Can't set imageview from Uri
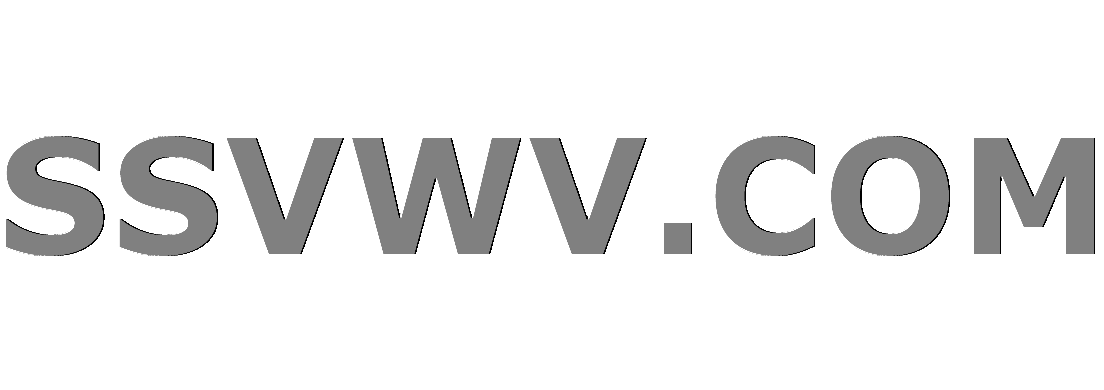
Multi tool use
In the MainActivity I take a picture from camera as the official documentation show:
private void dispatchTakePictureIntent() {
if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(MainActivity.this,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE}, REQUEST_PERMISSION_CAMERA);
} else {
if (Build.VERSION.SDK_INT >= 24) {
try {
Method m = StrictMode.class.getMethod("disableDeathOnFileUriExposure");
m.invoke(null);
} catch (Exception e) {
e.printStackTrace();
}
}
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// Ensure that there's a camera activity to handle the intent
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
// Create the File where the photo should go
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
// Error occurred while creating the File
}
// Continue only if the File was successfully created
if (photoFile != null) {
photoURI = FileProvider.getUriForFile(this,
"com.example.android.fileprovider",
photoFile);
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
startActivityForResult(takePictureIntent, 2);
}
}
}
private File createImageFile() throws IOException {
// Create an image file name
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = "JPEG_" + timeStamp + "_";
File storageDir = getExternalFilesDir(Environment.DIRECTORY_PICTURES);
File image = File.createTempFile(
imageFileName, /* prefix */
".jpg", /* suffix */
storageDir /* directory */
);
// Save a file: path for use with ACTION_VIEW intents
mCurrentPhotoPath = image.getAbsolutePath();
return image;
}
Then I save the photo to the gallery:
private Uri galleryAddPic() {
try{
file = new File(mCurrentPhotoPath);
MediaStore.Images.Media.insertImage(getContentResolver(),
file.getAbsolutePath(), file.getName(), null);
this.sendBroadcast(new Intent(
Intent.ACTION_MEDIA_SCANNER_SCAN_FILE, Uri.fromFile(file)));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
Log.v("UriTaken", Uri.fromFile(file).toString());
return Uri.fromFile(file);
}
Then, with putExtra, I pass to the next activity the Uri of the file.
The uri is this:
file:///storage/emulated/0/Android/data/com.my.name.myappname/files/Pictures/JPEG_20181231_002549_9133887087473873179.jpg
In the new activity, I retrieve the uri, and before I set the imageview with it(if I use image.setImageURI(imageUri); it works), I want to rotate the image to the exact orientation(I don't know why in the image view is always rotated).
I use this code:
if (getIntent().getExtras() != null) {
imageUri = Uri.parse(getIntent().getStringExtra("uri"));
path = getPath(imageUri);
try {
//filePath = getFileName(path);
ExifInterface exifInterface = new ExifInterface(path);
int orientation = exifInterface.getAttributeInt(ExifInterface.TAG_ORIENTATION,
ExifInterface.ORIENTATION_UNDEFINED);
Bitmap bitmap = BitmapFactory.decodeFile(path);
Bitmap rotatedBitmap = null;
switch (orientation) {
case ExifInterface.ORIENTATION_ROTATE_90:
rotatedBitmap = rotateImage(bitmap, 90);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_ROTATE_180:
rotatedBitmap = rotateImage(bitmap, 180);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_ROTATE_270:
rotatedBitmap = rotateImage(bitmap, 270);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_NORMAL:
image.setImageBitmap(bitmap);
default:
rotatedBitmap = bitmap;
image.setImageBitmap(rotatedBitmap);
}
} catch (IOException e) {
Log.w("TAG", "-- Error in setting image");
}
And here the method that cause the crash:
public String getPath(Uri imageUri) {
String wholeId = DocumentsContract.getDocumentId(imageUri);
String id = wholeId.split(":")[1];
String column = {MediaStore.Images.Media.DATA};
// where id is equal to
String sel = MediaStore.Images.Media._ID + "=?";
Cursor cursor = getContentResolver().
query(MediaStore.Images.Media.EXTERNAL_CONTENT_URI,
column, sel, new String{id}, null);
filePath = "";
int columnIndex = cursor.getColumnIndex(column[0]);
if (cursor.moveToFirst()) {
filePath = cursor.getString(columnIndex);
}
cursor.close();
return filePath;
}
On the first line of the method I got the error posted above, invalid uri: file///storage........etc
If I get the picture from gallery it works, because i get this Uri:
content://com.android.providers.media.documents/document/image%3A97897
I don't know if the problem is in the method that saves the image in the MainActivity or is in the getPath() method. What I need to do is to take a picture, save to the gallery and retrieve in the next activity.
java

add a comment |
In the MainActivity I take a picture from camera as the official documentation show:
private void dispatchTakePictureIntent() {
if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(MainActivity.this,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE}, REQUEST_PERMISSION_CAMERA);
} else {
if (Build.VERSION.SDK_INT >= 24) {
try {
Method m = StrictMode.class.getMethod("disableDeathOnFileUriExposure");
m.invoke(null);
} catch (Exception e) {
e.printStackTrace();
}
}
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// Ensure that there's a camera activity to handle the intent
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
// Create the File where the photo should go
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
// Error occurred while creating the File
}
// Continue only if the File was successfully created
if (photoFile != null) {
photoURI = FileProvider.getUriForFile(this,
"com.example.android.fileprovider",
photoFile);
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
startActivityForResult(takePictureIntent, 2);
}
}
}
private File createImageFile() throws IOException {
// Create an image file name
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = "JPEG_" + timeStamp + "_";
File storageDir = getExternalFilesDir(Environment.DIRECTORY_PICTURES);
File image = File.createTempFile(
imageFileName, /* prefix */
".jpg", /* suffix */
storageDir /* directory */
);
// Save a file: path for use with ACTION_VIEW intents
mCurrentPhotoPath = image.getAbsolutePath();
return image;
}
Then I save the photo to the gallery:
private Uri galleryAddPic() {
try{
file = new File(mCurrentPhotoPath);
MediaStore.Images.Media.insertImage(getContentResolver(),
file.getAbsolutePath(), file.getName(), null);
this.sendBroadcast(new Intent(
Intent.ACTION_MEDIA_SCANNER_SCAN_FILE, Uri.fromFile(file)));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
Log.v("UriTaken", Uri.fromFile(file).toString());
return Uri.fromFile(file);
}
Then, with putExtra, I pass to the next activity the Uri of the file.
The uri is this:
file:///storage/emulated/0/Android/data/com.my.name.myappname/files/Pictures/JPEG_20181231_002549_9133887087473873179.jpg
In the new activity, I retrieve the uri, and before I set the imageview with it(if I use image.setImageURI(imageUri); it works), I want to rotate the image to the exact orientation(I don't know why in the image view is always rotated).
I use this code:
if (getIntent().getExtras() != null) {
imageUri = Uri.parse(getIntent().getStringExtra("uri"));
path = getPath(imageUri);
try {
//filePath = getFileName(path);
ExifInterface exifInterface = new ExifInterface(path);
int orientation = exifInterface.getAttributeInt(ExifInterface.TAG_ORIENTATION,
ExifInterface.ORIENTATION_UNDEFINED);
Bitmap bitmap = BitmapFactory.decodeFile(path);
Bitmap rotatedBitmap = null;
switch (orientation) {
case ExifInterface.ORIENTATION_ROTATE_90:
rotatedBitmap = rotateImage(bitmap, 90);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_ROTATE_180:
rotatedBitmap = rotateImage(bitmap, 180);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_ROTATE_270:
rotatedBitmap = rotateImage(bitmap, 270);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_NORMAL:
image.setImageBitmap(bitmap);
default:
rotatedBitmap = bitmap;
image.setImageBitmap(rotatedBitmap);
}
} catch (IOException e) {
Log.w("TAG", "-- Error in setting image");
}
And here the method that cause the crash:
public String getPath(Uri imageUri) {
String wholeId = DocumentsContract.getDocumentId(imageUri);
String id = wholeId.split(":")[1];
String column = {MediaStore.Images.Media.DATA};
// where id is equal to
String sel = MediaStore.Images.Media._ID + "=?";
Cursor cursor = getContentResolver().
query(MediaStore.Images.Media.EXTERNAL_CONTENT_URI,
column, sel, new String{id}, null);
filePath = "";
int columnIndex = cursor.getColumnIndex(column[0]);
if (cursor.moveToFirst()) {
filePath = cursor.getString(columnIndex);
}
cursor.close();
return filePath;
}
On the first line of the method I got the error posted above, invalid uri: file///storage........etc
If I get the picture from gallery it works, because i get this Uri:
content://com.android.providers.media.documents/document/image%3A97897
I don't know if the problem is in the method that saves the image in the MainActivity or is in the getPath() method. What I need to do is to take a picture, save to the gallery and retrieve in the next activity.
java

add a comment |
In the MainActivity I take a picture from camera as the official documentation show:
private void dispatchTakePictureIntent() {
if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(MainActivity.this,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE}, REQUEST_PERMISSION_CAMERA);
} else {
if (Build.VERSION.SDK_INT >= 24) {
try {
Method m = StrictMode.class.getMethod("disableDeathOnFileUriExposure");
m.invoke(null);
} catch (Exception e) {
e.printStackTrace();
}
}
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// Ensure that there's a camera activity to handle the intent
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
// Create the File where the photo should go
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
// Error occurred while creating the File
}
// Continue only if the File was successfully created
if (photoFile != null) {
photoURI = FileProvider.getUriForFile(this,
"com.example.android.fileprovider",
photoFile);
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
startActivityForResult(takePictureIntent, 2);
}
}
}
private File createImageFile() throws IOException {
// Create an image file name
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = "JPEG_" + timeStamp + "_";
File storageDir = getExternalFilesDir(Environment.DIRECTORY_PICTURES);
File image = File.createTempFile(
imageFileName, /* prefix */
".jpg", /* suffix */
storageDir /* directory */
);
// Save a file: path for use with ACTION_VIEW intents
mCurrentPhotoPath = image.getAbsolutePath();
return image;
}
Then I save the photo to the gallery:
private Uri galleryAddPic() {
try{
file = new File(mCurrentPhotoPath);
MediaStore.Images.Media.insertImage(getContentResolver(),
file.getAbsolutePath(), file.getName(), null);
this.sendBroadcast(new Intent(
Intent.ACTION_MEDIA_SCANNER_SCAN_FILE, Uri.fromFile(file)));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
Log.v("UriTaken", Uri.fromFile(file).toString());
return Uri.fromFile(file);
}
Then, with putExtra, I pass to the next activity the Uri of the file.
The uri is this:
file:///storage/emulated/0/Android/data/com.my.name.myappname/files/Pictures/JPEG_20181231_002549_9133887087473873179.jpg
In the new activity, I retrieve the uri, and before I set the imageview with it(if I use image.setImageURI(imageUri); it works), I want to rotate the image to the exact orientation(I don't know why in the image view is always rotated).
I use this code:
if (getIntent().getExtras() != null) {
imageUri = Uri.parse(getIntent().getStringExtra("uri"));
path = getPath(imageUri);
try {
//filePath = getFileName(path);
ExifInterface exifInterface = new ExifInterface(path);
int orientation = exifInterface.getAttributeInt(ExifInterface.TAG_ORIENTATION,
ExifInterface.ORIENTATION_UNDEFINED);
Bitmap bitmap = BitmapFactory.decodeFile(path);
Bitmap rotatedBitmap = null;
switch (orientation) {
case ExifInterface.ORIENTATION_ROTATE_90:
rotatedBitmap = rotateImage(bitmap, 90);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_ROTATE_180:
rotatedBitmap = rotateImage(bitmap, 180);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_ROTATE_270:
rotatedBitmap = rotateImage(bitmap, 270);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_NORMAL:
image.setImageBitmap(bitmap);
default:
rotatedBitmap = bitmap;
image.setImageBitmap(rotatedBitmap);
}
} catch (IOException e) {
Log.w("TAG", "-- Error in setting image");
}
And here the method that cause the crash:
public String getPath(Uri imageUri) {
String wholeId = DocumentsContract.getDocumentId(imageUri);
String id = wholeId.split(":")[1];
String column = {MediaStore.Images.Media.DATA};
// where id is equal to
String sel = MediaStore.Images.Media._ID + "=?";
Cursor cursor = getContentResolver().
query(MediaStore.Images.Media.EXTERNAL_CONTENT_URI,
column, sel, new String{id}, null);
filePath = "";
int columnIndex = cursor.getColumnIndex(column[0]);
if (cursor.moveToFirst()) {
filePath = cursor.getString(columnIndex);
}
cursor.close();
return filePath;
}
On the first line of the method I got the error posted above, invalid uri: file///storage........etc
If I get the picture from gallery it works, because i get this Uri:
content://com.android.providers.media.documents/document/image%3A97897
I don't know if the problem is in the method that saves the image in the MainActivity or is in the getPath() method. What I need to do is to take a picture, save to the gallery and retrieve in the next activity.
java

In the MainActivity I take a picture from camera as the official documentation show:
private void dispatchTakePictureIntent() {
if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(MainActivity.this,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE}, REQUEST_PERMISSION_CAMERA);
} else {
if (Build.VERSION.SDK_INT >= 24) {
try {
Method m = StrictMode.class.getMethod("disableDeathOnFileUriExposure");
m.invoke(null);
} catch (Exception e) {
e.printStackTrace();
}
}
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// Ensure that there's a camera activity to handle the intent
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
// Create the File where the photo should go
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
// Error occurred while creating the File
}
// Continue only if the File was successfully created
if (photoFile != null) {
photoURI = FileProvider.getUriForFile(this,
"com.example.android.fileprovider",
photoFile);
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
startActivityForResult(takePictureIntent, 2);
}
}
}
private File createImageFile() throws IOException {
// Create an image file name
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = "JPEG_" + timeStamp + "_";
File storageDir = getExternalFilesDir(Environment.DIRECTORY_PICTURES);
File image = File.createTempFile(
imageFileName, /* prefix */
".jpg", /* suffix */
storageDir /* directory */
);
// Save a file: path for use with ACTION_VIEW intents
mCurrentPhotoPath = image.getAbsolutePath();
return image;
}
Then I save the photo to the gallery:
private Uri galleryAddPic() {
try{
file = new File(mCurrentPhotoPath);
MediaStore.Images.Media.insertImage(getContentResolver(),
file.getAbsolutePath(), file.getName(), null);
this.sendBroadcast(new Intent(
Intent.ACTION_MEDIA_SCANNER_SCAN_FILE, Uri.fromFile(file)));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
Log.v("UriTaken", Uri.fromFile(file).toString());
return Uri.fromFile(file);
}
Then, with putExtra, I pass to the next activity the Uri of the file.
The uri is this:
file:///storage/emulated/0/Android/data/com.my.name.myappname/files/Pictures/JPEG_20181231_002549_9133887087473873179.jpg
In the new activity, I retrieve the uri, and before I set the imageview with it(if I use image.setImageURI(imageUri); it works), I want to rotate the image to the exact orientation(I don't know why in the image view is always rotated).
I use this code:
if (getIntent().getExtras() != null) {
imageUri = Uri.parse(getIntent().getStringExtra("uri"));
path = getPath(imageUri);
try {
//filePath = getFileName(path);
ExifInterface exifInterface = new ExifInterface(path);
int orientation = exifInterface.getAttributeInt(ExifInterface.TAG_ORIENTATION,
ExifInterface.ORIENTATION_UNDEFINED);
Bitmap bitmap = BitmapFactory.decodeFile(path);
Bitmap rotatedBitmap = null;
switch (orientation) {
case ExifInterface.ORIENTATION_ROTATE_90:
rotatedBitmap = rotateImage(bitmap, 90);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_ROTATE_180:
rotatedBitmap = rotateImage(bitmap, 180);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_ROTATE_270:
rotatedBitmap = rotateImage(bitmap, 270);
image.setImageBitmap(rotatedBitmap);
break;
case ExifInterface.ORIENTATION_NORMAL:
image.setImageBitmap(bitmap);
default:
rotatedBitmap = bitmap;
image.setImageBitmap(rotatedBitmap);
}
} catch (IOException e) {
Log.w("TAG", "-- Error in setting image");
}
And here the method that cause the crash:
public String getPath(Uri imageUri) {
String wholeId = DocumentsContract.getDocumentId(imageUri);
String id = wholeId.split(":")[1];
String column = {MediaStore.Images.Media.DATA};
// where id is equal to
String sel = MediaStore.Images.Media._ID + "=?";
Cursor cursor = getContentResolver().
query(MediaStore.Images.Media.EXTERNAL_CONTENT_URI,
column, sel, new String{id}, null);
filePath = "";
int columnIndex = cursor.getColumnIndex(column[0]);
if (cursor.moveToFirst()) {
filePath = cursor.getString(columnIndex);
}
cursor.close();
return filePath;
}
On the first line of the method I got the error posted above, invalid uri: file///storage........etc
If I get the picture from gallery it works, because i get this Uri:
content://com.android.providers.media.documents/document/image%3A97897
I don't know if the problem is in the method that saves the image in the MainActivity or is in the getPath() method. What I need to do is to take a picture, save to the gallery and retrieve in the next activity.
java

java

asked Dec 30 '18 at 23:53


NicolaNicola
828
828
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982401%2fcant-set-imageview-from-uri%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982401%2fcant-set-imageview-from-uri%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
253EQ0s,UuJ,15fxjEoV