Java associations: How to convert String to another Class [closed]
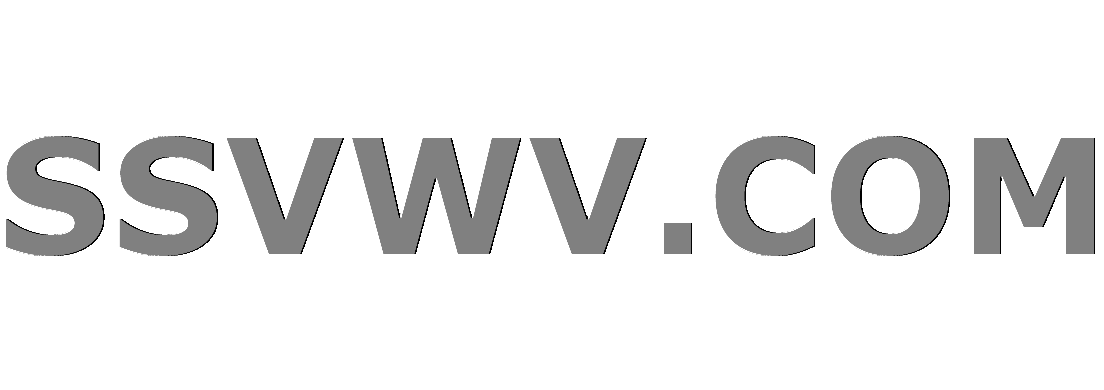
Multi tool use
I'm learning Java for school and started with a new chapter about associations. I have to make a program where 2 people enter their personal information and can marry. When I enter the name of the city, I get the error: "Expected: oef1_huwen.Gemeente (another exercise), Actual: java.lang.String. The city name has to be entered as a String but seen as Gemeente. Their information may only be entered using a constructor and I'm not allowed to use a setter for Gemeente.
I get an error on line 2 from HuwelijkApp
I really hope someone can help me, I'm studying for my exams right now... :s
Edit: Gemeente means city and Adres means address
This is some code from my class Persoon
package oef1_huwen;
public class Persoon {
private String naam;
private String voornaam;
private Datum geboortedatum;
private Adres adres;
public Persoon(String naam, String voornaam, int dag, int maand, int jaar,
String straat, String huisnummer, int postcode, String gemeentenaam) {
this.naam = naam;
this.voornaam = voornaam;
geboortedatum.setDag(dag);
geboortedatum.setMaand(maand);
geboortedatum.setJaar(jaar);
adres.setStraat(straat);
adres.setHuisNummer(huisnummer);
adres.getGemeente().setPostcode(postcode);
adres.getGemeente().setGemeenteNaam(gemeentenaam);
}
public Persoon(String naam, String voornaam, Datum geboortedatum, Adres
adres) {
this.naam = naam;
this.voornaam = voornaam;
this.geboortedatum = geboortedatum;
this.adres = adres;
}
This is from my class Gemeente
package oef1_huwen;
public class Gemeente {
private int postcode;
private String gemeenteNaam;
public Gemeente(int postcode, String gemeenteNaam) {
this.postcode = postcode;
this.gemeenteNaam = gemeenteNaam;
}
public int getPostcode() {
return postcode;
}
public void setPostcode(int postcode) {
this.postcode = postcode;
}
public String getGemeenteNaam() {
return gemeenteNaam;
}
public void setGemeenteNaam(String gemeenteNaam) {
this.gemeenteNaam = gemeenteNaam;
}
Code from my class Adres:
package oef1_huwen;
public class Adres {
private String straat;
private String huisNummer;
private Gemeente gemeente;
public Adres(String straat, String huisNummer, int postcode, Gemeente
gemeente) {
this.straat = straat;
this.huisNummer = huisNummer;
getGemeente().setPostcode(postcode);
this.gemeente = gemeente;
}
public String getStraat() {
return straat;
}
public void setStraat(String straat) {
this.straat = straat;
}
public String getHuisNummer() {
return huisNummer;
}
public void setHuisNummer(String huisNummer) {
this.huisNummer = huisNummer;
}
public Gemeente getGemeente() {
return gemeente;
}
public String toString() {
return straat + " " + huisNummer + "n" + gemeente;
}
}
And this is what I have in my class HuwelijkApp
Gemeente gemeente3 = new Gemeente(3290, "Diest");
Adres adres = new Adres("Kerkstraat", "12b", 3290, "Diest");
Persoon persoon = new Persoon("Aerts", "Jef", 29, 11, 1990, "Lindestraat", "23D", 3500, "Hasselt");
java
closed as off-topic by Dawood ibn Kareem, Mark Rotteveel, greg-449, leftjoin, EdChum Dec 29 '18 at 14:18
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Mark Rotteveel, greg-449, leftjoin, EdChum
If this question can be reworded to fit the rules in the help center, please edit the question.
|
show 13 more comments
I'm learning Java for school and started with a new chapter about associations. I have to make a program where 2 people enter their personal information and can marry. When I enter the name of the city, I get the error: "Expected: oef1_huwen.Gemeente (another exercise), Actual: java.lang.String. The city name has to be entered as a String but seen as Gemeente. Their information may only be entered using a constructor and I'm not allowed to use a setter for Gemeente.
I get an error on line 2 from HuwelijkApp
I really hope someone can help me, I'm studying for my exams right now... :s
Edit: Gemeente means city and Adres means address
This is some code from my class Persoon
package oef1_huwen;
public class Persoon {
private String naam;
private String voornaam;
private Datum geboortedatum;
private Adres adres;
public Persoon(String naam, String voornaam, int dag, int maand, int jaar,
String straat, String huisnummer, int postcode, String gemeentenaam) {
this.naam = naam;
this.voornaam = voornaam;
geboortedatum.setDag(dag);
geboortedatum.setMaand(maand);
geboortedatum.setJaar(jaar);
adres.setStraat(straat);
adres.setHuisNummer(huisnummer);
adres.getGemeente().setPostcode(postcode);
adres.getGemeente().setGemeenteNaam(gemeentenaam);
}
public Persoon(String naam, String voornaam, Datum geboortedatum, Adres
adres) {
this.naam = naam;
this.voornaam = voornaam;
this.geboortedatum = geboortedatum;
this.adres = adres;
}
This is from my class Gemeente
package oef1_huwen;
public class Gemeente {
private int postcode;
private String gemeenteNaam;
public Gemeente(int postcode, String gemeenteNaam) {
this.postcode = postcode;
this.gemeenteNaam = gemeenteNaam;
}
public int getPostcode() {
return postcode;
}
public void setPostcode(int postcode) {
this.postcode = postcode;
}
public String getGemeenteNaam() {
return gemeenteNaam;
}
public void setGemeenteNaam(String gemeenteNaam) {
this.gemeenteNaam = gemeenteNaam;
}
Code from my class Adres:
package oef1_huwen;
public class Adres {
private String straat;
private String huisNummer;
private Gemeente gemeente;
public Adres(String straat, String huisNummer, int postcode, Gemeente
gemeente) {
this.straat = straat;
this.huisNummer = huisNummer;
getGemeente().setPostcode(postcode);
this.gemeente = gemeente;
}
public String getStraat() {
return straat;
}
public void setStraat(String straat) {
this.straat = straat;
}
public String getHuisNummer() {
return huisNummer;
}
public void setHuisNummer(String huisNummer) {
this.huisNummer = huisNummer;
}
public Gemeente getGemeente() {
return gemeente;
}
public String toString() {
return straat + " " + huisNummer + "n" + gemeente;
}
}
And this is what I have in my class HuwelijkApp
Gemeente gemeente3 = new Gemeente(3290, "Diest");
Adres adres = new Adres("Kerkstraat", "12b", 3290, "Diest");
Persoon persoon = new Persoon("Aerts", "Jef", 29, 11, 1990, "Lindestraat", "23D", 3500, "Hasselt");
java
closed as off-topic by Dawood ibn Kareem, Mark Rotteveel, greg-449, leftjoin, EdChum Dec 29 '18 at 14:18
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Mark Rotteveel, greg-449, leftjoin, EdChum
If this question can be reworded to fit the rules in the help center, please edit the question.
When I enter the name of the city: what does that mean? Your code doesn't ask anyoe to enter anything. It seems like you have a compilation error. What exact and complete error message do you get, and what precise lineof code does it refer to?
– JB Nizet
Dec 28 '18 at 23:51
Can you show usGemeente
?
– Stalemate Of Tuning
Dec 28 '18 at 23:54
Can you post the full stack trace please?
– mypetlion
Dec 28 '18 at 23:57
And you seem to be mixing languages between your question and your code. Which of those variable names translates to "city"?
– mypetlion
Dec 29 '18 at 0:02
JB Nizet When I enter "Diest" in my Adres constructor
– user10615132
Dec 29 '18 at 0:02
|
show 13 more comments
I'm learning Java for school and started with a new chapter about associations. I have to make a program where 2 people enter their personal information and can marry. When I enter the name of the city, I get the error: "Expected: oef1_huwen.Gemeente (another exercise), Actual: java.lang.String. The city name has to be entered as a String but seen as Gemeente. Their information may only be entered using a constructor and I'm not allowed to use a setter for Gemeente.
I get an error on line 2 from HuwelijkApp
I really hope someone can help me, I'm studying for my exams right now... :s
Edit: Gemeente means city and Adres means address
This is some code from my class Persoon
package oef1_huwen;
public class Persoon {
private String naam;
private String voornaam;
private Datum geboortedatum;
private Adres adres;
public Persoon(String naam, String voornaam, int dag, int maand, int jaar,
String straat, String huisnummer, int postcode, String gemeentenaam) {
this.naam = naam;
this.voornaam = voornaam;
geboortedatum.setDag(dag);
geboortedatum.setMaand(maand);
geboortedatum.setJaar(jaar);
adres.setStraat(straat);
adres.setHuisNummer(huisnummer);
adres.getGemeente().setPostcode(postcode);
adres.getGemeente().setGemeenteNaam(gemeentenaam);
}
public Persoon(String naam, String voornaam, Datum geboortedatum, Adres
adres) {
this.naam = naam;
this.voornaam = voornaam;
this.geboortedatum = geboortedatum;
this.adres = adres;
}
This is from my class Gemeente
package oef1_huwen;
public class Gemeente {
private int postcode;
private String gemeenteNaam;
public Gemeente(int postcode, String gemeenteNaam) {
this.postcode = postcode;
this.gemeenteNaam = gemeenteNaam;
}
public int getPostcode() {
return postcode;
}
public void setPostcode(int postcode) {
this.postcode = postcode;
}
public String getGemeenteNaam() {
return gemeenteNaam;
}
public void setGemeenteNaam(String gemeenteNaam) {
this.gemeenteNaam = gemeenteNaam;
}
Code from my class Adres:
package oef1_huwen;
public class Adres {
private String straat;
private String huisNummer;
private Gemeente gemeente;
public Adres(String straat, String huisNummer, int postcode, Gemeente
gemeente) {
this.straat = straat;
this.huisNummer = huisNummer;
getGemeente().setPostcode(postcode);
this.gemeente = gemeente;
}
public String getStraat() {
return straat;
}
public void setStraat(String straat) {
this.straat = straat;
}
public String getHuisNummer() {
return huisNummer;
}
public void setHuisNummer(String huisNummer) {
this.huisNummer = huisNummer;
}
public Gemeente getGemeente() {
return gemeente;
}
public String toString() {
return straat + " " + huisNummer + "n" + gemeente;
}
}
And this is what I have in my class HuwelijkApp
Gemeente gemeente3 = new Gemeente(3290, "Diest");
Adres adres = new Adres("Kerkstraat", "12b", 3290, "Diest");
Persoon persoon = new Persoon("Aerts", "Jef", 29, 11, 1990, "Lindestraat", "23D", 3500, "Hasselt");
java
I'm learning Java for school and started with a new chapter about associations. I have to make a program where 2 people enter their personal information and can marry. When I enter the name of the city, I get the error: "Expected: oef1_huwen.Gemeente (another exercise), Actual: java.lang.String. The city name has to be entered as a String but seen as Gemeente. Their information may only be entered using a constructor and I'm not allowed to use a setter for Gemeente.
I get an error on line 2 from HuwelijkApp
I really hope someone can help me, I'm studying for my exams right now... :s
Edit: Gemeente means city and Adres means address
This is some code from my class Persoon
package oef1_huwen;
public class Persoon {
private String naam;
private String voornaam;
private Datum geboortedatum;
private Adres adres;
public Persoon(String naam, String voornaam, int dag, int maand, int jaar,
String straat, String huisnummer, int postcode, String gemeentenaam) {
this.naam = naam;
this.voornaam = voornaam;
geboortedatum.setDag(dag);
geboortedatum.setMaand(maand);
geboortedatum.setJaar(jaar);
adres.setStraat(straat);
adres.setHuisNummer(huisnummer);
adres.getGemeente().setPostcode(postcode);
adres.getGemeente().setGemeenteNaam(gemeentenaam);
}
public Persoon(String naam, String voornaam, Datum geboortedatum, Adres
adres) {
this.naam = naam;
this.voornaam = voornaam;
this.geboortedatum = geboortedatum;
this.adres = adres;
}
This is from my class Gemeente
package oef1_huwen;
public class Gemeente {
private int postcode;
private String gemeenteNaam;
public Gemeente(int postcode, String gemeenteNaam) {
this.postcode = postcode;
this.gemeenteNaam = gemeenteNaam;
}
public int getPostcode() {
return postcode;
}
public void setPostcode(int postcode) {
this.postcode = postcode;
}
public String getGemeenteNaam() {
return gemeenteNaam;
}
public void setGemeenteNaam(String gemeenteNaam) {
this.gemeenteNaam = gemeenteNaam;
}
Code from my class Adres:
package oef1_huwen;
public class Adres {
private String straat;
private String huisNummer;
private Gemeente gemeente;
public Adres(String straat, String huisNummer, int postcode, Gemeente
gemeente) {
this.straat = straat;
this.huisNummer = huisNummer;
getGemeente().setPostcode(postcode);
this.gemeente = gemeente;
}
public String getStraat() {
return straat;
}
public void setStraat(String straat) {
this.straat = straat;
}
public String getHuisNummer() {
return huisNummer;
}
public void setHuisNummer(String huisNummer) {
this.huisNummer = huisNummer;
}
public Gemeente getGemeente() {
return gemeente;
}
public String toString() {
return straat + " " + huisNummer + "n" + gemeente;
}
}
And this is what I have in my class HuwelijkApp
Gemeente gemeente3 = new Gemeente(3290, "Diest");
Adres adres = new Adres("Kerkstraat", "12b", 3290, "Diest");
Persoon persoon = new Persoon("Aerts", "Jef", 29, 11, 1990, "Lindestraat", "23D", 3500, "Hasselt");
java
java
edited Dec 29 '18 at 0:16
asked Dec 28 '18 at 23:47
user10615132
closed as off-topic by Dawood ibn Kareem, Mark Rotteveel, greg-449, leftjoin, EdChum Dec 29 '18 at 14:18
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Mark Rotteveel, greg-449, leftjoin, EdChum
If this question can be reworded to fit the rules in the help center, please edit the question.
closed as off-topic by Dawood ibn Kareem, Mark Rotteveel, greg-449, leftjoin, EdChum Dec 29 '18 at 14:18
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Mark Rotteveel, greg-449, leftjoin, EdChum
If this question can be reworded to fit the rules in the help center, please edit the question.
When I enter the name of the city: what does that mean? Your code doesn't ask anyoe to enter anything. It seems like you have a compilation error. What exact and complete error message do you get, and what precise lineof code does it refer to?
– JB Nizet
Dec 28 '18 at 23:51
Can you show usGemeente
?
– Stalemate Of Tuning
Dec 28 '18 at 23:54
Can you post the full stack trace please?
– mypetlion
Dec 28 '18 at 23:57
And you seem to be mixing languages between your question and your code. Which of those variable names translates to "city"?
– mypetlion
Dec 29 '18 at 0:02
JB Nizet When I enter "Diest" in my Adres constructor
– user10615132
Dec 29 '18 at 0:02
|
show 13 more comments
When I enter the name of the city: what does that mean? Your code doesn't ask anyoe to enter anything. It seems like you have a compilation error. What exact and complete error message do you get, and what precise lineof code does it refer to?
– JB Nizet
Dec 28 '18 at 23:51
Can you show usGemeente
?
– Stalemate Of Tuning
Dec 28 '18 at 23:54
Can you post the full stack trace please?
– mypetlion
Dec 28 '18 at 23:57
And you seem to be mixing languages between your question and your code. Which of those variable names translates to "city"?
– mypetlion
Dec 29 '18 at 0:02
JB Nizet When I enter "Diest" in my Adres constructor
– user10615132
Dec 29 '18 at 0:02
When I enter the name of the city: what does that mean? Your code doesn't ask anyoe to enter anything. It seems like you have a compilation error. What exact and complete error message do you get, and what precise lineof code does it refer to?
– JB Nizet
Dec 28 '18 at 23:51
When I enter the name of the city: what does that mean? Your code doesn't ask anyoe to enter anything. It seems like you have a compilation error. What exact and complete error message do you get, and what precise lineof code does it refer to?
– JB Nizet
Dec 28 '18 at 23:51
Can you show us
Gemeente
?– Stalemate Of Tuning
Dec 28 '18 at 23:54
Can you show us
Gemeente
?– Stalemate Of Tuning
Dec 28 '18 at 23:54
Can you post the full stack trace please?
– mypetlion
Dec 28 '18 at 23:57
Can you post the full stack trace please?
– mypetlion
Dec 28 '18 at 23:57
And you seem to be mixing languages between your question and your code. Which of those variable names translates to "city"?
– mypetlion
Dec 29 '18 at 0:02
And you seem to be mixing languages between your question and your code. Which of those variable names translates to "city"?
– mypetlion
Dec 29 '18 at 0:02
JB Nizet When I enter "Diest" in my Adres constructor
– user10615132
Dec 29 '18 at 0:02
JB Nizet When I enter "Diest" in my Adres constructor
– user10615132
Dec 29 '18 at 0:02
|
show 13 more comments
1 Answer
1
active
oldest
votes
Can you alter your Persoon
constructor? You have parameters int postcode
and String gemeentenaam
, but you could instead make one parameter Gemeente gemeentenaam
and add a new instance of Gemeente
. The Gemeente
constructor takes in the same two parameters.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Can you alter your Persoon
constructor? You have parameters int postcode
and String gemeentenaam
, but you could instead make one parameter Gemeente gemeentenaam
and add a new instance of Gemeente
. The Gemeente
constructor takes in the same two parameters.
add a comment |
Can you alter your Persoon
constructor? You have parameters int postcode
and String gemeentenaam
, but you could instead make one parameter Gemeente gemeentenaam
and add a new instance of Gemeente
. The Gemeente
constructor takes in the same two parameters.
add a comment |
Can you alter your Persoon
constructor? You have parameters int postcode
and String gemeentenaam
, but you could instead make one parameter Gemeente gemeentenaam
and add a new instance of Gemeente
. The Gemeente
constructor takes in the same two parameters.
Can you alter your Persoon
constructor? You have parameters int postcode
and String gemeentenaam
, but you could instead make one parameter Gemeente gemeentenaam
and add a new instance of Gemeente
. The Gemeente
constructor takes in the same two parameters.
edited Dec 29 '18 at 0:42
pzaenger
2,91211626
2,91211626
answered Dec 29 '18 at 0:41
mpiempie
134
134
add a comment |
add a comment |
lkkAP7guNKKWFEj748mYKt08yQI9oLesBd Y2TVj,jN7oC,LAJu9TepGJrHkdC jqE7 V8AeYblvDZk5AF jJPGFRuIu
When I enter the name of the city: what does that mean? Your code doesn't ask anyoe to enter anything. It seems like you have a compilation error. What exact and complete error message do you get, and what precise lineof code does it refer to?
– JB Nizet
Dec 28 '18 at 23:51
Can you show us
Gemeente
?– Stalemate Of Tuning
Dec 28 '18 at 23:54
Can you post the full stack trace please?
– mypetlion
Dec 28 '18 at 23:57
And you seem to be mixing languages between your question and your code. Which of those variable names translates to "city"?
– mypetlion
Dec 29 '18 at 0:02
JB Nizet When I enter "Diest" in my Adres constructor
– user10615132
Dec 29 '18 at 0:02