Select rows of a dataframe based on another dataframe in Python
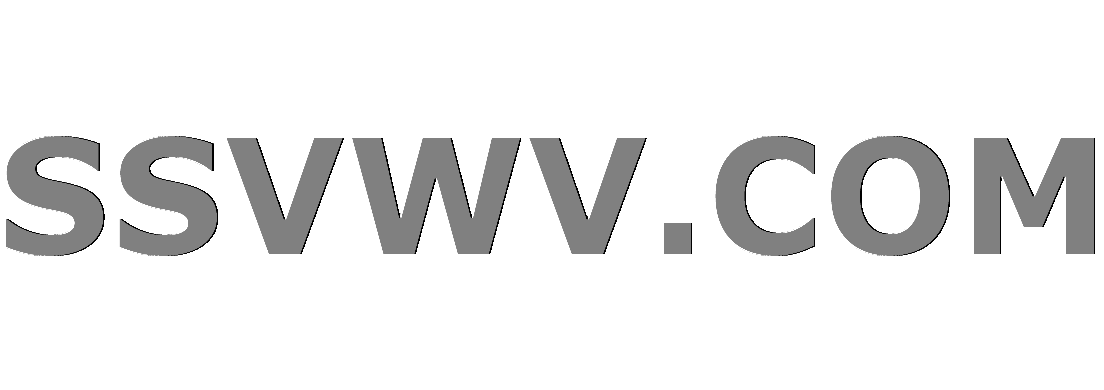
Multi tool use
I have the following dataframe:
import pandas as pd
import numpy as np
df1 = pd.DataFrame({'A': 'foo bar foo bar foo bar foo foo'.split(),
'B': 'one one two three two two one three'.split(),
'C': np.arange(8), 'D': np.arange(8) * 2})
print(df1)
A B C D
0 foo one 0 0
1 bar one 1 2
2 foo two 2 4
3 bar three 3 6
4 foo two 4 8
5 bar two 5 10
6 foo one 6 12
7 foo three 7 14
I hope to select rows in df1 by the df2 as follows:
df2 = pd.DataFrame({'A': 'foo bar'.split(),
'B': 'one two'.split()
})
print(df2)
A B
0 foo one
1 bar two
Here is what I have tried in Python, but I just wonder if there is another method. Thanks.
df = df1.merge(df2, on=['A','B'])
print(df)
Here is the outputs expected.
A B C D
0 foo one 0 0
1 bar two 5 10
2 foo one 6 12
Using pandas to select rows using two different columns from dataframe?
Select Columns of a DataFrame based on another DataFrame
python pandas dataframe
|
show 2 more comments
I have the following dataframe:
import pandas as pd
import numpy as np
df1 = pd.DataFrame({'A': 'foo bar foo bar foo bar foo foo'.split(),
'B': 'one one two three two two one three'.split(),
'C': np.arange(8), 'D': np.arange(8) * 2})
print(df1)
A B C D
0 foo one 0 0
1 bar one 1 2
2 foo two 2 4
3 bar three 3 6
4 foo two 4 8
5 bar two 5 10
6 foo one 6 12
7 foo three 7 14
I hope to select rows in df1 by the df2 as follows:
df2 = pd.DataFrame({'A': 'foo bar'.split(),
'B': 'one two'.split()
})
print(df2)
A B
0 foo one
1 bar two
Here is what I have tried in Python, but I just wonder if there is another method. Thanks.
df = df1.merge(df2, on=['A','B'])
print(df)
Here is the outputs expected.
A B C D
0 foo one 0 0
1 bar two 5 10
2 foo one 6 12
Using pandas to select rows using two different columns from dataframe?
Select Columns of a DataFrame based on another DataFrame
python pandas dataframe
what's wrong with current method?
– meW
Jan 2 at 12:22
3
In my opinion it is good solution.
– jezrael
Jan 2 at 12:23
I think maybe we can try with loc, iloc or isin function, what do you think?
– ahbon
Jan 2 at 12:25
stackoverflow.com/questions/13937022/…
– ahbon
Jan 2 at 12:25
1
Sorry maybe i ask a silly question.
– ahbon
Jan 2 at 12:26
|
show 2 more comments
I have the following dataframe:
import pandas as pd
import numpy as np
df1 = pd.DataFrame({'A': 'foo bar foo bar foo bar foo foo'.split(),
'B': 'one one two three two two one three'.split(),
'C': np.arange(8), 'D': np.arange(8) * 2})
print(df1)
A B C D
0 foo one 0 0
1 bar one 1 2
2 foo two 2 4
3 bar three 3 6
4 foo two 4 8
5 bar two 5 10
6 foo one 6 12
7 foo three 7 14
I hope to select rows in df1 by the df2 as follows:
df2 = pd.DataFrame({'A': 'foo bar'.split(),
'B': 'one two'.split()
})
print(df2)
A B
0 foo one
1 bar two
Here is what I have tried in Python, but I just wonder if there is another method. Thanks.
df = df1.merge(df2, on=['A','B'])
print(df)
Here is the outputs expected.
A B C D
0 foo one 0 0
1 bar two 5 10
2 foo one 6 12
Using pandas to select rows using two different columns from dataframe?
Select Columns of a DataFrame based on another DataFrame
python pandas dataframe
I have the following dataframe:
import pandas as pd
import numpy as np
df1 = pd.DataFrame({'A': 'foo bar foo bar foo bar foo foo'.split(),
'B': 'one one two three two two one three'.split(),
'C': np.arange(8), 'D': np.arange(8) * 2})
print(df1)
A B C D
0 foo one 0 0
1 bar one 1 2
2 foo two 2 4
3 bar three 3 6
4 foo two 4 8
5 bar two 5 10
6 foo one 6 12
7 foo three 7 14
I hope to select rows in df1 by the df2 as follows:
df2 = pd.DataFrame({'A': 'foo bar'.split(),
'B': 'one two'.split()
})
print(df2)
A B
0 foo one
1 bar two
Here is what I have tried in Python, but I just wonder if there is another method. Thanks.
df = df1.merge(df2, on=['A','B'])
print(df)
Here is the outputs expected.
A B C D
0 foo one 0 0
1 bar two 5 10
2 foo one 6 12
Using pandas to select rows using two different columns from dataframe?
Select Columns of a DataFrame based on another DataFrame
python pandas dataframe
python pandas dataframe
asked Jan 2 at 12:20


ahbonahbon
513211
513211
what's wrong with current method?
– meW
Jan 2 at 12:22
3
In my opinion it is good solution.
– jezrael
Jan 2 at 12:23
I think maybe we can try with loc, iloc or isin function, what do you think?
– ahbon
Jan 2 at 12:25
stackoverflow.com/questions/13937022/…
– ahbon
Jan 2 at 12:25
1
Sorry maybe i ask a silly question.
– ahbon
Jan 2 at 12:26
|
show 2 more comments
what's wrong with current method?
– meW
Jan 2 at 12:22
3
In my opinion it is good solution.
– jezrael
Jan 2 at 12:23
I think maybe we can try with loc, iloc or isin function, what do you think?
– ahbon
Jan 2 at 12:25
stackoverflow.com/questions/13937022/…
– ahbon
Jan 2 at 12:25
1
Sorry maybe i ask a silly question.
– ahbon
Jan 2 at 12:26
what's wrong with current method?
– meW
Jan 2 at 12:22
what's wrong with current method?
– meW
Jan 2 at 12:22
3
3
In my opinion it is good solution.
– jezrael
Jan 2 at 12:23
In my opinion it is good solution.
– jezrael
Jan 2 at 12:23
I think maybe we can try with loc, iloc or isin function, what do you think?
– ahbon
Jan 2 at 12:25
I think maybe we can try with loc, iloc or isin function, what do you think?
– ahbon
Jan 2 at 12:25
stackoverflow.com/questions/13937022/…
– ahbon
Jan 2 at 12:25
stackoverflow.com/questions/13937022/…
– ahbon
Jan 2 at 12:25
1
1
Sorry maybe i ask a silly question.
– ahbon
Jan 2 at 12:26
Sorry maybe i ask a silly question.
– ahbon
Jan 2 at 12:26
|
show 2 more comments
1 Answer
1
active
oldest
votes
Simpliest is use merge
with inner join.
Another solution with filtering:
arr = [np.array([df1[k] == v for k, v in x.items()]).all(axis=0) for x in df2.to_dict('r')]
df = df1[np.array(arr).any(axis=0)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Or create MultiIndex
and filter with Index.isin
:
df = df1[df1.set_index(['A','B']).index.isin(df2.set_index(['A','B']).index)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Sincere thanks and great respect to Python masters who give comments and helps here!! ;)
– ahbon
Jan 2 at 12:38
1
@ahbon - yes, and super thing is there is a lot of masters of pandas, so a lot of answers are answered ;) Happy coding!
– jezrael
Jan 2 at 12:39
Your second solution is awesome!! Solve problem on one line and it's the answer expected.
– ahbon
Jan 2 at 12:43
@ahbon - yes, it is better :)
– jezrael
Jan 2 at 12:47
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54006298%2fselect-rows-of-a-dataframe-based-on-another-dataframe-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Simpliest is use merge
with inner join.
Another solution with filtering:
arr = [np.array([df1[k] == v for k, v in x.items()]).all(axis=0) for x in df2.to_dict('r')]
df = df1[np.array(arr).any(axis=0)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Or create MultiIndex
and filter with Index.isin
:
df = df1[df1.set_index(['A','B']).index.isin(df2.set_index(['A','B']).index)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Sincere thanks and great respect to Python masters who give comments and helps here!! ;)
– ahbon
Jan 2 at 12:38
1
@ahbon - yes, and super thing is there is a lot of masters of pandas, so a lot of answers are answered ;) Happy coding!
– jezrael
Jan 2 at 12:39
Your second solution is awesome!! Solve problem on one line and it's the answer expected.
– ahbon
Jan 2 at 12:43
@ahbon - yes, it is better :)
– jezrael
Jan 2 at 12:47
add a comment |
Simpliest is use merge
with inner join.
Another solution with filtering:
arr = [np.array([df1[k] == v for k, v in x.items()]).all(axis=0) for x in df2.to_dict('r')]
df = df1[np.array(arr).any(axis=0)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Or create MultiIndex
and filter with Index.isin
:
df = df1[df1.set_index(['A','B']).index.isin(df2.set_index(['A','B']).index)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Sincere thanks and great respect to Python masters who give comments and helps here!! ;)
– ahbon
Jan 2 at 12:38
1
@ahbon - yes, and super thing is there is a lot of masters of pandas, so a lot of answers are answered ;) Happy coding!
– jezrael
Jan 2 at 12:39
Your second solution is awesome!! Solve problem on one line and it's the answer expected.
– ahbon
Jan 2 at 12:43
@ahbon - yes, it is better :)
– jezrael
Jan 2 at 12:47
add a comment |
Simpliest is use merge
with inner join.
Another solution with filtering:
arr = [np.array([df1[k] == v for k, v in x.items()]).all(axis=0) for x in df2.to_dict('r')]
df = df1[np.array(arr).any(axis=0)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Or create MultiIndex
and filter with Index.isin
:
df = df1[df1.set_index(['A','B']).index.isin(df2.set_index(['A','B']).index)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Simpliest is use merge
with inner join.
Another solution with filtering:
arr = [np.array([df1[k] == v for k, v in x.items()]).all(axis=0) for x in df2.to_dict('r')]
df = df1[np.array(arr).any(axis=0)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
Or create MultiIndex
and filter with Index.isin
:
df = df1[df1.set_index(['A','B']).index.isin(df2.set_index(['A','B']).index)]
print(df)
A B C D
0 foo one 0 0
5 bar two 5 10
6 foo one 6 12
answered Jan 2 at 12:34


jezraeljezrael
346k25301374
346k25301374
Sincere thanks and great respect to Python masters who give comments and helps here!! ;)
– ahbon
Jan 2 at 12:38
1
@ahbon - yes, and super thing is there is a lot of masters of pandas, so a lot of answers are answered ;) Happy coding!
– jezrael
Jan 2 at 12:39
Your second solution is awesome!! Solve problem on one line and it's the answer expected.
– ahbon
Jan 2 at 12:43
@ahbon - yes, it is better :)
– jezrael
Jan 2 at 12:47
add a comment |
Sincere thanks and great respect to Python masters who give comments and helps here!! ;)
– ahbon
Jan 2 at 12:38
1
@ahbon - yes, and super thing is there is a lot of masters of pandas, so a lot of answers are answered ;) Happy coding!
– jezrael
Jan 2 at 12:39
Your second solution is awesome!! Solve problem on one line and it's the answer expected.
– ahbon
Jan 2 at 12:43
@ahbon - yes, it is better :)
– jezrael
Jan 2 at 12:47
Sincere thanks and great respect to Python masters who give comments and helps here!! ;)
– ahbon
Jan 2 at 12:38
Sincere thanks and great respect to Python masters who give comments and helps here!! ;)
– ahbon
Jan 2 at 12:38
1
1
@ahbon - yes, and super thing is there is a lot of masters of pandas, so a lot of answers are answered ;) Happy coding!
– jezrael
Jan 2 at 12:39
@ahbon - yes, and super thing is there is a lot of masters of pandas, so a lot of answers are answered ;) Happy coding!
– jezrael
Jan 2 at 12:39
Your second solution is awesome!! Solve problem on one line and it's the answer expected.
– ahbon
Jan 2 at 12:43
Your second solution is awesome!! Solve problem on one line and it's the answer expected.
– ahbon
Jan 2 at 12:43
@ahbon - yes, it is better :)
– jezrael
Jan 2 at 12:47
@ahbon - yes, it is better :)
– jezrael
Jan 2 at 12:47
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54006298%2fselect-rows-of-a-dataframe-based-on-another-dataframe-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OibH4tDLL CS,bNdPS0,LaN9z ySqXru2O2Nf1,US g exbh,fO,9Oe8NG254,DuGdPY3X NbHCv2Pyf dAS
what's wrong with current method?
– meW
Jan 2 at 12:22
3
In my opinion it is good solution.
– jezrael
Jan 2 at 12:23
I think maybe we can try with loc, iloc or isin function, what do you think?
– ahbon
Jan 2 at 12:25
stackoverflow.com/questions/13937022/…
– ahbon
Jan 2 at 12:25
1
Sorry maybe i ask a silly question.
– ahbon
Jan 2 at 12:26