RangeError: Maximum call stack size exceeded Lazy routing Angular 2
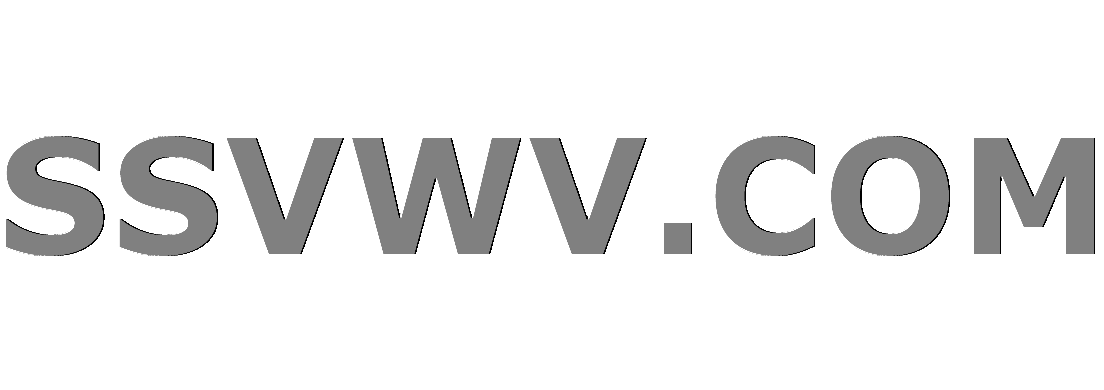
Multi tool use
I'm trying to implement lazy routing into my app.
I have a very big project and when it was at router-deprecated I used AsyncRoute, but now it was removed.
So I tried to implement newest lazy loading, but I got an issue
RangeError: Maximum call stack size exceeded
What I'm doing wrong? I did all code like in instructions.
Take a look please
EncountersModule
import { NgModule } from '@angular/core';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
@NgModule({
imports: [ SharedModule ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
Here is my app.routing.module
import { NgModule } from '@angular/core';
// import { ModuleWithProviders } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ImagingComponent } from '../modules/index';
import { DashboardComponent } from '../modules/index';
import { PrescriptionNoticesComponent } from '../modules/index';
// import { EncountersComponent } from "../modules/encounters/encounters.component";
import { ScheduleComponent } from "../modules/schedule/schedule.component";
import { AdminComponent } from '../modules/index';
@NgModule({
imports: [
RouterModule.forRoot([
{
path: '',
component: DashboardComponent,
data: { label: 'Dashboard' }
},
{
path: 'encounters',
// component: EncountersComponent,
loadChildren: 'production/modules/encounters/encounters.module#EncountersModule',
data: { label: 'Encounters' }
},
{
path: 'admin',
component: AdminComponent,
data: { label: 'Admin' }
}
])
],
exports: [
RouterModule
]
})
export class AppRoutingModule {}
// const appRoutes: Routes = [
// {
// path: 'imaging',
// component: ImagingComponent,
// data: { label: 'Imaging' }
// },
// {
// path: '',
// component: DashboardComponent,
// data: { label: 'Dashboard' }
// },
// {
// path: 'prescription_notices',
// component: PrescriptionNoticesComponent,
// data: { label: 'Prescription Notices' }
// },
// {
// path: 'encounters',
// component: EncountersComponent,
// data: { label: 'Encounters' }
// },
// {
// path: 'schedule',
// component: ScheduleComponent,
// data: { label: 'Schedule' }
// },
// {
// path: 'admin',
// component: AdminComponent,
// data: { label: 'Admin' }
// }
// ];
//
// export const appRoutingProviders: any = [
//
// ];
//
// export const routing: ModuleWithProviders = RouterModule.forRoot(appRoutes);
angular typescript angular2-routing
add a comment |
I'm trying to implement lazy routing into my app.
I have a very big project and when it was at router-deprecated I used AsyncRoute, but now it was removed.
So I tried to implement newest lazy loading, but I got an issue
RangeError: Maximum call stack size exceeded
What I'm doing wrong? I did all code like in instructions.
Take a look please
EncountersModule
import { NgModule } from '@angular/core';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
@NgModule({
imports: [ SharedModule ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
Here is my app.routing.module
import { NgModule } from '@angular/core';
// import { ModuleWithProviders } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ImagingComponent } from '../modules/index';
import { DashboardComponent } from '../modules/index';
import { PrescriptionNoticesComponent } from '../modules/index';
// import { EncountersComponent } from "../modules/encounters/encounters.component";
import { ScheduleComponent } from "../modules/schedule/schedule.component";
import { AdminComponent } from '../modules/index';
@NgModule({
imports: [
RouterModule.forRoot([
{
path: '',
component: DashboardComponent,
data: { label: 'Dashboard' }
},
{
path: 'encounters',
// component: EncountersComponent,
loadChildren: 'production/modules/encounters/encounters.module#EncountersModule',
data: { label: 'Encounters' }
},
{
path: 'admin',
component: AdminComponent,
data: { label: 'Admin' }
}
])
],
exports: [
RouterModule
]
})
export class AppRoutingModule {}
// const appRoutes: Routes = [
// {
// path: 'imaging',
// component: ImagingComponent,
// data: { label: 'Imaging' }
// },
// {
// path: '',
// component: DashboardComponent,
// data: { label: 'Dashboard' }
// },
// {
// path: 'prescription_notices',
// component: PrescriptionNoticesComponent,
// data: { label: 'Prescription Notices' }
// },
// {
// path: 'encounters',
// component: EncountersComponent,
// data: { label: 'Encounters' }
// },
// {
// path: 'schedule',
// component: ScheduleComponent,
// data: { label: 'Schedule' }
// },
// {
// path: 'admin',
// component: AdminComponent,
// data: { label: 'Admin' }
// }
// ];
//
// export const appRoutingProviders: any = [
//
// ];
//
// export const routing: ModuleWithProviders = RouterModule.forRoot(appRoutes);
angular typescript angular2-routing
1
probably it because I didn't have any routing for my encounters.module
– Velidan
Oct 19 '16 at 14:01
Possibly try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away. Although I had all of my comments at the top of my code so I am not sure if that applies here.
– Frank
Oct 19 '16 at 19:20
Thanks Frank for your help!
– Velidan
Oct 20 '16 at 11:37
Glad that worked! I posted the answer so people can find it easier. That same problem had me stumped for almost a whole day when I was updating.
– Frank
Oct 20 '16 at 18:03
add a comment |
I'm trying to implement lazy routing into my app.
I have a very big project and when it was at router-deprecated I used AsyncRoute, but now it was removed.
So I tried to implement newest lazy loading, but I got an issue
RangeError: Maximum call stack size exceeded
What I'm doing wrong? I did all code like in instructions.
Take a look please
EncountersModule
import { NgModule } from '@angular/core';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
@NgModule({
imports: [ SharedModule ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
Here is my app.routing.module
import { NgModule } from '@angular/core';
// import { ModuleWithProviders } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ImagingComponent } from '../modules/index';
import { DashboardComponent } from '../modules/index';
import { PrescriptionNoticesComponent } from '../modules/index';
// import { EncountersComponent } from "../modules/encounters/encounters.component";
import { ScheduleComponent } from "../modules/schedule/schedule.component";
import { AdminComponent } from '../modules/index';
@NgModule({
imports: [
RouterModule.forRoot([
{
path: '',
component: DashboardComponent,
data: { label: 'Dashboard' }
},
{
path: 'encounters',
// component: EncountersComponent,
loadChildren: 'production/modules/encounters/encounters.module#EncountersModule',
data: { label: 'Encounters' }
},
{
path: 'admin',
component: AdminComponent,
data: { label: 'Admin' }
}
])
],
exports: [
RouterModule
]
})
export class AppRoutingModule {}
// const appRoutes: Routes = [
// {
// path: 'imaging',
// component: ImagingComponent,
// data: { label: 'Imaging' }
// },
// {
// path: '',
// component: DashboardComponent,
// data: { label: 'Dashboard' }
// },
// {
// path: 'prescription_notices',
// component: PrescriptionNoticesComponent,
// data: { label: 'Prescription Notices' }
// },
// {
// path: 'encounters',
// component: EncountersComponent,
// data: { label: 'Encounters' }
// },
// {
// path: 'schedule',
// component: ScheduleComponent,
// data: { label: 'Schedule' }
// },
// {
// path: 'admin',
// component: AdminComponent,
// data: { label: 'Admin' }
// }
// ];
//
// export const appRoutingProviders: any = [
//
// ];
//
// export const routing: ModuleWithProviders = RouterModule.forRoot(appRoutes);
angular typescript angular2-routing
I'm trying to implement lazy routing into my app.
I have a very big project and when it was at router-deprecated I used AsyncRoute, but now it was removed.
So I tried to implement newest lazy loading, but I got an issue
RangeError: Maximum call stack size exceeded
What I'm doing wrong? I did all code like in instructions.
Take a look please
EncountersModule
import { NgModule } from '@angular/core';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
@NgModule({
imports: [ SharedModule ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
Here is my app.routing.module
import { NgModule } from '@angular/core';
// import { ModuleWithProviders } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ImagingComponent } from '../modules/index';
import { DashboardComponent } from '../modules/index';
import { PrescriptionNoticesComponent } from '../modules/index';
// import { EncountersComponent } from "../modules/encounters/encounters.component";
import { ScheduleComponent } from "../modules/schedule/schedule.component";
import { AdminComponent } from '../modules/index';
@NgModule({
imports: [
RouterModule.forRoot([
{
path: '',
component: DashboardComponent,
data: { label: 'Dashboard' }
},
{
path: 'encounters',
// component: EncountersComponent,
loadChildren: 'production/modules/encounters/encounters.module#EncountersModule',
data: { label: 'Encounters' }
},
{
path: 'admin',
component: AdminComponent,
data: { label: 'Admin' }
}
])
],
exports: [
RouterModule
]
})
export class AppRoutingModule {}
// const appRoutes: Routes = [
// {
// path: 'imaging',
// component: ImagingComponent,
// data: { label: 'Imaging' }
// },
// {
// path: '',
// component: DashboardComponent,
// data: { label: 'Dashboard' }
// },
// {
// path: 'prescription_notices',
// component: PrescriptionNoticesComponent,
// data: { label: 'Prescription Notices' }
// },
// {
// path: 'encounters',
// component: EncountersComponent,
// data: { label: 'Encounters' }
// },
// {
// path: 'schedule',
// component: ScheduleComponent,
// data: { label: 'Schedule' }
// },
// {
// path: 'admin',
// component: AdminComponent,
// data: { label: 'Admin' }
// }
// ];
//
// export const appRoutingProviders: any = [
//
// ];
//
// export const routing: ModuleWithProviders = RouterModule.forRoot(appRoutes);
angular typescript angular2-routing
angular typescript angular2-routing
edited Oct 19 '16 at 13:39
Velidan
asked Oct 19 '16 at 13:05
VelidanVelidan
1,40921634
1,40921634
1
probably it because I didn't have any routing for my encounters.module
– Velidan
Oct 19 '16 at 14:01
Possibly try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away. Although I had all of my comments at the top of my code so I am not sure if that applies here.
– Frank
Oct 19 '16 at 19:20
Thanks Frank for your help!
– Velidan
Oct 20 '16 at 11:37
Glad that worked! I posted the answer so people can find it easier. That same problem had me stumped for almost a whole day when I was updating.
– Frank
Oct 20 '16 at 18:03
add a comment |
1
probably it because I didn't have any routing for my encounters.module
– Velidan
Oct 19 '16 at 14:01
Possibly try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away. Although I had all of my comments at the top of my code so I am not sure if that applies here.
– Frank
Oct 19 '16 at 19:20
Thanks Frank for your help!
– Velidan
Oct 20 '16 at 11:37
Glad that worked! I posted the answer so people can find it easier. That same problem had me stumped for almost a whole day when I was updating.
– Frank
Oct 20 '16 at 18:03
1
1
probably it because I didn't have any routing for my encounters.module
– Velidan
Oct 19 '16 at 14:01
probably it because I didn't have any routing for my encounters.module
– Velidan
Oct 19 '16 at 14:01
Possibly try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away. Although I had all of my comments at the top of my code so I am not sure if that applies here.
– Frank
Oct 19 '16 at 19:20
Possibly try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away. Although I had all of my comments at the top of my code so I am not sure if that applies here.
– Frank
Oct 19 '16 at 19:20
Thanks Frank for your help!
– Velidan
Oct 20 '16 at 11:37
Thanks Frank for your help!
– Velidan
Oct 20 '16 at 11:37
Glad that worked! I posted the answer so people can find it easier. That same problem had me stumped for almost a whole day when I was updating.
– Frank
Oct 20 '16 at 18:03
Glad that worked! I posted the answer so people can find it easier. That same problem had me stumped for almost a whole day when I was updating.
– Frank
Oct 20 '16 at 18:03
add a comment |
4 Answers
4
active
oldest
votes
loadChildren needs to reference module with routing
By assigning a value to loadChildren property inside a route, you have to reference a module, which has a routing system implemented. In other words reference only a module that imports RoutingModule and configures it with forChild(routes) method.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
export const encountersModuleRoutes: Routes = [
/* configure routes here */
];
@NgModule({
imports: [ SharedModule, RouterModule.forChild(encountersModuleRoutes) ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
1
go f'n figure!!
– LastTribunal
Jul 6 '17 at 23:08
6
God bless you. Have a blissful life my friend. I was trying to find the error from 1 hour almost and you saved myn
numbers of hours :D
– Vikas Bansal
Oct 21 '17 at 17:17
Thanks , I was stuck it helps me cheers :)
– Ashutosh Jha
Nov 9 '17 at 10:35
Yep, this is definitely a module import/loading issue, also be careful with order of imports in the referenced module, I had random and annoying issues with the placement of the CommonModule.
– Michael Dausmann
Feb 22 '18 at 22:16
thanks, i got this error when i accidentally remove the import for my routing module. had no idea what it was about :)
– Graham Fowles
Jun 22 '18 at 12:53
|
show 2 more comments
I am not sure because it is not explicitly mentioned in the documentation (2.4.2), but from the examples in the "Angular Modules" and "Routing & Navigation" guides, I have induced the following pattern:
- The lazy module should have his own routing module.
- The routes array defined in the "lazy-routing.module" should have a single element; the
path
property of that element should be an empty string; thecomponent
property should be defined (necessary when the lazy module provides any service in order to injection works well) and the template of the referenced component should have an element with the<router-outlet>
directive. This route usually has achildren
property. - The value of the
path
property of the lazy route defined in the "app-routing.module" ("lazyModulePrefix" in my example) would be the prefix of all the paths defined in the ".lazy-routing.module".
For example:
///////////// app-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LoginComponent } from './login/login.component';
import { PageNotFoundComponent } from './page-not-found.component';
const appRoutes: Routes = [
{ path: 'login', component: LoginComponent },
{ path: 'lazyModulePrefix', loadChildren: 'app/lazyModulePath/lazy.module#LazyModule' }, //
{ path: '', redirectTo: 'login', pathMatch: 'full'},
{ path: '**', component: PageNotFoundComponent },
];
@NgModule({
imports: [RouterModule.forRoot(appRoutes)],
exports: [RouterModule]
})
export class AppRoutingModule {}
.
///////////// lazy-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
const lazyModuleRoutes: Routes = [ // IMPORTANT: this array should contain a single route element with an empty path. And optionally, as many children as desired.
{ path: '',
component: LazyModuleRootComponent, // the `component` property is necessary when the lazy module provides some service in order to injection work well. If defined, the referenced component's template should have an element with the `<router-outlet>` directive.
children: [
{ path: '', component: LazyModuleHomeComponent }, // this component has no diference with the other children except for the shorter route.
{ path: 'somePath1', component: AComponentDeclaredInTheLazyModule1 },
{ path: 'somePath2', component: AComponentDeclaredInTheLazyModule2 },
] }
];
@NgModule({
imports: [RouterModule.forChild(lazyModuleRoutes)],
exports: [RouterModule]
})
export class LazyRoutingModule { }
.
//////////////////// lazy.module.ts ////////////////////
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { SharedModule } from '../shared/shared.module';
import { LazyRoutingModule } from './lazy-routing.module';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
@NgModule({
imports: [
CommonModule,
SharedModule,
LazyRoutingModule,
],
declarations: [
LazyModuleRootComponent,
LazyModuleHomeComponent,
AComponentDeclaredInTheLazyModule1,
AComponentDeclaredInTheLazyModule2,
]
})
export class LazyModule { }
.
//////////////// lazy-module-root.component.ts //////////////////
import { Component } from '@angular/core';
@Component({
template: '<router-outlet></router-outlet>'
})
export class LazyModueRootComponent { }
With the above code, the routes mapping would be:
http://host/login -> LoginComponent
http://host/lazyModulePrefix -> LazyModuleHomeComponent
http://host/lazyModulePrefix/somePath1 -> AComponentDeclaredInTheLazyModule1
http://host/lazyModulePrefix/somePath2 -> AComponentDeclaredInTheLazyModule2
http://host/anythingElse -> PageNotFoundComponent
add a comment |
Try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away.
I did not work for me
– sudheer KB
Dec 16 '16 at 8:38
add a comment |
I was facing same issue and I had everything correct. The following worked for me
- Stop the angular server
- Start the server again with
ng serve
It started working again spontaneously. First make sure you have everything correct as mentioned in other answers and then try this.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f40132255%2frangeerror-maximum-call-stack-size-exceeded-lazy-routing-angular-2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
loadChildren needs to reference module with routing
By assigning a value to loadChildren property inside a route, you have to reference a module, which has a routing system implemented. In other words reference only a module that imports RoutingModule and configures it with forChild(routes) method.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
export const encountersModuleRoutes: Routes = [
/* configure routes here */
];
@NgModule({
imports: [ SharedModule, RouterModule.forChild(encountersModuleRoutes) ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
1
go f'n figure!!
– LastTribunal
Jul 6 '17 at 23:08
6
God bless you. Have a blissful life my friend. I was trying to find the error from 1 hour almost and you saved myn
numbers of hours :D
– Vikas Bansal
Oct 21 '17 at 17:17
Thanks , I was stuck it helps me cheers :)
– Ashutosh Jha
Nov 9 '17 at 10:35
Yep, this is definitely a module import/loading issue, also be careful with order of imports in the referenced module, I had random and annoying issues with the placement of the CommonModule.
– Michael Dausmann
Feb 22 '18 at 22:16
thanks, i got this error when i accidentally remove the import for my routing module. had no idea what it was about :)
– Graham Fowles
Jun 22 '18 at 12:53
|
show 2 more comments
loadChildren needs to reference module with routing
By assigning a value to loadChildren property inside a route, you have to reference a module, which has a routing system implemented. In other words reference only a module that imports RoutingModule and configures it with forChild(routes) method.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
export const encountersModuleRoutes: Routes = [
/* configure routes here */
];
@NgModule({
imports: [ SharedModule, RouterModule.forChild(encountersModuleRoutes) ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
1
go f'n figure!!
– LastTribunal
Jul 6 '17 at 23:08
6
God bless you. Have a blissful life my friend. I was trying to find the error from 1 hour almost and you saved myn
numbers of hours :D
– Vikas Bansal
Oct 21 '17 at 17:17
Thanks , I was stuck it helps me cheers :)
– Ashutosh Jha
Nov 9 '17 at 10:35
Yep, this is definitely a module import/loading issue, also be careful with order of imports in the referenced module, I had random and annoying issues with the placement of the CommonModule.
– Michael Dausmann
Feb 22 '18 at 22:16
thanks, i got this error when i accidentally remove the import for my routing module. had no idea what it was about :)
– Graham Fowles
Jun 22 '18 at 12:53
|
show 2 more comments
loadChildren needs to reference module with routing
By assigning a value to loadChildren property inside a route, you have to reference a module, which has a routing system implemented. In other words reference only a module that imports RoutingModule and configures it with forChild(routes) method.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
export const encountersModuleRoutes: Routes = [
/* configure routes here */
];
@NgModule({
imports: [ SharedModule, RouterModule.forChild(encountersModuleRoutes) ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
loadChildren needs to reference module with routing
By assigning a value to loadChildren property inside a route, you have to reference a module, which has a routing system implemented. In other words reference only a module that imports RoutingModule and configures it with forChild(routes) method.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
// import { CommonModule } from '@angular/common';
/* --------------- !System modules --------------- */
import { SharedModule } from 'sharedModule'; //There is a lot of shared components/directives/pipes (over 60) and it re-exports CommonModule so I can't avoid it
/* --------------- !App outer modules --------------- */
import { EncountersComponent } from './encounters.component';
// import { PassCodeComponent } from '../../shared/components/passcode/passcode.component';
export const encountersModuleRoutes: Routes = [
/* configure routes here */
];
@NgModule({
imports: [ SharedModule, RouterModule.forChild(encountersModuleRoutes) ],
declarations: [ EncountersComponent],
exports: [ EncountersComponent ],
})
export class EncountersModule { }
answered Dec 20 '16 at 10:23


Marek DulowskiMarek Dulowski
1,036514
1,036514
1
go f'n figure!!
– LastTribunal
Jul 6 '17 at 23:08
6
God bless you. Have a blissful life my friend. I was trying to find the error from 1 hour almost and you saved myn
numbers of hours :D
– Vikas Bansal
Oct 21 '17 at 17:17
Thanks , I was stuck it helps me cheers :)
– Ashutosh Jha
Nov 9 '17 at 10:35
Yep, this is definitely a module import/loading issue, also be careful with order of imports in the referenced module, I had random and annoying issues with the placement of the CommonModule.
– Michael Dausmann
Feb 22 '18 at 22:16
thanks, i got this error when i accidentally remove the import for my routing module. had no idea what it was about :)
– Graham Fowles
Jun 22 '18 at 12:53
|
show 2 more comments
1
go f'n figure!!
– LastTribunal
Jul 6 '17 at 23:08
6
God bless you. Have a blissful life my friend. I was trying to find the error from 1 hour almost and you saved myn
numbers of hours :D
– Vikas Bansal
Oct 21 '17 at 17:17
Thanks , I was stuck it helps me cheers :)
– Ashutosh Jha
Nov 9 '17 at 10:35
Yep, this is definitely a module import/loading issue, also be careful with order of imports in the referenced module, I had random and annoying issues with the placement of the CommonModule.
– Michael Dausmann
Feb 22 '18 at 22:16
thanks, i got this error when i accidentally remove the import for my routing module. had no idea what it was about :)
– Graham Fowles
Jun 22 '18 at 12:53
1
1
go f'n figure!!
– LastTribunal
Jul 6 '17 at 23:08
go f'n figure!!
– LastTribunal
Jul 6 '17 at 23:08
6
6
God bless you. Have a blissful life my friend. I was trying to find the error from 1 hour almost and you saved my
n
numbers of hours :D– Vikas Bansal
Oct 21 '17 at 17:17
God bless you. Have a blissful life my friend. I was trying to find the error from 1 hour almost and you saved my
n
numbers of hours :D– Vikas Bansal
Oct 21 '17 at 17:17
Thanks , I was stuck it helps me cheers :)
– Ashutosh Jha
Nov 9 '17 at 10:35
Thanks , I was stuck it helps me cheers :)
– Ashutosh Jha
Nov 9 '17 at 10:35
Yep, this is definitely a module import/loading issue, also be careful with order of imports in the referenced module, I had random and annoying issues with the placement of the CommonModule.
– Michael Dausmann
Feb 22 '18 at 22:16
Yep, this is definitely a module import/loading issue, also be careful with order of imports in the referenced module, I had random and annoying issues with the placement of the CommonModule.
– Michael Dausmann
Feb 22 '18 at 22:16
thanks, i got this error when i accidentally remove the import for my routing module. had no idea what it was about :)
– Graham Fowles
Jun 22 '18 at 12:53
thanks, i got this error when i accidentally remove the import for my routing module. had no idea what it was about :)
– Graham Fowles
Jun 22 '18 at 12:53
|
show 2 more comments
I am not sure because it is not explicitly mentioned in the documentation (2.4.2), but from the examples in the "Angular Modules" and "Routing & Navigation" guides, I have induced the following pattern:
- The lazy module should have his own routing module.
- The routes array defined in the "lazy-routing.module" should have a single element; the
path
property of that element should be an empty string; thecomponent
property should be defined (necessary when the lazy module provides any service in order to injection works well) and the template of the referenced component should have an element with the<router-outlet>
directive. This route usually has achildren
property. - The value of the
path
property of the lazy route defined in the "app-routing.module" ("lazyModulePrefix" in my example) would be the prefix of all the paths defined in the ".lazy-routing.module".
For example:
///////////// app-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LoginComponent } from './login/login.component';
import { PageNotFoundComponent } from './page-not-found.component';
const appRoutes: Routes = [
{ path: 'login', component: LoginComponent },
{ path: 'lazyModulePrefix', loadChildren: 'app/lazyModulePath/lazy.module#LazyModule' }, //
{ path: '', redirectTo: 'login', pathMatch: 'full'},
{ path: '**', component: PageNotFoundComponent },
];
@NgModule({
imports: [RouterModule.forRoot(appRoutes)],
exports: [RouterModule]
})
export class AppRoutingModule {}
.
///////////// lazy-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
const lazyModuleRoutes: Routes = [ // IMPORTANT: this array should contain a single route element with an empty path. And optionally, as many children as desired.
{ path: '',
component: LazyModuleRootComponent, // the `component` property is necessary when the lazy module provides some service in order to injection work well. If defined, the referenced component's template should have an element with the `<router-outlet>` directive.
children: [
{ path: '', component: LazyModuleHomeComponent }, // this component has no diference with the other children except for the shorter route.
{ path: 'somePath1', component: AComponentDeclaredInTheLazyModule1 },
{ path: 'somePath2', component: AComponentDeclaredInTheLazyModule2 },
] }
];
@NgModule({
imports: [RouterModule.forChild(lazyModuleRoutes)],
exports: [RouterModule]
})
export class LazyRoutingModule { }
.
//////////////////// lazy.module.ts ////////////////////
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { SharedModule } from '../shared/shared.module';
import { LazyRoutingModule } from './lazy-routing.module';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
@NgModule({
imports: [
CommonModule,
SharedModule,
LazyRoutingModule,
],
declarations: [
LazyModuleRootComponent,
LazyModuleHomeComponent,
AComponentDeclaredInTheLazyModule1,
AComponentDeclaredInTheLazyModule2,
]
})
export class LazyModule { }
.
//////////////// lazy-module-root.component.ts //////////////////
import { Component } from '@angular/core';
@Component({
template: '<router-outlet></router-outlet>'
})
export class LazyModueRootComponent { }
With the above code, the routes mapping would be:
http://host/login -> LoginComponent
http://host/lazyModulePrefix -> LazyModuleHomeComponent
http://host/lazyModulePrefix/somePath1 -> AComponentDeclaredInTheLazyModule1
http://host/lazyModulePrefix/somePath2 -> AComponentDeclaredInTheLazyModule2
http://host/anythingElse -> PageNotFoundComponent
add a comment |
I am not sure because it is not explicitly mentioned in the documentation (2.4.2), but from the examples in the "Angular Modules" and "Routing & Navigation" guides, I have induced the following pattern:
- The lazy module should have his own routing module.
- The routes array defined in the "lazy-routing.module" should have a single element; the
path
property of that element should be an empty string; thecomponent
property should be defined (necessary when the lazy module provides any service in order to injection works well) and the template of the referenced component should have an element with the<router-outlet>
directive. This route usually has achildren
property. - The value of the
path
property of the lazy route defined in the "app-routing.module" ("lazyModulePrefix" in my example) would be the prefix of all the paths defined in the ".lazy-routing.module".
For example:
///////////// app-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LoginComponent } from './login/login.component';
import { PageNotFoundComponent } from './page-not-found.component';
const appRoutes: Routes = [
{ path: 'login', component: LoginComponent },
{ path: 'lazyModulePrefix', loadChildren: 'app/lazyModulePath/lazy.module#LazyModule' }, //
{ path: '', redirectTo: 'login', pathMatch: 'full'},
{ path: '**', component: PageNotFoundComponent },
];
@NgModule({
imports: [RouterModule.forRoot(appRoutes)],
exports: [RouterModule]
})
export class AppRoutingModule {}
.
///////////// lazy-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
const lazyModuleRoutes: Routes = [ // IMPORTANT: this array should contain a single route element with an empty path. And optionally, as many children as desired.
{ path: '',
component: LazyModuleRootComponent, // the `component` property is necessary when the lazy module provides some service in order to injection work well. If defined, the referenced component's template should have an element with the `<router-outlet>` directive.
children: [
{ path: '', component: LazyModuleHomeComponent }, // this component has no diference with the other children except for the shorter route.
{ path: 'somePath1', component: AComponentDeclaredInTheLazyModule1 },
{ path: 'somePath2', component: AComponentDeclaredInTheLazyModule2 },
] }
];
@NgModule({
imports: [RouterModule.forChild(lazyModuleRoutes)],
exports: [RouterModule]
})
export class LazyRoutingModule { }
.
//////////////////// lazy.module.ts ////////////////////
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { SharedModule } from '../shared/shared.module';
import { LazyRoutingModule } from './lazy-routing.module';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
@NgModule({
imports: [
CommonModule,
SharedModule,
LazyRoutingModule,
],
declarations: [
LazyModuleRootComponent,
LazyModuleHomeComponent,
AComponentDeclaredInTheLazyModule1,
AComponentDeclaredInTheLazyModule2,
]
})
export class LazyModule { }
.
//////////////// lazy-module-root.component.ts //////////////////
import { Component } from '@angular/core';
@Component({
template: '<router-outlet></router-outlet>'
})
export class LazyModueRootComponent { }
With the above code, the routes mapping would be:
http://host/login -> LoginComponent
http://host/lazyModulePrefix -> LazyModuleHomeComponent
http://host/lazyModulePrefix/somePath1 -> AComponentDeclaredInTheLazyModule1
http://host/lazyModulePrefix/somePath2 -> AComponentDeclaredInTheLazyModule2
http://host/anythingElse -> PageNotFoundComponent
add a comment |
I am not sure because it is not explicitly mentioned in the documentation (2.4.2), but from the examples in the "Angular Modules" and "Routing & Navigation" guides, I have induced the following pattern:
- The lazy module should have his own routing module.
- The routes array defined in the "lazy-routing.module" should have a single element; the
path
property of that element should be an empty string; thecomponent
property should be defined (necessary when the lazy module provides any service in order to injection works well) and the template of the referenced component should have an element with the<router-outlet>
directive. This route usually has achildren
property. - The value of the
path
property of the lazy route defined in the "app-routing.module" ("lazyModulePrefix" in my example) would be the prefix of all the paths defined in the ".lazy-routing.module".
For example:
///////////// app-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LoginComponent } from './login/login.component';
import { PageNotFoundComponent } from './page-not-found.component';
const appRoutes: Routes = [
{ path: 'login', component: LoginComponent },
{ path: 'lazyModulePrefix', loadChildren: 'app/lazyModulePath/lazy.module#LazyModule' }, //
{ path: '', redirectTo: 'login', pathMatch: 'full'},
{ path: '**', component: PageNotFoundComponent },
];
@NgModule({
imports: [RouterModule.forRoot(appRoutes)],
exports: [RouterModule]
})
export class AppRoutingModule {}
.
///////////// lazy-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
const lazyModuleRoutes: Routes = [ // IMPORTANT: this array should contain a single route element with an empty path. And optionally, as many children as desired.
{ path: '',
component: LazyModuleRootComponent, // the `component` property is necessary when the lazy module provides some service in order to injection work well. If defined, the referenced component's template should have an element with the `<router-outlet>` directive.
children: [
{ path: '', component: LazyModuleHomeComponent }, // this component has no diference with the other children except for the shorter route.
{ path: 'somePath1', component: AComponentDeclaredInTheLazyModule1 },
{ path: 'somePath2', component: AComponentDeclaredInTheLazyModule2 },
] }
];
@NgModule({
imports: [RouterModule.forChild(lazyModuleRoutes)],
exports: [RouterModule]
})
export class LazyRoutingModule { }
.
//////////////////// lazy.module.ts ////////////////////
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { SharedModule } from '../shared/shared.module';
import { LazyRoutingModule } from './lazy-routing.module';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
@NgModule({
imports: [
CommonModule,
SharedModule,
LazyRoutingModule,
],
declarations: [
LazyModuleRootComponent,
LazyModuleHomeComponent,
AComponentDeclaredInTheLazyModule1,
AComponentDeclaredInTheLazyModule2,
]
})
export class LazyModule { }
.
//////////////// lazy-module-root.component.ts //////////////////
import { Component } from '@angular/core';
@Component({
template: '<router-outlet></router-outlet>'
})
export class LazyModueRootComponent { }
With the above code, the routes mapping would be:
http://host/login -> LoginComponent
http://host/lazyModulePrefix -> LazyModuleHomeComponent
http://host/lazyModulePrefix/somePath1 -> AComponentDeclaredInTheLazyModule1
http://host/lazyModulePrefix/somePath2 -> AComponentDeclaredInTheLazyModule2
http://host/anythingElse -> PageNotFoundComponent
I am not sure because it is not explicitly mentioned in the documentation (2.4.2), but from the examples in the "Angular Modules" and "Routing & Navigation" guides, I have induced the following pattern:
- The lazy module should have his own routing module.
- The routes array defined in the "lazy-routing.module" should have a single element; the
path
property of that element should be an empty string; thecomponent
property should be defined (necessary when the lazy module provides any service in order to injection works well) and the template of the referenced component should have an element with the<router-outlet>
directive. This route usually has achildren
property. - The value of the
path
property of the lazy route defined in the "app-routing.module" ("lazyModulePrefix" in my example) would be the prefix of all the paths defined in the ".lazy-routing.module".
For example:
///////////// app-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LoginComponent } from './login/login.component';
import { PageNotFoundComponent } from './page-not-found.component';
const appRoutes: Routes = [
{ path: 'login', component: LoginComponent },
{ path: 'lazyModulePrefix', loadChildren: 'app/lazyModulePath/lazy.module#LazyModule' }, //
{ path: '', redirectTo: 'login', pathMatch: 'full'},
{ path: '**', component: PageNotFoundComponent },
];
@NgModule({
imports: [RouterModule.forRoot(appRoutes)],
exports: [RouterModule]
})
export class AppRoutingModule {}
.
///////////// lazy-routing.module.ts /////////////////////
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
const lazyModuleRoutes: Routes = [ // IMPORTANT: this array should contain a single route element with an empty path. And optionally, as many children as desired.
{ path: '',
component: LazyModuleRootComponent, // the `component` property is necessary when the lazy module provides some service in order to injection work well. If defined, the referenced component's template should have an element with the `<router-outlet>` directive.
children: [
{ path: '', component: LazyModuleHomeComponent }, // this component has no diference with the other children except for the shorter route.
{ path: 'somePath1', component: AComponentDeclaredInTheLazyModule1 },
{ path: 'somePath2', component: AComponentDeclaredInTheLazyModule2 },
] }
];
@NgModule({
imports: [RouterModule.forChild(lazyModuleRoutes)],
exports: [RouterModule]
})
export class LazyRoutingModule { }
.
//////////////////// lazy.module.ts ////////////////////
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { SharedModule } from '../shared/shared.module';
import { LazyRoutingModule } from './lazy-routing.module';
import { LazyModuleRootComponent } from './lazy-module-root.component';
import { LazyModuleHomeComponent } from './lazy-module-home.component';
import { AComponentDeclaredInTheLazyModule1 } from './a-component-declared-in-the-lazy-module-1.component';
import { AComponentDeclaredInTheLazyModule2 } from './a-component-declared-in-the-lazy-module-2.component';
@NgModule({
imports: [
CommonModule,
SharedModule,
LazyRoutingModule,
],
declarations: [
LazyModuleRootComponent,
LazyModuleHomeComponent,
AComponentDeclaredInTheLazyModule1,
AComponentDeclaredInTheLazyModule2,
]
})
export class LazyModule { }
.
//////////////// lazy-module-root.component.ts //////////////////
import { Component } from '@angular/core';
@Component({
template: '<router-outlet></router-outlet>'
})
export class LazyModueRootComponent { }
With the above code, the routes mapping would be:
http://host/login -> LoginComponent
http://host/lazyModulePrefix -> LazyModuleHomeComponent
http://host/lazyModulePrefix/somePath1 -> AComponentDeclaredInTheLazyModule1
http://host/lazyModulePrefix/somePath2 -> AComponentDeclaredInTheLazyModule2
http://host/anythingElse -> PageNotFoundComponent
edited Aug 5 '17 at 8:06
answered Jan 11 '17 at 7:24
ReadrenReadren
445517
445517
add a comment |
add a comment |
Try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away.
I did not work for me
– sudheer KB
Dec 16 '16 at 8:38
add a comment |
Try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away.
I did not work for me
– sudheer KB
Dec 16 '16 at 8:38
add a comment |
Try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away.
Try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away.
answered Oct 20 '16 at 18:02


FrankFrank
846
846
I did not work for me
– sudheer KB
Dec 16 '16 at 8:38
add a comment |
I did not work for me
– sudheer KB
Dec 16 '16 at 8:38
I did not work for me
– sudheer KB
Dec 16 '16 at 8:38
I did not work for me
– sudheer KB
Dec 16 '16 at 8:38
add a comment |
I was facing same issue and I had everything correct. The following worked for me
- Stop the angular server
- Start the server again with
ng serve
It started working again spontaneously. First make sure you have everything correct as mentioned in other answers and then try this.
add a comment |
I was facing same issue and I had everything correct. The following worked for me
- Stop the angular server
- Start the server again with
ng serve
It started working again spontaneously. First make sure you have everything correct as mentioned in other answers and then try this.
add a comment |
I was facing same issue and I had everything correct. The following worked for me
- Stop the angular server
- Start the server again with
ng serve
It started working again spontaneously. First make sure you have everything correct as mentioned in other answers and then try this.
I was facing same issue and I had everything correct. The following worked for me
- Stop the angular server
- Start the server again with
ng serve
It started working again spontaneously. First make sure you have everything correct as mentioned in other answers and then try this.
answered Dec 30 '18 at 14:33


Hari DasHari Das
3,07842536
3,07842536
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f40132255%2frangeerror-maximum-call-stack-size-exceeded-lazy-routing-angular-2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4uWbh9 OmGCzCdvuIKyJhje,RD6iSbj1,OQ,Gcs,sT8J6ZXxIBfTA2lTu5g3nU rbbTGRX2jg51Yg,RQJTRHxdDJu Ct12X7qp,S7e,zb
1
probably it because I didn't have any routing for my encounters.module
– Velidan
Oct 19 '16 at 14:01
Possibly try removing comments. When I updated my router to current in the application I was working on I commented a bunch of stuff out from the old router because I didn't want to lose it. After removing comments some of the strange errors went away. Although I had all of my comments at the top of my code so I am not sure if that applies here.
– Frank
Oct 19 '16 at 19:20
Thanks Frank for your help!
– Velidan
Oct 20 '16 at 11:37
Glad that worked! I posted the answer so people can find it easier. That same problem had me stumped for almost a whole day when I was updating.
– Frank
Oct 20 '16 at 18:03