How to !rm python_var (in Jupyter notebooks)
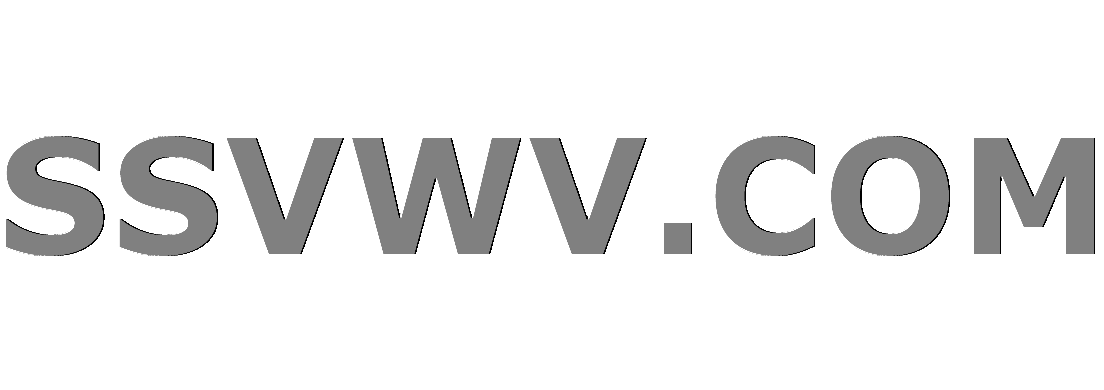
Multi tool use
I know I can do this:
CSV_Files = [file1.csv, file2.csv, etc...]
%rm file1.csv
!rm file2.csv
but how can I do this as a variable. eg.
TXT_Files = [ABC.txt, XYZ.txt, etc...]
for file in TXT_Files:
!rm file
python bash jupyter rm
add a comment |
I know I can do this:
CSV_Files = [file1.csv, file2.csv, etc...]
%rm file1.csv
!rm file2.csv
but how can I do this as a variable. eg.
TXT_Files = [ABC.txt, XYZ.txt, etc...]
for file in TXT_Files:
!rm file
python bash jupyter rm
Why do you need to use the shell?CSV_Files.map(os.remove)
– Barmar
Dec 28 '18 at 23:39
Just a newbie's peeve of not wanting to import os. Was wondering if I could do this in a loop. [os.remove(file) for file in Files] works great btw.
– Nate
Dec 29 '18 at 7:13
add a comment |
I know I can do this:
CSV_Files = [file1.csv, file2.csv, etc...]
%rm file1.csv
!rm file2.csv
but how can I do this as a variable. eg.
TXT_Files = [ABC.txt, XYZ.txt, etc...]
for file in TXT_Files:
!rm file
python bash jupyter rm
I know I can do this:
CSV_Files = [file1.csv, file2.csv, etc...]
%rm file1.csv
!rm file2.csv
but how can I do this as a variable. eg.
TXT_Files = [ABC.txt, XYZ.txt, etc...]
for file in TXT_Files:
!rm file
python bash jupyter rm
python bash jupyter rm
asked Dec 28 '18 at 23:35
NateNate
813
813
Why do you need to use the shell?CSV_Files.map(os.remove)
– Barmar
Dec 28 '18 at 23:39
Just a newbie's peeve of not wanting to import os. Was wondering if I could do this in a loop. [os.remove(file) for file in Files] works great btw.
– Nate
Dec 29 '18 at 7:13
add a comment |
Why do you need to use the shell?CSV_Files.map(os.remove)
– Barmar
Dec 28 '18 at 23:39
Just a newbie's peeve of not wanting to import os. Was wondering if I could do this in a loop. [os.remove(file) for file in Files] works great btw.
– Nate
Dec 29 '18 at 7:13
Why do you need to use the shell?
CSV_Files.map(os.remove)
– Barmar
Dec 28 '18 at 23:39
Why do you need to use the shell?
CSV_Files.map(os.remove)
– Barmar
Dec 28 '18 at 23:39
Just a newbie's peeve of not wanting to import os. Was wondering if I could do this in a loop. [os.remove(file) for file in Files] works great btw.
– Nate
Dec 29 '18 at 7:13
Just a newbie's peeve of not wanting to import os. Was wondering if I could do this in a loop. [os.remove(file) for file in Files] works great btw.
– Nate
Dec 29 '18 at 7:13
add a comment |
2 Answers
2
active
oldest
votes
rm
can remove several files per call:
In [80]: !touch a.t1 b.t1 c.t1
In [81]: !ls *.t1
a.t1 b.t1 c.t1
In [82]: !rm -r a.t1 b.t1 c.t1
In [83]: !ls *.t1
ls: cannot access '*.t1': No such file or directory
If the starting point is a list of file names:
In [116]: alist = ['a.t1', 'b.t1', 'c.t1']
In [117]: astr = ' '.join(alist) # make a string
In [118]: !echo $astr # variable substitution as in BASH
a.t1 b.t1 c.t1
In [119]: !touch $astr # make 3 files
In [120]: ls *.t1
a.t1 b.t1 c.t1
In [121]: !rm -r $astr # remove them
In [122]: ls *.t1
ls: cannot access '*.t1': No such file or directory
Working with Python's own OS functions is probably better, but you can do much of the same stuff with %magics - if you understand shell well enough.
To use 'magics' in a Python expression, I have to use the underlying functions, not the '!' or '%' syntax, e.g.
import IPython
for txt in ['a.t1','b.t1','c.t1']:
IPython.utils.process.getoutput('touch %s'%txt)
The getoutput
function is used by %sx
(which underlies !!
) which uses subprocess.Popen
. But if you go to all that work you might as well use the os
functions that Python itself provides.
The file names may need an added layer of quoting to ensure that the shell doesn't give a syntax error:
In [129]: alist = ['"a(1).t1"', '"b(2).t1"', 'c.t1']
In [130]: astr = ' '.join(alist)
In [131]: !touch $astr
In [132]: !ls *.t1
'a(1).t1' a.t1 'b(2).t1' b.t1 c.t1
that's great and all, but doesnt work in a loop. '/bin/sh: 1: Syntax error: "(" unexpected'
– Nate
Dec 29 '18 at 6:43
The magic syntax with '!' and '%' (and unquoted strings) isn't valid Python, so doesn't work within Python structures like loops. Those expressions have to be used at top level where the IPython REPL can catch and parse them.
– hpaulj
Dec 29 '18 at 7:35
owh wait, it's because I have parentheses in the str (in a list). eg.files = ['Hello(1).csv', 'Hello(2).csv']
– Nate
Dec 29 '18 at 7:43
otherwise it works flawlessly. Thanks! it's what i wanted
– Nate
Dec 29 '18 at 8:01
You may need an added layer of quotes, e.g.'"Hello(1).csv"'
.
– hpaulj
Dec 29 '18 at 8:17
|
show 1 more comment
You can handle this in Python without magic shell commands. I recommend using the pathlib
module, for a more modern approach. For what you're doing, it would be:
import pathlib
csv_files = pathlib.Path('/path/to/actual/files')
for csv_file in csv_files.glob('*.csv'):
csv_file.unlink()
Use the .glob()
method to filter only the files you want to use, and .unlink()
to delete them (which is similar to os.remove()
).
Avoid using file
as a variable, as it's a reserved word in the language.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965438%2fhow-to-rm-python-var-in-jupyter-notebooks%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
rm
can remove several files per call:
In [80]: !touch a.t1 b.t1 c.t1
In [81]: !ls *.t1
a.t1 b.t1 c.t1
In [82]: !rm -r a.t1 b.t1 c.t1
In [83]: !ls *.t1
ls: cannot access '*.t1': No such file or directory
If the starting point is a list of file names:
In [116]: alist = ['a.t1', 'b.t1', 'c.t1']
In [117]: astr = ' '.join(alist) # make a string
In [118]: !echo $astr # variable substitution as in BASH
a.t1 b.t1 c.t1
In [119]: !touch $astr # make 3 files
In [120]: ls *.t1
a.t1 b.t1 c.t1
In [121]: !rm -r $astr # remove them
In [122]: ls *.t1
ls: cannot access '*.t1': No such file or directory
Working with Python's own OS functions is probably better, but you can do much of the same stuff with %magics - if you understand shell well enough.
To use 'magics' in a Python expression, I have to use the underlying functions, not the '!' or '%' syntax, e.g.
import IPython
for txt in ['a.t1','b.t1','c.t1']:
IPython.utils.process.getoutput('touch %s'%txt)
The getoutput
function is used by %sx
(which underlies !!
) which uses subprocess.Popen
. But if you go to all that work you might as well use the os
functions that Python itself provides.
The file names may need an added layer of quoting to ensure that the shell doesn't give a syntax error:
In [129]: alist = ['"a(1).t1"', '"b(2).t1"', 'c.t1']
In [130]: astr = ' '.join(alist)
In [131]: !touch $astr
In [132]: !ls *.t1
'a(1).t1' a.t1 'b(2).t1' b.t1 c.t1
that's great and all, but doesnt work in a loop. '/bin/sh: 1: Syntax error: "(" unexpected'
– Nate
Dec 29 '18 at 6:43
The magic syntax with '!' and '%' (and unquoted strings) isn't valid Python, so doesn't work within Python structures like loops. Those expressions have to be used at top level where the IPython REPL can catch and parse them.
– hpaulj
Dec 29 '18 at 7:35
owh wait, it's because I have parentheses in the str (in a list). eg.files = ['Hello(1).csv', 'Hello(2).csv']
– Nate
Dec 29 '18 at 7:43
otherwise it works flawlessly. Thanks! it's what i wanted
– Nate
Dec 29 '18 at 8:01
You may need an added layer of quotes, e.g.'"Hello(1).csv"'
.
– hpaulj
Dec 29 '18 at 8:17
|
show 1 more comment
rm
can remove several files per call:
In [80]: !touch a.t1 b.t1 c.t1
In [81]: !ls *.t1
a.t1 b.t1 c.t1
In [82]: !rm -r a.t1 b.t1 c.t1
In [83]: !ls *.t1
ls: cannot access '*.t1': No such file or directory
If the starting point is a list of file names:
In [116]: alist = ['a.t1', 'b.t1', 'c.t1']
In [117]: astr = ' '.join(alist) # make a string
In [118]: !echo $astr # variable substitution as in BASH
a.t1 b.t1 c.t1
In [119]: !touch $astr # make 3 files
In [120]: ls *.t1
a.t1 b.t1 c.t1
In [121]: !rm -r $astr # remove them
In [122]: ls *.t1
ls: cannot access '*.t1': No such file or directory
Working with Python's own OS functions is probably better, but you can do much of the same stuff with %magics - if you understand shell well enough.
To use 'magics' in a Python expression, I have to use the underlying functions, not the '!' or '%' syntax, e.g.
import IPython
for txt in ['a.t1','b.t1','c.t1']:
IPython.utils.process.getoutput('touch %s'%txt)
The getoutput
function is used by %sx
(which underlies !!
) which uses subprocess.Popen
. But if you go to all that work you might as well use the os
functions that Python itself provides.
The file names may need an added layer of quoting to ensure that the shell doesn't give a syntax error:
In [129]: alist = ['"a(1).t1"', '"b(2).t1"', 'c.t1']
In [130]: astr = ' '.join(alist)
In [131]: !touch $astr
In [132]: !ls *.t1
'a(1).t1' a.t1 'b(2).t1' b.t1 c.t1
that's great and all, but doesnt work in a loop. '/bin/sh: 1: Syntax error: "(" unexpected'
– Nate
Dec 29 '18 at 6:43
The magic syntax with '!' and '%' (and unquoted strings) isn't valid Python, so doesn't work within Python structures like loops. Those expressions have to be used at top level where the IPython REPL can catch and parse them.
– hpaulj
Dec 29 '18 at 7:35
owh wait, it's because I have parentheses in the str (in a list). eg.files = ['Hello(1).csv', 'Hello(2).csv']
– Nate
Dec 29 '18 at 7:43
otherwise it works flawlessly. Thanks! it's what i wanted
– Nate
Dec 29 '18 at 8:01
You may need an added layer of quotes, e.g.'"Hello(1).csv"'
.
– hpaulj
Dec 29 '18 at 8:17
|
show 1 more comment
rm
can remove several files per call:
In [80]: !touch a.t1 b.t1 c.t1
In [81]: !ls *.t1
a.t1 b.t1 c.t1
In [82]: !rm -r a.t1 b.t1 c.t1
In [83]: !ls *.t1
ls: cannot access '*.t1': No such file or directory
If the starting point is a list of file names:
In [116]: alist = ['a.t1', 'b.t1', 'c.t1']
In [117]: astr = ' '.join(alist) # make a string
In [118]: !echo $astr # variable substitution as in BASH
a.t1 b.t1 c.t1
In [119]: !touch $astr # make 3 files
In [120]: ls *.t1
a.t1 b.t1 c.t1
In [121]: !rm -r $astr # remove them
In [122]: ls *.t1
ls: cannot access '*.t1': No such file or directory
Working with Python's own OS functions is probably better, but you can do much of the same stuff with %magics - if you understand shell well enough.
To use 'magics' in a Python expression, I have to use the underlying functions, not the '!' or '%' syntax, e.g.
import IPython
for txt in ['a.t1','b.t1','c.t1']:
IPython.utils.process.getoutput('touch %s'%txt)
The getoutput
function is used by %sx
(which underlies !!
) which uses subprocess.Popen
. But if you go to all that work you might as well use the os
functions that Python itself provides.
The file names may need an added layer of quoting to ensure that the shell doesn't give a syntax error:
In [129]: alist = ['"a(1).t1"', '"b(2).t1"', 'c.t1']
In [130]: astr = ' '.join(alist)
In [131]: !touch $astr
In [132]: !ls *.t1
'a(1).t1' a.t1 'b(2).t1' b.t1 c.t1
rm
can remove several files per call:
In [80]: !touch a.t1 b.t1 c.t1
In [81]: !ls *.t1
a.t1 b.t1 c.t1
In [82]: !rm -r a.t1 b.t1 c.t1
In [83]: !ls *.t1
ls: cannot access '*.t1': No such file or directory
If the starting point is a list of file names:
In [116]: alist = ['a.t1', 'b.t1', 'c.t1']
In [117]: astr = ' '.join(alist) # make a string
In [118]: !echo $astr # variable substitution as in BASH
a.t1 b.t1 c.t1
In [119]: !touch $astr # make 3 files
In [120]: ls *.t1
a.t1 b.t1 c.t1
In [121]: !rm -r $astr # remove them
In [122]: ls *.t1
ls: cannot access '*.t1': No such file or directory
Working with Python's own OS functions is probably better, but you can do much of the same stuff with %magics - if you understand shell well enough.
To use 'magics' in a Python expression, I have to use the underlying functions, not the '!' or '%' syntax, e.g.
import IPython
for txt in ['a.t1','b.t1','c.t1']:
IPython.utils.process.getoutput('touch %s'%txt)
The getoutput
function is used by %sx
(which underlies !!
) which uses subprocess.Popen
. But if you go to all that work you might as well use the os
functions that Python itself provides.
The file names may need an added layer of quoting to ensure that the shell doesn't give a syntax error:
In [129]: alist = ['"a(1).t1"', '"b(2).t1"', 'c.t1']
In [130]: astr = ' '.join(alist)
In [131]: !touch $astr
In [132]: !ls *.t1
'a(1).t1' a.t1 'b(2).t1' b.t1 c.t1
edited Dec 29 '18 at 8:16
answered Dec 29 '18 at 1:02
hpauljhpaulj
111k777145
111k777145
that's great and all, but doesnt work in a loop. '/bin/sh: 1: Syntax error: "(" unexpected'
– Nate
Dec 29 '18 at 6:43
The magic syntax with '!' and '%' (and unquoted strings) isn't valid Python, so doesn't work within Python structures like loops. Those expressions have to be used at top level where the IPython REPL can catch and parse them.
– hpaulj
Dec 29 '18 at 7:35
owh wait, it's because I have parentheses in the str (in a list). eg.files = ['Hello(1).csv', 'Hello(2).csv']
– Nate
Dec 29 '18 at 7:43
otherwise it works flawlessly. Thanks! it's what i wanted
– Nate
Dec 29 '18 at 8:01
You may need an added layer of quotes, e.g.'"Hello(1).csv"'
.
– hpaulj
Dec 29 '18 at 8:17
|
show 1 more comment
that's great and all, but doesnt work in a loop. '/bin/sh: 1: Syntax error: "(" unexpected'
– Nate
Dec 29 '18 at 6:43
The magic syntax with '!' and '%' (and unquoted strings) isn't valid Python, so doesn't work within Python structures like loops. Those expressions have to be used at top level where the IPython REPL can catch and parse them.
– hpaulj
Dec 29 '18 at 7:35
owh wait, it's because I have parentheses in the str (in a list). eg.files = ['Hello(1).csv', 'Hello(2).csv']
– Nate
Dec 29 '18 at 7:43
otherwise it works flawlessly. Thanks! it's what i wanted
– Nate
Dec 29 '18 at 8:01
You may need an added layer of quotes, e.g.'"Hello(1).csv"'
.
– hpaulj
Dec 29 '18 at 8:17
that's great and all, but doesnt work in a loop. '/bin/sh: 1: Syntax error: "(" unexpected'
– Nate
Dec 29 '18 at 6:43
that's great and all, but doesnt work in a loop. '/bin/sh: 1: Syntax error: "(" unexpected'
– Nate
Dec 29 '18 at 6:43
The magic syntax with '!' and '%' (and unquoted strings) isn't valid Python, so doesn't work within Python structures like loops. Those expressions have to be used at top level where the IPython REPL can catch and parse them.
– hpaulj
Dec 29 '18 at 7:35
The magic syntax with '!' and '%' (and unquoted strings) isn't valid Python, so doesn't work within Python structures like loops. Those expressions have to be used at top level where the IPython REPL can catch and parse them.
– hpaulj
Dec 29 '18 at 7:35
owh wait, it's because I have parentheses in the str (in a list). eg.
files = ['Hello(1).csv', 'Hello(2).csv']
– Nate
Dec 29 '18 at 7:43
owh wait, it's because I have parentheses in the str (in a list). eg.
files = ['Hello(1).csv', 'Hello(2).csv']
– Nate
Dec 29 '18 at 7:43
otherwise it works flawlessly. Thanks! it's what i wanted
– Nate
Dec 29 '18 at 8:01
otherwise it works flawlessly. Thanks! it's what i wanted
– Nate
Dec 29 '18 at 8:01
You may need an added layer of quotes, e.g.
'"Hello(1).csv"'
.– hpaulj
Dec 29 '18 at 8:17
You may need an added layer of quotes, e.g.
'"Hello(1).csv"'
.– hpaulj
Dec 29 '18 at 8:17
|
show 1 more comment
You can handle this in Python without magic shell commands. I recommend using the pathlib
module, for a more modern approach. For what you're doing, it would be:
import pathlib
csv_files = pathlib.Path('/path/to/actual/files')
for csv_file in csv_files.glob('*.csv'):
csv_file.unlink()
Use the .glob()
method to filter only the files you want to use, and .unlink()
to delete them (which is similar to os.remove()
).
Avoid using file
as a variable, as it's a reserved word in the language.
add a comment |
You can handle this in Python without magic shell commands. I recommend using the pathlib
module, for a more modern approach. For what you're doing, it would be:
import pathlib
csv_files = pathlib.Path('/path/to/actual/files')
for csv_file in csv_files.glob('*.csv'):
csv_file.unlink()
Use the .glob()
method to filter only the files you want to use, and .unlink()
to delete them (which is similar to os.remove()
).
Avoid using file
as a variable, as it's a reserved word in the language.
add a comment |
You can handle this in Python without magic shell commands. I recommend using the pathlib
module, for a more modern approach. For what you're doing, it would be:
import pathlib
csv_files = pathlib.Path('/path/to/actual/files')
for csv_file in csv_files.glob('*.csv'):
csv_file.unlink()
Use the .glob()
method to filter only the files you want to use, and .unlink()
to delete them (which is similar to os.remove()
).
Avoid using file
as a variable, as it's a reserved word in the language.
You can handle this in Python without magic shell commands. I recommend using the pathlib
module, for a more modern approach. For what you're doing, it would be:
import pathlib
csv_files = pathlib.Path('/path/to/actual/files')
for csv_file in csv_files.glob('*.csv'):
csv_file.unlink()
Use the .glob()
method to filter only the files you want to use, and .unlink()
to delete them (which is similar to os.remove()
).
Avoid using file
as a variable, as it's a reserved word in the language.
answered Dec 28 '18 at 23:57


Eron LloydEron Lloyd
11316
11316
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965438%2fhow-to-rm-python-var-in-jupyter-notebooks%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yAyoR52CnwS,lXzTo ecw,kycrg0 YMnWRReOki3STBndn,3uBtsn
Why do you need to use the shell?
CSV_Files.map(os.remove)
– Barmar
Dec 28 '18 at 23:39
Just a newbie's peeve of not wanting to import os. Was wondering if I could do this in a loop. [os.remove(file) for file in Files] works great btw.
– Nate
Dec 29 '18 at 7:13