c++ JsonCpp parse string with escaped quotes as array
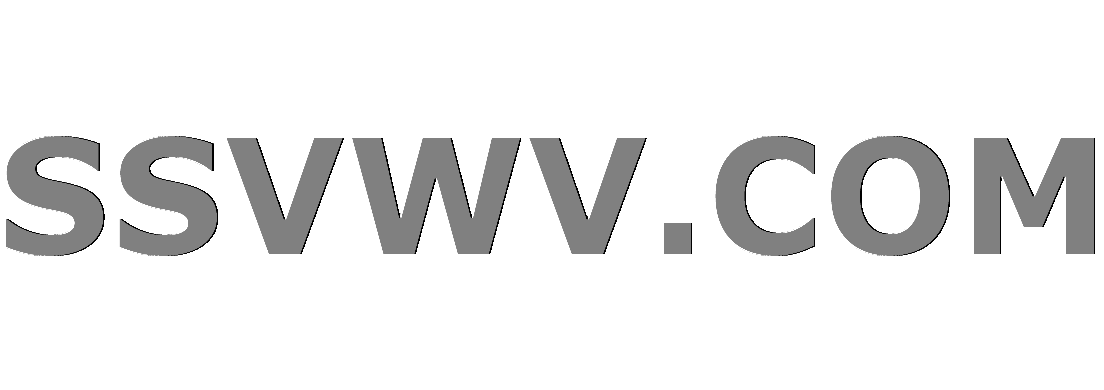
Multi tool use
I've got the following json string:
{
"data" :
[
{
"cart" : "[{"name":"Test item 1","price":15,"quantity":1,"sum":15,"tax":"none","payment_type":"advance","item_type":"service"},{"name":"Test item 2","price":13.01,"quantity":2,"sum":26.02,"tax":"none","payment_type":"part_prepay","item_type":"work"}]",
"contact" : "noname@google.com",
"p_id" : "603",
"sum" : "100.02",
"tax_system" : "osn"
}
],
"msg" : null,
"result" : "success"
}
I can parse cart as std::string after parsing input json string as stringstream:
const std::string ParseJsonData(std::stringstream ssJsonStream)
{
Json::Value jsonData;
Json::Value responseData;
Json::Value responseDataCart;
Json::CharReaderBuilder jsonReader;
std::string errs;
if (Json::parseFromStream(jsonReader, ssJsonStream, &jsonData, &errs)) {
responseData = jsonData["data"];
responseDataCart = responseData[0]["cart"];
return responseDataCart.toStyledString().c_str();
}
else
return "Could not parse HTTP data as JSON";
}
Please, tell me, how can i parse cart as array with JsonCpp?
c++ arrays jsoncpp
add a comment |
I've got the following json string:
{
"data" :
[
{
"cart" : "[{"name":"Test item 1","price":15,"quantity":1,"sum":15,"tax":"none","payment_type":"advance","item_type":"service"},{"name":"Test item 2","price":13.01,"quantity":2,"sum":26.02,"tax":"none","payment_type":"part_prepay","item_type":"work"}]",
"contact" : "noname@google.com",
"p_id" : "603",
"sum" : "100.02",
"tax_system" : "osn"
}
],
"msg" : null,
"result" : "success"
}
I can parse cart as std::string after parsing input json string as stringstream:
const std::string ParseJsonData(std::stringstream ssJsonStream)
{
Json::Value jsonData;
Json::Value responseData;
Json::Value responseDataCart;
Json::CharReaderBuilder jsonReader;
std::string errs;
if (Json::parseFromStream(jsonReader, ssJsonStream, &jsonData, &errs)) {
responseData = jsonData["data"];
responseDataCart = responseData[0]["cart"];
return responseDataCart.toStyledString().c_str();
}
else
return "Could not parse HTTP data as JSON";
}
Please, tell me, how can i parse cart as array with JsonCpp?
c++ arrays jsoncpp
Did you tryresponseDataCart = responseData[0]["cart"]["name"];
– Joey Mallone
Jan 2 at 12:29
Yes, i tried - it doesn't work.
– Michael K.
Jan 2 at 12:30
Your return statement is wasteful.toStyledString()
already gives you astd::string
, which only needs directly returning (which will move it); you're obtaining a C-string out of it then constructing a new string from that. It's not the end of the world, but it does require astrlen
invocation and prohibits moving the data.
– Lightness Races in Orbit
Jan 2 at 12:42
add a comment |
I've got the following json string:
{
"data" :
[
{
"cart" : "[{"name":"Test item 1","price":15,"quantity":1,"sum":15,"tax":"none","payment_type":"advance","item_type":"service"},{"name":"Test item 2","price":13.01,"quantity":2,"sum":26.02,"tax":"none","payment_type":"part_prepay","item_type":"work"}]",
"contact" : "noname@google.com",
"p_id" : "603",
"sum" : "100.02",
"tax_system" : "osn"
}
],
"msg" : null,
"result" : "success"
}
I can parse cart as std::string after parsing input json string as stringstream:
const std::string ParseJsonData(std::stringstream ssJsonStream)
{
Json::Value jsonData;
Json::Value responseData;
Json::Value responseDataCart;
Json::CharReaderBuilder jsonReader;
std::string errs;
if (Json::parseFromStream(jsonReader, ssJsonStream, &jsonData, &errs)) {
responseData = jsonData["data"];
responseDataCart = responseData[0]["cart"];
return responseDataCart.toStyledString().c_str();
}
else
return "Could not parse HTTP data as JSON";
}
Please, tell me, how can i parse cart as array with JsonCpp?
c++ arrays jsoncpp
I've got the following json string:
{
"data" :
[
{
"cart" : "[{"name":"Test item 1","price":15,"quantity":1,"sum":15,"tax":"none","payment_type":"advance","item_type":"service"},{"name":"Test item 2","price":13.01,"quantity":2,"sum":26.02,"tax":"none","payment_type":"part_prepay","item_type":"work"}]",
"contact" : "noname@google.com",
"p_id" : "603",
"sum" : "100.02",
"tax_system" : "osn"
}
],
"msg" : null,
"result" : "success"
}
I can parse cart as std::string after parsing input json string as stringstream:
const std::string ParseJsonData(std::stringstream ssJsonStream)
{
Json::Value jsonData;
Json::Value responseData;
Json::Value responseDataCart;
Json::CharReaderBuilder jsonReader;
std::string errs;
if (Json::parseFromStream(jsonReader, ssJsonStream, &jsonData, &errs)) {
responseData = jsonData["data"];
responseDataCart = responseData[0]["cart"];
return responseDataCart.toStyledString().c_str();
}
else
return "Could not parse HTTP data as JSON";
}
Please, tell me, how can i parse cart as array with JsonCpp?
c++ arrays jsoncpp
c++ arrays jsoncpp
asked Jan 2 at 12:23
Michael K.Michael K.
61
61
Did you tryresponseDataCart = responseData[0]["cart"]["name"];
– Joey Mallone
Jan 2 at 12:29
Yes, i tried - it doesn't work.
– Michael K.
Jan 2 at 12:30
Your return statement is wasteful.toStyledString()
already gives you astd::string
, which only needs directly returning (which will move it); you're obtaining a C-string out of it then constructing a new string from that. It's not the end of the world, but it does require astrlen
invocation and prohibits moving the data.
– Lightness Races in Orbit
Jan 2 at 12:42
add a comment |
Did you tryresponseDataCart = responseData[0]["cart"]["name"];
– Joey Mallone
Jan 2 at 12:29
Yes, i tried - it doesn't work.
– Michael K.
Jan 2 at 12:30
Your return statement is wasteful.toStyledString()
already gives you astd::string
, which only needs directly returning (which will move it); you're obtaining a C-string out of it then constructing a new string from that. It's not the end of the world, but it does require astrlen
invocation and prohibits moving the data.
– Lightness Races in Orbit
Jan 2 at 12:42
Did you try
responseDataCart = responseData[0]["cart"]["name"];
– Joey Mallone
Jan 2 at 12:29
Did you try
responseDataCart = responseData[0]["cart"]["name"];
– Joey Mallone
Jan 2 at 12:29
Yes, i tried - it doesn't work.
– Michael K.
Jan 2 at 12:30
Yes, i tried - it doesn't work.
– Michael K.
Jan 2 at 12:30
Your return statement is wasteful.
toStyledString()
already gives you a std::string
, which only needs directly returning (which will move it); you're obtaining a C-string out of it then constructing a new string from that. It's not the end of the world, but it does require a strlen
invocation and prohibits moving the data.– Lightness Races in Orbit
Jan 2 at 12:42
Your return statement is wasteful.
toStyledString()
already gives you a std::string
, which only needs directly returning (which will move it); you're obtaining a C-string out of it then constructing a new string from that. It's not the end of the world, but it does require a strlen
invocation and prohibits moving the data.– Lightness Races in Orbit
Jan 2 at 12:42
add a comment |
1 Answer
1
active
oldest
votes
The same way you parsed the outer JSON!
You started with a string (well, hidden by a stream) and turned it into JSON.
Now that JSON contains a property that is a string and, itself, contains JSON. The problem is recursive. The fact that the inner string originally came from JSON too can be ignored. Just pretend it's a string you typed in.
So, you can use JSON::Reader to in turn get the JSON out of that string.
Something like:
const std::string responseDataCartStr = responseData[0]["cart"].asString();
Json::Reader reader;
if (!reader.parse(responseDataCartStr, responseDataCart))
throw std::runtime_error("Parsing nested JSON failed");
JsonCpp provides a few ways to parse JSON and it's worth exploring them to find the most appropriate for your use case. The above is just an example.
Ignore the backslashes — the escaping was meaningful within the encapsulating JSON document, but the outermost parse stage should already have taken that into consideration. You'll see if you print responseDataCartStr
to console that it is a valid JSON document in its own right.
Thanks a lot for the reply! Works perfectly with Json::CharReader* reader = jsonReader.newCharReader();
– Michael K.
Jan 2 at 13:50
@MichaelK. Don't forget todelete
it! I like to usestd::unique_ptr
for the task. I had a memory leak in production where I was doingauto reader = Json::CharReaderBuilder().newCharReader();
and forgot that it returns a naked pointer :( Needless to say that area of code now has a massive comment warning slapped all over it
– Lightness Races in Orbit
Jan 2 at 13:52
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54006333%2fc-jsoncpp-parse-string-with-escaped-quotes-as-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The same way you parsed the outer JSON!
You started with a string (well, hidden by a stream) and turned it into JSON.
Now that JSON contains a property that is a string and, itself, contains JSON. The problem is recursive. The fact that the inner string originally came from JSON too can be ignored. Just pretend it's a string you typed in.
So, you can use JSON::Reader to in turn get the JSON out of that string.
Something like:
const std::string responseDataCartStr = responseData[0]["cart"].asString();
Json::Reader reader;
if (!reader.parse(responseDataCartStr, responseDataCart))
throw std::runtime_error("Parsing nested JSON failed");
JsonCpp provides a few ways to parse JSON and it's worth exploring them to find the most appropriate for your use case. The above is just an example.
Ignore the backslashes — the escaping was meaningful within the encapsulating JSON document, but the outermost parse stage should already have taken that into consideration. You'll see if you print responseDataCartStr
to console that it is a valid JSON document in its own right.
Thanks a lot for the reply! Works perfectly with Json::CharReader* reader = jsonReader.newCharReader();
– Michael K.
Jan 2 at 13:50
@MichaelK. Don't forget todelete
it! I like to usestd::unique_ptr
for the task. I had a memory leak in production where I was doingauto reader = Json::CharReaderBuilder().newCharReader();
and forgot that it returns a naked pointer :( Needless to say that area of code now has a massive comment warning slapped all over it
– Lightness Races in Orbit
Jan 2 at 13:52
add a comment |
The same way you parsed the outer JSON!
You started with a string (well, hidden by a stream) and turned it into JSON.
Now that JSON contains a property that is a string and, itself, contains JSON. The problem is recursive. The fact that the inner string originally came from JSON too can be ignored. Just pretend it's a string you typed in.
So, you can use JSON::Reader to in turn get the JSON out of that string.
Something like:
const std::string responseDataCartStr = responseData[0]["cart"].asString();
Json::Reader reader;
if (!reader.parse(responseDataCartStr, responseDataCart))
throw std::runtime_error("Parsing nested JSON failed");
JsonCpp provides a few ways to parse JSON and it's worth exploring them to find the most appropriate for your use case. The above is just an example.
Ignore the backslashes — the escaping was meaningful within the encapsulating JSON document, but the outermost parse stage should already have taken that into consideration. You'll see if you print responseDataCartStr
to console that it is a valid JSON document in its own right.
Thanks a lot for the reply! Works perfectly with Json::CharReader* reader = jsonReader.newCharReader();
– Michael K.
Jan 2 at 13:50
@MichaelK. Don't forget todelete
it! I like to usestd::unique_ptr
for the task. I had a memory leak in production where I was doingauto reader = Json::CharReaderBuilder().newCharReader();
and forgot that it returns a naked pointer :( Needless to say that area of code now has a massive comment warning slapped all over it
– Lightness Races in Orbit
Jan 2 at 13:52
add a comment |
The same way you parsed the outer JSON!
You started with a string (well, hidden by a stream) and turned it into JSON.
Now that JSON contains a property that is a string and, itself, contains JSON. The problem is recursive. The fact that the inner string originally came from JSON too can be ignored. Just pretend it's a string you typed in.
So, you can use JSON::Reader to in turn get the JSON out of that string.
Something like:
const std::string responseDataCartStr = responseData[0]["cart"].asString();
Json::Reader reader;
if (!reader.parse(responseDataCartStr, responseDataCart))
throw std::runtime_error("Parsing nested JSON failed");
JsonCpp provides a few ways to parse JSON and it's worth exploring them to find the most appropriate for your use case. The above is just an example.
Ignore the backslashes — the escaping was meaningful within the encapsulating JSON document, but the outermost parse stage should already have taken that into consideration. You'll see if you print responseDataCartStr
to console that it is a valid JSON document in its own right.
The same way you parsed the outer JSON!
You started with a string (well, hidden by a stream) and turned it into JSON.
Now that JSON contains a property that is a string and, itself, contains JSON. The problem is recursive. The fact that the inner string originally came from JSON too can be ignored. Just pretend it's a string you typed in.
So, you can use JSON::Reader to in turn get the JSON out of that string.
Something like:
const std::string responseDataCartStr = responseData[0]["cart"].asString();
Json::Reader reader;
if (!reader.parse(responseDataCartStr, responseDataCart))
throw std::runtime_error("Parsing nested JSON failed");
JsonCpp provides a few ways to parse JSON and it's worth exploring them to find the most appropriate for your use case. The above is just an example.
Ignore the backslashes — the escaping was meaningful within the encapsulating JSON document, but the outermost parse stage should already have taken that into consideration. You'll see if you print responseDataCartStr
to console that it is a valid JSON document in its own right.
answered Jan 2 at 12:37


Lightness Races in OrbitLightness Races in Orbit
292k54476808
292k54476808
Thanks a lot for the reply! Works perfectly with Json::CharReader* reader = jsonReader.newCharReader();
– Michael K.
Jan 2 at 13:50
@MichaelK. Don't forget todelete
it! I like to usestd::unique_ptr
for the task. I had a memory leak in production where I was doingauto reader = Json::CharReaderBuilder().newCharReader();
and forgot that it returns a naked pointer :( Needless to say that area of code now has a massive comment warning slapped all over it
– Lightness Races in Orbit
Jan 2 at 13:52
add a comment |
Thanks a lot for the reply! Works perfectly with Json::CharReader* reader = jsonReader.newCharReader();
– Michael K.
Jan 2 at 13:50
@MichaelK. Don't forget todelete
it! I like to usestd::unique_ptr
for the task. I had a memory leak in production where I was doingauto reader = Json::CharReaderBuilder().newCharReader();
and forgot that it returns a naked pointer :( Needless to say that area of code now has a massive comment warning slapped all over it
– Lightness Races in Orbit
Jan 2 at 13:52
Thanks a lot for the reply! Works perfectly with Json::CharReader* reader = jsonReader.newCharReader();
– Michael K.
Jan 2 at 13:50
Thanks a lot for the reply! Works perfectly with Json::CharReader* reader = jsonReader.newCharReader();
– Michael K.
Jan 2 at 13:50
@MichaelK. Don't forget to
delete
it! I like to use std::unique_ptr
for the task. I had a memory leak in production where I was doing auto reader = Json::CharReaderBuilder().newCharReader();
and forgot that it returns a naked pointer :( Needless to say that area of code now has a massive comment warning slapped all over it– Lightness Races in Orbit
Jan 2 at 13:52
@MichaelK. Don't forget to
delete
it! I like to use std::unique_ptr
for the task. I had a memory leak in production where I was doing auto reader = Json::CharReaderBuilder().newCharReader();
and forgot that it returns a naked pointer :( Needless to say that area of code now has a massive comment warning slapped all over it– Lightness Races in Orbit
Jan 2 at 13:52
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54006333%2fc-jsoncpp-parse-string-with-escaped-quotes-as-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZrOXCkMB0CZbSGWI6c HOWC3UiOPfVCL,vuhzEu,g8I2AfP2
Did you try
responseDataCart = responseData[0]["cart"]["name"];
– Joey Mallone
Jan 2 at 12:29
Yes, i tried - it doesn't work.
– Michael K.
Jan 2 at 12:30
Your return statement is wasteful.
toStyledString()
already gives you astd::string
, which only needs directly returning (which will move it); you're obtaining a C-string out of it then constructing a new string from that. It's not the end of the world, but it does require astrlen
invocation and prohibits moving the data.– Lightness Races in Orbit
Jan 2 at 12:42