python dataframe how to convert set column to list
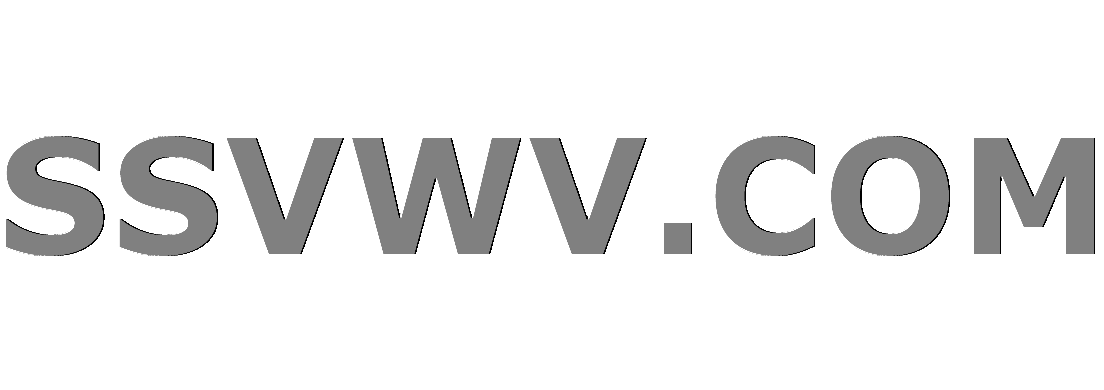
Multi tool use
I tried to convert a set column to list in python dataframe, but failed. Not sure what's best way to do so. Thanks.
Here is the example:
I tried to create a 'c' column which convert 'b' set column to list. but 'c' is still set.
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = list(tdf['b'])
tdf
a b c
0 [1, 2, 3] {33, 11, 22} {33, 11, 22}
1 [2, 3, 4] {222, 111} {222, 111}
python list dataframe set
add a comment |
I tried to convert a set column to list in python dataframe, but failed. Not sure what's best way to do so. Thanks.
Here is the example:
I tried to create a 'c' column which convert 'b' set column to list. but 'c' is still set.
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = list(tdf['b'])
tdf
a b c
0 [1, 2, 3] {33, 11, 22} {33, 11, 22}
1 [2, 3, 4] {222, 111} {222, 111}
python list dataframe set
1
This should never be a dataframe in the first place. You have non-scalar values in columns, you're making life harder for yourself. You will get no benefit of pandas at all through this structure.
– roganjosh
Dec 28 '18 at 23:51
Related meta: Generic “Don't Do It” Answer
– jpp
Dec 29 '18 at 0:46
add a comment |
I tried to convert a set column to list in python dataframe, but failed. Not sure what's best way to do so. Thanks.
Here is the example:
I tried to create a 'c' column which convert 'b' set column to list. but 'c' is still set.
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = list(tdf['b'])
tdf
a b c
0 [1, 2, 3] {33, 11, 22} {33, 11, 22}
1 [2, 3, 4] {222, 111} {222, 111}
python list dataframe set
I tried to convert a set column to list in python dataframe, but failed. Not sure what's best way to do so. Thanks.
Here is the example:
I tried to create a 'c' column which convert 'b' set column to list. but 'c' is still set.
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = list(tdf['b'])
tdf
a b c
0 [1, 2, 3] {33, 11, 22} {33, 11, 22}
1 [2, 3, 4] {222, 111} {222, 111}
python list dataframe set
python list dataframe set
asked Dec 28 '18 at 23:49
user1828513user1828513
10039
10039
1
This should never be a dataframe in the first place. You have non-scalar values in columns, you're making life harder for yourself. You will get no benefit of pandas at all through this structure.
– roganjosh
Dec 28 '18 at 23:51
Related meta: Generic “Don't Do It” Answer
– jpp
Dec 29 '18 at 0:46
add a comment |
1
This should never be a dataframe in the first place. You have non-scalar values in columns, you're making life harder for yourself. You will get no benefit of pandas at all through this structure.
– roganjosh
Dec 28 '18 at 23:51
Related meta: Generic “Don't Do It” Answer
– jpp
Dec 29 '18 at 0:46
1
1
This should never be a dataframe in the first place. You have non-scalar values in columns, you're making life harder for yourself. You will get no benefit of pandas at all through this structure.
– roganjosh
Dec 28 '18 at 23:51
This should never be a dataframe in the first place. You have non-scalar values in columns, you're making life harder for yourself. You will get no benefit of pandas at all through this structure.
– roganjosh
Dec 28 '18 at 23:51
Related meta: Generic “Don't Do It” Answer
– jpp
Dec 29 '18 at 0:46
Related meta: Generic “Don't Do It” Answer
– jpp
Dec 29 '18 at 0:46
add a comment |
2 Answers
2
active
oldest
votes
You could do:
import pandas as pd
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = [list(e) for e in tdf.b]
print(tdf)
1
@U9-Forward if you seemap
,apply
,lambda
,np.vectorize
(a complete misnomer)... it's running in Python time.
– roganjosh
Dec 28 '18 at 23:56
add a comment |
Use apply
:
tdf['c'] = tdf['b'].apply(list)
Because using list
is doing to whole column not one by one.
Or do:
tdf['c'] = tdf['b'].map(list)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965508%2fpython-dataframe-how-to-convert-set-column-to-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could do:
import pandas as pd
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = [list(e) for e in tdf.b]
print(tdf)
1
@U9-Forward if you seemap
,apply
,lambda
,np.vectorize
(a complete misnomer)... it's running in Python time.
– roganjosh
Dec 28 '18 at 23:56
add a comment |
You could do:
import pandas as pd
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = [list(e) for e in tdf.b]
print(tdf)
1
@U9-Forward if you seemap
,apply
,lambda
,np.vectorize
(a complete misnomer)... it's running in Python time.
– roganjosh
Dec 28 '18 at 23:56
add a comment |
You could do:
import pandas as pd
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = [list(e) for e in tdf.b]
print(tdf)
You could do:
import pandas as pd
data = [{'a': [1,2,3], 'b':{11,22,33}},{'a':[2,3,4],'b':{111,222}}]
tdf = pd.DataFrame(data)
tdf['c'] = [list(e) for e in tdf.b]
print(tdf)
answered Dec 28 '18 at 23:51


Daniel MesejoDaniel Mesejo
16k21130
16k21130
1
@U9-Forward if you seemap
,apply
,lambda
,np.vectorize
(a complete misnomer)... it's running in Python time.
– roganjosh
Dec 28 '18 at 23:56
add a comment |
1
@U9-Forward if you seemap
,apply
,lambda
,np.vectorize
(a complete misnomer)... it's running in Python time.
– roganjosh
Dec 28 '18 at 23:56
1
1
@U9-Forward if you see
map
, apply
, lambda
, np.vectorize
(a complete misnomer)... it's running in Python time.– roganjosh
Dec 28 '18 at 23:56
@U9-Forward if you see
map
, apply
, lambda
, np.vectorize
(a complete misnomer)... it's running in Python time.– roganjosh
Dec 28 '18 at 23:56
add a comment |
Use apply
:
tdf['c'] = tdf['b'].apply(list)
Because using list
is doing to whole column not one by one.
Or do:
tdf['c'] = tdf['b'].map(list)
add a comment |
Use apply
:
tdf['c'] = tdf['b'].apply(list)
Because using list
is doing to whole column not one by one.
Or do:
tdf['c'] = tdf['b'].map(list)
add a comment |
Use apply
:
tdf['c'] = tdf['b'].apply(list)
Because using list
is doing to whole column not one by one.
Or do:
tdf['c'] = tdf['b'].map(list)
Use apply
:
tdf['c'] = tdf['b'].apply(list)
Because using list
is doing to whole column not one by one.
Or do:
tdf['c'] = tdf['b'].map(list)
answered Dec 28 '18 at 23:51


U9-ForwardU9-Forward
14.1k21337
14.1k21337
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965508%2fpython-dataframe-how-to-convert-set-column-to-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S4rmkNweU
1
This should never be a dataframe in the first place. You have non-scalar values in columns, you're making life harder for yourself. You will get no benefit of pandas at all through this structure.
– roganjosh
Dec 28 '18 at 23:51
Related meta: Generic “Don't Do It” Answer
– jpp
Dec 29 '18 at 0:46