ForwardIterator combined with multiple bind's is iterating too fast
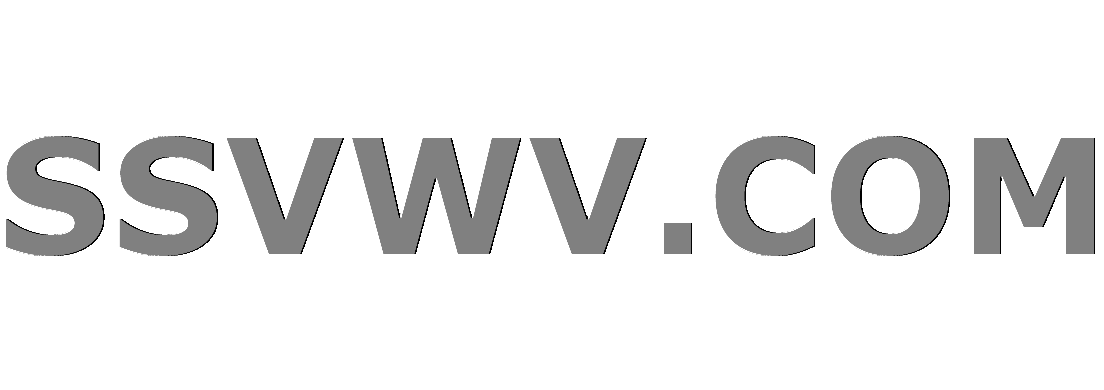
Multi tool use
i want to iterate over a vector
of int
s and remove all even numbers.
Example:
std::vector<int> v = {5,2,9,3,8}
auto it = std::remove_if(v.begin(),v.end(),
std::bind(std::bind(std::equal_to<int>(),_1,0),
std::bind(std::modulus<int>(),_1,2)));
The expected result should be {5,9,3}
But it is {5,8,9,3,8}
I think the Iterator is already at the end before performing all functions in bind and remove.
I know how to solve it differently but i want to know how to use the nested form and how it works with iterators
c++ stl
|
show 5 more comments
i want to iterate over a vector
of int
s and remove all even numbers.
Example:
std::vector<int> v = {5,2,9,3,8}
auto it = std::remove_if(v.begin(),v.end(),
std::bind(std::bind(std::equal_to<int>(),_1,0),
std::bind(std::modulus<int>(),_1,2)));
The expected result should be {5,9,3}
But it is {5,8,9,3,8}
I think the Iterator is already at the end before performing all functions in bind and remove.
I know how to solve it differently but i want to know how to use the nested form and how it works with iterators
c++ stl
2
Not an answer, but this would be much easier to read as a lambda instead ofstd::bind
expressions. Lambdas are available since C++11.
– user10605163
Dec 30 '18 at 23:40
Use lambdas.bind
insidebind
behaves counterintuitively (look for mention ofstd::is_bind_expression
)
– Igor Tandetnik
Dec 30 '18 at 23:43
@IgorTandetnik do u have a hint how to structure it as a lambda? Have not used them often yet
– Henne
Dec 30 '18 at 23:46
2
auto it = std::remove_if(..., (int val) { return val % 2 == 0; });
– Igor Tandetnik
Dec 30 '18 at 23:47
2
@IgorTandetnik Please, stop answering in comments. We are unable to peer review your contributions. Hopefully that is not your deliberate intention
– Lightness Races in Orbit
Dec 31 '18 at 1:05
|
show 5 more comments
i want to iterate over a vector
of int
s and remove all even numbers.
Example:
std::vector<int> v = {5,2,9,3,8}
auto it = std::remove_if(v.begin(),v.end(),
std::bind(std::bind(std::equal_to<int>(),_1,0),
std::bind(std::modulus<int>(),_1,2)));
The expected result should be {5,9,3}
But it is {5,8,9,3,8}
I think the Iterator is already at the end before performing all functions in bind and remove.
I know how to solve it differently but i want to know how to use the nested form and how it works with iterators
c++ stl
i want to iterate over a vector
of int
s and remove all even numbers.
Example:
std::vector<int> v = {5,2,9,3,8}
auto it = std::remove_if(v.begin(),v.end(),
std::bind(std::bind(std::equal_to<int>(),_1,0),
std::bind(std::modulus<int>(),_1,2)));
The expected result should be {5,9,3}
But it is {5,8,9,3,8}
I think the Iterator is already at the end before performing all functions in bind and remove.
I know how to solve it differently but i want to know how to use the nested form and how it works with iterators
c++ stl
c++ stl
edited Dec 30 '18 at 23:41
Sid S
3,7072923
3,7072923
asked Dec 30 '18 at 23:36
HenneHenne
142
142
2
Not an answer, but this would be much easier to read as a lambda instead ofstd::bind
expressions. Lambdas are available since C++11.
– user10605163
Dec 30 '18 at 23:40
Use lambdas.bind
insidebind
behaves counterintuitively (look for mention ofstd::is_bind_expression
)
– Igor Tandetnik
Dec 30 '18 at 23:43
@IgorTandetnik do u have a hint how to structure it as a lambda? Have not used them often yet
– Henne
Dec 30 '18 at 23:46
2
auto it = std::remove_if(..., (int val) { return val % 2 == 0; });
– Igor Tandetnik
Dec 30 '18 at 23:47
2
@IgorTandetnik Please, stop answering in comments. We are unable to peer review your contributions. Hopefully that is not your deliberate intention
– Lightness Races in Orbit
Dec 31 '18 at 1:05
|
show 5 more comments
2
Not an answer, but this would be much easier to read as a lambda instead ofstd::bind
expressions. Lambdas are available since C++11.
– user10605163
Dec 30 '18 at 23:40
Use lambdas.bind
insidebind
behaves counterintuitively (look for mention ofstd::is_bind_expression
)
– Igor Tandetnik
Dec 30 '18 at 23:43
@IgorTandetnik do u have a hint how to structure it as a lambda? Have not used them often yet
– Henne
Dec 30 '18 at 23:46
2
auto it = std::remove_if(..., (int val) { return val % 2 == 0; });
– Igor Tandetnik
Dec 30 '18 at 23:47
2
@IgorTandetnik Please, stop answering in comments. We are unable to peer review your contributions. Hopefully that is not your deliberate intention
– Lightness Races in Orbit
Dec 31 '18 at 1:05
2
2
Not an answer, but this would be much easier to read as a lambda instead of
std::bind
expressions. Lambdas are available since C++11.– user10605163
Dec 30 '18 at 23:40
Not an answer, but this would be much easier to read as a lambda instead of
std::bind
expressions. Lambdas are available since C++11.– user10605163
Dec 30 '18 at 23:40
Use lambdas.
bind
inside bind
behaves counterintuitively (look for mention of std::is_bind_expression
)– Igor Tandetnik
Dec 30 '18 at 23:43
Use lambdas.
bind
inside bind
behaves counterintuitively (look for mention of std::is_bind_expression
)– Igor Tandetnik
Dec 30 '18 at 23:43
@IgorTandetnik do u have a hint how to structure it as a lambda? Have not used them often yet
– Henne
Dec 30 '18 at 23:46
@IgorTandetnik do u have a hint how to structure it as a lambda? Have not used them often yet
– Henne
Dec 30 '18 at 23:46
2
2
auto it = std::remove_if(..., (int val) { return val % 2 == 0; });
– Igor Tandetnik
Dec 30 '18 at 23:47
auto it = std::remove_if(..., (int val) { return val % 2 == 0; });
– Igor Tandetnik
Dec 30 '18 at 23:47
2
2
@IgorTandetnik Please, stop answering in comments. We are unable to peer review your contributions. Hopefully that is not your deliberate intention
– Lightness Races in Orbit
Dec 31 '18 at 1:05
@IgorTandetnik Please, stop answering in comments. We are unable to peer review your contributions. Hopefully that is not your deliberate intention
– Lightness Races in Orbit
Dec 31 '18 at 1:05
|
show 5 more comments
1 Answer
1
active
oldest
votes
In VS2015, your code leaves v
containing {5, 9, 3, 3, 8}.
std::remove_if()
returns an iterator to the first unused element in the vector
, use that to truncate the vector
:
v.erase(it, v.end());
After that, v
contains {5, 9, 3}
On a side note, if you want to use a lambda instead of bind
you can do it like this:
std::vector<int> v = { 5, 2, 9, 3, 8 };
auto it = std::remove_if(v.begin(), v.end(), (int val) { return val % 2 == 0; });
v.erase(it, v.end());
Btw, en.cppreference.com/w/cpp/algorithm/remove has some excellent comments about all of this.
– Rob Starling
Dec 31 '18 at 6:57
As an additional side-note, the comment "The similarly-named container member functions list::remove, list::remove_if, forward_list::remove, and forward_list::remove_if erase the removed elements." is interesting.
– Rob Starling
Dec 31 '18 at 6:57
Also, you don't have to truncate the vector if you remember to stop atit
instead ofv.end()
. Something to remember if you're just using the results once and gonna let the whole vector get destroyed.
– Rob Starling
Dec 31 '18 at 7:00
You might want to edit the "In VS2015, your code leaves v containing {5, 9, 3, 3, 8}." part to note that the elements afterit
are in an undefined state as some of them weremove
d into the[v.begin(), it)
range.
– Rob Starling
Dec 31 '18 at 20:58
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982300%2fforwarditerator-combined-with-multiple-binds-is-iterating-too-fast%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In VS2015, your code leaves v
containing {5, 9, 3, 3, 8}.
std::remove_if()
returns an iterator to the first unused element in the vector
, use that to truncate the vector
:
v.erase(it, v.end());
After that, v
contains {5, 9, 3}
On a side note, if you want to use a lambda instead of bind
you can do it like this:
std::vector<int> v = { 5, 2, 9, 3, 8 };
auto it = std::remove_if(v.begin(), v.end(), (int val) { return val % 2 == 0; });
v.erase(it, v.end());
Btw, en.cppreference.com/w/cpp/algorithm/remove has some excellent comments about all of this.
– Rob Starling
Dec 31 '18 at 6:57
As an additional side-note, the comment "The similarly-named container member functions list::remove, list::remove_if, forward_list::remove, and forward_list::remove_if erase the removed elements." is interesting.
– Rob Starling
Dec 31 '18 at 6:57
Also, you don't have to truncate the vector if you remember to stop atit
instead ofv.end()
. Something to remember if you're just using the results once and gonna let the whole vector get destroyed.
– Rob Starling
Dec 31 '18 at 7:00
You might want to edit the "In VS2015, your code leaves v containing {5, 9, 3, 3, 8}." part to note that the elements afterit
are in an undefined state as some of them weremove
d into the[v.begin(), it)
range.
– Rob Starling
Dec 31 '18 at 20:58
add a comment |
In VS2015, your code leaves v
containing {5, 9, 3, 3, 8}.
std::remove_if()
returns an iterator to the first unused element in the vector
, use that to truncate the vector
:
v.erase(it, v.end());
After that, v
contains {5, 9, 3}
On a side note, if you want to use a lambda instead of bind
you can do it like this:
std::vector<int> v = { 5, 2, 9, 3, 8 };
auto it = std::remove_if(v.begin(), v.end(), (int val) { return val % 2 == 0; });
v.erase(it, v.end());
Btw, en.cppreference.com/w/cpp/algorithm/remove has some excellent comments about all of this.
– Rob Starling
Dec 31 '18 at 6:57
As an additional side-note, the comment "The similarly-named container member functions list::remove, list::remove_if, forward_list::remove, and forward_list::remove_if erase the removed elements." is interesting.
– Rob Starling
Dec 31 '18 at 6:57
Also, you don't have to truncate the vector if you remember to stop atit
instead ofv.end()
. Something to remember if you're just using the results once and gonna let the whole vector get destroyed.
– Rob Starling
Dec 31 '18 at 7:00
You might want to edit the "In VS2015, your code leaves v containing {5, 9, 3, 3, 8}." part to note that the elements afterit
are in an undefined state as some of them weremove
d into the[v.begin(), it)
range.
– Rob Starling
Dec 31 '18 at 20:58
add a comment |
In VS2015, your code leaves v
containing {5, 9, 3, 3, 8}.
std::remove_if()
returns an iterator to the first unused element in the vector
, use that to truncate the vector
:
v.erase(it, v.end());
After that, v
contains {5, 9, 3}
On a side note, if you want to use a lambda instead of bind
you can do it like this:
std::vector<int> v = { 5, 2, 9, 3, 8 };
auto it = std::remove_if(v.begin(), v.end(), (int val) { return val % 2 == 0; });
v.erase(it, v.end());
In VS2015, your code leaves v
containing {5, 9, 3, 3, 8}.
std::remove_if()
returns an iterator to the first unused element in the vector
, use that to truncate the vector
:
v.erase(it, v.end());
After that, v
contains {5, 9, 3}
On a side note, if you want to use a lambda instead of bind
you can do it like this:
std::vector<int> v = { 5, 2, 9, 3, 8 };
auto it = std::remove_if(v.begin(), v.end(), (int val) { return val % 2 == 0; });
v.erase(it, v.end());
edited Dec 31 '18 at 6:40
answered Dec 31 '18 at 1:39
Sid SSid S
3,7072923
3,7072923
Btw, en.cppreference.com/w/cpp/algorithm/remove has some excellent comments about all of this.
– Rob Starling
Dec 31 '18 at 6:57
As an additional side-note, the comment "The similarly-named container member functions list::remove, list::remove_if, forward_list::remove, and forward_list::remove_if erase the removed elements." is interesting.
– Rob Starling
Dec 31 '18 at 6:57
Also, you don't have to truncate the vector if you remember to stop atit
instead ofv.end()
. Something to remember if you're just using the results once and gonna let the whole vector get destroyed.
– Rob Starling
Dec 31 '18 at 7:00
You might want to edit the "In VS2015, your code leaves v containing {5, 9, 3, 3, 8}." part to note that the elements afterit
are in an undefined state as some of them weremove
d into the[v.begin(), it)
range.
– Rob Starling
Dec 31 '18 at 20:58
add a comment |
Btw, en.cppreference.com/w/cpp/algorithm/remove has some excellent comments about all of this.
– Rob Starling
Dec 31 '18 at 6:57
As an additional side-note, the comment "The similarly-named container member functions list::remove, list::remove_if, forward_list::remove, and forward_list::remove_if erase the removed elements." is interesting.
– Rob Starling
Dec 31 '18 at 6:57
Also, you don't have to truncate the vector if you remember to stop atit
instead ofv.end()
. Something to remember if you're just using the results once and gonna let the whole vector get destroyed.
– Rob Starling
Dec 31 '18 at 7:00
You might want to edit the "In VS2015, your code leaves v containing {5, 9, 3, 3, 8}." part to note that the elements afterit
are in an undefined state as some of them weremove
d into the[v.begin(), it)
range.
– Rob Starling
Dec 31 '18 at 20:58
Btw, en.cppreference.com/w/cpp/algorithm/remove has some excellent comments about all of this.
– Rob Starling
Dec 31 '18 at 6:57
Btw, en.cppreference.com/w/cpp/algorithm/remove has some excellent comments about all of this.
– Rob Starling
Dec 31 '18 at 6:57
As an additional side-note, the comment "The similarly-named container member functions list::remove, list::remove_if, forward_list::remove, and forward_list::remove_if erase the removed elements." is interesting.
– Rob Starling
Dec 31 '18 at 6:57
As an additional side-note, the comment "The similarly-named container member functions list::remove, list::remove_if, forward_list::remove, and forward_list::remove_if erase the removed elements." is interesting.
– Rob Starling
Dec 31 '18 at 6:57
Also, you don't have to truncate the vector if you remember to stop at
it
instead of v.end()
. Something to remember if you're just using the results once and gonna let the whole vector get destroyed.– Rob Starling
Dec 31 '18 at 7:00
Also, you don't have to truncate the vector if you remember to stop at
it
instead of v.end()
. Something to remember if you're just using the results once and gonna let the whole vector get destroyed.– Rob Starling
Dec 31 '18 at 7:00
You might want to edit the "In VS2015, your code leaves v containing {5, 9, 3, 3, 8}." part to note that the elements after
it
are in an undefined state as some of them were move
d into the [v.begin(), it)
range.– Rob Starling
Dec 31 '18 at 20:58
You might want to edit the "In VS2015, your code leaves v containing {5, 9, 3, 3, 8}." part to note that the elements after
it
are in an undefined state as some of them were move
d into the [v.begin(), it)
range.– Rob Starling
Dec 31 '18 at 20:58
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53982300%2fforwarditerator-combined-with-multiple-binds-is-iterating-too-fast%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rYnLso7wpzKwZdrrYwAxLj8GltLJKAsdy,7IAx0,rumdbEE,u,o
2
Not an answer, but this would be much easier to read as a lambda instead of
std::bind
expressions. Lambdas are available since C++11.– user10605163
Dec 30 '18 at 23:40
Use lambdas.
bind
insidebind
behaves counterintuitively (look for mention ofstd::is_bind_expression
)– Igor Tandetnik
Dec 30 '18 at 23:43
@IgorTandetnik do u have a hint how to structure it as a lambda? Have not used them often yet
– Henne
Dec 30 '18 at 23:46
2
auto it = std::remove_if(..., (int val) { return val % 2 == 0; });
– Igor Tandetnik
Dec 30 '18 at 23:47
2
@IgorTandetnik Please, stop answering in comments. We are unable to peer review your contributions. Hopefully that is not your deliberate intention
– Lightness Races in Orbit
Dec 31 '18 at 1:05