NodeJS (JavaScript) - Fastest method to convert date?
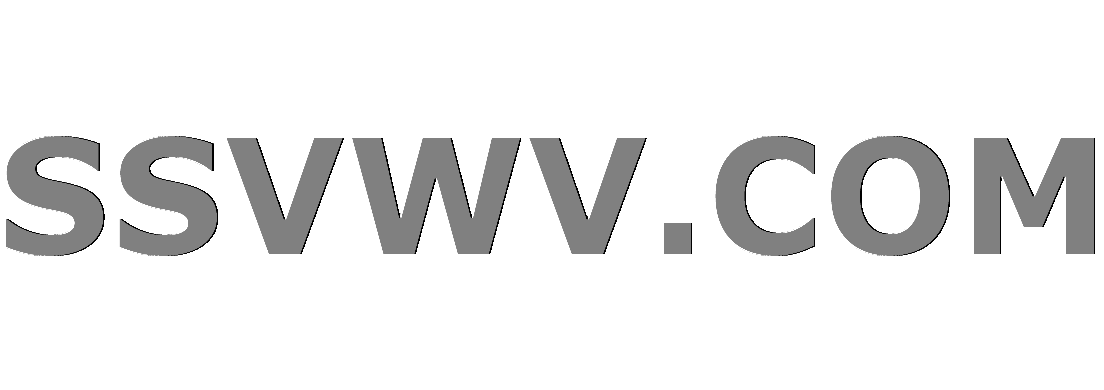
Multi tool use
In NodeJS, I'm reading and parsing a .txt file with more than 1.5M lines. Each line is in the format: date,number,number
, where each date is yyyyMMddhhmmss
. An example line is:
20170506014255,100.01,200.02
Using jFile, I can read and parse all 1.5M+ lines in about 2 seconds...
var jFile = require('jfile');
var data = ;
var dataFile = new jFile('./dataFile.txt');
dataFile.lines.forEach(function(line) {
data.push(line.split(','));
});
Works great! But, I'd like to change the date format to something else. For this, I am using date-format to do the conversion...
... same as previous, with new line within forEach() ...
var dateFormatter = require('date-format');
dataFile.lines.forEach(function(line) {
let tdata = line.split(',');
tdata[0] = dateFormatter('MM/dd/yy hh:mm:ss', dateFormatter.parse('yyyyMMddhhmmss', tdata[0]));
data.push(tdata);
});
Again, this works great! Mostly. What once took only 2 seconds now takes about 25 seconds. Gasp!
Ideally, the file would just have the dates formatted the correct way, but this is out of my hands.
Is there a faster way to do this conversion? Maybe there is a more native approach, or just a faster package?
Thanks for any insight!
javascript node.js date date-formatting
add a comment |
In NodeJS, I'm reading and parsing a .txt file with more than 1.5M lines. Each line is in the format: date,number,number
, where each date is yyyyMMddhhmmss
. An example line is:
20170506014255,100.01,200.02
Using jFile, I can read and parse all 1.5M+ lines in about 2 seconds...
var jFile = require('jfile');
var data = ;
var dataFile = new jFile('./dataFile.txt');
dataFile.lines.forEach(function(line) {
data.push(line.split(','));
});
Works great! But, I'd like to change the date format to something else. For this, I am using date-format to do the conversion...
... same as previous, with new line within forEach() ...
var dateFormatter = require('date-format');
dataFile.lines.forEach(function(line) {
let tdata = line.split(',');
tdata[0] = dateFormatter('MM/dd/yy hh:mm:ss', dateFormatter.parse('yyyyMMddhhmmss', tdata[0]));
data.push(tdata);
});
Again, this works great! Mostly. What once took only 2 seconds now takes about 25 seconds. Gasp!
Ideally, the file would just have the dates formatted the correct way, but this is out of my hands.
Is there a faster way to do this conversion? Maybe there is a more native approach, or just a faster package?
Thanks for any insight!
javascript node.js date date-formatting
add a comment |
In NodeJS, I'm reading and parsing a .txt file with more than 1.5M lines. Each line is in the format: date,number,number
, where each date is yyyyMMddhhmmss
. An example line is:
20170506014255,100.01,200.02
Using jFile, I can read and parse all 1.5M+ lines in about 2 seconds...
var jFile = require('jfile');
var data = ;
var dataFile = new jFile('./dataFile.txt');
dataFile.lines.forEach(function(line) {
data.push(line.split(','));
});
Works great! But, I'd like to change the date format to something else. For this, I am using date-format to do the conversion...
... same as previous, with new line within forEach() ...
var dateFormatter = require('date-format');
dataFile.lines.forEach(function(line) {
let tdata = line.split(',');
tdata[0] = dateFormatter('MM/dd/yy hh:mm:ss', dateFormatter.parse('yyyyMMddhhmmss', tdata[0]));
data.push(tdata);
});
Again, this works great! Mostly. What once took only 2 seconds now takes about 25 seconds. Gasp!
Ideally, the file would just have the dates formatted the correct way, but this is out of my hands.
Is there a faster way to do this conversion? Maybe there is a more native approach, or just a faster package?
Thanks for any insight!
javascript node.js date date-formatting
In NodeJS, I'm reading and parsing a .txt file with more than 1.5M lines. Each line is in the format: date,number,number
, where each date is yyyyMMddhhmmss
. An example line is:
20170506014255,100.01,200.02
Using jFile, I can read and parse all 1.5M+ lines in about 2 seconds...
var jFile = require('jfile');
var data = ;
var dataFile = new jFile('./dataFile.txt');
dataFile.lines.forEach(function(line) {
data.push(line.split(','));
});
Works great! But, I'd like to change the date format to something else. For this, I am using date-format to do the conversion...
... same as previous, with new line within forEach() ...
var dateFormatter = require('date-format');
dataFile.lines.forEach(function(line) {
let tdata = line.split(',');
tdata[0] = dateFormatter('MM/dd/yy hh:mm:ss', dateFormatter.parse('yyyyMMddhhmmss', tdata[0]));
data.push(tdata);
});
Again, this works great! Mostly. What once took only 2 seconds now takes about 25 seconds. Gasp!
Ideally, the file would just have the dates formatted the correct way, but this is out of my hands.
Is there a faster way to do this conversion? Maybe there is a more native approach, or just a faster package?
Thanks for any insight!
javascript node.js date date-formatting
javascript node.js date date-formatting
asked Dec 30 '18 at 23:56


BirrelBirrel
2,67742342
2,67742342
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
I guess it is faster to hardcode the parsing:
function fixFormat(date) {
const yy = date.slice(2, 4);
const MM = date.slice(4, 6);
const dd = date.slice(6, 8);
const hh = date.slice(8, 10);
const mm = date.slice(10, 12);
const ss = date.slice(12, 14);
return `${MM}/${dd}/${yy} ${hh}:${mm}:${ss}`;
}
Or really really ugly:
const y = 2, M = 4, d = 6, h = 8, m = 10, s = 12;
const pattern = fn => date => fn(p => date[p] + date[p + 1])
const fixFormat = pattern(p => p(M) + "/" + p(d) + "/" + p(y) + " " + p(h) + ":" + p(m) + ":" + p(s));
1
Boom! Only adds one second of processing time, for the entire set. I'm confident that every date will be formatted identically, so this is ideal. Thanks a tonne! I'll still accept your answer, but you haveyyyy
up top andyy
in the return. I just changed the first parse toconst yy = date.slice(2, 4);
, since I'm only interested in the last two characters anyway. Great fix though!
– Birrel
Dec 31 '18 at 0:10
Might a single regex match be faster than 6 consecutive calls to .slice()? Would have to run some benchmarks. /[0-9]{2,2}([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})/
– Eric Vautier
Dec 31 '18 at 0:24
@EricVautier—yes, but the regular expression can be very much simpler if combined with match. ;-)
– RobG
Jan 2 at 1:35
add a comment |
It seems you want to play a game of coding golf to see who can write the fastest code for you.
Anything that parses the string, creates a Date, then generates a string from if it going to be slower than something that just reformats the string. Jonas' approach is valid, and probably fast enough, but all those slices must take their toll. A single match should be faster, but it's up to you:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
function formatDate(s) {
var b = s.match(/dd/g);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
}
console.log(formatDate('20170506014255'));
I have no idea if that's faster, but it's certainly a lot less code. If you really want to make it fast, create the regular expression once:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
var formatDate = (function() {
var re = /dd/g;
return function (s) {
var b = s.match(re);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
};
}());
console.log(formatDate('20170506014255'));
We just need a testcase to see who wins ... :)
– Jonas Wilms
Jan 2 at 6:49
@JonasWilms—https://jsperf.com/reformat-date. It identified MS Edge as Chrome 64. Seems string operations are highly optimised. Unless you're running Edge…
– RobG
Jan 2 at 9:35
and I should definetly update my Firefox ... the next version seems to double the overall speed (or my cpu is just slow), however this seems like a clear win :)
– Jonas Wilms
Jan 2 at 11:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982415%2fnodejs-javascript-fastest-method-to-convert-date%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I guess it is faster to hardcode the parsing:
function fixFormat(date) {
const yy = date.slice(2, 4);
const MM = date.slice(4, 6);
const dd = date.slice(6, 8);
const hh = date.slice(8, 10);
const mm = date.slice(10, 12);
const ss = date.slice(12, 14);
return `${MM}/${dd}/${yy} ${hh}:${mm}:${ss}`;
}
Or really really ugly:
const y = 2, M = 4, d = 6, h = 8, m = 10, s = 12;
const pattern = fn => date => fn(p => date[p] + date[p + 1])
const fixFormat = pattern(p => p(M) + "/" + p(d) + "/" + p(y) + " " + p(h) + ":" + p(m) + ":" + p(s));
1
Boom! Only adds one second of processing time, for the entire set. I'm confident that every date will be formatted identically, so this is ideal. Thanks a tonne! I'll still accept your answer, but you haveyyyy
up top andyy
in the return. I just changed the first parse toconst yy = date.slice(2, 4);
, since I'm only interested in the last two characters anyway. Great fix though!
– Birrel
Dec 31 '18 at 0:10
Might a single regex match be faster than 6 consecutive calls to .slice()? Would have to run some benchmarks. /[0-9]{2,2}([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})/
– Eric Vautier
Dec 31 '18 at 0:24
@EricVautier—yes, but the regular expression can be very much simpler if combined with match. ;-)
– RobG
Jan 2 at 1:35
add a comment |
I guess it is faster to hardcode the parsing:
function fixFormat(date) {
const yy = date.slice(2, 4);
const MM = date.slice(4, 6);
const dd = date.slice(6, 8);
const hh = date.slice(8, 10);
const mm = date.slice(10, 12);
const ss = date.slice(12, 14);
return `${MM}/${dd}/${yy} ${hh}:${mm}:${ss}`;
}
Or really really ugly:
const y = 2, M = 4, d = 6, h = 8, m = 10, s = 12;
const pattern = fn => date => fn(p => date[p] + date[p + 1])
const fixFormat = pattern(p => p(M) + "/" + p(d) + "/" + p(y) + " " + p(h) + ":" + p(m) + ":" + p(s));
1
Boom! Only adds one second of processing time, for the entire set. I'm confident that every date will be formatted identically, so this is ideal. Thanks a tonne! I'll still accept your answer, but you haveyyyy
up top andyy
in the return. I just changed the first parse toconst yy = date.slice(2, 4);
, since I'm only interested in the last two characters anyway. Great fix though!
– Birrel
Dec 31 '18 at 0:10
Might a single regex match be faster than 6 consecutive calls to .slice()? Would have to run some benchmarks. /[0-9]{2,2}([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})/
– Eric Vautier
Dec 31 '18 at 0:24
@EricVautier—yes, but the regular expression can be very much simpler if combined with match. ;-)
– RobG
Jan 2 at 1:35
add a comment |
I guess it is faster to hardcode the parsing:
function fixFormat(date) {
const yy = date.slice(2, 4);
const MM = date.slice(4, 6);
const dd = date.slice(6, 8);
const hh = date.slice(8, 10);
const mm = date.slice(10, 12);
const ss = date.slice(12, 14);
return `${MM}/${dd}/${yy} ${hh}:${mm}:${ss}`;
}
Or really really ugly:
const y = 2, M = 4, d = 6, h = 8, m = 10, s = 12;
const pattern = fn => date => fn(p => date[p] + date[p + 1])
const fixFormat = pattern(p => p(M) + "/" + p(d) + "/" + p(y) + " " + p(h) + ":" + p(m) + ":" + p(s));
I guess it is faster to hardcode the parsing:
function fixFormat(date) {
const yy = date.slice(2, 4);
const MM = date.slice(4, 6);
const dd = date.slice(6, 8);
const hh = date.slice(8, 10);
const mm = date.slice(10, 12);
const ss = date.slice(12, 14);
return `${MM}/${dd}/${yy} ${hh}:${mm}:${ss}`;
}
Or really really ugly:
const y = 2, M = 4, d = 6, h = 8, m = 10, s = 12;
const pattern = fn => date => fn(p => date[p] + date[p + 1])
const fixFormat = pattern(p => p(M) + "/" + p(d) + "/" + p(y) + " " + p(h) + ":" + p(m) + ":" + p(s));
edited Jan 2 at 6:48
answered Dec 31 '18 at 0:04
Jonas WilmsJonas Wilms
57.1k43051
57.1k43051
1
Boom! Only adds one second of processing time, for the entire set. I'm confident that every date will be formatted identically, so this is ideal. Thanks a tonne! I'll still accept your answer, but you haveyyyy
up top andyy
in the return. I just changed the first parse toconst yy = date.slice(2, 4);
, since I'm only interested in the last two characters anyway. Great fix though!
– Birrel
Dec 31 '18 at 0:10
Might a single regex match be faster than 6 consecutive calls to .slice()? Would have to run some benchmarks. /[0-9]{2,2}([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})/
– Eric Vautier
Dec 31 '18 at 0:24
@EricVautier—yes, but the regular expression can be very much simpler if combined with match. ;-)
– RobG
Jan 2 at 1:35
add a comment |
1
Boom! Only adds one second of processing time, for the entire set. I'm confident that every date will be formatted identically, so this is ideal. Thanks a tonne! I'll still accept your answer, but you haveyyyy
up top andyy
in the return. I just changed the first parse toconst yy = date.slice(2, 4);
, since I'm only interested in the last two characters anyway. Great fix though!
– Birrel
Dec 31 '18 at 0:10
Might a single regex match be faster than 6 consecutive calls to .slice()? Would have to run some benchmarks. /[0-9]{2,2}([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})/
– Eric Vautier
Dec 31 '18 at 0:24
@EricVautier—yes, but the regular expression can be very much simpler if combined with match. ;-)
– RobG
Jan 2 at 1:35
1
1
Boom! Only adds one second of processing time, for the entire set. I'm confident that every date will be formatted identically, so this is ideal. Thanks a tonne! I'll still accept your answer, but you have
yyyy
up top and yy
in the return. I just changed the first parse to const yy = date.slice(2, 4);
, since I'm only interested in the last two characters anyway. Great fix though!– Birrel
Dec 31 '18 at 0:10
Boom! Only adds one second of processing time, for the entire set. I'm confident that every date will be formatted identically, so this is ideal. Thanks a tonne! I'll still accept your answer, but you have
yyyy
up top and yy
in the return. I just changed the first parse to const yy = date.slice(2, 4);
, since I'm only interested in the last two characters anyway. Great fix though!– Birrel
Dec 31 '18 at 0:10
Might a single regex match be faster than 6 consecutive calls to .slice()? Would have to run some benchmarks. /[0-9]{2,2}([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})/
– Eric Vautier
Dec 31 '18 at 0:24
Might a single regex match be faster than 6 consecutive calls to .slice()? Would have to run some benchmarks. /[0-9]{2,2}([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})([0-9]{2,2})/
– Eric Vautier
Dec 31 '18 at 0:24
@EricVautier—yes, but the regular expression can be very much simpler if combined with match. ;-)
– RobG
Jan 2 at 1:35
@EricVautier—yes, but the regular expression can be very much simpler if combined with match. ;-)
– RobG
Jan 2 at 1:35
add a comment |
It seems you want to play a game of coding golf to see who can write the fastest code for you.
Anything that parses the string, creates a Date, then generates a string from if it going to be slower than something that just reformats the string. Jonas' approach is valid, and probably fast enough, but all those slices must take their toll. A single match should be faster, but it's up to you:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
function formatDate(s) {
var b = s.match(/dd/g);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
}
console.log(formatDate('20170506014255'));
I have no idea if that's faster, but it's certainly a lot less code. If you really want to make it fast, create the regular expression once:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
var formatDate = (function() {
var re = /dd/g;
return function (s) {
var b = s.match(re);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
};
}());
console.log(formatDate('20170506014255'));
We just need a testcase to see who wins ... :)
– Jonas Wilms
Jan 2 at 6:49
@JonasWilms—https://jsperf.com/reformat-date. It identified MS Edge as Chrome 64. Seems string operations are highly optimised. Unless you're running Edge…
– RobG
Jan 2 at 9:35
and I should definetly update my Firefox ... the next version seems to double the overall speed (or my cpu is just slow), however this seems like a clear win :)
– Jonas Wilms
Jan 2 at 11:50
add a comment |
It seems you want to play a game of coding golf to see who can write the fastest code for you.
Anything that parses the string, creates a Date, then generates a string from if it going to be slower than something that just reformats the string. Jonas' approach is valid, and probably fast enough, but all those slices must take their toll. A single match should be faster, but it's up to you:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
function formatDate(s) {
var b = s.match(/dd/g);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
}
console.log(formatDate('20170506014255'));
I have no idea if that's faster, but it's certainly a lot less code. If you really want to make it fast, create the regular expression once:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
var formatDate = (function() {
var re = /dd/g;
return function (s) {
var b = s.match(re);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
};
}());
console.log(formatDate('20170506014255'));
We just need a testcase to see who wins ... :)
– Jonas Wilms
Jan 2 at 6:49
@JonasWilms—https://jsperf.com/reformat-date. It identified MS Edge as Chrome 64. Seems string operations are highly optimised. Unless you're running Edge…
– RobG
Jan 2 at 9:35
and I should definetly update my Firefox ... the next version seems to double the overall speed (or my cpu is just slow), however this seems like a clear win :)
– Jonas Wilms
Jan 2 at 11:50
add a comment |
It seems you want to play a game of coding golf to see who can write the fastest code for you.
Anything that parses the string, creates a Date, then generates a string from if it going to be slower than something that just reformats the string. Jonas' approach is valid, and probably fast enough, but all those slices must take their toll. A single match should be faster, but it's up to you:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
function formatDate(s) {
var b = s.match(/dd/g);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
}
console.log(formatDate('20170506014255'));
I have no idea if that's faster, but it's certainly a lot less code. If you really want to make it fast, create the regular expression once:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
var formatDate = (function() {
var re = /dd/g;
return function (s) {
var b = s.match(re);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
};
}());
console.log(formatDate('20170506014255'));
It seems you want to play a game of coding golf to see who can write the fastest code for you.
Anything that parses the string, creates a Date, then generates a string from if it going to be slower than something that just reformats the string. Jonas' approach is valid, and probably fast enough, but all those slices must take their toll. A single match should be faster, but it's up to you:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
function formatDate(s) {
var b = s.match(/dd/g);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
}
console.log(formatDate('20170506014255'));
I have no idea if that's faster, but it's certainly a lot less code. If you really want to make it fast, create the regular expression once:
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
var formatDate = (function() {
var re = /dd/g;
return function (s) {
var b = s.match(re);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
};
}());
console.log(formatDate('20170506014255'));
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
function formatDate(s) {
var b = s.match(/dd/g);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
}
console.log(formatDate('20170506014255'));
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
function formatDate(s) {
var b = s.match(/dd/g);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
}
console.log(formatDate('20170506014255'));
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
var formatDate = (function() {
var re = /dd/g;
return function (s) {
var b = s.match(re);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
};
}());
console.log(formatDate('20170506014255'));
// Convert a timestamp in yyyyMMddhhmmss format to MM/dd/yy hh:mm:ss
// where "hh" is assumed to be 24 hr
var formatDate = (function() {
var re = /dd/g;
return function (s) {
var b = s.match(re);
return `${b[2]}/${b[3]}/${b[1]} ${b[4]}:${b[5]}:${b[6]}`;
};
}());
console.log(formatDate('20170506014255'));
answered Jan 2 at 1:31
RobGRobG
97.9k19106145
97.9k19106145
We just need a testcase to see who wins ... :)
– Jonas Wilms
Jan 2 at 6:49
@JonasWilms—https://jsperf.com/reformat-date. It identified MS Edge as Chrome 64. Seems string operations are highly optimised. Unless you're running Edge…
– RobG
Jan 2 at 9:35
and I should definetly update my Firefox ... the next version seems to double the overall speed (or my cpu is just slow), however this seems like a clear win :)
– Jonas Wilms
Jan 2 at 11:50
add a comment |
We just need a testcase to see who wins ... :)
– Jonas Wilms
Jan 2 at 6:49
@JonasWilms—https://jsperf.com/reformat-date. It identified MS Edge as Chrome 64. Seems string operations are highly optimised. Unless you're running Edge…
– RobG
Jan 2 at 9:35
and I should definetly update my Firefox ... the next version seems to double the overall speed (or my cpu is just slow), however this seems like a clear win :)
– Jonas Wilms
Jan 2 at 11:50
We just need a testcase to see who wins ... :)
– Jonas Wilms
Jan 2 at 6:49
We just need a testcase to see who wins ... :)
– Jonas Wilms
Jan 2 at 6:49
@JonasWilms—https://jsperf.com/reformat-date. It identified MS Edge as Chrome 64. Seems string operations are highly optimised. Unless you're running Edge…
– RobG
Jan 2 at 9:35
@JonasWilms—https://jsperf.com/reformat-date. It identified MS Edge as Chrome 64. Seems string operations are highly optimised. Unless you're running Edge…
– RobG
Jan 2 at 9:35
and I should definetly update my Firefox ... the next version seems to double the overall speed (or my cpu is just slow), however this seems like a clear win :)
– Jonas Wilms
Jan 2 at 11:50
and I should definetly update my Firefox ... the next version seems to double the overall speed (or my cpu is just slow), however this seems like a clear win :)
– Jonas Wilms
Jan 2 at 11:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982415%2fnodejs-javascript-fastest-method-to-convert-date%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w,J4pL5,TCbaApNV,b,nn isPKD7dF,JJDN3fAEBgp0