Spring and AJAX Form - how to send an Object Field
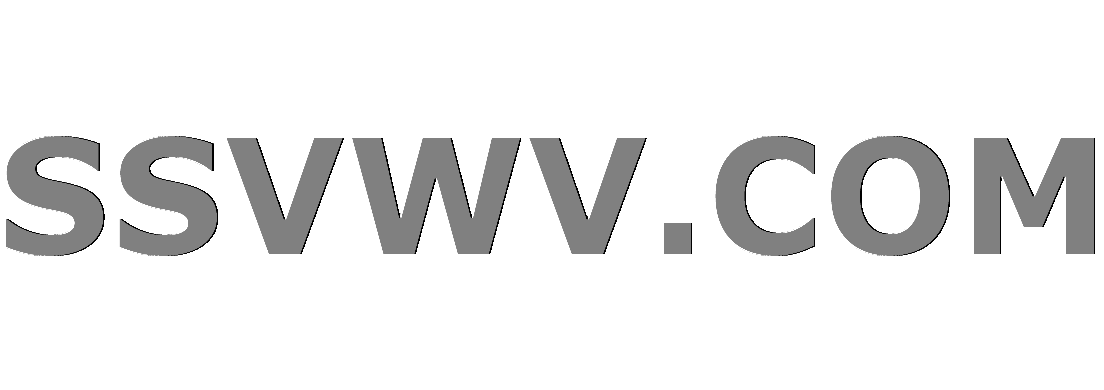
Multi tool use
I'm trying to send a DTO object via AJAX to my controller, but i don't know how to send an Object which is a field of my DTO because i can only send the id of the object...
My DTO is
public class ReservationDTO {
private Plate plate;
@Temporal(TemporalType.TIMESTAMP)
@DateTimeFormat(pattern="dd/MM/yyyy HH:mm")
@NotNull
private Date fromDate;
@Temporal(TemporalType.TIMESTAMP)
@DateTimeFormat(pattern="dd/MM/yyyy HH:mm")
@NotNull
private Date toDate;
private Park park;
//getters and setters
Then I have a form, with a select to choose the Plate
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group"> <label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
The script to send form data to the controller:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var reservationJson = $('#form-signin').serialize();
console.log(reservationJson);
$.ajax({
type: "POST",
url: "/book/getPrice",
data: reservationJson,
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
I would like to have this in my controller
public ResponseEntity<Integer> getReservationPrice(@RequestBody ReservationDTO dto){
// operations....
Submitting the form i get
plate.plateId=40&fromDate=26%2F04%2F2017+12%3A48&toDate=27%2F04%2F2017+12%3A49
which obviously isn't a ReservationDTO object
jquery ajax spring
add a comment |
I'm trying to send a DTO object via AJAX to my controller, but i don't know how to send an Object which is a field of my DTO because i can only send the id of the object...
My DTO is
public class ReservationDTO {
private Plate plate;
@Temporal(TemporalType.TIMESTAMP)
@DateTimeFormat(pattern="dd/MM/yyyy HH:mm")
@NotNull
private Date fromDate;
@Temporal(TemporalType.TIMESTAMP)
@DateTimeFormat(pattern="dd/MM/yyyy HH:mm")
@NotNull
private Date toDate;
private Park park;
//getters and setters
Then I have a form, with a select to choose the Plate
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group"> <label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
The script to send form data to the controller:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var reservationJson = $('#form-signin').serialize();
console.log(reservationJson);
$.ajax({
type: "POST",
url: "/book/getPrice",
data: reservationJson,
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
I would like to have this in my controller
public ResponseEntity<Integer> getReservationPrice(@RequestBody ReservationDTO dto){
// operations....
Submitting the form i get
plate.plateId=40&fromDate=26%2F04%2F2017+12%3A48&toDate=27%2F04%2F2017+12%3A49
which obviously isn't a ReservationDTO object
jquery ajax spring
add a comment |
I'm trying to send a DTO object via AJAX to my controller, but i don't know how to send an Object which is a field of my DTO because i can only send the id of the object...
My DTO is
public class ReservationDTO {
private Plate plate;
@Temporal(TemporalType.TIMESTAMP)
@DateTimeFormat(pattern="dd/MM/yyyy HH:mm")
@NotNull
private Date fromDate;
@Temporal(TemporalType.TIMESTAMP)
@DateTimeFormat(pattern="dd/MM/yyyy HH:mm")
@NotNull
private Date toDate;
private Park park;
//getters and setters
Then I have a form, with a select to choose the Plate
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group"> <label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
The script to send form data to the controller:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var reservationJson = $('#form-signin').serialize();
console.log(reservationJson);
$.ajax({
type: "POST",
url: "/book/getPrice",
data: reservationJson,
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
I would like to have this in my controller
public ResponseEntity<Integer> getReservationPrice(@RequestBody ReservationDTO dto){
// operations....
Submitting the form i get
plate.plateId=40&fromDate=26%2F04%2F2017+12%3A48&toDate=27%2F04%2F2017+12%3A49
which obviously isn't a ReservationDTO object
jquery ajax spring
I'm trying to send a DTO object via AJAX to my controller, but i don't know how to send an Object which is a field of my DTO because i can only send the id of the object...
My DTO is
public class ReservationDTO {
private Plate plate;
@Temporal(TemporalType.TIMESTAMP)
@DateTimeFormat(pattern="dd/MM/yyyy HH:mm")
@NotNull
private Date fromDate;
@Temporal(TemporalType.TIMESTAMP)
@DateTimeFormat(pattern="dd/MM/yyyy HH:mm")
@NotNull
private Date toDate;
private Park park;
//getters and setters
Then I have a form, with a select to choose the Plate
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group"> <label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
The script to send form data to the controller:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var reservationJson = $('#form-signin').serialize();
console.log(reservationJson);
$.ajax({
type: "POST",
url: "/book/getPrice",
data: reservationJson,
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
I would like to have this in my controller
public ResponseEntity<Integer> getReservationPrice(@RequestBody ReservationDTO dto){
// operations....
Submitting the form i get
plate.plateId=40&fromDate=26%2F04%2F2017+12%3A48&toDate=27%2F04%2F2017+12%3A49
which obviously isn't a ReservationDTO object
jquery ajax spring
jquery ajax spring
asked Apr 26 '17 at 10:50


besmartbesmart
65522455
65522455
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
I would do in the following way
HTML FORM:
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}" id="selectedPlate">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" id="fromDate" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" id="toDate" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
Then JS:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
It should be OK and create a correct JSON structure
Angelo
UPDATE
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate option:selected").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
Thanks Angelo, but there is something which is still wrong... because i'm getting all the plates in this way...{"fromDate":"26/04/2017 13:44","toDate":"27/04/2017 13:45","plate":{"id":"41","name":"ntttttttttt123456768899ntttttttttt123457ntttttttttt1234578910nttttttttttAAAAAntttttttttt123ntttttttttt111111111ntttttttttt"}
– besmart
Apr 26 '17 at 12:40
@besmart soorry i was wrong. You should use$("#selectedPlate option:selected").text();
in order to get the text of the selected plate. Let me know if it's OK. In the meantime I'll update the answer
– Angelo Immediata
Apr 26 '17 at 12:57
Yeh thanks, it's ok now!
– besmart
Apr 26 '17 at 12:59
You should put option:selected also on selectedPlate.id = $("#selectedPlate").val(); to be complete. thanks again
– besmart
Apr 26 '17 at 13:04
add a comment |
I found a much more simpler way to achieve this. Instead of passing the object as a JSON, you can simply use form.serializeArray()
as your data and expect a @ModelAttribute
in your controller. Something like this should do the trick.
jQuery
$.ajax({
url: "/book/getPrice",
data: $('#form-signin').serializeArray(),
type: "POST",
success: function() { ... }
});
Controller
@RequestMapping("/book/getPrice")
@ResponseBody
public ResponseEntity<Integer> getReservationPrice(@ModelAttribute ReservationDTO dto) {
// Operations.
}
Note: Remeber to add the @ResponseBody
annotation to your controller's method.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43632074%2fspring-and-ajax-form-how-to-send-an-object-field%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I would do in the following way
HTML FORM:
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}" id="selectedPlate">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" id="fromDate" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" id="toDate" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
Then JS:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
It should be OK and create a correct JSON structure
Angelo
UPDATE
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate option:selected").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
Thanks Angelo, but there is something which is still wrong... because i'm getting all the plates in this way...{"fromDate":"26/04/2017 13:44","toDate":"27/04/2017 13:45","plate":{"id":"41","name":"ntttttttttt123456768899ntttttttttt123457ntttttttttt1234578910nttttttttttAAAAAntttttttttt123ntttttttttt111111111ntttttttttt"}
– besmart
Apr 26 '17 at 12:40
@besmart soorry i was wrong. You should use$("#selectedPlate option:selected").text();
in order to get the text of the selected plate. Let me know if it's OK. In the meantime I'll update the answer
– Angelo Immediata
Apr 26 '17 at 12:57
Yeh thanks, it's ok now!
– besmart
Apr 26 '17 at 12:59
You should put option:selected also on selectedPlate.id = $("#selectedPlate").val(); to be complete. thanks again
– besmart
Apr 26 '17 at 13:04
add a comment |
I would do in the following way
HTML FORM:
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}" id="selectedPlate">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" id="fromDate" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" id="toDate" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
Then JS:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
It should be OK and create a correct JSON structure
Angelo
UPDATE
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate option:selected").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
Thanks Angelo, but there is something which is still wrong... because i'm getting all the plates in this way...{"fromDate":"26/04/2017 13:44","toDate":"27/04/2017 13:45","plate":{"id":"41","name":"ntttttttttt123456768899ntttttttttt123457ntttttttttt1234578910nttttttttttAAAAAntttttttttt123ntttttttttt111111111ntttttttttt"}
– besmart
Apr 26 '17 at 12:40
@besmart soorry i was wrong. You should use$("#selectedPlate option:selected").text();
in order to get the text of the selected plate. Let me know if it's OK. In the meantime I'll update the answer
– Angelo Immediata
Apr 26 '17 at 12:57
Yeh thanks, it's ok now!
– besmart
Apr 26 '17 at 12:59
You should put option:selected also on selectedPlate.id = $("#selectedPlate").val(); to be complete. thanks again
– besmart
Apr 26 '17 at 13:04
add a comment |
I would do in the following way
HTML FORM:
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}" id="selectedPlate">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" id="fromDate" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" id="toDate" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
Then JS:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
It should be OK and create a correct JSON structure
Angelo
UPDATE
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate option:selected").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
I would do in the following way
HTML FORM:
<form th:action="@{/book/new}" method="POST"
th:object="${reservation}"
id="form-signin">
<div class="form-group row">
<label class="col-sm-2 col-form-label" for="plate" th:text="#{reservation.plate }">Targa</label>
<div class="col-sm-4 input-group">
<select th:type="*{plate}" class="form-control" th:field="*{plate.plateId}" id="selectedPlate">
<option th:each="p : ${plates}" th:value="${p.plateId}" th:text="${p.plateNumber}">Opzione</option>
</select>
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="fromDate" th:text="#{reservation.from }"> </label>
<div class="col-sm-4 input-group" id="datetimepicker2">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{fromDate}" id="fromDate" />
</div>
</div>
<div class="row form-group">
<label class="col-sm-2 col-form-label" for="toDate" th:text="#{reservation.to }"> </label>
<div class="input-group col-sm-4" id="datetimepicker3">
<div class="input-group-addon">
<i class="fa fa-calendar"> </i>
</div>
<input type="text" th:field="*{toDate}" id="toDate" />
</div>
</div>
<div class="row col-sm-4">
<button type="submit" class="btn btn-success" th:text="#{reservation.getPrice}" id="priceButton">Conferma</button>
</div>
</form>
Then JS:
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
It should be OK and create a correct JSON structure
Angelo
UPDATE
$(function() {
$('#form-signin').submit(function(event) {
event.preventDefault();
var dati = new Object();
dati.fromDate = $("#fromDate").val();
dati.toDate = $("#toDate").val();
var selectedPlate = new Object();
selectedPlate.id = $("#selectedPlate").val();
selectedPlate.name = $("#selectedPlate option:selected").text();
dati.plate = selectedPlate;
dati.park = null;
$.ajax({
type: "POST",
url: "/book/getPrice",
data: JSON.stringify(dati),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data){
console.log(data);
}
});
return false;
});
});
edited Apr 26 '17 at 13:00
answered Apr 26 '17 at 11:24


Angelo ImmediataAngelo Immediata
4,14841637
4,14841637
Thanks Angelo, but there is something which is still wrong... because i'm getting all the plates in this way...{"fromDate":"26/04/2017 13:44","toDate":"27/04/2017 13:45","plate":{"id":"41","name":"ntttttttttt123456768899ntttttttttt123457ntttttttttt1234578910nttttttttttAAAAAntttttttttt123ntttttttttt111111111ntttttttttt"}
– besmart
Apr 26 '17 at 12:40
@besmart soorry i was wrong. You should use$("#selectedPlate option:selected").text();
in order to get the text of the selected plate. Let me know if it's OK. In the meantime I'll update the answer
– Angelo Immediata
Apr 26 '17 at 12:57
Yeh thanks, it's ok now!
– besmart
Apr 26 '17 at 12:59
You should put option:selected also on selectedPlate.id = $("#selectedPlate").val(); to be complete. thanks again
– besmart
Apr 26 '17 at 13:04
add a comment |
Thanks Angelo, but there is something which is still wrong... because i'm getting all the plates in this way...{"fromDate":"26/04/2017 13:44","toDate":"27/04/2017 13:45","plate":{"id":"41","name":"ntttttttttt123456768899ntttttttttt123457ntttttttttt1234578910nttttttttttAAAAAntttttttttt123ntttttttttt111111111ntttttttttt"}
– besmart
Apr 26 '17 at 12:40
@besmart soorry i was wrong. You should use$("#selectedPlate option:selected").text();
in order to get the text of the selected plate. Let me know if it's OK. In the meantime I'll update the answer
– Angelo Immediata
Apr 26 '17 at 12:57
Yeh thanks, it's ok now!
– besmart
Apr 26 '17 at 12:59
You should put option:selected also on selectedPlate.id = $("#selectedPlate").val(); to be complete. thanks again
– besmart
Apr 26 '17 at 13:04
Thanks Angelo, but there is something which is still wrong... because i'm getting all the plates in this way...
{"fromDate":"26/04/2017 13:44","toDate":"27/04/2017 13:45","plate":{"id":"41","name":"ntttttttttt123456768899ntttttttttt123457ntttttttttt1234578910nttttttttttAAAAAntttttttttt123ntttttttttt111111111ntttttttttt"}
– besmart
Apr 26 '17 at 12:40
Thanks Angelo, but there is something which is still wrong... because i'm getting all the plates in this way...
{"fromDate":"26/04/2017 13:44","toDate":"27/04/2017 13:45","plate":{"id":"41","name":"ntttttttttt123456768899ntttttttttt123457ntttttttttt1234578910nttttttttttAAAAAntttttttttt123ntttttttttt111111111ntttttttttt"}
– besmart
Apr 26 '17 at 12:40
@besmart soorry i was wrong. You should use
$("#selectedPlate option:selected").text();
in order to get the text of the selected plate. Let me know if it's OK. In the meantime I'll update the answer– Angelo Immediata
Apr 26 '17 at 12:57
@besmart soorry i was wrong. You should use
$("#selectedPlate option:selected").text();
in order to get the text of the selected plate. Let me know if it's OK. In the meantime I'll update the answer– Angelo Immediata
Apr 26 '17 at 12:57
Yeh thanks, it's ok now!
– besmart
Apr 26 '17 at 12:59
Yeh thanks, it's ok now!
– besmart
Apr 26 '17 at 12:59
You should put option:selected also on selectedPlate.id = $("#selectedPlate").val(); to be complete. thanks again
– besmart
Apr 26 '17 at 13:04
You should put option:selected also on selectedPlate.id = $("#selectedPlate").val(); to be complete. thanks again
– besmart
Apr 26 '17 at 13:04
add a comment |
I found a much more simpler way to achieve this. Instead of passing the object as a JSON, you can simply use form.serializeArray()
as your data and expect a @ModelAttribute
in your controller. Something like this should do the trick.
jQuery
$.ajax({
url: "/book/getPrice",
data: $('#form-signin').serializeArray(),
type: "POST",
success: function() { ... }
});
Controller
@RequestMapping("/book/getPrice")
@ResponseBody
public ResponseEntity<Integer> getReservationPrice(@ModelAttribute ReservationDTO dto) {
// Operations.
}
Note: Remeber to add the @ResponseBody
annotation to your controller's method.
add a comment |
I found a much more simpler way to achieve this. Instead of passing the object as a JSON, you can simply use form.serializeArray()
as your data and expect a @ModelAttribute
in your controller. Something like this should do the trick.
jQuery
$.ajax({
url: "/book/getPrice",
data: $('#form-signin').serializeArray(),
type: "POST",
success: function() { ... }
});
Controller
@RequestMapping("/book/getPrice")
@ResponseBody
public ResponseEntity<Integer> getReservationPrice(@ModelAttribute ReservationDTO dto) {
// Operations.
}
Note: Remeber to add the @ResponseBody
annotation to your controller's method.
add a comment |
I found a much more simpler way to achieve this. Instead of passing the object as a JSON, you can simply use form.serializeArray()
as your data and expect a @ModelAttribute
in your controller. Something like this should do the trick.
jQuery
$.ajax({
url: "/book/getPrice",
data: $('#form-signin').serializeArray(),
type: "POST",
success: function() { ... }
});
Controller
@RequestMapping("/book/getPrice")
@ResponseBody
public ResponseEntity<Integer> getReservationPrice(@ModelAttribute ReservationDTO dto) {
// Operations.
}
Note: Remeber to add the @ResponseBody
annotation to your controller's method.
I found a much more simpler way to achieve this. Instead of passing the object as a JSON, you can simply use form.serializeArray()
as your data and expect a @ModelAttribute
in your controller. Something like this should do the trick.
jQuery
$.ajax({
url: "/book/getPrice",
data: $('#form-signin').serializeArray(),
type: "POST",
success: function() { ... }
});
Controller
@RequestMapping("/book/getPrice")
@ResponseBody
public ResponseEntity<Integer> getReservationPrice(@ModelAttribute ReservationDTO dto) {
// Operations.
}
Note: Remeber to add the @ResponseBody
annotation to your controller's method.
answered Dec 28 '18 at 23:34


Alain CruzAlain Cruz
1,7683818
1,7683818
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43632074%2fspring-and-ajax-form-how-to-send-an-object-field%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Yz,XYfNz4ltKJXY1,IGFrg