How to Improve For Loop Performance [closed]
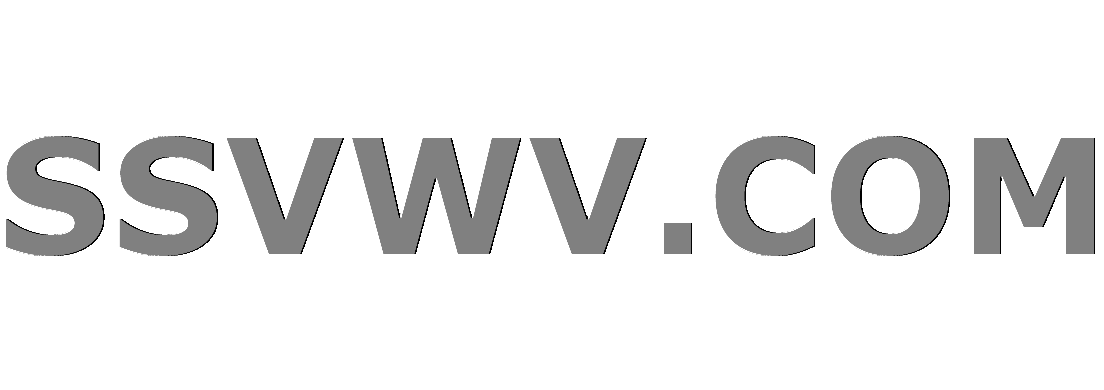
Multi tool use
This code is pretty fast but I would like to make it faster without using HashMap.
I have a list of Accounts and I am currently looping them to check if account.getAccountNumber().equals(accountNumber)
Is there a way to improve this using Java 8?
public Account getAccountFromCustomer(String customerID, String accountNumber) {
List<Account> accounts = getAccountsFromCustomer(customerID);
for(Account account : accounts) {
if(account.getAccountNumber().equals(accountNumber)) {
return account;
}
}
return null;
}
java
closed as too broad by Jens, Aomine, Reimeus, Michael, Tomasz Mularczyk Jan 2 at 13:45
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
|
show 10 more comments
This code is pretty fast but I would like to make it faster without using HashMap.
I have a list of Accounts and I am currently looping them to check if account.getAccountNumber().equals(accountNumber)
Is there a way to improve this using Java 8?
public Account getAccountFromCustomer(String customerID, String accountNumber) {
List<Account> accounts = getAccountsFromCustomer(customerID);
for(Account account : accounts) {
if(account.getAccountNumber().equals(accountNumber)) {
return account;
}
}
return null;
}
java
closed as too broad by Jens, Aomine, Reimeus, Michael, Tomasz Mularczyk Jan 2 at 13:45
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
2
"I would like to make it faster without using HashMap." Why would you use a HashMap?
– Andy Turner
Jan 2 at 12:21
2
without using HashMap
, where are you usingHashMap
?
– Sid
Jan 2 at 12:22
2
No, there is no way.
– JB Nizet
Jan 2 at 12:23
1
Ifaccounts
is very large, try outparallelStream
. It also depends ongetAccountsFromCustomer
method.
– Sid
Jan 2 at 12:24
3
@Sid "It won't have better performance".
– Andy Turner
Jan 2 at 12:26
|
show 10 more comments
This code is pretty fast but I would like to make it faster without using HashMap.
I have a list of Accounts and I am currently looping them to check if account.getAccountNumber().equals(accountNumber)
Is there a way to improve this using Java 8?
public Account getAccountFromCustomer(String customerID, String accountNumber) {
List<Account> accounts = getAccountsFromCustomer(customerID);
for(Account account : accounts) {
if(account.getAccountNumber().equals(accountNumber)) {
return account;
}
}
return null;
}
java
This code is pretty fast but I would like to make it faster without using HashMap.
I have a list of Accounts and I am currently looping them to check if account.getAccountNumber().equals(accountNumber)
Is there a way to improve this using Java 8?
public Account getAccountFromCustomer(String customerID, String accountNumber) {
List<Account> accounts = getAccountsFromCustomer(customerID);
for(Account account : accounts) {
if(account.getAccountNumber().equals(accountNumber)) {
return account;
}
}
return null;
}
java
java
asked Jan 2 at 12:21
TiagoTiago
112
112
closed as too broad by Jens, Aomine, Reimeus, Michael, Tomasz Mularczyk Jan 2 at 13:45
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
closed as too broad by Jens, Aomine, Reimeus, Michael, Tomasz Mularczyk Jan 2 at 13:45
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
2
"I would like to make it faster without using HashMap." Why would you use a HashMap?
– Andy Turner
Jan 2 at 12:21
2
without using HashMap
, where are you usingHashMap
?
– Sid
Jan 2 at 12:22
2
No, there is no way.
– JB Nizet
Jan 2 at 12:23
1
Ifaccounts
is very large, try outparallelStream
. It also depends ongetAccountsFromCustomer
method.
– Sid
Jan 2 at 12:24
3
@Sid "It won't have better performance".
– Andy Turner
Jan 2 at 12:26
|
show 10 more comments
2
"I would like to make it faster without using HashMap." Why would you use a HashMap?
– Andy Turner
Jan 2 at 12:21
2
without using HashMap
, where are you usingHashMap
?
– Sid
Jan 2 at 12:22
2
No, there is no way.
– JB Nizet
Jan 2 at 12:23
1
Ifaccounts
is very large, try outparallelStream
. It also depends ongetAccountsFromCustomer
method.
– Sid
Jan 2 at 12:24
3
@Sid "It won't have better performance".
– Andy Turner
Jan 2 at 12:26
2
2
"I would like to make it faster without using HashMap." Why would you use a HashMap?
– Andy Turner
Jan 2 at 12:21
"I would like to make it faster without using HashMap." Why would you use a HashMap?
– Andy Turner
Jan 2 at 12:21
2
2
without using HashMap
, where are you using HashMap
?– Sid
Jan 2 at 12:22
without using HashMap
, where are you using HashMap
?– Sid
Jan 2 at 12:22
2
2
No, there is no way.
– JB Nizet
Jan 2 at 12:23
No, there is no way.
– JB Nizet
Jan 2 at 12:23
1
1
If
accounts
is very large, try out parallelStream
. It also depends on getAccountsFromCustomer
method.– Sid
Jan 2 at 12:24
If
accounts
is very large, try out parallelStream
. It also depends on getAccountsFromCustomer
method.– Sid
Jan 2 at 12:24
3
3
@Sid "It won't have better performance".
– Andy Turner
Jan 2 at 12:26
@Sid "It won't have better performance".
– Andy Turner
Jan 2 at 12:26
|
show 10 more comments
2 Answers
2
active
oldest
votes
Maybe I understood the question wrong but there is a performance improvement if I use parallel
:
@Test
public void testIt(){
Integer accountNumberLookingFor = 50001;
List<Account> accounts = new ArrayList<>();
for (int i = 0 ; i < 100000; i++){
accounts.add(new Account(i));
}
Stopwatch stopwatch = Stopwatch.createStarted();
accounts.stream().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
stopwatch = Stopwatch.createStarted();
accounts.stream().parallel().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
}
private class Account{
Integer accountNumber;
private Account(Integer accountNumber){
this.accountNumber = accountNumber;
}
@Override
public String toString(){
return "Account number: ".concat(String.valueOf(accountNumber));
}
}
leads to:
Time of execution in milliseconds without parallel:50
Time of execution in milliseconds with parallel:9
Is there any disadvantages usign this? Why isn't everyone using that parallel stuff?
– Tiago
Jan 2 at 12:48
Usingparallel
here will use the default thread pool, shared by the entire application. So you might get contention - and actually be slower - if there are other things also using the default thread pool.
– Andy Turner
Jan 2 at 12:49
@Tiago "Why isn't everyone using that parallel stuff" you should perhaps read Effective Java 3rd Ed which has a lot to say about why it's not something you should choose by default.
– Andy Turner
Jan 2 at 12:52
@AndyTurner In my dockerized application I dont care about that. The thread pool is only reservated for my service
– Stefan Beike
Jan 2 at 13:05
add a comment |
I'm not familiar with the classes, used in this example, but from an algorithm point of view, it looks like your are doing the following:
// Create an unordered list.
getAccountsFromCustomer();
// Run through it
for(Account account : accounts) {
if matches()...
}
The speed of this algorithm is O(n)
.
If you put the results in an ordered list, you might use a binary search. You'll need a O(n*log n)
algorithm to create that list (not just adding account names at the end of the list, but inserting in an alphabetical ordered way), and afterards you use a binary search for finding the account name within that list (performance O(log n)
.
Obviously, you'll need two separate functions for that:
- One function which creates the list (this function is called only once).
- One function for finding the account number (this function is called every time you need to find the account, based on its account number).
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Maybe I understood the question wrong but there is a performance improvement if I use parallel
:
@Test
public void testIt(){
Integer accountNumberLookingFor = 50001;
List<Account> accounts = new ArrayList<>();
for (int i = 0 ; i < 100000; i++){
accounts.add(new Account(i));
}
Stopwatch stopwatch = Stopwatch.createStarted();
accounts.stream().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
stopwatch = Stopwatch.createStarted();
accounts.stream().parallel().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
}
private class Account{
Integer accountNumber;
private Account(Integer accountNumber){
this.accountNumber = accountNumber;
}
@Override
public String toString(){
return "Account number: ".concat(String.valueOf(accountNumber));
}
}
leads to:
Time of execution in milliseconds without parallel:50
Time of execution in milliseconds with parallel:9
Is there any disadvantages usign this? Why isn't everyone using that parallel stuff?
– Tiago
Jan 2 at 12:48
Usingparallel
here will use the default thread pool, shared by the entire application. So you might get contention - and actually be slower - if there are other things also using the default thread pool.
– Andy Turner
Jan 2 at 12:49
@Tiago "Why isn't everyone using that parallel stuff" you should perhaps read Effective Java 3rd Ed which has a lot to say about why it's not something you should choose by default.
– Andy Turner
Jan 2 at 12:52
@AndyTurner In my dockerized application I dont care about that. The thread pool is only reservated for my service
– Stefan Beike
Jan 2 at 13:05
add a comment |
Maybe I understood the question wrong but there is a performance improvement if I use parallel
:
@Test
public void testIt(){
Integer accountNumberLookingFor = 50001;
List<Account> accounts = new ArrayList<>();
for (int i = 0 ; i < 100000; i++){
accounts.add(new Account(i));
}
Stopwatch stopwatch = Stopwatch.createStarted();
accounts.stream().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
stopwatch = Stopwatch.createStarted();
accounts.stream().parallel().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
}
private class Account{
Integer accountNumber;
private Account(Integer accountNumber){
this.accountNumber = accountNumber;
}
@Override
public String toString(){
return "Account number: ".concat(String.valueOf(accountNumber));
}
}
leads to:
Time of execution in milliseconds without parallel:50
Time of execution in milliseconds with parallel:9
Is there any disadvantages usign this? Why isn't everyone using that parallel stuff?
– Tiago
Jan 2 at 12:48
Usingparallel
here will use the default thread pool, shared by the entire application. So you might get contention - and actually be slower - if there are other things also using the default thread pool.
– Andy Turner
Jan 2 at 12:49
@Tiago "Why isn't everyone using that parallel stuff" you should perhaps read Effective Java 3rd Ed which has a lot to say about why it's not something you should choose by default.
– Andy Turner
Jan 2 at 12:52
@AndyTurner In my dockerized application I dont care about that. The thread pool is only reservated for my service
– Stefan Beike
Jan 2 at 13:05
add a comment |
Maybe I understood the question wrong but there is a performance improvement if I use parallel
:
@Test
public void testIt(){
Integer accountNumberLookingFor = 50001;
List<Account> accounts = new ArrayList<>();
for (int i = 0 ; i < 100000; i++){
accounts.add(new Account(i));
}
Stopwatch stopwatch = Stopwatch.createStarted();
accounts.stream().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
stopwatch = Stopwatch.createStarted();
accounts.stream().parallel().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
}
private class Account{
Integer accountNumber;
private Account(Integer accountNumber){
this.accountNumber = accountNumber;
}
@Override
public String toString(){
return "Account number: ".concat(String.valueOf(accountNumber));
}
}
leads to:
Time of execution in milliseconds without parallel:50
Time of execution in milliseconds with parallel:9
Maybe I understood the question wrong but there is a performance improvement if I use parallel
:
@Test
public void testIt(){
Integer accountNumberLookingFor = 50001;
List<Account> accounts = new ArrayList<>();
for (int i = 0 ; i < 100000; i++){
accounts.add(new Account(i));
}
Stopwatch stopwatch = Stopwatch.createStarted();
accounts.stream().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
stopwatch = Stopwatch.createStarted();
accounts.stream().parallel().filter(account -> account.accountNumber.equals(accountNumberLookingFor)).findFirst().orElse(null);
System.out.println("Time of execution in milliseconds:" + stopwatch.stop().elapsed(TimeUnit.MILLISECONDS));
}
private class Account{
Integer accountNumber;
private Account(Integer accountNumber){
this.accountNumber = accountNumber;
}
@Override
public String toString(){
return "Account number: ".concat(String.valueOf(accountNumber));
}
}
leads to:
Time of execution in milliseconds without parallel:50
Time of execution in milliseconds with parallel:9
answered Jan 2 at 12:45


Stefan BeikeStefan Beike
9,89173956
9,89173956
Is there any disadvantages usign this? Why isn't everyone using that parallel stuff?
– Tiago
Jan 2 at 12:48
Usingparallel
here will use the default thread pool, shared by the entire application. So you might get contention - and actually be slower - if there are other things also using the default thread pool.
– Andy Turner
Jan 2 at 12:49
@Tiago "Why isn't everyone using that parallel stuff" you should perhaps read Effective Java 3rd Ed which has a lot to say about why it's not something you should choose by default.
– Andy Turner
Jan 2 at 12:52
@AndyTurner In my dockerized application I dont care about that. The thread pool is only reservated for my service
– Stefan Beike
Jan 2 at 13:05
add a comment |
Is there any disadvantages usign this? Why isn't everyone using that parallel stuff?
– Tiago
Jan 2 at 12:48
Usingparallel
here will use the default thread pool, shared by the entire application. So you might get contention - and actually be slower - if there are other things also using the default thread pool.
– Andy Turner
Jan 2 at 12:49
@Tiago "Why isn't everyone using that parallel stuff" you should perhaps read Effective Java 3rd Ed which has a lot to say about why it's not something you should choose by default.
– Andy Turner
Jan 2 at 12:52
@AndyTurner In my dockerized application I dont care about that. The thread pool is only reservated for my service
– Stefan Beike
Jan 2 at 13:05
Is there any disadvantages usign this? Why isn't everyone using that parallel stuff?
– Tiago
Jan 2 at 12:48
Is there any disadvantages usign this? Why isn't everyone using that parallel stuff?
– Tiago
Jan 2 at 12:48
Using
parallel
here will use the default thread pool, shared by the entire application. So you might get contention - and actually be slower - if there are other things also using the default thread pool.– Andy Turner
Jan 2 at 12:49
Using
parallel
here will use the default thread pool, shared by the entire application. So you might get contention - and actually be slower - if there are other things also using the default thread pool.– Andy Turner
Jan 2 at 12:49
@Tiago "Why isn't everyone using that parallel stuff" you should perhaps read Effective Java 3rd Ed which has a lot to say about why it's not something you should choose by default.
– Andy Turner
Jan 2 at 12:52
@Tiago "Why isn't everyone using that parallel stuff" you should perhaps read Effective Java 3rd Ed which has a lot to say about why it's not something you should choose by default.
– Andy Turner
Jan 2 at 12:52
@AndyTurner In my dockerized application I dont care about that. The thread pool is only reservated for my service
– Stefan Beike
Jan 2 at 13:05
@AndyTurner In my dockerized application I dont care about that. The thread pool is only reservated for my service
– Stefan Beike
Jan 2 at 13:05
add a comment |
I'm not familiar with the classes, used in this example, but from an algorithm point of view, it looks like your are doing the following:
// Create an unordered list.
getAccountsFromCustomer();
// Run through it
for(Account account : accounts) {
if matches()...
}
The speed of this algorithm is O(n)
.
If you put the results in an ordered list, you might use a binary search. You'll need a O(n*log n)
algorithm to create that list (not just adding account names at the end of the list, but inserting in an alphabetical ordered way), and afterards you use a binary search for finding the account name within that list (performance O(log n)
.
Obviously, you'll need two separate functions for that:
- One function which creates the list (this function is called only once).
- One function for finding the account number (this function is called every time you need to find the account, based on its account number).
add a comment |
I'm not familiar with the classes, used in this example, but from an algorithm point of view, it looks like your are doing the following:
// Create an unordered list.
getAccountsFromCustomer();
// Run through it
for(Account account : accounts) {
if matches()...
}
The speed of this algorithm is O(n)
.
If you put the results in an ordered list, you might use a binary search. You'll need a O(n*log n)
algorithm to create that list (not just adding account names at the end of the list, but inserting in an alphabetical ordered way), and afterards you use a binary search for finding the account name within that list (performance O(log n)
.
Obviously, you'll need two separate functions for that:
- One function which creates the list (this function is called only once).
- One function for finding the account number (this function is called every time you need to find the account, based on its account number).
add a comment |
I'm not familiar with the classes, used in this example, but from an algorithm point of view, it looks like your are doing the following:
// Create an unordered list.
getAccountsFromCustomer();
// Run through it
for(Account account : accounts) {
if matches()...
}
The speed of this algorithm is O(n)
.
If you put the results in an ordered list, you might use a binary search. You'll need a O(n*log n)
algorithm to create that list (not just adding account names at the end of the list, but inserting in an alphabetical ordered way), and afterards you use a binary search for finding the account name within that list (performance O(log n)
.
Obviously, you'll need two separate functions for that:
- One function which creates the list (this function is called only once).
- One function for finding the account number (this function is called every time you need to find the account, based on its account number).
I'm not familiar with the classes, used in this example, but from an algorithm point of view, it looks like your are doing the following:
// Create an unordered list.
getAccountsFromCustomer();
// Run through it
for(Account account : accounts) {
if matches()...
}
The speed of this algorithm is O(n)
.
If you put the results in an ordered list, you might use a binary search. You'll need a O(n*log n)
algorithm to create that list (not just adding account names at the end of the list, but inserting in an alphabetical ordered way), and afterards you use a binary search for finding the account name within that list (performance O(log n)
.
Obviously, you'll need two separate functions for that:
- One function which creates the list (this function is called only once).
- One function for finding the account number (this function is called every time you need to find the account, based on its account number).
answered Jan 2 at 12:36
DominiqueDominique
2,12841942
2,12841942
add a comment |
add a comment |
m,GttW2ND1TYAC,4kGW3nrhFpQIGF4mC5gUQZR,E0 KgccLvXQ bdV
2
"I would like to make it faster without using HashMap." Why would you use a HashMap?
– Andy Turner
Jan 2 at 12:21
2
without using HashMap
, where are you usingHashMap
?– Sid
Jan 2 at 12:22
2
No, there is no way.
– JB Nizet
Jan 2 at 12:23
1
If
accounts
is very large, try outparallelStream
. It also depends ongetAccountsFromCustomer
method.– Sid
Jan 2 at 12:24
3
@Sid "It won't have better performance".
– Andy Turner
Jan 2 at 12:26