POST method for elements in collection
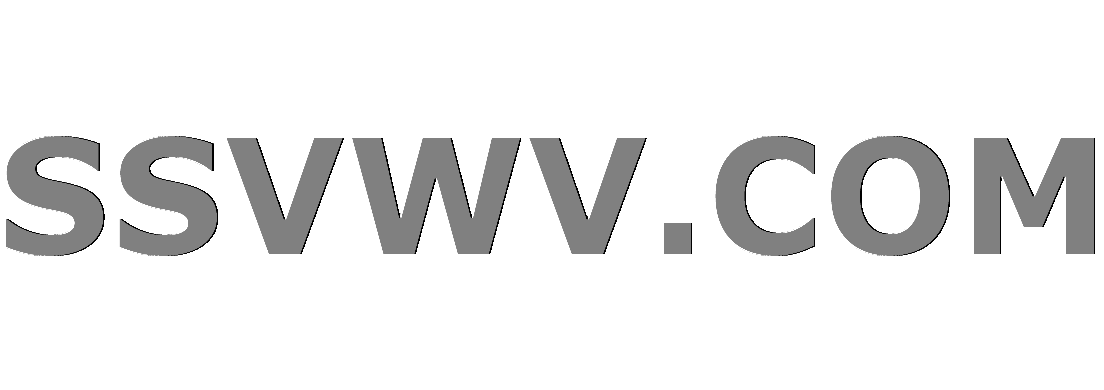
Multi tool use
I'm on a .NET platform, writing an MVC application for registering attendances of Departments' Chiefs and their staff. Each of them will log into the webpage, will have a list of some people in their staff shown, then they shall check some checkboxes and select some elements from dropdownlists, and all need to be posted back to the server.
First, I have the Entities:
public class Attendance
{
public DateTime Date { get; set; }
public Chief Appointed { get; set; }
public bool Present { get; set; }
public LeaveType Leave { get; set; }
public Chief Deputy { get; set; }
}
public class Department
{
public string Name { get; set; }
public int ID { get; set; }
public Chief Head { get; set; }
public Department Parent { get; set; }
public List<Department> Children { get; set; }
}
These entities, stored in a SQL Server database, make up a repository so that when anyone logs into the web page, the Controller populates the ViewModel with a list of people in their staff only.
This filtered repository is wrapped up in the ViewModel that, for other reasons, has a constructor method:
public class ViewModel
{
public List<Attendance> Attendances { get; set; }
public List<Department> Departments { get; set; }
public ViewModel()
{
// some code
}
}
This is (part of) the View code - important note: I'm using this boostrap-switch javascript library for turning checkboxes into switches, so I can't rely on helper methods for the input elements.
@model ViewModel
@using (Html.BeginForm("Index", "Attendances", FormMethod.Post))
{
foreach (Department department in Model.Departments)
{
<script type="text/javascript">
$(document).ready(function () {
$("[id='@department.ID']").bootstrapSwitch()
});
</script>
<div>
<div>
@department.Name
</div>
</div>
<div>
<div>
@department.Head
</div>
<div>
<input type="checkbox" id="@department.ID" name="Department.ID" checked />
</div>
</div>
}
<input type="submit" value="Submit"/>
}
Now, this is where I have problems: in the controller, I have this POST method that create a new instance of the ViewModel every time is called.
[HttpPost]
public ActionResult Index(ViewModel viewModel)
{
// Register the submitted data
return View(viewModel);
}
How do I tell the code to take the collection submitted by the user into the new (empty) collection created by the controller when the user clicks on the submit button?
Thanks, Davide.
c# post model-view-controller collections
add a comment |
I'm on a .NET platform, writing an MVC application for registering attendances of Departments' Chiefs and their staff. Each of them will log into the webpage, will have a list of some people in their staff shown, then they shall check some checkboxes and select some elements from dropdownlists, and all need to be posted back to the server.
First, I have the Entities:
public class Attendance
{
public DateTime Date { get; set; }
public Chief Appointed { get; set; }
public bool Present { get; set; }
public LeaveType Leave { get; set; }
public Chief Deputy { get; set; }
}
public class Department
{
public string Name { get; set; }
public int ID { get; set; }
public Chief Head { get; set; }
public Department Parent { get; set; }
public List<Department> Children { get; set; }
}
These entities, stored in a SQL Server database, make up a repository so that when anyone logs into the web page, the Controller populates the ViewModel with a list of people in their staff only.
This filtered repository is wrapped up in the ViewModel that, for other reasons, has a constructor method:
public class ViewModel
{
public List<Attendance> Attendances { get; set; }
public List<Department> Departments { get; set; }
public ViewModel()
{
// some code
}
}
This is (part of) the View code - important note: I'm using this boostrap-switch javascript library for turning checkboxes into switches, so I can't rely on helper methods for the input elements.
@model ViewModel
@using (Html.BeginForm("Index", "Attendances", FormMethod.Post))
{
foreach (Department department in Model.Departments)
{
<script type="text/javascript">
$(document).ready(function () {
$("[id='@department.ID']").bootstrapSwitch()
});
</script>
<div>
<div>
@department.Name
</div>
</div>
<div>
<div>
@department.Head
</div>
<div>
<input type="checkbox" id="@department.ID" name="Department.ID" checked />
</div>
</div>
}
<input type="submit" value="Submit"/>
}
Now, this is where I have problems: in the controller, I have this POST method that create a new instance of the ViewModel every time is called.
[HttpPost]
public ActionResult Index(ViewModel viewModel)
{
// Register the submitted data
return View(viewModel);
}
How do I tell the code to take the collection submitted by the user into the new (empty) collection created by the controller when the user clicks on the submit button?
Thanks, Davide.
c# post model-view-controller collections
After user clicks on submit, viewmodel collection values will come to controller. What you want to do now?
– sri harsha
Jan 2 at 12:59
How aboutRequest.Form
indexer to get checkbox value, such likeviewModel.DepartmentID = Request.Form["Department.ID"].ToString()
? I think you can still use HTML helpers with bootstrap-switch, just sethtmlAttributes
withnew { @class = "..." }
to style into switches.
– Tetsuya Yamamoto
Jan 3 at 4:17
In the end, the problem was the default ViewModel constructor preventing the model binding. Thanks anyway.
– Davide Vitali
Jan 3 at 14:39
add a comment |
I'm on a .NET platform, writing an MVC application for registering attendances of Departments' Chiefs and their staff. Each of them will log into the webpage, will have a list of some people in their staff shown, then they shall check some checkboxes and select some elements from dropdownlists, and all need to be posted back to the server.
First, I have the Entities:
public class Attendance
{
public DateTime Date { get; set; }
public Chief Appointed { get; set; }
public bool Present { get; set; }
public LeaveType Leave { get; set; }
public Chief Deputy { get; set; }
}
public class Department
{
public string Name { get; set; }
public int ID { get; set; }
public Chief Head { get; set; }
public Department Parent { get; set; }
public List<Department> Children { get; set; }
}
These entities, stored in a SQL Server database, make up a repository so that when anyone logs into the web page, the Controller populates the ViewModel with a list of people in their staff only.
This filtered repository is wrapped up in the ViewModel that, for other reasons, has a constructor method:
public class ViewModel
{
public List<Attendance> Attendances { get; set; }
public List<Department> Departments { get; set; }
public ViewModel()
{
// some code
}
}
This is (part of) the View code - important note: I'm using this boostrap-switch javascript library for turning checkboxes into switches, so I can't rely on helper methods for the input elements.
@model ViewModel
@using (Html.BeginForm("Index", "Attendances", FormMethod.Post))
{
foreach (Department department in Model.Departments)
{
<script type="text/javascript">
$(document).ready(function () {
$("[id='@department.ID']").bootstrapSwitch()
});
</script>
<div>
<div>
@department.Name
</div>
</div>
<div>
<div>
@department.Head
</div>
<div>
<input type="checkbox" id="@department.ID" name="Department.ID" checked />
</div>
</div>
}
<input type="submit" value="Submit"/>
}
Now, this is where I have problems: in the controller, I have this POST method that create a new instance of the ViewModel every time is called.
[HttpPost]
public ActionResult Index(ViewModel viewModel)
{
// Register the submitted data
return View(viewModel);
}
How do I tell the code to take the collection submitted by the user into the new (empty) collection created by the controller when the user clicks on the submit button?
Thanks, Davide.
c# post model-view-controller collections
I'm on a .NET platform, writing an MVC application for registering attendances of Departments' Chiefs and their staff. Each of them will log into the webpage, will have a list of some people in their staff shown, then they shall check some checkboxes and select some elements from dropdownlists, and all need to be posted back to the server.
First, I have the Entities:
public class Attendance
{
public DateTime Date { get; set; }
public Chief Appointed { get; set; }
public bool Present { get; set; }
public LeaveType Leave { get; set; }
public Chief Deputy { get; set; }
}
public class Department
{
public string Name { get; set; }
public int ID { get; set; }
public Chief Head { get; set; }
public Department Parent { get; set; }
public List<Department> Children { get; set; }
}
These entities, stored in a SQL Server database, make up a repository so that when anyone logs into the web page, the Controller populates the ViewModel with a list of people in their staff only.
This filtered repository is wrapped up in the ViewModel that, for other reasons, has a constructor method:
public class ViewModel
{
public List<Attendance> Attendances { get; set; }
public List<Department> Departments { get; set; }
public ViewModel()
{
// some code
}
}
This is (part of) the View code - important note: I'm using this boostrap-switch javascript library for turning checkboxes into switches, so I can't rely on helper methods for the input elements.
@model ViewModel
@using (Html.BeginForm("Index", "Attendances", FormMethod.Post))
{
foreach (Department department in Model.Departments)
{
<script type="text/javascript">
$(document).ready(function () {
$("[id='@department.ID']").bootstrapSwitch()
});
</script>
<div>
<div>
@department.Name
</div>
</div>
<div>
<div>
@department.Head
</div>
<div>
<input type="checkbox" id="@department.ID" name="Department.ID" checked />
</div>
</div>
}
<input type="submit" value="Submit"/>
}
Now, this is where I have problems: in the controller, I have this POST method that create a new instance of the ViewModel every time is called.
[HttpPost]
public ActionResult Index(ViewModel viewModel)
{
// Register the submitted data
return View(viewModel);
}
How do I tell the code to take the collection submitted by the user into the new (empty) collection created by the controller when the user clicks on the submit button?
Thanks, Davide.
c# post model-view-controller collections
c# post model-view-controller collections
asked Jan 2 at 12:21


Davide VitaliDavide Vitali
445212
445212
After user clicks on submit, viewmodel collection values will come to controller. What you want to do now?
– sri harsha
Jan 2 at 12:59
How aboutRequest.Form
indexer to get checkbox value, such likeviewModel.DepartmentID = Request.Form["Department.ID"].ToString()
? I think you can still use HTML helpers with bootstrap-switch, just sethtmlAttributes
withnew { @class = "..." }
to style into switches.
– Tetsuya Yamamoto
Jan 3 at 4:17
In the end, the problem was the default ViewModel constructor preventing the model binding. Thanks anyway.
– Davide Vitali
Jan 3 at 14:39
add a comment |
After user clicks on submit, viewmodel collection values will come to controller. What you want to do now?
– sri harsha
Jan 2 at 12:59
How aboutRequest.Form
indexer to get checkbox value, such likeviewModel.DepartmentID = Request.Form["Department.ID"].ToString()
? I think you can still use HTML helpers with bootstrap-switch, just sethtmlAttributes
withnew { @class = "..." }
to style into switches.
– Tetsuya Yamamoto
Jan 3 at 4:17
In the end, the problem was the default ViewModel constructor preventing the model binding. Thanks anyway.
– Davide Vitali
Jan 3 at 14:39
After user clicks on submit, viewmodel collection values will come to controller. What you want to do now?
– sri harsha
Jan 2 at 12:59
After user clicks on submit, viewmodel collection values will come to controller. What you want to do now?
– sri harsha
Jan 2 at 12:59
How about
Request.Form
indexer to get checkbox value, such like viewModel.DepartmentID = Request.Form["Department.ID"].ToString()
? I think you can still use HTML helpers with bootstrap-switch, just set htmlAttributes
with new { @class = "..." }
to style into switches.– Tetsuya Yamamoto
Jan 3 at 4:17
How about
Request.Form
indexer to get checkbox value, such like viewModel.DepartmentID = Request.Form["Department.ID"].ToString()
? I think you can still use HTML helpers with bootstrap-switch, just set htmlAttributes
with new { @class = "..." }
to style into switches.– Tetsuya Yamamoto
Jan 3 at 4:17
In the end, the problem was the default ViewModel constructor preventing the model binding. Thanks anyway.
– Davide Vitali
Jan 3 at 14:39
In the end, the problem was the default ViewModel constructor preventing the model binding. Thanks anyway.
– Davide Vitali
Jan 3 at 14:39
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54006308%2fpost-method-for-elements-in-collection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54006308%2fpost-method-for-elements-in-collection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Rp0Cobb,9b,cSU,Ho34LgrbSafdR4H5WWmhkF,0E,mq,61e2AuPma3KMGhPRwMb,lh XjqELU 8pG,leH9z8qLh z3WB6o7vWWNsELgWNLOg1
After user clicks on submit, viewmodel collection values will come to controller. What you want to do now?
– sri harsha
Jan 2 at 12:59
How about
Request.Form
indexer to get checkbox value, such likeviewModel.DepartmentID = Request.Form["Department.ID"].ToString()
? I think you can still use HTML helpers with bootstrap-switch, just sethtmlAttributes
withnew { @class = "..." }
to style into switches.– Tetsuya Yamamoto
Jan 3 at 4:17
In the end, the problem was the default ViewModel constructor preventing the model binding. Thanks anyway.
– Davide Vitali
Jan 3 at 14:39