Mapping a case class to a mongodb document using reactive mongo
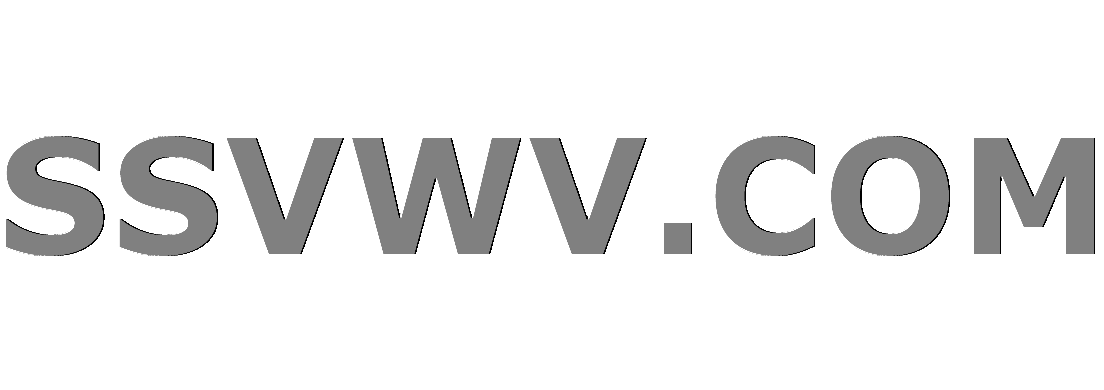
Multi tool use
Below is my simple document that represents a Link. I am using reactivemongo in scala for this.
I am getting this error during compilation:
app/components/Link.scala:60:11: No Json deserializer found for type
components.Link. Try to implement an implicit Reads or Format for this
type. [error] .one[Link]) [error] ^ [error] one error
found
I created the implicits in my Link companion object, which I also imported into my LinkRepo class.
Am I handling the mongo document _id correctly?
Should I use a String to map to the document ID, confused as to what is the best practise? Do I have to convert the String to the BSONObjectID at some point?
package components
import javax.inject.Inject
import reactivemongo.bson._
import reactivemongo.api.ReadPreference
import reactivemongo.api.collections.bson.BSONCollection
import reactivemongo.bson.{ BSONDocument, BSONObjectID }
import reactivemongo.api.commands.{ UpdateWriteResult, WriteResult, Upserted }
import reactivemongo.api.commands.bson.BSONUpdateCommand._
import reactivemongo.api.commands.bson.BSONUpdateCommandImplicits._
case class Link(id: Link.ID,
name: String,
url: String)
object Link {
type ID = String
implicit val linkReader: BSONDocumentReader[Link] =
BSONDocumentReader[Link] { doc: BSONDocument =>
Link(
doc.getAs[String]("id").getOrElse(""),
doc.getAs[String]("name").getOrElse(""),
doc.getAs[String]("url").getOrElse(""))
}
implicit val linkWriter: BSONDocumentWriter[Link] =
BSONDocumentWriter[Link] { link: Link =>
BSONDocument(
"id" -> link.id,
"name" -> link.name,
"url" -> link.url)
}
}
import scala.concurrent.{ ExecutionContext, Future }
import reactivemongo.bson.{ BSONDocument, BSONObjectID }
import reactivemongo.api.{ Cursor, ReadPreference }
import reactivemongo.api.commands.WriteResult
import reactivemongo.play.json._
import reactivemongo.play.json.collection.JSONCollection
import play.modules.reactivemongo.ReactiveMongoApi
class LinkRepo @Inject()(implicit ec: ExecutionContext, reactiveMongoApi: ReactiveMongoApi) {
import Link._
def linksCol: Future[JSONCollection] = reactiveMongoApi.database.map(_.collection("links"))
def byId(id: Link.ID): Future[Option[Link]] = {
linksCol.flatMap(_.find(
selector = BSONDocument("_id" -> id),
projection = Option.empty[BSONDocument])
.one[Link])
}
}
My sbt has these lib versions:
scalaVersion := "2.12.7"
libraryDependencies += guice
libraryDependencies ++= Seq(
guice,
"joda-time" % "joda-time" % "2.9.9",
"net.ruippeixotog" %% "scala-scraper" % "2.1.0",
"org.reactivemongo" %% "play2-reactivemongo" % "0.16.0-play26",
"org.scalatestplus.play" %% "scalatestplus-play" % "3.1.2" % Test
)
plugins:
addSbtPlugin("com.typesafe.play" % "sbt-plugin" % "2.6.20")
mongodb scala playframework play-reactivemongo
add a comment |
Below is my simple document that represents a Link. I am using reactivemongo in scala for this.
I am getting this error during compilation:
app/components/Link.scala:60:11: No Json deserializer found for type
components.Link. Try to implement an implicit Reads or Format for this
type. [error] .one[Link]) [error] ^ [error] one error
found
I created the implicits in my Link companion object, which I also imported into my LinkRepo class.
Am I handling the mongo document _id correctly?
Should I use a String to map to the document ID, confused as to what is the best practise? Do I have to convert the String to the BSONObjectID at some point?
package components
import javax.inject.Inject
import reactivemongo.bson._
import reactivemongo.api.ReadPreference
import reactivemongo.api.collections.bson.BSONCollection
import reactivemongo.bson.{ BSONDocument, BSONObjectID }
import reactivemongo.api.commands.{ UpdateWriteResult, WriteResult, Upserted }
import reactivemongo.api.commands.bson.BSONUpdateCommand._
import reactivemongo.api.commands.bson.BSONUpdateCommandImplicits._
case class Link(id: Link.ID,
name: String,
url: String)
object Link {
type ID = String
implicit val linkReader: BSONDocumentReader[Link] =
BSONDocumentReader[Link] { doc: BSONDocument =>
Link(
doc.getAs[String]("id").getOrElse(""),
doc.getAs[String]("name").getOrElse(""),
doc.getAs[String]("url").getOrElse(""))
}
implicit val linkWriter: BSONDocumentWriter[Link] =
BSONDocumentWriter[Link] { link: Link =>
BSONDocument(
"id" -> link.id,
"name" -> link.name,
"url" -> link.url)
}
}
import scala.concurrent.{ ExecutionContext, Future }
import reactivemongo.bson.{ BSONDocument, BSONObjectID }
import reactivemongo.api.{ Cursor, ReadPreference }
import reactivemongo.api.commands.WriteResult
import reactivemongo.play.json._
import reactivemongo.play.json.collection.JSONCollection
import play.modules.reactivemongo.ReactiveMongoApi
class LinkRepo @Inject()(implicit ec: ExecutionContext, reactiveMongoApi: ReactiveMongoApi) {
import Link._
def linksCol: Future[JSONCollection] = reactiveMongoApi.database.map(_.collection("links"))
def byId(id: Link.ID): Future[Option[Link]] = {
linksCol.flatMap(_.find(
selector = BSONDocument("_id" -> id),
projection = Option.empty[BSONDocument])
.one[Link])
}
}
My sbt has these lib versions:
scalaVersion := "2.12.7"
libraryDependencies += guice
libraryDependencies ++= Seq(
guice,
"joda-time" % "joda-time" % "2.9.9",
"net.ruippeixotog" %% "scala-scraper" % "2.1.0",
"org.reactivemongo" %% "play2-reactivemongo" % "0.16.0-play26",
"org.scalatestplus.play" %% "scalatestplus-play" % "3.1.2" % Test
)
plugins:
addSbtPlugin("com.typesafe.play" % "sbt-plugin" % "2.6.20")
mongodb scala playframework play-reactivemongo
1
You're using a JSON collection, but the provided one are BSON, so you need to make sure conversions fromImplicitBSONHandlers
is imported fromreactivemongo.play.json
.
– cchantep
Jan 3 at 16:19
I added bothimport reactivemongo.play.json.ImplicitBSONHandlers
andimport reactivemongo.play.json._
but I still get the same error.
– Blankman
Jan 3 at 16:37
Check the versions
– cchantep
Jan 3 at 16:38
@cchantep I added my versions to my question. I'm using reactivemongo 0.16.0-play26.
– Blankman
Jan 4 at 12:46
add a comment |
Below is my simple document that represents a Link. I am using reactivemongo in scala for this.
I am getting this error during compilation:
app/components/Link.scala:60:11: No Json deserializer found for type
components.Link. Try to implement an implicit Reads or Format for this
type. [error] .one[Link]) [error] ^ [error] one error
found
I created the implicits in my Link companion object, which I also imported into my LinkRepo class.
Am I handling the mongo document _id correctly?
Should I use a String to map to the document ID, confused as to what is the best practise? Do I have to convert the String to the BSONObjectID at some point?
package components
import javax.inject.Inject
import reactivemongo.bson._
import reactivemongo.api.ReadPreference
import reactivemongo.api.collections.bson.BSONCollection
import reactivemongo.bson.{ BSONDocument, BSONObjectID }
import reactivemongo.api.commands.{ UpdateWriteResult, WriteResult, Upserted }
import reactivemongo.api.commands.bson.BSONUpdateCommand._
import reactivemongo.api.commands.bson.BSONUpdateCommandImplicits._
case class Link(id: Link.ID,
name: String,
url: String)
object Link {
type ID = String
implicit val linkReader: BSONDocumentReader[Link] =
BSONDocumentReader[Link] { doc: BSONDocument =>
Link(
doc.getAs[String]("id").getOrElse(""),
doc.getAs[String]("name").getOrElse(""),
doc.getAs[String]("url").getOrElse(""))
}
implicit val linkWriter: BSONDocumentWriter[Link] =
BSONDocumentWriter[Link] { link: Link =>
BSONDocument(
"id" -> link.id,
"name" -> link.name,
"url" -> link.url)
}
}
import scala.concurrent.{ ExecutionContext, Future }
import reactivemongo.bson.{ BSONDocument, BSONObjectID }
import reactivemongo.api.{ Cursor, ReadPreference }
import reactivemongo.api.commands.WriteResult
import reactivemongo.play.json._
import reactivemongo.play.json.collection.JSONCollection
import play.modules.reactivemongo.ReactiveMongoApi
class LinkRepo @Inject()(implicit ec: ExecutionContext, reactiveMongoApi: ReactiveMongoApi) {
import Link._
def linksCol: Future[JSONCollection] = reactiveMongoApi.database.map(_.collection("links"))
def byId(id: Link.ID): Future[Option[Link]] = {
linksCol.flatMap(_.find(
selector = BSONDocument("_id" -> id),
projection = Option.empty[BSONDocument])
.one[Link])
}
}
My sbt has these lib versions:
scalaVersion := "2.12.7"
libraryDependencies += guice
libraryDependencies ++= Seq(
guice,
"joda-time" % "joda-time" % "2.9.9",
"net.ruippeixotog" %% "scala-scraper" % "2.1.0",
"org.reactivemongo" %% "play2-reactivemongo" % "0.16.0-play26",
"org.scalatestplus.play" %% "scalatestplus-play" % "3.1.2" % Test
)
plugins:
addSbtPlugin("com.typesafe.play" % "sbt-plugin" % "2.6.20")
mongodb scala playframework play-reactivemongo
Below is my simple document that represents a Link. I am using reactivemongo in scala for this.
I am getting this error during compilation:
app/components/Link.scala:60:11: No Json deserializer found for type
components.Link. Try to implement an implicit Reads or Format for this
type. [error] .one[Link]) [error] ^ [error] one error
found
I created the implicits in my Link companion object, which I also imported into my LinkRepo class.
Am I handling the mongo document _id correctly?
Should I use a String to map to the document ID, confused as to what is the best practise? Do I have to convert the String to the BSONObjectID at some point?
package components
import javax.inject.Inject
import reactivemongo.bson._
import reactivemongo.api.ReadPreference
import reactivemongo.api.collections.bson.BSONCollection
import reactivemongo.bson.{ BSONDocument, BSONObjectID }
import reactivemongo.api.commands.{ UpdateWriteResult, WriteResult, Upserted }
import reactivemongo.api.commands.bson.BSONUpdateCommand._
import reactivemongo.api.commands.bson.BSONUpdateCommandImplicits._
case class Link(id: Link.ID,
name: String,
url: String)
object Link {
type ID = String
implicit val linkReader: BSONDocumentReader[Link] =
BSONDocumentReader[Link] { doc: BSONDocument =>
Link(
doc.getAs[String]("id").getOrElse(""),
doc.getAs[String]("name").getOrElse(""),
doc.getAs[String]("url").getOrElse(""))
}
implicit val linkWriter: BSONDocumentWriter[Link] =
BSONDocumentWriter[Link] { link: Link =>
BSONDocument(
"id" -> link.id,
"name" -> link.name,
"url" -> link.url)
}
}
import scala.concurrent.{ ExecutionContext, Future }
import reactivemongo.bson.{ BSONDocument, BSONObjectID }
import reactivemongo.api.{ Cursor, ReadPreference }
import reactivemongo.api.commands.WriteResult
import reactivemongo.play.json._
import reactivemongo.play.json.collection.JSONCollection
import play.modules.reactivemongo.ReactiveMongoApi
class LinkRepo @Inject()(implicit ec: ExecutionContext, reactiveMongoApi: ReactiveMongoApi) {
import Link._
def linksCol: Future[JSONCollection] = reactiveMongoApi.database.map(_.collection("links"))
def byId(id: Link.ID): Future[Option[Link]] = {
linksCol.flatMap(_.find(
selector = BSONDocument("_id" -> id),
projection = Option.empty[BSONDocument])
.one[Link])
}
}
My sbt has these lib versions:
scalaVersion := "2.12.7"
libraryDependencies += guice
libraryDependencies ++= Seq(
guice,
"joda-time" % "joda-time" % "2.9.9",
"net.ruippeixotog" %% "scala-scraper" % "2.1.0",
"org.reactivemongo" %% "play2-reactivemongo" % "0.16.0-play26",
"org.scalatestplus.play" %% "scalatestplus-play" % "3.1.2" % Test
)
plugins:
addSbtPlugin("com.typesafe.play" % "sbt-plugin" % "2.6.20")
mongodb scala playframework play-reactivemongo
mongodb scala playframework play-reactivemongo
edited Jan 4 at 12:46
Blankman
asked Jan 3 at 14:41
BlankmanBlankman
98.6k2706641043
98.6k2706641043
1
You're using a JSON collection, but the provided one are BSON, so you need to make sure conversions fromImplicitBSONHandlers
is imported fromreactivemongo.play.json
.
– cchantep
Jan 3 at 16:19
I added bothimport reactivemongo.play.json.ImplicitBSONHandlers
andimport reactivemongo.play.json._
but I still get the same error.
– Blankman
Jan 3 at 16:37
Check the versions
– cchantep
Jan 3 at 16:38
@cchantep I added my versions to my question. I'm using reactivemongo 0.16.0-play26.
– Blankman
Jan 4 at 12:46
add a comment |
1
You're using a JSON collection, but the provided one are BSON, so you need to make sure conversions fromImplicitBSONHandlers
is imported fromreactivemongo.play.json
.
– cchantep
Jan 3 at 16:19
I added bothimport reactivemongo.play.json.ImplicitBSONHandlers
andimport reactivemongo.play.json._
but I still get the same error.
– Blankman
Jan 3 at 16:37
Check the versions
– cchantep
Jan 3 at 16:38
@cchantep I added my versions to my question. I'm using reactivemongo 0.16.0-play26.
– Blankman
Jan 4 at 12:46
1
1
You're using a JSON collection, but the provided one are BSON, so you need to make sure conversions from
ImplicitBSONHandlers
is imported from reactivemongo.play.json
.– cchantep
Jan 3 at 16:19
You're using a JSON collection, but the provided one are BSON, so you need to make sure conversions from
ImplicitBSONHandlers
is imported from reactivemongo.play.json
.– cchantep
Jan 3 at 16:19
I added both
import reactivemongo.play.json.ImplicitBSONHandlers
and import reactivemongo.play.json._
but I still get the same error.– Blankman
Jan 3 at 16:37
I added both
import reactivemongo.play.json.ImplicitBSONHandlers
and import reactivemongo.play.json._
but I still get the same error.– Blankman
Jan 3 at 16:37
Check the versions
– cchantep
Jan 3 at 16:38
Check the versions
– cchantep
Jan 3 at 16:38
@cchantep I added my versions to my question. I'm using reactivemongo 0.16.0-play26.
– Blankman
Jan 4 at 12:46
@cchantep I added my versions to my question. I'm using reactivemongo 0.16.0-play26.
– Blankman
Jan 4 at 12:46
add a comment |
1 Answer
1
active
oldest
votes
As far as I know, you do not NEED TO use BSONObjectID, but it would be recommended.
But one thins you should use "_id" field in mongo, otherwise the default behaviour will be to create an _id on your document when you create a new one... so your document will have _id and id.
So you should get id from "_id" and write it into "_id" even if your case class is id.
As per handlers, for basic case classes you can use their provided macros:
implicit val linkHandler: BSONDocumentHandler[Link] = Macros.handler
this will generate the Reader and Writer for your Link.
When using the macros, you have an annotations that you can use to highlight your id will be "_id" on mongo:
import reactivemongo.bson.Macros.Annotations.Key
case class Link(
@Key("_id")
id: Link.ID,
name: String,
url: String
)
But since I have my own handler in this case, why isn't it finding the implicit?
– Blankman
Jan 3 at 15:30
I realise you are using JSONCollection.. this is specific of Play... which I guess converts the BSON into JSON so you are not providing Json readers for thos documents... but not sure as I haven't use reactiveMongo with play, I use it in akka-http... sorry about that
– FerranJr
Jan 3 at 16:36
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54024490%2fmapping-a-case-class-to-a-mongodb-document-using-reactive-mongo%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
As far as I know, you do not NEED TO use BSONObjectID, but it would be recommended.
But one thins you should use "_id" field in mongo, otherwise the default behaviour will be to create an _id on your document when you create a new one... so your document will have _id and id.
So you should get id from "_id" and write it into "_id" even if your case class is id.
As per handlers, for basic case classes you can use their provided macros:
implicit val linkHandler: BSONDocumentHandler[Link] = Macros.handler
this will generate the Reader and Writer for your Link.
When using the macros, you have an annotations that you can use to highlight your id will be "_id" on mongo:
import reactivemongo.bson.Macros.Annotations.Key
case class Link(
@Key("_id")
id: Link.ID,
name: String,
url: String
)
But since I have my own handler in this case, why isn't it finding the implicit?
– Blankman
Jan 3 at 15:30
I realise you are using JSONCollection.. this is specific of Play... which I guess converts the BSON into JSON so you are not providing Json readers for thos documents... but not sure as I haven't use reactiveMongo with play, I use it in akka-http... sorry about that
– FerranJr
Jan 3 at 16:36
add a comment |
As far as I know, you do not NEED TO use BSONObjectID, but it would be recommended.
But one thins you should use "_id" field in mongo, otherwise the default behaviour will be to create an _id on your document when you create a new one... so your document will have _id and id.
So you should get id from "_id" and write it into "_id" even if your case class is id.
As per handlers, for basic case classes you can use their provided macros:
implicit val linkHandler: BSONDocumentHandler[Link] = Macros.handler
this will generate the Reader and Writer for your Link.
When using the macros, you have an annotations that you can use to highlight your id will be "_id" on mongo:
import reactivemongo.bson.Macros.Annotations.Key
case class Link(
@Key("_id")
id: Link.ID,
name: String,
url: String
)
But since I have my own handler in this case, why isn't it finding the implicit?
– Blankman
Jan 3 at 15:30
I realise you are using JSONCollection.. this is specific of Play... which I guess converts the BSON into JSON so you are not providing Json readers for thos documents... but not sure as I haven't use reactiveMongo with play, I use it in akka-http... sorry about that
– FerranJr
Jan 3 at 16:36
add a comment |
As far as I know, you do not NEED TO use BSONObjectID, but it would be recommended.
But one thins you should use "_id" field in mongo, otherwise the default behaviour will be to create an _id on your document when you create a new one... so your document will have _id and id.
So you should get id from "_id" and write it into "_id" even if your case class is id.
As per handlers, for basic case classes you can use their provided macros:
implicit val linkHandler: BSONDocumentHandler[Link] = Macros.handler
this will generate the Reader and Writer for your Link.
When using the macros, you have an annotations that you can use to highlight your id will be "_id" on mongo:
import reactivemongo.bson.Macros.Annotations.Key
case class Link(
@Key("_id")
id: Link.ID,
name: String,
url: String
)
As far as I know, you do not NEED TO use BSONObjectID, but it would be recommended.
But one thins you should use "_id" field in mongo, otherwise the default behaviour will be to create an _id on your document when you create a new one... so your document will have _id and id.
So you should get id from "_id" and write it into "_id" even if your case class is id.
As per handlers, for basic case classes you can use their provided macros:
implicit val linkHandler: BSONDocumentHandler[Link] = Macros.handler
this will generate the Reader and Writer for your Link.
When using the macros, you have an annotations that you can use to highlight your id will be "_id" on mongo:
import reactivemongo.bson.Macros.Annotations.Key
case class Link(
@Key("_id")
id: Link.ID,
name: String,
url: String
)
answered Jan 3 at 15:21
FerranJrFerranJr
31518
31518
But since I have my own handler in this case, why isn't it finding the implicit?
– Blankman
Jan 3 at 15:30
I realise you are using JSONCollection.. this is specific of Play... which I guess converts the BSON into JSON so you are not providing Json readers for thos documents... but not sure as I haven't use reactiveMongo with play, I use it in akka-http... sorry about that
– FerranJr
Jan 3 at 16:36
add a comment |
But since I have my own handler in this case, why isn't it finding the implicit?
– Blankman
Jan 3 at 15:30
I realise you are using JSONCollection.. this is specific of Play... which I guess converts the BSON into JSON so you are not providing Json readers for thos documents... but not sure as I haven't use reactiveMongo with play, I use it in akka-http... sorry about that
– FerranJr
Jan 3 at 16:36
But since I have my own handler in this case, why isn't it finding the implicit?
– Blankman
Jan 3 at 15:30
But since I have my own handler in this case, why isn't it finding the implicit?
– Blankman
Jan 3 at 15:30
I realise you are using JSONCollection.. this is specific of Play... which I guess converts the BSON into JSON so you are not providing Json readers for thos documents... but not sure as I haven't use reactiveMongo with play, I use it in akka-http... sorry about that
– FerranJr
Jan 3 at 16:36
I realise you are using JSONCollection.. this is specific of Play... which I guess converts the BSON into JSON so you are not providing Json readers for thos documents... but not sure as I haven't use reactiveMongo with play, I use it in akka-http... sorry about that
– FerranJr
Jan 3 at 16:36
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54024490%2fmapping-a-case-class-to-a-mongodb-document-using-reactive-mongo%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pZ LfXPKddTl
1
You're using a JSON collection, but the provided one are BSON, so you need to make sure conversions from
ImplicitBSONHandlers
is imported fromreactivemongo.play.json
.– cchantep
Jan 3 at 16:19
I added both
import reactivemongo.play.json.ImplicitBSONHandlers
andimport reactivemongo.play.json._
but I still get the same error.– Blankman
Jan 3 at 16:37
Check the versions
– cchantep
Jan 3 at 16:38
@cchantep I added my versions to my question. I'm using reactivemongo 0.16.0-play26.
– Blankman
Jan 4 at 12:46