Error when trying to use PIL image in Pyglet
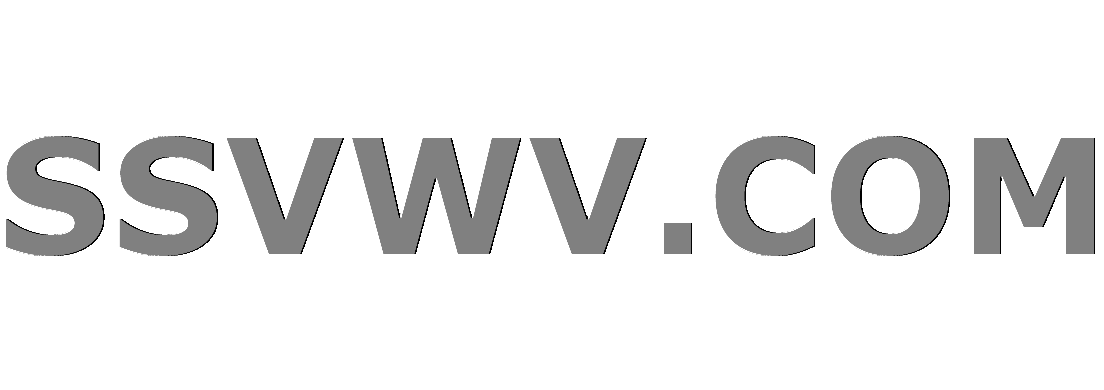
Multi tool use
I have a function which is suposed to generate a random star background using pillow which is then suposed to be used in pyglet:
from pyglet.image.codecs.png import PILImageDecoder
from PIL import Image, ImageDraw
import pyglet
import random
def generate_stars(map_size):
img = Image.new('RGB', map_size, color='black')
draw = ImageDraw.Draw(img)
for _ in range(3):
for x in range(0, map_size[0] + 1, 3):
for b in range(400, map_size[1] + 1, 400):
y = random.randint(b - 400, b)
draw.rectangle((x,y,x,y),fill='white')
return pyglet.image.load(img, decoder=PILImageDecoder())
window = pyglet.window.Window(1280,800)
stars = generate_stars((1280,800))
However executing this gets me the error:
Traceback (most recent call last):
File "/Users/jonaskosinski/Desktop/Python/Game/main_loop.py", line 18, in <module>
stars = generate_stars((1280,800))
File "/Users/jonaskosinski/Desktop/Python/Game/main_loop.py", line 15, in generate_stars
return pyglet.image.load(img, decoder=PILImageDecoder())
File "/usr/local/lib/python3.7/site-packages/pyglet/image/__init__.py", line 180, in load
file = open(filename, 'rb')
TypeError: expected str, bytes or os.PathLike object, not Image
python-3.x python-imaging-library pyglet
add a comment |
I have a function which is suposed to generate a random star background using pillow which is then suposed to be used in pyglet:
from pyglet.image.codecs.png import PILImageDecoder
from PIL import Image, ImageDraw
import pyglet
import random
def generate_stars(map_size):
img = Image.new('RGB', map_size, color='black')
draw = ImageDraw.Draw(img)
for _ in range(3):
for x in range(0, map_size[0] + 1, 3):
for b in range(400, map_size[1] + 1, 400):
y = random.randint(b - 400, b)
draw.rectangle((x,y,x,y),fill='white')
return pyglet.image.load(img, decoder=PILImageDecoder())
window = pyglet.window.Window(1280,800)
stars = generate_stars((1280,800))
However executing this gets me the error:
Traceback (most recent call last):
File "/Users/jonaskosinski/Desktop/Python/Game/main_loop.py", line 18, in <module>
stars = generate_stars((1280,800))
File "/Users/jonaskosinski/Desktop/Python/Game/main_loop.py", line 15, in generate_stars
return pyglet.image.load(img, decoder=PILImageDecoder())
File "/usr/local/lib/python3.7/site-packages/pyglet/image/__init__.py", line 180, in load
file = open(filename, 'rb')
TypeError: expected str, bytes or os.PathLike object, not Image
python-3.x python-imaging-library pyglet
Looking at the PIL documentation, maybe you needtobitmap
:pyglet.image.load(img.tobitmap(), decoder=PILImageDecoder())
– solarc
Dec 28 '18 at 23:28
Although looking at the PILImageDecoder code it seems it constructs the Image object from a filename. You might want to create your own Decoder based on this where you can pass the Image yourself. If your objective is to create the image in code, why not use pyglet.image.create?
– solarc
Dec 28 '18 at 23:37
@solarc That solved that error but gives me ValueError: not a bitmap
– Jonas
Dec 28 '18 at 23:48
Yes, see my second comment. The PILImageDecoder also expects the first argument ofpyglet.image.load
to be a filename. If you still want to create theImage
object yourself you'll have to create your own Decoder that skips loading from the filename and instead creates theImage
itself.
– solarc
Dec 28 '18 at 23:56
add a comment |
I have a function which is suposed to generate a random star background using pillow which is then suposed to be used in pyglet:
from pyglet.image.codecs.png import PILImageDecoder
from PIL import Image, ImageDraw
import pyglet
import random
def generate_stars(map_size):
img = Image.new('RGB', map_size, color='black')
draw = ImageDraw.Draw(img)
for _ in range(3):
for x in range(0, map_size[0] + 1, 3):
for b in range(400, map_size[1] + 1, 400):
y = random.randint(b - 400, b)
draw.rectangle((x,y,x,y),fill='white')
return pyglet.image.load(img, decoder=PILImageDecoder())
window = pyglet.window.Window(1280,800)
stars = generate_stars((1280,800))
However executing this gets me the error:
Traceback (most recent call last):
File "/Users/jonaskosinski/Desktop/Python/Game/main_loop.py", line 18, in <module>
stars = generate_stars((1280,800))
File "/Users/jonaskosinski/Desktop/Python/Game/main_loop.py", line 15, in generate_stars
return pyglet.image.load(img, decoder=PILImageDecoder())
File "/usr/local/lib/python3.7/site-packages/pyglet/image/__init__.py", line 180, in load
file = open(filename, 'rb')
TypeError: expected str, bytes or os.PathLike object, not Image
python-3.x python-imaging-library pyglet
I have a function which is suposed to generate a random star background using pillow which is then suposed to be used in pyglet:
from pyglet.image.codecs.png import PILImageDecoder
from PIL import Image, ImageDraw
import pyglet
import random
def generate_stars(map_size):
img = Image.new('RGB', map_size, color='black')
draw = ImageDraw.Draw(img)
for _ in range(3):
for x in range(0, map_size[0] + 1, 3):
for b in range(400, map_size[1] + 1, 400):
y = random.randint(b - 400, b)
draw.rectangle((x,y,x,y),fill='white')
return pyglet.image.load(img, decoder=PILImageDecoder())
window = pyglet.window.Window(1280,800)
stars = generate_stars((1280,800))
However executing this gets me the error:
Traceback (most recent call last):
File "/Users/jonaskosinski/Desktop/Python/Game/main_loop.py", line 18, in <module>
stars = generate_stars((1280,800))
File "/Users/jonaskosinski/Desktop/Python/Game/main_loop.py", line 15, in generate_stars
return pyglet.image.load(img, decoder=PILImageDecoder())
File "/usr/local/lib/python3.7/site-packages/pyglet/image/__init__.py", line 180, in load
file = open(filename, 'rb')
TypeError: expected str, bytes or os.PathLike object, not Image
python-3.x python-imaging-library pyglet
python-3.x python-imaging-library pyglet
edited Dec 28 '18 at 23:42
Jonas
asked Dec 28 '18 at 23:22


JonasJonas
305
305
Looking at the PIL documentation, maybe you needtobitmap
:pyglet.image.load(img.tobitmap(), decoder=PILImageDecoder())
– solarc
Dec 28 '18 at 23:28
Although looking at the PILImageDecoder code it seems it constructs the Image object from a filename. You might want to create your own Decoder based on this where you can pass the Image yourself. If your objective is to create the image in code, why not use pyglet.image.create?
– solarc
Dec 28 '18 at 23:37
@solarc That solved that error but gives me ValueError: not a bitmap
– Jonas
Dec 28 '18 at 23:48
Yes, see my second comment. The PILImageDecoder also expects the first argument ofpyglet.image.load
to be a filename. If you still want to create theImage
object yourself you'll have to create your own Decoder that skips loading from the filename and instead creates theImage
itself.
– solarc
Dec 28 '18 at 23:56
add a comment |
Looking at the PIL documentation, maybe you needtobitmap
:pyglet.image.load(img.tobitmap(), decoder=PILImageDecoder())
– solarc
Dec 28 '18 at 23:28
Although looking at the PILImageDecoder code it seems it constructs the Image object from a filename. You might want to create your own Decoder based on this where you can pass the Image yourself. If your objective is to create the image in code, why not use pyglet.image.create?
– solarc
Dec 28 '18 at 23:37
@solarc That solved that error but gives me ValueError: not a bitmap
– Jonas
Dec 28 '18 at 23:48
Yes, see my second comment. The PILImageDecoder also expects the first argument ofpyglet.image.load
to be a filename. If you still want to create theImage
object yourself you'll have to create your own Decoder that skips loading from the filename and instead creates theImage
itself.
– solarc
Dec 28 '18 at 23:56
Looking at the PIL documentation, maybe you need
tobitmap
: pyglet.image.load(img.tobitmap(), decoder=PILImageDecoder())
– solarc
Dec 28 '18 at 23:28
Looking at the PIL documentation, maybe you need
tobitmap
: pyglet.image.load(img.tobitmap(), decoder=PILImageDecoder())
– solarc
Dec 28 '18 at 23:28
Although looking at the PILImageDecoder code it seems it constructs the Image object from a filename. You might want to create your own Decoder based on this where you can pass the Image yourself. If your objective is to create the image in code, why not use pyglet.image.create?
– solarc
Dec 28 '18 at 23:37
Although looking at the PILImageDecoder code it seems it constructs the Image object from a filename. You might want to create your own Decoder based on this where you can pass the Image yourself. If your objective is to create the image in code, why not use pyglet.image.create?
– solarc
Dec 28 '18 at 23:37
@solarc That solved that error but gives me ValueError: not a bitmap
– Jonas
Dec 28 '18 at 23:48
@solarc That solved that error but gives me ValueError: not a bitmap
– Jonas
Dec 28 '18 at 23:48
Yes, see my second comment. The PILImageDecoder also expects the first argument of
pyglet.image.load
to be a filename. If you still want to create the Image
object yourself you'll have to create your own Decoder that skips loading from the filename and instead creates the Image
itself.– solarc
Dec 28 '18 at 23:56
Yes, see my second comment. The PILImageDecoder also expects the first argument of
pyglet.image.load
to be a filename. If you still want to create the Image
object yourself you'll have to create your own Decoder that skips loading from the filename and instead creates the Image
itself.– solarc
Dec 28 '18 at 23:56
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965347%2ferror-when-trying-to-use-pil-image-in-pyglet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965347%2ferror-when-trying-to-use-pil-image-in-pyglet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
o7sM22U7yd84lEkZatAwBSAtmGHEZaadNs,YfWFHO F9,EW,YCdr,5,ePxI1xNwovNj0YzzJ8Fw,4p
Looking at the PIL documentation, maybe you need
tobitmap
:pyglet.image.load(img.tobitmap(), decoder=PILImageDecoder())
– solarc
Dec 28 '18 at 23:28
Although looking at the PILImageDecoder code it seems it constructs the Image object from a filename. You might want to create your own Decoder based on this where you can pass the Image yourself. If your objective is to create the image in code, why not use pyglet.image.create?
– solarc
Dec 28 '18 at 23:37
@solarc That solved that error but gives me ValueError: not a bitmap
– Jonas
Dec 28 '18 at 23:48
Yes, see my second comment. The PILImageDecoder also expects the first argument of
pyglet.image.load
to be a filename. If you still want to create theImage
object yourself you'll have to create your own Decoder that skips loading from the filename and instead creates theImage
itself.– solarc
Dec 28 '18 at 23:56