How to fix MongoError: no geo indices for geoNear
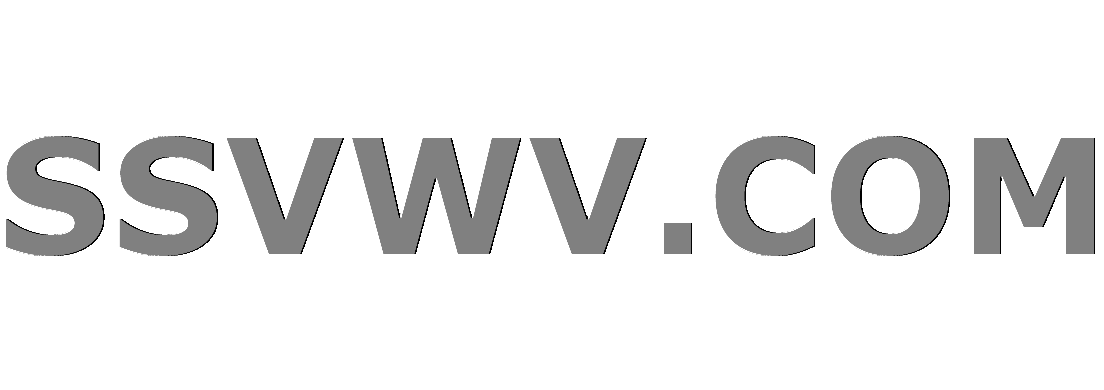
Multi tool use
I have a collection with a Geometry type. Now I would like, for a given point, get the nearest document. I am trying to use $geoNear operator with MongoDb. But in my callback I catch an error "MongoError: no geo indices for geoNear". I suppose than I have to define an index to my collection.
So I tried to launch this command :
$ db..createIndex({locationCoordinate:"2dsphere"});
But that doesn't change nothing.
This is my schema definition:
const MySchema = new mongoose.Schema({
logo: { type: String, required: true },
locationCoordinate: {
type: {
type: String,
enum: ['Point'],
default: 'Point'
},
coordinates: {
type: [Number],
required: true,
unique: true
}
},
name: { type: String, required: true }
}, {
writeConcern: 'majority',
});
And this is my aggregate function:
myModel.aggregate([
{
$geoNear: {
near: { type: "Point", coordinates: [ 17 , 65 ] },
distanceField: "distance",
spherical: true
}
}
], (err, results) => {
console.log("Err", err)
})
What I did wrong?
node.js mongoose
add a comment |
I have a collection with a Geometry type. Now I would like, for a given point, get the nearest document. I am trying to use $geoNear operator with MongoDb. But in my callback I catch an error "MongoError: no geo indices for geoNear". I suppose than I have to define an index to my collection.
So I tried to launch this command :
$ db..createIndex({locationCoordinate:"2dsphere"});
But that doesn't change nothing.
This is my schema definition:
const MySchema = new mongoose.Schema({
logo: { type: String, required: true },
locationCoordinate: {
type: {
type: String,
enum: ['Point'],
default: 'Point'
},
coordinates: {
type: [Number],
required: true,
unique: true
}
},
name: { type: String, required: true }
}, {
writeConcern: 'majority',
});
And this is my aggregate function:
myModel.aggregate([
{
$geoNear: {
near: { type: "Point", coordinates: [ 17 , 65 ] },
distanceField: "distance",
spherical: true
}
}
], (err, results) => {
console.log("Err", err)
})
What I did wrong?
node.js mongoose
add a comment |
I have a collection with a Geometry type. Now I would like, for a given point, get the nearest document. I am trying to use $geoNear operator with MongoDb. But in my callback I catch an error "MongoError: no geo indices for geoNear". I suppose than I have to define an index to my collection.
So I tried to launch this command :
$ db..createIndex({locationCoordinate:"2dsphere"});
But that doesn't change nothing.
This is my schema definition:
const MySchema = new mongoose.Schema({
logo: { type: String, required: true },
locationCoordinate: {
type: {
type: String,
enum: ['Point'],
default: 'Point'
},
coordinates: {
type: [Number],
required: true,
unique: true
}
},
name: { type: String, required: true }
}, {
writeConcern: 'majority',
});
And this is my aggregate function:
myModel.aggregate([
{
$geoNear: {
near: { type: "Point", coordinates: [ 17 , 65 ] },
distanceField: "distance",
spherical: true
}
}
], (err, results) => {
console.log("Err", err)
})
What I did wrong?
node.js mongoose
I have a collection with a Geometry type. Now I would like, for a given point, get the nearest document. I am trying to use $geoNear operator with MongoDb. But in my callback I catch an error "MongoError: no geo indices for geoNear". I suppose than I have to define an index to my collection.
So I tried to launch this command :
$ db..createIndex({locationCoordinate:"2dsphere"});
But that doesn't change nothing.
This is my schema definition:
const MySchema = new mongoose.Schema({
logo: { type: String, required: true },
locationCoordinate: {
type: {
type: String,
enum: ['Point'],
default: 'Point'
},
coordinates: {
type: [Number],
required: true,
unique: true
}
},
name: { type: String, required: true }
}, {
writeConcern: 'majority',
});
And this is my aggregate function:
myModel.aggregate([
{
$geoNear: {
near: { type: "Point", coordinates: [ 17 , 65 ] },
distanceField: "distance",
spherical: true
}
}
], (err, results) => {
console.log("Err", err)
})
What I did wrong?
node.js mongoose
node.js mongoose
asked Jan 3 at 14:45
popideppopidep
73
73
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can wait for the index like this:
function waitForIndex() {
return new Promise((resolve, reject) => {
YourModel.on('index', error => error ? reject(error) : resolve());
});
}
And then perform the async operations.
Thanks. I add waitForIndex function and I execute myModel.agregate when waitForIndex is finished but I still have the same error.
– popidep
Jan 3 at 15:54
you should use it before doing the aggregation. i.emyModel.save().then(waitForIndex).then(aggregate).catch(err=> ...)
@popidep
– Adonis Murati
Jan 3 at 19:30
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54024555%2fhow-to-fix-mongoerror-no-geo-indices-for-geonear%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can wait for the index like this:
function waitForIndex() {
return new Promise((resolve, reject) => {
YourModel.on('index', error => error ? reject(error) : resolve());
});
}
And then perform the async operations.
Thanks. I add waitForIndex function and I execute myModel.agregate when waitForIndex is finished but I still have the same error.
– popidep
Jan 3 at 15:54
you should use it before doing the aggregation. i.emyModel.save().then(waitForIndex).then(aggregate).catch(err=> ...)
@popidep
– Adonis Murati
Jan 3 at 19:30
add a comment |
You can wait for the index like this:
function waitForIndex() {
return new Promise((resolve, reject) => {
YourModel.on('index', error => error ? reject(error) : resolve());
});
}
And then perform the async operations.
Thanks. I add waitForIndex function and I execute myModel.agregate when waitForIndex is finished but I still have the same error.
– popidep
Jan 3 at 15:54
you should use it before doing the aggregation. i.emyModel.save().then(waitForIndex).then(aggregate).catch(err=> ...)
@popidep
– Adonis Murati
Jan 3 at 19:30
add a comment |
You can wait for the index like this:
function waitForIndex() {
return new Promise((resolve, reject) => {
YourModel.on('index', error => error ? reject(error) : resolve());
});
}
And then perform the async operations.
You can wait for the index like this:
function waitForIndex() {
return new Promise((resolve, reject) => {
YourModel.on('index', error => error ? reject(error) : resolve());
});
}
And then perform the async operations.
edited Jan 3 at 15:04
answered Jan 3 at 14:57
Adonis MuratiAdonis Murati
716
716
Thanks. I add waitForIndex function and I execute myModel.agregate when waitForIndex is finished but I still have the same error.
– popidep
Jan 3 at 15:54
you should use it before doing the aggregation. i.emyModel.save().then(waitForIndex).then(aggregate).catch(err=> ...)
@popidep
– Adonis Murati
Jan 3 at 19:30
add a comment |
Thanks. I add waitForIndex function and I execute myModel.agregate when waitForIndex is finished but I still have the same error.
– popidep
Jan 3 at 15:54
you should use it before doing the aggregation. i.emyModel.save().then(waitForIndex).then(aggregate).catch(err=> ...)
@popidep
– Adonis Murati
Jan 3 at 19:30
Thanks. I add waitForIndex function and I execute myModel.agregate when waitForIndex is finished but I still have the same error.
– popidep
Jan 3 at 15:54
Thanks. I add waitForIndex function and I execute myModel.agregate when waitForIndex is finished but I still have the same error.
– popidep
Jan 3 at 15:54
you should use it before doing the aggregation. i.e
myModel.save().then(waitForIndex).then(aggregate).catch(err=> ...)
@popidep– Adonis Murati
Jan 3 at 19:30
you should use it before doing the aggregation. i.e
myModel.save().then(waitForIndex).then(aggregate).catch(err=> ...)
@popidep– Adonis Murati
Jan 3 at 19:30
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54024555%2fhow-to-fix-mongoerror-no-geo-indices-for-geonear%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0kE407b,xqk