Hibernate delete content of database table
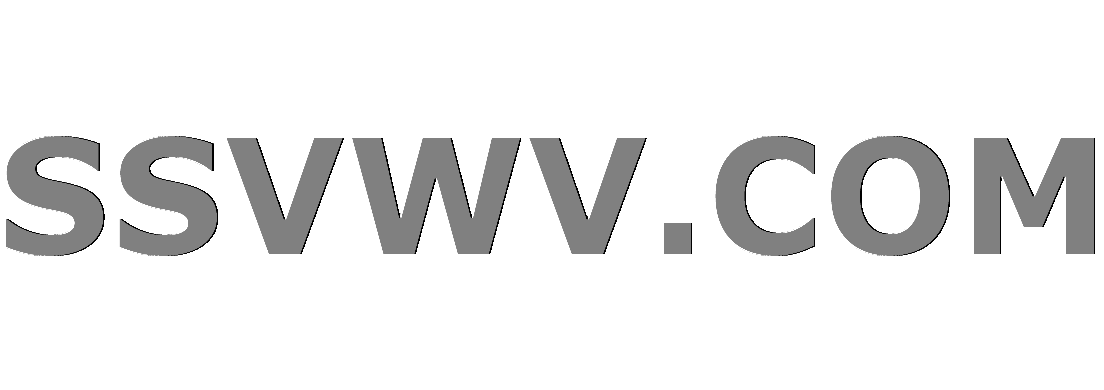
Multi tool use
I try to implement a CRUD application in Java using JPA with Hibernate.
I have two independent tests that act on two different database tables. When I run one of these tests, the corresponding table is populated correctly, but when I run the other test the table that was previously populated is emptied and the other table is filled.
This is my configuration.cfg.xml
file for the configuration of hibernate:
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">org.sqlite.JDBC</property>
<property name="hibernate.connection.url">
jdbc:sqlite:/Users/th3g3ntl3man/Repository/assignment3/src/main/resources/database.db
</property>
<property name="dialect">com.enigmabridge.hibernate.dialect.SQLiteDialect</property>
<property name="show_sql">true</property>
<property name="hibernate.hbm2ddl.auto">create</property>
<property name="hibernate.current_session_context_class">
org.hibernate.context.internal.ThreadLocalSessionContext
</property>
<mapping class="entity.User"/>
<mapping class="entity.Topic"/>
<mapping class="entity.Image"/>
</session-factory>
</hibernate-configuration>
I suppose that the problem was in the property hbm2ddl.auto
, thus I try to change the value from create
to update
but when I compile one test I works correctly but when I run a second test the test crash with this stack trace:
java.lang.ExceptionInInitializerError
at util.Operation.getByPrimaryKey(Operation.java:34)
at dao.TopicDAO.create(TopicDAO.java:22)
at entity.TopicTest.setUp(TopicTest.java:22)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:566)
at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50)
at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12)
at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47)
at org.junit.internal.runners.statements.RunBefores.evaluate(RunBefores.java:24)
at org.junit.runners.ParentRunner.run(ParentRunner.java:363)
at org.junit.runner.JUnitCore.run(JUnitCore.java:137)
at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:68)
at com.intellij.rt.execution.junit.IdeaTestRunner$Repeater.startRunnerWithArgs(IdeaTestRunner.java:47)
at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:242)
at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:70)
Caused by: java.util.NoSuchElementException
at java.base/java.util.StringTokenizer.nextToken(StringTokenizer.java:349)
at org.hibernate.tool.schema.extract.internal.InformationExtractorJdbcDatabaseMetaDataImpl.populateTablesWithColumns(InformationExtractorJdbcDatabaseMetaDataImpl.java:369)
at org.hibernate.tool.schema.extract.internal.InformationExtractorJdbcDatabaseMetaDataImpl.getTables(InformationExtractorJdbcDatabaseMetaDataImpl.java:337)
at org.hibernate.tool.schema.extract.internal.DatabaseInformationImpl.getTablesInformation(DatabaseInformationImpl.java:120)
at org.hibernate.tool.schema.internal.GroupedSchemaMigratorImpl.performTablesMigration(GroupedSchemaMigratorImpl.java:65)
at org.hibernate.tool.schema.internal.AbstractSchemaMigrator.performMigration(AbstractSchemaMigrator.java:207)
at org.hibernate.tool.schema.internal.AbstractSchemaMigrator.doMigration(AbstractSchemaMigrator.java:114)
at org.hibernate.tool.schema.spi.SchemaManagementToolCoordinator.performDatabaseAction(SchemaManagementToolCoordinator.java:183)
at org.hibernate.tool.schema.spi.SchemaManagementToolCoordinator.process(SchemaManagementToolCoordinator.java:72)
at org.hibernate.internal.SessionFactoryImpl.<init>(SessionFactoryImpl.java:309)
at org.hibernate.boot.internal.SessionFactoryBuilderImpl.build(SessionFactoryBuilderImpl.java:462)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:708)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:724)
at util.HibernateUtil.init(HibernateUtil.java:11)
at util.HibernateUtil.<clinit>(HibernateUtil.java:8)
For the all code, this is the repository link
java hibernate
add a comment |
I try to implement a CRUD application in Java using JPA with Hibernate.
I have two independent tests that act on two different database tables. When I run one of these tests, the corresponding table is populated correctly, but when I run the other test the table that was previously populated is emptied and the other table is filled.
This is my configuration.cfg.xml
file for the configuration of hibernate:
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">org.sqlite.JDBC</property>
<property name="hibernate.connection.url">
jdbc:sqlite:/Users/th3g3ntl3man/Repository/assignment3/src/main/resources/database.db
</property>
<property name="dialect">com.enigmabridge.hibernate.dialect.SQLiteDialect</property>
<property name="show_sql">true</property>
<property name="hibernate.hbm2ddl.auto">create</property>
<property name="hibernate.current_session_context_class">
org.hibernate.context.internal.ThreadLocalSessionContext
</property>
<mapping class="entity.User"/>
<mapping class="entity.Topic"/>
<mapping class="entity.Image"/>
</session-factory>
</hibernate-configuration>
I suppose that the problem was in the property hbm2ddl.auto
, thus I try to change the value from create
to update
but when I compile one test I works correctly but when I run a second test the test crash with this stack trace:
java.lang.ExceptionInInitializerError
at util.Operation.getByPrimaryKey(Operation.java:34)
at dao.TopicDAO.create(TopicDAO.java:22)
at entity.TopicTest.setUp(TopicTest.java:22)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:566)
at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50)
at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12)
at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47)
at org.junit.internal.runners.statements.RunBefores.evaluate(RunBefores.java:24)
at org.junit.runners.ParentRunner.run(ParentRunner.java:363)
at org.junit.runner.JUnitCore.run(JUnitCore.java:137)
at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:68)
at com.intellij.rt.execution.junit.IdeaTestRunner$Repeater.startRunnerWithArgs(IdeaTestRunner.java:47)
at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:242)
at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:70)
Caused by: java.util.NoSuchElementException
at java.base/java.util.StringTokenizer.nextToken(StringTokenizer.java:349)
at org.hibernate.tool.schema.extract.internal.InformationExtractorJdbcDatabaseMetaDataImpl.populateTablesWithColumns(InformationExtractorJdbcDatabaseMetaDataImpl.java:369)
at org.hibernate.tool.schema.extract.internal.InformationExtractorJdbcDatabaseMetaDataImpl.getTables(InformationExtractorJdbcDatabaseMetaDataImpl.java:337)
at org.hibernate.tool.schema.extract.internal.DatabaseInformationImpl.getTablesInformation(DatabaseInformationImpl.java:120)
at org.hibernate.tool.schema.internal.GroupedSchemaMigratorImpl.performTablesMigration(GroupedSchemaMigratorImpl.java:65)
at org.hibernate.tool.schema.internal.AbstractSchemaMigrator.performMigration(AbstractSchemaMigrator.java:207)
at org.hibernate.tool.schema.internal.AbstractSchemaMigrator.doMigration(AbstractSchemaMigrator.java:114)
at org.hibernate.tool.schema.spi.SchemaManagementToolCoordinator.performDatabaseAction(SchemaManagementToolCoordinator.java:183)
at org.hibernate.tool.schema.spi.SchemaManagementToolCoordinator.process(SchemaManagementToolCoordinator.java:72)
at org.hibernate.internal.SessionFactoryImpl.<init>(SessionFactoryImpl.java:309)
at org.hibernate.boot.internal.SessionFactoryBuilderImpl.build(SessionFactoryBuilderImpl.java:462)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:708)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:724)
at util.HibernateUtil.init(HibernateUtil.java:11)
at util.HibernateUtil.<clinit>(HibernateUtil.java:8)
For the all code, this is the repository link
java hibernate
The JPA API does not use hibernate specific antiquated files. Usepersistence.xml
otherwise remove the JPA tag
– Billy Frost
Dec 29 '18 at 9:09
@BillyFrost Sorry, I just edit the post
– th3g3ntl3man
Dec 29 '18 at 14:00
add a comment |
I try to implement a CRUD application in Java using JPA with Hibernate.
I have two independent tests that act on two different database tables. When I run one of these tests, the corresponding table is populated correctly, but when I run the other test the table that was previously populated is emptied and the other table is filled.
This is my configuration.cfg.xml
file for the configuration of hibernate:
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">org.sqlite.JDBC</property>
<property name="hibernate.connection.url">
jdbc:sqlite:/Users/th3g3ntl3man/Repository/assignment3/src/main/resources/database.db
</property>
<property name="dialect">com.enigmabridge.hibernate.dialect.SQLiteDialect</property>
<property name="show_sql">true</property>
<property name="hibernate.hbm2ddl.auto">create</property>
<property name="hibernate.current_session_context_class">
org.hibernate.context.internal.ThreadLocalSessionContext
</property>
<mapping class="entity.User"/>
<mapping class="entity.Topic"/>
<mapping class="entity.Image"/>
</session-factory>
</hibernate-configuration>
I suppose that the problem was in the property hbm2ddl.auto
, thus I try to change the value from create
to update
but when I compile one test I works correctly but when I run a second test the test crash with this stack trace:
java.lang.ExceptionInInitializerError
at util.Operation.getByPrimaryKey(Operation.java:34)
at dao.TopicDAO.create(TopicDAO.java:22)
at entity.TopicTest.setUp(TopicTest.java:22)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:566)
at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50)
at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12)
at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47)
at org.junit.internal.runners.statements.RunBefores.evaluate(RunBefores.java:24)
at org.junit.runners.ParentRunner.run(ParentRunner.java:363)
at org.junit.runner.JUnitCore.run(JUnitCore.java:137)
at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:68)
at com.intellij.rt.execution.junit.IdeaTestRunner$Repeater.startRunnerWithArgs(IdeaTestRunner.java:47)
at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:242)
at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:70)
Caused by: java.util.NoSuchElementException
at java.base/java.util.StringTokenizer.nextToken(StringTokenizer.java:349)
at org.hibernate.tool.schema.extract.internal.InformationExtractorJdbcDatabaseMetaDataImpl.populateTablesWithColumns(InformationExtractorJdbcDatabaseMetaDataImpl.java:369)
at org.hibernate.tool.schema.extract.internal.InformationExtractorJdbcDatabaseMetaDataImpl.getTables(InformationExtractorJdbcDatabaseMetaDataImpl.java:337)
at org.hibernate.tool.schema.extract.internal.DatabaseInformationImpl.getTablesInformation(DatabaseInformationImpl.java:120)
at org.hibernate.tool.schema.internal.GroupedSchemaMigratorImpl.performTablesMigration(GroupedSchemaMigratorImpl.java:65)
at org.hibernate.tool.schema.internal.AbstractSchemaMigrator.performMigration(AbstractSchemaMigrator.java:207)
at org.hibernate.tool.schema.internal.AbstractSchemaMigrator.doMigration(AbstractSchemaMigrator.java:114)
at org.hibernate.tool.schema.spi.SchemaManagementToolCoordinator.performDatabaseAction(SchemaManagementToolCoordinator.java:183)
at org.hibernate.tool.schema.spi.SchemaManagementToolCoordinator.process(SchemaManagementToolCoordinator.java:72)
at org.hibernate.internal.SessionFactoryImpl.<init>(SessionFactoryImpl.java:309)
at org.hibernate.boot.internal.SessionFactoryBuilderImpl.build(SessionFactoryBuilderImpl.java:462)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:708)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:724)
at util.HibernateUtil.init(HibernateUtil.java:11)
at util.HibernateUtil.<clinit>(HibernateUtil.java:8)
For the all code, this is the repository link
java hibernate
I try to implement a CRUD application in Java using JPA with Hibernate.
I have two independent tests that act on two different database tables. When I run one of these tests, the corresponding table is populated correctly, but when I run the other test the table that was previously populated is emptied and the other table is filled.
This is my configuration.cfg.xml
file for the configuration of hibernate:
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">org.sqlite.JDBC</property>
<property name="hibernate.connection.url">
jdbc:sqlite:/Users/th3g3ntl3man/Repository/assignment3/src/main/resources/database.db
</property>
<property name="dialect">com.enigmabridge.hibernate.dialect.SQLiteDialect</property>
<property name="show_sql">true</property>
<property name="hibernate.hbm2ddl.auto">create</property>
<property name="hibernate.current_session_context_class">
org.hibernate.context.internal.ThreadLocalSessionContext
</property>
<mapping class="entity.User"/>
<mapping class="entity.Topic"/>
<mapping class="entity.Image"/>
</session-factory>
</hibernate-configuration>
I suppose that the problem was in the property hbm2ddl.auto
, thus I try to change the value from create
to update
but when I compile one test I works correctly but when I run a second test the test crash with this stack trace:
java.lang.ExceptionInInitializerError
at util.Operation.getByPrimaryKey(Operation.java:34)
at dao.TopicDAO.create(TopicDAO.java:22)
at entity.TopicTest.setUp(TopicTest.java:22)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:566)
at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50)
at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12)
at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47)
at org.junit.internal.runners.statements.RunBefores.evaluate(RunBefores.java:24)
at org.junit.runners.ParentRunner.run(ParentRunner.java:363)
at org.junit.runner.JUnitCore.run(JUnitCore.java:137)
at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:68)
at com.intellij.rt.execution.junit.IdeaTestRunner$Repeater.startRunnerWithArgs(IdeaTestRunner.java:47)
at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:242)
at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:70)
Caused by: java.util.NoSuchElementException
at java.base/java.util.StringTokenizer.nextToken(StringTokenizer.java:349)
at org.hibernate.tool.schema.extract.internal.InformationExtractorJdbcDatabaseMetaDataImpl.populateTablesWithColumns(InformationExtractorJdbcDatabaseMetaDataImpl.java:369)
at org.hibernate.tool.schema.extract.internal.InformationExtractorJdbcDatabaseMetaDataImpl.getTables(InformationExtractorJdbcDatabaseMetaDataImpl.java:337)
at org.hibernate.tool.schema.extract.internal.DatabaseInformationImpl.getTablesInformation(DatabaseInformationImpl.java:120)
at org.hibernate.tool.schema.internal.GroupedSchemaMigratorImpl.performTablesMigration(GroupedSchemaMigratorImpl.java:65)
at org.hibernate.tool.schema.internal.AbstractSchemaMigrator.performMigration(AbstractSchemaMigrator.java:207)
at org.hibernate.tool.schema.internal.AbstractSchemaMigrator.doMigration(AbstractSchemaMigrator.java:114)
at org.hibernate.tool.schema.spi.SchemaManagementToolCoordinator.performDatabaseAction(SchemaManagementToolCoordinator.java:183)
at org.hibernate.tool.schema.spi.SchemaManagementToolCoordinator.process(SchemaManagementToolCoordinator.java:72)
at org.hibernate.internal.SessionFactoryImpl.<init>(SessionFactoryImpl.java:309)
at org.hibernate.boot.internal.SessionFactoryBuilderImpl.build(SessionFactoryBuilderImpl.java:462)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:708)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:724)
at util.HibernateUtil.init(HibernateUtil.java:11)
at util.HibernateUtil.<clinit>(HibernateUtil.java:8)
For the all code, this is the repository link
java hibernate
java hibernate
edited Dec 29 '18 at 14:00
th3g3ntl3man
asked Dec 28 '18 at 23:44


th3g3ntl3manth3g3ntl3man
3681310
3681310
The JPA API does not use hibernate specific antiquated files. Usepersistence.xml
otherwise remove the JPA tag
– Billy Frost
Dec 29 '18 at 9:09
@BillyFrost Sorry, I just edit the post
– th3g3ntl3man
Dec 29 '18 at 14:00
add a comment |
The JPA API does not use hibernate specific antiquated files. Usepersistence.xml
otherwise remove the JPA tag
– Billy Frost
Dec 29 '18 at 9:09
@BillyFrost Sorry, I just edit the post
– th3g3ntl3man
Dec 29 '18 at 14:00
The JPA API does not use hibernate specific antiquated files. Use
persistence.xml
otherwise remove the JPA tag– Billy Frost
Dec 29 '18 at 9:09
The JPA API does not use hibernate specific antiquated files. Use
persistence.xml
otherwise remove the JPA tag– Billy Frost
Dec 29 '18 at 9:09
@BillyFrost Sorry, I just edit the post
– th3g3ntl3man
Dec 29 '18 at 14:00
@BillyFrost Sorry, I just edit the post
– th3g3ntl3man
Dec 29 '18 at 14:00
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965479%2fhibernate-delete-content-of-database-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53965479%2fhibernate-delete-content-of-database-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
U13,3 Y3JOaiHXZPS8dsTdfGj6Ok1PRk t,PrKEQTxRPptMc8HxrNHr1tNmC2sXR1E,6ErlE9GhD9ND92uM6k0CLoi1l,wmd9
The JPA API does not use hibernate specific antiquated files. Use
persistence.xml
otherwise remove the JPA tag– Billy Frost
Dec 29 '18 at 9:09
@BillyFrost Sorry, I just edit the post
– th3g3ntl3man
Dec 29 '18 at 14:00