Alert if radios unchecked on form submit
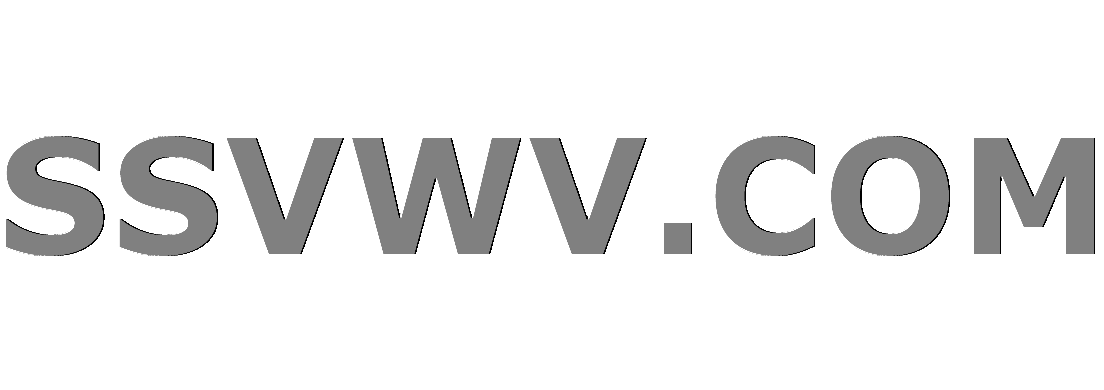
Multi tool use
I am attempting to check if a one of two radio buttons have been checked when submitting a form to validate before submitting.
<body onload="init();">
<form name="myForm" onsubmit="return validateForm();">
<label for="cName">Client Name*</label><br>
<div style="width:74.8%; display:inline-block;">
<input type="text" id="cName" name="cName" placeholder="Client Name..." required>
</div><div style="width:25.2%; display:inline-block;">
<select class="select1" id="cRecord" name="cRecord" required>
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
</div>
Yes <input required type="radio" onclick="javascript:smeCheck();" value="Yes" name="TL/SME" id="yesCheck">
No <input type="radio" onclick="javascript:smeCheck();" value="No" name="TL/SME" id="noCheck"><br>
<div id="ifyes" style="display:none">
<div class="buttons">
<input type="button" id="formOut" onclick="this.form.submit();" value="Submit">
</div>
<script>
$('#formOut').click(function validateForm() {
var Name = document.forms["myForm"]["cName"].value;
var Record = document.forms["myForm"]["cRecord"].value;
var yesSME = $("#yesCheck").prop("checked");
var noSME = $("#noCheck").prop("checked");
if (Name == "") {
alert("//Alert Here");
return false;
}
if (Record == "") {
alert("//Alert Here");
return false;
}
if (yesSME == false || noSME == false) {
alert("//Alert Here");
return false;
} else if (cName != "" && cRecord != "" && ((yesSME == true) || (noSME == true))) {
// do things here
}
});
<script>
</form>
Currently I find with what I have above the user receives an alert upon form submission even when a radio option is selected.
Edit:
Desired behaviour - Upon the user clicking the "Submit" button if the fields cName
, cRecord
and one of the radios has been selected a Modal will open.
Current Behaviour - When the user clicks the "Submit" Button if the fields cName
, cRecord
and one of the radios have been selected an alert is given for the radio buttons.
If one or both of the fields cName
or cRecord
have no user input when clicking the "Submit" button an alert is given for the cName or cRecord field.
The function (opening the modal) works without any issues if I remove the check for the radio button being checked,
The only issue seems to be with the check for if a radio button is selected/unselected
Any assistance would be appreciated.
javascript jquery
add a comment |
I am attempting to check if a one of two radio buttons have been checked when submitting a form to validate before submitting.
<body onload="init();">
<form name="myForm" onsubmit="return validateForm();">
<label for="cName">Client Name*</label><br>
<div style="width:74.8%; display:inline-block;">
<input type="text" id="cName" name="cName" placeholder="Client Name..." required>
</div><div style="width:25.2%; display:inline-block;">
<select class="select1" id="cRecord" name="cRecord" required>
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
</div>
Yes <input required type="radio" onclick="javascript:smeCheck();" value="Yes" name="TL/SME" id="yesCheck">
No <input type="radio" onclick="javascript:smeCheck();" value="No" name="TL/SME" id="noCheck"><br>
<div id="ifyes" style="display:none">
<div class="buttons">
<input type="button" id="formOut" onclick="this.form.submit();" value="Submit">
</div>
<script>
$('#formOut').click(function validateForm() {
var Name = document.forms["myForm"]["cName"].value;
var Record = document.forms["myForm"]["cRecord"].value;
var yesSME = $("#yesCheck").prop("checked");
var noSME = $("#noCheck").prop("checked");
if (Name == "") {
alert("//Alert Here");
return false;
}
if (Record == "") {
alert("//Alert Here");
return false;
}
if (yesSME == false || noSME == false) {
alert("//Alert Here");
return false;
} else if (cName != "" && cRecord != "" && ((yesSME == true) || (noSME == true))) {
// do things here
}
});
<script>
</form>
Currently I find with what I have above the user receives an alert upon form submission even when a radio option is selected.
Edit:
Desired behaviour - Upon the user clicking the "Submit" button if the fields cName
, cRecord
and one of the radios has been selected a Modal will open.
Current Behaviour - When the user clicks the "Submit" Button if the fields cName
, cRecord
and one of the radios have been selected an alert is given for the radio buttons.
If one or both of the fields cName
or cRecord
have no user input when clicking the "Submit" button an alert is given for the cName or cRecord field.
The function (opening the modal) works without any issues if I remove the check for the radio button being checked,
The only issue seems to be with the check for if a radio button is selected/unselected
Any assistance would be appreciated.
javascript jquery
Possible duplicate of How can I check whether a radio button is selected with JavaScript?
– chbchb55
Dec 30 '18 at 23:37
@chbchb55 I have made some edits to give some further context, from what I can see there isn't an example I can apply/get working from the solution linked.
– user10073459
Dec 31 '18 at 0:23
add a comment |
I am attempting to check if a one of two radio buttons have been checked when submitting a form to validate before submitting.
<body onload="init();">
<form name="myForm" onsubmit="return validateForm();">
<label for="cName">Client Name*</label><br>
<div style="width:74.8%; display:inline-block;">
<input type="text" id="cName" name="cName" placeholder="Client Name..." required>
</div><div style="width:25.2%; display:inline-block;">
<select class="select1" id="cRecord" name="cRecord" required>
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
</div>
Yes <input required type="radio" onclick="javascript:smeCheck();" value="Yes" name="TL/SME" id="yesCheck">
No <input type="radio" onclick="javascript:smeCheck();" value="No" name="TL/SME" id="noCheck"><br>
<div id="ifyes" style="display:none">
<div class="buttons">
<input type="button" id="formOut" onclick="this.form.submit();" value="Submit">
</div>
<script>
$('#formOut').click(function validateForm() {
var Name = document.forms["myForm"]["cName"].value;
var Record = document.forms["myForm"]["cRecord"].value;
var yesSME = $("#yesCheck").prop("checked");
var noSME = $("#noCheck").prop("checked");
if (Name == "") {
alert("//Alert Here");
return false;
}
if (Record == "") {
alert("//Alert Here");
return false;
}
if (yesSME == false || noSME == false) {
alert("//Alert Here");
return false;
} else if (cName != "" && cRecord != "" && ((yesSME == true) || (noSME == true))) {
// do things here
}
});
<script>
</form>
Currently I find with what I have above the user receives an alert upon form submission even when a radio option is selected.
Edit:
Desired behaviour - Upon the user clicking the "Submit" button if the fields cName
, cRecord
and one of the radios has been selected a Modal will open.
Current Behaviour - When the user clicks the "Submit" Button if the fields cName
, cRecord
and one of the radios have been selected an alert is given for the radio buttons.
If one or both of the fields cName
or cRecord
have no user input when clicking the "Submit" button an alert is given for the cName or cRecord field.
The function (opening the modal) works without any issues if I remove the check for the radio button being checked,
The only issue seems to be with the check for if a radio button is selected/unselected
Any assistance would be appreciated.
javascript jquery
I am attempting to check if a one of two radio buttons have been checked when submitting a form to validate before submitting.
<body onload="init();">
<form name="myForm" onsubmit="return validateForm();">
<label for="cName">Client Name*</label><br>
<div style="width:74.8%; display:inline-block;">
<input type="text" id="cName" name="cName" placeholder="Client Name..." required>
</div><div style="width:25.2%; display:inline-block;">
<select class="select1" id="cRecord" name="cRecord" required>
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
</div>
Yes <input required type="radio" onclick="javascript:smeCheck();" value="Yes" name="TL/SME" id="yesCheck">
No <input type="radio" onclick="javascript:smeCheck();" value="No" name="TL/SME" id="noCheck"><br>
<div id="ifyes" style="display:none">
<div class="buttons">
<input type="button" id="formOut" onclick="this.form.submit();" value="Submit">
</div>
<script>
$('#formOut').click(function validateForm() {
var Name = document.forms["myForm"]["cName"].value;
var Record = document.forms["myForm"]["cRecord"].value;
var yesSME = $("#yesCheck").prop("checked");
var noSME = $("#noCheck").prop("checked");
if (Name == "") {
alert("//Alert Here");
return false;
}
if (Record == "") {
alert("//Alert Here");
return false;
}
if (yesSME == false || noSME == false) {
alert("//Alert Here");
return false;
} else if (cName != "" && cRecord != "" && ((yesSME == true) || (noSME == true))) {
// do things here
}
});
<script>
</form>
Currently I find with what I have above the user receives an alert upon form submission even when a radio option is selected.
Edit:
Desired behaviour - Upon the user clicking the "Submit" button if the fields cName
, cRecord
and one of the radios has been selected a Modal will open.
Current Behaviour - When the user clicks the "Submit" Button if the fields cName
, cRecord
and one of the radios have been selected an alert is given for the radio buttons.
If one or both of the fields cName
or cRecord
have no user input when clicking the "Submit" button an alert is given for the cName or cRecord field.
The function (opening the modal) works without any issues if I remove the check for the radio button being checked,
The only issue seems to be with the check for if a radio button is selected/unselected
Any assistance would be appreciated.
javascript jquery
javascript jquery
edited Dec 31 '18 at 1:02
user10073459
asked Dec 30 '18 at 23:35
user10073459user10073459
609
609
Possible duplicate of How can I check whether a radio button is selected with JavaScript?
– chbchb55
Dec 30 '18 at 23:37
@chbchb55 I have made some edits to give some further context, from what I can see there isn't an example I can apply/get working from the solution linked.
– user10073459
Dec 31 '18 at 0:23
add a comment |
Possible duplicate of How can I check whether a radio button is selected with JavaScript?
– chbchb55
Dec 30 '18 at 23:37
@chbchb55 I have made some edits to give some further context, from what I can see there isn't an example I can apply/get working from the solution linked.
– user10073459
Dec 31 '18 at 0:23
Possible duplicate of How can I check whether a radio button is selected with JavaScript?
– chbchb55
Dec 30 '18 at 23:37
Possible duplicate of How can I check whether a radio button is selected with JavaScript?
– chbchb55
Dec 30 '18 at 23:37
@chbchb55 I have made some edits to give some further context, from what I can see there isn't an example I can apply/get working from the solution linked.
– user10073459
Dec 31 '18 at 0:23
@chbchb55 I have made some edits to give some further context, from what I can see there isn't an example I can apply/get working from the solution linked.
– user10073459
Dec 31 '18 at 0:23
add a comment |
4 Answers
4
active
oldest
votes
** Another answer (I'd like to keep first one for reference)
you can achieve what you want like :
HTML:
<!DOCTYPE html>
<html>
<body>
<form id="form1" action="javascript:void(0);">
<label for="cName">Client Name*</label><br>
<input type="text" id="cName" name="cName" placeholder="Client Name..."> <br><br>
<select class="select1" id="cRecord" name="cRecord">
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
<br>
Yes <input type="radio" value="Yes" name="TLSME" id="yesCheck">
No <input type="radio" value="No" name="TLSME" id="noCheck"><br>
<input type="submit" id="formSubmit" value="Submit">
</form>
</body>
</html>
JS (JQuery):
$("#form1").submit(function(){
if (!$("input[name='TLSME']").is(':checked') || $("#cName").val() === '' || $("#cRecord").val() === '') {
alert('Please complete required fields!');
} else {
// your code here
}
});
Thank you, I was able to get this to work as I intended with your assistance.
– user10073459
Dec 31 '18 at 1:55
add a comment |
if(!document.getElementById('yesCheck').checked || !document.getElementById('noCheck').checked) {
alert('Not checked');
}
Should do the trick
This gives the alert when unchecked but still gives an alert when an option has been checked when I useelse if((document.getElementById('yesCheck').checked) || (document.getElementById('noCheck').checked))
– user10073459
Dec 30 '18 at 23:56
You don't need to do else if. Just use } else { and process the form in the else statement.
– James Grimshaw
Dec 31 '18 at 0:26
add a comment |
You can do that easily using JQuery:
HTML:
<html>
<body>
<form id="form1" action="javascript:void(0);">
<input type="radio" name="gender" value="male"> Male<br>
<input type="radio" name="gender" value="female"> Female<br>
<input type="radio" name="gender" value="other"> Other <br>
<input type="submit">
</form>
</body>
</html>
Javascript:
$("#form1").on("submit", function(){
//Code: Action (like ajax...)
if ($('input[name=gender]:checked').length <= 0) {
alert("No radio checked")
}
})
** Note:
action="javascript:void(0);"
was added to prevent form submission (do nothing), you can remove it when you have action to do.
Thank you for your assistance, You solution while working with the two radios I can't seem to get working with the other variables I have, would you be able to advise how I could implement the solution to work with my edits above? Thank you
– user10073459
Dec 31 '18 at 0:27
Please post your HTML and JS, so i can investigate
– Tarreq
Dec 31 '18 at 0:29
I have updated my post with a cutdown copy of the HTML to where should be relevant, Thank you
– user10073459
Dec 31 '18 at 0:41
ok, your edit didn't say what you want your code to do, please explain in detail
– Tarreq
Dec 31 '18 at 0:51
My apologies, I have added the current and expected behaviour to my edit, The only issue I am having with the code is getting an alert if no radio is checked (and all fields have user input) and opening the modal if all fields have user input and a radio option is selected.
– user10073459
Dec 31 '18 at 1:06
|
show 1 more comment
Why not make the radio buttons required
It would seem that you're trying to do the job of the browser. You don't need to display an alert, which are generally bad UX, because you can use built-in form validation via the required
attribute like so:
<form onsubmit="...">
<input type="radio" name="group" value="1" required />
<input type="radio" name="group" value="2" />
...
<button type="submit">Submit</button>
<form />
NOTE: you only need one required
attribute and it will apply to all inputs in said group.
This is something I have already done but the form submit is calling a function to open a Modal on the same page. using a<submit>
just opens the Modal over the top of the page even when theRequired
fields are empty, I apologise I forgot to add this too my original question.
– user10073459
Dec 31 '18 at 0:44
Theonsubmit
event should never even fire if you've set it up correctly @user10073459
– chbchb55
Dec 31 '18 at 1:09
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982298%2falert-if-radios-unchecked-on-form-submit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
** Another answer (I'd like to keep first one for reference)
you can achieve what you want like :
HTML:
<!DOCTYPE html>
<html>
<body>
<form id="form1" action="javascript:void(0);">
<label for="cName">Client Name*</label><br>
<input type="text" id="cName" name="cName" placeholder="Client Name..."> <br><br>
<select class="select1" id="cRecord" name="cRecord">
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
<br>
Yes <input type="radio" value="Yes" name="TLSME" id="yesCheck">
No <input type="radio" value="No" name="TLSME" id="noCheck"><br>
<input type="submit" id="formSubmit" value="Submit">
</form>
</body>
</html>
JS (JQuery):
$("#form1").submit(function(){
if (!$("input[name='TLSME']").is(':checked') || $("#cName").val() === '' || $("#cRecord").val() === '') {
alert('Please complete required fields!');
} else {
// your code here
}
});
Thank you, I was able to get this to work as I intended with your assistance.
– user10073459
Dec 31 '18 at 1:55
add a comment |
** Another answer (I'd like to keep first one for reference)
you can achieve what you want like :
HTML:
<!DOCTYPE html>
<html>
<body>
<form id="form1" action="javascript:void(0);">
<label for="cName">Client Name*</label><br>
<input type="text" id="cName" name="cName" placeholder="Client Name..."> <br><br>
<select class="select1" id="cRecord" name="cRecord">
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
<br>
Yes <input type="radio" value="Yes" name="TLSME" id="yesCheck">
No <input type="radio" value="No" name="TLSME" id="noCheck"><br>
<input type="submit" id="formSubmit" value="Submit">
</form>
</body>
</html>
JS (JQuery):
$("#form1").submit(function(){
if (!$("input[name='TLSME']").is(':checked') || $("#cName").val() === '' || $("#cRecord").val() === '') {
alert('Please complete required fields!');
} else {
// your code here
}
});
Thank you, I was able to get this to work as I intended with your assistance.
– user10073459
Dec 31 '18 at 1:55
add a comment |
** Another answer (I'd like to keep first one for reference)
you can achieve what you want like :
HTML:
<!DOCTYPE html>
<html>
<body>
<form id="form1" action="javascript:void(0);">
<label for="cName">Client Name*</label><br>
<input type="text" id="cName" name="cName" placeholder="Client Name..."> <br><br>
<select class="select1" id="cRecord" name="cRecord">
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
<br>
Yes <input type="radio" value="Yes" name="TLSME" id="yesCheck">
No <input type="radio" value="No" name="TLSME" id="noCheck"><br>
<input type="submit" id="formSubmit" value="Submit">
</form>
</body>
</html>
JS (JQuery):
$("#form1").submit(function(){
if (!$("input[name='TLSME']").is(':checked') || $("#cName").val() === '' || $("#cRecord").val() === '') {
alert('Please complete required fields!');
} else {
// your code here
}
});
** Another answer (I'd like to keep first one for reference)
you can achieve what you want like :
HTML:
<!DOCTYPE html>
<html>
<body>
<form id="form1" action="javascript:void(0);">
<label for="cName">Client Name*</label><br>
<input type="text" id="cName" name="cName" placeholder="Client Name..."> <br><br>
<select class="select1" id="cRecord" name="cRecord">
<option value="" disabled selected>Advised Recording...
</option>
<option value="Yes">Yes</option>
<option value="No">No</option>
</select>
<br>
Yes <input type="radio" value="Yes" name="TLSME" id="yesCheck">
No <input type="radio" value="No" name="TLSME" id="noCheck"><br>
<input type="submit" id="formSubmit" value="Submit">
</form>
</body>
</html>
JS (JQuery):
$("#form1").submit(function(){
if (!$("input[name='TLSME']").is(':checked') || $("#cName").val() === '' || $("#cRecord").val() === '') {
alert('Please complete required fields!');
} else {
// your code here
}
});
answered Dec 31 '18 at 1:44


TarreqTarreq
553212
553212
Thank you, I was able to get this to work as I intended with your assistance.
– user10073459
Dec 31 '18 at 1:55
add a comment |
Thank you, I was able to get this to work as I intended with your assistance.
– user10073459
Dec 31 '18 at 1:55
Thank you, I was able to get this to work as I intended with your assistance.
– user10073459
Dec 31 '18 at 1:55
Thank you, I was able to get this to work as I intended with your assistance.
– user10073459
Dec 31 '18 at 1:55
add a comment |
if(!document.getElementById('yesCheck').checked || !document.getElementById('noCheck').checked) {
alert('Not checked');
}
Should do the trick
This gives the alert when unchecked but still gives an alert when an option has been checked when I useelse if((document.getElementById('yesCheck').checked) || (document.getElementById('noCheck').checked))
– user10073459
Dec 30 '18 at 23:56
You don't need to do else if. Just use } else { and process the form in the else statement.
– James Grimshaw
Dec 31 '18 at 0:26
add a comment |
if(!document.getElementById('yesCheck').checked || !document.getElementById('noCheck').checked) {
alert('Not checked');
}
Should do the trick
This gives the alert when unchecked but still gives an alert when an option has been checked when I useelse if((document.getElementById('yesCheck').checked) || (document.getElementById('noCheck').checked))
– user10073459
Dec 30 '18 at 23:56
You don't need to do else if. Just use } else { and process the form in the else statement.
– James Grimshaw
Dec 31 '18 at 0:26
add a comment |
if(!document.getElementById('yesCheck').checked || !document.getElementById('noCheck').checked) {
alert('Not checked');
}
Should do the trick
if(!document.getElementById('yesCheck').checked || !document.getElementById('noCheck').checked) {
alert('Not checked');
}
Should do the trick
answered Dec 30 '18 at 23:41


James GrimshawJames Grimshaw
10618
10618
This gives the alert when unchecked but still gives an alert when an option has been checked when I useelse if((document.getElementById('yesCheck').checked) || (document.getElementById('noCheck').checked))
– user10073459
Dec 30 '18 at 23:56
You don't need to do else if. Just use } else { and process the form in the else statement.
– James Grimshaw
Dec 31 '18 at 0:26
add a comment |
This gives the alert when unchecked but still gives an alert when an option has been checked when I useelse if((document.getElementById('yesCheck').checked) || (document.getElementById('noCheck').checked))
– user10073459
Dec 30 '18 at 23:56
You don't need to do else if. Just use } else { and process the form in the else statement.
– James Grimshaw
Dec 31 '18 at 0:26
This gives the alert when unchecked but still gives an alert when an option has been checked when I use
else if((document.getElementById('yesCheck').checked) || (document.getElementById('noCheck').checked))
– user10073459
Dec 30 '18 at 23:56
This gives the alert when unchecked but still gives an alert when an option has been checked when I use
else if((document.getElementById('yesCheck').checked) || (document.getElementById('noCheck').checked))
– user10073459
Dec 30 '18 at 23:56
You don't need to do else if. Just use } else { and process the form in the else statement.
– James Grimshaw
Dec 31 '18 at 0:26
You don't need to do else if. Just use } else { and process the form in the else statement.
– James Grimshaw
Dec 31 '18 at 0:26
add a comment |
You can do that easily using JQuery:
HTML:
<html>
<body>
<form id="form1" action="javascript:void(0);">
<input type="radio" name="gender" value="male"> Male<br>
<input type="radio" name="gender" value="female"> Female<br>
<input type="radio" name="gender" value="other"> Other <br>
<input type="submit">
</form>
</body>
</html>
Javascript:
$("#form1").on("submit", function(){
//Code: Action (like ajax...)
if ($('input[name=gender]:checked').length <= 0) {
alert("No radio checked")
}
})
** Note:
action="javascript:void(0);"
was added to prevent form submission (do nothing), you can remove it when you have action to do.
Thank you for your assistance, You solution while working with the two radios I can't seem to get working with the other variables I have, would you be able to advise how I could implement the solution to work with my edits above? Thank you
– user10073459
Dec 31 '18 at 0:27
Please post your HTML and JS, so i can investigate
– Tarreq
Dec 31 '18 at 0:29
I have updated my post with a cutdown copy of the HTML to where should be relevant, Thank you
– user10073459
Dec 31 '18 at 0:41
ok, your edit didn't say what you want your code to do, please explain in detail
– Tarreq
Dec 31 '18 at 0:51
My apologies, I have added the current and expected behaviour to my edit, The only issue I am having with the code is getting an alert if no radio is checked (and all fields have user input) and opening the modal if all fields have user input and a radio option is selected.
– user10073459
Dec 31 '18 at 1:06
|
show 1 more comment
You can do that easily using JQuery:
HTML:
<html>
<body>
<form id="form1" action="javascript:void(0);">
<input type="radio" name="gender" value="male"> Male<br>
<input type="radio" name="gender" value="female"> Female<br>
<input type="radio" name="gender" value="other"> Other <br>
<input type="submit">
</form>
</body>
</html>
Javascript:
$("#form1").on("submit", function(){
//Code: Action (like ajax...)
if ($('input[name=gender]:checked').length <= 0) {
alert("No radio checked")
}
})
** Note:
action="javascript:void(0);"
was added to prevent form submission (do nothing), you can remove it when you have action to do.
Thank you for your assistance, You solution while working with the two radios I can't seem to get working with the other variables I have, would you be able to advise how I could implement the solution to work with my edits above? Thank you
– user10073459
Dec 31 '18 at 0:27
Please post your HTML and JS, so i can investigate
– Tarreq
Dec 31 '18 at 0:29
I have updated my post with a cutdown copy of the HTML to where should be relevant, Thank you
– user10073459
Dec 31 '18 at 0:41
ok, your edit didn't say what you want your code to do, please explain in detail
– Tarreq
Dec 31 '18 at 0:51
My apologies, I have added the current and expected behaviour to my edit, The only issue I am having with the code is getting an alert if no radio is checked (and all fields have user input) and opening the modal if all fields have user input and a radio option is selected.
– user10073459
Dec 31 '18 at 1:06
|
show 1 more comment
You can do that easily using JQuery:
HTML:
<html>
<body>
<form id="form1" action="javascript:void(0);">
<input type="radio" name="gender" value="male"> Male<br>
<input type="radio" name="gender" value="female"> Female<br>
<input type="radio" name="gender" value="other"> Other <br>
<input type="submit">
</form>
</body>
</html>
Javascript:
$("#form1").on("submit", function(){
//Code: Action (like ajax...)
if ($('input[name=gender]:checked').length <= 0) {
alert("No radio checked")
}
})
** Note:
action="javascript:void(0);"
was added to prevent form submission (do nothing), you can remove it when you have action to do.
You can do that easily using JQuery:
HTML:
<html>
<body>
<form id="form1" action="javascript:void(0);">
<input type="radio" name="gender" value="male"> Male<br>
<input type="radio" name="gender" value="female"> Female<br>
<input type="radio" name="gender" value="other"> Other <br>
<input type="submit">
</form>
</body>
</html>
Javascript:
$("#form1").on("submit", function(){
//Code: Action (like ajax...)
if ($('input[name=gender]:checked').length <= 0) {
alert("No radio checked")
}
})
** Note:
action="javascript:void(0);"
was added to prevent form submission (do nothing), you can remove it when you have action to do.
answered Dec 30 '18 at 23:59


TarreqTarreq
553212
553212
Thank you for your assistance, You solution while working with the two radios I can't seem to get working with the other variables I have, would you be able to advise how I could implement the solution to work with my edits above? Thank you
– user10073459
Dec 31 '18 at 0:27
Please post your HTML and JS, so i can investigate
– Tarreq
Dec 31 '18 at 0:29
I have updated my post with a cutdown copy of the HTML to where should be relevant, Thank you
– user10073459
Dec 31 '18 at 0:41
ok, your edit didn't say what you want your code to do, please explain in detail
– Tarreq
Dec 31 '18 at 0:51
My apologies, I have added the current and expected behaviour to my edit, The only issue I am having with the code is getting an alert if no radio is checked (and all fields have user input) and opening the modal if all fields have user input and a radio option is selected.
– user10073459
Dec 31 '18 at 1:06
|
show 1 more comment
Thank you for your assistance, You solution while working with the two radios I can't seem to get working with the other variables I have, would you be able to advise how I could implement the solution to work with my edits above? Thank you
– user10073459
Dec 31 '18 at 0:27
Please post your HTML and JS, so i can investigate
– Tarreq
Dec 31 '18 at 0:29
I have updated my post with a cutdown copy of the HTML to where should be relevant, Thank you
– user10073459
Dec 31 '18 at 0:41
ok, your edit didn't say what you want your code to do, please explain in detail
– Tarreq
Dec 31 '18 at 0:51
My apologies, I have added the current and expected behaviour to my edit, The only issue I am having with the code is getting an alert if no radio is checked (and all fields have user input) and opening the modal if all fields have user input and a radio option is selected.
– user10073459
Dec 31 '18 at 1:06
Thank you for your assistance, You solution while working with the two radios I can't seem to get working with the other variables I have, would you be able to advise how I could implement the solution to work with my edits above? Thank you
– user10073459
Dec 31 '18 at 0:27
Thank you for your assistance, You solution while working with the two radios I can't seem to get working with the other variables I have, would you be able to advise how I could implement the solution to work with my edits above? Thank you
– user10073459
Dec 31 '18 at 0:27
Please post your HTML and JS, so i can investigate
– Tarreq
Dec 31 '18 at 0:29
Please post your HTML and JS, so i can investigate
– Tarreq
Dec 31 '18 at 0:29
I have updated my post with a cutdown copy of the HTML to where should be relevant, Thank you
– user10073459
Dec 31 '18 at 0:41
I have updated my post with a cutdown copy of the HTML to where should be relevant, Thank you
– user10073459
Dec 31 '18 at 0:41
ok, your edit didn't say what you want your code to do, please explain in detail
– Tarreq
Dec 31 '18 at 0:51
ok, your edit didn't say what you want your code to do, please explain in detail
– Tarreq
Dec 31 '18 at 0:51
My apologies, I have added the current and expected behaviour to my edit, The only issue I am having with the code is getting an alert if no radio is checked (and all fields have user input) and opening the modal if all fields have user input and a radio option is selected.
– user10073459
Dec 31 '18 at 1:06
My apologies, I have added the current and expected behaviour to my edit, The only issue I am having with the code is getting an alert if no radio is checked (and all fields have user input) and opening the modal if all fields have user input and a radio option is selected.
– user10073459
Dec 31 '18 at 1:06
|
show 1 more comment
Why not make the radio buttons required
It would seem that you're trying to do the job of the browser. You don't need to display an alert, which are generally bad UX, because you can use built-in form validation via the required
attribute like so:
<form onsubmit="...">
<input type="radio" name="group" value="1" required />
<input type="radio" name="group" value="2" />
...
<button type="submit">Submit</button>
<form />
NOTE: you only need one required
attribute and it will apply to all inputs in said group.
This is something I have already done but the form submit is calling a function to open a Modal on the same page. using a<submit>
just opens the Modal over the top of the page even when theRequired
fields are empty, I apologise I forgot to add this too my original question.
– user10073459
Dec 31 '18 at 0:44
Theonsubmit
event should never even fire if you've set it up correctly @user10073459
– chbchb55
Dec 31 '18 at 1:09
add a comment |
Why not make the radio buttons required
It would seem that you're trying to do the job of the browser. You don't need to display an alert, which are generally bad UX, because you can use built-in form validation via the required
attribute like so:
<form onsubmit="...">
<input type="radio" name="group" value="1" required />
<input type="radio" name="group" value="2" />
...
<button type="submit">Submit</button>
<form />
NOTE: you only need one required
attribute and it will apply to all inputs in said group.
This is something I have already done but the form submit is calling a function to open a Modal on the same page. using a<submit>
just opens the Modal over the top of the page even when theRequired
fields are empty, I apologise I forgot to add this too my original question.
– user10073459
Dec 31 '18 at 0:44
Theonsubmit
event should never even fire if you've set it up correctly @user10073459
– chbchb55
Dec 31 '18 at 1:09
add a comment |
Why not make the radio buttons required
It would seem that you're trying to do the job of the browser. You don't need to display an alert, which are generally bad UX, because you can use built-in form validation via the required
attribute like so:
<form onsubmit="...">
<input type="radio" name="group" value="1" required />
<input type="radio" name="group" value="2" />
...
<button type="submit">Submit</button>
<form />
NOTE: you only need one required
attribute and it will apply to all inputs in said group.
Why not make the radio buttons required
It would seem that you're trying to do the job of the browser. You don't need to display an alert, which are generally bad UX, because you can use built-in form validation via the required
attribute like so:
<form onsubmit="...">
<input type="radio" name="group" value="1" required />
<input type="radio" name="group" value="2" />
...
<button type="submit">Submit</button>
<form />
NOTE: you only need one required
attribute and it will apply to all inputs in said group.
answered Dec 31 '18 at 0:35
chbchb55chbchb55
1,5071723
1,5071723
This is something I have already done but the form submit is calling a function to open a Modal on the same page. using a<submit>
just opens the Modal over the top of the page even when theRequired
fields are empty, I apologise I forgot to add this too my original question.
– user10073459
Dec 31 '18 at 0:44
Theonsubmit
event should never even fire if you've set it up correctly @user10073459
– chbchb55
Dec 31 '18 at 1:09
add a comment |
This is something I have already done but the form submit is calling a function to open a Modal on the same page. using a<submit>
just opens the Modal over the top of the page even when theRequired
fields are empty, I apologise I forgot to add this too my original question.
– user10073459
Dec 31 '18 at 0:44
Theonsubmit
event should never even fire if you've set it up correctly @user10073459
– chbchb55
Dec 31 '18 at 1:09
This is something I have already done but the form submit is calling a function to open a Modal on the same page. using a
<submit>
just opens the Modal over the top of the page even when the Required
fields are empty, I apologise I forgot to add this too my original question.– user10073459
Dec 31 '18 at 0:44
This is something I have already done but the form submit is calling a function to open a Modal on the same page. using a
<submit>
just opens the Modal over the top of the page even when the Required
fields are empty, I apologise I forgot to add this too my original question.– user10073459
Dec 31 '18 at 0:44
The
onsubmit
event should never even fire if you've set it up correctly @user10073459– chbchb55
Dec 31 '18 at 1:09
The
onsubmit
event should never even fire if you've set it up correctly @user10073459– chbchb55
Dec 31 '18 at 1:09
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982298%2falert-if-radios-unchecked-on-form-submit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9GxOJY3pAU KTjEESzmDBhRL ABb6C8QODHexODcliVbYcR,Vp5s6zOY y2znL
Possible duplicate of How can I check whether a radio button is selected with JavaScript?
– chbchb55
Dec 30 '18 at 23:37
@chbchb55 I have made some edits to give some further context, from what I can see there isn't an example I can apply/get working from the solution linked.
– user10073459
Dec 31 '18 at 0:23