Draw overlapping lines with same transparency
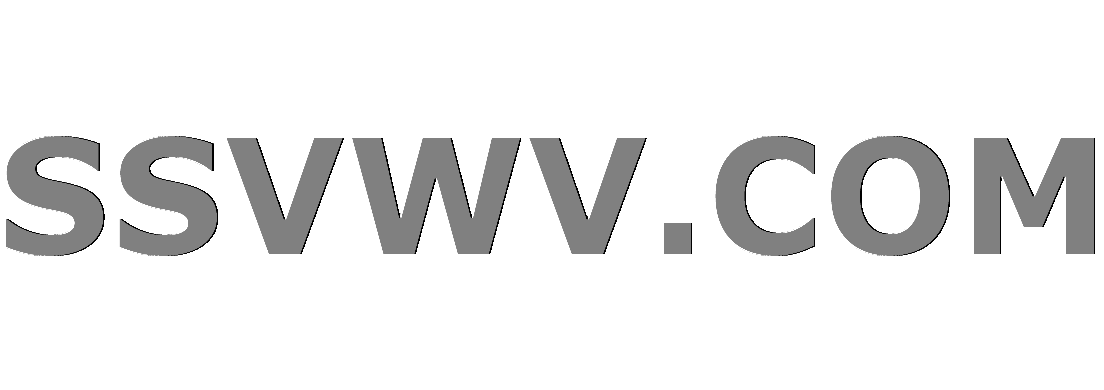
Multi tool use
I have roughly this logic:
Bitmap bmp = ....
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15);
var graphics = Graphics.FromImage(bmp);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
The problem is, that points1 and points2 contain some line segments that are overlaping.
If I draw this lines, the overlapping part have a different color then the rest, due to the blending of the same segments (first, 1 with background and later 2 with already blended 1 with background). Is there a way, how to achieve the effect, that the overlapping parts have the same color as a single non-overlapping segmens?
c# graphics gdi+
add a comment |
I have roughly this logic:
Bitmap bmp = ....
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15);
var graphics = Graphics.FromImage(bmp);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
The problem is, that points1 and points2 contain some line segments that are overlaping.
If I draw this lines, the overlapping part have a different color then the rest, due to the blending of the same segments (first, 1 with background and later 2 with already blended 1 with background). Is there a way, how to achieve the effect, that the overlapping parts have the same color as a single non-overlapping segmens?
c# graphics gdi+
add a comment |
I have roughly this logic:
Bitmap bmp = ....
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15);
var graphics = Graphics.FromImage(bmp);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
The problem is, that points1 and points2 contain some line segments that are overlaping.
If I draw this lines, the overlapping part have a different color then the rest, due to the blending of the same segments (first, 1 with background and later 2 with already blended 1 with background). Is there a way, how to achieve the effect, that the overlapping parts have the same color as a single non-overlapping segmens?
c# graphics gdi+
I have roughly this logic:
Bitmap bmp = ....
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15);
var graphics = Graphics.FromImage(bmp);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
The problem is, that points1 and points2 contain some line segments that are overlaping.
If I draw this lines, the overlapping part have a different color then the rest, due to the blending of the same segments (first, 1 with background and later 2 with already blended 1 with background). Is there a way, how to achieve the effect, that the overlapping parts have the same color as a single non-overlapping segmens?
c# graphics gdi+
c# graphics gdi+
edited Feb 2 at 17:59
Uwe Keim
27.6k32132213
27.6k32132213
asked Jan 2 at 12:22


Martin PerryMartin Perry
5,14933165
5,14933165
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
DrawLines
will not work in this case as it will only draw connected lines in one go.
You need to add the line sets to one GraphicsPath
using StartFigure
to separate the two sets.
Example, Drawline
to the left, DrawPath
to the right:
Here is the code for both:
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
..
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15)
{ LineJoin = LineJoin.Round };
var graphics = Graphics.FromImage(bmp);
graphics.Clear(Color.White);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
bmp.Save("D:\__x19DL", ImageFormat.Png);
graphics.Clear(Color.White);
using (GraphicsPath gp = new GraphicsPath())
{
gp.AddLines(points1);
gp.StartFigure();
gp.AddLines(points2);
graphics.DrawPath(pen, gp);
bmp.Save("D:\__x19GP", ImageFormat.Png);
}
Don't forget to Dispose
of the Pen
and the Graphics
object, or, better, put them in using
clauses!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54006327%2fdraw-overlapping-lines-with-same-transparency%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
DrawLines
will not work in this case as it will only draw connected lines in one go.
You need to add the line sets to one GraphicsPath
using StartFigure
to separate the two sets.
Example, Drawline
to the left, DrawPath
to the right:
Here is the code for both:
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
..
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15)
{ LineJoin = LineJoin.Round };
var graphics = Graphics.FromImage(bmp);
graphics.Clear(Color.White);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
bmp.Save("D:\__x19DL", ImageFormat.Png);
graphics.Clear(Color.White);
using (GraphicsPath gp = new GraphicsPath())
{
gp.AddLines(points1);
gp.StartFigure();
gp.AddLines(points2);
graphics.DrawPath(pen, gp);
bmp.Save("D:\__x19GP", ImageFormat.Png);
}
Don't forget to Dispose
of the Pen
and the Graphics
object, or, better, put them in using
clauses!
add a comment |
DrawLines
will not work in this case as it will only draw connected lines in one go.
You need to add the line sets to one GraphicsPath
using StartFigure
to separate the two sets.
Example, Drawline
to the left, DrawPath
to the right:
Here is the code for both:
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
..
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15)
{ LineJoin = LineJoin.Round };
var graphics = Graphics.FromImage(bmp);
graphics.Clear(Color.White);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
bmp.Save("D:\__x19DL", ImageFormat.Png);
graphics.Clear(Color.White);
using (GraphicsPath gp = new GraphicsPath())
{
gp.AddLines(points1);
gp.StartFigure();
gp.AddLines(points2);
graphics.DrawPath(pen, gp);
bmp.Save("D:\__x19GP", ImageFormat.Png);
}
Don't forget to Dispose
of the Pen
and the Graphics
object, or, better, put them in using
clauses!
add a comment |
DrawLines
will not work in this case as it will only draw connected lines in one go.
You need to add the line sets to one GraphicsPath
using StartFigure
to separate the two sets.
Example, Drawline
to the left, DrawPath
to the right:
Here is the code for both:
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
..
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15)
{ LineJoin = LineJoin.Round };
var graphics = Graphics.FromImage(bmp);
graphics.Clear(Color.White);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
bmp.Save("D:\__x19DL", ImageFormat.Png);
graphics.Clear(Color.White);
using (GraphicsPath gp = new GraphicsPath())
{
gp.AddLines(points1);
gp.StartFigure();
gp.AddLines(points2);
graphics.DrawPath(pen, gp);
bmp.Save("D:\__x19GP", ImageFormat.Png);
}
Don't forget to Dispose
of the Pen
and the Graphics
object, or, better, put them in using
clauses!
DrawLines
will not work in this case as it will only draw connected lines in one go.
You need to add the line sets to one GraphicsPath
using StartFigure
to separate the two sets.
Example, Drawline
to the left, DrawPath
to the right:
Here is the code for both:
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
..
Pen pen = new Pen(Color.FromArgb(125, 0, 0, 255), 15)
{ LineJoin = LineJoin.Round };
var graphics = Graphics.FromImage(bmp);
graphics.Clear(Color.White);
graphics.DrawLines(pen, points1);
graphics.DrawLines(pen, points2);
bmp.Save("D:\__x19DL", ImageFormat.Png);
graphics.Clear(Color.White);
using (GraphicsPath gp = new GraphicsPath())
{
gp.AddLines(points1);
gp.StartFigure();
gp.AddLines(points2);
graphics.DrawPath(pen, gp);
bmp.Save("D:\__x19GP", ImageFormat.Png);
}
Don't forget to Dispose
of the Pen
and the Graphics
object, or, better, put them in using
clauses!
edited Jan 2 at 18:17
answered Jan 2 at 15:39


TaWTaW
41.7k62866
41.7k62866
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54006327%2fdraw-overlapping-lines-with-same-transparency%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DbzH,z2iiEsIDUBZ h0T6pY8EEL0me,Z v3e2t0nNcOi 8usHQ6Z,SmQEQjKbBWSp4tBN