How do I change my encryption code so that it will only encrypt the given string, and not encrypt what was...
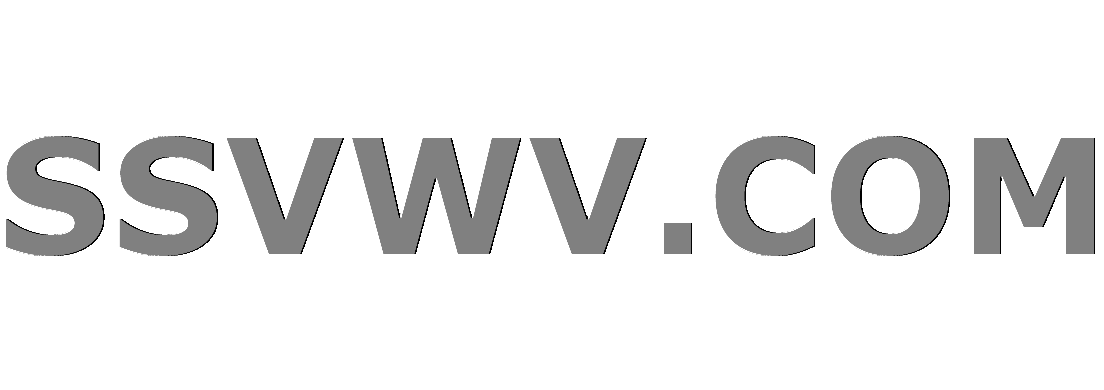
Multi tool use
My code encrypts the previously encrypted phrase in an inputted sentence. I want it so that it encrypts only the letters v, m, g, and b in the original string, not the previously encrypted letters, if that makes any sense. So with a string such as "Very good", it would encrypt the letter v into the correct phrase, but then it would find the letter 'b' within that encrypted phrase, then further encrypt it, which is want I don't want. And obviously the decryption wouldn't work as well, because it needs the specific phrase to turn it back into the correct letter, but it can't because the phrase has been encrypted even further than it should have.
I've tried to change the order of what letter gets encrypted first, hoping that it would avoid conflicts, but that hasn't worked at all.
Crypto Class
public String encryptdSntnc;
public String decryptdSntnc;
public String sntnc;
public String encrypt(String sntnc)
{
String encrypt1 = sntnc.replace("v", "ag',r");
String encrypt2 = encrypt1.replace("V", "ag',r");
String encrypt3 = encrypt2.replace("m", "ssad");
String encrypt4 = encrypt3.replace("M", "ssad");
String encrypt5 = encrypt4.replace("g", "jeb..w");
String encrypt6 = encrypt5.replace("G", "jeb..w");
String encrypt7 = encrypt6.replace("b", "dug>?/");
encryptdSntnc = encrypt7.replace("B", "dug>?/");
return encryptdSntnc;
}
public String decrypt(String encryptdSntnc)
{
String decrypt1 = encryptdSntnc.replace("dug>?/", "B");
String decrypt2 = decrypt1.replace("dug>?/", "b");
String decrypt3 = decrypt2.replace("jeb..w", "G");
String decrypt4 = decrypt3.replace("jeb..w", "g");
String decrypt5 = decrypt4.replace("ssad", "M");
String decrypt6 = decrypt5.replace("ssad", "m");
String decrypt7 = decrypt6.replace("ag',r", "V");
decryptdSntnc = decrypt7.replace("ag',r", "v");
return decryptdSntnc;
}
Tester Class
Scanner kbReader = new Scanner(System.in);
System.out.print("Enter a sentence that is to be encrypted: ");
String sntnc = kbReader.nextLine();
Crypto myCryptObj = new Crypto();
String encryptdSntnc = myCryptObj.encrypt(sntnc);
System.out.println("Encrypted sentence = " + encryptdSntnc);
String decryptdSntnc = myCryptObj.decrypt(encryptdSntnc);
System.out.println("Decrypted sentence = " + decryptdSntnc);
What I expect is if you type in "Very good", the encrypted sentence would be "ag',rery jeb..wood", and the decrypted sentence would be "Very good".
But instead, I get "ajedug>?/..w',rery jedug>?/..wood" for the encryption, and "ajeB..w',rery jeB..wood" for the decryption, using the same "Very good".
java
add a comment |
My code encrypts the previously encrypted phrase in an inputted sentence. I want it so that it encrypts only the letters v, m, g, and b in the original string, not the previously encrypted letters, if that makes any sense. So with a string such as "Very good", it would encrypt the letter v into the correct phrase, but then it would find the letter 'b' within that encrypted phrase, then further encrypt it, which is want I don't want. And obviously the decryption wouldn't work as well, because it needs the specific phrase to turn it back into the correct letter, but it can't because the phrase has been encrypted even further than it should have.
I've tried to change the order of what letter gets encrypted first, hoping that it would avoid conflicts, but that hasn't worked at all.
Crypto Class
public String encryptdSntnc;
public String decryptdSntnc;
public String sntnc;
public String encrypt(String sntnc)
{
String encrypt1 = sntnc.replace("v", "ag',r");
String encrypt2 = encrypt1.replace("V", "ag',r");
String encrypt3 = encrypt2.replace("m", "ssad");
String encrypt4 = encrypt3.replace("M", "ssad");
String encrypt5 = encrypt4.replace("g", "jeb..w");
String encrypt6 = encrypt5.replace("G", "jeb..w");
String encrypt7 = encrypt6.replace("b", "dug>?/");
encryptdSntnc = encrypt7.replace("B", "dug>?/");
return encryptdSntnc;
}
public String decrypt(String encryptdSntnc)
{
String decrypt1 = encryptdSntnc.replace("dug>?/", "B");
String decrypt2 = decrypt1.replace("dug>?/", "b");
String decrypt3 = decrypt2.replace("jeb..w", "G");
String decrypt4 = decrypt3.replace("jeb..w", "g");
String decrypt5 = decrypt4.replace("ssad", "M");
String decrypt6 = decrypt5.replace("ssad", "m");
String decrypt7 = decrypt6.replace("ag',r", "V");
decryptdSntnc = decrypt7.replace("ag',r", "v");
return decryptdSntnc;
}
Tester Class
Scanner kbReader = new Scanner(System.in);
System.out.print("Enter a sentence that is to be encrypted: ");
String sntnc = kbReader.nextLine();
Crypto myCryptObj = new Crypto();
String encryptdSntnc = myCryptObj.encrypt(sntnc);
System.out.println("Encrypted sentence = " + encryptdSntnc);
String decryptdSntnc = myCryptObj.decrypt(encryptdSntnc);
System.out.println("Decrypted sentence = " + decryptdSntnc);
What I expect is if you type in "Very good", the encrypted sentence would be "ag',rery jeb..wood", and the decrypted sentence would be "Very good".
But instead, I get "ajedug>?/..w',rery jedug>?/..wood" for the encryption, and "ajeB..w',rery jeB..wood" for the decryption, using the same "Very good".
java
Did you try a loop instead of String.replace?
– SHoko
Dec 30 '18 at 23:33
No, I haven't actually. How would I go about doing that?
– Nathan Kim
Dec 30 '18 at 23:53
add a comment |
My code encrypts the previously encrypted phrase in an inputted sentence. I want it so that it encrypts only the letters v, m, g, and b in the original string, not the previously encrypted letters, if that makes any sense. So with a string such as "Very good", it would encrypt the letter v into the correct phrase, but then it would find the letter 'b' within that encrypted phrase, then further encrypt it, which is want I don't want. And obviously the decryption wouldn't work as well, because it needs the specific phrase to turn it back into the correct letter, but it can't because the phrase has been encrypted even further than it should have.
I've tried to change the order of what letter gets encrypted first, hoping that it would avoid conflicts, but that hasn't worked at all.
Crypto Class
public String encryptdSntnc;
public String decryptdSntnc;
public String sntnc;
public String encrypt(String sntnc)
{
String encrypt1 = sntnc.replace("v", "ag',r");
String encrypt2 = encrypt1.replace("V", "ag',r");
String encrypt3 = encrypt2.replace("m", "ssad");
String encrypt4 = encrypt3.replace("M", "ssad");
String encrypt5 = encrypt4.replace("g", "jeb..w");
String encrypt6 = encrypt5.replace("G", "jeb..w");
String encrypt7 = encrypt6.replace("b", "dug>?/");
encryptdSntnc = encrypt7.replace("B", "dug>?/");
return encryptdSntnc;
}
public String decrypt(String encryptdSntnc)
{
String decrypt1 = encryptdSntnc.replace("dug>?/", "B");
String decrypt2 = decrypt1.replace("dug>?/", "b");
String decrypt3 = decrypt2.replace("jeb..w", "G");
String decrypt4 = decrypt3.replace("jeb..w", "g");
String decrypt5 = decrypt4.replace("ssad", "M");
String decrypt6 = decrypt5.replace("ssad", "m");
String decrypt7 = decrypt6.replace("ag',r", "V");
decryptdSntnc = decrypt7.replace("ag',r", "v");
return decryptdSntnc;
}
Tester Class
Scanner kbReader = new Scanner(System.in);
System.out.print("Enter a sentence that is to be encrypted: ");
String sntnc = kbReader.nextLine();
Crypto myCryptObj = new Crypto();
String encryptdSntnc = myCryptObj.encrypt(sntnc);
System.out.println("Encrypted sentence = " + encryptdSntnc);
String decryptdSntnc = myCryptObj.decrypt(encryptdSntnc);
System.out.println("Decrypted sentence = " + decryptdSntnc);
What I expect is if you type in "Very good", the encrypted sentence would be "ag',rery jeb..wood", and the decrypted sentence would be "Very good".
But instead, I get "ajedug>?/..w',rery jedug>?/..wood" for the encryption, and "ajeB..w',rery jeB..wood" for the decryption, using the same "Very good".
java
My code encrypts the previously encrypted phrase in an inputted sentence. I want it so that it encrypts only the letters v, m, g, and b in the original string, not the previously encrypted letters, if that makes any sense. So with a string such as "Very good", it would encrypt the letter v into the correct phrase, but then it would find the letter 'b' within that encrypted phrase, then further encrypt it, which is want I don't want. And obviously the decryption wouldn't work as well, because it needs the specific phrase to turn it back into the correct letter, but it can't because the phrase has been encrypted even further than it should have.
I've tried to change the order of what letter gets encrypted first, hoping that it would avoid conflicts, but that hasn't worked at all.
Crypto Class
public String encryptdSntnc;
public String decryptdSntnc;
public String sntnc;
public String encrypt(String sntnc)
{
String encrypt1 = sntnc.replace("v", "ag',r");
String encrypt2 = encrypt1.replace("V", "ag',r");
String encrypt3 = encrypt2.replace("m", "ssad");
String encrypt4 = encrypt3.replace("M", "ssad");
String encrypt5 = encrypt4.replace("g", "jeb..w");
String encrypt6 = encrypt5.replace("G", "jeb..w");
String encrypt7 = encrypt6.replace("b", "dug>?/");
encryptdSntnc = encrypt7.replace("B", "dug>?/");
return encryptdSntnc;
}
public String decrypt(String encryptdSntnc)
{
String decrypt1 = encryptdSntnc.replace("dug>?/", "B");
String decrypt2 = decrypt1.replace("dug>?/", "b");
String decrypt3 = decrypt2.replace("jeb..w", "G");
String decrypt4 = decrypt3.replace("jeb..w", "g");
String decrypt5 = decrypt4.replace("ssad", "M");
String decrypt6 = decrypt5.replace("ssad", "m");
String decrypt7 = decrypt6.replace("ag',r", "V");
decryptdSntnc = decrypt7.replace("ag',r", "v");
return decryptdSntnc;
}
Tester Class
Scanner kbReader = new Scanner(System.in);
System.out.print("Enter a sentence that is to be encrypted: ");
String sntnc = kbReader.nextLine();
Crypto myCryptObj = new Crypto();
String encryptdSntnc = myCryptObj.encrypt(sntnc);
System.out.println("Encrypted sentence = " + encryptdSntnc);
String decryptdSntnc = myCryptObj.decrypt(encryptdSntnc);
System.out.println("Decrypted sentence = " + decryptdSntnc);
What I expect is if you type in "Very good", the encrypted sentence would be "ag',rery jeb..wood", and the decrypted sentence would be "Very good".
But instead, I get "ajedug>?/..w',rery jedug>?/..wood" for the encryption, and "ajeB..w',rery jeB..wood" for the decryption, using the same "Very good".
java
java
edited Dec 30 '18 at 23:49
Nathan Kim
asked Dec 30 '18 at 23:18


Nathan KimNathan Kim
112
112
Did you try a loop instead of String.replace?
– SHoko
Dec 30 '18 at 23:33
No, I haven't actually. How would I go about doing that?
– Nathan Kim
Dec 30 '18 at 23:53
add a comment |
Did you try a loop instead of String.replace?
– SHoko
Dec 30 '18 at 23:33
No, I haven't actually. How would I go about doing that?
– Nathan Kim
Dec 30 '18 at 23:53
Did you try a loop instead of String.replace?
– SHoko
Dec 30 '18 at 23:33
Did you try a loop instead of String.replace?
– SHoko
Dec 30 '18 at 23:33
No, I haven't actually. How would I go about doing that?
– Nathan Kim
Dec 30 '18 at 23:53
No, I haven't actually. How would I go about doing that?
– Nathan Kim
Dec 30 '18 at 23:53
add a comment |
1 Answer
1
active
oldest
votes
Your approach to your encryption will not give you what you desire. You want the modifications to affect the original string. However, your step-by-step approach means you get something different. Let's consider just the first two changes:
Very good
becomes
ag',rery good
based on the first pair of rules for replacing v
or V
.
So encrypt2
has this value and then you apply the next pair of rules for replacing g
or G
to this value. This has the effect of replacing the first g
which is not from the original string, but has been added by the first step. Thus encrypt5
has the value:
ajeb..w',rery good
Your encryption will continue in this fashion and you get the undesired result.
You might want to try a loop over your original string instead. Something like:
public String encrypt(String sntnc) {
StringBuilder encryptdSntnc = new StringBuilder();
for (char c : sntnc.toCharArray()) {
c = Character.toLowerCase(c);
switch (c) {
case 'v' :
encryptdSntnc.append("ag',r");
break;
case 'm' :
encryptdSntnc.append("ssad");
break;
case 'g' :
encryptdSntnc.append("jeb..w");
break;
case 'b' :
encryptdSntnc.append("dug>?/");
break;
default :
encryptdSntnc.append(c);
break;
}
}
return encryptdSntnc.toString();
}
Decryption will be more complicated as you need to match strings and replace them with a character. However a similar approach using a loop should be possible. Instead of a switch
statement, you could search for each string that can be replaced by a character.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982222%2fhow-do-i-change-my-encryption-code-so-that-it-will-only-encrypt-the-given-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your approach to your encryption will not give you what you desire. You want the modifications to affect the original string. However, your step-by-step approach means you get something different. Let's consider just the first two changes:
Very good
becomes
ag',rery good
based on the first pair of rules for replacing v
or V
.
So encrypt2
has this value and then you apply the next pair of rules for replacing g
or G
to this value. This has the effect of replacing the first g
which is not from the original string, but has been added by the first step. Thus encrypt5
has the value:
ajeb..w',rery good
Your encryption will continue in this fashion and you get the undesired result.
You might want to try a loop over your original string instead. Something like:
public String encrypt(String sntnc) {
StringBuilder encryptdSntnc = new StringBuilder();
for (char c : sntnc.toCharArray()) {
c = Character.toLowerCase(c);
switch (c) {
case 'v' :
encryptdSntnc.append("ag',r");
break;
case 'm' :
encryptdSntnc.append("ssad");
break;
case 'g' :
encryptdSntnc.append("jeb..w");
break;
case 'b' :
encryptdSntnc.append("dug>?/");
break;
default :
encryptdSntnc.append(c);
break;
}
}
return encryptdSntnc.toString();
}
Decryption will be more complicated as you need to match strings and replace them with a character. However a similar approach using a loop should be possible. Instead of a switch
statement, you could search for each string that can be replaced by a character.
add a comment |
Your approach to your encryption will not give you what you desire. You want the modifications to affect the original string. However, your step-by-step approach means you get something different. Let's consider just the first two changes:
Very good
becomes
ag',rery good
based on the first pair of rules for replacing v
or V
.
So encrypt2
has this value and then you apply the next pair of rules for replacing g
or G
to this value. This has the effect of replacing the first g
which is not from the original string, but has been added by the first step. Thus encrypt5
has the value:
ajeb..w',rery good
Your encryption will continue in this fashion and you get the undesired result.
You might want to try a loop over your original string instead. Something like:
public String encrypt(String sntnc) {
StringBuilder encryptdSntnc = new StringBuilder();
for (char c : sntnc.toCharArray()) {
c = Character.toLowerCase(c);
switch (c) {
case 'v' :
encryptdSntnc.append("ag',r");
break;
case 'm' :
encryptdSntnc.append("ssad");
break;
case 'g' :
encryptdSntnc.append("jeb..w");
break;
case 'b' :
encryptdSntnc.append("dug>?/");
break;
default :
encryptdSntnc.append(c);
break;
}
}
return encryptdSntnc.toString();
}
Decryption will be more complicated as you need to match strings and replace them with a character. However a similar approach using a loop should be possible. Instead of a switch
statement, you could search for each string that can be replaced by a character.
add a comment |
Your approach to your encryption will not give you what you desire. You want the modifications to affect the original string. However, your step-by-step approach means you get something different. Let's consider just the first two changes:
Very good
becomes
ag',rery good
based on the first pair of rules for replacing v
or V
.
So encrypt2
has this value and then you apply the next pair of rules for replacing g
or G
to this value. This has the effect of replacing the first g
which is not from the original string, but has been added by the first step. Thus encrypt5
has the value:
ajeb..w',rery good
Your encryption will continue in this fashion and you get the undesired result.
You might want to try a loop over your original string instead. Something like:
public String encrypt(String sntnc) {
StringBuilder encryptdSntnc = new StringBuilder();
for (char c : sntnc.toCharArray()) {
c = Character.toLowerCase(c);
switch (c) {
case 'v' :
encryptdSntnc.append("ag',r");
break;
case 'm' :
encryptdSntnc.append("ssad");
break;
case 'g' :
encryptdSntnc.append("jeb..w");
break;
case 'b' :
encryptdSntnc.append("dug>?/");
break;
default :
encryptdSntnc.append(c);
break;
}
}
return encryptdSntnc.toString();
}
Decryption will be more complicated as you need to match strings and replace them with a character. However a similar approach using a loop should be possible. Instead of a switch
statement, you could search for each string that can be replaced by a character.
Your approach to your encryption will not give you what you desire. You want the modifications to affect the original string. However, your step-by-step approach means you get something different. Let's consider just the first two changes:
Very good
becomes
ag',rery good
based on the first pair of rules for replacing v
or V
.
So encrypt2
has this value and then you apply the next pair of rules for replacing g
or G
to this value. This has the effect of replacing the first g
which is not from the original string, but has been added by the first step. Thus encrypt5
has the value:
ajeb..w',rery good
Your encryption will continue in this fashion and you get the undesired result.
You might want to try a loop over your original string instead. Something like:
public String encrypt(String sntnc) {
StringBuilder encryptdSntnc = new StringBuilder();
for (char c : sntnc.toCharArray()) {
c = Character.toLowerCase(c);
switch (c) {
case 'v' :
encryptdSntnc.append("ag',r");
break;
case 'm' :
encryptdSntnc.append("ssad");
break;
case 'g' :
encryptdSntnc.append("jeb..w");
break;
case 'b' :
encryptdSntnc.append("dug>?/");
break;
default :
encryptdSntnc.append(c);
break;
}
}
return encryptdSntnc.toString();
}
Decryption will be more complicated as you need to match strings and replace them with a character. However a similar approach using a loop should be possible. Instead of a switch
statement, you could search for each string that can be replaced by a character.
edited Dec 31 '18 at 1:41
answered Dec 31 '18 at 0:28
davedave
8,51552950
8,51552950
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982222%2fhow-do-i-change-my-encryption-code-so-that-it-will-only-encrypt-the-given-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s k6W9A15HC5gkXD2
Did you try a loop instead of String.replace?
– SHoko
Dec 30 '18 at 23:33
No, I haven't actually. How would I go about doing that?
– Nathan Kim
Dec 30 '18 at 23:53