success', $user->getEmail() . ' ' . $this->get('translator')->trans('have been added successfully'));
return $this->redirect($this->generateUrl('app-users-index'));
}
$title = $this->get('translator')->trans('Users › Add');
$user = array(
'id' => '',
'saeid' => '',
'showpro' => '',
'name' => '',
'titreid' => '',
'expertise' => '',
'notes' => '',
'sae' => '',
'email' => '',
'phone' => '',
'extension' => '',
'fax' => '',
'address' => '',
'city' => '',
'zipcode' => ''
);
$userAddon = array(
'tags' => '',
'secteurs' => ''
);
$categories = $this->get('app.professional')->getAllCategories();
$tags = $this->get('app.professional')->getAllTags();
$saes = $this->get('app.sae')->getAllSaes();
$titres = $this->get('app.professional')->getAllTitres();
$secteurs = $this->get('app.professional')->getAllSecteurs();
return $this->render('Users/show.html.twig', [
'title' => $title,
'user' => $user,
'user_addon' => $userAddon,
'categories' => $categories,
'tags' => $tags,
'saes' => $saes,
'titres' => $titres,
'secteurs' => $secteurs,
'users' => $users
]);
}
}
It uses renderView which I never got working but render did the trick and now the get-> part that does not seem to work. I extended the controller the same way and did not find anything in the service file that would suggest I need to do something else.
php symfony
I am working on the last part of my reset password system. All I need is for Symfony to send the URL that contain the token by email. When I execute the controller I get the following message:
Call to a member function get() on null (500 Internal Server Error)
If I remouve the "extends Controller" i get this error
What I don't get is that I copied the code from an other controller in the same project that sends email with out any problems.
My controller
<?php
namespace AppBundleEvent;
use CoopTilleulsForgotPasswordBundleEventForgotPasswordEvent;
use AppBundleAppBundle;
use AppBundleEntityUser;
use AppBundleFormImportForm;
use AppBundleFormUserRegistrationForm;
use AppBundleFormUserEditForm;
use SensioBundleFrameworkExtraBundleConfigurationRoute;
use SensioBundleFrameworkExtraBundleConfigurationSecurity;
use SymfonyBundleFrameworkBundleControllerController;
use SymfonyComponentHttpFoundationFileFile;
use SymfonyComponentHttpFoundationRequest;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentHttpFoundationFileUploadedFile;
use SymfonyComponentHttpFoundationStreamedResponse;
class ForgotPasswordEventListener extends Controller
{
private $templating;
private $manager;
public function __construct($templating, DoctrineORMEntityManager $manager)
{
$this->templating = $templating;
$this->manager=$manager;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$passwordToken = $event->getPasswordToken();
$user = $passwordToken->getUser();
$message = $this->templating->render(
'_Emails/change-password.html.twig',
[
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
]
);
$this->get('app.mailer')->send([
'from' => 'noreply@quebecenreseau.ca',
'to' => $user->getEmail(),
'subject' => 'Intranet Québec en réseau : Mot de passe oublier',
'body' => $message
]);
}
public function onUpdatePassword(ForgotPasswordEvent $event)
{
$passwordToken = $event->getPasswordToken();
$user = $passwordToken->getUser();
$user->setPlainPassword($event->getPassword());
$this->manager->persist($user);
}
}
My service file:
# Learn more about services, parameters and containers at
# http://symfony.com/doc/current/book/service_container.html
parameters:
# parameter_name: value
services:
app.security.login_form_authenticator:
class: AppBundleSecurityLoginFormAuthenticator
autowire: true
app.doctrine.hash_password_listener:
class: AppBundleDoctrineHashPasswordListener
autowire: true
tags:
- { name: doctrine.event_subscriber }
app.locale_listener:
class: AppBundleEventListenerLocaleListener
arguments: ['%kernel.default_locale%','@security.authorization_checker']
tags:
- { name: kernel.event_subscriber }
app.login_listener:
class: AppBundleEventListenerLoginListener
arguments: ['@session']
tags:
- { name: kernel.event_listener, event: security.interactive_login, method: onInteractiveLogin }
app.mailer:
class: AppBundleServiceMailer
arguments: ['@mailer','@templating']
app.utility:
class: AppBundleServiceUtility
arguments: ['@doctrine.orm.default_entity_manager']
app.filemanager:
class: AppBundleServiceFileManager
app.twig_extension:
class: AppBundleTwigAppExtension
arguments: ['@translator.default','@request_stack']
public: false
tags:
- { name: twig.extension }
app.sae:
class: AppBundleServiceEntitySae
arguments: ['@doctrine.orm.default_entity_manager']
app.document:
class: AppBundleServiceEntityDocument
arguments: ['@doctrine.orm.default_entity_manager']
app.professional:
class: AppBundleServiceEntityProfessional
arguments: ['@doctrine.orm.default_entity_manager']
app.article:
class: AppBundleServiceEntityArticle
arguments: ['@doctrine.orm.default_entity_manager']
app.course:
class: AppBundleServiceEntityCourse
arguments: ['@doctrine.orm.default_entity_manager']
app.useraddon:
class: AppBundleServiceEntityUserAddon
arguments: ['@doctrine.orm.default_entity_manager']
app.jwt_token_authenticator:
class: AppBundleSecurityJwtAuthenticator
arguments: ['@doctrine.orm.entity_manager', '@lexik_jwt_authentication.encoder']
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
app.ResettingController:
class: AppBundleControllerResettingController
tags:
- { name: kernel.event_listener, event: app.ResettingController }
kernel.event_listener.json_request_transformer:
class: QandidateCommonSymfonyHttpKernelEventListenerJsonRequestTransformerListener
tags:
- { name: kernel.event_listener, event: kernel.request, method: onKernelRequest, priority: 100 }
And this is the controller i based my code on
<?php
namespace AppBundleController;
use AppBundleAppBundle;
use AppBundleEntityUser;
use AppBundleFormImportForm;
use AppBundleFormUserRegistrationForm;
use AppBundleFormUserEditForm;
use SensioBundleFrameworkExtraBundleConfigurationRoute;
use SensioBundleFrameworkExtraBundleConfigurationSecurity;
use SymfonyBundleFrameworkBundleControllerController;
use SymfonyComponentHttpFoundationFileFile;
use SymfonyComponentHttpFoundationRequest;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentHttpFoundationFileUploadedFile;
use SymfonyComponentHttpFoundationStreamedResponse;
class UserController extends Controller
{
/**
* @Route("/users", name="app-users-index")
*/
public function userListAction(Request $request)
{
$em = $this->getDoctrine()->getManager();
$users = $em->getRepository('AppBundleEntityUser')
->findAll();
$title = $this->get('translator')->trans('Users');
return $this->render('Users/index.html.twig', [
'title' => $title,
'users' => $users
]);
}
/**
* @Route("/users/add", name="app-users-add")
*/
public function userAddAction(Request $request)
{
$em = $this->getDoctrine()->getManager();
$user = $this->getUser();
$usergroup = $user->getUsergroup();
$users = $this->get('app.utility')->getUsersByUsergroupId($usergroup->getId());
if ($request->get('insert')) {
$creator = $user;
$password = $this->get('app.utility')->randomPassword();
$user = new User();
$user->setPlainPassword( $password );
$user->setUsergroup( $creator->getUsergroup() );
// user
$user->setShowpro( ($request->get('showpro')) ? $request->get('showpro') : 0 );
$user->setName( $request->get('name') );
$user->setTitreid( $request->get('titreid') );
$user->setExpertise( $request->get('expertise') );
$user->setNotes( $request->get('notes') );
$user->setSaeid( $request->get('sae') );
$user->setEmail( $request->get('email') );
$user->setPhone( $request->get('phone') );
$user->setExtension( $request->get('extension') );
$user->setFax( $request->get('fax') );
$user->setAddress( $request->get('address') );
$user->setCity( $request->get('city') );
$user->setZipcode( $request->get('zipcode') );
$user->setProvince( 'QC' );
$user->setCountry( 'CA' );
$user->setLocale( 'fr' );
$user->setLanguage( 'fr' );
$em->persist($user);
$em->flush();
// user addons
// create and associate tags
$tags = $request->get('tags');
$tagsToAssociate = ;
$i = 0;
if($tags)
{
foreach($tags as $tag)
{
$checkTag = $this->get('app.professional')->getTagById($tag);
if( ! $checkTag )
{
$tag = $this->get('app.professional')->addTagByName($tag);
}
$tagsToAssociate[$i] = $tag;
$i++;
}
$this->get('app.useraddon')->associateUserTags( $user->getId(), $tagsToAssociate );
}
$secteurs = $request->get('secteurs');
if($secteurs)
{
$this->get('app.useraddon')->associateUserSecteurs( $user->getId(), $secteurs );
}
$message = $this->renderView(
'_Emails/new-account.html.twig',
[
'name' => $user->getName(),
'creator' => $creator->getName(),
'group' => $creator->getUsergroup()->getName(),
'username' => $user->getEmail(),
'password' => $password
]
);
$this->get('app.mailer')->send([
'from' => 'noreply@quebecenreseau.ca',
'to' => $user->getEmail(),
'subject' => 'Intranet Québec en réseau : Nouveau compte',
'body' => $message
]);
$this->addFlash('success', $user->getEmail() . ' ' . $this->get('translator')->trans('have been added successfully'));
return $this->redirect($this->generateUrl('app-users-index'));
}
$title = $this->get('translator')->trans('Users › Add');
$user = array(
'id' => '',
'saeid' => '',
'showpro' => '',
'name' => '',
'titreid' => '',
'expertise' => '',
'notes' => '',
'sae' => '',
'email' => '',
'phone' => '',
'extension' => '',
'fax' => '',
'address' => '',
'city' => '',
'zipcode' => ''
);
$userAddon = array(
'tags' => '',
'secteurs' => ''
);
$categories = $this->get('app.professional')->getAllCategories();
$tags = $this->get('app.professional')->getAllTags();
$saes = $this->get('app.sae')->getAllSaes();
$titres = $this->get('app.professional')->getAllTitres();
$secteurs = $this->get('app.professional')->getAllSecteurs();
return $this->render('Users/show.html.twig', [
'title' => $title,
'user' => $user,
'user_addon' => $userAddon,
'categories' => $categories,
'tags' => $tags,
'saes' => $saes,
'titres' => $titres,
'secteurs' => $secteurs,
'users' => $users
]);
}
}
It uses renderView which I never got working but render did the trick and now the get-> part that does not seem to work. I extended the controller the same way and did not find anything in the service file that would suggest I need to do something else.
php symfony
php symfony
edited Dec 31 '18 at 7:44
marc_s
573k12811071254
573k12811071254
asked Dec 28 '18 at 23:27


Patrick SimardPatrick Simard
1,05911024
1,05911024
1
Do you need to inject the Service Container into the EventListener (as explained in this answer stackoverflow.com/a/15472533/2915099) and then use that$container
variable to get the object? Also I don't thinkForgotPasswordEventListener
needs to extend the Controller class
– Richie Hughes
Dec 28 '18 at 23:47
Can you identify the line that is causing the error? Looking at your controller I only see aget()
call here:$this->get('app.mailer')
but the error message doesn't make sense in this context since$this
would not benull
here.
– Darragh Enright
Dec 28 '18 at 23:56
Thats exacly where the error occurs. I added a picture in the question.
– Patrick Simard
Dec 29 '18 at 0:04
there only are$this->get
and$request->get
. usexdebug
instead of guessing. see wiki.php.net/rfc/incompat_ctx
– Martin Zeitler
Dec 29 '18 at 0:05
The only thing i see diffrent is that i use namespace AppBundleEvent; could it be related? As for xdebug, i can't run in dev envirement... I have an open question here stackoverflow.com/questions/53948241/…
– Patrick Simard
Dec 29 '18 at 0:19
add a comment |
1
Do you need to inject the Service Container into the EventListener (as explained in this answer stackoverflow.com/a/15472533/2915099) and then use that$container
variable to get the object? Also I don't thinkForgotPasswordEventListener
needs to extend the Controller class
– Richie Hughes
Dec 28 '18 at 23:47
Can you identify the line that is causing the error? Looking at your controller I only see aget()
call here:$this->get('app.mailer')
but the error message doesn't make sense in this context since$this
would not benull
here.
– Darragh Enright
Dec 28 '18 at 23:56
Thats exacly where the error occurs. I added a picture in the question.
– Patrick Simard
Dec 29 '18 at 0:04
there only are$this->get
and$request->get
. usexdebug
instead of guessing. see wiki.php.net/rfc/incompat_ctx
– Martin Zeitler
Dec 29 '18 at 0:05
The only thing i see diffrent is that i use namespace AppBundleEvent; could it be related? As for xdebug, i can't run in dev envirement... I have an open question here stackoverflow.com/questions/53948241/…
– Patrick Simard
Dec 29 '18 at 0:19
1
1
Do you need to inject the Service Container into the EventListener (as explained in this answer stackoverflow.com/a/15472533/2915099) and then use that
$container
variable to get the object? Also I don't think ForgotPasswordEventListener
needs to extend the Controller class– Richie Hughes
Dec 28 '18 at 23:47
Do you need to inject the Service Container into the EventListener (as explained in this answer stackoverflow.com/a/15472533/2915099) and then use that
$container
variable to get the object? Also I don't think ForgotPasswordEventListener
needs to extend the Controller class– Richie Hughes
Dec 28 '18 at 23:47
Can you identify the line that is causing the error? Looking at your controller I only see a
get()
call here: $this->get('app.mailer')
but the error message doesn't make sense in this context since $this
would not be null
here.– Darragh Enright
Dec 28 '18 at 23:56
Can you identify the line that is causing the error? Looking at your controller I only see a
get()
call here: $this->get('app.mailer')
but the error message doesn't make sense in this context since $this
would not be null
here.– Darragh Enright
Dec 28 '18 at 23:56
Thats exacly where the error occurs. I added a picture in the question.
– Patrick Simard
Dec 29 '18 at 0:04
Thats exacly where the error occurs. I added a picture in the question.
– Patrick Simard
Dec 29 '18 at 0:04
there only are
$this->get
and $request->get
. use xdebug
instead of guessing. see wiki.php.net/rfc/incompat_ctx– Martin Zeitler
Dec 29 '18 at 0:05
there only are
$this->get
and $request->get
. use xdebug
instead of guessing. see wiki.php.net/rfc/incompat_ctx– Martin Zeitler
Dec 29 '18 at 0:05
The only thing i see diffrent is that i use namespace AppBundleEvent; could it be related? As for xdebug, i can't run in dev envirement... I have an open question here stackoverflow.com/questions/53948241/…
– Patrick Simard
Dec 29 '18 at 0:19
The only thing i see diffrent is that i use namespace AppBundleEvent; could it be related? As for xdebug, i can't run in dev envirement... I have an open question here stackoverflow.com/questions/53948241/…
– Patrick Simard
Dec 29 '18 at 0:19
add a comment |
2 Answers
2
active
oldest
votes
You're trying to call the method get() of ContainerInterface.
To be able to use this method, add ContainerInterface as a part of your service dependencies. So, you can use this attributes to call the get() function
for example:
$this->container->get('app.mailer')
add a comment |
Ok so I played arround and readed a lot and never understood why get-> is not working in my controller ... but I managed to go arround it like so.
class ForgotPasswordEventListener
{
private $templating;
private $manager;
protected $mailer;
public function __construct($templating, DoctrineORMEntityManager $manager, Swift_Mailer $mailer)
{
$this->templating = $templating;
$this->manager=$manager;
$this->mailer=$mailer;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$message = (new Swift_Message('Intranet Québec en réseau : Mot de passe oublier'))
->setFrom('noreply@quebecenreseau.ca')
->setTo($user->getEmail())
->setBody(
$this->templating->render(
// templates/emails/registration.html.twig
'_Emails/change-password.html.twig',
array(
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
)
),
'text/html'
);
if (0 === $this->mailer->send($message)){
throw new RuntimeException('Unable to send email');
}else{
echo "done";
}
[...]
As for my service i did this:
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
- "@mailer"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
I wish i could of understood why but this will do for now. Hope this helps sombody else in the future!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965377%2fsymfony-3-4-sending-out-an-email-call-to-a-member-function-get-on-null-500%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You're trying to call the method get() of ContainerInterface.
To be able to use this method, add ContainerInterface as a part of your service dependencies. So, you can use this attributes to call the get() function
for example:
$this->container->get('app.mailer')
add a comment |
You're trying to call the method get() of ContainerInterface.
To be able to use this method, add ContainerInterface as a part of your service dependencies. So, you can use this attributes to call the get() function
for example:
$this->container->get('app.mailer')
add a comment |
You're trying to call the method get() of ContainerInterface.
To be able to use this method, add ContainerInterface as a part of your service dependencies. So, you can use this attributes to call the get() function
for example:
$this->container->get('app.mailer')
You're trying to call the method get() of ContainerInterface.
To be able to use this method, add ContainerInterface as a part of your service dependencies. So, you can use this attributes to call the get() function
for example:
$this->container->get('app.mailer')
answered Dec 29 '18 at 19:30


JessGabrielJessGabriel
1194
1194
add a comment |
add a comment |
Ok so I played arround and readed a lot and never understood why get-> is not working in my controller ... but I managed to go arround it like so.
class ForgotPasswordEventListener
{
private $templating;
private $manager;
protected $mailer;
public function __construct($templating, DoctrineORMEntityManager $manager, Swift_Mailer $mailer)
{
$this->templating = $templating;
$this->manager=$manager;
$this->mailer=$mailer;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$message = (new Swift_Message('Intranet Québec en réseau : Mot de passe oublier'))
->setFrom('noreply@quebecenreseau.ca')
->setTo($user->getEmail())
->setBody(
$this->templating->render(
// templates/emails/registration.html.twig
'_Emails/change-password.html.twig',
array(
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
)
),
'text/html'
);
if (0 === $this->mailer->send($message)){
throw new RuntimeException('Unable to send email');
}else{
echo "done";
}
[...]
As for my service i did this:
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
- "@mailer"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
I wish i could of understood why but this will do for now. Hope this helps sombody else in the future!
add a comment |
Ok so I played arround and readed a lot and never understood why get-> is not working in my controller ... but I managed to go arround it like so.
class ForgotPasswordEventListener
{
private $templating;
private $manager;
protected $mailer;
public function __construct($templating, DoctrineORMEntityManager $manager, Swift_Mailer $mailer)
{
$this->templating = $templating;
$this->manager=$manager;
$this->mailer=$mailer;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$message = (new Swift_Message('Intranet Québec en réseau : Mot de passe oublier'))
->setFrom('noreply@quebecenreseau.ca')
->setTo($user->getEmail())
->setBody(
$this->templating->render(
// templates/emails/registration.html.twig
'_Emails/change-password.html.twig',
array(
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
)
),
'text/html'
);
if (0 === $this->mailer->send($message)){
throw new RuntimeException('Unable to send email');
}else{
echo "done";
}
[...]
As for my service i did this:
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
- "@mailer"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
I wish i could of understood why but this will do for now. Hope this helps sombody else in the future!
add a comment |
Ok so I played arround and readed a lot and never understood why get-> is not working in my controller ... but I managed to go arround it like so.
class ForgotPasswordEventListener
{
private $templating;
private $manager;
protected $mailer;
public function __construct($templating, DoctrineORMEntityManager $manager, Swift_Mailer $mailer)
{
$this->templating = $templating;
$this->manager=$manager;
$this->mailer=$mailer;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$message = (new Swift_Message('Intranet Québec en réseau : Mot de passe oublier'))
->setFrom('noreply@quebecenreseau.ca')
->setTo($user->getEmail())
->setBody(
$this->templating->render(
// templates/emails/registration.html.twig
'_Emails/change-password.html.twig',
array(
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
)
),
'text/html'
);
if (0 === $this->mailer->send($message)){
throw new RuntimeException('Unable to send email');
}else{
echo "done";
}
[...]
As for my service i did this:
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
- "@mailer"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
I wish i could of understood why but this will do for now. Hope this helps sombody else in the future!
Ok so I played arround and readed a lot and never understood why get-> is not working in my controller ... but I managed to go arround it like so.
class ForgotPasswordEventListener
{
private $templating;
private $manager;
protected $mailer;
public function __construct($templating, DoctrineORMEntityManager $manager, Swift_Mailer $mailer)
{
$this->templating = $templating;
$this->manager=$manager;
$this->mailer=$mailer;
}
/**
* @param ForgotPasswordEvent $event
*/
public function onCreateToken(ForgotPasswordEvent $event)
{
$message = (new Swift_Message('Intranet Québec en réseau : Mot de passe oublier'))
->setFrom('noreply@quebecenreseau.ca')
->setTo($user->getEmail())
->setBody(
$this->templating->render(
// templates/emails/registration.html.twig
'_Emails/change-password.html.twig',
array(
'name' => $user->getName(),
'tokenLink' => "https://www.quebecenreseau.ca/intranet/password-change/".$user->getId()."/".$passwordToken->getToken()
)
),
'text/html'
);
if (0 === $this->mailer->send($message)){
throw new RuntimeException('Unable to send email');
}else{
echo "done";
}
[...]
As for my service i did this:
app.listener.forgot_password:
class: AppBundleEventForgotPasswordEventListener
arguments:
- "@templating"
- "@doctrine.orm.entity_manager"
- "@mailer"
tags:
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: templating.helper, alias: templating, event: coop_tilleuls_forgot_password.create_token, method: onCreateToken}
- { name: kernel.event_listener, event: coop_tilleuls_forgot_password.update_password, method: onUpdatePassword }
I wish i could of understood why but this will do for now. Hope this helps sombody else in the future!
answered Dec 29 '18 at 13:04


Patrick SimardPatrick Simard
1,05911024
1,05911024
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965377%2fsymfony-3-4-sending-out-an-email-call-to-a-member-function-get-on-null-500%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
A0u8,j,EIFVFfEk0,5vdoibWwsSY 61ueThRqi D,JqXq,prj
1
Do you need to inject the Service Container into the EventListener (as explained in this answer stackoverflow.com/a/15472533/2915099) and then use that
$container
variable to get the object? Also I don't thinkForgotPasswordEventListener
needs to extend the Controller class– Richie Hughes
Dec 28 '18 at 23:47
Can you identify the line that is causing the error? Looking at your controller I only see a
get()
call here:$this->get('app.mailer')
but the error message doesn't make sense in this context since$this
would not benull
here.– Darragh Enright
Dec 28 '18 at 23:56
Thats exacly where the error occurs. I added a picture in the question.
– Patrick Simard
Dec 29 '18 at 0:04
there only are
$this->get
and$request->get
. usexdebug
instead of guessing. see wiki.php.net/rfc/incompat_ctx– Martin Zeitler
Dec 29 '18 at 0:05
The only thing i see diffrent is that i use namespace AppBundleEvent; could it be related? As for xdebug, i can't run in dev envirement... I have an open question here stackoverflow.com/questions/53948241/…
– Patrick Simard
Dec 29 '18 at 0:19